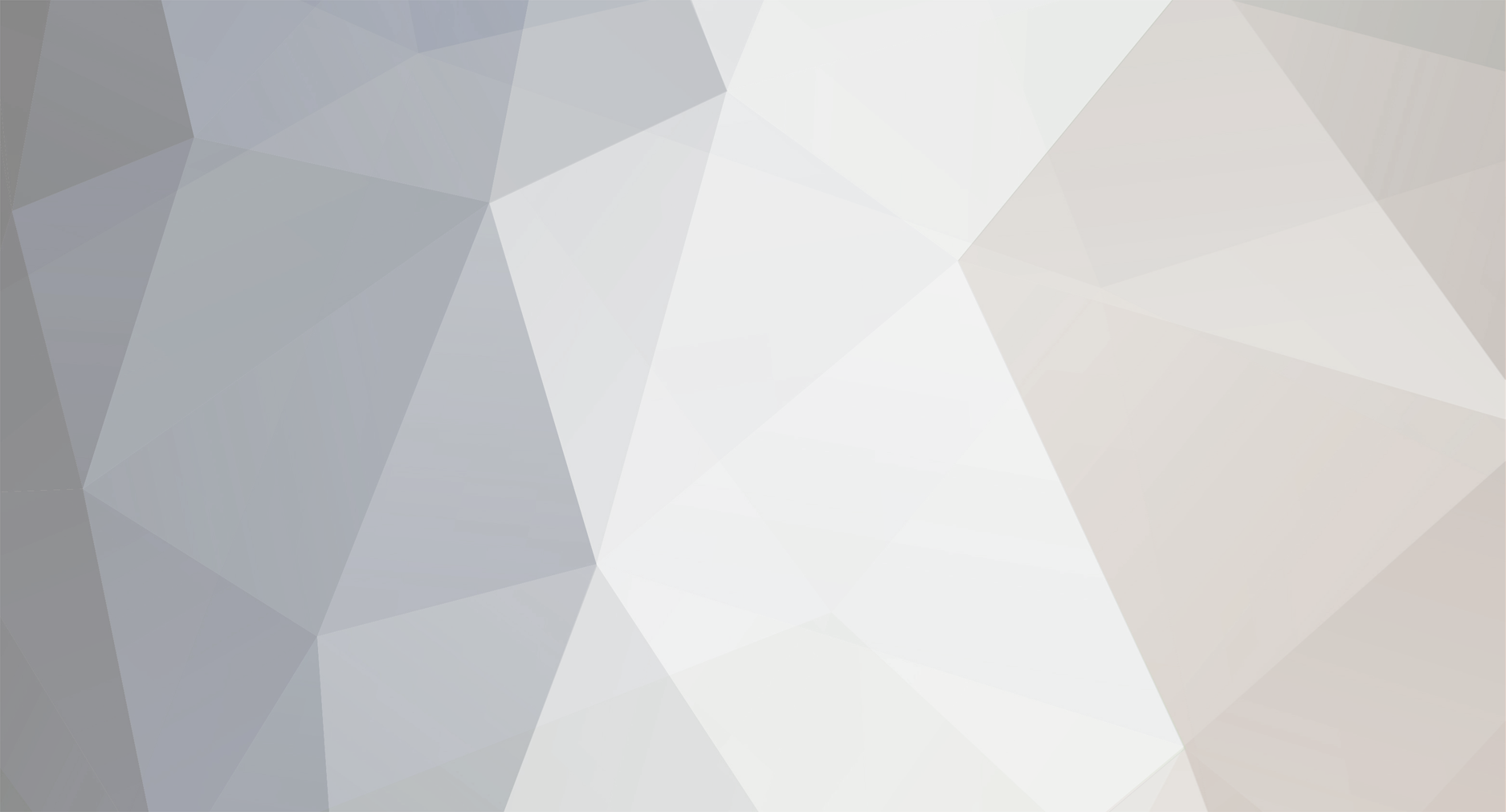
Th3Boss
-
Posts
33 -
Joined
-
Last visited
Posts posted by Th3Boss
-
-
Flip the parameter order of both array_filter() function calls. That should fix all of the warnings.
E.x.
$textLines = array_filter('trim', $textLines);
to
$textLines = array_filter($textLines, 'trim');
nbst
Yeah that fixed the errors.
echo $query looks like this:
INSERT INTO Table2 (`ID`,`Name`) VALUES ('50', 'Name1'), ('50', 'Name2'), ('50', 'Name3')
Though it doesnt insert it into the table. It does insert the 50 into Table1 though from.
$query = "INSERT INTO table1 (ID) VALUES (NULL)";
-
Try something like this:
<?php //Convert text input into lines based upon newline $textLines = explode("\n", $_POST['TextArea']); //Trim all the values $textLines = array_map('trim', $textLines); //Remove empty line $textLines = array_filter('trim', $textLines); //Escape input for query $textLines = array_filter('mysql_real_escape_string', $textLines); //Create parent record in table 1 $query = "INSERT INTO table1 (field1) VALUES ('value1')"; $result = mysql_query($query) or die(mysql_error()); $table1ID = mysql_insert_id(); //Process the lines into separate values for INSERT query $insertValues = array(); foreach($textLines as $line) { $insertValues[] = "('{$table1ID}', '{$line}')"; } //Create INSERT query $query = "INSERT INTO table2 (ID, Name) VALUES " . implode(', ', $insertValues); ?>
Warning: array_filter() expects parameter 1 to be array, string given in on line 60
$textLines = array_filter('trim', $textLines);
Warning: array_filter() expects parameter 1 to be array, string given on line 62
$textLines = array_filter('mysql_real_escape_string', $textLines);
Warning: Invalid argument supplied for foreach() on line 71
foreach($textLines as $line)
And should field1 be a new column for the parent record or should that be the auto increment ID from table 1?
Try something like this:
//Create parent record in table 1 $query = "INSERT INTO table1 (field1) VALUES ('value1')"; $result = mysql_query($query) or die(mysql_error()); $table1ID = mysql_insert_id();
Like:
//Create parent record in table 1 $query = "INSERT INTO table1 (ID) VALUES (NULL)"; $result = mysql_query($query) or die(mysql_error()); $table1ID = mysql_insert_id();
-
oh I just noticed I have that wrong.
The ID needs to be the same as the ID from another table that is auto increment, the structure is this.
It inserts the ID row # for Table 1 as auto increment and then inserts the ID from Table 1 into Table 2 with the data from the textarea.
From Textarea:
Info1
Info2
Info3
Info4
Info5
Table 1:
ID
1
2
3
4
Into Table2:
ID | Name
4 | Info1
4 | Info2
4 | Info3
4 | Info4
4 | Info5
-
A. You need to normalize your data.
B. what debugging have you done? Echo the query, run it in MySQL/phpMyAdmin, etc?
But ignore B until after you do A.
Edit: That's assuming your structure looks like that. You said rows, but your code doesn't look like it's trying to do rows. Do you have many COLUMNS with those names, or you want many rows? (Hint: rows is right.)
What do you mean normalize the data?
the structure I want is this
From Textarea:
Info1
Info2
Info3
Info4
Info5
Into Table:
ID | Name
1 | Info1
1 | Info2
1 | Info3
1 | Info4
1 | Info5
-
Well I replied to an old thread but as it is old it doesnt seem to be getting any activity as it is marked answered.
The thread is: http://forums.phpfreaks.com/topic/228584-insert-different-lines-from-textarea-to-different-mysql-rows/
This doesnt work. I have tried it so many different ways.
I have a textarea that has data like
Info1
Info2
Info3
Info4
Info5
I have a table that has an auto increment ID and a Name column and I want each line from the textarea in its own row inserted in the Name column.
The only reply to the thread says to try:
$lines = array_map('mysql_real_escape_string', explode("\n", $_POST['textarea'])); $values = "VALUES ('" . implode("'), ('", $lines) . "')"; $query = "INSERT INTO table_name (column_name) $values";
Which didnt work. Ive tried a bunch of different things and cant get this to work.
Here is my last attempt of trying to get the above to work:
$lines = array_map('mysql_real_escape_string', explode("\n", $_POST['TextArea'])); $values = implode("'), ('", $lines); $query = "INSERT INTO Table (`ID`,`Name`) VALUES (NULL, '$values')";
-
One way:
$lines = array_map('mysql_real_escape_string', explode("\n", $_POST['textarea'])); $values = "VALUES ('" . implode("'), ('", $lines) . "')"; $query = "INSERT INTO table_name (column_name) $values";
This doesnt work. I have tried it so many different ways.
I have a textarea that has data like
Info1
Info2
Info3
Info4
Info5
I have a table that has an auto increment ID and a Name column and I want each line from the textarea in its own row inserted in the Name column.
I tried like posted above and so many other ways. Here is my last attempt.
$lines = array_map('mysql_real_escape_string', explode("\n", $_POST['TextArea']));
$values = implode("'), ('", $lines);
$query = "INSERT INTO Table (`ID`,`Name`) VALUES (NULL, '$values')";
-
after lots or searching and reading I finally figured it out.
http://stackoverflow.com/questions/10371502/storing-multiple-rows-from-mysql-in-separate-variables
-
anyone able to help?
-
Or make your code use arrays. That's what they're there for.
Can you do an example of how I can do this with arrays?
-
Why don't you select those where slot = 'high' in the query and set LIMIT 8?
Right now in the query it selects all of that data i need from the database and now I need to set all of that data as the correct variables.
-
Pretty sure im doing this completely wrong.
while($row = mysql_fetch_array($result)){ if($row['slot'] == 'high') { $i=1; while($i<= { $name[$i] .= $row['name']."" ; $typeID[$i] .= $row['typeID']."" ; $i++; } } else { } }
There will always be 0 to 8 items that are 'high' in the database $row['slot'].
I want all of the 'name' and 'typeID' to be as:
$name1
$typeID1$name2
$typeID2$name3
$typeID3and so on up to how ever many there is, but no more than 8
How do I do something like this? (Sorry if that is a terrible explanation)
-
I would guess that you already have a subs table in your database which does not have the drone column defined. Drop the table before you run that SQL script.
Thanks, that was it.
-
I keep getting #1054 - Unknown column 'drone' in 'field list' but dont see whats wrong with it.
Anyone see whats wrong?
-
Probably the last line where you have a comma and nothing after it.q
Thanks.
-
This is the error I keep getting and I cant figure out where the error is.
SQL query:
-- -- Dumping data for table `ships` -- INSERT INTO `ships` (`Ship_Name`, `typeID`, `high`, `mid`, `low`, `rig`, `drone`) VALUES ('Bantam', 582, 3, 4, 2, 3, 5, ''), ('Condor', 583, 4, 4, 2, 3, 0, ''), ('Griffin', 584, 2, 5, 2, 3, 5, ''), ('slasher', 585, 4, 4, 2, 3, 0, ''), ('Probe', 586, 3, 4, 3, 3, 5, ''), ('Rifter', 587, 4, 3, 3, 3, 0, ''), ('Executioner', 589, 4, 3, 3, 3, 0, ''), ('Inquisitor', 590, 3, 2, 4, 3, 5, ''), ('Tormentor', 591, 3, 3, 4, 3, 5, ''), ('Navitas', 592, 3, 3, 3, 3, 5, ''), ('Tristan', 593, 3, 3, 3, 3, 5, ''), ('Incursus', 594, 3, 3, 4, 3, 5, ''), ('Punisher', 597, 4, 2, 4, 3, 0, ''), ('Breacher', 598, 3, 4, 3, 3, 5, ''), ('Burst', 599, 3, 3, 3, 3, 5, ''), ('Kestrel', 602, 4, 4, 2, 3, 0, ''), ('Merlin', 603, 3, 4, 3, 3, 0, ''), ('Heron', 605, 3, 5, 2, 3, 5, ''), ('Imicus', 607, 3, 4, 3, 3, 5, ''), ('Atron', 608, 4, 3, 3, 3, 0, ''), ('Maulus', 609, 2, 4, 3, 3, 5, ''), ('Crucifier', 2161, 2, 4, 3, 3, 5, ''), ('Echelon', 3532, 0, 1, 0, 0, 0, ''), ('Vigil', 3766,[...]
#1064 - You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near '' at line 293Anyone see what is wrong with it? -
output = '$' + templateName + ';' + "\\\n";
worked with
"\n"
It left a \ visible the other way. Thanks!
-
Im trying to get it to place a line break after each output
function generateTemplate() { output = '$' + templateName + ';' + "<br>"; output += '$ship=' + fit.shipName + ';' + "<br>"; output += '$shipTypeID=' + fit.shipTypeID + ';' + "<br>"; output += '$fitName=' + fit.name + ';' + "<br>"; output += '$fitID=' + fit.fitID + ';' + "<br>"; }
output is displayed in a textarea
How do I do this?
-
Figured it out finally.
-
You bet. Compare that with
class="Fitting"
class="Info"
title="View"
See a pattern in there?I see they are all =""
-
well you can start by explaining what it is you are tying to accomplish...
On click of class="Info" it uses $DataTypeID for data-typeid from page 1 and does showItemInfo using that data form data-typeid as typeID.
-
Never used javascript before so trying to figure this out.
Page 1:
<?php $DataTypeID="599"; include("Page2.php"); ?>
Page 2:
<head> <script type="text/javascript"> $(function() { $('.Fitting .Info').on('click', showItemInfo); }); function showItemInfo() { var typeID = $(this).attr('data-typeid'); CCPEVE.showInfo(typeID); } </script> </head> <body> <div class="Fitting"> <div class="Info" data-typeid == <?$DataTypeID?> title="View">INFO</div> </div> </body>
Pretty sure this is wrong
data-typeid == <?$DataTypeID?>
How do I get this to work?
-
You shouldn't jump in and out of PHP tags like that, it makes for messy code.
Try this:
<?PHP if($highname1 == 'open') { $output = '<div class="module high open"> <img src="Icon_hi_slot.png" width="46" height="46"> </div>'; } else if(strlen(trim($highname1))) { $output = '<div class="module high inactive"> </div>'; } else { $output = '<div class="module high" data-typeid=$high1typeID data-name=$high1name> <img src="http://image.website.com/Type/'. $high1typeID .'_64.png"> </div>'; } echo $output; ?>
That worked though they were switched around. Works as wanted like this:
<?PHP if($high2name == 'open') { $output = '<div class="module high open"> <img src="Icon_hi_slot.png" width="46" height="46"> </div>'; } else if(strlen(trim($high2name))) { $output = '<div class="module high" data-typeid=$high2typeID data-name=$high2name> <img src="http://image.website.com/Type/'. $high2typeID .'_64.png"> </div>'; } else { $output = '<div class="module high inactive"> </div>'; } echo $output; ?>
Thanks
-
Would it be best to use the if statements or switch in this situation?
-
ok and it seems this does the same thing but as an if statement
<?php if ($high1name == "open") { ?> <div class="module high open"> <img src="Icon_hi_slot.png" width="46" height="46"> </div> <?php } elseif ($high1name == "") { ?> <div class="module high inactive" > </div> <?php } else { ?> <div class="module high" data-typeid=$high1typeID data-name=$high1name> <img src="http://image.website.com/Type/<?php print($high1typeID);?>_64.png"> </div> <? } ?>
insert different lines from <textarea> to different mysql rows
in PHP Coding Help
Posted
Ok got this all working. Thanks a lot everyone who helped.