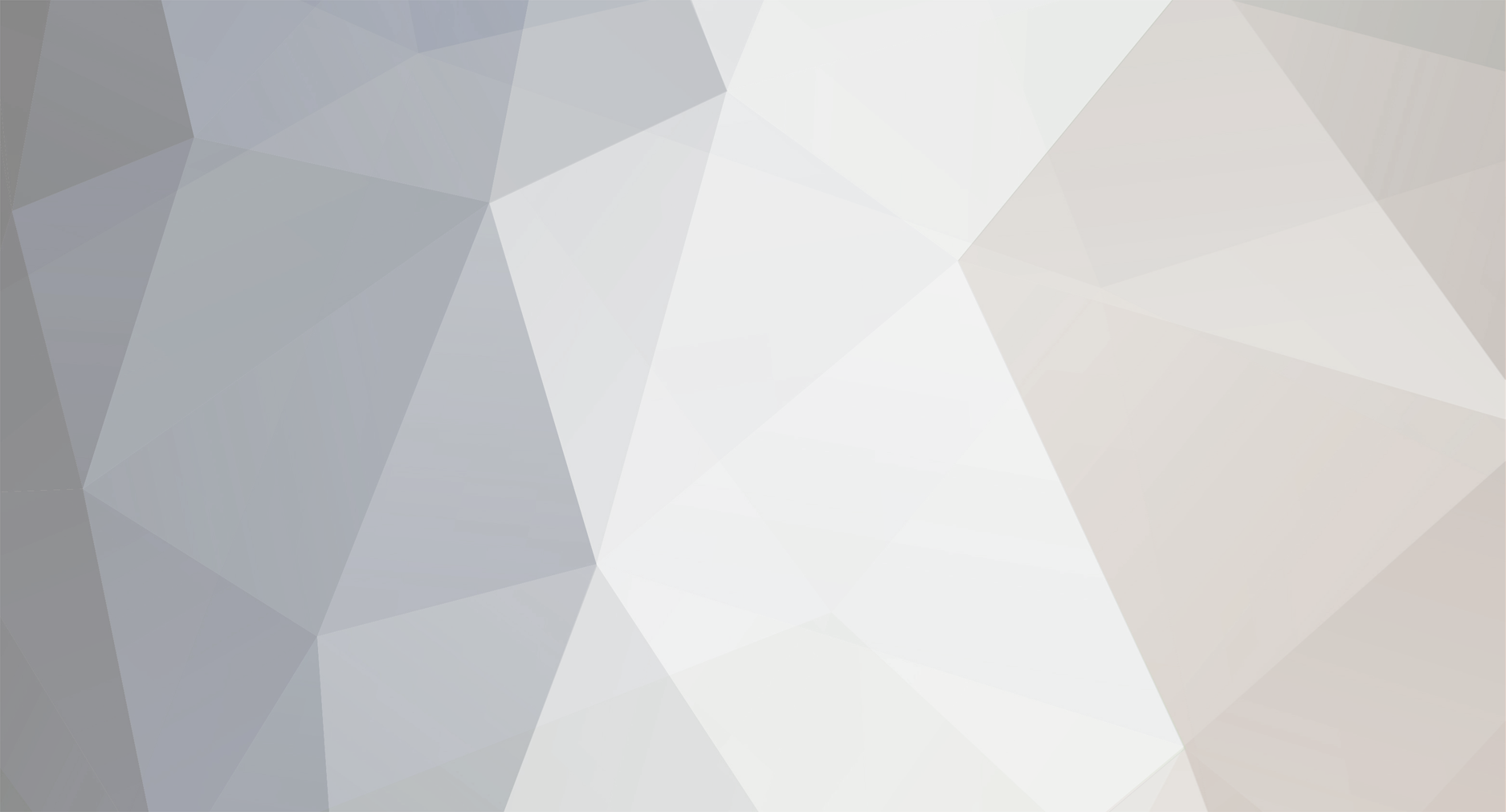
Drummin
Members-
Posts
1,004 -
Joined
-
Last visited
Everything posted by Drummin
-
... OK, you said the `image_path` is in categorys table. Now if you don't need the `area_name`, then just remove that one field from the query. $sql= "SELECT p.id, p.location_id, p.catagory_id, p.type, p.bedrooms, p.bathrooms, p.receptions, p.parking, p.garden, p.market_type, p.asking_price, p.pay_interval, p.url, p.summary, p.full_description, c.image_path FROM property as p "; $sql .= "LEFT JOIN catagorys AS c "; $sql .= "ON "; $sql .= "(c.property_id = p.id) "; $sql .= "WHERE p.market_type='sale'";
-
Double check each of these fields against your table field names. The query is failing because something doesn't match. p.id, p.location_id, p.catagory_id, p.type, p.bedrooms, p.bathrooms, p.receptions, p.parking, p.garden, p.market_type, p.asking_price, p.pay_interval, p.url, p.summary, p.full_description, c.image_path, c.area_name I suspect it's `area_name` from `categorys` but that's just a guess.
-
Just wrapping the insert in a simple IF statement should do the job. <?php function insert_tel_order(){ $lastname = $_POST['lastname']; $orderid = strtoupper(substr($lastname,0,3)).(substr(time(),-4)); //Creates a unique order number $customerid = $_POST['customerid']; $pickupdate = $_POST['pickupdate']; $deliverylocationid = $_POST['deliverylocationid']; $orderdate = $_POST['orderdate']; foreach ($_POST['prodid'] as $arrkey => $prodid){ $quantity = $_POST['quantity'][$arrkey]; $prodid = $_POST['prodid'][$arrkey]; $price = $_POST['price'][$arrkey]; $ordervalue = $quantity * $price; if ($quantity>=1){ $sql =" INSERT INTO `order` (id, orderid,orderdate,customerid, deliverylocationid,pickupdate,prodid,price, quantity, ordervalue, status) VALUES ('', '$orderid',$orderdate,$customerid,$deliverylocationid,$pickupdate,$prodid,$price,$quantity,$ordervalue,'')"; $this->db->query($sql); } } } ?>
-
One more copy of your full page based on view source code of your page. Adjust as needed. <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>Mumtaz Properties</title> <link type="text/css" rel="stylesheet" href="http://www.mumtazproperties.hostei.com/cutouts/style.css"/> </head> <body> <!--Main Div Tag--> <div id="wrapper"> <div id="header"> <div id="logo"><a href="index.php"><img src="http://www.mumtazproperties.hostei.com/cutouts/Homepage/logo.png" alt=""/></a></div> </div> <div id="navigation"> <a id="Home" href="index.php" title="home"><span>home</span></a> <a id="Sale" href="forsale.php" title="for sale"><span>for sale</span></a> <a id="Rent" href="forrent.php" title="for rent"><span>for rent</span></a> <a id="Contact" href="contact.html" title="contact us"><span>contact us</span></a> </div> <div id="main"> <table border="0" cellpadding="0" cellspacing="0"> <tr> <td> <div id="filter"><p class="houses" style="font-family:helvetica;color:#0155a1;font-size:14px;background:url(http://www.mumtazproperties.hostei.com/cutouts/forsale/filter.jpg) no-repeat;"><a href="forsale.php"><b><u>All</u></b></a> <br /> <a href="forsale.php?type=detached"><span class="dh"><b><u>Detached Houses</u></b></span></a> <br /> <a href="forsale.php?type=semi-detached"><span class="dh"><b><u>Semi-detached houses</u></b></span></a> <br /> <a href="forsale.php?type=terraced"><span class="dh"><b><u>Terraced houses</u></b></span></a> <br /> <br /> <a href="forsale.php?type=flats"><span class="dh"><b><u>Flats / Apartments</u></b></span></a> </p></div> </td> <td> <?php $host = "localhost"; //MySQL Database user name. $login = ""; //Password for MySQL. $dbpass = ""; //MySQL Database name. $db = ""; //Make connection to DB try { $dbh = new PDO("mysql:host=localhost;dbname=$db", $login, $dbpass); } catch (PDOException $e) { print "Error!: " . $e->getMessage() . "<br/>"; die(); } //Make query. Adjust table/field names as needed. //Basic query for properies for sale $sql= "SELECT p.id, p.location_id, p.catagory_id, p.type, p.bedrooms, p.bathrooms, p.receptions, p.parking, p.garden, p.market_type, p.asking_price, p.pay_interval, p.url, p.summary, p.full_description, c.image_path, c.area_name FROM property as p "; $sql .= "LEFT JOIN catagorys AS c "; $sql .= "ON "; $sql .= "(c.property_id = p.id) "; $sql .= "WHERE p.market_type='sale'"; //Make array of filter allowed types $types=array("detached","semi-detached","terraced","flats"); //Check for GET and if allowed type if(isset($_GET['type']) && in_array($_GET['type'], $types)){ //Add filter type to our query $sql .="AND p.type='{$_GET['type']}'"; } //Add order by $sql .=" ORDER BY p.id"; //execute query $result = $dbh->query($sql); //get result count $resultcount = $result->rowCount(); //check count for no results if ($resultcount < 1){ echo '<div class="error">Sorry, No Results Match Your Search.</div>'; } while($row = $result->fetch(PDO::FETCH_BOTH)){ echo '<div class="container" style="float:left;">'; echo '<div class="imageholder" style="float:left;">'; echo "<a href='{$row['url']}'><img class='image1' src='{$row['image_path']}' alt='{$row['summary']}' /></a> <br />"; echo '</div>'; echo '<div class="textholder" style="font-family:helvetica; font-size:14px; float:left; padding-top:10px;">'; echo "{$row['summary']}"; echo "<span style=\"color:#63be21;\"><br /><br /><b>{$row['bedrooms']} bedroom(s) {$row['bathrooms']} bathroom(s) {$row['receptions']} reception room(s)</b></span>"; if($row['parking'] != null){ echo "<span style=\"color:#63be21;\"><b> {$row['parking']} parking space(s)</b></span>"; echo '<div class="sline"><img src="cutouts/search/sline.png" alt=""/></div>'; } echo '</div>'; echo '<div style="clear:both"></div>'; echo '</div>'; } ?></td> </tr> </table> <div id="footer"> <a id="fHome" href="index.php" title="homepage"><span>homepage</span></a> <a id="fSale" href="forsale.html" title="for sale"><span>for sale</span></a> <a id="fRent" href="forrent.html" title="for rent"><span>for rent</span></a> <a id="fContact" href="contact.html" title="contact us"><span>contact us</span></a> </div> </div><!-- main --> </div><!-- wrapper --> <!-- www.000webhost.com Analytics Code --> <script type="text/javascript" src="http://stats.hosting24.com/count.php"></script> <noscript><a href="http://www.hosting24.com/"><img src="http://stats.hosting24.com/count.php" alt="web hosting" /></a></noscript> <!-- End Of Analytics Code --> </body> </html>
-
Here's a full updated copy, that works on my test db. <?php $host = "localhost"; //MySQL Database user name. $login = ""; //Password for MySQL. $dbpass = ""; //MySQL Database name. $db = ""; //Make connection to DB try { $dbh = new PDO("mysql:host=localhost;dbname=$db", $login, $dbpass); } catch (PDOException $e) { print "Error!: " . $e->getMessage() . "<br/>"; die(); } //Make query. Adjust table/field names as needed. //Basic query for properies for sale $sql= "SELECT p.id, p.location_id, p.catagory_id, p.type, p.bedrooms, p.bathrooms, p.receptions, p.parking, p.garden, p.market_type, p.asking_price, p.pay_interval, p.url, p.summary, p.full_description, c.image_path, c.area_name FROM property as p "; $sql .= "LEFT JOIN catagorys AS c "; $sql .= "ON "; $sql .= "(c.property_id = p.id) "; $sql .= "WHERE p.market_type='sale'"; //Make array of filter allowed types $types=array("detached","semi-detached","terraced","flats"); //Check for GET and if allowed type if(isset($_GET['type']) && in_array($_GET['type'], $types)){ //Add filter type to our query $sql .="p.type='{$_GET['type']}'"; } //Add order by $sql .=" ORDER BY p.id"; //execute query $result = $dbh->query($sql); //get result count $resultcount = $result->rowCount(); //check count for no results if ($resultcount < 1){ echo '<div class="error">Sorry, No Results Match Your Search.</div>'; } while($row = $result->fetch(PDO::FETCH_BOTH)){ echo '<div class="container" style="float:left;">'; echo '<div class="imageholder" style="float:left;">'; echo "<a href='{$row['url']}'><img class='image1' src='{$row['image_path']}' alt='{$row['summary']}' /></a> <br />"; echo '</div>'; echo '<div class="textholder" style="font-family:helvetica; font-size:14px; float:left; padding-top:10px;">'; echo "{$row['summary']}"; echo "<span style=\"color:#63be21;\"><br><br><b>{$row['bedrooms']} bedroom(s) {$row['bathrooms']} bathroom(s) {$row['receptions']} reception room(s)</b></span>"; if($row['parking'] != null){ echo "<span style=\"color:#63be21;\"><b> {$row['parking']} parking space(s)</b></span>"; echo '<div class="sline"><img src="cutouts/search/sline.png" alt=""/></div>'; } echo '</div>'; echo '<div style="clear:both"></div>'; } ?>
-
Being used to mysql I can't imagine querying the entire DB without defining the table and field names. See if this is closer. Let me know what errors you get. Note only replaing the upper section of the query. $sql= "SELECT p.id, p.location_id, p.catagory_id, p.type, p.bedrooms, p.bathrooms, p.receptions, p.parking, p.garden, p.market_type, p.asking_price, p.pay_interval, p.url, p.summary, p.full_description, c.image_path, c.area_name FROM property as p "; $sql .= "LEFT JOIN catagorys AS c "; $sql .= "ON "; $sql .= "(c.property_id = p.id) "; $sql .= "WHERE p.market_type='For Sale'"; IF you get an error like below, a table field in the query doesn't match DB field name. Fatal error: Call to a member function rowCount() on a non-object in /home/a2221438/public_html/forsale.php on line 102
-
Yes, sorry about that. Needs to be more like this. p.id, p.location_id, p.catagory_id, p.type, p.bedrooms, p.bathrooms, p.receptions, p.parking, p.garden, p.market_type, p.asking_price, p.pay_interval, p.summary, p.full_description, c.image_path, c.area_name Be back in 30 minutes. Hope you get it working.
-
See about modifying this section of the code. Again note I used property_id to identify table row in categorys. Change if needed. //Basic query for properies for sale $sql= "SELECT * FROM property as p "; $sql .= "LEFT JOIN catagorys AS c "; $sql .= "ON "; $sql .= "(c.property_id = p.id) "; $sql .= "WHERE p.market_type='For Sale'"; //Make array of filter allowed types $types=array("detached","semi-detached","terraced","flats"); //Check for GET and if allowed type if(isset($_GET['type']) && in_array($_GET['type'], $types)){ //Add filter type to our query $sql .="p.type='{$_GET['type']}'"; } //Add order by $sql .=" ORDER BY p.id";
-
So table `property` doesn't hold `image_path`. What table holds this?
-
...And from the same table `property`? Looks like you're getting $row['summary'], so it seems odd you're not getting $row['image_path'].
-
Is `image_path` the name for your field?
-
I see you still have body tag within <tr> tag. <body onload="load()" onunload="GUnload()"> Fix this.
-
What's the URL of your test page? When viewing source can you see the image path?
-
Does the field `image_path` have the correct value?
-
Check all the field names and variables so they match what you have. market_type='For Sale' Maybe is market_type='sale' Not sure exactly what you're using. Also it doesn't look like you've updated your search links. <td> <div id="filter"><p class="houses" style="font-family:helvetica;color:#0155a1;font-size:14px;background:url(http://www.mumtazproperties.hostei.com/cutouts/forsale/filter.jpg) no-repeat;"><a href="forsale.html"><b><u>All</u></b></a> <br /> <a href="forsale.php?type=detached"><span class="dh"><b><u>Detached Houses</u></b></span></a> <br /> <a href="forsale.php?type=semi-detached"><span class="dh"><b><u>Semi-detached houses</u></b></span></a> <br /> <a href="forsale.php?type=terraced"><span class="dh"><b><u>Terraced houses</u></b></span></a> <br /> <br /> <a href="forsale.php?type=flats"><span class="dh"><b><u>Flats / Apartments</u></b></span></a> </p></div> </td>
-
Still getting used to PDO queries. See if you can make this work. Check table and field names before testing. <?php $host = "localhost"; //MySQL Database user name. $login = ""; //Password for MySQL. $dbpass = ""; //MySQL Database name. $db = ""; //Make connection to DB try { $dbh = new PDO("mysql:host=localhost;dbname=$db", $login, $dbpass); } catch (PDOException $e) { print "Error!: " . $e->getMessage() . "<br/>"; die(); } //Make query. Adjust table/field names as needed. //Basic query for properties for sale $sql= "SELECT * FROM property WHERE market_type='For Sale'"; //Make array of filter allowed types $types=array("detached","semi-detached","terraced","flats"); //Check for GET and if allowed type if(isset($_GET['type']) && in_array($_GET['type'], $types)){ //Add filter type to our query $sql .="type='{$_GET['type']}'"; } //Add order by $sql .=" ORDER BY id"; //execute query $result = $dbh->query($sql); //get result count $resultcount = $result->rowCount(); //check count for no results if ($resultcount < 1){ echo '<div class="error">Sorry, No Results Match Your Search.</div>'; } while($row = $result->fetch(PDO::FETCH_BOTH)){ echo '<div class="container" style="float:left;">'; echo '<div class="imageholder" style="float:left;">'; echo "<a href='{$row['url']}'><img class='image1' src='{$row['image_path']}' alt='{$row['summary']}' /></a> <br />"; echo '</div>'; echo '<div class="textholder" style="font-family:helvetica; font-size:14px; float:left; padding-top:10px;">'; echo "{$row['summary']}"; echo "<span style=\"color:#63be21;\"><br><br><b>{$row['bedrooms']} bedroom(s) {$row['bathrooms']} bathroom(s) {$row['receptions']} reception room(s)</b></span>"; if($row['parking'] != null){ echo "<span style=\"color:#63be21;\"><b> {$row['parking']} parking space(s)</b></span>"; echo '<div class="sline"><img src="cutouts/search/sline.png" alt=""/></div>'; } echo '</div>'; echo '<div style="clear:both"></div>'; } ?>
-
You're going to want to move this body tag out of the table. Have this one with the onload properties replace your regular <body> tag. <tr> <th><div id="key"><img src="cutouts/maps/key.jpg" alt=""/></div></th> <body onload="load()" onunload="GUnload()"> <th><div id="map" style="width: 780px; height: 480px"></div></th> </tr>
-
You're right, it is. But there is more to this than his current HTML markup. That's why I asked him to simply see if the 'Logout' text was in fact clickable as I fear, and have seen a million times before in similar cases, that the containing DIV simply has a text-decoration:none style attached to it for all hyperlinks. Might not be the case, but it needs to be ruled out for my sanity. Aside from that, I fail to see how a DIV could otherwise manipulate the content within and break links without it being controlled by javascript. I agree. It must be the css for the containing div or its parent that causing the problem. Showing relevant code, div/wrappers etc and css would shed some light on the problem.
-
Yes an obvious over site on my part when making the conversion. However <font> is an old depreciated coding style.
-
Hows it work with full quotes? <img src="images/username.png" height="20" width="20"> <b>Welcome</b> <?php echo "<span style=\"color=#9e0219;\">$username</span>"; ?>, <?php echo "<a href=\"logout.php\">Logout</a>"; ?>
-
OK. After some testing option two. $sql = "SELECT settings_value from `settings` WHERE settings_name='sitename'"; $result = $dbh->query($sql); $row = $result->fetch(PDO::FETCH_ASSOC); Sorry, just trying to get my head around this.
-
Which is a better way to make queries? $sql=$dbh->prepare("SELECT settings_value from `settings` WHERE settings_name='sitename'"); $row = $sql->fetch(PDO::FETCH_ASSOC); OR $sql = "SELECT settings_value from `settings` WHERE settings_name='sitename'"; $result = $dbh->query($sql); $row = $result->fetch(PDO::FETCH_ASSOC);
-
Can I ask your opinion? This project has 100+ pages that would need to be converted? Do you think it's worth the effort to convert to PDO? Do you think at some time, mysql will not be available?
-
Do you think it's a good Idea to wrap all results in a check like this adding a second wrapper? if ($sql == FALSE){ while ($row = $sql->fetch(PDO::FETCH_BOTH)){ } } I guess that would be NOT false. if ($sql != FALSE){ while ($row = $sql->fetch(PDO::FETCH_BOTH)){ } }