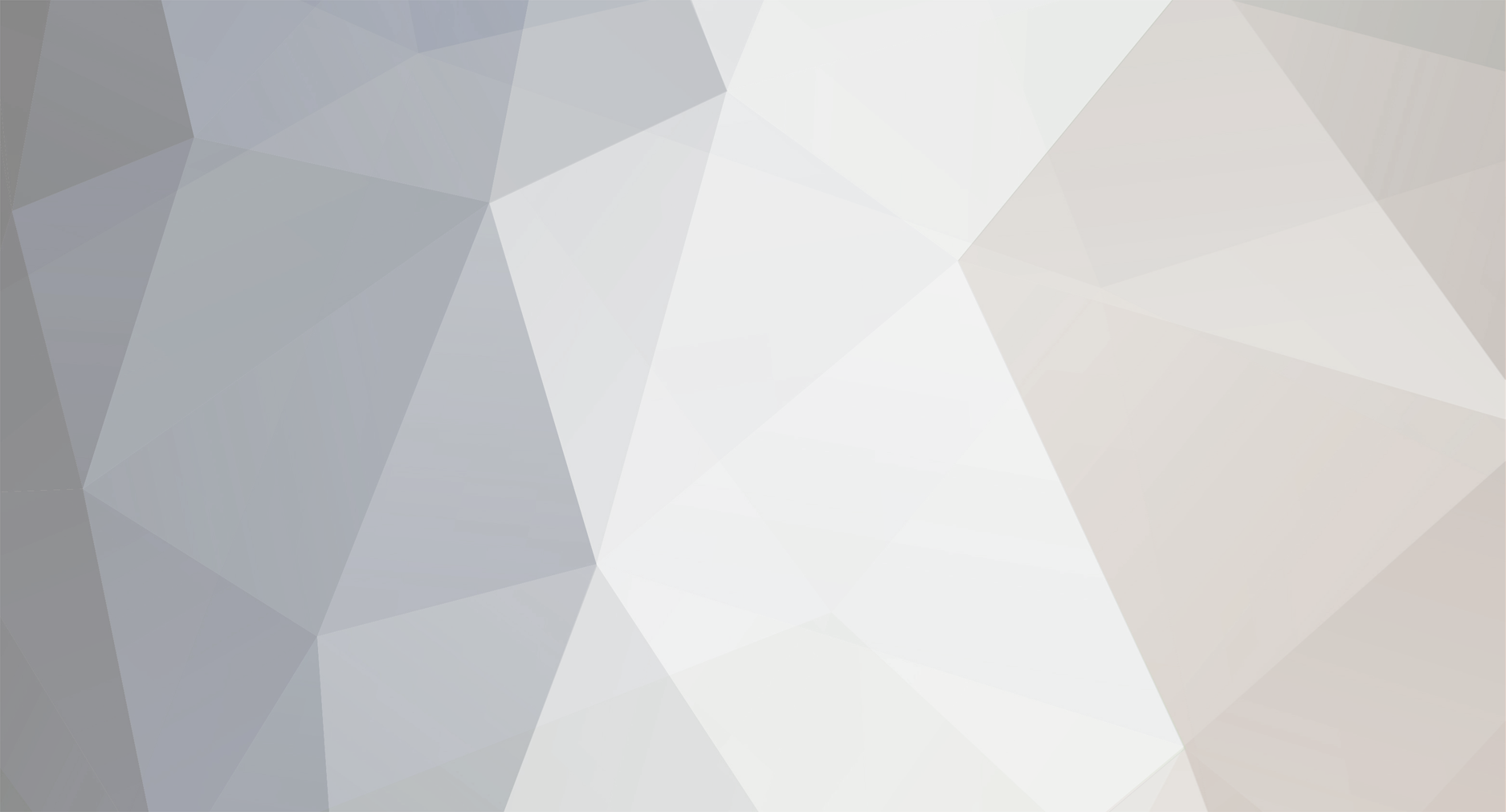
TDotBoy2011
New Members-
Posts
8 -
Joined
-
Last visited
Never
Profile Information
-
Gender
Not Telling
TDotBoy2011's Achievements

Newbie (1/5)
0
Reputation
-
It was set to 250 so I am going to guess that was the issue. I've increased it but my luck I will never see one that long again.
-
Yes. It's all controlled by me.
-
<?php // Declare variables // $debug = false; //$url = "http://www.mto.gov.on.ca/english/traveller/conditions/rdclosure.htm"; $url = "http://www.mto.gov.on.ca/english/traveller/trip/road_closures.shtml"; //$url = "./road_closures.html"; $email_recip = ""; #$email_recip = "t"; $email_from = "s"; $email_from_addr = "t"; $message_header = ""; $message_footer = ""; $arfield1 = array("summary","highway","direction","fromat","to","lanesaffected","trafficimpact","reason","others","eventstart","eventend"); $cntarfield1 = count($arfield1); $timenow = date("Y-m-d H:i:s"); // Connect to database $dbname = "mevents"; $dbuser = ""; $dbpass = ""; $link = mysql_connect('localhost',$dbuser,$dbpass); if (!$link) { die('Could not connect: ' . mysql_error()); } mysql_select_db($dbname); // DON'T EDIT PAST HERE UNLESS YOU KNOW WHAT YOU'RE DOING // // MAIN PROGRAM /// $webpage = read_web($url); $content = find_data($webpage, "<!-- MIDDLE COLUMN", "</html>"); $content = find_data($content, "</div><a ", "</html>"); $curr_events = read_html_table($content,true); // Parse the events HTML table if ($curr_events["totalevents"]>0) { $sql = "update mEvents set eventid = 'xRoadInfo' where eventid like 'RoadInfo%'"; $result = mysql_query($sql); } for ($curr_event_num = 1; $curr_event_num <= $curr_events["totalevents"]; $curr_event_num++) { $send_email = false; // Flag used to see if an email should be sent for new closure events $event_message = $message_header; $curr_event_found = false; if ($curr_events[$curr_event_num]["unscheduledevents"]==0) { $sql = "select * from mEvents where eventid = '".($curr_events[$curr_event_num]["eventid"])."' limit 1"; } else { $unscd = explode("\n",$curr_events[$curr_event_num]["others"]["data"]); $sql = "select * from mEvents where eventid='xRoadInfo' and highway='".$curr_events[$curr_event_num]["highway"]["data"]."' and direction='".$curr_events[$curr_event_num]["direction"]["data"]."' and fromat='".$curr_events[$curr_event_num]["fromat"]["data"]."'"; if ($unscd[0]!="") $sql .= " and others like '".$unscd[0]."%'"; } $result = mysql_query($sql); if (mysql_num_rows($result)>0) $curr_event_found = true; if (!$curr_event_found) { // Add the not found current event to the message $send_email = true; if ($curr_events[$curr_event_num]["eventend"]["data"]=="") { $event_message .= "*-NEW-*\n"; } else { $event_message .= "-CLEARED-\n"; } if ($debug) $event_message .= $curr_events[$curr_event_num]["eventid"]; $sqlquery = "insert into mEvents (eventid,date_insert"; $sqlval = "('".$curr_events[$curr_event_num]["eventid"]."','".$timenow."'"; for ($a=0;$a<$cntarfield1;$a++) { $sqlquery .= ",`".$arfield1[$a]."`"; $sqlval .= ",'".addslashes($curr_events[$curr_event_num][$arfield1[$a]]["data"])."'"; if ($curr_events[$curr_event_num][$arfield1[$a]]["data"]!="") { $event_message .= $curr_events[$curr_event_num][$arfield1[$a]]["field"]."".$curr_events[$curr_event_num][$arfield1[$a]]["data"]."\n"; } if ($debug) $event_message .= "<br/>"; } $sqlquery .= ") values".$sqlval.")"; mysql_query($sqlquery); $event_message .= "====END====\n"; $email_subject = "*-NEW-* "; $email_subject .= $curr_events[$curr_event_num]["summary"]["data"]; } else { $row = mysql_fetch_array($result); if ($row["eventend"]=="") { if ($curr_events[$curr_event_num]["unscheduledevents"]==0) { if ($curr_events[$curr_event_num]["eventend"]["data"]!="") { $send_email = true; $event_message .= "-CLEARED-\n"; if ($debug) $event_message .= $curr_events[$curr_event_num]["eventid"]; $sqlquery = "update mEvents set date_update='$timenow'"; for ($a=0;$a<$cntarfield1;$a++) { $sqlquery .= ",`".$arfield1[$a]."`='".addslashes($curr_events[$curr_event_num][$arfield1[$a]]["data"])."'"; if ($curr_events[$curr_event_num][$arfield1[$a]]["data"]!="") { $event_message .= $curr_events[$curr_event_num][$arfield1[$a]]["field"]."".$curr_events[$curr_event_num][$arfield1[$a]]["data"]."\n"; } if ($debug) $event_message .= "<br/>"; } $sqlquery .= " where eventid='".$curr_events[$curr_event_num]["eventid"]."'"; mysql_query($sqlquery); $event_message .= "====END====\n"; $email_subject = "*-CLR-* "; $email_subject .= $curr_events[$curr_event_num]["summary"]["data"]; } } else { $sqlquery = "update mEvents set eventid='".$curr_events[$curr_event_num]["eventid"]."' where id=".$row["id"]; mysql_query($sqlquery); } } } $event_message .= $message_footer; //Send an email if there is a new/resolved event if ($send_email) { if ($debug) print ("Sending the message below to " . $email_recip . ", from \"" . $email_from . "\" <" .$email_from_addr . ">.\n" . $event_message . "\n"); else mail ($email_recip, $email_subject, $event_message, "From: \"" . $email_from . "\" <" .$email_from_addr . ">"); } } // FOUR LINES BELOW COMMENTED OUT TO STOP REPEATING MESSAGE //if ($curr_events["totalevents"]>0) //{ // $sql = "delete from mEvents where eventid = 'xRoadInfo'"; //$result = mysql_query($sql); //} mysql_close(); // Functions // //read_web - Read the web page // $strURL ==> URL of the webpage function read_web($strURL) { global $debug; $buffer = ""; if($debug){ print("Reading \"$strURL\".\n"); } $fh = fopen($strURL, "rb"); if ($fh === false) { return ""; } while (!feof($fh)) { $buffer .= fread($fh, 1024); } fclose($fh); return $buffer; } // end function read_web // find)data - Gets a substring of the webpage by scraping the data // $strFile ==> text of the webpage // $strStart_Pattern ==> start of the substring // $strEnd_Pattern ==> end of the substring function find_data($strFile,$strStart_Pattern,$strEnd_Pattern, $intStart_Position = 0) { global $debug; if($debug){ print("Searching for \"$strStart_Pattern\"...\"$strEnd_Pattern\".\n<!-- //-->"); } $first_match = strpos($strFile,$strStart_Pattern, $intStart_Position); if ($first_match) { # find the begining of the tag for ($i = $first_match; $i>0;$i--) { $char = $strFile[$i]; if ($char == "<" ) { $first_match = $i; //record the location of the tag break; } } $partialbuffer = substr($strFile,$first_match,strlen($strFile) - $first_match); # find the end of the sub string $second_match = strpos($partialbuffer,$strEnd_Pattern); return substr($partialbuffer,0,$second_match + strlen($strEnd_Pattern)); } else { return(false); } } //end function find_data // read_html_table - Read the contents of an HTML table and return the array(s) // strHTMLTable ==> HTML table text // boolSkipFirstRow ==> Skip the first row if it contains column titles (true/false) function read_html_table($strHTMLTable, $boolSkipFirstRow) { global $debug,$arfield1,$cntarfield1; $arrevents = array(); preg_match_all('/<a name="Event.+<\/table>/Us',$strHTMLTable,$dataevents); $dataevents = $dataevents[0]; $countdataevents = count($dataevents); $j = 0; for($i=0; $i<$countdataevents; $i++) { preg_match('/<a name="Event(.+)" id/', $dataevents[$i], $matches); $eventid = $matches[1]; //if ($eventid>0) { $j++; $arrevents[$j]["eventid"] = $eventid; preg_match('/summary="(.+)" style/', $dataevents[$i], $matches); $arrevents[$j]["summary"]["field"] = ""; $arrevents[$j]["summary"]["data"] = $matches[1]; preg_match_all('/<tr>.+<\/tr>/Us',$dataevents[$i],$tr); $tr = $tr[0]; $counttr = count($tr); for ($k=1;$k<$cntarfield1;$k++) { $arrevents[$j][$arfield1[$k]]["field"] = ""; $arrevents[$j][$arfield1[$k]]["data"] = ""; } if ($eventid>0) $arrevents[$j]["unscheduledevents"] = 0; else $arrevents[$j]["unscheduledevents"] = 1; for ($k=1;$k<$counttr;$k++) { preg_match_all('/<td.+<\/td>/Us',$tr[$k],$td); $td = $td[0]; $td1 = cleandatafield(cleandata($td[0])); if (checkdatafield($td1)==1) { $arrevents[$j][$td1]["field"] = cleandata($td[0])." "; $arrevents[$j][$td1]["data"] = cleandata($td[1]); } else { if (cleandata($td[1])!="") { $arrevents[$j]["others"]["field"] = ""; $arrevents[$j]["others"]["data"] .= cleandata($td[0])." ".cleandata($td[1])."\n"; } } } } } echo "<br/>\ntotal ".$j; $arrevents["totalevents"] = $j; return $arrevents; } //end function readhtmltable function cleandata($x) { $y = $x; $y = preg_replace('/<td.+>/U',"",$y); $y = str_replace("</td>","",$y); $y = str_replace("<strong>","",$y); $y = str_replace("</strong>","",$y); $y = str_replace("/r/n","",$y); $y = trim($y); return $y; } function cleandatafield($x) { $y = $x; $y = str_replace("/","",$y); $y = str_replace(":","",$y); $y = str_replace(" ","",$y); $y = strtolower($y); return $y; } function checkdatafield($x) { global $debug,$arfield1,$cntarfield1; $found = 0; for ($i=0;$i<$cntarfield1;$i++) { if ($x==$arfield1[$i]) $found = 1; } return $found; } print ("ClosedRoadScript"); print date(" G:i:s"); ?> I have the above script that reads this website http://www.mto.gov.on.ca/english/traveller/trip/road_closures.shtml and emails out the changes. Up until this morning it has been working fine but then it started repeating the same one over and over. It didn't put the normal line in the database it would for any other event which would explain why the same email got sent over and over but I am trying to figure out why the database was empty for this event. The only idea I have is something to do with this line and the script not liking it "VASEY ROAD (WAVERLEY)/SIMCOE ROAD 27/SIMCOE ROAD 23" or that it's too long for database. Someone on this site has been helping me with this for the last couple of years but he has since passed away. I've been making small adjustments myself with my limited php know how but this one has me stumped.
-
The org. one is back not that it matters. <?php $curl_handle=curl_init(); curl_setopt($curl_handle,CURLOPT_URL,'http://api.radioreference.com/audio/listeners.php?feedId=2798'); curl_exec($curl_handle); curl_close($curl_handle); ?>
-
if I put in http://api.radioreference.com/audio/listeners.php?feedId=763 it shows 222 at the moment I am typing this. document.write('222'); the 222 is the number I am trying to grab.
-
Looks like that feed went down. This one works. <?php $curl_handle=curl_init(); curl_setopt($curl_handle,CURLOPT_URL,'http://api.radioreference.com/audio/listeners.php?feedId=763'); curl_exec($curl_handle); curl_close($curl_handle); ?>
-
I got this. <?php $curl_handle=curl_init(); curl_setopt($curl_handle,CURLOPT_URL,'http://api.radioreference.com/audio/listeners.php?feedId=2798'); curl_exec($curl_handle); curl_close($curl_handle); ?> It outputs the page but I cannot figure out how to only get the numbers.
-
Hi all, I'm php stupid but from what I read its what I need. I am looking to grab just the number this page outputs http://api.radioreference.com/audio/listeners.php?feedId=2798 and put it on a page for some tracking software. When you view the source page of the page it needs to show the number and not the coding for it so Javascripting is out of the question. Can anyone help me?