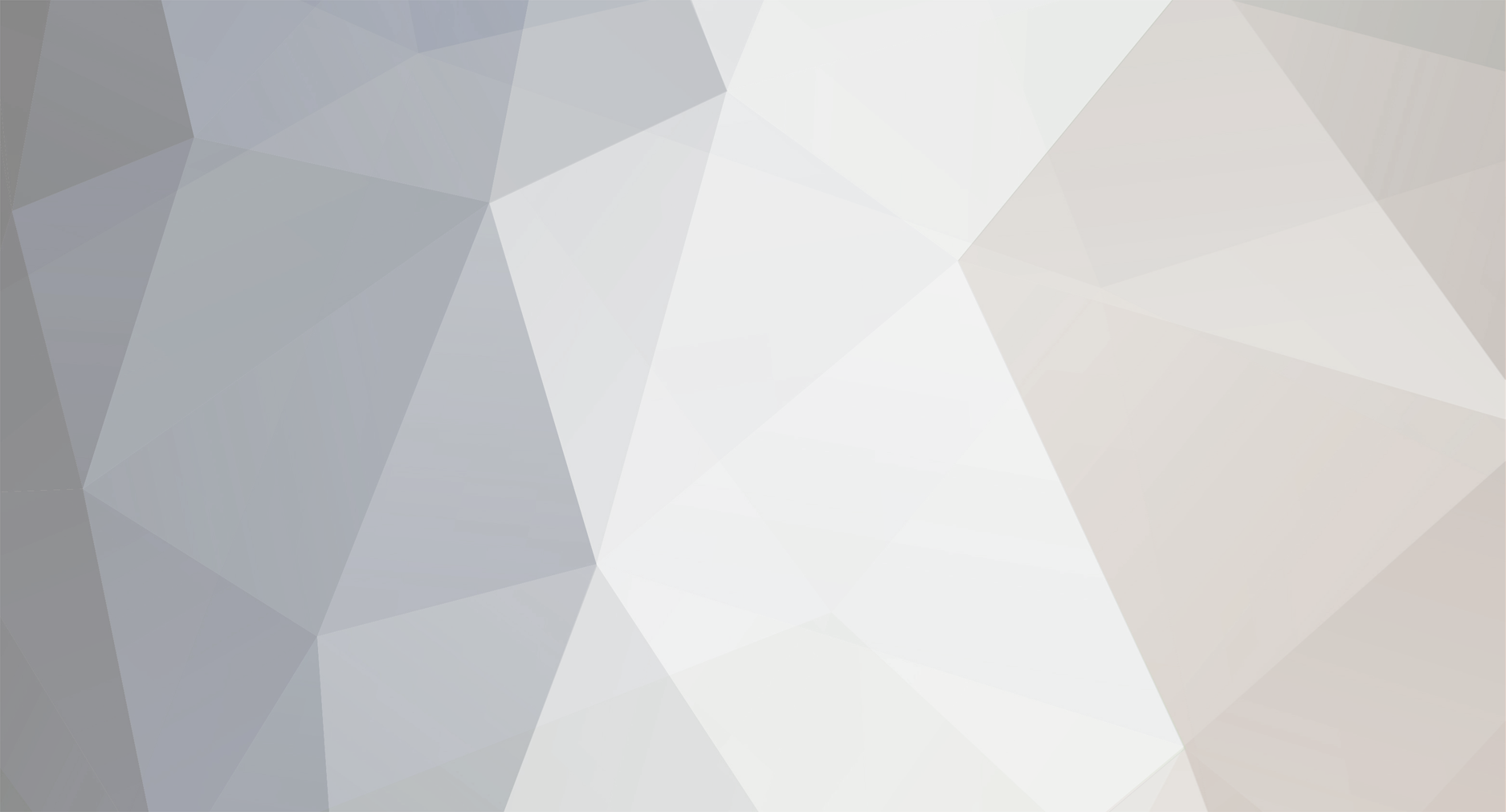
chris57828
Members-
Posts
14 -
Joined
-
Last visited
Everything posted by chris57828
-
Hi, This is a really easy one but for the life of me I cannot fathom it out. All I want to do is format numbers to have a decimal point in the right place. For I example I want the number 9550 to be formatted as 95.50 or 1250 as 12.50 or 10000 as 100.00 I search google using 'formatting to two decimal places' but in the case of the first example all I get returned is formatting numbers such as 9500 to 9500.00 which I don't want. Help would be greatly appreciated Regards, Chris.
-
Can someone help? I am new to PHP and trying to get some training in before I commence study in September. Below you will see the code for a very simple Lucky Lotto number generator, which generates six numbers at random from the $numbers array via the shuffle(). Being that I am a learner, in the most simple way possible I want to place the resulting 6 numbers in a new array, so I can use other functions to ascertain comparisons and results etc. <?php class Game { public $count; public $draw = array(1,15,16,27,30,18); public $numbers = array (1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20,21,22,23,24,25,26,27,28,29,30,31,32,33,34,35 ,36,37,38,39,10,41,42,43,44,45,46,47,48,49); function display($sub1) { $this->count = $sub1; while ($this->count>0) { shuffle($this->numbers); for($j=0;$j<6;$j++) echo $this->numbers[$j] . " "; echo "<br />"; shuffle($this->numbers); $this->count--; } } } $object1 = new Game(); $object1->display(1); ?>
-
Thanks wildteen88, I have replaced the 'for' loop with a 'for..each' loop, and it seems to be working, when I have finished I'll post the code
-
Hold on, I'll have a look! :'(
-
If that’s the case why does the code in my initial post not work, only one element is ever displayed?
-
Hi, yeah I did see your reply last time, sorry for not thanking you, only problem is your code is quite different from mine, and at present because I am learning I don’t want to simply cut and paste someone else’s code. Since I started this thread I have learnt that the explode() is for strings only and not numbers but there is a workaround. Do you know how I could explode whole numbers into an array, but for instance if the numbers are 1, 15, 25, 28, I want them placed in an array in that format, not splitting them up into single integers?
-
Hi, I am new to programming hence why I am unfamiliar with built in PHP functions and my code is rather unsophisticated, however, I am attempting to write a small ‘Lucky Lotto’ program which involves me predetermining the winning numbers manually via the array $draw. Via display() a user can decide how many ‘attempts’ they want. I want to be able to ascertain how many winning numbers each draw has (if any) so I have constructed a rather long IF function whereby the results are ‘exploded’ into an array namely $temp or $temp1 then via count() or sizeof() I should be able to determine the results. The problem I am having is that the arrays I am ‘exploding’ to namely $temp and $temp1 only ever appear to hold one element at position zero, which is obviously contrary to what arrays are for. By looking at the code below can someone show me in laymen’s terms how I can get $temp and $temp1 to hold and output more than one element, thanks! <?php class Game { public $temp; public $temp1; public $result; public $result1; public $draw = array(15,11,27,9,5,25); public $numbers = array(1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20,21,22,23,24,25,26,27,28,29,30, 31,32,33,34,35,36,37,38,39,40,41,42,43,44,45,46,47,48,49); public $age; public $count; function __construct($sub1, $sub2) { $this->name = $sub1; $this->age = $sub2; return $this->name;age; } function checkAge() { if ($this->age <18) { return "Sorry $this->name, you are too young to gamble"; } else { return "Hi $this->name welcome to Lucky Lotto Dip, how many draws would you like?"; } } function display($sub3) { shuffle ($this->numbers); $this->count = $sub3; echo "<pre>"; echo "*********************"; echo "<br />"; echo "* Lucky Lotto *"; echo "<br />"; echo "*********************"; while ($this->count >0) { echo "<br />"; echo "* "; for ($j =0; $j <6; $j++) // For loop if ($this->numbers[$j] <10) { echo " " . $this->numbers[$j] . " "; if ($this->numbers[$j] == $this->draw[0] or $this->numbers[$j] == $this->draw[1] or $this->numbers[$j] == $this->draw[2] or $this->numbers[$j] == $this->draw[3] or $this->numbers[$j] == $this->draw[4] or $this->numbers[$j] == $this->draw[5] ) { $temp = explode(' ', $this->numbers[$j]); for ($a =0; $a <$temp.length; $j++); } } else { echo $this->numbers[$j] . " "; if ($this->numbers[$j] == $this->draw[0] or $this->numbers[$j] == $this->draw[1] or $this->numbers[$j] == $this->draw[2] or $this->numbers[$j] == $this->draw[3] or $this->numbers[$j] == $this->draw[4] or $this->numbers[$j] == $this->draw[5] ) { $temp1 = explode(' ', $this->numbers[$j]); for ($a =0; $a <$temp1.length; $j++); } } echo "* "; echo "<br />"; echo "<br />"; echo $temp[$a]; echo "<br />"; echo $temp1[$a]; echo "<br />"; echo "*********************"; echo "<br />"; echo " "; echo "<br />"; echo "*********************"; $this->count --; shuffle ($this->numbers); $temp1= 0; $temp = 0; } } } $object2 = new Game("Chris", 36); echo $object2->checkAge(); echo $object2->display(3); ?>
-
Hi everyone, this is quite a big one, and I am new to programming. In the code below you will see I have created a 'Lucky Lotto Dip'. The display function allows the user to choose how many draws they want then by way of the shuffle() 6 numbers are generated randomly for each line. I have two questions. (1) I have managed by way of an if statement to automatically check the first number in the array $draw, how do I get it to check for 2 match's, 3 match's and so on up to 6 match's. And secondly if the first number is matched I have managed to get it to repeat itself and if to show a match, but how do I get it to repeat itself on the line below the selected numbers? I know this is difficult but if anyone can help I will be very grateful! <?php class Game { public $draw = array(10,25,36,3,12,13); public $numbers = array(1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20,21,22,23,24,25,26,27,28,29,30, 31,32,33,34,35,36,37,38,39,40,41,42,43,44,45,46,47,48,49); public $age; public $count; function __construct($sub1, $sub2) { $this->name = $sub1; $this->age = $sub2; return $this->name;age; } function checkAge() { if ($this->age <18) { return "Sorry $this->name, you are too young to gamble"; } else { return "Hi $this->name welcome to Lucky Lotto Dip, how many draws would you like?"; } } function display($sub3) { shuffle ($this->numbers); $this->count = $sub3; echo "<pre>"; echo "*********************"; echo "<br />"; echo "* Lucky Lotto *"; echo "<br />"; echo "*********************"; while ($this->count >0) { echo "<br />"; echo "* "; for ($j =0; $j <6; $j++) // For loop if ($this->numbers[$j] <10) { echo " " . $this->numbers[$j] . " "; if ($this->numbers[$j] == $this->draw[0]) { echo $this->numbers[$j] = $this->draw[0]; } } else { echo $this->numbers[$j] . " "; if ($this->numbers[$j] == $this->draw[0]) { echo $this->numbers[$j] = $this->draw[0]; } } echo "* "; echo "<br />"; echo "*********************"; echo "<br />"; echo " "; echo "<br />"; echo "*********************"; $this->count --; shuffle ($this->numbers); } } } $object2 = new Game("Chris", 36); echo $object2->checkAge(); echo $object2->display(4); ?>
-
Multidimensional Arrays & foreach....loop?
chris57828 replied to chris57828's topic in PHP Coding Help
Thanks babe. this forum is so cooooooooooooooool -
Hi everyone, If you take a look at my code below I am having trouble echoing the key/ value pair of the 'sex' array which is a sub array of 'pens'. I have tried so many different ways but to no avail, thanks Chris! <?php $products = array( 'paper' => array( 'copier' => "Copier & Multipurpose", 'inkjet' => "Inkjet Printer", 'laser' => "Laser Printer", 'photo' => "Photographic Paper"), 'pens' => array( 'ball' => "Ball Point", 'hilite' => "Highlighters", 'marker' => "Markers", 'sex' => array ( 'condom' => "Protection")), 'misc' => array( 'tape' => "Sticky Tape", 'glue' => "Adhesives", 'clips' => "Paperclips") ); echo "<pre>"; foreach ($products as $section => $items ) foreach ($items as $key => $value) echo "$section:\t$key\t($value)<br>"; echo "</pre>"; ?> :'(
-
I'm having trouble ascertaining the difference between 'echo' and 'return'?
-
Thanks babe..................it works! YIPPEE
-
Can someone tell me by looking at the following code why function pcust() does not work, I am new to programming? :'( $firstname = "chris"; $surname = "reynolds"; $address1 = "12 birch end"; $town = "Wallington"; $county = "surrey"; $postcode = "wh20 3bg"; $telephone = "01372 854785"; $cust_details = fix_cust($firstname, $surname, $address1,$town, $county, $postcode, $telephone ); function fix_cust($n1, $n2, $n3, $n4, $n5, $n6, $n7) { $n1 = ucfirst(strtolower($n1)); $n2 = ucfirst(strtolower($n2)); $n3 = ucwords(strtolower($n3)); $n4 = ucfirst(strtolower($n4)); $n5 = ucfirst(strtolower($n5)); $n6 = ucfirst(strtoupper($n6)); $n7 = ucfirst(strtolower($n7)); return array($n1, $n2, $n3, $n4, $n5, $n6, $n7); } echo $cust_details[0] . " " . $cust_details[1]; echo "<br />"; echo $cust_details[2]; echo "<br />"; echo $cust_details[3]; echo "<br />"; echo $cust_details[4]; echo "<br />"; echo $cust_details[5]; echo "<br />"; echo $cust_details[6]; echo "<br />"; function pcust(){ $length = count($cust_details ); for ($i = 0; $i < $length; $i++) { echo $cust_details[$i]; echo "<br />"; } } pcust();