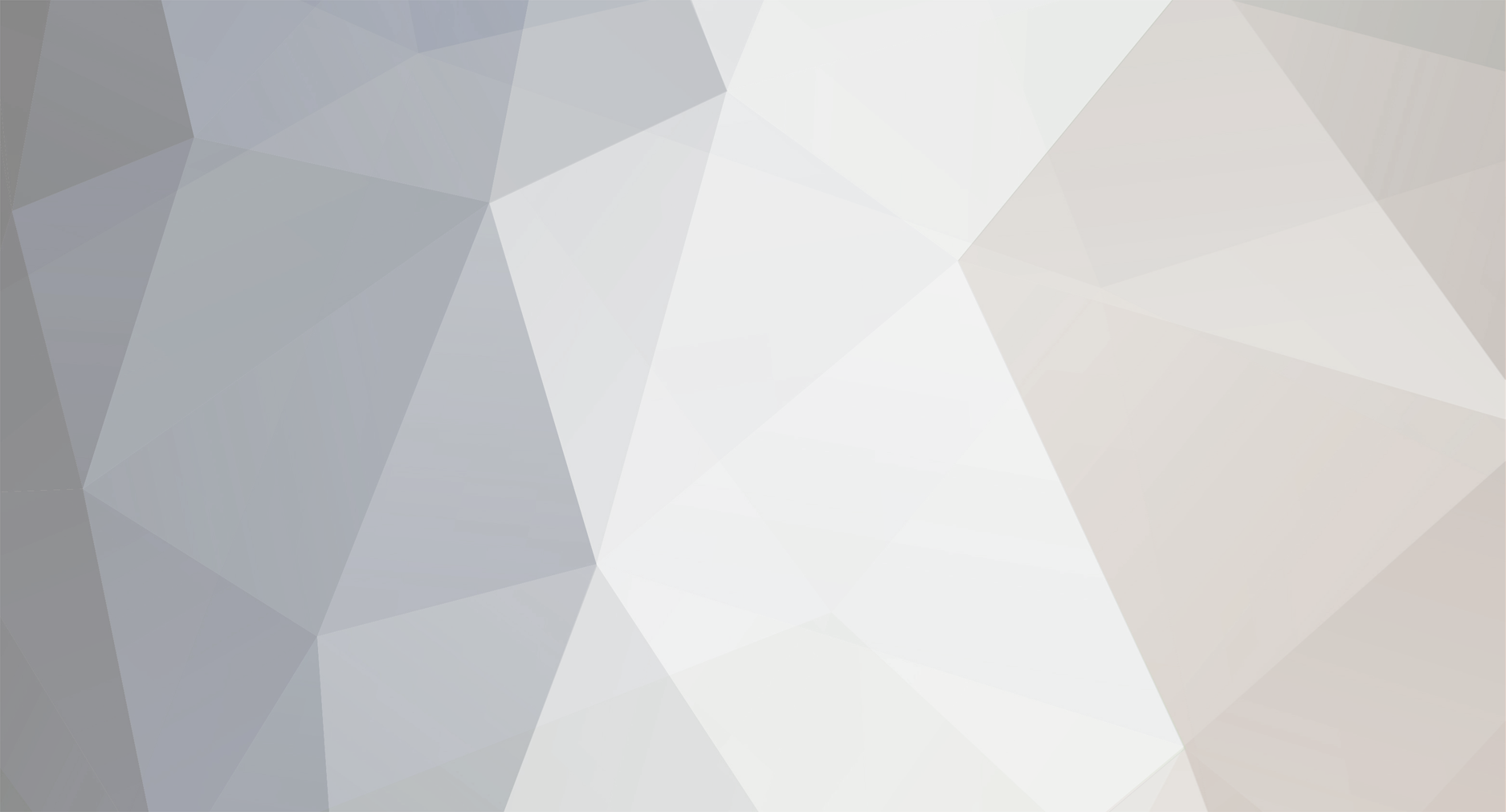
Failing_Solutions
-
Posts
109 -
Joined
-
Last visited
Posts posted by Failing_Solutions
-
-
Hi Matt,
You could easily select the products with SQL, but what happens after that is a little unknown. Are you trying to direct customers to other peoples sites ? Allow them to buy it on your site?
Are you factoring in the shipping cost? If product 1 from Company A is more then Company B, but Product 2 is cheaper at Company A who has cheaper shipping rates, then it "may" make more since to buy both products at company A.
-
Hi Guys and Gals,
I run a simple fansite for some of my fellow MTG Tactics players at www.onlinegamekey.com
I recently made a Booster Page value page here: http://www.onlinegamekey.com/booster-packs.php
And it seems the page loads really slow, but I kind of understand that since its doing a lot of math and dealing with a lot of data.
I am wondering if there is a way to possibly optimize the page and querys in such a way that it doesn't take so long to load.
I'm basically using 4 queries to get the value of each pack and 1 function to average the query data. And I add them up as I go.
This is my function to find average values
///AVERAGE FUNCTION function calculate_average($arr) { $count = count($arr); //total numbers in array foreach ($arr as $value) { $total = $total + $value; // total value of array numbers } $average = ($total/$count); // get average value return $average; }
These are the queries to get the Average Values
<?php ///SETTING THE DAY $today = date("Y/m/d"); ///ALTERING DAY QUERY TIME (20 Days of Data) $minusday = mktime(0,0,0,date("m"),date("d")-20,date("Y")); ///SETTING THE DAY FOR THE QUERIES $queryday = date("Y/m/d",$minusday); ///DATA SET FOR BOOSTER PACK 1 //GET THE MYTHIC AVERAGE VALUE $sqlMythic=mysql_query("SELECT AVG( ab.Price_Per ) AS Aaverage FROM auctions AS ab INNER JOIN cardlist AS c ON c.card_name = ab.Card_Name WHERE ab.Day >= '$queryday' AND c.rarity = 'Mythic Rare' AND c.set = '1' GROUP BY c.card_name, ab.Card_Name") or die; while ($row=mysql_fetch_array($sqlMythic)) { $MythicValues[]=$row['Aaverage']; } ///MYTHIC VALUE IS 10% OF AVERAGE VALUE $Mythic = calculate_average($MythicValues) * .1; //GET THE RARE AVERAGE VALUE $sqlRare=mysql_query("SELECT AVG( ab.Price_Per ) AS Aaverage FROM auctions AS ab INNER JOIN cardlist AS c ON c.card_name = ab.Card_Name WHERE ab.Day >= '$queryday' AND c.rarity = 'Rare' AND c.set='1' GROUP BY c.card_name, ab.Card_Name") or die; while ($row=mysql_fetch_array($sqlRare)) { $RareValues[]=$row['Aaverage']; } ///RARE VALUE IS 90% OF AVERAGE RARE VALUE $Rare = calculate_average($RareValues) *.9; ///TOTAL CONTRIBUTION TO PACK 100% IS 10% MYTHIC + 90% RARE $raremythic= $Mythic + $Rare; ///GET THE UNCOMMON AVERAGE VALUE $sqlUncommon=mysql_query("SELECT AVG( ab.Price_Per ) AS Aaverage FROM auctions AS ab INNER JOIN cardlist AS c ON c.card_name = ab.Card_Name WHERE ab.Day >= '$queryday' AND c.rarity = 'Uncommon' AND c.set='1' GROUP BY c.card_name, ab.Card_Name") or die; while ($row=mysql_fetch_array($sqlUncommon)) { $ucValues[]=$row['Aaverage']; } ///UNCOMMON VALUE IS VALUE * 3 $Uncommon = calculate_average($ucValues); $urm= ($Uncommon * 3) + $raremythic; ///GET THE COMMON VALUE $sqlCommon=mysql_query("SELECT AVG( ab.Price_Per ) AS Aaverage FROM auctions AS ab INNER JOIN cardlist AS c ON c.card_name = ab.Card_Name WHERE ab.Day >= '$queryday' AND c.rarity = 'Common' AND c.set='1' GROUP BY c.card_name, ab.Card_Name") or die; while ($row=mysql_fetch_array($sqlCommon)) { $cValues[]=$row['Aaverage']; } ///COMMON VALUE IS VALUE * 10 $common = calculate_average($cValues); //TOTAL SET 1 VALUE IS ALL VALUES ADDED UP $curm= ($common * 10) + $urm; ?>
But I'm not real sure where the strain is coming from. The page seems to load really slow, and I'm not sure if I should just try and write all these queries as a separate function then have it write the data to a separate table, or if there is another way to optimize this code.
I'm still have a lot to learn so I would appreciate any thoughts or ideas you folks can offer me.
Many thanks
-
I have been researching a job listing software suit for my corporate site for a few weeks. I have seen a lot of Job Boards or employment listing suits but that is a little more extravagant then we need. We aren't interested in building a site for allowing companies to list jobs rather just something we can use for our own company jobs, manage applicants, and assign them to the correct department head. Ideally an interface with different level of admin privileges and a front end for users to submit applications and resumes, which will notify the correct person via email that there is an application awaiting review.
So I'm looking for suggestions, on what you guys have used or would recommend. Our site is not built on any CMS system it is a ground up design so I was hoping to find something that will provide the source code so we can modify it to fit into our existing templates.
I have no qualms about software that cost money, but I'm not interested in a hosted solution, we would want it on our server.
Any recommendations, suggestions, or thoughts is appreciated.
Thank you.
-
Found the solution,
min: 0;
max: MinvalMap.length -1,
values [0, MinvalMap.length -1]
Was all I needed..
-
I'm trying to setup a range slider. However my initial value is always starting at 4.00 which is not the first value in the value map I setup. It increments correctly but is just starting at the wrong value. I have included notes in the code part as to what I was trying to do.
I'm using PHP to get the values for the increments and it is looping through the correct values in the var MinvalMap
When I view the source I see the following values in the var:
var MinvalMap= [2.50,3.00,3.71,4.00,4.50,5.00,6.00,6.50,7.00,8.00,8.40,8.50,9.00,9.50,10.00,11.00,12.00,12.50,13.50,14.00,15.00,15.50,15.80,16.00,17.00,18.00,18.50,19.00,19.50,20.00,20.50,21.00,21.50,22.00,23.00,24.00,25.00,26.00,27.00,28.00,28.50,29.00,30.00,31.00,32.00,34.00,35.00,36.00,37.00,38.00,39.00,39.50,40.00,42.00,43.00,43.40,45.00,46.00,47.00,48.00,50.00,52.00,55.00,56.00,58.00,59.50,60.00,61.00,64.00,68.00,70.00,75.00,78.00,80.00,81.00,86.00,88.00,90.00,94.00,100.00,108.00,109.00,112.00,118.00,125.00,126.00,128.00,143.00,190.00,200.00,208.00,];
Which is correct but the min value is always starting at 4.00
So I'm thinking that this is not the correct way to reference the first value
min: MinvalMap[0],
Seems like it should be but .. I'm learning still..
Any help is appreciated.
The HTML part
<p>Range Slider Here</p> <form> <fieldset> <legend>Connector 1</legend> <p> <label for="rangeAmount">Range</label> Min:<input type="text" id="rangeAmountMin" style="border:0; color:#f6931f; font-weight:bold;" onfocus="TheMin(this.value)"/> to Max:<input type="text" id="rangeAmountMax" style="border:0; color:#f6931f; font-weight:bold;" onchange="TheMax(this.value)" /> </p> <div id="slider-range"></div>
The Jquery Slider Range getting values from database...
<?php /// CON 1 Array $Con1minquery=mysql_query("SELECT DISTINCT m_connector_1 FROM products where m_connector_1 IS NOT NULL ORDER BY m_connector_1 ASC"); while ($aquery=mysql_fetch_assoc($Con1minquery)) { $newArray[]=$aquery['m_connector_1']; asort($newArray); } ?> <script type="text/javascript"> jQuery("#jQueryUISlider").slider(); //create a value array map echo the sql array and add a comma to each value var MinvalMap= [<?php foreach($newArray as $key => $val) {echo "$val,"; }?>]; jQuery( "#slider-range" ).slider({ range: true, //get the first value of the MinvalMap min: MinvalMap[0], //get the last value of the MinvalMap max: MinvalMap.length -1, //setting the ranges to lowest to highest of the MinvalMap values: [MinvalMap[0], MinvalMap.length-1], slide: function( event, ui ) { $( "#rangeAmountMin" ).val(MinvalMap[ui.values[ 0 ]].toFixed(2)); $( "#rangeAmountMax" ).val(MinvalMap[ui.values[ 1 ]].toFixed(2)); } }); jQuery( "#rangeAmountMin" ).val(MinvalMap[$( "#slider-range" ).slider( "values", 0 )].toFixed(2)); jQuery( "#rangeAmountMax" ).val(MinvalMap[$( "#slider-range" ).slider( "values", 1 )].toFixed(2)); </script>
Attached is an image of how the page looks on Fresh (CRTL+F5) load.. always starts with min value 4.00
-
Yes this was very helpful,
I'm at the point now where I've got one my first slider accepting the ranges from an initial query and incrementing correctly.
I was using thinking of using XHTML to pass the slider values to the php processing page. Which would execute the query then pass back the table with the matching products back to the page.
Now I'm stuck on how to pass multiple queries though the Form post / URL. I got an example from w3schools which shows passing one value via javascript function, I understand that I need to build the URL to get multiple parameters to be passed but that is proving to be a little of a challenge for me.
I'm going to mark this one as a resolved and appreciate your help.
I may need some more help but will provided specific code and a specific questions to help illustrate where I'm stuck.
Thanks again.
-
Hi Nogray,
I appreciate you taking the time to comment. That seems like good technical information I may reference. Perhaps you know of some live examples or tutorials you can point me to?
-
I'm looking for some advice, and help with using range sliders as filters for data on a product-line we offer.
The sliders will allow users to set a min & max acceptable values for a particular section of the part.
An example: we have a dust boot which can expand and contract and has 2 different end sizes. All this data is stored in our sql database.
What I want to do is basically like Google Finance Does with their stock screener. http://www.google.com/finance/stockscreener
I would like to have 4 sliders.
Slider 1: Top End Size Range
Slider 2: Bottom End Size Range
Slider 3: Maximum Allowable Length
Slider 4: Minimum Allowable Size (Collapsed)
Below the sliders I want to display a table with all parts that match the selections as the sliders change I want it to filter out / in parts, like is done with the Google Wave-Like slider here http://demo.tutorialzine.com/2009/10/google-wave-history-slider-jquery/demo.php
Sorry for such a long explanation but here is where I'm a little stuck.
The sliders I understand, fairly simple with Jquery.
Getting the data from the database seems pretty simple with MYSQL / PHP
Now if I only had access to these 3 languages I think I could make it work, but it would require a lot of re-quering the database every time somebody mouses out on a slider and thus requiring a page refresh to display the query results.
That doesn't seem like the best way to do it, both the examples above simply change the page contents of the table based on the position of the slider. I think I need to use AJAX .. but honestly that is one I haven't used before and I am very open to any help / advice / examples you can point to.
Thank you for your time
-
Hi Xyph,
I appreciate you taking the time to reply. Its a tough one for me, because I'm working inside oscommerce and have to be senstive to its requirements.
I am already posting the form from the index page to the actual product search page, so unfortuantely I can't just use $_GET. The results from the selections on the page are passed to the product search page for SEO purposes, we don't want a bunch o random stuff getting seen on our Index page so we pass it to the product searh page, which isn't a SEO page rather a functionality page. T
I was hopeing there was a Firefox equivlent to the .htaccess fix for IE that would prevent this.
On our site the only time a duplicate post would matter would be in our checkout which is HTTPS so I can put a port check on the statement....
Thanks again, I'll keep digging.
-
Ref Site: www. rubberstore .com
A new problem has come up with a recent upgrade to the latest Firefox.
The problem which used to be exclusive to IE 7/8 was when you use the ProductSearch on the bottom of the page hit the search then you use the browsers "Back" button the page shows expired. Which forces you to refresh then retry to get the page to reload.
We fixed the problem in the past for IE by including
BrowserMatch MSIE force-no-vary #in our htaccess
But there doesn't appear to be an equivalent statement for Firefox.
We also have a page dedicated for ProductSearch, which we were able to use cache private to prevent the problem, however on our homepage (index.php) this is not an option because this page does so many other things.
Has anybody found a way around this issue with FireFox?
Please let me know if my question and issue aren't clear, thank you!
-
Well I managed to find a solution for this.
In case anybody else runs into this problem..
By adding:
BrowserMatch MSIE force-no-vary
to your .htaccess file you can prevent IE from caching http pages thus resulting in no webpage expired messages when you hit the back button. It isn't suppose to work on https pages which is actually good news as this is where you want the page to be cached to prevent duplicate submissions of orders and such. Either make sure you test it on your server before running with it.
Thanks
-
Forgive me if I'm in the wrong area, but not sure what forum this was most appropriate for.
This community has always been the most helpful for me so I'm hoping I can find an answer.
We have a form that uses multiple selects as filters for our products. Upon submission it retrieves matching products and displays a list. This all works great until you hit the "Back" button on IE 7/8/9 the page drops and displays the message "The Webpage Has Expired" which forces the user to have to refresh the page and click retry to get the page to reload. This is horrible behavior and a pain the butt for the user.
We did find a work around which is declaring the page header private like this
header( 'Cache-Control: private, max-age=10800, pre-check=10800' );
Which has served us well over the last year, however now we want to move the search functionality to our homepage in which we can't use a private header, its an online shop and the homepage (index.php) is the base url for so many sub pages.
Is there any other way anybody knows to stop IE from throwing the webpage expired message?
In my perfect world IE would stop being supported, and Microsoft would concede the browser market to FF / Safari / Chrome.. until then.. I must deal with this.. Your help is as always, very valued, and very much appreciated.
-
Thank you very much.
I made a table backup, did a SQL replace for all my dates ie (12/31/2011 to 2011-12-31), then converted the column into data type DATE.
Changed my lookup functions to pull the correct data
$today = date("Y/m/d"); $minusday = mktime(0,0,0,date("m"),date("d")-14,date("Y")); $queryday = date("Y/m/d",$minusday);
Then used php to pretty up the date.
date('m-d-Y',strtotime($today))
Wasn't as bad as I thought, thanks for the help and previous post that put me on the right track!
-
Okay so it sounds like I have to reformat all my data dumps, truncate my data, re-upload, and recode my page so the date is outputted in the correct pattern instead of a database 0000-00-00 pattern.
Was trying to avoid that.
-
Problem: My query is set to get rows with date after x, as of today (January 1st, 2012) my query doesn't recognize 01/01/2012 as greater than day 12/31/2011.
Webpage: http://www.onlinegamekey. com/ MTGT-Auction.php
Execution Code:
<?php ///Setting The Date & Query Range $today = date("m/d/Y"); $minusday = mktime(0,0,0,date("m"),date("d")-14,date("Y")); $queryday = date("m/d/Y",$minusday); ///Getting The Data $quer2=mysql_query("SELECT * FROM auctions WHERE Card_Name ='$cards' AND Day >='$queryday' ORDER BY Price_Per") or die; ?>
Additional Notes: My database stores the date in this format mm/dd/yyyy in column `Day` stored as a VARCHAR.
Testing on the site: Search for Air Elemental this card is new today (Auction ID)543716 (Card Name)Air Elemental (Cards Per Auction)1 (Seller)Edwitdahead (Auction Price)7 (Price Per)7.00 (Date)01/01/2012
I would like to avoid changing the database format if possible, and I assume this is likely a common issue so any help pointers or links you can provide to help me fix this is Greatly Appreciated.
Thank you
-
Wow, just re-looking at my post I took a guess
I was using ' in my Select box option values just changed them to \" and that fixed the issue...
Yep that's what mysql_real_escape_string does. You should be calling that on any data that comes near your database.
As in when the data is written to the database? Or coming from it? Or both?
Right now I'm uploading data via phpMyAdmin but do plan on having the site parse flat dump files.
-
Wow, just re-looking at my post I took a guess
I was using ' in my Select box option values just changed them to \" and that fixed the issue...
-
onlinegamekey. com/MTGT-Auction.php is the page I'm working on.
The problem I'm having is cards with an apostrophe in the name breaks the operation.
I am populating the Select Box with the Card Names and those are coming in fine, its not until I try to use the select value to get that specific card data do I have an issue.
This query specifically
$quer2=mysql_query("SELECT * FROM auctions WHERE Card_Name ='$cards' Order By Price_Per") or die;
I've tried
$quer2=mysql_query("SELECT * FROM auctions WHERE Card_Name =" . htmlspecialchars($cards) . " Order By Price_Per") or die;
but then I get no data for any card.
Here is the page code I'm working with.
<?php $cards = $_POST['cards']; //SELECTING DATA FOR THE DROPDOWN $sql = "Select Card_Name From auctions Group BY Card_Name ASC" or die; $result = mysql_query($sql); ?> <script type="text/javascript"> <!-- var optList; var optsValue = new Array(); var optsText = new Array(); //when the page loads get the original options values and text and store them in arrays window.onload = function() { optList = document.getElementsByTagName("option"); for(var i=0; i<optList.length; i++) { optsValue[i] = optList[i].value; optsText[i] = optList[i].text.toLowerCase(); } } function searchSel(txtSearch) { //clear all the current options document.getElementById("items").options.length = 0; var count = 0; for(var i=0; i < optsValue.length; i=i+1) { if(optsText[i].indexOf(txtSearch.toLowerCase()) == 0) { //match found //add this option to the select list options var newOpt = new Option(optsValue[i],optsText[i],false,false); document.getElementById("items").options[count] = newOpt; count = count+1; } } } function reload(form) { var f1 = document.forms['f1'] var val=f1.cards.options[f1.cards.options.selectedIndex].value; self.location='MTGT-Auction.php?card=' + val ; } //--> </script> <style type="text/css"> body { background-color:#000000; } .row-one { background-color: #666666; font-family: Arial, Helvetica, sans-serif; font-size:12px; font-weight: bold; line-height: 17px; color:#CCFF33; } .row-two { background-color: #333333; font-family: Arial, Helvetica, sans-serif; font-size:12px; font-weight: bold; line-height: 17px; color: #FF0; } .th { background-color:#000000; font-family:Arial, Helvetica, sans-serif; font-size:14px; font-weight:bold; color:#CC0000; padding: 2; } </style> <!-- CREATE FORM & SELECT BOX --> <form method="post" name="f1" action="MTGT-Auction.php"> <select name="cards" id="items"> <option value='0'>Select...</option> <?php while ($row=mysql_fetch_array($result)) { if ($row['Card_Name']==@$cards) { echo "<option selected value='$row[Card_Name]'>$row[Card_Name]</option>"; } else { echo "<option value='$row[Card_Name]'>$row[Card_Name]</option>"; } } ?> </select> <br /> <input type="text" id="txt" value="Card Name?" onfocus="this.value==this.defaultValue?this.value='' :null" onkeyup="searchSel(this.value);" style="color:#000000; font:Arial; font-size:12px; background-color:#e1e1e1;" /> <BR /> <input type="submit" value="Submit" name="submit" /> <input type=button onClick="location.href='MTGT-Auction.php'" value='Reset' /> </form> <!-- CREATE TABLE WHERE DATA GOES --> <table border="1" bordercolor="#000000"> <tr align="center"> <th class="th">Auction ID</th> <th class="th">Card Name</th> <th class="th">Cards Per Auction</th> <th class="th">Auction Price</th> <th class="th">Cost Per Card</th> <th class="th">Date Listed</th> <th class="th">Seller Name</th> </tr> <?php //GET DATA FOR TABLE BASED ON SELECTED CARD & LOOP THROUGH $quer2=mysql_query("SELECT * FROM auctions WHERE Card_Name ='$cards' Order By Price_Per") or die; $i =1; WHILE($row = mysql_fetch_array($quer2)) { if ($i%2 !=0) $rowColor = "class='row-one'"; else $rowColor = "class='row-two'"; echo "<tr $rowColor>" . "<td>" . $row[Auction_ID] . "</td><td>" . $row[Card_Name] . "</td><td>" . $row[Qty_Listed] . "</td><td>" . $row[Price] . "</td><td>" . $row[Price_Per] . "</td><td>" . $row[Date] . "</td><td>" . $row[seller] . "</td></tr>"; $i++; } //} ?> <?php //QUICK CHECK IS OUR VARIABLE SET??? echo "<font color=\"#FFFFFF\">". $cards . "</font>"; ?> </table>
I image this is probably a very common problem & easy fix that has been answered many times, but I haven't found any thing that worked for me so any help.. or links to similar issues would really be appreciated.
Thank you,
-
Turns out the problem wasn't with the permissions, I had actually deleted the folder in which the files were suppose to be uploaded to. Permissions at 755 work fine with the script.
/facepalm
-
The other question you had in regards to "other" permissions, is that if you have path where I give you 7 perms to a particular directory, and from there the script creates a directory, then the owner of that directory is going to be the user that created it. So, if the script creates its own directories, then use those directories to store the image files, and you don't need to give "other" the 7 permission, because it already owns the directory.
There has to be at some point, the full set of RWX perms given to the user that php will be running as --- there is again, no way around that fact if your script needs to write files.
I've seen some scripts that creates a directory, then writes the files, then chmods it back down to secure levels. So there is no real way to get all the uploads into 1 directory without opening it up. I'll just end up having a main directory, with a bunch of sub-folders each containing the specific upload from that submission, is that correct?
-
Dwilliams and gizmola thank you both for the info.
I will admit that I have had 2 courses in Linux and understand permission to some degree. What I have trouble wrapping my head around is some of the Open Source packages that allow for image upload from a user that don't require the folder to have the public / other RWX permission. This to me says that somehow these packages are taking ownership of the folder they are uploading the images to.
To the security part, giving the public the ability to RWX inside a folder, at first thought to me seemed okay because they were limited to the scope of that folder. The problem then became they were somehow able to execute scripts inside that folder that actually affected all the folders on the user account of the server (meaning my account on my host shared server). Thus, it becomes a problem and after the last hack my host told me to remove all xx7 permissions.
I will continue to digest the information you both provided and do research myself to learn what I can.
I'm wondering if I move the php script inside the folder it is uploading to and simply change my form on submit to point to the Upload folder if I can skip this issue? My thinking is that since the file is in the folder then it won't need a public X permission.
Again thanks for the info, I'll continue to work on this and update my solution and mark the question answered when it is solved.
-
Here is the script if it will help:
<?php include 'dbc.php'; //////// End of connecting to database //////// /// INSERTING DATA TO DATABASE if(!isset($rfq_part) && !isset($rfq_name) && !isset($rfq_company)&& !isset($rfq_phone) && !isset($rfq_email)&& !isset($rfq_proto) && !isset($rfq_eau)) { echo "Sorry this page is only available for submissions<br><br>"; echo "Please visit our <a href='orings.php'>Oring Selector</a> to submit and RFQ"; echo "<div align='right'><table border='0'><tr><td valign='bottom'><A href=\"javascript: window.close()\">X Close</a></td></tr></table></div>"; }else{ ///Writing the file to the database///// // Configuration - Your Options $allowed_filetypes = array('','.jpg','.pdf','.pjpg','.jpeg','.gif','.bmp','.png','.ppf','.tiff','.pdp','.csv','.dxf','.ppt','.xls','.avi','.doc','.stp','.step','.igs','.iges','.x_t','.dwg'); $max_filesize = 2024288; // Maximum filesize in BYTES (currently 0.5MB). $upload_path = './upload/'; // The place the files will be uploaded to (currently a 'files' directory). $filename = $_FILES['file']['name']; // Get the name of the file (including file extension). $ext = substr($filename, strpos($filename,'.'), strlen($filename)-1); // Get the extension from the filename. // Check if the filetype is allowed, if not DIE and inform the user. if(!in_array($ext,$allowed_filetypes)) die('The file you attempted to upload is not allowed.'); // Now check the filesize, if it is too large then DIE and inform the user. if(filesize($_FILES['file']['tmp_name']) > $max_filesize) die('The file you attempted to upload is too large.'); // Check if we can upload to the specified path, if not DIE and inform the user. if(!is_writable($upload_path)) die('You cannot upload to the specified directory, please CHMOD it to 777.'); // Upload the file to your specified path. if(move_uploaded_file($_FILES['file']['tmp_name'],$upload_path . $filename)) echo ''; //'Your file upload was successful,';// view the file <a href="' . $upload_path . $filename . '" title="Your File">here</a>'; // It worked. else echo ''; //There was an error during the file upload. Please try again.'; // It failed . $query="INSERT INTO rfq(rfq_id,rfq_model,rfq_name,rfq_company,rfq_phone,rfq_email,rfq_prototype,rfq_eau,notes,upload,rfq_date) VALUES ('','$rfq_part','$rfq_name','$rfq_company','$rfq_phone','$rfq_email','$rfq_proto','$rfq_eau','$rfq_note','$rfq_upload','$rfq_date')"; mysql_query($query) or die ('Error trying to update database'); //// SELECTING RFQ NUMBER $rfq_number=0; $rfq_number=mysql_query("SELECT rfq_id FROM rfq where rfq_id=(SELECT MAX(rfq_id) FROM rfq)"); $rfq_number=mysql_fetch_array($rfq_number); $rfq_id=$rfq_number['rfq_id']; ?>
-
Awhile ago I wrote a simple form to except image specific uploads from users and chmoded the directory to 777... worked great...
Till there was a hack and then I found that chmod 777 is bad
So changed the folder to 775, but now the upload script won't work.
Can somebody point me to a post or article on how to give a file ownership or group permissions so it can safely run its uploads to the folder?
I have tried google but keep getting Linux and Window OS results.. its a tough question to google.
Any help is very much appreciated.
-
sorry I never coded anything this way. I always create all my elements either on the page or externally then use a php or javascript to show them when needed.
You can of course do it but I have no experience with it.
This site may help: http://www.javascriptkit.com/javatutors/dom2.shtml
How do I format checkboxes to input them into MySQL database
in PHP Coding Help
Posted
Something like this maybe of some help.. wrote it real quick and I've seen more optimized examples but I tend to code in a way that makes sense to me...
Then to record a Yes or No into the database is fairly simple.. on your processing page just grab the variable...
Hope that helps