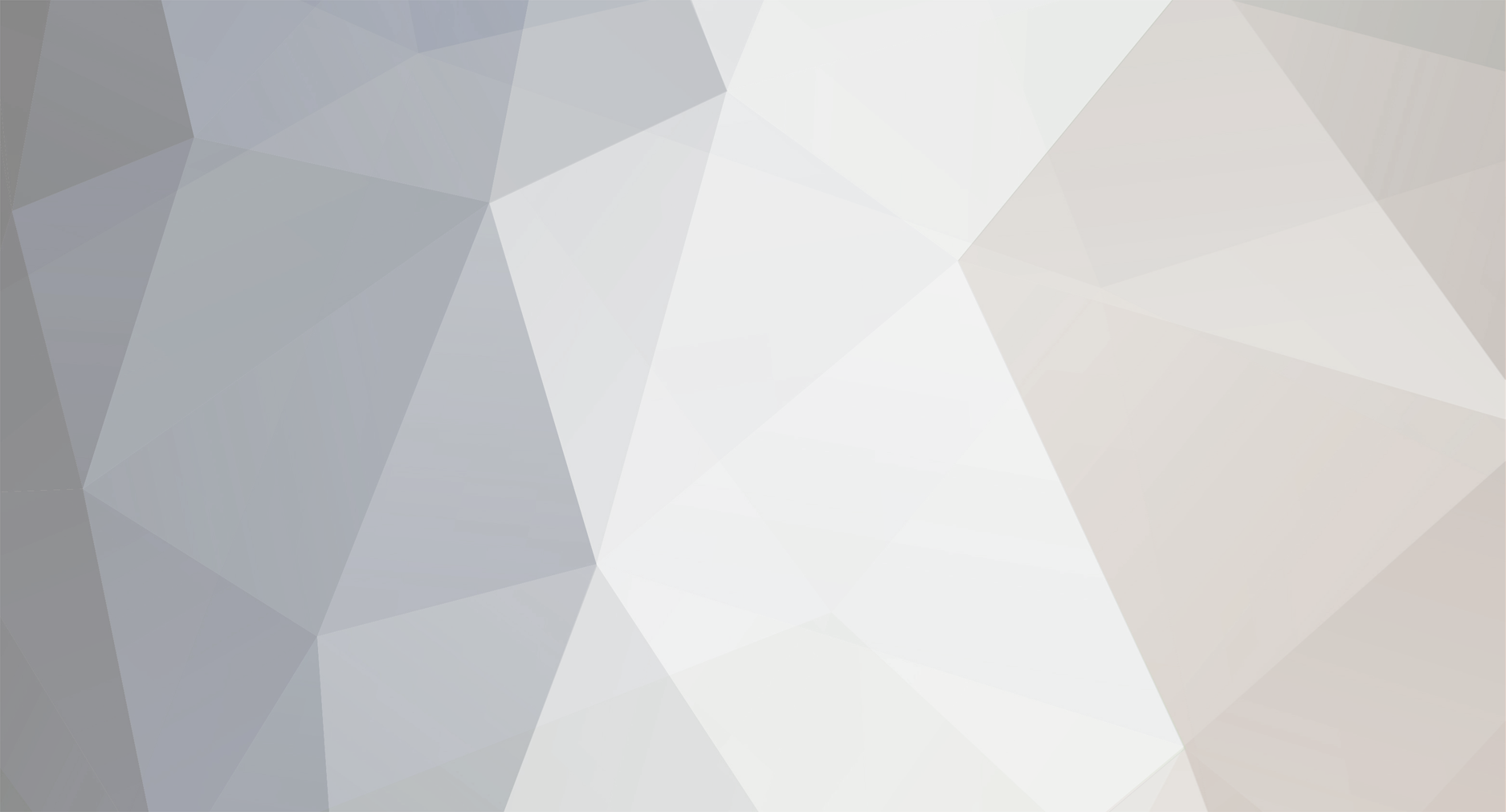
HDFilmMaker2112
Members-
Posts
547 -
Joined
-
Last visited
Never
Everything posted by HDFilmMaker2112
-
PHP PDO quote() returning error
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
Because you need to escape incoming user submitted data when not using prepared statements. I have no reason to use prepared statements; as I'm not iterating through anything that would cause the need for duplicate queries (the point of prepared statements). Not to mention prepared statements are approximately 2 to 3 times slower than quoted/regular queries via PDO (when running single queries, multiple queries are faster). Honestly, in this specific situation, if it weren't for the transaction features of PDO, I'd actually use MySQLi. -
The PDO quote() function is returning an error for me, lost as to why. Fatal error: Call to a member function quote() on a non-object else{ $register_name ="$register_fname $register_lname"; $register_birthday ="$register_year - $register_month - $register_day"; $register_date=date('Y-m-d H:i:s'); SafePDOCOE(db_name); $quoted_account_type = $DB->quote($register_account_type); $quoted_email = $DB->quote($register_email); $quoted_fname = $DB->quote($register_fname); $quoted_lname = $DB->quote($register_lname); $quoted_name = $DB->quote($register_name); $encoded_password = kam3($register_password); $quoted_gender = $DB->quote($register_gender); $quoted_birthday = $DB->quote($register_birthday); $quoted_membership_type = $DB->quote($register_membership_type); try{ $DB->beginTransaction(); $DB->query("INSERT INTO user (email_address, password, user_level, name, membership_type, join_date) VALUES ($quoted_email, $encoded_password, '1', $quoted_name, $quoted_membership_type, $register_date)"); $userid = $DB->lastInsertId(); $DB->query("INSERT INTO user_profile (user_id, birthday, gender, first_name, last_name) VALUES ($userid, $quoted_birthday, $quoted_gender, $quoted_fname, $quoted_lname)"); $DB->commit(); echo "Data Entered."; } catch(PDOException $e){ $DB->rollBack(); echo "Query Error: ". $e->getMessage(); } } All the variables I'm quoting are coming from a form. Here's the function: function SafePDOCOE($dbname){ $DBconnect = new SafePDO_errordisplay("mysql:host=localhost;dbname=$dbname", "user", "pass"); return $DBconnect; } Should I be using $DBconnect instead of $DB? $DB is used in the SafePDO class. (EDIT: Tried changing the variable in the function to $DB, not the issue. Still have the same problem.)
-
Convert this into a function or object?
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
I guess for those few other situations I could just do the checking manually and append to the $error array as follows?: $error() = (int)hasEmptyFields($_POST, $fields) if($register_email!=$register_check_email){ $error[]=1; } else{ $error[]=0; } if($register_password!=$register_check_password){ $error[]=1; } else{ $error[]=0; } $number_of_zeros=str_repeat('0', count($error)); if ($number_of_zeros != $error){ header(Location: ); } Just looking for confirmation that would work. I'm hesitant to test, as I don't want to screw up any other code. -
Convert this into a function or object?
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
That's nearly perfect. Is there any way to add in a variable matching comparison? As per the last two if statements in my original code: if($register_email!=$register_check_email){ $error[13]=1; } else{ $error[13]=0; } if($register_password!=$register_check_password){ $error[14]=1; } else{ $error[14]=0; } -
Convert this into a function or object?
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
Basically I want it to function close to the following way. I would generate an array in this format: array_name($variable_name, array_of_type_of_error_checking(Could check for isset, empty, blank($variable_name==""), or match(compare two variables to see if they're the same)), if match variable to compare it to); From there, it would need to pull that data into a function, have it trigger the correct error checking called for and output an array $error with either a 0 or 1 based on the conditions evaluating to true or not. I could use a little help on polishing up how this would actually function, and how to implement it. This is what I have so far as an example for the data input side: $register_account_type_array=array($register_account_type, array("isset","empty","blank")); $register_fname_array=array($register_fname, array("isset","empty","blank")); $register_lname_array=array($register_lane, array("isset","empty","blank")); $register_email_array=array($register_email, array("isset","empty","blank","match"), $register_check_email); $merged_array=array_merge($register_account_type_array, $register_fname_array, $register_lname_array, $register_email_array); $error = error_check($merged_array); I need a way to split the merged_array once it gets into the function. No idea how to do this. It needs to essentially split it back to each individual array before it got merged into one array. And then break off the "error check type assigning" sub-array to trigger their specific error checking job. -
Code for a Changeable Birthday Date
HDFilmMaker2112 replied to timothyarden's topic in PHP Coding Help
You can call this function from your month menu, and year menu. function generateBirthDayMenu($month,$year){ session_start(); $_SESSION['birthday_month']=$month; $_SESSION['birthday_year']=$year; $day_array=array(1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20,21,22,23,24,25,26,27,28); $month_array1=array("April", "June", "September", "November"); $month_array2=array("January", "March", "May", "July", "August", "October", "December"); if($month=="February" && ($year % 4) ==0 && (($year % 100) !=0 || ($year % 400) ==0)){ array_push($day_array, "29"); } elseif(!isset($month)){ array_push($day_array, "29", "30", "31"); } else{ } foreach($month_array1 as $month_value){ if($month==$month_value){ array_push($day_array, "29", "30"); } } foreach($month_array2 as $month_value){ if($month==$month_value){ array_push($day_array, "29", "30", "31"); } } foreach($day_array as $day_item){ $day.='<option value="'.$day_item.'"'; if(isset($_SESSION['birthday_day']) && $_SESSION['birthday_day']==$day_item){ $day.=' selected="selected"'; } $day.='>'.$day_item.'</option>'."\n"; } return $day; } You need to use AJAX, and send the values of the month and year selected with a onclick change in your year and month select menus. For example the month drop down would need to have this added to the select tag: onchange="sndReq(register_year.value,this.value); The year menu would have to have this added to the select tag: onchange="sndReq(this.value,register_month.value); This is the page I use for my ajax call. <?php require_once 'function.php'; session_start(); if(!isset($month) || (isset($_GET['month']) && $_GET['month']!=$month)){ $month=$_GET['month']; } if(!isset($year) || (isset($_GET['year']) && $_GET['year']!=$year0)){ $year=$_GET['year']; } $form_day_menu=generateBirthDayMenu($month,$year); echo "<select name=\"day\"> <option>Day</option> $form_day_menu </select>"; ?> You may or may not want to remove the sessions. -
In the past this is how I've done most of my error checking: if(empty($register_account_type) || !isset($register_account_type)){ $error[0]=1; } else{ $error[0]=0; } if(empty($register_fname) || !isset($register_fname)){ $error[1]=1; } else{ $error[1]=0; } if(empty($register_lname) || !isset($register_lname)){ $error[2]=1; } else{ $error[2]=0; } if(empty($register_email) || !isset($register_email)){ $error[3]=1; } else{ $error[3]=0; } if(empty($register_check_email) || !isset($register_check_email)){ $error[4]=1; } else{ $error[4]=0; } if(empty($register_password) || !isset($register_password)){ $error[5]=1; } else{ $error[5]=0; } if(empty($register_check_password) || !isset($register_check_password)){ $error[6]=1; } else{ $error[6]=0; } if(empty($register_gender) || !isset($register_register)){ $error[7]=1; } else{ $error[7]=0; } if(empty($register_month) || !isset($register_month)){ $error[8]=1; } else{ $error[8]=0; } if(empty($register_day) || !isset($register_day)){ $error[9]=1; } else{ $error[9]=0; } if(empty($register_year) || !isset($register_year)){ $error[10]=1; } else{ $error[10]=0; } if(empty($register_membership_type) || !isset($register_membership_type)){ $error[11]=1; } else{ $error[11]=0; } if(empty($register_submit_button) || !isset($register_submit_button)){ $error[12]=1; } else{ $error[12]=0; } if($register_email!=$register_check_email){ $error[13]=1; } else{ $error[13]=0; } if($register_password!=$register_check_password){ $error[14]=1; } else{ $error[14]=0; } if($error!=="000000000000000"){ $_SESSION['error']=$error; header("Location: redirect back to form"); } Would there be some way to simply this to a function or a class?
-
I'm just hard coding the username and password into each function. It just seems like a waste to include a file into each function every time a new connection needs to be established. If I ever decide to change the username and password I need to update it in 4 functions; but, oh well.
-
Yes, I'm perfectly aware of that. And that's what I'm trying to avoid. I'm going to be coding with a team of coders, and I don't want them to know the MySQL Username or Password. Thus I made this function so they would only need to know the Database name. You're the one that told me to pass the variables in the argument (instead of using global), and I see no way of doing that.
-
That's what I posted in my last post: This is line 53: class mysqli_errordisplay extends mysqli { this is line 71: return $DBconnect; The php file calling the function is simply this right now: <?php include 'db_select.php'; MysqliCOE("db_name"); ?>
-
Yep, that's pretty much exactly what I had Before I switch it to global. I used global after trying it that way. In the example I posted, I forgot to remove the globals. I just copied what I currently have and changed the function argument. Here's the function with variables: $DBusername="user"; $DBpassword="password"; class mysqli_errordisplay extends mysqli { public function __construct($host, $user, $pass, $db) { parent::__construct($host, $user, $pass, $db); if (mysqli_connect_error()) { die('Connect Error (' . mysqli_connect_errno() . ') ' . mysqli_connect_error()); } } } function MysqliPersist($dbname, $DBusername, $DBpassword){ $DBconnect = new mysqli_errordisplay('p:localhost', $DBusername, $DBpassword, $dbname); return $DBconnect; } function MysqliCOE($dbname, $DBusername, $DBpassword){ $DBconnect = new mysqli_errordisplay('localhost', $DBusername, $DBpassword, $dbname); return $DBconnect; } This is what I get in return: Warning: Missing argument 2 for MysqliCOE(), called in /home/zyquo/public_html/beta/test.php on line 3 and defined in /home/zyquo/public_html/beta/db_select.php on line 69 Warning: Missing argument 3 for MysqliCOE(), called in /home/zyquo/public_html/beta/test.php on line 3 and defined in /home/zyquo/public_html/beta/db_select.php on line 69 Warning: mysqli::mysqli() [mysqli.mysqli]: (28000/1045): Access denied for user 'root'@'localhost' (using password: NO) in /home/zyquo/public_html/beta/db_select.php on line 55 Connect Error (1045) Access denied for user 'root'@'localhost' (using password: NO)
-
I tried that and it didn't work. function MysqliPersist($dbname, $DBUsername, $DBpassword){ global $DBusername, $DBpassword; $DBconnect = new mysqli_errordisplay('p:localhost', $DBusername, $DBpassword, $dbname); return $DBconnect; } Gives me an error when I use MysqliPersist("some_db_name"); in the actual page. It says something along the lines of argument 2 and 3 are invalid or missing. The whole point of the function is so the other coders on the team don't need to know the mysql login details. Is there another way to pass the login details to the argument? I also tried: function MysqliPersist($dbname, $DBUsername=$DBusername, $DBpassword=$DBpassword){ global $DBusername, $DBpassword; $DBconnect = new mysqli_errordisplay('p:localhost', $DBusername, $DBpassword, $dbname); return $DBconnect; }
-
Alright, solved with setting the variables to global. Now I'm getting the following warning: Warning: Unexpected character in input: ''' (ASCII=39) state=1 in /home/zyquo/public_html/beta/db_select.php on line 71 Line 71: $DBconnect = new mysqli_errordisplay('localhost', $DBusername, $DBpassword, $dbname)'; full code: class mysqli_errordisplay extends mysqli { public function __construct($host, $user, $pass, $db) { parent::__construct($host, $user, $pass, $db); if (mysqli_connect_error()) { die('Connect Error (' . mysqli_connect_errno() . ') ' . mysqli_connect_error()); } } } function MysqliPersist($dbname){ global $DBusername, $DBpassword; $DBconnect = new mysqli_errordisplay('p:localhost', $DBusername, $DBpassword, $dbname); return $DBconnect; } function MysqliCOE($dbname){ global $DBusername, $DBpassword; $DBconnect = new mysqli_errordisplay('localhost', $DBusername, $DBpassword, $dbname)'; } EDIT: Crap. Had a quote on the end. I seem to always solve my own posts...
-
The below is giving me the following: Warning: mysqli::mysqli() [mysqli.mysqli]: (28000/1045): Access denied for user 'root'@'localhost' (using password: NO) in /home/zyquo/public_html/beta/db_select.php on line 53 Connect Error (1045) Access denied for user 'root'@'localhost' (using password: NO) $DBUsername and $DBPassword are set at the top of the file and they're correct. class mysqli_errordisplay extends mysqli { public function __construct($host, $user, $pass, $db) { parent::__construct($host, $user, $pass, $db); if (mysqli_connect_error()) { die('Connect Error (' . mysqli_connect_errno() . ') ' . mysqli_connect_error()); } } } function MysqliPersist($dbname){ $DBconnect = new mysqli_errordisplay('p:localhost', $DBusername, $DBpassword, $dbname); return $DBconnect; } function MysqliCOE($dbname){ $DBconnect = new mysqli_errordisplay('localhost', $DBusername, $DBpassword, $dbname); return $DBconnect; }
-
This is the first time I wrote a class, so I want to make sure this is right. Under "class SafePDO_errordisplay extends SafePDO" am I using "parent::__construct($dsn, $username, $password, $driver_options);" correctly? It should be sending the connection data to SafePDO to start the connection. The errordisplay class is there to catch any error on the connection. class SafePDO extends PDO { public static function exception_handler($exception) { // Output the exception details die('Uncaught exception: '. $exception->getMessage()); } public function __construct($dsn, $username='', $password='', $driver_options=array()) { // Temporarily change the PHP exception handler while we . . . set_exception_handler(array(__CLASS__, 'exception_handler')); // . . . create a PDO object parent::__construct($dsn, $username, $password, $driver_options); // Change the exception handler back to whatever it was before restore_exception_handler(); } } class SafePDO_errordisplay extends SafePDO { public function connect_db($dsn, $username='', $password='', $driver_options=array()){ parent::__construct($dsn, $username, $password, $driver_options); try { $DB = new SafePDO($dsn, $user, $password, $driver_options); } catch (PDOException $e) { echo 'Connection failed: ' . $e->getMessage(); } } } // Connect to the database - So my other coders only need to know the dbname to connect, not the MySQL log-in details. function SafePDOPersist($dbname){ $DB = new SafePDO_errordisplay("mysql:host=localhost;dbname=$dbname", $DBusername, $DBpassword, array(PDO::ATTR_PERSISTENT => true)); } function SafePDOCOE($dbname){ $DB = new SafePDO_errordisplay("mysql:host=localhost;dbname=$dbname", $DBusername, $DBpassword); }
-
including specific data from another file
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
Using this: if($_GET['signup_type']=="music"){ $_GET['form_type']="music"; $content.= include 'signup.php'; } does work. I was just trying to use the wrong URL. I was using signup?signup_type=$form_type should have been signup/?signup_type=$form_type. Forgot the slash. -
including specific data from another file
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
solved. -
I need to pull in specific data under if/else statement in another file. The data is pulled via AJAX to create a dynamic form, but I'm also trying to pull the same data to create a static form for people without javascript enabled. So they would click a link and reload the entire page instead of a radio button and AJAX. The AJAX is trigger via signup.php?ajax&signup_type=$form_type. Right now the else statement is triggering and I am getting the base form to display. But if I add ?signup_type=music to the URL, nothing shows up other than the elements that are already hard coded into the primary page. Be sure to turn Javascript off: http://beta.area51entertainment.co/signup Here's what I'm trying to do: <noscript>'; if($_GET['signup_type']=="music"){ $form_type="music"; $content.= include 'signup.php'; } else{ $content.= include 'signup.php'; } $content.=' </noscript> Here's signup.php <?php if($form_type=="music"){ $content2.='Test'; } elseif($form_type=="film"){ $content2.='Test2'; } elseif($form_type=="business"){ $content2.='Test3'; } elseif($form_type=="other"){ $content2.='Test4 G'; } else{ $year_menu=generateBirthYearMenu(date("Y")); $day_menu=generateBirthDayMenu(null,null); $content2.='<div class="register_form_wrapper"> <p class="register_form_position"> <label for="register_email"><span class="register_form_text">Email:</span></label> <input type="email" value="'.$_SESSION['register_email'].'" name="register_email" id="register_email" class="register_form" /> </p> <p class="register_form_position"> <label for="register_fname"><span class="register_form_text">First Name:</span></label> <input type="text" value="'.$_SESSION['register_fname'].'" name="register_fname" id="register_fname" class="register_form" /> </p> <p class="register_form_position"> <label for="register_lname"><span class="register_form_text">Last Name:</span></label> <input type="text" value="'.$_SESSION['register_lname'].'" name="register_lname" id="register_lname" class="register_form" /> </p> <p class="register_form_position"> <label for="register_check_email"><span class="register_form_text">Re-enter Email:</span></label> <input type="email" value="'.$_SESSION['register_check_email'].'" name="register_check_email" id="register_check_email" class="register_form" /> </p> <p class="register_form_position"> <label for="register_password"><span class="register_form_text">New Password:</span></label> <input type="password" value="'.$_SESSION['register_password'].'" name="register_password" id="register_password" class="register_form" /> </p> <p class="register_form_position"> <label for="register_check_password"><span class="register_form_text">Re-enter Password:</span></label> <input type="password" value="'.$_SESSION['register_check_password'].'" name="register_check_password" id="register_check_password" class="register_form" /> </p> <p class="register_form_position_gender"> <label for="register_gender">Gender:</label> <select name="register_gender" id="register_gender"> <option value=""></option> <option value="Female">Female</option> <option value="Male">Male</option> </select> </p> <p class="register_form_position_birthday"> Birthday: <select name="register_month" id="register_month" onchange="sndReq(register_year.value,this.value);"> <option value="">Month</option> <option value="January">January</option> <option value="February">February</option> <option value="March">March</option> <option value="April">April</option> <option value="June">June</option> <option value="July">July</option> <option value="August">August</option> <option value="September">September</option> <option value="October">October</option> <option value="November">November</option> <option value="December">December</option> </select> <select name="register_day" id="register_day"> <option>Day</option>'."\n". $day_menu .'</select> <select name="register_year" id="register_year" onchange="sndReq(this.value,register_month.value);"> <option>Year</option>'."\n". $year_menu .'</select> </p> </div>'; } if(isset($_GET['ajax'])){ echo $content2; } return $content2; ?> I tried using a full URL with the query string directly in an include, and my webhost has that kind of include turned off.
-
setting a form selection to a php session
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
hidden div solved it. -
I need help setting a form selection to a php session. I'm generating one of my form dropdown menus dynamically, based on the selection of two other drop down menus. But I need the value selected of the generated drop down menu stored so the value be recalled when one of the two other menus change. See this page: http://beta.area51entertainment.co/signup Change the birthday setting to what ever you want. You'll see that if you set the Month, Then the Day, then the year, the day resets back to "Day" because the year menus onchange call triggers the menu to regenerate. I some how need the day selected to be stored in a session and reselected once the year or month menu changes (unless the day option selected does fit within the new menu generated. I.E; someone selects the 31, but then selects a month with only 30 days.). It maybe that I'm not doing anything with the ajax response, but since I'm triggering a PHP Session variable do I need to? I can't send the response back to the menu id tag, since that'll wipe out the menu generation AJAX since it uses the same id. Maybe send it back to a hidden div? Here's the current code: Form: elseif(isset($_GET['signup'])){ session_start(); $_SESSION['register_user']="add"; $year_menu=generateBirthYearMenu(date("Y")); $day_menu=generateBirthDayMenu(null,null); $switch="notloggedin"; $content.='<div class="register_ad_wrapper"> <div class="register_wrapper"> <form name="sign_up_type" action="./signup.php" method="post"> <input type="hidden" name="newuser" id="newuser" value="add" /> <div class="register_account_type_position"> <p class="signup_title">Sign-Up for - Step One</p> <p class="register_account_type_title">Account Type:</p> <span class="register_account_type_personal_position"><input type="radio" value="personal" name="register_account_type" id="register_account_type_personal" class="register_account_type_personal" onclick="sendReq(\'personal\');" '; if((isset($_SESSION['account_type']) && $_SESSION['account_type']=="personal") || !isset($_SESSION['account_type'])){ $content.='checked="checked" '; } $content.='/> <label for="register_account_type_personal"><span class="register_radio_text">Personal</span></label></span> <span class="register_account_type_music_position"><input type="radio" value="music" name="register_account_type" id="register_account_type_music" class="register_account_type_music" onclick="sendReq(\'music\');" '; if(isset($_SESSION['account_type']) && $_SESSION['account_type']=="music"){ $content.='checked="checked" '; } $content.='/> <label for="register_account_type_music"><span class="register_radio_text">Band/Recording Artist</span></label></span> <span class="register_account_type_film_position"><input type="radio" value="film" name="register_account_type" id="register_account_type_film" class="register_account_type_film" onclick="sendReq(\'film\');" '; if(isset($_SESSION['account_type']) && $_SESSION['account_type']=="film"){ $content.='checked="checked" '; } $content.='/> <label for="register_account_type_film"><span class="register_radio_text">Filmmaker/Actor</span></label></span> <span class="register_account_type_business_position"><input type="radio" value="business" name="register_account_type" id="register_account_type_business" class="register_account_type_business" onclick="sendReq(\'business\');" '; if(isset($_SESSION['account_type']) && $_SESSION['account_type']=="business"){ $content.='checked="checked" '; } $content.='/> <label for="register_account_type_business"><span class="register_radio_text">Business</span></label></span> <span class="register_account_type_other_position"><input type="radio" value="business" name="register_account_type" id="register_account_type_other" class="register_account_type_other" onclick="sendReq(\'other\');" '; if(isset($_SESSION['account_type']) && $_SESSION['account_type']=="other"){ $content.='checked="checked" '; } $content.='/> <label for="register_account_type_other"><span class="register_radio_text">Other</span></label></span> </div> <div id="signup_forms"> <div class="register_form_wrapper"> <p class="register_form_position"> <label for="register_email"><span class="register_form_text">Email:</span></label> <input type="email" value="'.$_SESSION['register_email'].'" name="register_email" id="register_email" class="register_form" /> </p> <p class="register_form_position"> <label for="register_fname"><span class="register_form_text">First Name:</span></label> <input type="text" value="'.$_SESSION['register_fname'].'" name="register_fname" id="register_fname" class="register_form" /> </p> <p class="register_form_position"> <label for="register_lname"><span class="register_form_text">Last Name:</span></label> <input type="text" value="'.$_SESSION['register_lname'].'" name="register_lname" id="register_lname" class="register_form" /> </p> <p class="register_form_position"> <label for="register_check_email"><span class="register_form_text">Re-enter Email:</span></label> <input type="email" value="'.$_SESSION['register_check_email'].'" name="register_check_email" id="register_check_email" class="register_form" /> </p> <p class="register_form_position"> <label for="register_password"><span class="register_form_text">New Password:</span></label> <input type="password" value="'.$_SESSION['register_password'].'" name="register_password" id="register_password" class="register_form" /> </p> <p class="register_form_position"> <label for="register_check_password"><span class="register_form_text">Re-enter Password:</span></label> <input type="password" value="'.$_SESSION['register_check_password'].'" name="register_check_password" id="register_check_password" class="register_form" /> </p> <p class="register_form_position_gender"> <label for="register_gender">Gender:</label> <select name="register_gender" id="register_gender"> <option value=""></option> <option value="Female">Female</option> <option value="Male">Male</option> </select> </p> <p class="register_form_position_birthday"> Birthday: <select name="register_month" id="register_month" onchange="sndReq(register_year.value,this.value);"> <option value="">Month</option> <option value="January">January</option> <option value="February">February</option> <option value="March">March</option> <option value="April">April</option> <option value="June">June</option> <option value="July">July</option> <option value="August">August</option> <option value="September">September</option> <option value="October">October</option> <option value="November">November</option> <option value="December">December</option> </select> <select name="register_day" id="register_day" onchange="sendRequest(this.value);"> <option>Day</option>'."\n". $day_menu .'</select> <select name="register_year" id="register_year" onchange="sndReq(this.value,register_month.value);"> <option>Year</option>'."\n". $year_menu .'</select> </p> </div> </div> <div class="register_membership_type_position"> <span class="register_membership_type_title">Membership Type:</span> <span class="register_membership_type_basic_position"><input type="radio" value="basic" name="register_membership_type" id="register_membership_type_basic" class="register_membership_type_basic" onchange="changeButton(\'basic\');" '; if((isset($_SESSION['membership_type']) && $_SESSION['membership_type']=="basic") || !isset($_SESSION['membership_type'])){ $content.='checked="checked" '; } $content.='/> <label for="register_membership_type_basic"><span class="register_radio_text">Basic</span></label></span> <span class="register_membership_type_premium_position"><input type="radio" value="premium" name="register_membership_type" id="register_membership_type_premium" class="register_membership_type_premium" onchange="changeButton(\'premium\');" '; if(isset($_SESSION['membership_type']) && $_SESSION['membership_type']=="premium"){ $content.='checked="checked" '; } $content.='/> <label for="register_membership_type_premium"><span class="register_radio_text">Premium</span></label></span> <span class="register_membership_type_platinum_position"><input type="radio" value="platinum" name="register_membership_type" id="register_membership_type_platinum" class="register_membership_type_platinum" onchange="changeButton(\'platinum\');" '; if(isset($_SESSION['membership_type']) && $_SESSION['membership_type']=="platinum"){ $content.='checked="checked" '; } $content.='/> <label for="register_membership_type_platinum"><span class="register_radio_text">Platinum</span></label></span> <span class="learn_more_position">(<a href="/signup?memberships">Learn More About Membership Types</a>)</span> </div> <div class="form_question_wrapper"> <p class="register_form_question_title">Random Question (Bot Screener):</p> <p class="register_form_question_position"> <label for="register_bot"><span class="register_form_question_text">What is the primary color of this page (starts with "G"):</span></label> <input type="text" value="'.$_SESSION['register_bot'].'" name="register_bot" id="register_bot" class="register_question_form" /> </p> </div> <p class="signup_agreement"> By clicking the sign-up button below you are agreeing to our Terms of Services and our Privacy Policy. </p> <p class="register_form_position register_submission"> <input type="submit" value="Sign Up" class="register_button_position" id="register_submit_button" name="register_submit_button" /> </p> </form> </div> <div class="ad_wrapper"> Ad </div> </div> '; } AJAX call: function requestObj() { var xmlhttp; if (window.XMLHttpRequest) {// code for IE7+, Firefox, Chrome, Opera, Safari xmlhttp=new XMLHttpRequest(); } else {// code for IE6, IE5 xmlhttp=new ActiveXObject("Microsoft.XMLHTTP"); } return xmlhttp; } var http3 = requestObj(); function sendRequest(day_selected){ http3.open('get', 'signup_sessionset.php?day_selected='+day_selected, true); http3.onreadystatechange = handleResquest; http3.send(null); } Function that generates the menu: function generateBirthDayMenu($month,$year){ session_start(); $_SESSION['month']=$month; $_SESSION['year']=$year; $day_array=array(1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20,21,22,23,24,25,26,27,28); $month_array1=array("April", "June", "September", "November"); $month_array2=array("January", "March", "May", "July", "August", "October", "December"); if($month=="February" && ($year % 4) ==0 && (($year % 100) !=0 || ($year % 400) ==0)){ array_push($day_array, "29"); } elseif(!isset($month)){ array_push($day_array, "29", "30", "31"); } else{ } foreach($month_array1 as $month_value){ if($month==$month_value){ array_push($day_array, "29", "30"); } } foreach($month_array2 as $month_value){ if($month==$month_value){ array_push($day_array, "29", "30", "31"); } } foreach($day_array as $day_item){ $day.='<option value="'.$day_item.'"'; if(isset($_SESSION['birthday_day']) && $_SESSION['birthday_day']==$day_item){ $day.=' selected="selected"'; } $day.='>'.$day_item.'</option>'."\n"; } return $day; } php page where the session should be set, but is not being set: <?php session_start(); if(isset($_GET['day_selected'])){ $_SESSION['birthday_day']=$_GET['day_selected']; } echo $_SESSION['birthday_day']; ?>
-
Form not trigger right if statement on submit
HDFilmMaker2112 replied to HDFilmMaker2112's topic in PHP Coding Help
Forgot session_start(); in the top of the signup.php page. -
I don't know if this is a PHP issue or a HTML form issue, but thought I'd post here: When I click the submit button my form, it sends the data to the right page, but it loads the else in the if/else statement. <?php require_once 'function.php'; $form_type=$_GET['signup_type']; $register_user=$_SESSION['register_user']; $newuser=$_POST['newuser']; if($register_user=="add" && $newuser=="add"){ $register_account_type=$_POST['register_account_type']; $register_email=$_POST['register_email']; $register_fname=$_POST['register_fname']; $register_lname=$_POST['register_lname']; $register_check_email=$_POST['register_check_email']; $register_password=$_POST['register_password']; $register_check_password=$_POST['register_check_password']; $register_gender=$_POST['register_gender']; $register_month=$_POST['register_month']; $register_day=$_POST['register_day']; $register_year=$_POST['register_year']; $register_membership_type=$_POST['register_membership_type']; $register_bot=$_POST['register_bot']; $register_submit_button=$_POST['register_submit_button']; echo " Register Account Type: $register_account_type <br /> Register Email: $register_email <br /> Register First Name: $register_fname <br /> Register Last Name: $register_lname <br /> Register Check Email: $register_check_email <br /> Register Password: $register_password <br /> Register Password Check: $register_check_password <br /> Register Gender: $register_gender <br /> Register Birthday: $register_month / $register_day / $register_year <br /> Register Bot: $register_bot <br /> Register Submit: $register_submit_button "; } if($form_type=="music"){ $content.='Test'; } elseif($form_type=="film"){ $content.='Test2'; } elseif($form_type=="business"){ $content.='Test3'; } elseif($form_type=="other"){ $content.='Test4 G'; } else{ //other data } echo $content; echo $_SESSION['register_user']; echo $newuser; ?> Here's the form page: elseif(isset($_GET['signup'])){ session_start(); $_SESSION['register_user']="add"; $year_menu=generateBirthYearMenu(date("Y")); $day_menu=generateBirthDayMenu(null,null); $switch="notloggedin"; $content.='<div class="register_ad_wrapper"> <div class="register_wrapper"> <form name="sign_up_type" action="./signup.php" method="post"> <input type="hidden" name="newuser" id="newuser" value="add" /> <div class="register_account_type_position"> <p class="signup_title">Sign-Up for - Step One</p> <p class="register_account_type_title">Account Type:</p> <span class="register_account_type_personal_position"><input type="radio" value="personal" name="register_account_type" id="register_account_type_personal" class="register_account_type_personal" onclick="sendReq(\'personal\');" '; if((isset($_SESSION['account_type']) && $_SESSION['account_type']=="personal") || !isset($_SESSION['account_type'])){ $content.='checked="checked" '; } $content.='/> <label for="register_account_type_personal"><span class="register_radio_text">Personal</span></label></span> <span class="register_account_type_music_position"><input type="radio" value="music" name="register_account_type" id="register_account_type_music" class="register_account_type_music" onclick="sendReq(\'music\');" '; if(isset($_SESSION['account_type']) && $_SESSION['account_type']=="music"){ $content.='checked="checked" '; } $content.='/> <label for="register_account_type_music"><span class="register_radio_text">Band/Recording Artist</span></label></span> <span class="register_account_type_film_position"><input type="radio" value="film" name="register_account_type" id="register_account_type_film" class="register_account_type_film" onclick="sendReq(\'film\');" '; if(isset($_SESSION['account_type']) && $_SESSION['account_type']=="film"){ $content.='checked="checked" '; } $content.='/> <label for="register_account_type_film"><span class="register_radio_text">Filmmaker/Actor</span></label></span> <span class="register_account_type_business_position"><input type="radio" value="business" name="register_account_type" id="register_account_type_business" class="register_account_type_business" onclick="sendReq(\'business\');" '; if(isset($_SESSION['account_type']) && $_SESSION['account_type']=="business"){ $content.='checked="checked" '; } $content.='/> <label for="register_account_type_business"><span class="register_radio_text">Business</span></label></span> <span class="register_account_type_other_position"><input type="radio" value="business" name="register_account_type" id="register_account_type_other" class="register_account_type_other" onclick="sendReq(\'other\');" '; if(isset($_SESSION['account_type']) && $_SESSION['account_type']=="other"){ $content.='checked="checked" '; } $content.='/> <label for="register_account_type_other"><span class="register_radio_text">Other</span></label></span> </div> <div id="signup_forms"> <div class="register_form_wrapper"> <p class="register_form_position"> <label for="register_email"><span class="register_form_text">Email:</span></label> <input type="email" value="'.$_SESSION['register_email'].'" name="register_email" id="register_email" class="register_form" /> </p> <p class="register_form_position"> <label for="register_fname"><span class="register_form_text">First Name:</span></label> <input type="text" value="'.$_SESSION['register_fname'].'" name="register_fname" id="register_fname" class="register_form" /> </p> <p class="register_form_position"> <label for="register_lname"><span class="register_form_text">Last Name:</span></label> <input type="text" value="'.$_SESSION['register_lname'].'" name="register_lname" id="register_lname" class="register_form" /> </p> <p class="register_form_position"> <label for="register_check_email"><span class="register_form_text">Re-enter Email:</span></label> <input type="email" value="'.$_SESSION['register_check_email'].'" name="register_check_email" id="register_check_email" class="register_form" /> </p> <p class="register_form_position"> <label for="register_password"><span class="register_form_text">New Password:</span></label> <input type="password" value="'.$_SESSION['register_password'].'" name="register_password" id="register_password" class="register_form" /> </p> <p class="register_form_position"> <label for="register_check_password"><span class="register_form_text">Re-enter Password:</span></label> <input type="password" value="'.$_SESSION['register_check_password'].'" name="register_check_password" id="register_check_password" class="register_form" /> </p> <p class="register_form_position_gender"> <label for="register_gender">Gender:</label> <select name="register_gender" id="register_gender"> <option value=""></option> <option value="Female">Female</option> <option value="Male">Male</option> </select> </p> <p class="register_form_position_birthday"> Birthday: <select name="register_month" id="register_month" onchange="sndReq(register_year.value,this.value);"> <option value="">Month</option> <option value="January">January</option> <option value="February">February</option> <option value="March">March</option> <option value="April">April</option> <option value="June">June</option> <option value="July">July</option> <option value="August">August</option> <option value="September">September</option> <option value="October">October</option> <option value="November">November</option> <option value="December">December</option> </select> <select name="register_day" id="register_day"> <option>Day</option>'."\n". $day_menu .'</select> <select name="register_year" id="register_year" onchange="sndReq(this.value,register_month.value);"> <option>Year</option>'."\n". $year_menu .'</select> </p> </div> </div> <div class="register_membership_type_position"> <span class="register_membership_type_title">Membership Type:</span> <span class="register_membership_type_basic_position"><input type="radio" value="basic" name="register_membership_type" id="register_membership_type_basic" class="register_membership_type_basic" onchange="changeButton(\'basic\');" '; if((isset($_SESSION['membership_type']) && $_SESSION['membership_type']=="basic") || !isset($_SESSION['membership_type'])){ $content.='checked="checked" '; } $content.='/> <label for="register_membership_type_basic"><span class="register_radio_text">Basic</span></label></span> <span class="register_membership_type_premium_position"><input type="radio" value="premium" name="register_membership_type" id="register_membership_type_premium" class="register_membership_type_premium" onchange="changeButton(\'premium\');" '; if(isset($_SESSION['membership_type']) && $_SESSION['membership_type']=="premium"){ $content.='checked="checked" '; } $content.='/> <label for="register_membership_type_premium"><span class="register_radio_text">Premium</span></label></span> <span class="register_membership_type_platinum_position"><input type="radio" value="platinum" name="register_membership_type" id="register_membership_type_platinum" class="register_membership_type_platinum" onchange="changeButton(\'platinum\');" '; if(isset($_SESSION['membership_type']) && $_SESSION['membership_type']=="platinum"){ $content.='checked="checked" '; } $content.='/> <label for="register_membership_type_platinum"><span class="register_radio_text">Platinum</span></label></span> <span class="learn_more_position">(<a href="/signup?memberships">Learn More About Membership Types</a>)</span> </div> <div class="form_question_wrapper"> <p class="register_form_question_title">Random Question (Bot Screener):</p> <p class="register_form_question_position"> <label for="register_bot"><span class="register_form_question_text">What is the primary color of this page (starts with "G"):</span></label> <input type="text" value="'.$_SESSION['register_bot'].'" name="register_bot" id="register_bot" class="register_question_form" /> </p> </div> <p class="signup_agreement"> By clicking the sign-up button below you are agreeing to our Terms of Services and our Privacy Policy. </p> <p class="register_form_position register_submission"> <input type="submit" value="Sign Up" class="register_button_position" id="register_submit_button" name="register_submit_button" /> </p> </form> </div> <div class="ad_wrapper"> Ad </div> </div> '; } $_SESSION['register_user']; and $_POST['newuser']; are both set, as I can see the values echoed out when the page loads. When I try echoing out $register_user the value is not displayed. So some how passing the session variable data to the $register_user variable is not working.
-
Works perfectly, thanks. Now is there anyway to make it so it loads "Sign Up for Basic Membership" right when the page loads? I have the button set just to "Sign Up" for users without Javascript enabled. Any way to use window.onload=changeButton(basic); (tried this and doesn't work) or something like that?
-
I'm looking to change a submit buttons text based on which radio button is checked. Here's the form data: <div class="register_membership_type_position"> <span class="register_membership_type_title">Membership Type:</span> <span class="register_membership_type_basic_position"><input type="radio" value="basic" name="register_membership_type" id="register_membership_type_basic" class="register_membership_type_basic" onchange="changeButton('basic');" checked="checked" /> <label for="register_membership_type_basic"><span class="register_radio_text">Basic</span></label></span> <span class="register_membership_type_premium_position"><input type="radio" value="premium" name="register_membership_type" id="register_membership_type_premium" class="register_membership_type_premium" onchange="changeButton('premium');" /> <label for="register_membership_type_premium"><span class="register_radio_text">Premium</span></label></span> <span class="register_membership_type_platinum_position"><input type="radio" value="platinum" name="register_membership_type" id="register_membership_type_platinum" class="register_membership_type_platinum" onchange="changeButton('platinum');" /> <label for="register_membership_type_platinum"><span class="register_radio_text">Platinum</span></label></span> <span class="learn_more_position">(<a href="/signup?memberships">Learn More About Membership Types</a>)</span> </div> <p class="register_form_position register_submission"> <input type="submit" value="Sign Up" class="register_button_position" id="submit_button" /> </p> Here's the javascript I've been trying, but haven't had much luck. function changeButton(selection) { selection.charAt(0).toUpperCase() + selection.slice(1); el = document.getElementById(submit_button); el.value = 'Sign Up for '+selection+' Membership'; }