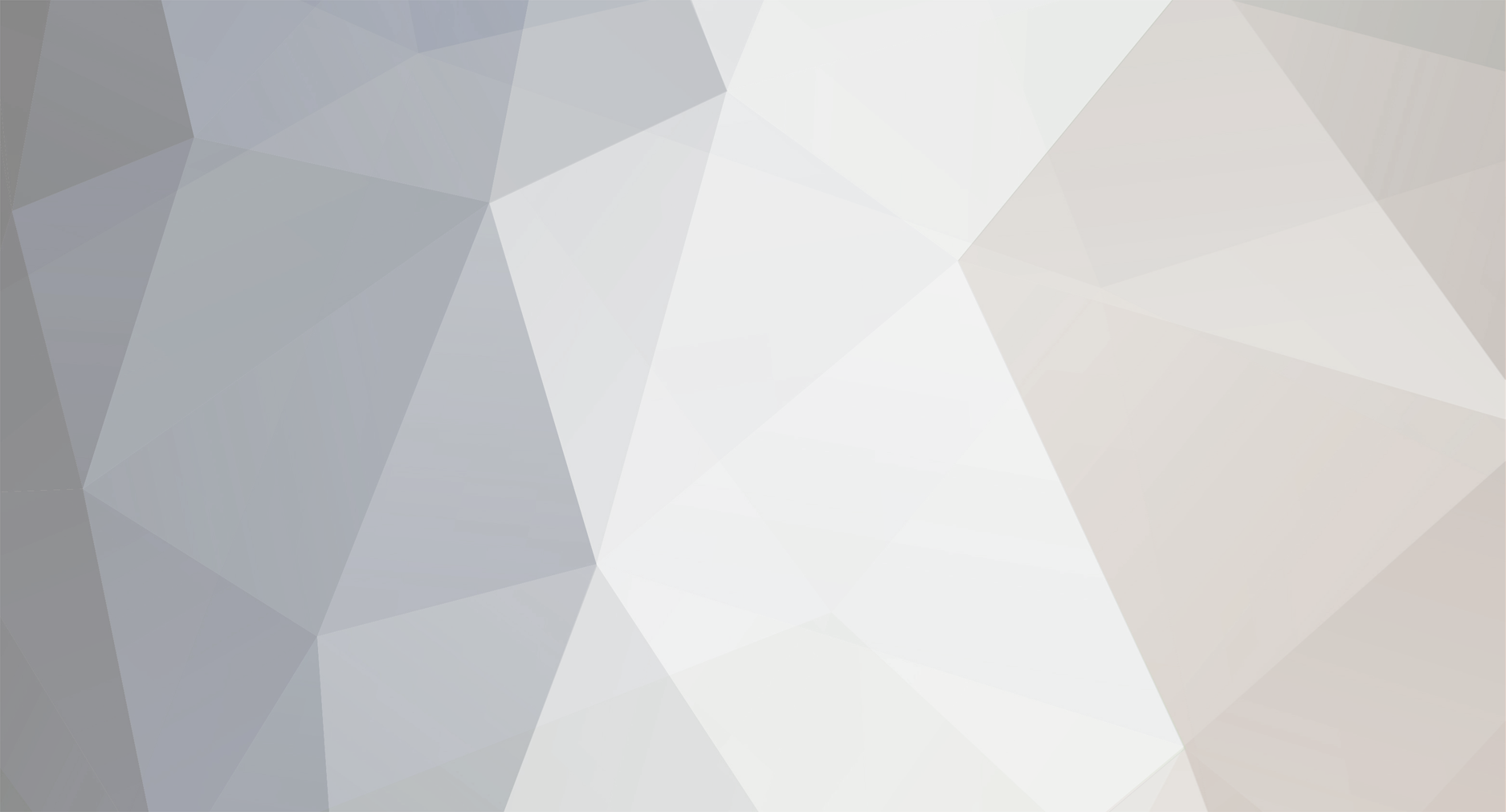
Freedom-n-Democrazy
-
Posts
295 -
Joined
-
Last visited
Never
Posts posted by Freedom-n-Democrazy
-
-
I'm getting this error when I try to fwrite to a directory:
failed to open stream: Permission denied
I have added the user www-data to the group which has permission to the directory?
The directory is this:
/home/directory1/documents
directory1 = drwxr-x---
documents = drwxrwx---
Does anyone know whats going on?
-
Lets say I have a MySQL value of 4... and I have a HTML INPUT field.. Is there a way to make it so that if a client tries to submit a value higher than 4, then they will be returned a message? Something like:
<SCRIPT type="text/javascript"> function validateForm() { if (document.forms["form"]["quantity"].value== (+$row['quantity']) { alert ("Cannot submit because the quantity specified is not available."); return false; } } </SCRIPT> <INPUT name="quantity">
-
Your welcome.
-
Your right. Next time..
-
I'm 99% sure its necessary in that case. Try it, see what happens. If still failure, check your servers error log to see what PHP says.
-
I normally don't suggest long shots but you've had a marathon of zero responses, so:
From my memory, PHP cannot get returned system verbose, but I could be wrong:
If your on Linux, try and get PHP to use a system command to return located text from a file.
-
Going over the code 10 times is due diligence.
-
In your Javascript..
<?php echo $r_admin ?>
should be
<?php echo $r_admin; ?>
-
Yeah, I know I just noticed that.
Well, I went over the code about 10 times.
Please note: This is a help forum. Thanks.
-
Whats wrong with this arithmetic code?
$sizestotal = $content['sizes'] * $row['price']; $sizeltotal = $content['sizem'] * $row['price']; $sizeltotal = $content['sizel'] * $row['price']; $sizeltotal = $content['sizexl'] * $row['price']; $idtotal = $sizestotal + $sizemtotal + $sizeltotal + $sizexltotal; $total += $idtotal;
-
Mistake detected!
There was an extra
';
that should not have been there.
-
Yep, thats the exact code I used from the start. I suspect the error is else where:
<?php session_start(); if (empty($_SESSION['cart']['content'])) { echo '<DIV class="nocart">No product in cart.</DIV>'; } else { echo ' <DIV class="cartcontent">'; $link = mysql_connect('localhost', 'testusr', 'testpw'); mysql_select_db('testdb', $link); foreach ($_SESSION['cart']['content'] as $content) { $query = "select * from products where id='{$content['id']}'"; $result = mysql_query($query); $row = mysql_fetch_array($result); echo ' <DIV class="cartproduct"> <DIV class="productdescriptor"> '.$row['id'].' <BR> <IMG alt="" class="thumbnail" src="../products/'.$row['fordir'].'/'.$row['categorydir'].'/'.$row['id'].'/thumbnail.png"> </DIV> <DIV class="cartproductdata"> '; '; if ($content['sizes'] == 0) {goto sizem;} else {echo "<B>Small:</B> ".$content['sizes'];} echo "<BR>"; sizem: if ($content['sizem'] == 0) {goto sizel;} else {echo "<B>Medium:</B> ".$content['sizem'];} echo "<BR>"; sizel: if ($content['sizel'] == 0) {goto sizexl;} else {echo "<B>Large:</B> ".$content['sizel'];} echo "<BR>"; sizexl: if ($content['sizexl'] == 0) {goto endsizes;} else {echo "<B>Extra large:</B> ".$content['sizexl'];} echo "<BR>"; endsizes: echo ' <BR> <BR> <B>Unit price:</B> $'.$row[price].' AUD <BR> <BR> <INPUT type="submit" value="REMOVE FROM CART"> </DIV> </DIV> '; $sizestotal = $content['sizes'] * $row['price']; $sizeltotal = $content['sizel'] * $row['price']; $idtotal = $sizestotal + $sizeltotal; $total = $idtotal; } echo " </DIV>"; mysql_query ($query); mysql_close($link); echo ' <DIV class="totalandproceed"> Total: $'.$total.' <BR> <INPUT type="submit" value="PROCEED TO PAYMENT"> </DIV> '; } ?>
-
Hey Buddski,
Not one of those lines worked - still getting the same error.
-
What is a T_STRING? google.com cannot find anything about it in the PHP manual.
... and, why am I getting "unexpected T_STRING" for this line?
if ($content['sizes'] == 0) {goto sizem;} else {echo "<B>Small:</B> '.$content['sizes'].'";}
-
You all good?
-
There is. I just re-wrote my code:
if ($_POST['size'] == "Small") { if (isset($_SESSION['cart']['content'][$_POST[id]])) { $_SESSION['cart']['content'][$_POST[id]]['sizes'] += $_POST['quantity']; } else { $_SESSION['cart']['content'][$_POST[id]] = array ('id' => $_POST['id'], 'sizes' => $_POST['quantity']); } } elseif ($_POST['size'] == "Large") { if (isset($_SESSION['cart']['content'][$_POST[id]])) { $_SESSION['cart']['content'][$_POST[id]]['sizel'] += $_POST['quantity']; } else { $_SESSION['cart']['content'][$_POST[id]] = array ('id' => $_POST['id'], 'sizel' => $_POST['quantity']); } }
-
Yeah, you need to create a HTML form and name your INPUT accordingly:
<INPUT name="subject">
No, the client cannot see your PHP code. Web server software will not send it.
-
I see. Do you mean something like this?:
$all = $_SESSION['cart']['content'][]; foreach ($_SESSION['cart']['content']['$all']['sizel'] as $content) {
-
$from = $_POST['from']; $subject = $_POST['subject']; $body = $_POST['body']; $header = 'From: Website form - $from'; mail(your@email.com, $from, $subject, $body, $header);
There.
-
Is there an equivalent to * (any) in a $_SESSION? Something like this:
foreach ($_SESSION['cart']['content'][*]['Large'] as $content) {
-
No problem. Thanks, Nightslyr.
-
Is there a shorter way to achieve this in a single foreach()?
foreach ($_SESSION['cart']['content']['sizes'] as $content); foreach ($_SESSION['cart']['content']['sizem'] as $content); foreach ($_SESSION['cart']['content']['sizel'] as $content);
I am thinking something like:
foreach ($_SESSION['cart']['content']['sizes'] && $_SESSION['cart']['content']['sizem'] && $_SESSION['cart']['content']['sizel'] as $content);
or
foreach ($_SESSION['cart']['content'] = ['sizes'] || ['sizem'] || ['sizel'] as $content);
-
Never mind. I have re-wrote the way entities are stored in the session. They are now stored as:
$_SESSION['cart']['content'][$_POST[id]][$_POST[size]] = array ('quantity' => $_POST['quantity'])
opposed to:
$_SESSION['cart']['content'][] = array ('id' => $_POST['id'], 'size' => $_POST['size'], 'quantity' => $_POST['quantity'])
EDIT: Well, this is flawed. But I will figure it out.
-
Unfortunately, I spoke too soon. Now another problem has developed.
If I add an entity with a quantity of 1, and then add the same entity with a quantity of 1, I get a single entity with a quantity of 2, however,
If I add an entity with a quantity of 1, and then add the same entity with a quantity of 3, I get two entities - entity A/quantity-1 & entity A/quantity-3.
Why is this happening?
http://i52.tinypic.com/30bgtww.png
<?php session_start(); if (isset($_SESSION['cart']['content'][$_POST['id']])) { $_SESSION['cart']['content'][$_POST[id]]['quantity'] += $_POST['quantity']; } else { $_SESSION['cart']['content'][] = array ('id' => $_POST['id'], 'size' => $_POST['size'], 'quantity' => $_POST['quantity']); } echo '<DIV class="result">Added to cart.</DIV>'; echo $_SESSION['cart']['content'][$_POST['id']]['quantity']; ?>
Getting "failed to open stream: Permission denied", but I'm sure process is runn
in PHP Coding Help
Posted
No.