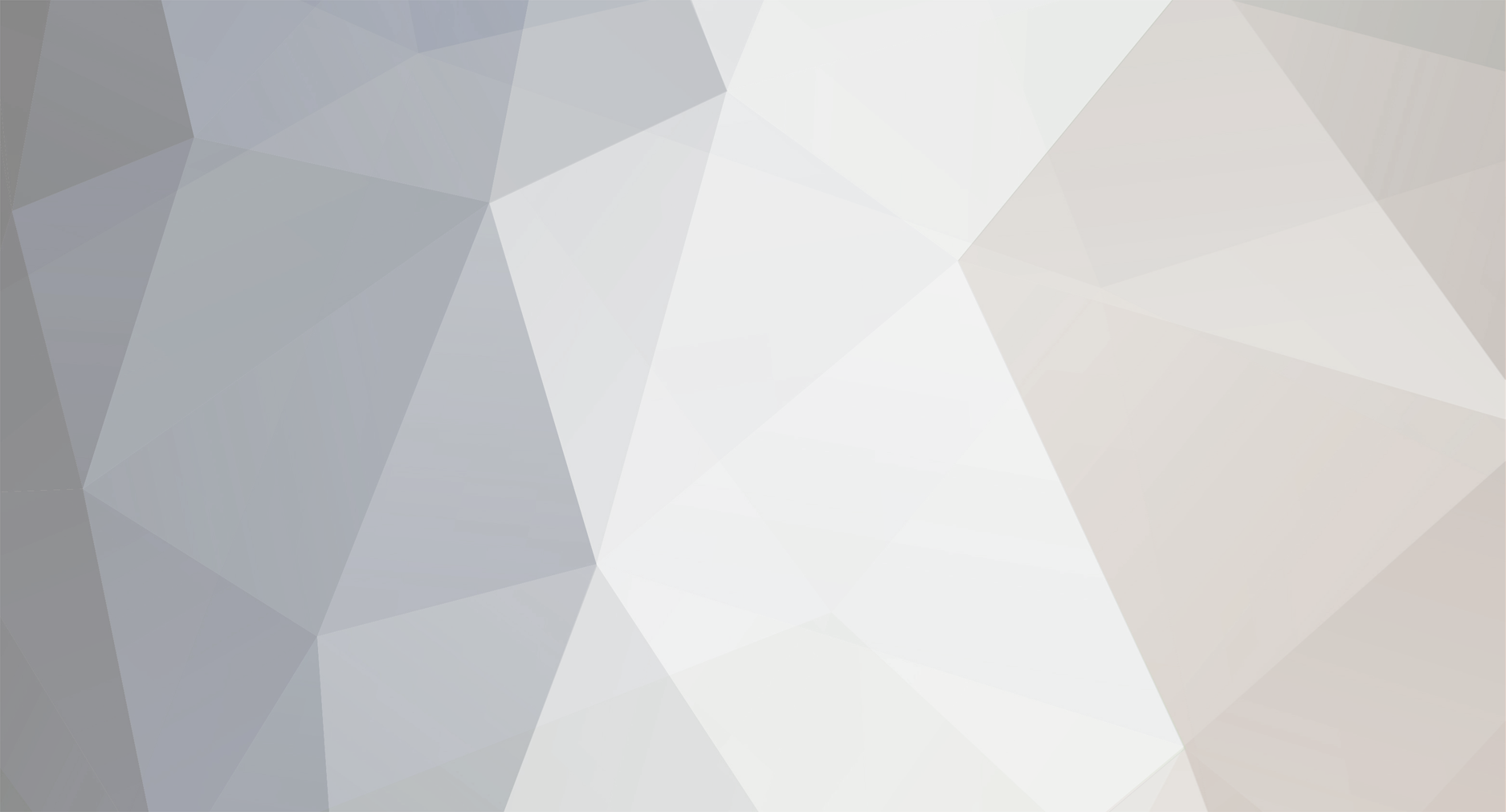
Kush
Members-
Posts
39 -
Joined
-
Last visited
Never
Everything posted by Kush
-
Okay. Is it common for the socket to take 4 seconds in total for each connection? It's recommended on 1and1 that you only run a cron job every 5 minutes, because it could queue up the server a lot, but I want to run the script every minute or minute and a half so the database is semi-current... do you think that would be too much stress on the server?
-
Hello, I'm trying to update my database with current statistics from my gameservers, but running the loop of socket connections to all of the servers on page load takes too long. For every server in the array it takes roughly 4 seconds to complete each one.. with a massive list of say 42 it takes awhile. However, I'm running from shared hosting and was wondering if that was the reason each server takes so long to query. I was thinking about running a cron job every 1 minute to update the information so it's still relativity new and current. Will the 1 minute cron job affect anything from the shared hosting? I'm using 1and1 web-hosting on a 1&1 Business Package. Thanks
-
btherl, thank you!
-
So what do I do now?
-
Apparently so.. results:
-
When doing this: var_dump($command); echo "<br>"; var_dump($status); It output the same exact string (visibly at least), but the starting of the var_dump was different.. $command had string(228) "hostname: ..etc..etc.. this all was exactly the same" $status had string(238) "hostname: ..etc..etc.. this all was exactly the same" When I tried doing: $serverInformation = getServerInformation($command); This was the result: Array ( [hostname] => Hooter's GG 5.1 Elimination HLstatsX:CE [version] => 1.0.0.58/15 4426 [secure ip] => 25.2.132.39:27015 [map] => fy_dustdm [at] => 0 x, 0 y, 0 z [players] => 0 (20 max) ) This is the result when I tried getServerInformation() with the variable $status: Array ( [hostname] => [version] => [secure ip] => [map] => [at] => [players] => )
-
Whoops, accidentally removed the ending bracket in the rcon class when copy and pasting.. added the ?> myself.. sorry. The class works fine and the bracket is there.. just missed it copying and pasting.
-
Oh, forgot to add the "getServerInformation()", but that's just the regex part: function getServerInformation($string) { $data = array( 'hostname' => "~hostname: (.*?) version :~", 'version' => "~version : (.*?) secure udp/ip :~", 'secure ip' => "~secure udp/ip : (.*?) map :~", 'map' => "~map : (.*?) at:~", 'at' => "~at: (.*?) players :~", 'players' => "~players : (.*) #~" ); foreach($data as $varName => $pattern) { preg_match($pattern, $string, $matches); $data[$varName] = $matches[1]; } return $data; } Also, in the code provided when I use the var $command to getServerInformation() it works just fine, but it's supposed to be the same string the socket returns..
-
$r = new rcon("25.2.132.39", 27015, "serverpasswordherebutiremovedit"); if(!$r->isValid()) die("Unable to connect to server."); if(!$r->Auth()) die("Unable to authenticate! Wrong password?"); $command = "hostname: Hooter's GG 5.1 Elimination HLstatsX:CE version : 1.0.0.58/15 4426 secure udp/ip : 25.2.132.39:27015 map : fy_dustdm at: 0 x, 0 y, 0 z players : 0 (20 max) # userid name uniqueid connected ping loss state adr "; $status = $r->sendRconCommand("status"); var_dump($status); $serverInformation = getServerInformation($status); Here's the rcon class: <?php /* CS:S Rcon PHP Class - code by 1FO|zyzko 01/12/2005 www.1formatik.com - www.1fogames.com -------------------------------------------------- */ define("SERVERDATA_EXECCOMMAND", 02); define("SERVERDATA_AUTH", 03); class rcon { var $Password; var $Host; var $Port = 27015; var $_Sock = null; var $_Id = 0; var $valid = false; function __construct($Host,$Port,$Password) { return $this->init($Host,$Port,$Password); } function rcon ($Host,$Port,$Password) { return $this->init($Host,$Port,$Password); } function init($Host,$Port,$Password) { $this->Password = $Password; $this->Host = $Host; $this->Port = $Port; $this->_Sock = @fsockopen($this->Host,$this->Port, $errno, $errstr, 30);// or //die("Unable to open the port: $errstr ($errno)\n"+$this->Host+":"+$this->Port); if($this->_Sock) { $this->_Set_Timeout($this->_Sock,2,500); $this->valid = true; } } function isValid() { return $this->valid; } function Auth() { $PackID = $this->_Write(SERVERDATA_AUTH, $this->Password); $ret = $this->_PacketRead(); if ($ret[1]['ID'] == -1) { return 0; } else { return 1; } } function _Set_Timeout(&$res,$s,$m=0) { if (version_compare(phpversion(),'4.3.0','<')) { return socket_set_timeout($res,$s,$m); } return stream_set_timeout($res,$s,$m); } function _Write($cmd, $s1='', $s2='') { $id = ++$this->_Id; $data = pack("VV",$id,$cmd).$s1.chr(0).$s2.chr(0); $data = pack("V",strlen($data)).$data; fwrite($this->_Sock,$data,strlen($data)); return $id; } function _PacketRead() { $retarray = array(); while ($size = @fread($this->_Sock,4)) { $size = unpack('V1Size',$size); if ($size["Size"] > 4096) { $packet = "\x00\x00\x00\x00\x00\x00\x00\x00".fread($this->_Sock,4096); } else { $packet = fread($this->_Sock,$size["Size"]); } array_push($retarray,unpack("V1ID/V1Reponse/a*S1/a*S2",$packet)); } return $retarray; } function Read() { $Packets = $this->_PacketRead(); foreach($Packets as $pack) { if (isset($ret[$pack['ID']])) { $ret[$pack['ID']]['S1'] .= $pack['S1']; $ret[$pack['ID']]['S2'] .= $pack['S1']; } else { $ret[$pack['ID']] = array( 'Reponse' => $pack['Reponse'], 'S1' => $pack['S1'], 'S2' => $pack['S2'], ); } } return $ret; } /** * Send an rcon command the server. The command must be properly formatted before * sending to this method. This means that variables that require quotes must have * quotes put around them. * Ex. $command = "kickid ". "\"".$steamId."\" ".$banReason */ function sendRconCommand($command) { $this->_Write(SERVERDATA_EXECCOMMAND, $command, ''); $ret = $this->Read(); return $ret[$this->_Id]['S1']; } ?>
-
I'm trying to do some regex, but I'm having trouble with my input string for the regex. I'm querying a server and getting results from that via socket and trying to regex the results and extract content. I've got the regex working (thanks to mjdamato ), but it only works when I have the regex string like this: $input = "hostname: Hooter's GG 5.1 Elimination HLstatsX:CE version : 1.0.0.58/15 4426 secure udp/ip : 25.2.132.39:27015 map : fy_dustdm at: 0 x, 0 y, 0 z players : 0 (20 max) # userid name uniqueid connected ping loss state adr "; When I get the results from my socket it's stored in a variable and it doesn't work when it's exactly the same. I did var_dump on the results from the socket and this is what it outputs: string(229) "hostname: Hooter's GG 5.1 Elimination HLstatsX:CE version : 1.0.0.58/15 4426 secure udp/ip : 25.2.132.39:27015 map : fy_dustdm at: 0 x, 0 y, 0 z players : 0 (20 max) # userid name uniqueid connected ping loss state adr " Since it's exactly the same why doesn't the second way work? Let me know if this is too confusing..
-
I'm reading this string from a socket and apparently it's not working. When testing and using it as a string like you did in the actual script like: $input = "hostname: Hooter's GG 5.1 Elimination HLstatsX:CE version : 1.0.0.58/15 4426 secure udp/ip : 25.2.132.39:27015 map : fy_dustdm at: 0 x, 0 y, 0 z players : 0 (20 max) # userid name uniqueid connected ping loss state adr "; It works, but when I pass in what comes back from the socket it returns with an empty array: Array ( [hostname] => [version] => [secure ip] => [map] => [at] => [players] => ) I do a var dump and this is what comes back: string(229) "hostname: Hooter's GG 5.1 Elimination HLstatsX:CE version : 1.0.0.58/15 4426 secure udp/ip : 25.2.132.39:27015 map : fy_dustdm at: 0 x, 0 y, 0 z players : 0 (20 max) # userid name uniqueid connected ping loss state adr " Is there a way I can make it a string like the way I did in the script?
-
I'm trying to extract information from a string that's going to have changing content. There's a possibility that more useless content added to the end of this string, but that stuff doesn't matter. I've put the content I want to extract in bold & underline. The content I want to extract is going to be different for each time though, but some of the stuff is going to be constant. Here's what would remain constant: Everything else will be different (need to extract multiple strings of the same format with different content for what I want to extract) so I'm not exactly sure how to handle this. For example, the "hostname" ([GE] 24/7 OFFICE N00b Training Facility - GangsterElite.com) which I want to extract could be anything same with the "map" and "players". I'm guessing that I would have to use regex or split the string somehow, but I've never really been good at that and I absolutely dread it. Help? :'(