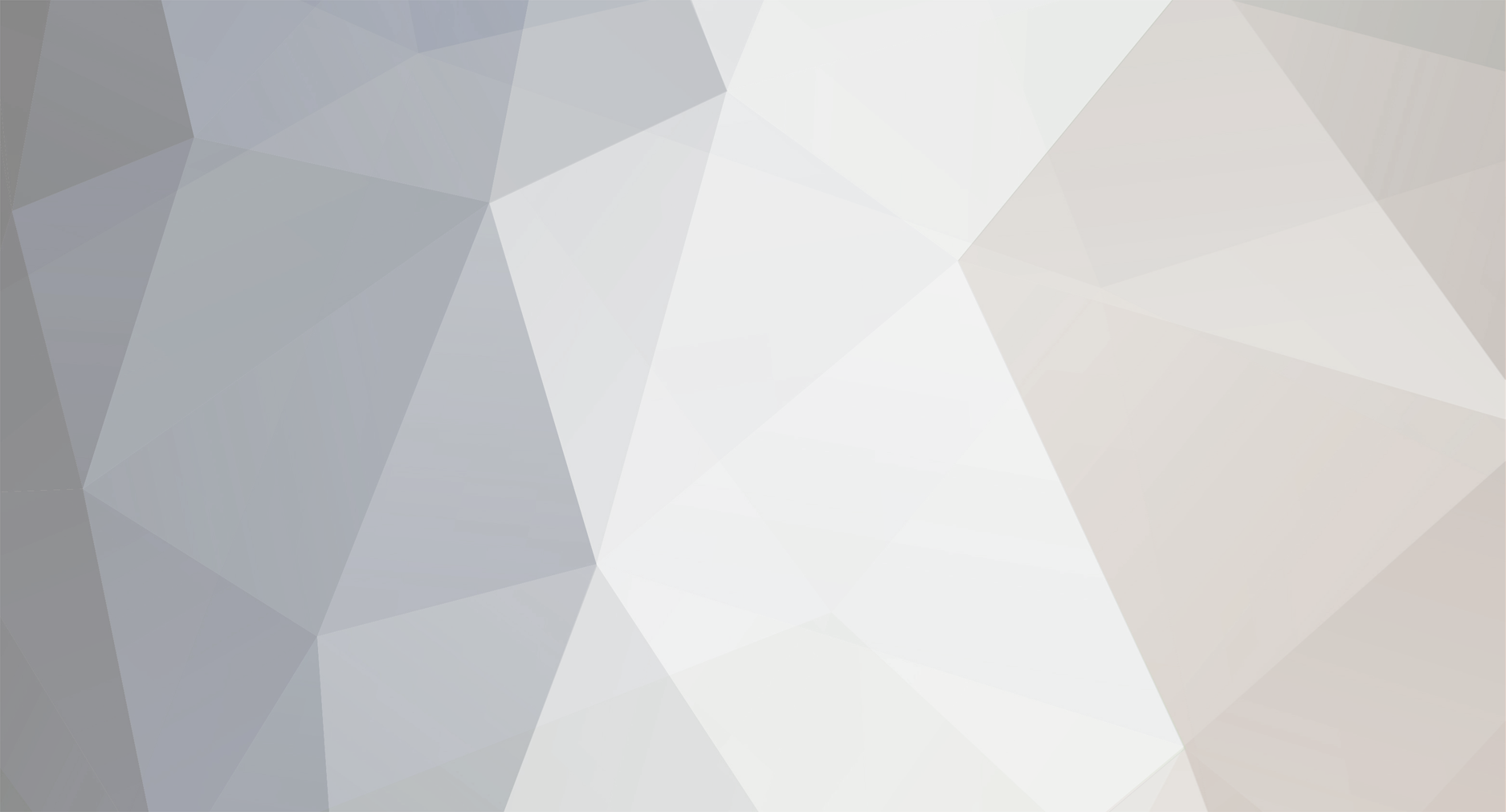
mpsn
Members-
Posts
264 -
Joined
-
Last visited
Everything posted by mpsn
-
Hi, I am building function to convert the db table to an XML file. But I want to retrieve the root node value from the FIRST row in that db table before I build a for loop to traverse that db table. Let's say I have this table NodeInfo: node_Id source target ====== ===== ===== 1 email to 2 to toFirstName pseudocode for what I want: 1) store root (the source field of table NodeInfo 2)for each entry in table NodeInfo, do: build XML END FOR-EACH Any help much appreciated!
-
Hi xyph, you're right but when I was trying to build an xml shredder using SimpleXML, I didn't know an easy way to test if the current node is text node but DOM has if($dom->nodeType=XML_TEXT_NODE). Anyways, does anyone know why it just outputs Bob, rather than the entire XML document? I though saveXML() is supposed to convert the entire XML to a string? But I do see the xml file when I View Source though. Any help much appreciated!
-
Hi, I want this output for an XML doc I create via DOM functions. <?xml version="1.0" ?> <letter> <to> <toFirstContact>Bob</toFirstContact> </to> </letter> BUT it outputs nothing to browser screen! Please see code below: <?php //TEST for REPEAT APPENDAGE $aDoc=new DOMDocument(); $root=$aDoc->createElement("letter"); //create child elem to append to root elem node $newChild1=$aDoc->createElement("to"); //append new child elem <to> to root <letter> $root->appendChild($newChild1); //make elem<toFirstContact> and also create a text, then append to <to> $toFirstContactTag=$aDoc->createElement("toFirstContact"); $textNode1=$aDoc->createTextNode("Bob"); $toFirstContactTag->appendChild($textNode1); //now append new child <toFirstContact> to <to> $newChild1->appendChild($toFirstContactTag); print $aDoc->saveXML(); ?> Any help much appreciated!
-
Ok, I got it to work!
-
As you can see above, for some reason, in browser output, it is ALMOST correct except that it is printing whatever the current node's parent, two times: Notice: Trying to get property of non-object to/message/message/email/email/#document/#document// Actually it's b/c I update $dom1=$dom1->parentNode, BUT I'm still unsure as to why it is printing #document, how do I use break once I'm at the root?
-
Now I realized I have no end condition so infinite loop, please see updated code again. <?php $pathway=array(); $strPathway=""; $dom1=$doc->getElementsByTagName("toLastName")->item(0); while($dom1->parentNode!=NULL) { $dom1=$dom1->parentNode;//NEW: update $dom1 $pathway[]=$dom1->nodeName."/".$dom1->parentNode->nodeName."/"; } $strPathway=implode("",$pathway); print $strPathway; ?> So my code ALMOST works, but I get this notice. I guess the most top level is document which HAS NO PARENTS so how do I indicate that once current node is now the actual document to stop. Any help much appreciated! Notice: Trying to get property of non-object in to/message/message/email/email/#document/#document//
-
I have changed while to: while($dom1->parentNode!=NULL) { $pathway[]=$dom->nodeName."/".$dom->parentNode->nodeName."/"; } but still no luck
-
Ok, I don't know how to use rsort, so I used array_reverse: $testArray=array("toLastName","to","message","email"); $reversedTestArray=array_reverse($testArray);//rsort returns TRUE on success $strTestArrayReversed=implode(",",$reversedTestArray); print $strTestArrayReversed; Thanks.
-
It just print: 1... Doesn't that just mean TRUE?
-
Thanks for that. I will re-code and go from there!
-
Hi, when I output this, it's same order, I want it to be: email/message/to/toLastName please see code: $testArray=array("toLastName","to","message","email"); $reversedTestArray=rsort($testArray);//rsort returns TRUE on success //$strTestArrayReversed=implode(",",$testArray); print_r($testArray); Any idea what I am doingwrong, it looks fairly straightforward. Any help much appreciated!
-
Hi, starting at a given node, I want to be able to build a pathway from that current node all the way to the root node. Let's say I have this email.xml file: <?xml version="1.0" encoding="ISO-8859-1" ?> <email> <message> <to> <toFirstName>Bob</toFirstName> <toLastName type="common">Smith</toLastName> </to> </message> </email> Here is my script which btw is infinite loop: <?php $doc=new DOMDocument(); $doc->load("email.xml"); $pathway=array(); $dom1=$doc->getElementsByTagName("toLastName")->item(0); while(!$dom1->documentElement->nodeName) { $pathway[]=$dom1->nodeName."/".$dom1->parentNode->nodeName."/"; $strPathway=implode("",$pathway); print $strPathway; } ?> The output I want is in this format: email/message/to/toLastName (I will figure out how to use rsort since in the while loop it appends as: toLastName/to/message/email Any help much appreciated!
-
Ok, let's use this simple email.xml: <?xml version="1.0" encoding="ISO-8859-1" ?> <email> <message> <to> <toFirstName>Bob</toFirstName> </to> </message> </email> For table edge: ============ edge_id source target flag ---------- -------- ------- ------ 1 email message Ref 2 message to Ref 3 to toFirstName Ref 4 toFirstName Bob Val flag refers to the current node's child ("Ref" for if child node is an element and "Val" if current node's child is a text node) I hope this is more clear. Thanks.
-
to be more specific, here is the partial code: <?php //CHECK/DEAL w/ set up for current node's source/parent $currentIdQuery=mysql_query("SELECT ");//HOW DO I GET current row's edge_id?? $nextRowQuery=mysql_query("SELECT source FROM edge WHERE edge_id>$currentIDQuery LIMIT 1"); $nextRow=mysql_fetch_assoc($nextRowQuery); if($row['target']==$nextRow['source']) { //append current row's next row as child node of current row } ?> Any help much appreciated!
-
I think I will try: $nextRow=mysql_query("SELECT source FROM edge WHERE edge_id>$currentEdge_id LIMIT 1"); but how do I get the current table edge.edge_id to store in $currentEdge_id for this comparison. I know I'll have to use mysql_fetch_assoc to retrieve the actual id BUT how do I even use the SELECT query to ask for the current edge_id I mean. Any help much appreciated!
-
Hi, I am trying to write an SQL to XML converter, so so far I have all the records inputted into the db tables, and now I want to append a child, but I don't know how to compare current row's field value with the next row below it, specifically I want to check if a current row is parent of the next row. I have already got the XML to SQL function working (some bugs but for the most part it's working) So for eg: <?php $query=mysql_query("SELECT * FROM edge"); while($row=mysql_fetch_assoc($query) ) { if($row['target'] is parent of the next row), then append the next row as child of current row } ?> Table edge is one of the tables that hold target node/source(aka: parent) nodes. Any help much appreciated!
-
Yes, I understand that, that's actually a typo, I meant searchFolders! Any help much appreciated!
-
There are two methods I know, SimpleXML (built into php core libraries) and pure DOM (I prefer DOM b/c more advanced), anyways here is snippet to give you idea what to do: $dom=new DOMDocument(); $dom->loadXML(THIS IS THE TEXT from text area when user submits);//and I think you need to link with $_POST[] to retrieve that info (loadXML will load a string as a dom document to parse) now build a loop to traverse each node starting with $dom (the root element node): function recursionTraverse($dom) { //check if attributes if($dom->hasAttributes() ), then: for($i=0;$i<$dom->attributes->length;$i++), do: print $dom->attributes->item($i) //check if text nodes (remember, white space is considered text node so use trim() to not output blank text nodes and print the //text node and element/tag node at the same time for($i=0;$i<$dom->childNodes->length;$i++), do: print $dom->childNodes->item($i)->nodeName//elem/tag node print $dom->childNodes->item($i)->nodeValue//Text node if($dom->hasChildNodes) recursionTraverse($dom->childNodes->item($i)) }END FUNCTION So something like this, the important thing to understand is that b/c of nature of an xml document, recursion is essential to traverse these kind of files!
-
Sorry I will be more clear, what I mean is that I have 4 db tables, table1 has PK, table2 has PK, table3 has PK and refs table1,table2 (so it has 2 FKs) and table4 has PK and refs table3 In short, I want to store xml to db and each node component is stored in these tables but table1 just stores the xml file name (something.xml and filepath: c:\dir\dir1\something.xml) As you can see above, I have an if($isXdocExist) that ensures just one of the something.xml is loaded b/c each time I reload the browser, the script re-enters SAME entries for table3, table4 and NOT table1, table2, so that is why I suspect is the FK causing issue. Any help much appreciated!
-
Hi, I am frustrated with getting db to store just unique entries, b/c every time I refresh the browser, the script runs and re-inserts the SAME INSERT query (but with different primary key of course which I don't want). My ques is is there a way upon the first insertion to tell it that this will be only instance of this record entered for the specified db table. So I don't want to have to encapsulate each INSERT statement in an if block to make sure that only one record exists so now I am doing it incorrectly with exit statement. please see code below: <?php $_filePath="C:\\\dir\\\email.xml"; $node=basename($_filePath); $dom=new DOMDocument(); $dom->load($node); $labelPath=array(); $connection=mysql_connect("localhost","root"); mysql_select_db("dummydpev999"); $isXdocExist=mysql_query("SELECT file_Path,file_Name FROM xdocument WHERE file_Path='$_filePath' AND file_Name='$node'"); $docId=0; if(mysql_num_rows($isXdocExist)==1) { print "Entry already exists!"; $docId=mysql_next_id("xdocument")-1; print "<br />".$docId; exit; } else { mysql_query("INSERT INTO xdocument (file_Path,file_Name) VALUES ('$_filePath','$node')"); $docId=mysql_next_id("xdocument")-1; print "<br />".$docId; } writeXMLtoDBViaDOM($dom->documentElement,$labelPath,$docId); ?> Sorry I should elaborate, I think my problem is that I have tables with foreign keys rather than all tables with just primary keys, I am unsure... Any help much appreciated.
-
Hi, I want to be able to retrieve records from db tables to build an xml file. The problem I am having is that I don't know how to retrieve a value after using foreign key to get appropriate value. You see below I want to make a new attribute to store to var $newAttr. <?php $doc=new DOMDocument(); mysql_connect("localhost","root"); mysql_select_db("xmlDB"); $edges=mysql_query("SELECT * FROM edge"); while($row=mysql_fetch_assoc($edges)) { if($row['flag']=='val') { $curValue=mysql_query("SELECT val FROM value,edge WHERE val.edge_id=edge.edge_id"); if($curRowIsAttribute=mysql_query("SELECT is_Attribute FROM value,edge WHERE value.is_Attribute='Y' AND value.edge_id=edge.edge_id")) { //create attribute node $newAttr=$dom->createAttribute();//HELP HERE PLEASE THEN I SHOULD BE ABLE TO COMPLETE NEXT TWO LINES BELOW! //set attribute value ... //append attribute to current element ... }//END IF }//END IF }//END BIG WHILE ?> Any help much appreciated!
-
My recursion is not that good, so now I get an infinite loop for the following: <?php $dir = "C:/dir1/dir2/dir3/"; function searchFolders($dir) { //FIRST get root dir and put into an array $startDir=scandir($dir); $filePathsArray=array(); foreach($startDir AS $curDir) { if(is_dir($dir)) { searchFoldersRecursively($curDir); } else if(is_file($dir)) {//BASE CASE when no more sub-dir for current dir $xmlFiles=glob($dir."*.xml"); //FIRST STORE EACH file foreach($xmlFiles AS $curXMLfile) { $filePathsArray[]=$curXMLfile; //NOW STORE EACH XML file of this current dir foreach($filePathsArray AS $_filePath) { writeXMLtoDBviaDOM($_filePath); }//END FOR-EACH LOOP store XML to DB }//END ELSE-IF }//END BIG FOR-EACH LOOP }//END FCN searchFoldersRecursively */ searchFolders($dir); ?> Any help much appreciated!
-
Hi, currently I have a function searchFolder that simply takes in ONE directory which holds all the xml files I wish to store to db via function writeXMLtoDBviaDOM. I want to make it more robust(realistic) to be able to also search complex directories (so the main directory I pass as parameter to searchFolders) in turn holds sub-directory (which in turn holds further sub-directory) which finally holds all the xml files. here is code: <?php $dir = "C:/dir/dir2/dir3/"; function searchFolders($dir) { $_filePath=""; $filePathsArray=array();//stores each file $xmlFiles = glob($dir . '*.xml'); if(is_dir($dir)) { // list files $xmlFiles = glob($dir . '*.xml'); //print each file name foreach($xmlFiles as $xmlFile) { print $xmlFile."<br />"; $filePathsArray[]=$xmlFile; } }//END BIG IF retreiving all files in dir param foreach($filePathsArray AS $curFile) { //set up db connection mysql_connect("localhost","root"); mysql_select_db("someDb"); $_filePath=$curFile; $dom=new DOMDocument(); $node=basename($_filePath);//$node is the file name only $dom->load($node); writeXMLtoDBViaDOM($dom->documentElement,$_filePath); }//END FOR-EACH LOOP for each XML file }//END FCN searchFolders VERSION 0 searchFolders($dir);//VERSION 0 ONLY searched 1 simple dir ?> Any help much appreciated!
-
Yes, I also found out about glob just now. Apparently it's good for directories with < 100 files, this is what I used: <?php $dir = "C:/xampp/htdocs/proj_ath/"; $xmlFiles = glob($dir . "*.xml"); //print each file name foreach($xmlFiles as $xmlFile) { print $xmlFile."<br />"; } ?> Thanks.
-
Hi, how do I output the total files in a given directory? Here is code I cannot get to work: <?php $file=fopen('C:\\dir\\folder\\proj_ath\\', 'a+'); for($i=0;$i<count($file);$i++) { print "tot files so far: $i <br />"; } ?> Any help much appreciated!