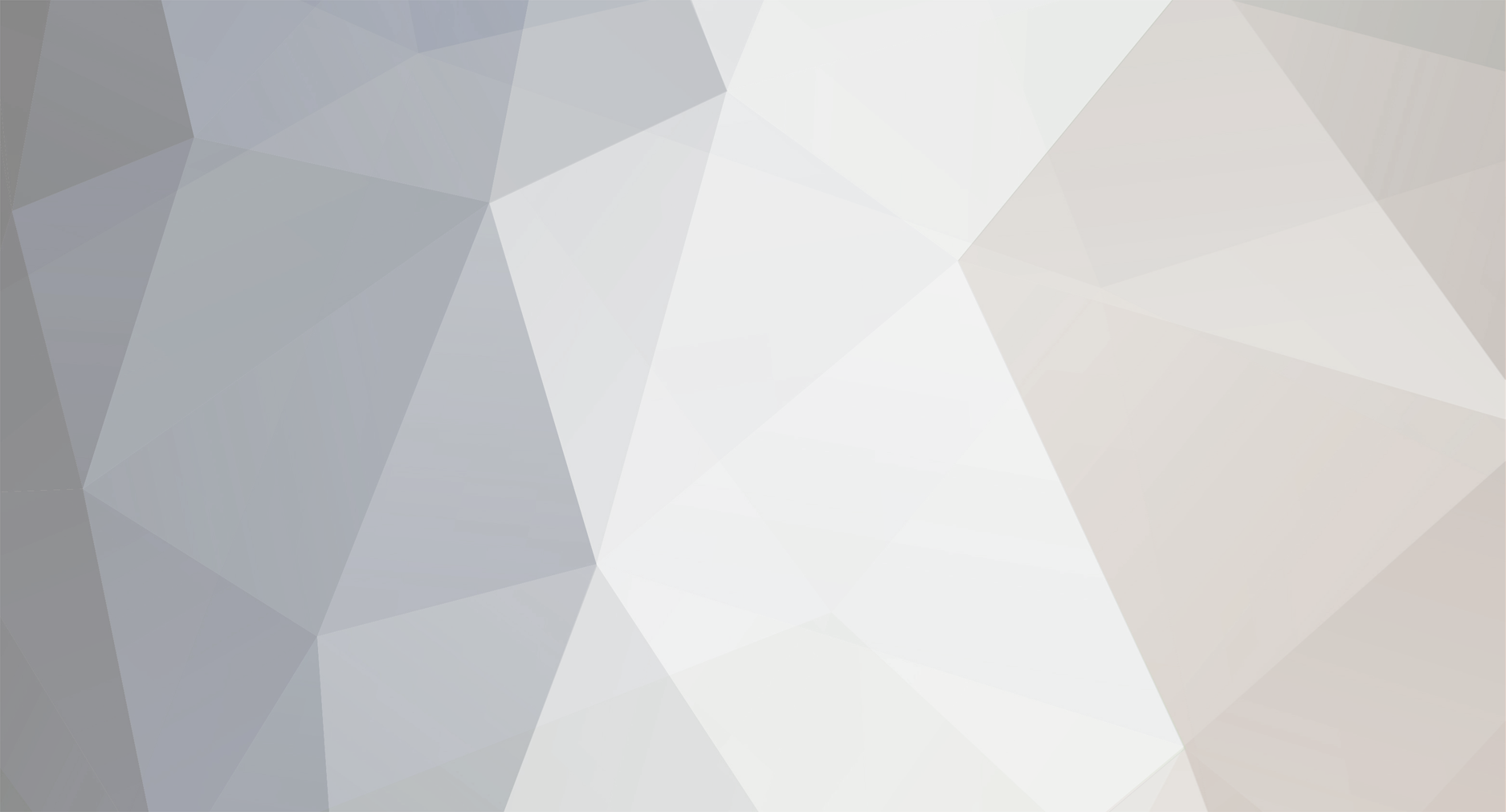
Drummin
Members-
Posts
1,004 -
Joined
-
Last visited
Everything posted by Drummin
-
Having an issue with UPDATING SQL Database via PHP Table
Drummin replied to mbb87's topic in PHP Coding Help
You are executing your query twice. $query = "UPDATE 'players' SET `block` = '$block' WHERE `pl_id` = '$pl_id'"; mysql_query($query) OR DIE .mysql_error(); -
You want to quote the "text" not the mysql_error(). $result = mysql_query($query) or die("cannot get results!" .mysql_error()); Edit: I made my own event calendar not long ago. Image attached.
-
So, how's things working? Any errors?
-
Depending on how you are saving the date in the DB you might need to change the format in the query. I would probably use YYYY-mm-dd in which case the query should be. $query = "SELECT title, DATE_FORMAT(event_date,'%Y-%m-%d') AS event_date FROM events WHERE event_date LIKE '$year-$month%'";
-
Actually, once you get things setup, you can change this line. $result = mysql_query($query) or die('cannot get results!');
-
Have you created a database and user with password for this DB with your hosting service? It's not too hard to do if you have Cpanal on your hosting service. Just click create database and give it a name. (write it down) Then create a user who has access for this DB, giving it a name and password. (write these down) These three "names" need to be added to that opening DB connection script. IF your event calendar came with a SQL file to create tables then you should be able to go to your Cpanal, select your DB and click the import tab and upload the table(s) to your DB. Otherwise you will need to create the tables adding the needed field names and types. The table is called "events" without quotes and the obvious fields would be id int(11) AUTO_INCREMENT title varchar(25) [/td] event_date varchar(10) [td] After adding the table has been added, see about running your script again. Note the script really should have come with documentation, needed files and an example.
-
You need to make a database connection. Near the top of the page add this filling in missing values. $host = "localhost"; //MySQL Database user name. $login = ""; //Password for MySQL. $dbpass = ""; //MySQL Database name. $dbcnx = ""; //Make connection to DB $oConn=mysql_connect("$host","$login","$dbpass") OR DIE ("There is a problem with the system. Please notify your system administrator." .mysql_error()); mysql_select_db($dbcnx,$oConn) OR DIE ("There is a problem with the system. Please notify your system administrator." .mysql_error());
-
Change the GET date section to this. /* date settings */ $month = (int) (isset($_GET['month']) ? $_GET['month'] : date('m')); $year = (int) (isset($_GET['year']) ? $_GET['year'] : date('Y'));
-
Writing a review script from scratch, am I doing it correctly?
Drummin replied to a topic in PHP Coding Help
Your form currently doesn't have input fields for the city etc. You need to add those in. Name: <input type="text" name="name" /><br /> Address: <input type="text" name="address" /><br /> City: <input type="text" name="city" /><br /> State: <input type="text" name="state" /><br /> Website: <input type="text" name="website" /><br /> -
Ya, my bad not escaping those inner quotes. Glad you got it working.
-
Don't use short tags, eg. <?. Use full opening tag <?php <?php //assuming variable $remember is set by session or other query $query = "SELECT shout_id, shout_photo_caption FROM myShoutout ORDER BY shout_listorder"; $result = mysql_query($query); echo '<select>'; while ($row=mysql_fetch_array($result)) { $selected =(isset($remember) && $remember==$row['shout_id'] ? ' selected="selected"' : ''); echo "<option value="{$row['shout_id']}"$selected>{$row['shout_photo_caption']}</option>"; } echo '</select>'; ?>
-
This will work as well. <?php $results = Array ( 0 => array ( 'id'=>101, 'year'=>2011, 'month'=>11, 'in'=>25 ), 2 => array ( 'id'=>105, 'year'=>2011, 'month'=>12, 'in'=>3 ) ); echo "<pre>"; print_r($results); echo "</pre>"; $search_in=25; foreach($results as $k1 => $a1){ if ($results[$k1]['in']==$search_in){ //Display each item in this array for $search_in key echo "ID = {$results[$k1]['id']}<br />"; echo "Year = {$results[$k1]['year']}<br />"; echo "Month = {$results[$k1]['month']}<br />"; echo "IN = {$results[$k1]['in']}<br />"; } } ?>
-
How To: Show Table TITLE Values; and Hide/Show DETAIL Value
Drummin replied to EmperorJazzy's topic in Javascript Help
It's not a hosting issue. I'm no expert in jQuery but I know you need required files. Read documentation, download files and make sure you link to them correctly and things should work fine. -
If you notice in my samples, I just echo the query so you can SEE that the values are as expected. It's a good practice especially when having problems to echo the query to make sure all values are as expected. You can comment out or remove that "test" echo and un comment the query execution lines so it actually should do the query.
-
Need Help (please) w redirect after form submission.
Drummin replied to Rossp2000's topic in PHP Coding Help
I am no expert in this area, so I apologize if this doesn't work. I assume the idea is to get the name of the subdomain and rebuild the path. This is what I came up with. <?php $request_method = $_SERVER["REQUEST_METHOD"]; if($request_method == "GET"){ $query_vars = $_GET; } elseif ($request_method == "POST"){ $query_vars = $_POST; } reset($query_vars); $t = date("U"); $file = $_SERVER['DOCUMENT_ROOT'] . "/../data/gdform_" . $t; $fp = fopen($file,"w"); while (list ($key, $val) = each ($query_vars)) { fputs($fp,"<GDFORM_VARIABLE NAME=$key START>\n"); fputs($fp,"$val\n"); fputs($fp,"<GDFORM_VARIABLE NAME=$key END>\n"); if ($key == "redirect") { $landing_page = $val;} } fclose($fp); //find subdomain and rebuild path $subdomain = strpos($_SERVER['HTTP_HOST'], "."); $subdomain = substr($_SERVER['HTTP_HOST'], 0, $subdomain); $path = "$subdomain/{$_SERVER['HTTP_HOST']}"; if ($landing_page != ""){ header("Location: http://$path/$landing_page"); exit; } else { header("Location: http://$path/"); exit; } ?> EDIT: My sample didn't work when I just tested it. Sorry about that. $_SERVER['HTTP_HOST'] is showing the subdomain path already so I'm not sure what the issue is with your code. I would probably use relative paths anyway. -
How To: Show Table TITLE Values; and Hide/Show DETAIL Value
Drummin replied to EmperorJazzy's topic in Javascript Help
Probably jQuery is what you need if not wishing to refresh the page. http://webcloud.se/jQuery-Collapse/ -
Well it's your html markup that really needed help. A good IDE editor will tell you when you have errors in your php. NetBeans is one option. Most of the errors I noticed had to do with nesting. For example if you want to "position" a selection box you don't put div tags around the <option> tags but rather the <selection> tags and you certainly need to watch nesting for example <b><i>word</i></b> not <b><i>word</b></i>.
-
php script just inserts one row. there is an auto increment id
Drummin replied to rahul19dj's topic in PHP Coding Help
IF you echo $query_insert_user by adding this line below setting the variable, does it show expected variables for each field? echo "$query_insert_user"; By the way, you don't need all that extra markup in there. $query_insert_user = "INSERT INTO userdtls (mobileno,pass,fname,lname,email,MUM,PUN,BNG,MYS) VALUES ('$mobile','$password','$fname','$lname','$email','$mumbai','$pune','$banglore','$mysore')"; And if you haven't fixed the "checkbox" section with this code, then all those variables may not be getting set, also causing the query to fail. Echo the query to see if all values are passed to the query. if (isset($_POST['checkbox'])){ $mumbai=(in_array("mumbai",$_POST['checkbox']) ? 1 : 0); $pune=(in_array("pune",$_POST['checkbox']) ? 1 : 0); $banglore=(in_array("banglore",$_POST['checkbox']) ? 1 : 0); $mysore=(in_array(1,$_POST['checkbox']) ? 1 : 0); } -
insert the checkbox value from php form to mysql
Drummin replied to rahul19dj's topic in PHP Coding Help
Answered in your other post. http://forums.phpfreaks.com/index.php?topic=363369.msg1719607#msg1719607 if (isset($_POST['checkbox'])){ $mumbai=(in_array("mumbai",$_POST['checkbox']) ? 1 : 0); $pune=(in_array("pune",$_POST['checkbox']) ? 1 : 0); $banglore=(in_array("banglore",$_POST['checkbox']) ? 1 : 0); $mysore=(in_array(1,$_POST['checkbox']) ? 1 : 0); } -
I mentioned a few and also told you how you can see these for yourself by getting a validator add-on for firefox.
-
php script just inserts one row. there is an auto increment id
Drummin replied to rahul19dj's topic in PHP Coding Help
Besides dealing with your current issue with the query failing (more than likely due to $_POST['mobileno']), the value $_POST['checkbox'] is an array and needs to be handled as such. This should work. if (isset($_POST['checkbox'])){ $mumbai=(in_array("mumbai",$_POST['checkbox']) ? 1 : 0); $pune=(in_array("pune",$_POST['checkbox']) ? 1 : 0); $banglore=(in_array("banglore",$_POST['checkbox']) ? 1 : 0); $mysore=(in_array(1,$_POST['checkbox']) ? 1 : 0); } -
And with the str_replace() and strtolower() option as suggested several times. <?php include "access.php"; if(isset($_POST['category'])){ $_POST['category'] = trim($_POST['category']); if(!empty($_POST['category'])) { $error = "Please select a category."; } if(!isset($error)) { $category = mysql_real_escape_string($categories[$_POST['category']]); $linkcategory = str_replace(' ', '-',strtolower($_POST['category']); $linkcategory = mysql_real_escape_string($linkcategory); $sql = "INSERT INTO organiserdbase (category, linkcategory) VALUES ('$category','$linkcategory')"; echo "$sql"; /* $query = mysql_query($sql); if($query) { } else { $error = "There was a problem with the submission. Please try again."; } */ } } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=windows-1252" /> <title></title> </head> <body> <div id="registerpagecell"> <div id="registerpageheaderorganiser"> Create Your Organiser Profile </div> <div id="registerform"> <form id="form_id" name="form_id" class="appnitro" method="post" action=""> <?php if(isset($error)){ echo "<span style=\"color:#ff0000;\">".$error."</span><br /><br />";}?> <div id="forminputcell"> <div id="forminputleft"> <label class="description" for="element_3">Your Job Role:</label> </div> <div id="forminputright"> <select class="element select medium" name="category" id="category" > <option value="" selected="selected">Venue:</option> <option value="Event Manager" >Event Manager</option> <option value="Event Managent Courses" >Event Managent Courses</option> <option value="Event Management Software" >Event Management Software</option> <option value="Entertainment Staff" >Entertainment Staff</option> </select> </div> </div> <input type="submit" value="Submit" /> </form> </div> </div> </body> </html>
-
Yes, even in the few lines of form code you've posted there are many errors. <div> within <ul> and "nesting" issues with </div> within <select> tags etc. Get tidy validator for firefox or another validator to check your code. Another issue is the <select> tag is not named. Any input field should be uniquely named. I'm sure it's been mentioned that creating an array would solve many of the issues you are trying to answer in having both a category name and link value. You can then easily check posted value against this array and use the array key to get the name. Here's a sample. <?php include "access.php"; $categories = array("reception-room"=>"Reception Room","hotel"=>"Hotel","night-club"=>"Night Club"); if(isset($_POST['category'])){ $_POST['category'] = trim($_POST['category']); if(!array_key_exists($_POST['category'],$categories)) { $error = "Please select a category."; } if(!isset($error)) { $category = mysql_real_escape_string($categories[$_POST['category']]); $linkcategory = mysql_real_escape_string($_POST['category']); $sql = "INSERT INTO organiserdbase (category, linkcategory) VALUES ('$category','$linkcategory')"; echo "$sql"; /* $query = mysql_query($sql); if($query) { } else { $error = "There was a problem with the submission. Please try again."; } */ } } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=windows-1252" /> <title></title> </head> <body> <div id="registerpagecell"> <div id="registerpageheaderorganiser"> Create Your Organiser Profile </div> <div id="registerform"> <form id="form_id" name="form_id" class="appnitro" method="post" action=""> <?php if(isset($error)){ echo "<span style=\"color:#ff0000;\">".$error."</span><br /><br />";}?> <div id="forminputcell"> <div id="forminputleft"> <label class="description" for="element_3">Your Job Role:</label> </div> <div id="forminputright"> <select class="element select medium" name="category" id="category" > <option value="" selected="selected">Venue:</option> <?php foreach($categories as $key => $value){ $selected=(isset($_POST['category']) && $_POST['category']==$key ? ' selected="selected"' : ''); echo "<option value=\"$key\"$selected>$value</option>"; } ?> </select> </div> </div> <input type="submit" value="Submit" /> </form> </div> </div> </body> </html>
-
OK well sure... you're setting these variables to empty values then checking to see if they are empty. Try wrapping the whole thing in an isset() statement. EDIT: Use one of your field names that's always posted if you don't have a "submit" named button. <?php if (isset($_POST['name'])){ $errors=0; $error="The following errors occured while processing your form input."; $name= isset($_POST['name']) ? $_POST['name'] : ''; $company= isset($_POST['company']) ? $_POST['company'] : ''; $telephone=isset($_POST['telephone']) ? $_POST['telephone'] : ''; $email=isset($_POST['email']) ? $_POST['email'] : ''; $message=isset($_POST['message']) ? $_POST['message'] : ''; if(empty($name) || empty($company) || empty($telephone) || empty($email) || empty($message)){ $errors=1; $error.="You did not complete one or more fields, please go back and try again!"; } if($errors==1) echo $error; else{ $where_form_is="http".($HTTP_SERVER_VARS["HTTPS"]=="on"?"s":"")."://".$SERVER_NAME.strrev(strstr(strrev($PHP_SELF),"/")); $message="Name: ".$name." Company: ".$company." Telephone: ".$telephone." E-mail: ".$email." Message: ".$message." "; $message = stripslashes($message); mail("[email protected]","Form Submitted at your website",$message,"From: xyz.com"); header('Refresh: 0; http://www.xyz.com/thanks.html'); } } ?>
-
Try adding brackets to your IF statement. if($errors==1){ echo $error; }else{ $where_form_is="http".($HTTP_SERVER_VARS["HTTPS"]=="on"?"s":"")."://".$SERVER_NAME.strrev(strstr(strrev($PHP_SELF),"/")); $message="Name: ".$name." Company: ".$company." Telephone: ".$telephone." E-mail: ".$email." Message: ".$message." "; $message = stripslashes($message); mail("[email protected]","Form Submitted at your website",$message,"From: xyz.com"); header('Refresh: 0; http://www.xyz.com/thanks.html'); } Also the variable $errors don't need to be changed to an array, but could be done that way as darkfreaks suggested, but then you would need to use a foreach statement to display it to the user. Also you don't usually echo an array variable while setting the value.