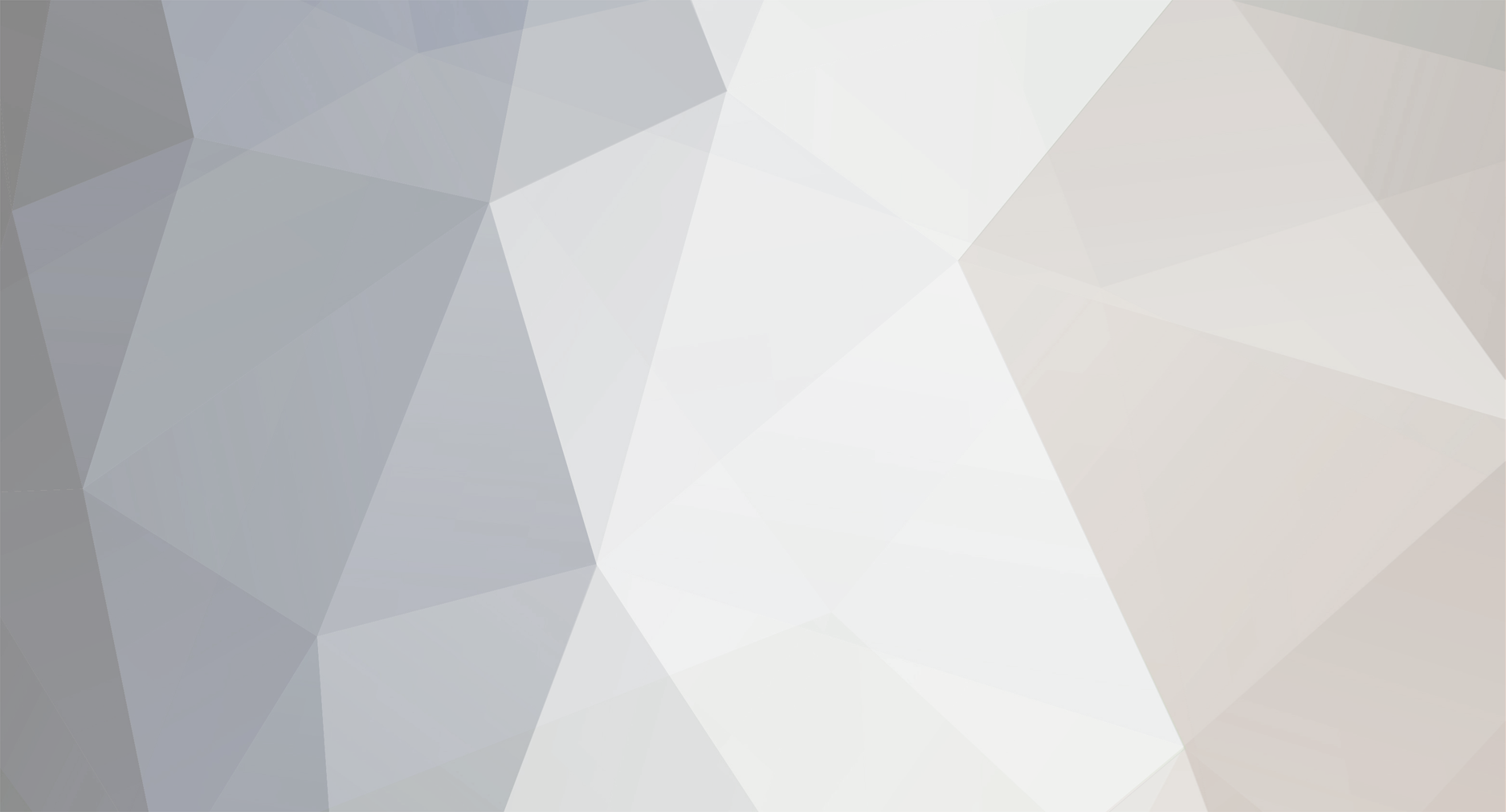
crmamx
-
Posts
417 -
Joined
-
Last visited
Posts posted by crmamx
-
-
Example 2, here is one way.
http://www.beginnersphp.co.uk/mathoptut.php
Google search is very helpful for questions like yours. I try to only bother the phpFreaks as a last resort.
-
Well, I can now add/change/delete data in my db. But then I find I have no security at all. I know that IE/View/Source will display the code, but I did not know that right click on a frame would display that particular frameset code.
Can you point me to a simple php security script. I have looked at many of them and they are pretty complicated. No need for me to reinvent the wheel and not sure I could do it anyway. I have many other programs I can be working on. I don't need anything extreme as the data is only phone nos. and email addresses. It is just that the site members don't want others to be able to view it.
What if I created a db password table, then simply verified that the entered password was in the file. What kind of security would I have?
Thanks
-
Damn, this stuff is killing me! I finally got it but only after hours of struggle.
It isn't the
].,} stuff that seems to get me. I just don't seem to understand the flow logic of php.
Is this the way it works?
($row = mysql_fetch_array( $result )); // $row is initially 1 and the result row name/s and value/s are stored in $result // so I would have row1,name1,name1-value,name2,name2-value, ect // the program continues and drops to the end (somewhere), and if I call for an echo // of row1 by name1 it will output name1-value // and if I think it might find more than one record then I simply add the while statement
Now to go back and clean it all up. Changing the db will be duck soup.
Thanks again guys.
-
I don't understand the code, but it fixed the first problem. The form only displays if select finds a record.
What does php and the right curly bracket do here?
</form> <?php } ?> </body>
Now for the second problem. It is obvious that php is not passing my form the variables I need. I know how to pass them from a form to php but how do I pass them from php to a form.
Simply...how do I make the data show up on the form?
-
Whoever programmed this forum is amazing.
I was typing a reply and got a message that a new reply had been posted while I was typing. WOW! Off the scale!
-
Ok, here is all the code.
<?php // change record in database // Connect to database ============================================ include("connect_db.php"); // retrieve form data from form2.html =================================== $id = $_POST['id']; // Send query =================================================== $query = "SELECT * FROM airplanes WHERE id='$id'"; if (!mysql_query($query)){ die('Error :' .mysql_error()); } $result = mysql_query ($query); $num = mysql_num_rows($result); // Check to see if record exists or not ==================================== if (mysql_num_rows($result) == 1) { mysql_query("Select FROM airplanes WHERE id='$id'"); print 'Record successfully retreived'; } // Record not in db =============================================== if (mysql_num_rows($result) == 0) { print '<big style="color: red;"><span style="font-weight: bold;"> ERROR MESSAGE:</span></big> Change ID number not found'; echo "<br />"; echo "<br />"; print 'Use the BACK button on your browser to try again'; echo "<br />"; } ?> <h3>Change this record</h3> <form action="insert.php" method="post"> AMA #: <input name="ama" type="text" value="<?php echo $ama; ?>"><br> Model Name: <input name="model_name" type="text" value="<?php echo model_name; ?>"><br> Model Mfg: <input name="model_mfg" type="text" value="<?php echo $model_mfg; ?>"><br> Wingspan: <input name="wingspan" type="text" value="<?php echo $wingspan; ?>"><br> Engine Mfg & Size: <input name="engine" type="text" value="<?php echo $engine; ?>"><br> Sound Reading: <input name="decibels" type="text" value="<?php echo $decibels; ?>"> Leave blank if you don't know it.<br> <input value="Send" type="submit"> </form> </body> </html>
This actually works, or my version does. Did a lot of deleting of test stuff like echos.
I actually have 2 problems but was trying to solve them one at a time.
1. The form will display whether I find a record or not. I only want it to display if I find a record.
2. When I find a record the form does not display any values.
I appreciate your patience.
-
I like your code better, but until then:
// form display logic if( empty($errors) ) { // include your form here. }
I still don't understand the include your form here.
I new this wouldn't work, which I interpret to be what you suggested.
if (mysql_num_rows($result) == 1) { mysql_query("Select FROM airplanes WHERE id='$id'"); <form action="insert.php" method="post"> AMA #: <input name="ama" type="text" value="<? echo $ama; ?>"> </form> }
And sure enough it didn't so I know I am missing something.
Let's say I delete the value=, still I am executing php and don't understand how to get the form to display inside the if the statement.
-
I am running IE7 (because my expensive HP printer will not work with IE8 and there is no fix for it) and it displays just fine.
-
select_display.php
// I am selecting an ID value from the db, result can only be 0 or 1 if (mysql_num_rows($result) == 0) { print '<big style="color: red;"><span style="font-weight: bold;"> ERROR MESSAGE:</span></big> Update ID number not found'; // I don't want my form to be displayed, just the error message } if (mysql_num_rows($result) == 1) { // Here I want to jump to html <form action="insert.php" method="post"> // I don't want to echo the whole form from within php // Now it displays on either compare because I am displaying it after ?> }
-
Many Thanks!
-
Well you can't have form1.html as a HTML file, it will need to be php. You then include retreive.php.
If that is the case, why do I need an include?
Example: You pull a name from the database and display it in the form
//connect to mysql //run query to grab the information from the database //loop through the query result set, putting each column into it's own variable //you should have a variable $name = $row["name"]; //start printing all of your form code //the HTML/PHP code for the name text field will be<input type="text" name="name" value="<?php echo $name; ?>"/> //end your form printing.
Can I do it like this?
retrieve_data.php
// php retrieve data // html create form and echo data into form, action=update.php method=post
update.php
//grab variables using $_POST['var_name']; //Update db
-
Thanks Denno, but I guess my request was not clear.
retrieve.php will get the data from the db.
form1.html will display the retrieved data.
update.php will update the db.
How do I get the retrieved variables from retrieve.php to form1.html?
-
I can add and delete data from my table. Now I need to be able to change one or more fields in an entry. So I want to retrieve a row from the db, display that data on a form where the user can change any field and then pass the changed data to an update.php program.
I know how to go from form to php. But how do I pass the data from retrieve.php to a form so it will display? Do I use a URL and Get? Can I put the retrieve and form in the same program?
-
As usual, you're dead on the money.
Thanks
-
Where is the setting for adding a signature or quote automatically to a reply?
For adding a picture in the left frame of a reply?
-
I appreciate you insight and time.
-
Sounds like you are doing exactly what I did when I wrote my first php program a couple of weeks ago. Since a .html program would execute and display in the browser, I figured a .php program would do the same thing. Doesn't exactly work that way if you don't have all the "goodies" installed on your PC.
I changed my file associations to open .php with wordpad because notepad screws up the format.
-
Oh my gosh! There it is for the whole world to see.
Will I ever learn all there is to learn? You people are killing me....haha I am struggling to program adds/changes/deletions to my db and now I have to go study server side validation.
One further question. All they would see in the protected area are names, phone numbers and email addresses and for sale/swap. Are there really people out there that look for this to spam emails?
-
I have a site designed with 3 frames: top=messages, left=navigation and right=content. All my programs use <base target="content"> so I never open a new window except to link to an outside site.
I have a simple password.html program written in javascript and the password is embedded in the program for comparison. I read where this is like no security at all. But no matter what program is displaying on the content.html frame, if you do a view/source from the browser, you always see the same thing, that is the frameset code.
So I am wondering where the security issue is.
Thanks
-
I know this is bad code but I got it to work. I tried using only the delete statement but could find no way to see if the record existed. I had a heck of a time getting the number of rows (there can only be one unique id). And finally, I could not get the if/else to work.
Would anyone care to show me how a pro would do it?
Thanks
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <?php // delete airplane in database // Connect to database ===================================================== include("connect_db.php"); // retrieve form data from form2.html ========================================== $id = $_POST['id']; // Send query =========================================================== $query = "SELECT * FROM airplanes WHERE id='$id'"; if (!mysql_query($query)){ die('Error :' .mysql_error()); } $result = mysql_query ($query); $num = mysql_num_rows($result); // Check to see if record exists ============================================== if (mysql_num_rows($result) == 1) { mysql_query("DELETE FROM airplanes WHERE id='$id'"); print 'Airplane successfully deleted'; } // Record not in db ========================================================= if (mysql_num_rows($result) == 0) { print '<big style="color: red;"><span style="font-weight: bold;"> ERROR MESSAGE:</span></big> ID number not found'; echo "<br />"; echo "<br />"; print 'Use the BACK button on your browser to try again'; echo "<br />"; } echo "<br />"; echo '<p>Return to <a href="members_menu.html">Members Menu Here</a></p>'; ?> </body> </html>
-
I previously was seeking advice on good php tutorials and where I could find good php scripts. Javascripts are plentiful but php scripts seem to be scarce.
I get the impression most don't value the tutorials and I was referred to a couple of books. I looked at them and like Larry Ullman's PHP 3rd edition and have ordered it.
But what I also found is he has over 100 free downloadable scripts here if you are interestred.
http://www.larryullman.com/books/php-for-the-web-visual-quickstart-guide-3rd-edition/
-
Thanks guys! I have looked at Larry Ullman's php 3rd edition and think I will try that for a starter.
-
Bingo!
That's some deep stuff. My hosts phpMyAdmin could not fix it.
I had two old fast cars, 68 and 69 AMX. Wish the hell I had kept them.
Thanks a jillion.
-
Using phpMyAdmin I loaded 6 test records with the id set to auto_increment and it loaded all the data correctly with id # 1-6.
Then from somewhere it got the number 333353 and auto_increments it as the value for the id. So now I have id's 1-6 and 333353, 333354, ect. For every record I add it increments it.
I deleted all but records 1-6 and tried again but it has the last value of 3333xx stored somewhere and increments it. Deleted them again, closed the program, came back and it still does it.
Can I create an executable link from a value in an output table?
in PHP Coding Help
Posted
In this output table, I would like to be able to click on any number in the ama row, add .html to that value and then execute the link.
Example: If the ama number in row 3 is 890543, add .html to the number = 890543.html, then click on it to link to that web page.