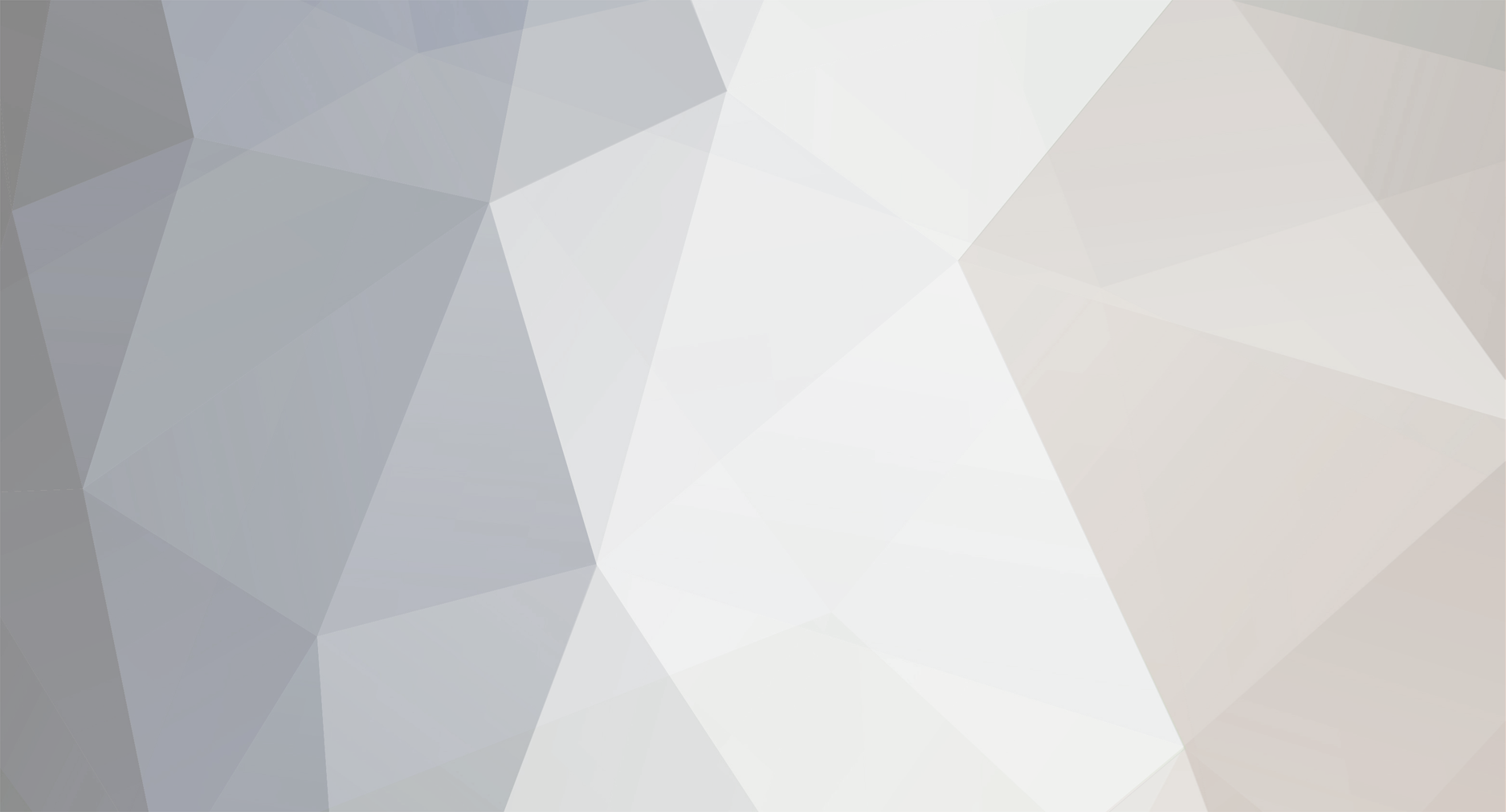
HDFilmMaker2112
Members-
Posts
547 -
Joined
-
Last visited
Never
Everything posted by HDFilmMaker2112
-
if(isset($_GET['cat'])){ $cat=$_GET['cat']; $cat2=$_GET['cat']; $cat=str_replace("-"," ", $cat); if(isset($_GET['num_products']) && ctype_digit($_GET['num_products'])){ $num_products_per_page=(int)$_GET['num_products']; $_SESSION[$cat2]['num_products_per_page'] = $num_products_per_page; $num_products_per_page = stripslashes($num_products_per_page); $num_products_per_page = mysql_real_escape_string($num_products_per_page); } else{ $num_products_per_page="20"; } $num_products=' <form action="" method="GET"> <label>Display:</label> <select id="num_products" onchange="this.form.submit();" disabled="disabled"> <option value="8">8 Items per Page</option> <option value="12">12 Items per Page</option> <option value="16">16 Items per Page</option> <option value="20" selected="selected">20 Items per Page</option> <option value="24">24 Items per Page</option> <option value="32">32 Items per Page</option> <option value="40">40 Items per Page</option> <option value="48">48 Items per Page</option> </select> <noscript> <input type="submit" value="Go" /> </noscript> </form> '; if(isset($_GET['sort_by'])){ $sort_by_selected=$_GET['sort_by']; $_SESSION[$cat2]['sort_by_selected'] = $sort_by_selected; $sort_by_selected = stripslashes($sort_by_selected); $sort_by_selected = mysql_real_escape_string($sort_by_selected); if(isset($sort_by_selected)){ $sort_by_selected2=$sort_by_selected; if($sort_by_selected2=="product_price_hl"){ $sort_by_selected2="ABS(product_price) DESC"; } elseif($sort_by_selected2=="product_price_lh"){ $sort_by_selected2="ABS(product_price) ASC"; } } } else{ $sort_by_selected2="product_id"; } $sort_by=' <form action="store.php?cat='.$cat2.'" method="GET"> <label>Sort By:</label> <select id="sort_by" onchange="this.form.submit();"> <option value="relevance">Relevance</option> <option value="product_price_hl">Price: High to Low</option> <option value="product_price_lh">Price: Low to High</option> </select> <noscript> <input type="submit" value="Go" /> </noscript> </form> '; } else{ header("Location: ./index.php"); } For some reason the Sort By: form is redirecting to index.php...
-
Adding all the results from all the fields.
HDFilmMaker2112 replied to u214's topic in PHP Coding Help
mysql_query only gives you the id location in the database. You have to apply that to an array to actually pull data out of it. Use: $total_kills=mysql_fetch_array($total_kills) directly after $total_kills = mysql_query("SELECT SUM(kills) FROM playerinfo", $connect); -
Adding all the results from all the fields.
HDFilmMaker2112 replied to u214's topic in PHP Coding Help
Try adding SUM(kills) to the SQL query. -
The issue is, what I'm coding will eventually be out for other people to use; It's cart software. They might think they have to escape stuff when they type it in the admin panel.
-
Alright made a test table and test code: <?php function sanitize($formValue){ if( function_exists(get_magic_quotes_gpc()) && get_magic_quotes_gpc() ) { $formValue = stripslashes($formValue); } $formValue = mysql_real_escape_string($formValue); return $formValue; } $string="Is your name O\'reilly?"; $string=sanitize($string); $sql="INSERT INTO $tbl_name (test123) VALUES ('$string')"; mysql_query($sql) or die("Problem with the query: $sql<br />" . mysql_error()); echo "Inserted: $string <br /><br />"; $sql2="SELECT * FROM $tbl_name"; $result2=mysql_query($sql2); while($row2=mysql_fetch_array($result2)){ extract($row2); echo $test123; } ?> That echo's out: So how would I remove the \ from the data pulled from database?
-
But once mysql_real_escape_string($formValue); is used, wouldn't that add another backslash to "O\'reilly" resulting in "O\\'reilly"?
-
If I do that I get this: From this: <?php function sanitize($formValue){ if( function_exists(get_magic_quotes_gpc()) && get_magic_quotes_gpc() ) { $formValue = stripslashes($formValue); } //$formValue = mysql_real_escape_string($formValue); return $formValue; } $string="Is your name O\'reilly?"; $string=sanitize($string); echo $string; $string2="abssagasdfasdf \' \ asdfjkla\\\ asdala\?"; $string2=sanitize($string2); echo "<br />"; echo $string2; ?> It's only removing one slash.
-
Alright, thanks. One more question... should the function go before or after it's used? I'm assuming before, but double checking.
-
First time I'm looking into creating my own functions, so I'm making sure I'm getting this right: If I have the following: <?php function formSanitize($formValue){ $formValue = stripslashes($formValue); $formValue = mysql_real_escape_string($formValue); } ?> Then if I just use this: <?php $product_name=formSanitize($_POST['product_name']); ?> It should do the same this as this: $product_name=$_POST['product_name']; $product_name = stripslashes($product_name); $product_name = mysql_real_escape_string($product_name);
-
I'm trying to make it so somebody can't do an SQL injection on my script, by using is_int and setting the $_GET['id'] to an integer with (int). Now even when the URL contains product=4 it pulls from the database as product=1. If I use is_numeric all that has to be done is include a number in the SQL injection and then is_numeric will return true. As per the PHP manual: elseif(isset($_GET['product'])){ $product_id=is_int((int)$_GET['product']); $sql500="SELECT * FROM $tbl_name3 WHERE product_id='$product_id' AND review_show='y' ORDER BY review_date"; $result500=mysql_query($sql500); $num_rows500=mysql_num_rows($result500); if($num_rows500==0){ $average_rating=0; } else{ while($row500=mysql_fetch_array($result500)){ extract($row500); if($review_show=="y"){ $total = $total + $review_product_rating; $review.=' <div class="review_container"> <div class="review_title">'.$review_title.'</div> <div><img src="'.$review_product_rating.'.png" alt="'.$review_product_rating.' Stars" /></div> <div><span class="bold">By '.$review_name.' from '.$review_location.' on '.$review_date.'</span></div> <div><span class="bold">Describe Yourself:</span> '.$review_describe.'</div> <div><span class="bold">Best Use:</span> '.$review_best_use.'</div> <div><span class="bold">Pros:</span> '.$review_pros.'</div> <div><span class="bold">Cons:</span> '.$review_cons.'</div> <div><span class="bold">Comments</span></div><div>'.$review_text.'</div> </div> <hr /> '; $review_product_rating_total=$total; $a=$review_product_rating_total/$num_rows500; $i=round($a, 1); $b=$i; $i=explode(".", $i); $decimal=$i[1]; if($decimal < 5){ $j=floor($b); $i= $decimal > 3 ? $j + .5 : $j; $average_rating=$i; } else{ $j=ceil($b); $i= $decimal < 8 ? $j - .5 : $j; $average_rating=$i; } } } } $sql50="SELECT * FROM $tbl_name WHERE product_id='$product_id'"; $result50=mysql_query($sql50); while($row50=mysql_fetch_array($result50)){
-
I'm trying to back engineer the sort by and items per page drop down menus on this website: http://www.bhphotovideo.com/c/buy/Music-Production-Software/ci/14793/N/4294550038 I found their javascript file: function setItemsPerPage(form, itemsPerPage) { form.itemsPerPage.value = itemsPerPage; form.submit(); } function setSortBy(form, sortBy) { form.sortBy.value = sortBy; form.submit(); } $(document).ready(function(){ $(".jqDropDownLayer").bhLayer({ openerSelector:"sibling .jqDropDownOpener", closerSelector: "label", modal: true }).bind("onBeforeShow", function(){ $(this).siblings(".jqDropDownOpener").addClass("darker1"); $(".productBlock:first").css("z-index","-1"); }).bind("onAfterHide", function(){ $(this).siblings(".jqDropDownOpener").removeClass("darker1"); $(".productBlock:first").css("z-index","0"); }); $(".jqDropDown").mouseleave(function(){ var hideLayer = $(this).find(".jqDropDownLayer"); if(hideLayer.hasClass("openBhLayer")) hideLayer.bhLayer("hide"); }); }); This is their HTML/CSS: <script type="text/javascript" src="/FrameWork/js/sortby.js"> </script> <form name="sortBy" method="get" action="http://www.bhphotovideo.com/c/search" style="float: left;" class="jqDropDown"> <input type="hidden" name="Ns" id="sortBy" value="" /> <div class="sortMain"><div class="sortby1 jqDropDownOpener"><a><label class="sortDisplay">Relevance </label><span>Sort by:</span></a></div> <input type="hidden" name="ci" value="14793" /> <input type="hidden" name="N" value="4294550038" /> <div class="sortby2 jqDropDownLayer"> <ul> <li><a><label onClick="setSortBy(this.form,'')">Relevance</label></a></li> <li><a><label onClick="setSortBy(this.form,'p_PRICE_2|0')">Price: Low to High</label></a></li> <li><a><label onClick="setSortBy(this.form,'p_PRICE_2|1')">Price: High to Low</label></a></li> <li><a><label onClick="setSortBy(this.form,'p_PRODUCT_SHORT_DESCR|0')">Brand: A to Z</label></a></li> <li><a><label onClick="setSortBy(this.form,'p_PRODUCT_SHORT_DESCR|1')">Brand: Z to A</label></a></li> <li><a><label onClick="setSortBy(this.form,'p_OVER_ALL_RATE|1')">Top Rated</label></a></li> </ul> </div> </div> <noscript> <input type="image" src="/images/searcharrow.gif" border=0 name="image" alt="Search" align="absmiddle" hspace="2"> </noscript> </form> <form method="get" action="http://www.bhphotovideo.com/c/search" style="float: left;" class="jqDropDown" > <input type="hidden" name="ipp" id="itemsPerPage" value="" /> <div class="displayMain"><div class="display1 jqDropDownOpener"><a><label class="sortDisplay">75</label><span>Display:</span></a></div> <input type="hidden" name="ci" value="14793" /> <input type="hidden" name="N" value="4294550038" /> <div class="display2 jqDropDownLayer"> <ul> <li><a><label onClick="setItemsPerPage(this.form,25);">25</label></a></li> <li><a><label onClick="setItemsPerPage(this.form,50);">50</label></a></li> <li><a><label onClick="setItemsPerPage(this.form,75);">75</label></a></li> <li><a><label onClick="setItemsPerPage(this.form,100);">100</label></a></li> </ul> </div> </div> </form> Could I get an overview of what's doing what here? I've never used jQuery before. Basically in the end I want it to function where it says: Display: then a drop down of numbers; just like they have.
-
Not returning result from 2nd time in loop
HDFilmMaker2112 replied to tanveer's topic in PHP Coding Help
You need a while loop: http://www.php.net/manual/en/control-structures.while.php -
Well I just added is_numeric(); to all calls to an ID in the database and all the places where I get a LIMIT setting from an external source. Is that what you were referring to? if(isset($_GET['num_products']) && is_numeric($_GET['num_products'])){ $num_products_per_page=$_GET['num_products']; $num_products_per_page = stripslashes($num_products_per_page); $num_products_per_page = mysql_real_escape_string($num_products_per_page); }
-
Okay, so how do I prevent it, because I don't know of any other way?
-
I need a way to store the settings for a specific category that user is viewing. Say they click into category "A", and choose Sort By: Name and Products per Page: 24 and then click into category "C", and choose Sort By: Price and Products per Page: 48. Then click back into category "A", I want their initial settings choices of Sort By: Name and Products per Page: 24 to come back up. I also want to keep this in the URL as best as possible so they can bookmark it. How do I do this? if(isset($_GET['cat'])){ $cat=$_GET['cat']; $cat=str_replace("-"," ", $cat); session_start(); if(isset($_GET['num_products'])){ $num_products_per_page=$_GET['num_products']; $num_products_per_page = stripslashes($num_products_per_page); $num_products_per_page = mysql_real_escape_string($num_products_per_page); } else{ $num_products_per_page="20"; } $num_products=''; if(isset($_GET['sort_by'])){ $sort_by_selected=$_GET['sort_by']; $sort_by_selected = stripslashes($sort_by_selected); $sort_by_selected = mysql_real_escape_string($sort_by_selected); } else{ $sort_by_selected="product_id"; } $sort_by=''; }
-
Alternating Color and adding a spacer/divider
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
I guess my only option is something like this?: $pagination= $counter%2 == 0 ? '<span style="font-weight: bold;">' : '<span style="font-weight: bold; font-color: grey;">'; $pagination.=' | </span>'; Inside each if/else statement under each for statement. Would be easier if I could apply that to variable once and just place the variable under each for statement; but there seems to be no way to carry an if/else statement in a variable and then have it function as a statement, not a string. -
I need help adding the following into my pagination code: This first snippet formats a divider "|" into a certain color: (Includes question marks because I'm not sure what to use.) $i = 0; for($i=?; $i=?; $i++){ echo ""; if($i%2 == 0){ echo '<span class="bold"> | </span>'; $i++; } else { echo '<span class="bold grey"> | </span>'; $i++; } } I need to some how integrate that into this: // How many adjacent pages should be shown on each side? $adjacents = 3; /* First get total number of rows in data table. If you have a WHERE clause in your query, make sure you mirror it here. */ $query5 = "SELECT COUNT(*) as num FROM $tbl_name WHERE product_category='$cat'"; $total_pages = mysql_fetch_array(mysql_query($query5)); $total_pages = $total_pages[num]; /* Setup vars for query. */ $targetpage = "store.php?cat=".$_GET['cat']; //your file name (the name of this file) $limit = $num_products_per_page; //how many items to show per page $page = $_GET['page']; if($page){ $start = ($page - 1) * $limit; //first item to display on this page } else{ $start = 0; //if no page var is given, set start to 0 } /* Get data. */ $sql30 = "SELECT * FROM $tbl_name WHERE product_category='$cat' ORDER BY $sort_by_selected LIMIT $start, $limit"; $result30 = mysql_query($sql30) or die("Problem with the query: $sql30<br>" . mysql_error()); /* Setup page vars for display. */ if ($page == 0){ $page = 1; } //if no page var is given, default to 1. $prev = $page - 1; //previous page is page - 1 $next = $page + 1; //next page is page + 1 $lastpage = ceil($total_pages/$limit); //lastpage is = total pages / items per page, rounded up. $lpm1 = $lastpage - 1; //last page minus 1 /* Now we apply our rules and draw the pagination object. We're actually saving the code to a variable in case we want to draw it more than once. */ $pagination = ""; if($lastpage > 1){ $pagination .= "<div class=\"pagination\">"; //previous button if ($page > 1){ $pagination.= '<a href="'.$targetpage.'&page='.$prev.'">« Previous</a> '; } else{ $pagination.= ""; } //pages if ($lastpage < 7 + ($adjacents * 2)) //not enough pages to bother breaking it up { for ($counter = 1; $counter <= $lastpage; $counter++) { if ($counter == $page){ $pagination.= "<span class=\"current\">$counter</span>"; } else{ $pagination.= '<a href="'.$targetpage.'&page='.$counter.'">'.$counter.'</a>'; } } } elseif($lastpage > 5 + ($adjacents * 2)) //enough pages to hide some { //close to beginning; only hide later pages if($page < 1 + ($adjacents * 2)){ for ($counter = 1; $counter < 4 + ($adjacents * 2); $counter++){ if ($counter == $page){ $pagination.= "<span class=\"current\">$counter</span>"; } else{ $pagination.= '<a href="'.$targetpage.'&page='.$counter.'">'.$counter.'</a>'; } } $pagination.= "..."; $pagination.= '<a href="'.$targetpage.'&page='.$lpm1.'">'.$lpm1.'</a>'; $pagination.= '<a href="'.$targetpage.'&page='.$lastpage.'">'.$lastpage.'</a>'; } //in middle; hide some front and some back elseif($lastpage - ($adjacents * 2) > $page && $page > ($adjacents * 2)){ $pagination.= '<a href="'.$targetpage.'&page=1">1</a>'; $pagination.= '<a href="'.$targetpage.'&page=2">2</a>'; $pagination.= "..."; for ($counter = $page - $adjacents; $counter <= $page + $adjacents; $counter++){ if ($counter == $page){ $pagination.= "<span class=\"current\">$counter</span>"; } else{ $pagination.= '<a href="'.$targetpage.'&page='.$counter.'">'.$counter.'</a>'; } } $pagination.= "..."; $pagination.= '<a href="'.$targetpage.'&page='.$lpm1.'">'.$lpm1.'</a>'; $pagination.= '<a href="'.$targetpage.'&page='.$lastpage.'">'.$lastpage.'</a>'; } //close to end; only hide early pages else { $pagination.= '<a href="'.$targetpage.'&page=1">1</a>'; $pagination.= '<a href="'.$targetpage.'&page=2">2</a>'; $pagination.= "..."; for ($counter = $lastpage - (2 + ($adjacents * 2)); $counter <= $lastpage; $counter++) { if ($counter == $page){ $pagination.= '<span class="current">'.$counter.'</span>'; } else{ $pagination.= '<a href="'.$targetpage.'&page='.$counter.'">'.$counter.'</a>'; } } } } //next button if ($page < $counter - 1){ $pagination.= ' <a href="'.$targetpage.'&page='.$next.'">Next »</a>'; $pagination.= "</div>\n"; } else{ $pagination.= ""; $pagination.= "</div>\n"; } } So I end up with it formatted like this: 1 | 2 | 3 Next >> With every other "|" divider line being grey-ish color.
-
Got it... Who ever wrote the script I'm using, forgot to close the first div if($page < $counter - 1); evaluated to true.
-
Here's the PHP: if(isset($_GET['cat'])){ $cat=$_GET['cat']; $cat=str_replace("-"," ", $cat); if(isset($_POST['num_products'])){ $num_product_per_page=$_POST['num_products']; $num_product_per_page = stripslashes($num_product_per_page); $num_product_per_page = mysql_real_escape_string($num_product_per_page); } else{ $num_products_per_page="4"; } $num_products=''; if(isset($_POST['sort_by'])){ $sort_by_selected=$_POST['sort_by']; $sort_by_selected = stripslashes($sort_by_selected); $sort_by_selected = mysql_real_escape_string($sort_by_selected); } else{ $sort_by_selected="product_id"; } $sort_by=''; // How many adjacent pages should be shown on each side? $adjacents = 3; /* First get total number of rows in data table. If you have a WHERE clause in your query, make sure you mirror it here. */ $query5 = "SELECT COUNT(*) as num FROM $tbl_name WHERE product_category='$cat'"; $total_pages = mysql_fetch_array(mysql_query($query5)); $total_pages = $total_pages[num]; /* Setup vars for query. */ $targetpage = "store.php?cat=".$_GET['cat']; //your file name (the name of this file) $limit = $num_products_per_page; //how many items to show per page $page = $_GET['page']; if($page){ $start = ($page - 1) * $limit; //first item to display on this page } else{ $start = 0; //if no page var is given, set start to 0 } /* Get data. */ $sql30 = "SELECT * FROM $tbl_name WHERE product_category='$cat' ORDER BY $sort_by_selected LIMIT $start, $limit"; $result30 = mysql_query($sql30) or die("Problem with the query: $sql30<br>" . mysql_error()); /* Setup page vars for display. */ if ($page == 0){ $page = 1; } //if no page var is given, default to 1. $prev = $page - 1; //previous page is page - 1 $next = $page + 1; //next page is page + 1 $lastpage = ceil($total_pages/$limit); //lastpage is = total pages / items per page, rounded up. $lpm1 = $lastpage - 1; //last page minus 1 /*Now we apply our rules and draw the pagination object. We're actually saving the code to a variable in case we want to draw it more than once. */ $pagination = ""; if($lastpage > 1){ $pagination .= "<div class=\"pagination\">"; //previous button if ($page > 1){ $pagination.= '<a href="'.$targetpage.'&page='.$prev.'">« Previous</a>'; } else{ $pagination.= ""; } //pages if ($lastpage < 7 + ($adjacents * 2)) //not enough pages to bother breaking it up { for ($counter = 1; $counter <= $lastpage; $counter++) { if ($counter == $page){ $pagination.= "<span class=\"current\">$counter</span>"; } else{ $pagination.= '<a href="'.$targetpage.'&page='.$counter.'">'.$counter.'</a>'; } } } elseif($lastpage > 5 + ($adjacents * 2)) //enough pages to hide some { //close to beginning; only hide later pages if($page < 1 + ($adjacents * 2)){ for ($counter = 1; $counter < 4 + ($adjacents * 2); $counter++){ if ($counter == $page){ $pagination.= "<span class=\"current\">$counter</span>"; } else{ $pagination.= '<a href="'.$targetpage.'&page='.$counter.'">'.$counter.'</a>'; } } $pagination.= "..."; $pagination.= '<a href="'.$targetpage.'&page='.$lpm1.'">'.$lpm1.'</a>'; $pagination.= '<a href="'.$targetpage.'&page='.$lastpage.'">'.$lastpage.'</a>'; } //in middle; hide some front and some back elseif($lastpage - ($adjacents * 2) > $page && $page > ($adjacents * 2)){ $pagination.= '<a href="'.$targetpage.'&page=1">1</a>'; $pagination.= '<a href="'.$targetpage.'&page=2">2</a>'; $pagination.= "..."; for ($counter = $page - $adjacents; $counter <= $page + $adjacents; $counter++){ if ($counter == $page){ $pagination.= "<span class=\"current\">$counter</span>"; } else{ $pagination.= '<a href="'.$targetpage.'&page='.$counter.'">'.$counter.'</a>'; } } $pagination.= "..."; $pagination.= '<a href="'.$targetpage.'&page='.$lpm1.'">'.$lpm1.'</a>'; $pagination.= '<a href="'.$targetpage.'&page='.$lastpage.'">'.$lastpage.'</a>'; } //close to end; only hide early pages else { $pagination.= '<a href="'.$targetpage.'&page=1">1</a>'; $pagination.= '<a href="'.$targetpage.'&page=2">2</a>'; $pagination.= "..."; for ($counter = $lastpage - (2 + ($adjacents * 2)); $counter <= $lastpage; $counter++) { if ($counter == $page){ $pagination.= '<span class="current">'.$counter.'</span>'; } else{ $pagination.= '<a href="'.$targetpage.'&page='.$counter.'">'.$counter.'</a>'; } } } } } //next button if ($page < $counter - 1){ $pagination.= '<a href="'.$targetpage.'&page='.$next.'">Next »</a>'; } else{ $pagination.= ""; $pagination.= "</div>\n"; } } $cat2=str_replace(" ","-", $cat); while($row30=mysql_fetch_array($result30)){ extract($row30); $section=ucwords($product_category); $crumbs='<a href="./index.php">Home</a> <span class="eleven">»</span> <a href="./store.php?cat='.$cat2.'">'.$section.'</a>'; $section=" - ".$section; $product_name=ucwords($product_name); $content.='<div class="content_text2"><div class="product"><div><a href="./store.php?product='.$product_id.'"><img src="/images/'.$product_image.'" alt="'.$product_name.'" class="product_image" /></a></div> <div><a href="./store.php?product='.$product_id.'">'.$product_name.'</a></div> <div>Price: $'.number_format($product_price, 2, '.', ',').'</div> <div>'.$product_link.'</div></div></div>'; } $records_on_page = mysql_num_rows($result30); $start_record = $start + 1; $end_record = $start_record + $records_on_page -1; $products_on_page="Showing ".$start_record." - ".$end_record." out of ".$total_pages." product"; if($total_pages > 1){ $products_on_page.="s"; } } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.1//EN" "http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en"> <head> <base href="http://ghosthuntersportal.com/" /> <title>Ghost Hunter's Portal <?php echo "$section"; ?></title> <meta http-equiv="content-type" content="text/html; charset=utf-8" /> <meta name="verify-v1" content="" /> <meta name="keywords" content="ghost, hunters, hunter, ghosts, spirit, spirits, paranormal, investigation, investigator, investigators, k2, emf, meter, kii" /> <meta name="description" content="Ghost Hunters Potal. Parnormal research equipment store." /> <meta name="author" content="Andrew McCarrick" /> <meta name="robots" content="index, follow" /> <?php if($_GET['donate']=="thankyou"){ echo '<meta http-equiv="refresh" content="5;url=http://makethemoviehappen.com/index.php">'; } elseif($_GET['donate']=="cancel"){ echo '<meta http-equiv="refresh" content="1;url=http://makethemoviehappen.com/index.php">'; } else{ } ?> <style type="text/css"> <?php if (strstr($_SERVER["HTTP_USER_AGENT"], "MSIE 7")) { echo 'body {background-color: #000000; color: #FFFFFF; font-family: Tahoma; margin-top: 10px; margin-right:auto;margin-left:auto;max-width:1000px;}'; } else { echo 'body {background-color: #000000; color: #FFFFFF; font-family: Verdana; margin-top: 10px; margin-right:auto;margin-left:auto;max-width:1000px;}'; } ?> </style> <link rel="stylesheet" type="text/css" href="style.css" /> <link rel="shortcut icon" href="./favicon.png" /> <? if(isset($_GET['product'])){ include 'ajax.php'; } ?> </head> <body <? if(isset($_GET['product'])){ echo 'onload="load();"';} ?>> <div class="header"> <a href="./index.php"> <div class="logo"><img src="./logo.png" alt="Ghost Hunter's Portal" /></div> <div class="tag"><img src="./tag.png" alt="Ghost Hunter's Portal" /></div> </a> <div class="header_right"> <div class="ad"> </div> <div class="header_links"> <a href="./index.php">Home</a> | <a href="https://www.paypal.com/cgi-bin/webscr?cmd=_cart&business=A2YAZNFRRYNFG&display=1">View Cart</a> | <a href="./index.php?returns">Returns</a> | <a href="./index.php?aboutus">About Us</a> | <a href="./index.php?contactus">Contact Us</a> </div> </div> </div> <div class="content_wrapper"> <div class="links"> <div class="categories">Categories</div> <?php $sql100="SELECT DISTINCT product_category FROM $tbl_name"; $result100=mysql_query($sql100); while($row100=mysql_fetch_array($result100)){ $product_category=$row100['product_category']; $product_category_link=$product_category; $product_category_link=strtolower($product_category_link); $product_category_link=str_replace(" ","-", $product_category_link); $product_category=ucwords($product_category); echo '<div><a href="store.php?cat='.$product_category_link.'">'.$product_category.'</a></div>'."\n"; } ?> <div class="sig"><img src="./sig.png" /></div> </div> <div class="data_wrapper"> <div class="search_crumbs"> <div class="crumbs"> <? echo $crumbs; ?> </div> <div class="search"> <form action="./index.php?search" method="post"> <label>Search</label> <input type="text" name="search" size="30" /> <input type="submit" value="Go" name="Go" /> </form> </div> </div> <? if(isset($products_on_page)){ echo " <div class=\"number_pages_wrapper\"> <div class=\"number_products\">$products_on_page</div> <div class=\"page_numbers\">$pagination</div> </div> <div class=\"number_pages_wrapper\"> <div class=\"number_products\">$sort_by</div> <div class=\"page_numbers\">$num_products</div> </div> "; } ?> <div class="content"> <? echo $content; ?> </div> <? echo " <div class=\"number_pages_wrapper\"> <div class=\"number_products\"> </div> <div class=\"page_numbers\">$pagination</div> </div>" ?> <br /> </div> </div> <div class="footer"> © 2011 <?php echo $copyrightdate; ?> Ghost Hunter's Portal, L.L.C. </div> </body> </html>
-
If you go to this page: http://www.ghosthuntersportal.com/store.php?cat=emf-meters You can see that the page is misformatted, but if you click the link to page 2 or the Next Link, that page is perfectly fine. Ideas on why the first page is misformatted? They're both coming from the same PHP script, and the CSS defined in those div tag and span tags doesn't exist yet.
-
Well if the error is printing out "product_id" wouldn't that mean it's echoing out? Anyway, moved the ORDER BY before the LIMIT and it's working now... doesn't make much sense to me. Thought it really didn't matter about the order so long as the query started with a SELECT, INSERT, or DELETE statement. Thanks for the help.