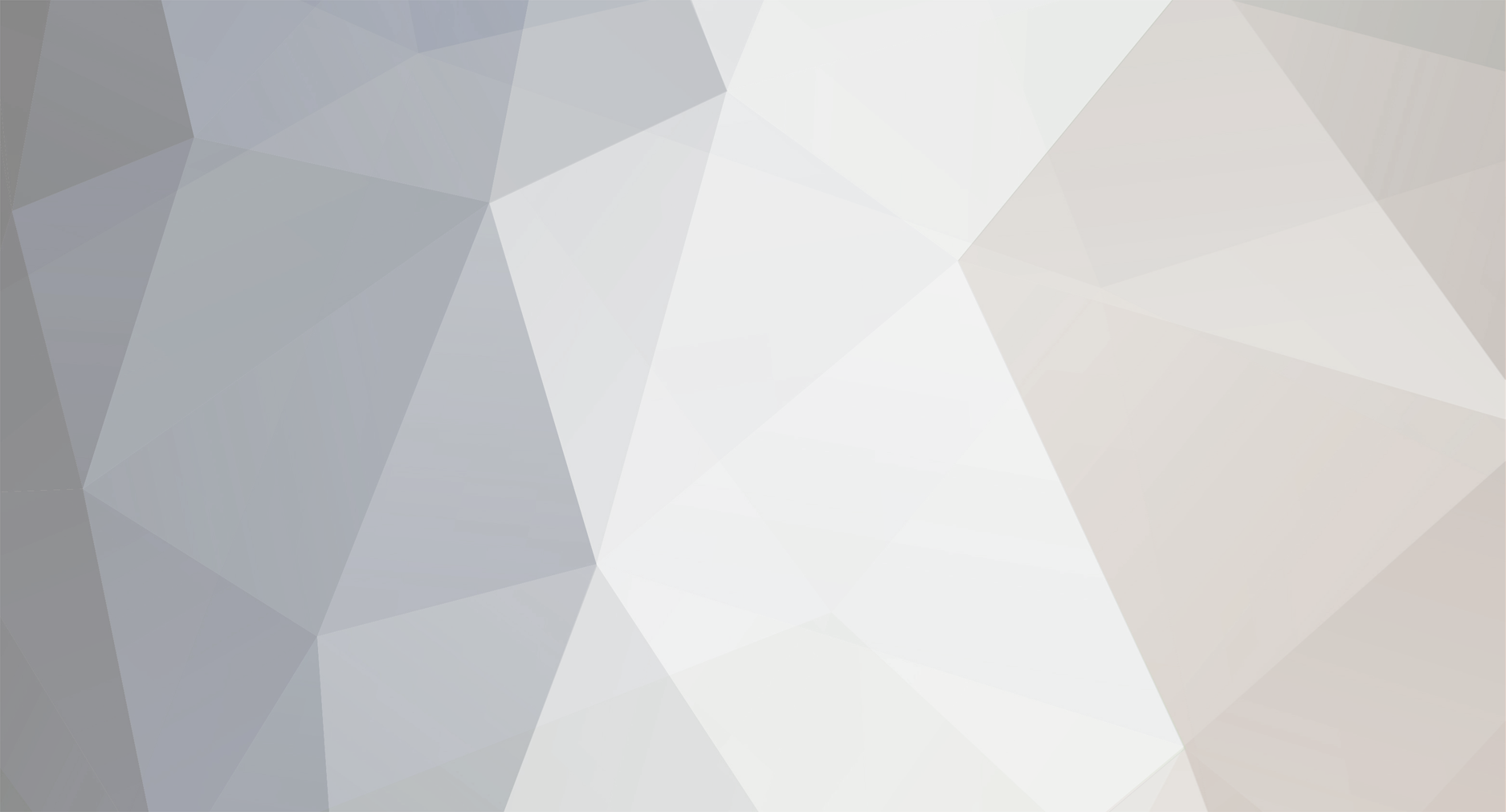
Chanpa
Members-
Posts
10 -
Joined
-
Last visited
Never
Everything posted by Chanpa
-
Hey, I'm making sort of a betting system for me and my friends and I've run into a bit of a snag. The first part of it is fairly easy it is a simple 1 x 2 betting system like this: <?php $grp_a_t1 = array("team" => "Sverige", "pts" => 0); $grp_a_t2 = array("team" => "Norge", "pts" => 0); $grp_a_t3 = array("team" => "Danmark", "pts" => 0); $grp_a_t4 = array("team" => "Finland", "pts" => 0); ?> Round 1 <?php echo $grp_a_t1["team"]." - ".$grp_a_t2["team"]; ?> <select name="grp_a_r1_m1"> <option>1</option> <option>x</option> <option>2</option> </select> <?php echo $grp_a_t3["team"]." - ".$grp_a_t4["team"]; ?> <select name="grp_a_r1_m2"> <option>1</option> <option>x</option> <option>2</option> </select> Now what I want to know how to do (if possible) is to update the $team["pts"] depending on what you select. For instance if you choose "1" in the first match(name="grp_a_r1_m1"), I want to do: $grp_a_t1["pts"] += 3; I would prefer it to update as soon as you select something, however I will settle for "at the press of a button" if it turns out it's to hard to have it update instantly. I'm pretty sure I can't make this with PHP alone, so Currently I have done this: <button id="grp_a_upd">Update this group</button> <div id="grp_a"> <?php echo $grp_a_t1["team"]." with ".$grp_a_t1["pts"]; ?><br /> <?php echo $grp_a_t2["team"]." with ".$grp_a_t2["pts"]; ?><br /> <?php echo $grp_a_t3["team"]." with ".$grp_a_t3["pts"]; ?><br /> <?php echo $grp_a_t4["team"]." with ".$grp_a_t4["pts"]; ?><br /> </div> <script language="javascript" type="text/javascript"> $('#grp_a_upd').click(function() { // Is it possible to update the variables here? }); </script> So as is infered in the code snippet above, is it possible to update php-variables with jQuery like that? If it is, how? Or do I need AJAX? Thanks, Chanpa
-
I want to use the same script to upload pictures to other places aswell. images/articles for example. So doing it the 2nd way I would add something like this at the start of the file?: if ($location != "images/slideshow"){ echo "That location isn't allowed"; exit(); } // The script And I changed from using blacklist to using a whitelist, don't even know why I used blacklist to start with. Really big thanks for all your help
-
Thanks for the reply! I updated the first post with some new info, basically that the error code for the $_FILES returned 0 which means ok. Your post really helped! I solved it so now it works. What I did was: $target_path=$_SERVER['DOCUMENT_ROOT']; $name=mysql_real_escape_string($_POST['name']); $text=mysql_real_escape_string($_POST['text']); $dbtable=mysql_real_escape_string($_POST['dbtable']); $location=mysql_real_escape_string($_POST['location']); $location.=basename($_FILES['uploadedfile']['name']); $target_path.=$location; And then changed the if-statement to use the $target_path variable. Again your post was extremely helpful. Now I want to ask you about your other pointers How do I "completely" validate it? Ok, I added this after the if(!getimagesize)-statement: $blacklist = array(".php", ".phtml", ".php3", ".php4", ".js", ".shtml", ".pl" ,".py"); foreach ($blacklist as $file){ if(preg_match("/$file\$/i", $_FILES['uploadedfile']['name'])){ echo "ERROR: That filetype is NOT allowed.\n"; exit; } } Is that sort of what you mean? Again thanks for all your input!
-
Hey, I'm creating a script so my clients can upload pictures to their site and I had it working but then I think I broke it somehow. This is what it's looks like: The Form: <form enctype='multipart/form-data' id='uploadPic' action='../php-bin/uploader.php' method='post'> Namn: <input type='text' name='name' value=''><br /> Text: <input type='text' name='text' value=''><br /> <input name='uploadedfile' type='file' /><br /> <input type='hidden' name='location' value='/images/slideshow/'> <input type='hidden' name='dbtable' value='Slideshow'>"<input type='submit' value='Submit'></form> And this is the uploader.php script: <?php include $_SERVER['DOCUMENT_ROOT']."/php-bin/var.php"; $name=mysql_real_escape_string($_POST['name']); $text=mysql_real_escape_string($_POST['text']); $dbtable=mysql_real_escape_string($_POST['dbtable']); $location=mysql_real_escape_string($_POST['location']); $location.=basename($_FILES['uploadedfile']['name']); // Check if it's an image: if (!getimagesize($_FILES['uploadedfile']['tmp_name'])){ echo "Bara bilder kan laddas upp."; exit(); } // Restrict width and height of the image is for slideshow: if($dbtable == "Slideshow"){ list($width, $height, $type, $attr) = getimagesize($_FILES['uploadedfile']['tmp_name']); if (!($width == 710)){ echo "Bilder till bildspelet måste vara exakt 710 pixlar breda. Filen laddades INTE upp."; exit(); } if (!($height == 420)){ echo "Bilder till bildspelet måste vara exakt 420 pixlar höga. Filen laddades INTE upp."; exit(); } } // The file is a picture. (With both correct height and width if its for the slideshow) // Upload it: if(move_uploaded_file($_FILES['uploadedfile']['tmp_name'], $location)){ echo "The file ". basename( $_FILES['uploadedfile']['name']). " has been uploaded"; } else { echo "Bilden laddades INTE upp.<br />". "location = $location<br />". "tmp_name = ".$_FILES['uploadedfile']['tmp_name']."<br />". "Error: ".$_FILES['uploadedfile']['error']; exit(); } $query="INSERT INTO $dbtable VALUES ('$name','$location','$text','')"; mysql_query($query) or die("Could not execute query: $query." . mysql_error()); mysql_close(); ?> The script works to a certain degree, but the picture doesn't actually upload, it goes into the last else statement; if(move_uploaded_file($_FILES['uploadedfile']['tmp_name'], $location)){ echo "The file ". basename( $_FILES['uploadedfile']['name']). " has been uploaded"; } [b]else { echo "Bilden laddades INTE upp.<br />". "location = $location<br />". "tmp_name = ".$_FILES['uploadedfile']['tmp_name']."<br />". "Error: ".$_FILES['uploadedfile']['error']; exit(); }[/b] This is what the page says when I run: So I looked up error: 0 and it found: And since the else-statement only triggers when the move fails, shouldn't this be impossible? As I said it worked awhile then it stopped, so I though I had reached max_file_uploads so I raised that from 20 to 1000 and it still doesn't work. If I try to upload other filetypes, it does into the correct if-statement and exits, same for the height and width if I try to upload for the slideshow, so those two checks are working, so the file must be uploaded to the temp location but then it doesn't get moved to the $location. Any light on this is greatly appreciated.
-
Where I read about it it didn't say anything about that. Care to explain what you mean or link to what you're talking about?
-
Hi, I am running an Apache webserver with MoWeS and I'm protecting my admin section of the site with this .htaccess file: AuthName "Admin Area" AuthType Basic AuthUserFile ../.htpasswd Require valid-user On the tutorials I read it said that the password in my .htpasswd file had to be encrypted for it to work so I did that(I used a script on the site I was learning from) and had something like: Admin:dhAJHD82zxyA in my .htpasswd file and it didn't work, the error.log said there was a user/pass mismatch. So I changed it to Admin:plaintextpass and it worked! However this is obviously less secure (although I don't have the .htpasswd -file on the webserver) so I was wondering how I can make it "accept" or work with the encrypted versions of a password. Thanks, Chanpa
-
Thanks for the reply. I made the changes you said. And the reason I don't have static login is because I plan on experimenting with multiple users with different access-levels. Thanks for the help, this topic can be closed/solved.
-
Actually the above code didn't work, I got Could not connect: Access denied for user 'www-data'@'localhost' (using password: NO) because of the mysql_real_escape_string. Anyone know why? (It works if I remove mysql_real_escape_string)
-
I solved it temporarily like this: if (isset($_GET['createDb'])) { $user = mysql_real_escape_string($_POST['user']); $pass = mysql_real_escape_string($_POST['pass']); $dbName = mysql_real_escape_string($_POST['dbName']); $test->createDb($user,$pass,$dbName); } else { echo " <form name='formCreateDb' method='post' action='?createDb'> dbName: <input type='text' name='dbName'><br> User: <input type='text' name='user'><br> Password: <input type='password' name='pass'><br> <input type='submit' value='Create DB'><br> </form>"; } But I think it's kind of ugly and I'm stil wondering if there is another way to do it.
-
Hi, I'm creating a PHP application to handle my SQL server and I've run into a bit of a problem; I have two files atm: mainClass.php and testSite.php My mainClass.php looks like this: class mainClass { private $host = 'localhost'; public function createDb($user,$pass,$dbName) { $con = mysql_connect($host, $user, $pass); if (!$con){ die('Could not connect: '.mysql_error()); } $sql = "CREATE DATABASE `$dbName`;"; if (!mysql_query($sql)){ die('Error 1: '.mysql_error()); } mysql_close(); } } and testSite.php looks like this: <!DOCTYPE HTML> <html lang="en"> <head> <meta charset="UTF-8"> </head> <body> <h1>testSite for my PHP app</h1> <?php function __autoload($className){ require_once "./classes/{$className}.php"; } $test = new mainClass(); ?> <form name='createDb' method='post' action=''> User: <input type='text' name='user'><br> Password: <input type='password' name='pass'><br> dbName: <input type='text' name='dbName'><br> <input type='submit' value='Create DB'> </form> </body> </html> What I'm asking is if it is possible to make the form-action from testSite.php run the createDb function from mainClass.php I have pretty much no idea how to do it but I tried like this: <form name='createDb' method='post' action="<?php $test->createDb($_POST['user'],$_POST['pass'],$_POST['dbName']); ?>"> User: <input type='text' name='user'><br> Password: <input type='password' name='pass'><br> dbName: <input type='text' name='dbName'><br> <input type='submit' value='Log in'> </form> But that just made the whole form disappear so now I'm completely lost, any help greatly appreciated. PS: I'm doing this to get better at PHP so please don't come with advice like "use a framework" or "there already are applications that handles this", I know there is.