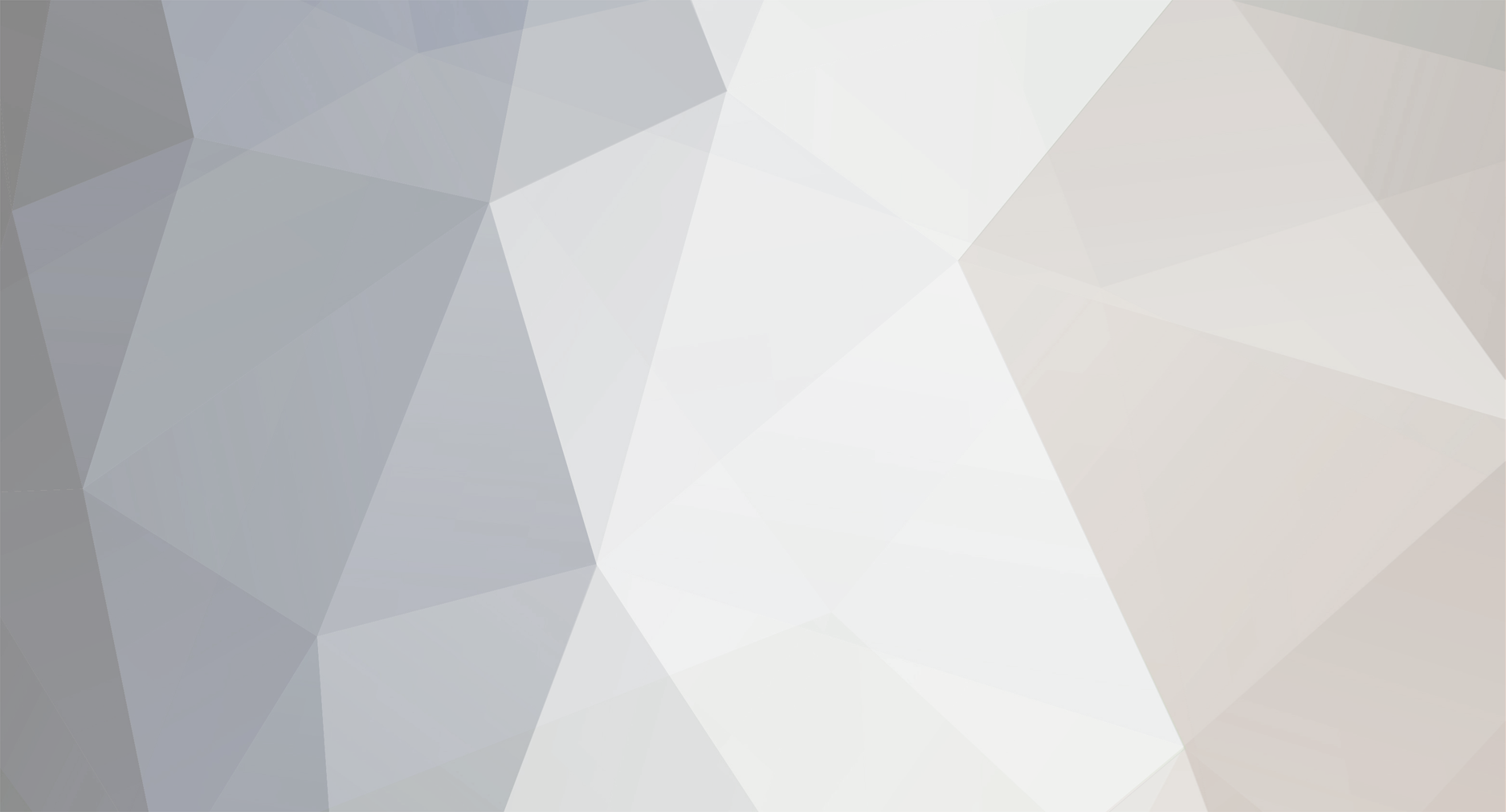
Bravat
Members-
Posts
69 -
Joined
-
Last visited
Never
Everything posted by Bravat
-
I found class on the net, and i am having a bit of a problem to understand how does update method works. Here is the code: public function update() { global $database; // Don't forget your SQL syntax and good habits: // - UPDATE table SET key='value', key='value' WHERE condition // - single-quotes around all values // - escape all values to prevent SQL injection $attributes = $this->sanitized_attributes(); $attribute_pairs = array(); foreach($attributes as $key => $value) { $attribute_pairs[] = "{$key}='{$value}'"; } $sql = "UPDATE ".self::$table_name." SET "; $sql .= join(", ", $attribute_pairs); $sql .= " WHERE id=". $database->escape_value($this->id); $database->query($sql); return ($database->affected_rows() == 1) ? true : false; } I have form like this to deal with update: <form action="index.php?page=languages" enctype="multipart/form-data" method="POST"> <?php foreach($language as $lang){ ?> <input type="hidden" name="MAX_FILE_SIZE" value="<?php echo $max_file_size; ?>" /> <label>Jezik</label><input type="text" size="50" name="language" value="<?php echo $lang->lang; ?>" /><br> <input type="hidden" name="id_lang" value="<?php echo $lang->id_lang; ?>" /> <label>Slika</label><input type="file" name="image"><?php echo "<img src=\"../images/"; echo $lang->image; echo "\">"; ?> <br> <label>Pozicija</label><input type="text" name="pozicija" value="<?php echo $lang->pozicija; ?>" size="2" /></p> <br> <input type="submit" name="submit_update" value="Unesi"> <?php } ?> </form> and code to start the function: if(isset($_POST['submit_update'])) { $language = new Jezik(); $language->update(); } What next???
-
I think the problem is that it only get one product_id, and when new one is set it erase the old one. How to keep adding new product_id data into $_SESSION['poredi']?
-
My bad, didn't delete the old session. There is no error, but when i add new product_id it just replace old one, instead of adding new one to the list.
-
That does the job, but now i am stumble upon the query. Here is code (not working): <?php if(isset($_SESSION['poredi'])){ $idp = $_SESSION['poredi']; $sql="SELECT * FROM product WHERE product_id IN ("; foreach($_SESSION['poredi'] as $id) { $sql.=$id.","; } $sql=substr($sql, 0, -1).") ORDER BY model ASC"; $query=mysql_query($sql); while($row=mysql_fetch_array($query)){ ?> <?php echo "<span class=\"korpa\">" . $row['model'] ?> <?php } ?>
-
How to add more data into $_SESSION? I have following code: if(isset($_GET['poredi']) && $_GET['poredi'] == "upo"){ $idp = intval($_POST['idp']); $sql = "SELECT * FROM product WHERE product_id={$idp}"; $query=mysql_query($sql); if(mysql_num_rows($query)!=0){ $row=mysql_fetch_array($query); $_SESSION['poredi'] = $row['product_id']; } } On click it need to store more product_id, and after that i have to be able to do query with data (find all data with product_id)?
-
That the thing i want. I need to mail order to the buyer, and in the while loop is all the data from the cart. I want that data to be subject in the mail function.
-
Here is the while loop: <?php require_once("../includes/initialize.php"); $sql="SELECT * FROM product WHERE product_id IN ("; foreach($_SESSION['cart'] as $id => $value) { $sql.=$id.","; } $sql=substr($sql, 0, -1).") ORDER BY model ASC"; $query=mysql_query($sql); $totalprice=0; while($row=mysql_fetch_array($query)){ $subtotal=$_SESSION['cart'][$row['product_id']]['quantity']*$row['price']; $totalprice+=$subtotal; ?> <tr> <td><?php echo $row['model'] ?></td> <td><?php echo $_SESSION['cart'][$row['product_id']]['quantity'] ?></td> <td><?php echo number_format($row['price'], 2, ',', '.'); ?>din</td> <td><?php $cena = $_SESSION['cart'][$row['product_id']]['quantity']*$row['price']; echo number_format($cena, 2, ',', '.'); ?>din</td> </tr> <?php } ?> How to get data out of the loop? I need it to be same as data echoed (this is the echo result: BFG 225/30 ZR 20 4 21.667,00din 86.668,00din).
-
I am wondering about the following problem: I have two sessions, one for the user ($_SESSION['user_id']) and second one for the products inside cart ($_SESSION['cart']). I need to insert data into table racun. I did it this way and it works, but i want to know if this can be done better? while ($row=mysql_fetch_array($query)){ $id = $_SESSION['korisnik_id']; $idp = $row['product_id']; $kol = $_SESSION['cart'][$row['product_id']]['quantity'] ."<br>"; $insert = "INSERT INTO racun (product_id, quantity, korisnik_id) VALUES ('$idp', '$kol', '$id') "; $result = mysql_query($insert); }
-
I followed tutorial from youtube http://www.youtube.com/watch?v=5b3TcoeY7Bs and made shopping cart. Everything is working fine, but there is one part I want to add. I want user to be able to set the quantity of products without going to the cart (user have to be able to enter quantity himself). At the moment I have no idea how to do this, i am still researching this topic, but wanted to try to ask for help from the pros . This is the complete code (a bit large): index.php: <?php session_start(); require("includes/connection.php"); if(isset($_GET['page'])){ $pages=array("products", "cart"); if(in_array($_GET['page'], $pages)) { $_page=$_GET['page']; }else{ $_page="products"; } }else{ $_page="products"; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <link rel="stylesheet" href="css/reset.css" /> <link rel="stylesheet" href="css/style.css" /> <title>Shopping Cart</title> </head> <body> <div id="container"> <div id="main"> <?php require($_page.".php"); ?> </div><!--end of main--> <div id="sidebar"> <h1>Cart</h1> <?php if(isset($_SESSION['cart'])){ $sql="SELECT * FROM products WHERE id_product IN ("; foreach($_SESSION['cart'] as $id => $value) { $sql.=$id.","; } $sql=substr($sql, 0, -1).") ORDER BY name ASC"; $query=mysql_query($sql); while($row=mysql_fetch_array($query)){ ?> <p><?php echo $row['name'] ?> x <?php echo $_SESSION['cart'][$row['id_product']]['quantity'] ?></p> <?php } ?> <hr /> <a href="index.php?page=cart">Go to cart</a> <?php }else{ echo "<p>Your Cart is empty. Please add some products.</p>"; } ?> </div><!--end of sidebar--> </div><!--end container--> </body> </html> cart.php: <?php if(isset($_POST['submit'])){ foreach($_POST['quantity'] as $key => $val) { if($val==0) { unset($_SESSION['cart'][$key]); }else{ $_SESSION['cart'][$key]['quantity']=$val; } } } ?> <h1>View cart</h1> <a href="index.php?page=products">Go back to products page</a> <form method="post" action="index.php?page=cart"> <table> <tr> <th>Name</th> <th>Quantity</th> <th>Price</th> <th>Items Price</th> </tr> <?php $sql="SELECT * FROM products WHERE id_product IN ("; foreach($_SESSION['cart'] as $id => $value) { $sql.=$id.","; } $sql=substr($sql, 0, -1).") ORDER BY name ASC"; $query=mysql_query($sql); $totalprice=0; while($row=mysql_fetch_array($query)){ $subtotal=$_SESSION['cart'][$row['id_product']]['quantity']*$row['price']; $totalprice+=$subtotal; ?> <tr> <td><?php echo $row['name'] ?></td> <td><input type="text" name="quantity[<?php echo $row['id_product'] ?>]" size="5" value="<?php echo $_SESSION['cart'][$row['id_product']]['quantity'] ?>" /></td> <td><?php echo $row['price'] ?>$</td> <td><?php echo $_SESSION['cart'][$row['id_product']]['quantity']*$row['price'] ?>$</td> </tr> <?php } ?> <tr> <td>Total Price: <?php echo $totalprice ?></td> </tr> </table> <br /> <button type="submit" name="submit">Update Cart</button> </form> <br /> <p>To remove an item set it's quantity to 0. </p> products.php: <?php if(isset($_GET['action']) && $_GET['action']=="add"){ $id=intval($_GET['id']); if(isset($_SESSION['cart'][$id])){ $_SESSION['cart'][$id]['quantity']++; }else{ $sql_s="SELECT * FROM products WHERE id_product={$id}"; $query_s=mysql_query($sql_s); if(mysql_num_rows($query_s)!=0){ $row_s=mysql_fetch_array($query_s); $_SESSION['cart'][$row_s['id_product']]=array( "quantity" => 1, "price" => $row_s['price'] ); }else{ $message="This product id it's invalid!"; } } } ?> <h1>Product List</h1> <?php if(isset($message)){ echo "<h2>$message</h2>"; } ?> <table> <tr> <th>Name</th> <th>Description</th> <th>Price</th> <th>Action</th> </tr> <?php $sql="SELECT * FROM products ORDER BY name ASC"; $query=mysql_query($sql); while ($row=mysql_fetch_array($query)) { ?> <tr> <td><?php echo $row['name'] ?></td> <td><?php echo $row['description'] ?></td> <td><?php echo $row['price'] ?>$</td> <td><a href="index.php?page=products&action=add&id=<?php echo $row['id_product'] ?>">Add to cart</a></td> </tr> <?php } ?> </table>
-
I have stored a text into DB with HTML tag. Is it possible to read it from DB and display in browser as tagged? Exemple of text: <p> O nama<br /> Iza Online prodaje guma stoji grupa mladih ljudi čiji je cilj da Vam omogući da na što jednostavniji način dođete do Vama potrebnih proizvoda. Za početak smo se odlučili za gume najpoznatijih svetskih proizvođača. U perspektivi nam je proširenje asortimana proizvoda na prateću opremu, auto-kozmetiku i sve ostalo što ide uz vašeg metalnog ljubimca.</p> <p> </p> <p> </p> <table cellpadding="2" cellspacing="2" width="100%"> <tbody> <tr> <td width="32%"> Naziv firme:</td> <td width="68%"> <img alt="" height="25" src="http://localhost/GumeOnline/image/data/petrovicLogo.jpg" width="194" /></td> </tr> <tr> <td> Poslovno sedište:</td> <td> Sutjeska 64, Pirot, Srbija</td> </tr> <tr> <td> Delatnost:</td> <td> Trgovina na malo posredstvom pošte ili preko interneta</td> </tr> <tr> <td> Šifra delatnosti:</td> <td> 4791</td> </tr> <tr> <td> Matični broj:</td> <td> 62244089</td> </tr> <tr> <td> PIB:</td> <td> 106760742</td> </tr> <tr> <td> Br. računa:</td> <td> INTESA 160-341869-50</td> </tr> <tr> <td> Web adresa:</td> <td> <a href="http://www.gumeonline.co.rs/">www.gumeonline.co.rs</a></td> </tr> <tr> <td> Kontakt telefon:</td> <td> 0616860008</td> </tr> <tr> <td> Kontakt e-mail adresa:</td> <td> <a href="mailto:info@gumeonline.co.rs">nfo@gumeonline.co.rs</a><br /> <a href="mailto:komercijala@gumeonline.co.rs">komercijala@gumeonline.co.rs</a></td> </tr> </tbody> </table> <p> </p> <table cellpadding="2" cellspacing="2" width="100%"> </table> <p> Za sva pitanja i sugestije kontaktirajte nas putem mail-a na<br /> info@gumeonline.co.rs</p>
-
Well... human stupidity is so infinitive . Problem was in == (wonder why i put the). Just to say this is the best forum for helping novice PHP "programmers" . Thank you for help.
-
Only one record. This is an attempt of login system.
-
But when i use this line: echo '<br>$row[\'ime\'] = [ ' . $ime . ' ]<br>'; there is a positive result: $row['ime'] = [ Sasha ]
-
This is the result: $row['ime'] = [ ]
-
The same error is reported: Here is the code again ( basically changed first line and the $_SESSION lines): <?php session_start(); ?> <?php require_once("public/includes/connection.php"); ?> <?php if(!$_POST['submit']){ header('refresh:0; url=index.php');} else { $korisnik = $_POST['korisnicko_ime']; $lozinka = $_POST['lozinka']; $lozinka_db = sha1($lozinka); if ($korisnik && $lozinka){ $query = mysql_query("SELECT * FROM korisnik WHERE korisnicko_ime = '$korisnik' "); $numrow = mysql_num_rows($query); if($numrow != 0){ while ($row = mysql_fetch_assoc($query)){ $korisnik_db = $row['korisnicko_ime']; $db_lozinka = $row['lozinka']; $ime = $row['ime']; } if ($korisnik == $korisnik_db && $lozinka_db == $db_lozinka){ echo "Uspesno ste ulogovani"; $_SESSION['username'] == $ime; echo $_SESSION['username']; } else { echo "Lozinka je netacna"; } } else {die("Korisnik ne postoji");} } } ?>
-
Changed it to: $_SESSION['username'] == $ime; echo $_SESSION['username']; and it still does not work
-
I have this error, and I cann't find soluiton: Notice: Undefined index: ime in C:\wamp\www\web\login_public.php on line 26 Notice: Undefined index: ime in C:\wamp\www\web\login_public.php on line 27 This is the code: <?php require_once("public/includes/session.php"); ?> <?php require_once("public/includes/connection.php"); ?> <?php if(!$_POST['submit']){ header('refresh:0; url=index.php');} else { $korisnik = $_POST['korisnicko_ime']; $lozinka = $_POST['lozinka']; $lozinka_db = sha1($lozinka); if ($korisnik && $lozinka){ $query = mysql_query("SELECT * FROM korisnik WHERE korisnicko_ime = '$korisnik' "); $numrow = mysql_num_rows($query); if($numrow != 0){ while ($row = mysql_fetch_assoc($query)){ $korisnik_db = $row['korisnicko_ime']; $db_lozinka = $row['lozinka']; $ime = $row['ime']; } if ($korisnik == $korisnik_db && $lozinka_db == $db_lozinka){ echo "Uspesno ste ulogovani"; $_SESSION['ime'] == $ime; echo $_SESSION['ime']; } else { echo "Lozinka je netacna"; } } else {die("Korisnik ne postoji");} } } ?> What seems to be the problem
-
Solved the problem. I created new file that does only inserting and redirect back. It work fine. Thank you all for help
-
None of the data is inserted. This is redirect_to function: function redirect_to( $location = NULL ) { if ($location != NULL) { header("Location: {$location}"); exit; } } Also tried to move header.php after INSERT part. still won't work.
-
I don't get it (maybe becouse of late hours and not sleeping for 30h...). I deleted include/header.php but it stil wont work???
-
Well this is the header: <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <link href="stylesheets/public.css" rel="stylesheet"/> <script src="SpryAssets/SpryValidationTextField.js" type="text/javascript"></script> <link href="SpryAssets/SpryValidationTextField.css" rel="stylesheet" type="text/css" /> </head> <body> <div id="wrapper"> <div id="header"> <div id="headerWrapper"> <div id="slikaHeader"> <?php $query = "SELECT slika FROM prodavnica"; $result = mysql_query($query); $row = mysql_fetch_array($result); ?> <a href="index.php"><img src="<?php echo $row['slika'];?>" /></a> </div> <!--Kraj na slikaHeader id--> <div id="loginHeader"> <form action="login_public.php" method="post"> <label>Korisnicko ime:</label> <input name="korisnicko_ime" type="text" /> <br /> <label>Lozinka:</label> <input name="lozinka" type="password" /> <br /> <label><a href="new_user.php">Kreiraj novog korisnika</a></label> <input type="submit" name="submit" value="Prijava" style="float:right" /> </form> </div> </div> <!--Kraj na headerWrapper id--> </div> <!--Kraj na header id--> connection.php is just conn definition, and other includes are with out html. I will try something. Hope it will work.
-
I have problem with this code. It does absolutely nothing. When INSERT is over it should redirect to index.php but it does nothing. There is no error, when the submit is clicked the page just refresh itself and because of echo function it write all the values. What seems to be the problem (I going slightly mad ) <?php require_once("public/includes/session.php"); ?> <?php require_once("public/includes/connection.php"); ?> <?php require_once("public/includes/functions.php"); ?> <?php include_once("public/includes/form_functions.php"); include_once("public/includes/header.php"); if (isset($_POST['submit'])) { // Form has been submitted. $errors = array(); $required_fields = array('nik', 'lozinka', 'ime', 'prezime', 'adresa', 'grad', 'postanskiBroj', 'fiskni', 'moblini', 'email'); $errors = array_merge($errors, check_required_fields($required_fields, $_POST)); $username = trim(mysql_prep($_POST['nik'])); $password = trim(mysql_prep($_POST['lozinka'])); $hashed_password = sha1($password); $ime = trim(mysql_prep($_POST['ime'])); $prezime = trim(mysql_prep($_POST['prezime'])); $adresa = trim(mysql_prep($_POST['adresa'])); $grad = trim(mysql_prep($_POST['grad'])); $postanskiBroj = trim(mysql_prep($_POST['postanskiBroj'])); $fiskni = trim(mysql_prep($_POST['fiksni'])); $moblini = trim(mysql_prep($_POST['mobilni'])); $email = trim(mysql_prep($_POST['email'])); echo $username . $hashed_password . $ime . $prezime . $adresa . $grad . $postanskiBroj . $fiskni . $moblini . $email; if ( empty($errors) ) { $query = " INSERT INTO `gume`.`korisnik` (`id`, `korisnicko_ime`, `lozinka`, `ime`, `prezime`, `adresa`, `grad`, `postanskiBroj`, `fiksni_telefon`, `mobilni_telefon`, `email`) VALUES (NULL, '$username', '$hashed_password', '$ime', '$prezime', '$adresa', '$grad', '$postanskiBroj', '$fiskni, $moblini', '$email' )"; $result = mysql_query($query, $connection) or die(mysql_error); if ($result) { redirect_to("index.php"); } else { $message = "The user could not be created."; $message .= "<br />" . mysql_error(); } } else { if (count($errors) == 1) { $message = "There was 1 error in the form."; } else { $message = "There were " . count($errors) . " errors in the form."; } } } else { // Form has not been submitted. $username = ""; $password = ""; } ?> <div id="telo"> <div id="kreiranjeNaloga"> <script src="SpryAssets/SpryValidationTextField.js" type="text/javascript"></script> <link href="SpryAssets/SpryValidationTextField.css" rel="stylesheet" type="text/css" /> <script src="SpryAssets/SpryValidationPassword.js" type="text/javascript"></script> <script src="SpryAssets/SpryValidationConfirm.js" type="text/javascript"></script> <link href="SpryAssets/SpryValidationPassword.css" rel="stylesheet" type="text/css" /> <link href="SpryAssets/SpryValidationConfirm.css" rel="stylesheet" type="text/css" /> <p>Polja sa * su obavezna</p> <form action="new_user.php" method="post"> <span id="sprytextfield1"> <label>Korisnicko ime: </label> <input type="text" name="nik" id="nik" size="40" value=""/> *<span class="textfieldMinCharsMsg">Korisnicko ime ne moze imati manje od 5 karaktera</span><span class="textfieldMaxCharsMsg">Korisnicko ime moze imati najvise 30 karaktera.</span></span><br /> <span id="sprypassword1"> <label>Lozinka:</label> <input type="password" name="lozinka" id="lozinka" size="40" value=""/> *<span class="passwordMinCharsMsg">Sifra mora sadrzati najmanje 5 karaktera.</span><span class="passwordMaxCharsMsg">Sifra moze imati najvise 30 karaktera.</span></span> <br /> <span id="spryconfirm1"> <label>Potvrdite lozinku:</label> <input type="password" name="password1" id="password1" size="40" value=""/> <span class="confirmRequiredMsg">*</span>Obe lozinke moraju da budu iste.</span> <br /> <span id="sprytextfield2"> <label>Ime:</label> <input type="text" name="ime" id="ime" size="40" value=""/> * </span> <br /> <span id="sprytextfield3"> <label>Prezima</label> <input type="text" name="prezime" id="prezime"size="40" value="" /> *</span> <br /> <span id="sprytextfield4"> <label>Adresa:</label> <input type="text" name="adresa" id="adresa" size="40" value=""/> * </span> <br /> <span id="sprytextfield7"> <label>Grad:</label> <input type="text" name="grad" id="grad" size="40" value="" /> * </span> <br /> </span><span id="sprytextfield9"> <label>Postanski Broj: </label> <input type="text" name="postanskiBroj" id="postanskiBroj" size="10" value=""/> * <span class="textfieldInvalidFormatMsg">Postanski broj nije pravilno upisan.</span></span><br /> <span id="sprytextfield5"> <label>Broj fiksnog telefona: </label> <input type="text" name="fiksni" id="Broj fiksnog telefona" size="40" value="" /> *<span class="textfieldInvalidFormatMsg">Broj telefona nije pravilno upisan</span></span> <br /> <span id="sprytextfield6"> <label>Broj mobilnog telefona: </label> <input type="text" name="mobilni" id="mobilni" size="40" value="" /> *<span class="textfieldInvalidFormatMsg">Broj telefona nije pravilno upisan</span></span><br /> <span id="sprytextfield10"> <label>Email:</label> <input type="text" name="email" id="email" size="40" value="" /> *<span class="textfieldInvalidFormatMsg">Email adresa nija pravilno upisana.</span></span><br /> <input name="submit" type="submit" id="submit" value="Kreiraj korisnika" /> </form> </div> </div> <?php include("public/includes/footer.php"); ?>
-
How to define default folder. For example I have folder images and in that folder specific image. In the root directory I have index.php with <?php require_once("public/includes/header.php"); ?> and in index.php there are links which goes to different folder: <a href="public/sajt/kategorija.php?id=<?php echo $id; ?>"> which also have header.php, but there is no image. How to make default folder for image, or some similar solution?
-
Well this is an embarrassing moment Thank you