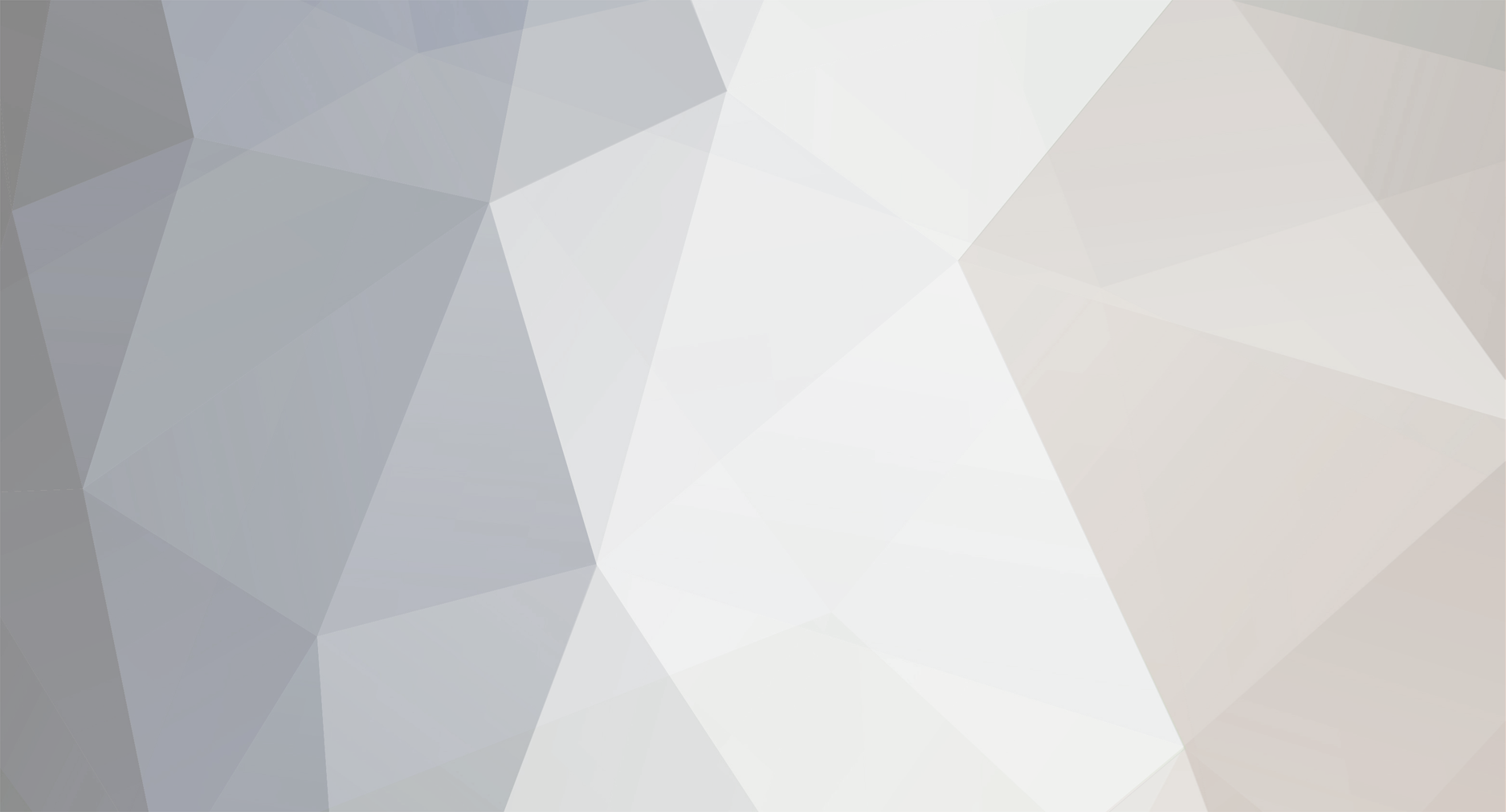
analog
Members-
Posts
45 -
Joined
-
Last visited
Never
Everything posted by analog
-
It's being encoded for the URL. You can use urldecode to convert it back.
-
Adding clear:both; to the .col1 class should stop it from trying to fit below the last one. #col1 { clear:both; float:left; margin-left: 6%; max-width: 350px; /* OLD 200 */ min-width: 350px; /* OLD 200 */ border: 1px solid grey; background-color: #5b5b5b; } Swedish! Jeg snakker ikke Svensk.
-
You need to add table-layout: fixed; to the .tabela class for it size the cells correctly. So it would become: .tabela { width: 100%; cellpading: 0; cellspacing: 0; table-layout: fixed; } That should make it work how you want. More information: http://www.w3schools.com/css/pr_tab_table-layout.asp
-
A lot of queries for a single page would be quite bad for performance and scalability. You can load all the language strings into an assoc array and use that. Something like this (Untested code): <?php $query_language = "SELECT lang_entry_data, lang_entry_code FROM lang_entries WHERE lang_id = '1'"; $language = mysql_query($query_language, $db) or die(mysql_error()); $lang = array(); while($row = mysql_fetch_assoc($language)) $lang[$row_language['lang_entry_data']] = $row_language['lang_entry_data']; echo $lang['AA0005']; // output the lang string for AA0005 ?>
-
You could change the CWD to the directory of the current file using __FILE__ like this: <?php $old_dir = getcwd(); chdir(dirname(__FILE__)); echo getcwd(); // do your stuff exec("../bin/code.cur"); chdir($old_dir); echo getcwd(); // all back to what it was again ?>
-
The error: Warning: Cannot modify header information - headers already sent by (output started at header.php:62) in file.php on line 65 Is due to the fact that header.php is sending output to the browser. Once output has been sent you can no longer set HTTP headers so this: header("Location: thisfile.php?var1=$plant_id"); is failing. One easy fix is to call ob_start at the top of the header file like this: <?php ob_start(); ?> // rest of header here That will stop any output being sent until either the script has finished or you call ob_end_flush.
-
alternate the <title> between original and new title
analog replied to jason310771's topic in Javascript Help
You could use AJAX to do that. Have it fetch the output of a PHP script that outputs how many messages a user has and then display that. Just have it on an interval of a few seconds and it will check without the user needing to refresh. -
whoops there was a space between the backslash and single quote near text/javascript. Try: 556: var s = d.createElement(t); s.type = \'text/javascript\'; s.async = true; 557: s.src = (\'https:\' == document.location.protocol ? vglnk.api_url : 558: \'//cdn.viglink.com/api\') + \'/vglnk.js\'; 559: var r = d.getElementsByTagName(t)[0]; r.parentNode.insertBefore(s, r); 560: }(document, \'script\'));
-
alternate the <title> between original and new title
analog replied to jason310771's topic in Javascript Help
Just changing document.title should change the title. To make it switch between two different titles over and over you could use setInterval. Something like: <html> <head> <title>Original</title> </head> <body> <script language="javascript"> var originalTitle = document.title; var newTitle = "You have a message"; function toggleTitle() { if(document.title == originalTitle) document.title = newTitle; else document.title = originalTitle; } var interval = setInterval("toggleTitle()", 1000); // clearInterval(interval); call this to stop it toggling the title </script> </body> </html> -
556: var s = d.createElement(t); s.type =\ 'text/javascript'; s.async = true; The single quote after javascript needs to be escaped as well as the others. Try: 556: var s = d.createElement(t); s.type =\ 'text/javascript\'; s.async = true; 557: s.src = (\'https:\' == document.location.protocol ? vglnk.api_url : 558: \'//cdn.viglink.com/api\') + \'/vglnk.js\'; 559: var r = d.getElementsByTagName(t)[0]; r.parentNode.insertBefore(s, r); 560: }(document, \'script\'));
-
Just looked it up in the manual and it says this: By default, microtime() returns a string in the form "msec sec", where sec is the current time measured in the number of seconds since the Unix epoch (0:00:00 January 1, 1970 GMT), and msec is the number of microseconds that have elapsed since sec expressed in seconds. So only the first would be accurate. You can use sleep to check it by making it sleep for more than 1 second. I would use the code from the manual though that way you don't need to explode and then add the results together.
-
I think the microseconds part is how many microseconds have elapsed since the seconds part. So if you excluded the seconds and the script took more than 1 second it would be out by however many full seconds it took to execute. I'm not completely sure but I think that's what would happen.
-
This would do it: <?php for($i=1;$i<=100;++$i) { if($i % 3 == 0 && $i % 5 == 0) echo "FizzBuzz\r\n"; elseif($i % 3 == 0) // if remainder of i divided by 3 is 0 then i is a multiple of 3. See http://en.wikipedia.org/wiki/Modulo_operation echo "Fizz\r\n"; elseif($i % 5 == 0) echo "Buzz\r\n"; else echo $i . "\r\n"; } ?> You could probably do it a bit nicer than that but it's one way.
-
Put die after the header and it will stop. Header() doesn't stop the processing it just sets HTTP headers.
-
One thing would be to use mod_rewrite if you are going to be using Apache server. You could then keep the old links the same (best not to break old links) and make the URLs easier for people to read. Although if you won't be using query strings it won't have that big an effect.
-
The HTML is being rendered in Quirks Mode which causes some legacy behavior meaning that the font size isn't inherited properly. See http://devedge-temp.mozilla.org/viewsource/2002/table-inherit/ If you put a doctype at the top it should fix it. e.g. put: <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd"> at the very top of the HTML that should fix it.
-
You can use mysql_affected_rows after 2 to check the number of rows it affected (deleted).
-
You need to change the # in the forms HTML action attribute to the URL of the PHP script you want the post data sent to. So instead of: <form action="#" method="post" enctype="multipart/form-data"> it would be: <form action="http://www.example.com/handleform.php" method="post" enctype="multipart/form-data">
-
If you only used the microseconds part and your script took longer than a second to execute it would give the wrong result wouldn't it? But why are you exploding the output at all? Why not just do: // From of the PHP manual: <?php $time_start = microtime(true); ?> //rest of code <?php $time_end = microtime(true); $time = $time_end - $time_start; echo "This page was created in {$time} seconds";?>
-
The first few lines of the code you posted have the single quites escaped: 545: subMenusContainerId:\'admsubMenus\', 546: duration:\'150\' Which might be because the JavaScript is inside a PHP string. If you escape the other single quotes like this: subMenusContainerId:\'admsubMenus\', duration:\'150\' }); }); } </script> <script type="text/javascript"> var vglnk = { api_url: \'//api.viglink.com/api\', key: \'ad93febdf4e33c034d9635037b687fb3\' }; (function(d, t) { var s = d.createElement(t); s.type =\ 'text/javascript\'; s.async = true; s.src = (\'https:\' == document.location.protocol ? vglnk.api_url : Dose it fix the error?