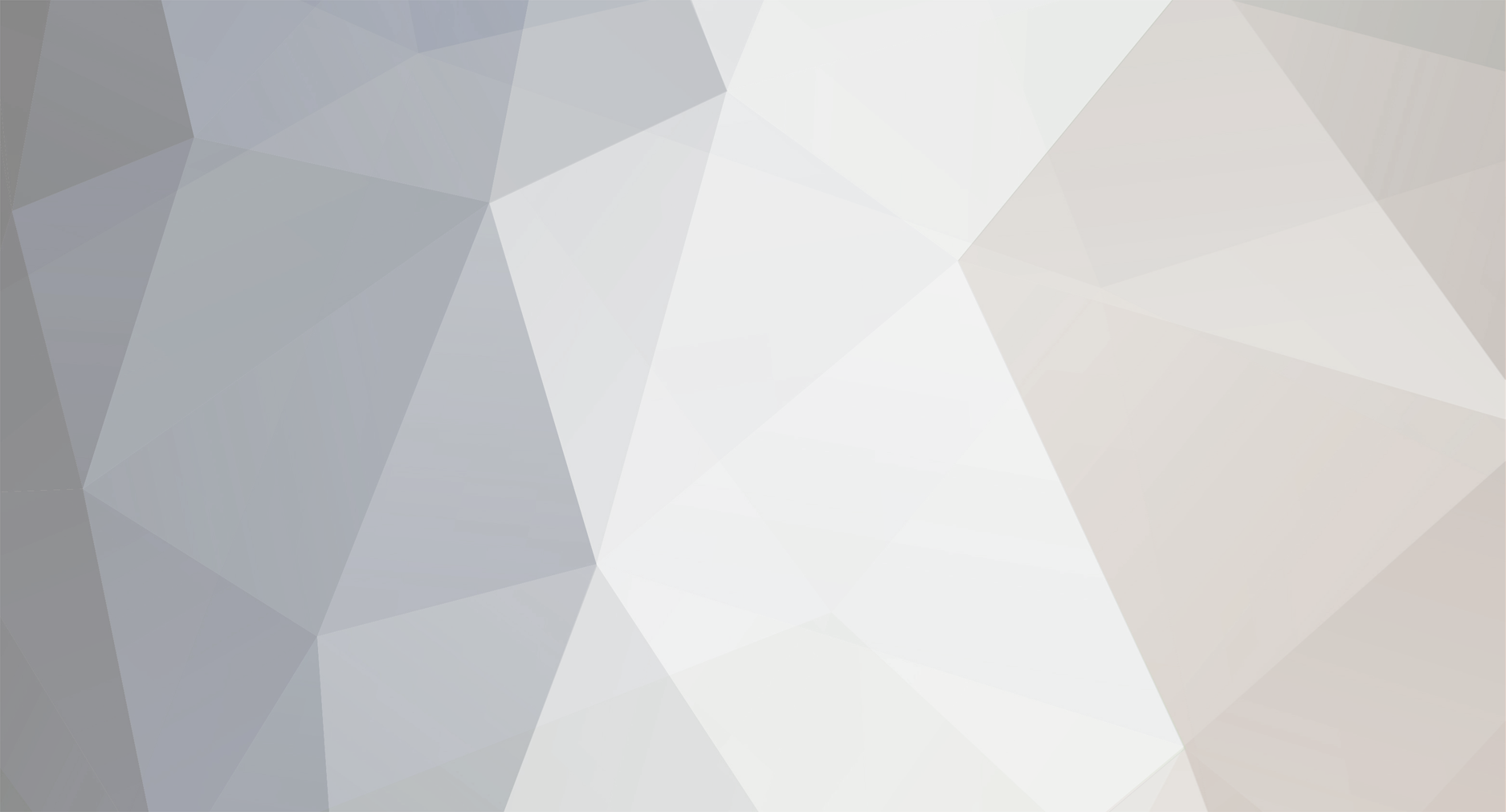
webby121
Members-
Posts
15 -
Joined
-
Last visited
Never
Profile Information
-
Gender
Not Telling
webby121's Achievements

Newbie (1/5)
0
Reputation
-
This is my code! <?php // Set error message as blank upon arrival to page $errorMsg = ""; // First we check to see if the form has been submitted if (isset($_POST['firstname'])){ //Connect to the database through our include include_once "connect_to_mysql.php"; // Filter the posted variables $accounttype = ereg_replace("[^a-z]", "", $_POST['accounttype']); // filter everything but lowercase letters $companyname = ereg_replace("[^A-Za-z]", "", $_POST['companyname']); // filter everything but letters $firstname = ereg_replace("[^A-Za-z]", "", $_POST['firstname']); // filter everything but letters $lastname = ereg_replace("[^A-Za-z]", "", $_POST['lastname']); // filter everything but letters $d_m = ereg_replace("[^A-Za-z]", "", $_POST['d_m']); // filter everything but letters $d_d = ereg_replace("[^0-9]", "", $_POST['d_d']); // filter everything but number $d_y = ereg_replace("[^0-9]", "", $_POST['d_y']); // filter everything but number $housenumber = ereg_replace("[^0-9]", "", $_POST['housenumber']); // filter everything but number $address = ereg_replace("[^A-za-z]", "", $_POST['address']); // filter everything but number $address1 = ereg_replace("[^A-za-z]", "", $_POST['address1']); // filter everything but number $town = ereg_replace("[^A-Za-z]", "", $_POST['town']); // filter everything but letters $postcode = ereg_replace("[^A-Z a-z0-9]", "", $_POST['postcode']); // filter everything but letters and numbers and spaces $phone = ereg_replace("[^0-9]", "", $_POST['postcode']); // filter everything but numbers $studentnumber = ereg_replace("[^0-9]", "", $_POST['studentnumber']); // filter everything but numbers $username = ereg_replace("[^A-Za-z0-9]", "", $_POST['username']); // filter everything but numbers and letters $email = stripslashes($_POST['email']); $email = strip_tags($email); $email = mysql_real_escape_string($email); $password = ereg_replace("[^A-Za-z0-9]", "", $_POST['password']); // filter everything but numbers and letters // Check to see if the user filled all fields with // the "Required"(*) symbol next to them in the join form // and print out to them what they have forgotten to put in if((!$accounttype) || (!$firstname) || (!$lastname) || (!$d_d) || (!$d_m) || (!$d_y) || (!$housenumber)|| (!$address)|| (!$town)|| (!$postcode)|| (!$phone) || (!$username)|| (!$email)|| (!$confirmemail)|| (!$password) || (!$confirmpassword) ){ $errorMsg = "You did not submit the following required information!<br /><br />"; if(!$accounttype){ $errorMsg .= "--- Account Type"; } else if(!$firstname){ $errorMsg .= "--- First Name"; } else if(!$lastname){ $errorMsg .= "--- Last Name"; } else if(!$d_d){ $errorMsg .= "--- Date of Birth Day"; } else if(!$d_m){ $errorMsg .= "--- Date of Birth Month"; } else if(!$d_y){ $errorMsg .= "--- Date of Birth Year"; } else if(!$housenumber){ $errorMsg .= "--- Housenumber"; } else if(!$address){ $errorMsg .= "--- Address"; }else if(!$town){ $errorMsg .= "--- Town"; }else if(!$postcode){ $errorMsg .= "--- Postcode"; }else if(!$phone){ $errorMsg .= "--- Phone"; }else if(!$username){ $errorMsg .= "--- Username"; }else if(!$email){ $errorMsg .= "--- Email"; }else if(!$password){ $errorMsg .= "--- Password"; } } else { // Database duplicate Fields Check $sql_username_check = mysql_query("SELECT id FROM member WHERE username='$username' LIMIT 1"); $sql_email_check = mysql_query("SELECT id FROM members WHERE email='$email' LIMIT 1"); $username_check = mysql_num_rows($sql_username_check); $email_check = mysql_num_rows($sql_email_check); } if ($username_check > 0){ $errorMsg = "<u>ERROR:</u><br />Your User Name is already in use inside our system. Please try another."; } else if ($email_check > 0){ $errorMsg = "<u>ERROR:</u><br />Your Email address is already in use inside our system. Please try another."; } if($password != $confirmpassword) { echo "Passwords do not match. Please try again."; } if($email != $confirmemail) { echo "Emails do not match. Please try again."; } else { // Add MD5 Hash to the password variable $hashedPass = md5($password); // Convert Birthday to a DATE field type format(YYYY-MM-DD) out of the month, day, and year supplied $dateofbirth = "$d_y-$d_m-$d_d"; // Add user info into the database table, claim your fields then values $sql = mysql_query("INSERT INTO member (username, companyname, firstname, lastname, dateofbirth, accounttype, email, password, lastlogin, phone) VALUES('$username','$companyname','$firstname','$lastname','$dateofbirth','$accounttype','$email', '$hashedPass',now(),'$phone')") or die (mysql_error()); $sql = mysql_query("INSERT INTO address (housenumber, postcode, town, address, address1) VALUES('$housenumber','$postcode','$town','$address','$address1')") or die (mysql_error()); $sql = mysql_query("INSERT INTO student (studentnumber) VALUES('$studentnumber')") or die (mysql_error()); // Get the inserted ID here to use in the activation email $id = mysql_insert_id(); // Create directory(folder) to hold each user files(pics, MP3s, etc.) mkdir("memberFiles/$id", 0755); // Start assembly of Email Member the activation link $to = "$email"; // From $from = "http://users.ecs.soton.ac.uk/alw3g08/index.php"; $subject = "Complete your registration"; //Begin HTML Email Message where you need to change the activation URL inside $message = '<html> <body bgcolor="#FFFFFF"> Hi ' . $username . ', <br /><br /> You must complete this step to activate your account with us. <br /><br /> Please click here to activate now >> <a href="http://users.ecs.soton.ac.uk/alw3g08/activation.php?id=' . $id . '"> ACTIVATE NOW</a> <br /><br /> Your Login Data is as follows: <br /><br /> E-mail Address: ' . $email . ' <br /> Password: ' . $password . ' <br /><br /> Thanks! </body> </html>'; // end of message $headers = "From: $from\r\n"; $headers .= "Content-type: text/html\r\n"; $to = "$to"; // Finally send the activation email to the member mail($to, $subject, $message, $headers); // Then print a message to the browser for the joiner print "<br /><br /><br /><h4>OK $firstname, one last step to verify your email identity:</h4><br /> We just sent an Activation link to: $email<br /><br /> <strong><font color=\"#990000\">Please check your email inbox in a moment</font></strong> to click on the Activation <br /> Link inside the message. After email activation you can log in."; exit(); // Exit so the form and page does not display, just this success message } // Close else after database duplicate field value checks } // Close else after missing vars check //Close if $_POST ?>
-
Anyone willing to offer some advice?
-
Hi there, im trying to create a sign up page for my site! I have used confirm password and email fields on the form but the code still shows the error message even if the passwords and emails match! Can anyone advice me please? [attachment deleted by admin]
-
dont think i am! any ideas of where the other brace should be?
-
Can anyone tell me where the missing brace is please? thanks
-
sorry im a very basic programmer as you can tell so can you please show me where i need to change it please? Thanks
-
Hi nothing seems to work! No error messages appear and no data enters my database! Can people please help me please! Needs to be solved by today! Thanks <?php //connect to db $connect = mysql_connect("l", "", ""); mysql_select_db("", $connect); //if submit button gets pressed if(isset($_POST['submit'])){ //Grab data from the form $username = preg_replace('#[^A-Za-z0-9]#i','', $_POST['username']); // filter everything but letters and numbers $firstname = preg_replace('#[^A-Za-z]#i', '', $_POST['firstname']); // filter everything but Letters $lastname = preg_replace('#[^A-Za-z]#i', '', $_POST['lastname']); // filter everything but Letters $phone = preg_replace('#[^0-9]#i', '', $_POST['phone']); // filter everything but numbers $address= preg_replace('#[^A-Za-z]#i', '', $_POST['address']); // filter everything but Letters $postcode= preg_replace('#[^A-Za-z]#i', '', $_POST['postcode']); // filter everything but Letters $town= preg_replace('#[^A-Za-z]#i', '', $_POST['town']); // filter everything but Letters $housenumber= preg_replace('#[^0-9]#i', '', $_POST['housenumber']); // filter everything but numbers $b_m = preg_replace('#[^0-9]#i', '', $_POST['birth_month']); // filter everything but numbers $b_d = preg_replace('#[^0-9]#i', '', $_POST['birth_day']); // filter everything but numbers $b_y = preg_replace('#[^0-9]#i', '', $_POST['birth_year']); // filter everything but numbers $email1 = mysql_real_escape_string (stripslahes (strip_tags($_POST['email1']))); $email2 = mysql_real_escape_string (stripslahes (strip_tags($_POST['email2']))); $pass1 = md5(mysql_real_escape_string (stripslahes (strip_tags($_POST['pass1'])))); $pass2 = stripslashes(strip_tags($_POST['pass2'])); $emailCHecker = mysql_real_escape_string($email1); $emailCHecker = str_replace("`", "", $emailCHecker); // Database duplicate username check setup for use below in the error handling if else conditionals $sql_uname_check = mysql_query("SELECT username FROM member WHERE username='$username'"); $uname_check = mysql_num_rows($sql_uname_check); // Database duplicate e-mail check setup for use below in the error handling if else conditionals $sql_email_check = mysql_query("SELECT email FROM member WHERE email='$emailCHecker'"); $email_check = mysql_num_rows($sql_email_check); // Convert Birthday to a DATE field type format(YYYY-MM-DD) out of the month, day, and year supplied $dateofbirth = "$b_y-$b_m-$b_d"; //If any errors have been found, DO NOT register the member, and instead, redisplay the form } if (!isset($username) || !isset($firstname) || !isset ($lastname) || !isset($address) || !isset($postcode) || !isset($town) || !isset($b_m) || !isset($b_d) || !isset($b_y) || !isset($email1) || !isset($email2) || !isset($pass1) || !isset($pass2)) { $errorMsg = 'ERROR: You did not submit the following required information:<br /><br />'; if(!isset($username)){ $errorMsg .= ' * User Name<br />'; } if(!isset($firstname)){ $errorMsg .= ' * First Name<br />'; } if(!isset($lastname)){ $errorMsg .= ' * Last Name<br />'; } if(!isset($address)){ $errorMsg .= ' * Address<br />'; } if(!isset($postcode)){ $errorMsg .= ' * postcode<br />'; } if(!isset($town)){ $errorMsg .= ' * town<br />'; } if(!isset($b_m)){ $errorMsg .= ' * Birth Month<br />'; } if(!isset($b_d)){ $errorMsg .= ' * Birth Day<br />'; } if(!isset($b_y)){ $errorMsg .= ' * Birth year<br />'; } if(!isset($email1)){ $errorMsg .= ' * Email Address<br />'; } if(!isset($email2)){ $errorMsg .= ' * Confirm Email Address<br />'; } if(!isset($pass1)){ $errorMsg .= ' * Login Password<br />'; } if(!isset($pass2)){ $errorMsg .= ' * Confirm Login Password<br />'; } if ($email1!= $email2){ $errorMsg.='ERROR: Your email fields below do not match<br />'; } if ($pass1!= $pass2){ $errorMsg.='ERROR: Your password fields below do not match<br />'; } if(strlen($username)<6){ $errorMsg.="<u>ERROR:</u><br/>Your User Name is too short. 6-20 characters please. <br/>"; } if(strlen($username)>20){ $errorMsg.="<u>ERROR:</u><br/>Your User Name is too long. 6-20 characters please. <br/>"; } if($username_check>0){ $errorMsg.="<u>ERROR:</u><br/> Your User Name is already in use inside of our system. Please try another.<br/>"; } if($email_check >0){ $errorMsg.="<u>ERROR:</u><br/>Your Email address is already in use inside of our system. Please use another.<br/>"; } } else{ mysql_query("INSERT INTO member (username, firstname, lastname, email, password, dateofbirth, phone, lastlogin) VALUES('$username','$firstname','$lastname','$email1','$password', '$dateofbirth','$phone', now())") or die (mysql_error()); $sql = mysql_query("INSERT INTO address (address, postcode, town, housenumber) VALUES('$adress','$postcode,'$town','$housenumber'") or die (mysql_error()); mysql_close(); Echo "Welcome to my site, $username! You may now <a href=\"index.php\">login</a>."; } ?>
-
Hi James is there a way of contacting you directly?
-
Your right there im only a basic programmer! Can you go through my code if you dont mind and show me all the errors you can find? Regards Alex
-
The database connection is provided but im not sure if it is the right area? The user signs up there information on a form! Can you please re write my script?
-
sorry im not to sure what you mean? how do you change it to on? Thanks for the reply
-
At the moment there is no error message! When I select the sign up page nothing appears apart from a blank page? Regards Alex
-
Hi Im having an issue with it as it seems none of it works! I havent worked with more then one table before so im not sure how you input data into multiple tables that contain foreign keys. Can you please check by code and re write any issues that you may find! Regards
-
Hi im trying to create a sign up page for my website that contains different paths dependin on the membership that you select. I am an unexperienced programmer and need help as nothing is working at the moment. I would appreciate if people could reply to this post as soon as possible as I need it sorted today! Below is my code! Can you please send me suggestive improvement? Thanks <?php if (isset ($_POST['firstname'])){ //grab data from the form $username = preg_replace('#[^A-Za-z0-9]#i', '', $_POST['username']); // filter everything but letters and numbers $firstname = preg_replace('#[^A-Za-z]#i', '', $_POST['firstname']); // filter everything but Letters $lastname = preg_replace('#[^A-Za-z]#i', '', $_POST['lastname']); // filter everything but Letters $phone = preg_replace('#[^0-9]#i', '', $_POST['phone']); // filter everything but numbers $address= preg_replace('#[^A-Za-z]#i', '', $_POST['address']); // filter everything but Letters $postcode= preg_replace('#[^A-Za-z]#i', '', $_POST['postcode']); // filter everything but Letters $town= preg_replace('#[^A-Za-z]#i', '', $_POST['town']); // filter everything but Letters $housenumber= preg_replace('#[^0-9]#i', '', $_POST['housenumber']); // filter everything but numbers $b_m = preg_replace('#[^0-9]#i', '', $_POST['birth_month']); // filter everything but numbers $b_d = preg_replace('#[^0-9]#i', '', $_POST['birth_day']); // filter everything but numbers $b_y = preg_replace('#[^0-9]#i', '', $_POST['birth_year']); // filter everything but numbers $email1 = $_POST['email1']; $email2 = $_POST['email2']; $pass1 = $_POST['pass1']; $pass2 = $_POST['pass2']; $email1 = stripslashes($email1); $pass1 = stripslashes($pass1); $email2 = stripslashes($email2); $pass2 = stripslashes($pass2); $email1 = strip_tags($email1); $pass1 = strip_tags($pass1); $email2 = strip_tags($email2); $pass2 = strip_tags($pass2); //connect to db $connection = mysql_connect('linuxproj.ecs.soton.ac.uk', 'db_alw3g08', 'pasta'); $db = mysql_select_db('db_alw3g08', $connection); $emailCHecker = mysql_real_escape_string($email1); $emailCHecker = str_replace("`", "", $emailCHecker); // Database duplicate username check setup for use below in the error handling if else conditionals $sql_uname_check = mysql_query("SELECT username FROM Members WHERE username='$username'"); $uname_check = mysql_num_rows($sql_uname_check); // Database duplicate e-mail check setup for use below in the error handling if else conditionals $sql_email_check = mysql_query("SELECT email FROM Members WHERE email='$emailCHecker'"); $email_check = mysql_num_rows($sql_email_check); // Error handling for missing data if ((!$username) || (!$firstname) || (!$lastname) || (!$address) || (!$postcode) || (!$town) || (!$b_m) || (!$b_d) || (!$b_y) || (!$email1) || (!$email2) || (!$pass1) || (!$pass2)) { $errorMsg = 'ERROR: You did not submit the following required information:<br /><br />'; if(!$username){ $errorMsg .= ' * User Name<br />'; } if(!$firstname){ $errorMsg .= ' * First Name<br />'; } if(!$lastname){ $errorMsg .= ' * Last Name<br />'; } if(!$address){ $errorMsg .= ' * Address<br />'; } if(!$postcode){ $errorMsg .= ' * postcode<br />'; } if(!$town){ $errorMsg .= ' * town<br />'; } if(!$b_m){ $errorMsg .= ' * Birth Month<br />'; } if(!$b_d){ $errorMsg .= ' * Birth Day<br />'; } if(!$b_y){ $errorMsg .= ' * Birth year<br />'; } if(!$email1){ $errorMsg .= ' * Email Address<br />'; } if(!$email2){ $errorMsg .= ' * Confirm Email Address<br />'; } if(!$pass1){ $errorMsg .= ' * Login Password<br />'; } if(!$pass2){ $errorMsg .= ' * Confirm Login Password<br />'; } } else if ($email1 != $email2) { $errorMsg = 'ERROR: Your Email fields below do not match<br />'; } else if ($pass1 != $pass2) { $errorMsg = 'ERROR: Your Password fields below do not match<br />'; } else if (strlen($username) < 6) { $errorMsg = "<u>ERROR:</u><br />Your User Name is too short. 6 - 20 characters please.<br />"; } else if (strlen($username) > 20) { $errorMsg = "<u>ERROR:</u><br />Your User Name is too long. 6 - 20 characters please.<br />"; } else if ($uname_check > 0){ $errorMsg = "<u>ERROR:</u><br />Your User Name is already in use inside of our system. Please try another.<br />"; } else if ($email_check > 0){ $errorMsg = "<u>ERROR:</u><br />Your Email address is already in use inside of our system. Please use another.<br />"; } else { // Error handling is ended, process the data and add member to database $email1 = mysql_real_escape_string($email1); $pass1 = mysql_real_escape_string($pass1); // Add MD5 Hash to the password variable $password = md5($pass1); // Convert Birthday to a DATE field type format(YYYY-MM-DD) out of the month, day, and year supplied $dateofbirth = "$b_y-$b_m-$b_d"; // Add user info into the database table for the main site table $sql = mysql_query("INSERT INTO members (username, firstname, lastname, email, password, dateofbirth, phone, lastlogin) VALUES('$username','$firstname','$lastname','$email1','$password', '$dateofbirth','$phone', now())") or die (mysql_error()); $sql = mysql_query("INSERT INTO address (address, postcode, town, housenumber) VALUES('$adress','$postcode,'$town','$housenumber'") or die (mysql_error()); $id = mysql_insert_id() } else { // if the form is not posted with variables, place default empty variables so no warnings or errors show $errorMsg = ""; $username = ""; $firstname = ""; $lastname = ""; $phone = ""; $address = ""; $postcode = ""; $town = ""; $housenumber = ""; $b_m = ""; $b_d = ""; $b_y = ""; $email1 = ""; $email2 = ""; $pass1 = ""; $pass2 = ""; }