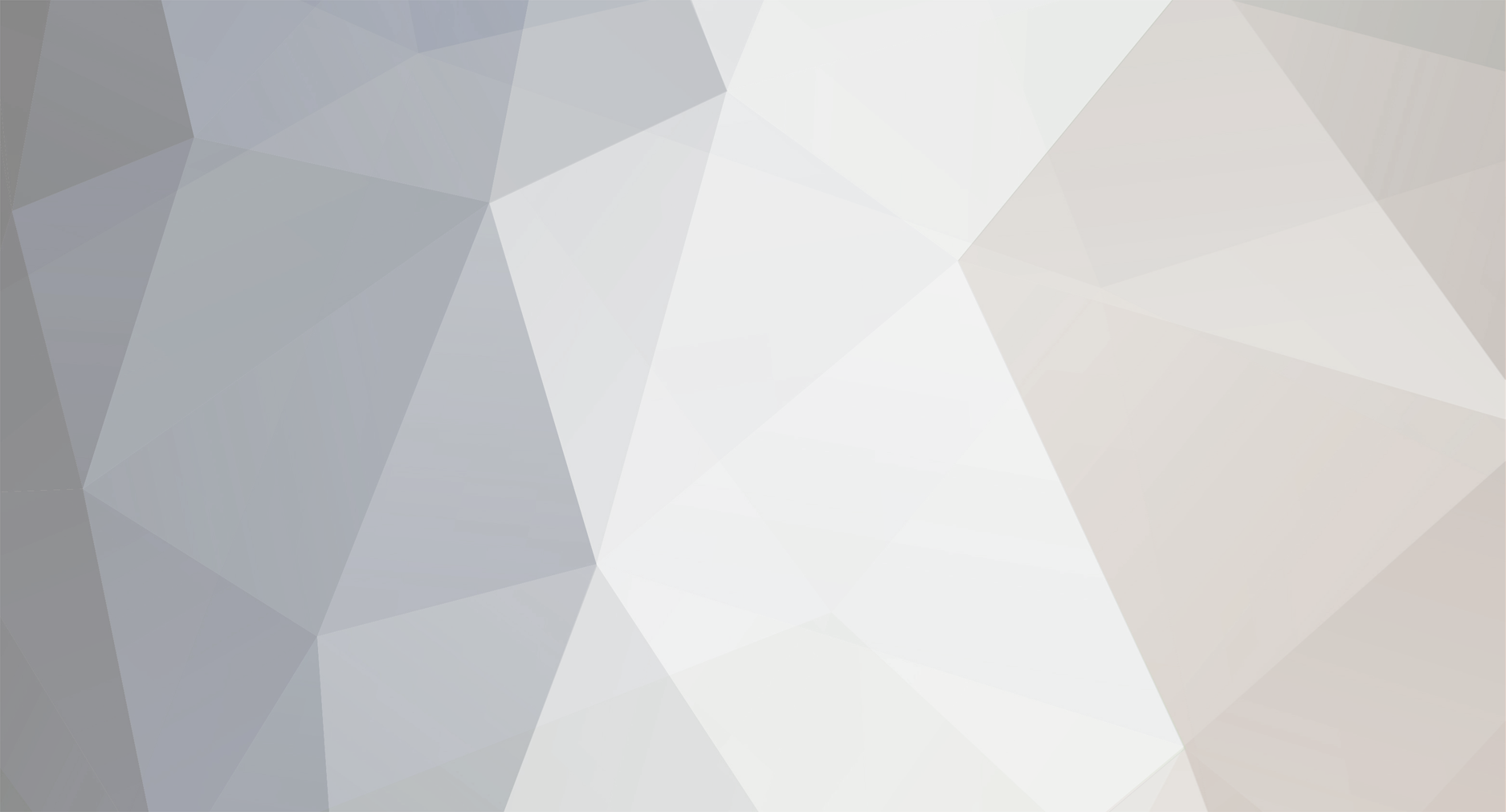
Bl4ckMaj1k
-
Posts
93 -
Joined
-
Last visited
Never
Posts posted by Bl4ckMaj1k
-
-
So... you want an 'add tool' button.
The user can pick from existing tools, then names of which are stored in a db.
or/
The user can enter their own name of the tool?
Wow you summed that up a lot better than I did. In a word, yes, that's what I am looking to create. Can you point me in the right direction? Is this possible?
-
Good afternoon fellas!! Got another JS question for ya...let me know if I am making any sense :-)
So I have the following form elements
<div id="tool1" class="check box"> <input name="form_checkboxes[]" type="checkbox" value="Some_Tool" /><br /> </div> <div id="tool1_label" class="form_label"> Some Tool Name </div> <div id="tool_quantity" class="form_quantity"> Quantity </div> <div id="tool_textfield" class="form_quantity_textfield"> <input name="quantity[some_Tool]" type="text" size="3" /> </div><br />
On the screen, this form would look similar to the following
o Some Tool Name Quantity ___Assume that the circle in the beginning is a check box and the underscore after 'Quantity' is a text field.
I have some static tools on the form but I want the user to be able to add as many additional fields as they need to. In other words, I want some fields marked 'Other' or something like that. So the form may look something like this.
o Hammer Quantity ___o Wrench Quantity ___
o Drill Quantity ___
o Screw Driver Quantity ___
Then when the user has filled out the on screen fields, there should be a button at the bottom marked 'add tool' or something like that. Upon clicking that button, the form will change to the following (for this example, lets assume the button was clicked 3 times)
o Hammer Quantity ___o Wrench Quantity ___
o Drill Quantity ___
o Screw Driver Quantity ___
o Other 1 Quantity ___
o Other 2 Quantity ___
0 Other 3 Quantity ___
As you can say, clicking three times created 3 additional fields to the original form. The user should be able to type in a specific name for his/her tool though. So, instead of saying 'Other 1', 'Other 2', or 'Other 3', it would read whatever the name of his added tool is. Is this something I need to do in Javascript or PHP? I can't figure any PHP for this process and I am complete idiot when it comes to JS lol. Any help would be greatly appreciated as I can honestly say I wouldn't even know how to Google this LOL.
Also, I am writing this stuff to a database so I need some way to keep these fields unique. I figure there is some type of JS function that will allow the 'Value' for my inputs to be whatever the user types in and that will create my unique ID. I can run a check of some sort that says if those 2 fields are equal then an alert pops us saying something like 'hey, those have to all be unique dude'...!!!
So, what do you all think? Am I out of my mind to even think this is possible or should I get punched in the face for not knowing how to do this in my sleep??
-
Wow I didn't know you replied. You REALLY should consider being a teacher, you explanations make more sense than anything I have read on any forum to date. Thanks again bro, your method really just helped me with a large percentage of my project.
SOLVED.
-
Thank you all so much for the information!!!!! This can be marked as SOLVED!!!! Another one bites the dust :-)
-
P.S. I just realized you want to tell the code to either edit or delete a group....I would handle this on a different page. Ideally you will want your users to be able to delete from blah.php and edit from somewhereelse.php
Regarding editing, what exactly are the users editing? The user data? Some name and email data? Depending on what you are editing, I can assist with a script that will allow you to edit multiple rows of user information in one form.
-
While loops are always fun, but for what you wish to achieve, you want to look at another member in the loop family. The 'foreach' loop. This will allow for each individual id in your array to be stored in a single variable. See below example
while($rows=mysql_fetch_array($result)){
$theId = $row['id'];
$theFname = $row['name'];
$theLname = $row['lastname'];
$theEmail = $row['email'];
$deleteThese .= '<input name="contacts[]" type="checkbox" value="' . $theId . '" /> ' . $theFname . ' ' . $theLname . '<br />' . $theEmail . '<br /><br />';
}
Now we want to give a rule for our checkbox function. We want to capture whichever checkbox was ticked, and do something to that particular piece of data in the database. We know which piece of data in the database we will be working with because of our variable $theID declared in each input checkbox. See below how we make the foreach function work for us here
if(isset($_POST['delete_contacts']) && !empty($_POST['contacts'])) {
I always like to run the above if statement to check whether or not our submit button was pressed, and to ensure that there was actually some piece of information checked on our form before we move forward.
foreach($_POST['contacts'] as $allchecked) {
Now we do a foreach function. This will grab the value of each checked box on our form. Remember, the value of each checkbox is the current ID that we are working with. This is how we will tell our database what and where to delete.
$query = "SELECT id FROM your_table_name WHERE id='$allchecked";
$sql = mysql_query($query) or die (mysql_error());
Here I like to do one more check to make sure our database found someone with the same ID as the checkbox value.
$existCount = mysql_num_rows($sql);
if($existCount > 0) {
After our count of the rows, I do the final query to the database, which is find the contact in the database, and delete that person from the database.
$lastQuery = "DELETE FROM your_table_name WHERE id='$allchecked';
$sql2 = mysql_query($lastQuery);
Overall, the code will be the following:
while($rows=mysql_fetch_array($result)){
$theId = $row['id'];
$theFname = $row['name'];
$theLname = $row['lastname'];
$theEmail = $row['email'];
$deleteThese .= '<input name="contacts[]" type="checkbox" value="' . $theId . '" /> ' . $theFname . ' ' . $theLname . '<br />' . $theEmail . '<br /><br />';
}
if(isset($_POST['delete_contacts']) && !empty($_POST['contacts'])) {
foreach($_POST['contacts'] as $allchecked) {
$query = "SELECT id FROM your_table_name WHERE id='$allchecked";
$sql = mysql_query($query) or die (mysql_error());
$existCount = mysql_num_rows($sql);
if($existCount > 0) {
$lastQuery = "DELETE FROM your_table_name WHERE id='$allchecked';
$sql2 = mysql_query($lastQuery);
}
}
}
echo 'anything you want here and be sure to exit when you are done.';
exit();
The above is the simple PHP. Wherever you wish to display the list of options that will be deleted, echo this function in the HTML. See below
<?php echo "$deleteThese"; ?>
This will give you your list of checkboxes and names with emails listed below. Also, don't forget to add your form and button somewhere on the html.
<form action="some_page.php" method="post" enctype="multipart/form-data">
<input type="submit" name="delete_contacts" value"Delete Contacts" /> (NOTE: we establish the 'name' of this button here and it has to be the same name in the php where you are checking to see if this button was clicked)
</form>
And you are done. The code you are using is not necessary as far as I know. The above is all you need to capture the data from your database and remove it if its been checked. Hope this works, good luck!!
-
preg functions need pattern delimiters:
$customer_contact_id = preg_replace("/`/", "", $customer_contact_id);
However, if you don't need regex, this is easier and faster:
$customer_contact_id = str_replace("`", "", $customer_contact_id);
Worked just fine, thanks!!
The video told you to do that because eregi has been deprecated since 5.3.
Hmm....why do they deprecate functions anyway? eregi_replace still got the job done...what made someone make another function which does the exact same thing?
-
$customer_contact_id = preg_replace("`", "", $customer_contact_id);
Am I doing something wrong? Every page that used the eregi_function, I changed to have it utilize the preg_function (because I saw a Youtube video telling me to do so). Now, every variable I perform the above on acts as if it doesn't exist. This has totally destroyed my system. What do I need to do here to fix things?
-
You ever consider being a teacher??? That explanation was spot on. I understand it in its entirety. Thanks for taking the time to explain.
The query you are running has implode() which you state handles inserting multiple records into the database. Lets assume I want to record the 5 pieces of data into that field. 2 of which are captured on the form, and 3 that we define in our PHP. For this example I will say:
$variable1 = 1;
$variable2 = 2;
$variable3 = 3;
How would we also add this information to our database?
Also, I notice you have the following
implode('', '', $variable)
What is the purpose of the 2 null values?
Once again thanks for the previous explanation.
-
Wow this just blew my mind. I think I understand whats going on. I am going to make an attempt at explaining and you just correct me if I am confused anywhere...
So first, you establish the radio button, or checkbox...whichever I decide to use. The name has the array [] brackets meaning that whenever thats checked, the value of that will be recorded. The value in this case being hammer ID. Next you work out the input for the texfield. This is where I am kind of confused (please see below questions). I believe you need a reference point so your code knows what quantity to associate that tool with??
Next, you bust out my favorite part, the PHP. You establish that the variable queryValues is an array....understood. Then you crank out a foreach loop which will capture everything thats been stored in our tools array (which is all the tools we have selected) and store them in the variable itemID. Now this is really where the confusion returns for me. The line '$quantity = (int) $_POST['quantity'][$itemID];' is one I have never seen. Doing research I understand its some sort of count function, but I can't honestly say I get how it works. Then you run an if statement which basically says, if there is any time the itemID belongs to the quantity field that we are capturing && that there was actually some data in it, then do this next part?? Is that correct? Anywho, after that if statement if verified as 'yes, its greater than 0', you do another line of code that, once again, I don't understand how it works....quite frankly, here is where it gets completely dark for me. I don't understand what any of the remaining code is doing.
So there you have it...my version of what's going on is probably no where near correct. Below I have a couple direct questions along with the questions I have asked in the above recap of events. I know this is asking a lot, but it would be amazing (if you wouldn't mind) if you could answer these questions/explain this stuff to me.
1.) Can I just simply change the tool ID (ex. hammerID) to just the name of the tool (ex. hammer) ??
2.) What is the point of the array function in the textfield input? You call the value of the checkbox hammerID and then call the name inside the array hammerID. I don't think I understand this part, you mind explaining?
-
Oops!!! Correction to my post. I am not using radio buttons. I am actually using checkboxes!!! I am so sorry...does this change your suggested code?
-
I have a form which has both radio buttons and textfields. Each individual radio button is associated with a form field. For this example, lets say the Radio button references a tool name and the form field represents a quantity, or number of tools needed for a certain job. So the form would look as follows:
o Some Tool Quantity ___ (<-- lets pretend thats a form field)The question is, how would I go about adding the tool and quantity to the same row in the database if I am creating a new row? The simple way would be have each radio button and quantity be its own entity in the HTML. For example:
<input name="tool1" type="radio" value="Hammer" /> <input name="tool_quantity1" type="text" /> <input name="tool2" type="radio" value="Wrench" /> <input name="tool_quantity2" type="text" />
However easy this method may be, and however functional it may work, it is not how I wish to achieve this because we are talking maybe 100 tools. I think it would be easier using some kind of loop function that goes through an array of anything that has some form of data stored in it. For example, instead of the code being the above, I would like to change it to the following:
<input name="tools[]" type="radio" value="Hammer" /> <input name="tools_quantity[]" type="text" />
I understand the basic foreach function
foreach ($_POST['tools'] as $someVariableName) { //do some stuff }
But I don't understand how I can get the value of 2 different form fields and have them associated with one another. I want the tool and the quantity of tools to be stored in the same row. How is this done? Is this even possible using a loop function like foreach or while? Please help! As always, any help would be greatly appreciated!!
Bl4ck Maj1k
-
Darnnnn!!!! I figured someone would say the answer I was dreading the whole time. And I know you are right, this would actually be much easier if I just checked the assigned project with some record in the database and ensure that the $_GET['pid'] was equal to whatever is in the database. The only question is, would it be a good idea to query a table in the database every time a new page is loaded? That's ultimately what we are saying. I have a table that I have my employees related to all the projects. There is a field for project ID and a field for employee ID. So what I would do is say the following (note this is a question)
$current_proj_id = $_GET['pid]; $current_employee_id = $_SESSION['eid']; $query = "SELECT project_id FROM someTableWithEmps&Projs WHERE proj_id='$current_proj_id' AND employee_id = '$current_employee_id' "; $sql = mysql_query($query) or die (mysql_error()); $row_count = mysql_num_rows($sql); if ($row_count <='0') { //some error }
-
I still don't fully understand why you would want to do this, but what you are saying is that you don't want anyone to access information unless you specifically provide them with an HTML link to the information. That's not security. The only way that comes to mind is adding the allowable values to the session and checking on the next page. Assuming you are getting these from a database or somewhere in an array and outputing links:
//page1.php $_SESSION['cids'] = array(); foreach($rows as $row) { $_SESSION['cids'][] = $row['cid']; echo '<a href="page2.php?cid=' . $row['cid'] . '">click</a><br>'; }
//page2.php if(!in_array($_GET['cid'], $_SESSION['cids'])) { //error } $_SESSION['cids'] = array(); //let em get the information
This code is awesome....I will say that first. Didn't even know it was possible.
Anyway, this is not what I am saying I want to do. Lets take an example directly from my system and you will understand the importance of URL security for me. Lets take the example that there are 3 employees in the system.
Employee 1Employee 2
Employee 3
There are 2 Companies in the system
Company 1Company 2
Companies are assigned employees. We will distribute our employees like so:
Company 1Employee 1 and Employee 3
Company 2
Employee 2
Now each company is assigned several different projects. Lets create a couple projects and give them all IDs. We will say IDs are generated based on ID of project created in the database and company it belongs to. (Example, if we are dealing with company 2 project 6, the ID would be 2-6. This way each project ID remains unique.)
Company 1Project 1 - PID=1-1
Project 2 - PID=1-2
Project 3 - PID=1-3
Company 2
Project 4 - PID=2-4
Project 5 - PID=2-5
Now we have the employees that each company is associated with. Once they have been associated with a company, we can then associate them with a project within that company. So lets do that
Employee 1Project 1 and 3
Employee 2
Project 5
Employee 3
Project 2
Now we have all the information we need to run our example of the security I need. Following my data above, lets assume I am employee 1. This means I belong to company 1 and I should only be able to view projects 1 and 3. So when I click my URL, I will get the following information using the $_GET function in the PHP of the code and store those variables into local variables. My link will look like this
proj_prof.php?eid=1&cid=1&pid=1-1Now this is captured by me clicking on a link in the previous page, whatever that may be. Somewhere on that page I do a $_GET['eid'], $_GET['cid'], and lastly a $_GET['pid']. I store those in local variables. So if I link to the following page and do some sql statement, I make sure results are only associated with that project, that employee, that company. Now I have stored the employee ID and company ID in $_SESSION and transferred them to local PHP variables as those will never change. An employee will always, as long as he/she is logged into his/her account, will belong to the same company, have the same user ID. On the other hand, projects are created on the fly. Employees are assigned new projects randomly. They won't know when they are to work on a new project until the project is assigned to them. With that being said, here is what I am afraid of.
Employee 1 and 3 work for the same company. However, Employee 3 has access to a project that Employee 1 does not. Project 2. Now if I am employee 1, and I know anything about PHP, I can simply go to any project that I do have access to. Then, in the URL, I can simply change the value of pid from 1-3 to 1-2. This will direct me to all the information being pulled thats associated with the project ID of 1-2, even though I don't have access. This is where I need to say "Hey A****LE!!!! Get out of that URL!!!!!".
-
What makes you think that using $_GET is insecure? I can manipulate $_POST as much as I could with $_GET even if you add SSL. Your real core problem is that you should sanitize and validate your data before using it.
Sanitize and Validate??? Hmm maybe I am already doing this. What does this consist of? I Google'd but I am guessing you are using some PHP slang. do you mind explaining?
Also, I agree that $_GET and $_POST are both just as secure or just as insecure as one another....that's not what I am referring to. I am referring to the fact that I have my system set up in such a way where I am storing variable values in my URL. Unfortunately what this does is allows other people to see those variables as well. I just need a way to hide this from the public eye. If I can't hide this, I just need a way to say, "Hey!!! Don't edit the URL A****LE!!!!". I'm sure you get my drift.
Bl4ck Maj1k
-
Please define your meaning of "retreive a stored $_POST variable"
$_POST variable is the same as a normal PHP variable.
SSL: http://www.flatmtn.com/article/setting-ssl-certificates-apache
Thanks for the links to tuts guys! I have them bookmarked and already started trying a few things here and there. Mod_rewrite is ridiculous!!!
As for the 'retreive a stored $_POST variable', what I mean is this...
When something is under the $_POST array, how can you pull that from a URL? For example, if I have a variable of id in the URL, can I say the following:
$user_id = $_POST['id'];
That's basically what I mean. I thought this could only be achieved through the $_GET method.
-
OK I am not that advanced....kinda newb. Do you guys mind giving an explanation? I understand $_POST but how would I retreive a stored $_POST variable? And how would the SSL work?? Also confused about the mod_rewrite function. If you can give a few examples that would be great!!!! Thanks again.
Bl4ck Maj1k
-
Hi all. I have a question and don't know if it belongs in the PHP section or the Javascript section so I apologize in advance if I am in the wrong place. Basically what I am trying to do is change a URL with the following variables
index.php?id=16&?pid=23-P27-16&cid=1324353245
to something more secure....like
index.php
I think doing it this way would add an extra layer of security to my website. If this is not possible, is there a way I can track whether or not a user attempted to edit something in the URL. For example, if the cid=13243543 and they go in a change it to 13243654 instead, can I have PHP check that? I have a great deal of security already, but I think this would truly be the highest layer, completely controlling what happens in the user's address bar.
As always, thanks in advance!
Bl4ck Maj1k
-
You guys are nothing short of GENIUSES!!!!!! Man I love this place. Thanks again boyz and girlz....another one for the books! SOLVED!!!!
-
Got it, works perfectly...thanks!!! One question. Do you mind explaining the following line to me. I can honestly say I have never seen this done before:
$current_staff_id = (isset($_POST['staff_id'])) ? $_POST['staff_id'] : NULL;
I understand you are saying that the current_staff_id variable will be equal to whatever variable is stored in the hidden div. But what is the ? $_POST['staff_id'] : NULL; ?? Can you explain this line?
-
I don't mean to nag but can someone please help me with this issue? I really can't seem to understand storing each of these IDs as a separate entity. I keep pulling the last ID that was stored in the database. I think my new confusion is how to work the hidden form function so that would grab the variables for me and separate each form or ID.
-
Where exactly in the code are you saying I need to place the form and the hidden form? Sorry but I just don't understand where exactly in the code you are saying the changes need to be made. Can you give me an example?
-
EDIT: Just tested and it still doesnt work. Unfortunately, i believe I will need to do some type of foreach statement. I say unfortunately because I have no idea how that works. Anyone wanna help me out in changing this code in such a way that will deliver me each individual user id depending on which div I have selected?
Hmmm....I don't know how I would go about doing that...would I just place the form tags in the PHP function that calls all the divs? I thought about doing this but I still don't understand how that would allow me to separate the individual User IDs. In any case, I will test this and let you know if it works. Thanks.
-
Good afternoon all!! I have a very specific issue that, in my opinion, is quite complicated. In words, here is what I wish to achieve. I would like a page which displays users from the database. Each individual user has their own Div hidden below their name. Within this div, there is a form. The form is full of radio buttons. Once the user's name is clicked and form submitted, I wish to write the form data to the database for the specific user that was selected. See below example for better understanding:
Dale Gibbs
Chris Hansen
Steve Jobs
If I click Chris Hansen, the following happens
Dale Gibbs
==============
Chris Hansen
HIDDEN DIV FORM CONTENT
HIDDEN DIV FORM CONTENT
HIDDEN DIV FORM CONTENT
HIDDEN DIV FORM CONTENT
HIDDEN DIV FORM CONTENT
Submit Button
==============
Steve Jobbs
So as of now, the display is correct. I am seeing exactly what I want to see from my database. Also, my div IDs work just fine as well as the Javascript toggleSlidebox function. The only issue is for some reason, whenever I submit the form (no matter which user I select from my list), I can only write to the last inputted ID. So for example, if the last ID entered into the database was 6, then thats the only ID that will be returned when my form is submitted and the only place data will be written to, even if I select a user with ID 2. Please see below code for more information
<?php $staff_display_query = "SELECT staff_info.id, staff_info.fname, staff_info.lname FROM staff_info, staff_projects WHERE staff_info.id = staff_projects.staff_id AND staff_projects.proj_id = '$c_project_id'"; $staff_display_sql = mysql_query($staff_display_query) or die (mysql_error()); while ($row = mysql_fetch_array($staff_display_sql)) { $current_staff_id = $row['id']; $staff_fname = $row['fname']; $staff_lname = $row['lname']; $list_staff .= ' ' . $current_staff_id . '<br /> <a href="#" onclick="return false" onmousedown="javascript:toggleSlideBox(' . $current_staff_id . ');">' . $staff_fname . ' ' . $staff_lname . '</a> <div class="hiddenDiv" id="' . $current_staff_id . '" style="border:#FFF 2px solid; width:553px;"> <!--TASK 1--> <div id="task_1_permissions" class="task_permissions"> <input name="permissions_1" type="radio" value="1"/> <input name="permissions_1" type="radio" value="2" /> <input name="permissions_1" type="radio" value="3" /> <input name="permissions_1" type="radio" value="0" /> </div> <!--TASK 2--> <div id="task_2_permissions" class="task_permissions"> <input name="permissions_2" type="radio" value="1"/> <input name="permissions_2" type="radio" value="2" /> <input name="permissions_2" type="radio" value="3" /> <input name="permissions_2" type="radio" value="0" /> </div> <!--TASK 3--> <div id="task_3_permissions" class="task_permissions"> <input name="permissions_3" type="radio" value="1"/> <input name="permissions_3" type="radio" value="2" /> <input name="permissions_3" type="radio" value="3" /> <input name="permissions_3" type="radio" value="0" /> </div> <!--TASK 4--> <div id="task_4_permissions" class="task_permissions"> <input name="permissions_4" type="radio" value="1"/> <input name="permissions_4" type="radio" value="2" /> <input name="permissions_4" type="radio" value="3" /> <input name="permissions_4" type="radio" value="0" /> </div> <input name="submit_user_permissions" type="submit" value="Submit Permissions" /> </div> </div> <br /><br /> '; } if (isset($_POST['submit_user_permissions'])) { $permissions_1 = $_POST['permissions_1']; $permissions_2 = $_POST['permissions_2']; $permissions_3 = $_POST['permissions_3']; $permissions_4 = $_POST['permissions_4']; $query = "UPDATE staff_projects SET task_1='$permissions_1', task_2='$permissions_2', task_3='$permissions_3', task_4='$permissions_4' WHERE proj_id='$c_project_id' AND staff_id='$current_staff_id'"; $sql = mysql_query($query) or die (mysql_error()); echo 'Permissions set successfully.<br />'; }
After this PHP I have my standard HTML. Below is the javascript function I use for the slide box:
<script src="js/jquery-1.5.js" type="text/javascript"></script> <script language="javascript" type="text/javascript"> function toggleSlideBox(x) { if ($('#'+x).is(":hidden")) { $(".hiddenDiv").slideUp(200); $('#'+x).slideDown(300); } else { $('#'+x).slideUp(300); } } </script>
Javascript works just fine by the way. Below is the form I have in the HTML of the code.
<form action="" method="post" enctype="multipart/form-data"> <?php echo "$list_staff"; ?> </form>
This is of course wrapped around body tags and all the other necessary HTML. The view of the form is working right, the functions within the form are also working correctly. I just cant seem to separate the variables for each individual user. If I am in Chris Hansen's div, it should be his ID number that is being referenced for the database, not the ID number of the last person entered into the system. Any ideas? As always, thanks in advance guys!!!
Bl4ck Maj1k
Total n00b trying to build website. Plz help :(
in PHP Coding Help
Posted
http://www.developphp.com/list_php_video.php
The videos on that website made me the PHP semi-ok dude that I am today. I can now bang out exactly what you are asking for in about 2-3 hours...if even that long.
You are creating 3 pages, 2 tables, and a directory for each individual user. I would make one index page which displays every image in the system, another page for user specific images, and a final page for a users profile. Tables would be 1 for contact/profile information and another to hold the data for the uploaded art.
Once you go through the site I just posted and review the videos, you should be good to go. The guy teaching the stuff is extremely thorough and assumes anyone looking at the videos are EXTREMELY dumb lol. At times you will find yourself sighing at how lower-level he takes some of his explanations, but other times you will appreciate his sense of depth. Good luck!!
P.S. I wish the project I am working on was something a bit more simple. For a first project, I think I went a bit too complex.