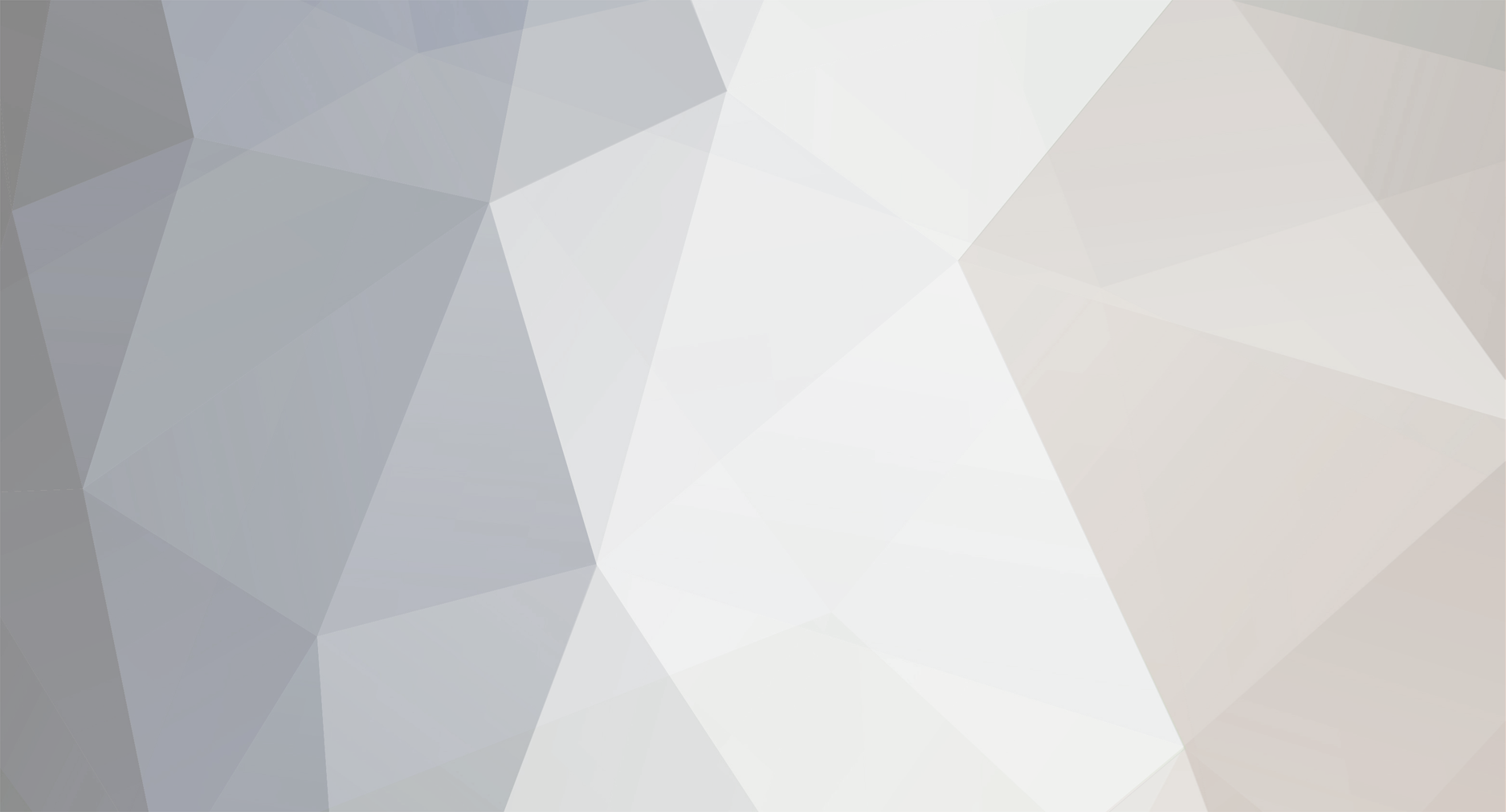
joe92
-
Posts
304 -
Joined
-
Last visited
Posts posted by joe92
-
-
OK, after a morning of reading and learning new JavaScript functions and tricks I have come up with the following to try and fix it.
function cancelBubble(e) { var evt = e || window.event; /*ff/chrome etc. route*/ if (evt.stopPropagation) { evt.stopPropagation(); } /*ie route*/ else{ evt.cancelBubble = true; } } function setActiveThis(tg){ /*add custom event listener to the element to prevent event propagation*/ if(tg && tg.addEventListener) { tg.addEventListener('click', cancelBubble, false); } else if(tg && tg.attachEvent) { tg.attachEvent('onclick', cancelBubble); } /*start event*/ var container = document.getElementById('activeContainer'); var containerEls = container.getElementsByTagName('*'); var theClass = ''; var newClass = ''; for(var i=0;i<containerEls.length;i++) { /*get the class of the current element*/ theClass = containerEls[i].className; /*check the class is not blank*/ if(theClass != '') { /*check if this element is the active one*/ if(theClass.match(/active/)) { /*remove the active class from it*/ newClass = theClass.replace(/\s?active/, ''); containerEls[i].className = newClass; /*since there is only ever one active class break out of the loop*/ i = containerEls.length; } } } var tgClass = tg.className; tgClass += ' active'; tg.className = tgClass; }
This works perfectly, absolutely what I needed. However, it only works after the first click. I.e. the first click still applies the active class to the highest element with the event attached to it, but all subsequent clicks go in their intended positions. Any idea why that's happening and how I can fix it? I'm guessing that it may be to do with the timing of when I add the event listeners?
Thanks for all you help. I've nearly got this one sorted,
Joe
-
You have two problems:
1. The child elements are INSIDE the parent elements. So, when you click on the child you are also clicking on the parent. If you put an alert in the function you will see it popup multiple times based upon which child element you select.
2. The child elements will inherit the styles of the parent elements.
The easy fix is to not have nested divs.
Problem 2 is actually a desired result - when they click the parent it highlights them all by carrying the style through and when they click the child it only highlights the child. It's problem 1 that I'm trying to get around. I just don't know how yet.
You can just stop the event propagation.
Thank you for pointing me in the right direction. Google's given me this tutorial, which describes my exact problem in the first sentence, from a quick search so I'll see what I can do. I wasn't even aware of event propagation so I might be back soon with another question
Looks interesting though, thanks again!
-
Where's your pound symbol?!
and single- and double-quotes aren't on the same key!
That's what makes it so much better!
Granted, as King Phillip said, our pipe and backslash key is in the most annoying place ever. But I much prefer having the @ symbol where it is. I guess it's all about what your used to though. I also enjoy having the hash and tilde as their own key above the right hand shift key, quite useful.
-
Background colour is a problem. It is denoted in javascript as
backgroundColor
. In fact, I think all javascript notations of a css style use camels as opposed to the dash.Also, you will need to pick a position of the getElementsByTagName array for the javascript to get the parent of it. parentNode works by getting the parent of a specific element. Not an array of elements.
Try:
function changeIt() { var node; node = document.getElementsByTagName("p")[0].parentNode; node.style.backgroundColor = '#0033dd'; }
Hope that helps you,
Joe
-
Ah, thought it did have a location. I'm from the UK. I have this layout:
I actually also only just discovered that euro sign today too whiles experimenting with every single key combination wondering what Alt Gr actually did
-
Ahh right. Always wanted to know. Thanks for the information
(experimenting with smileys, I don't think this one fits the needs but oh well).
-
Right, I've glanced at them every day for the past year and have no idea what they are?
The symbols in question are:
¬ - Shift + that button above tab
¦ - Alt Grrrrrrrrrrrrrrrrrrrr + that button above tab
Please help me put this morbid curiosity to sleep
Cheers!
Joe
-
Hi,
I am writing a function which will apply an active class to a div that has been clicked. I am running into a problem in that the function that is called on all the elements involved doesn't know how to differentiate between the elements, and will therefore jump to the highest element with the call. If that's clear enough?
The function is written to first remove the active class from whichever element it used to be on, and then apply it to our current element.
Here is the javascript function:
function setActiveThis(el){ var container = document.getElementById('activeContainer'); var containerEls = container.getElementsByTagName('*'); var theClass = ''; var newClass = ''; for(var i=0;i<containerEls.length;i++) { /*get the class of the current element*/ theClass = containerEls[i].className; /*check the class is not blank*/ if(theClass != '') { /*check if this element is the active one*/ if(theClass.match(/active/)) { /*remove the active class from it*/ newClass = theClass.replace(/\s?active/, ''); containerEls[i].className = newClass; /*since there is only ever one active class break out of the loop*/ i = containerEls.length; } } } var elClass = el.className; elClass += ' active'; el.className = elClass; }
And here is the HTML that I am testing it with:
<div id="activeContainer"> <div onclick="setActiveThis(this);"> Content1 <div onclick="setActiveThis(this);"> Content2 <div onclick="setActiveThis(this);"> Content3 <div onclick="setActiveThis(this);"> Testing </div> </div> </div> </div> <div onclick="setActiveThis(this);"> I work because there are no higher elements with the setActiveThis function around me </div> </div>
What I need is for when I click on a div, for example the word testing, the active class be applied to ONLY the div which directly contains it. Currently, it is applying it to the highest element that wraps around it with the setActiveThis function, in this example applying it to the div with content1. How can I get it to apply the active class to the 'testing' div only?
If this isn't clear enough, please say so and I will try to reword my problem. Any and all help is appreciated!
Cheers,
Joe
-
Hey playful,
From what I've seen of tdl's, the optional extension on the end is usually the shorter of the two tdl components. That's why I made it the shorter one. It's neither here nor there though. Once you start trying to match url's in a block of text you can't even end at the tdl, for the url might be pointing to a file 3 sub directories down the tree. This is all specific to the OP's needs.
And true, I stand by the second version of my original regex
haha
Joe
-
Hi playful,
That should say:[a-z]{2,10}(\.[a-z]{2,5})?
But that matches aa
(No tld needed.)
Yes, that alone does, but not when included in the entire regex.
So my question still stands: did you mean to say
if(preg_match("/^[a-z0-9-]+\.[a-z]{2,5}$/", $domain))
No, I meant what I posted. I'll explain. The OP states:
A user should submit domain such as abc123-abc.tld
That means the the domain can consist of three parts:
abc123-abd
.
tld
Each part is:
[td].
The reason the tdl contains so much is because of how many types of tdl's there are. If we limit the tdl to just [a-z]{2,5} as you are suggesting then we are ruling out co.uk addresses etc., they will fail on the extra dot. However, saying that, the .uk is an optional part of the tdl as it .com's and .net's etc don't include them. That is why I encase it in parenthesis followed by a question mark. If it's there, include it, else if the regex fails here it doesn't matter as it's not imperative to the entire match.
Hence I stand by my original regex. It will allow for every type of tdl available and must match characters and a dot before it:
if(preg_match("/^[a-z0-9-]+\.[a-z]{2,10}(\.[a-z]{2,5})?$/", $domain))
Hope I explained myself properly,
Joe
-
Oops! It's always a damned typo! Haha.
That isn't supposed to be used as a capturing parenthesis. It was a questionable string and it was meant to start with a dot. I personally don't see the point in telling the regex engine not to remember a parenthesis when we're talking about a maximum of 5-10 bytes. That should say:
[a-z]{2,10}(\.[a-z]{2,5})?
That is to cover tdl's such as a .co.uk address or a .gov.uk address. Also, I was recently reading that personal tdl strings are very soon going to be launched onto the world wide web. In fact, I think the 'reveal' date of all the new one's is going to be 1st May. Tdl's such as .museum and .aero have already been introduced, although are reserved in this case for museum's and aerospace firms. Let's not forget the .name which allows individuals (I'm guessing just the fabulously wealthy/famous ones) to have their name in a tdl. Once they start becoming more common you could have tdl's such as .hammersmith or .frankenstein. Got to accommodate for the future now.
Anyway, good spot playful! Here is the complete code I suggest you use ankur. It will allow some wrong ones through (e.g. .zzzzzz.zz), but unless you put a very big OR statement at the end to capture every type of legal tdl ((com?|co\.uk|info|..etc.)) you won't be able to get around it I'm afraid.
if(preg_match("/^[a-z0-9-]+\.[a-z]{2,10}(\.[a-z]{2,5})?$/", $domain))
Here's the Root Zone Database if you do wish to do that though
Joe
-
Hmm, well from the code you have provided that error should not be coming up. Are you using any third party libraries like jQuery or Mootools? They often write their own custom event listeners and that could be causing the error.
Or are you using any third party text editor tools such as Nicedit or CKeditor? They too might be writing custom event listeners which might be causing the error. Just clutching at straws here. Are you using any third party packages at all?
Also, what tool/browser are you seeing the error in? Firefox's firebug is quite useful in that it tells you the line, column and script of the JavaScript error.
-
Will domains be suffixed with the http:// and www.? Will they be submitted with trailing GET information such as '.tdl/?index.php'?
Both those questions will change the regex. However, from what you have said so far, the following should suffice:
if(preg_match("/^[a-z0-9-]+\.[a-z]{2,10}([a-z]{2,5})?$/", $domain))
Hope that helps you,
Joe
Edit:: Noticed you said small alpha's so removed case insensitivity
-
<FORM action="result.html" method="post" name="form" validateForm()">
That is causing the error I would presume. You cannot just type a JavaScript function into the tag. You have to place it in an event listener. By not doing so it seems to have caused some sort of parsing error, I think. Judging from the function, I would say you need to use onsumbit:
<FORM action="result.html" method="post" name="form" onsubmit="validateForm();">
-
Haven't read through the entire thread but I could offer an alternative way of thinking for this...
-Run through the post and replace all quotes (from opening tag to it's closing tag) with a unique identifier and storing the quote in an array. E.g. '[|||QUOTE]##' for unique identifier, where ## represents which quote this is in terms of array position, so the first is 0.
-Run through the post and replace all the youtube tags that are present with function A which turns them into embedded videos.
-Run a for loop to put all the quotes back in in there original positions from the array we stored them in.
-Run through the post and replace all the remaining youtube tags with function B which turns them into links. (The only remaining one's will have only just appeared if they were in quotes)
-Now do all other replacements you might have.
Hope that helps you,
Joe
-
Also, if they are already on a higher level, calculate the starting cost with:
$level = 4; $cost = 5 + ($level * 5);
That means the first cost here for a level 4 is 25. A level 10 would be 55 etc..
-
I don't think there is a function for that, but a simple while loop will solve that for you.
<?php $level = 1; $cost = 10; $money = 100; while($money > $cost) { $money -= $cost; //remove cost from money left $cost += 5; //increase base cost ++$level; //increment your level } echo $level;
That will output 5 levels for 100 bucks.
Edit:: Removed a pointless step.
-
Could be no spaces, could be over a dozen spaces, haha. Oh well, thanks for taking the time to respond.
Joe
-
I may be wrong but I remember hearing or reading somewhere that a foreach loop makes an identical copy of the array, and then works on that copy leaving the original intact. If that is right, then what you are seeing is the delay to create the copy.
Hope that helps,
Joe
-
I wish to extract the data between the square brackets and replace the spaces with underscores in one go?
E.g. I have the string
location=[location to go to]
I need to get it to,
location_to_go_to
Currently I am using a preg_replace_callback with a str_replace to change the spaces into underscores, but it seems like a long winded route to take. Is there a way I can do this in one clean sweep of a preg_replace?
Here is the existing code:
<?php function removeSpace($match){ $string = $match[1]; $string = str_replace(' ', '_', $string); return $string; } $location = 'location=[location to go to]'; echo $location.'<br/>into<br/>'; $location = preg_replace_callback("~location=\[([\w\s-]+)\]~ism", "removeSpace", $location); echo $location;
If not, then I suppose it's no great loss. I'm just constantly looking for ways to improve my regex skills
Cheers,
Joe
-
$correct_numbers += $count2;
Is equivalent to
$correct_numbers = $correct_numbers + $count2;
-
Hi there, I am working on a little CMS site and I have run into a bit of a problem. When the user comes to publish the changes they have made I need to push the new files onto their server. I don't know whether they are on a shared host or not though, and if they are they won't be the root user and so functions like chmod and rmdir will be disallowed. Even if they weren't on a shared host I doubt whether they would be running scripts as root anyway.
I cannot ask the user to set the file permissions to 0777 to use the CMS as I know that most people would not do that, I know I wouldn't. So my plan of action was to have the permissions set to 0644 and temporarily change them to 0777 whiles I push the changes then change it back again afterwards. I have a tiny website on a shared host so I am able to test this in the worst of scenarios and these are the errors I get:
Warning: chmod() [function.chmod]: Operation not permitted in ******** on line 20Warning: fopen(********) [function.fopen]: failed to open stream: Permission denied in ******** on line 35
Warning: fwrite() expects parameter 1 to be resource, boolean given in ******** on line 39
Warning: fclose() expects parameter 1 to be resource, boolean given in ******** on line 40
Because I am not the root user chmod is not permitted and because the file permissions are set to 0644 I cannot execute the file system functions which alter the files. It all relies on the permissions being set right. I tried using umask but as someone says in a note on php.net, umask can only remove/delete permissions, it can't grant them. So that seems like a dead end. I can't change ownership either with chown as that requires you to be the root to use too.
Wondering what was going on I used fileperms to find out what the permissions were according to the php and got the number 33188. Turns out after a bit of research that this is an octal number, so I converted it and got the number 100644. That looks about right, but I don't know what the 10 is doing before the permissions?
Does anyone know how can I get around this problem? I would like to avoid using FTP functions if I can.
Thanks for any help,
Joe
-
$string = preg_replace("/©([a-z0-9]+)/i","©\\1",$string);
That should do it. That is looking for a copyright sign followed by a number or any letter between 1 and infinity times in lower or upper case and will replace it with ©\\1. The \\1 is the first capturing parenthesis (the round brackets) within the pattern so in your first example it will change too '©1'. If it detects a space the pattern will fail and so won't replace it.
Hope that helps you,
Joe
-
Mozilla says this about oninput
This event is sent when a user enters text in a textbox . This event is only called when the text displayed would change, thus it is not called when the user presses non-displayable keys.Whereas the onkeyup is described as
The keyup event is raised when the user releases a key that's been pressed.So there is your difference.
Know whether I am on the child element, not the parent, with the same function
in Javascript Help
Posted
And it was entirely to do with the timing. To get round it, I've attached a class of stopPropagation to all the elements that have the setActiveThis function attached to them. Then on window.load I run a function that loop's through all elements with this class and attaches the needed event listener to stop event propagation.
Problem solved and I've learnt a lot! Thank you,
Joe