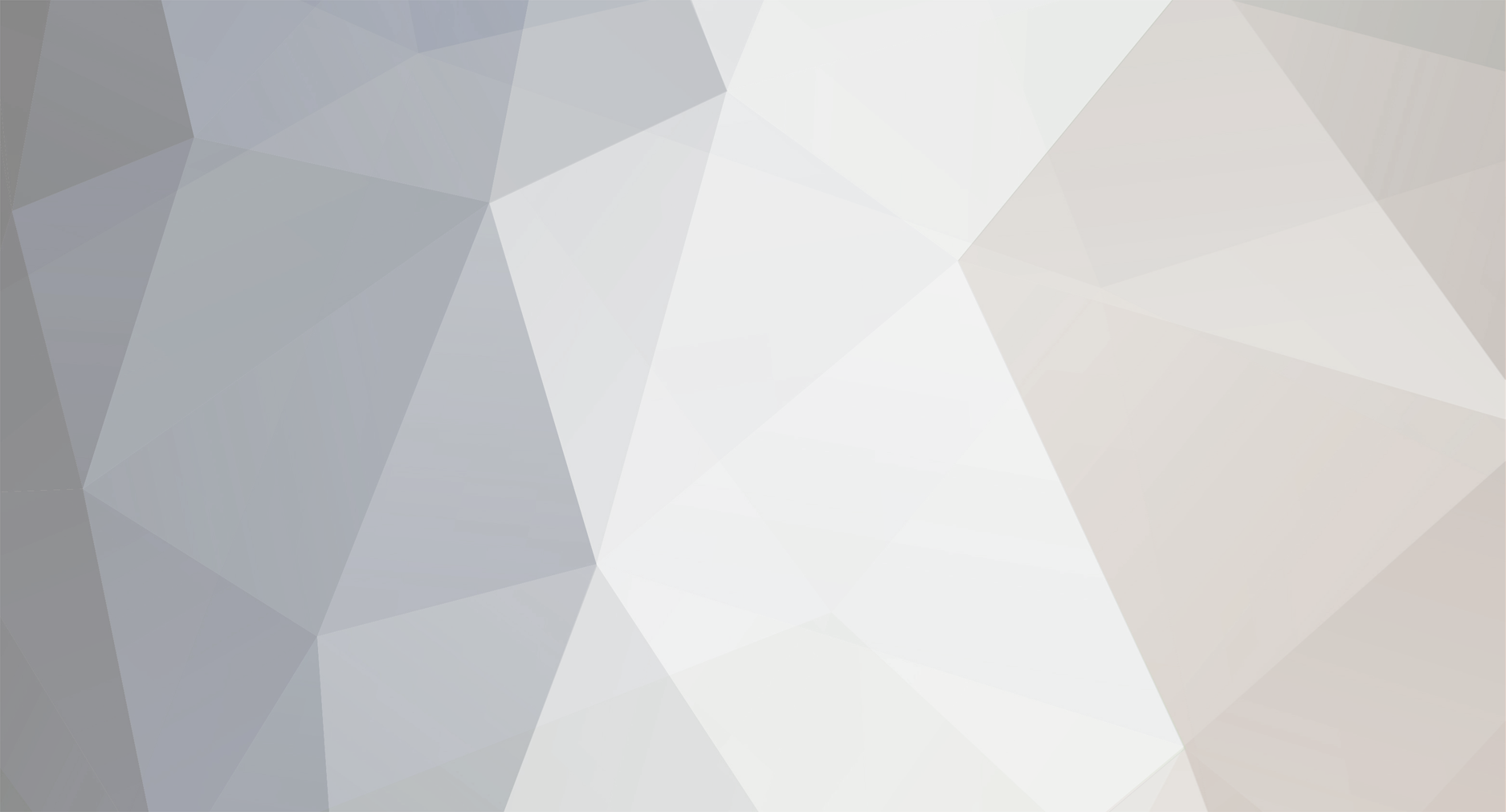
andy_b_1502
Members-
Posts
397 -
Joined
-
Last visited
Everything posted by andy_b_1502
-
Revised register01.php it still doesn't add to the db <?PHP session_start(); include('db.php'); /* set some validation variables */ $error_message = ""; /* DEFINE THE FUNCTION */ /* ============================================== */ /* ============================================== */ /* DO NOT MODIFY THIS FUNCTION */ function Resize_Image($save,$file,$t_w,$t_h,$s_path,$o_path) { $s_path = trim($s_path); $o_path = trim($o_path); $save = $s_path . $save; $file = $o_path . $file; $ext = strtolower(end(explode('.',$save))); list($width, $height) = getimagesize($file) ; if(($width>$t_w) OR ($height>$t_h)) { $r1 = $t_w/$width; $r2 = $t_h/$height; if($r1<$r2) { $size = $t_w/$width; }else{ $size = $t_h/$height; } }else{ $size=1; } $modwidth = $width * $size; $modheight = $height * $size; $tn = imagecreatetruecolor($modwidth, $modheight) ; switch ($ext) { case 'jpg': case 'jpeg': $image = imagecreatefromjpeg($file) ; break; case 'gif': $image = imagecreatefromgif($file) ; break; case 'png': $image = imagecreatefrompng($file) ; break; } imagecopyresampled($tn, $image, 0, 0, 0, 0, $modwidth, $modheight, $width, $height) ; imagejpeg($tn, $save, 100) ; return; } /* END OF RESIZE FUNCTION */ //This is the directory where images will be saved $target = "/home/users/web/b109/ipg.removalspacecom/images/COMPANIES/"; $target = $target . basename( $_FILES['upload']['name']); //This gets all the other information from the form /* ============================================== */ /* ============================================== */ /* YOU NEED TO DO SOME VALIDATION AND SANITIZING OF YOUR FORM DATA */ /* DO NOT MODIFY THESE NEXT 4 VARIABLES */ $normal_Pattern = "/[^a-zA-Z0-9\s\-\'\,\.\_\(\)\&\"\!\`\~\!\@\#\$\%\^\*\+\[\]\{\}\:\;\?\/]/"; $username_Pattern = "/[^a-zA-Z0-9\_]/"; $password_Pattern= "/[^a-zA-Z0-9\_]/"; $phone_Pattern= "/[^0-9\s]/"; if((!isset($_POST['company_name'])) || (strlen(trim($_POST['company_name'])) <5) || (trim($_POST['company_name']) != preg_replace($normal_Pattern, "", trim($_POST['company_name'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid company name<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['company_name'] . "</font><hr>"; }else{ $company_name = trim($_POST['company_name']); } /* END validating company_name */ /* =============================================== */ if((!isset($_POST['contact_name'])) || (strlen(trim($_POST['contact_name'])) <5) || (trim($_POST['contact_name']) != preg_replace($normal_Pattern, "", trim($_POST['contact_name'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid contact name<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['contact_name'] . "</font><hr>"; }else{ $contact_name = trim($_POST['contact_name']); } /* END validating contact_name */ /* =============================================== */ if((!isset($_POST['phone'])) || (strlen(trim($_POST['phone'])) <5) || (trim($_POST['phone']) != preg_replace($phone_Pattern, "", trim($_POST['phone'])))) { /* if it is NOT set, then set the error variable and start building the error message */ $error_message = $error_message . "You must enter a valid phone<br>"; $error_message = $error_message . 'Your invalid phone was: <font color="red">' . $_POST['phone'] . "</font><hr>"; }else{ $phone = trim($_POST['phone']); } /* END validating phone */ /* =============================================== */ /* =============================================== */ /* validating the email */ /* create a function */ function validateEmailAddress($email) { return filter_var($email, FILTER_VALIDATE_EMAIL) && preg_match('/@.+\./', $email); } if(!isset($_POST['email']) || validateEmailAddress($_POST['email']) !=1) { $error_message = $error_message . "You must enter a valid email address<br>"; $error_message = $error_message . 'The invalid email was: <font color="red">' . $_POST['email'] . "</font><hr>"; }else{ $email = $_POST['email']; } /* END validating email */ /* =============================================== */ if((!isset($_POST['street1'])) || (strlen(trim($_POST['street1'])) <5) || (trim($_POST['street1']) != preg_replace($normal_Pattern, "", trim($_POST['street1'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid address<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['street1'] . "</font><hr>"; }else{ $street1 = trim($_POST['street1']); } /* END validating street1 */ /* =============================================== */ if((!isset($_POST['street2'])) || (strlen(trim($_POST['street2'])) <5) || (trim($_POST['street2']) != preg_replace($normal_Pattern, "", trim($_POST['street2'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid address<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['street2'] . "</font><hr>"; }else{ $street2 = trim($_POST['street2']); } /* END validating street2 */ /* =============================================== */ if((!isset($_POST['premiumuser_description'])) || (strlen(trim($_POST['premiumuser_description'])) <5) || (trim($_POST['premiumuser_description']) != preg_replace($normal_Pattern, "", trim($_POST['premiumuser_description'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a description<br>" . "<hr>"; } else{ $premiumuser_description = trim($_POST['premiumuser_description']); } /* END validating premiumuser_description */ /* =============================================== */ if((!isset($_POST['basicpackage_description'])) || (strlen(trim($_POST['basicpackage_description'])) <5) || (trim($_POST['basicpackage_description']) != preg_replace($normal_Pattern, "", trim($_POST['basicpackage_description'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a description<br>" . "<hr>"; } else { $basicpackage_description = trim($_POST['basicpackage_description']); } /* END validating basicpackage_description */ /* =============================================== * /* =============================================== */ /* this section of code will set up an error message for the username if ANY of the conditions occur 1) checks to see if $_POST['username'] is NOT set 2) if length of username is less than 5 3) if username has anything other than letter, numbers or underscores */ if((!isset($_POST['username'])) || (strlen(trim($_POST['username'])) <5) || (trim($_POST['username']) != preg_replace($username_Pattern, "", trim($_POST['username'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid username<br>"; $error_message = $error_message . "Valid names are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['username'] . "</font><hr>"; } /* END validating username */ /* =============================================== */ /* =============================================== */ /* this section of code will set up an error message for the password if ANY of the conditions occur 1) checks to see if $_POST['upassword'] is NOT set 2) if length of upassword is less than 5 3) if upassword has anything other than letter, numbers or underscores */ if((!isset($_POST['password'])) || (strlen(trim($_POST['password'])) <5) || (trim($_POST['password']) != preg_replace($pawword_Pattern, "", trim($_POST['password'])))) { /* if it is NOT set, then set the error variable and start building the error message */ $error_message = $error_message . "You must enter a valid password<br>"; $error_message = $error_message . "Valid passwords are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid password was: <font color="red">' . $_POST['password'] . "</font><hr>"; }else{ $password = trim($_POST['password']); } /* END validating password */ /* =============================================== */ /* =============================================== */ /* check to see if username is already taken */ $username = mysql_real_escape_string(trim($_POST['username'])); $query1 = "SELECT username from companies WHERE username = '$username'"; $result1 = mysql_query($query1) or die(mysql_error()); $count = mysql_num_rows($result1); if($count>0) { $error_message = $error_message . 'The username: <font color="red">' . $_POST['username'] . "</font> is taken.<hr>"; } /* =============================================== */ /* if any of the post variables are invalid */ /* set the session variable and send back to the form page */ if(strlen(trim($error_message))>0) { $_SESSION['error_message'] =$error_message; header("Location: register00.php"); exit(); } /* =============================================== */ $uploadDir = 'images/COMPANIES'; /* main picture folder */ $max_height = 450; /* largest height you allowed; 0 means any */ $max_width = 450; /* largest width you allowed; 0 means any */ $max_file = 2000000; /* set the max file size in bytes */ $image_overwrite = 1; /* 0 means overwite; 1 means new name */ /* add or delete allowed image types */ $allowed_type01 = array( "image/gif", "image/pjpeg", "image/jpeg", "image/png", "image/x-png", "image/jpg"); $do_thumb = 1; /* 1 make thumbnails; 0 means do NOT make */ $thumbDir = "/images/thumbs"; /* thumbnail folder */ $thumb_prefix = ""; /* prefix for thumbnails */ $thumb_width = 90; /* max thumb width */ $thumb_height = 70; // max thumb height //Writes the photo to the server if(move_uploaded_file($_FILES['upload']['tmp_name'], $target)) { /* HERE IS WHERE WE WILL DO THE ACTUAL RESIZING */ /* ============================================== */ /* ============================================== */ /* THESE SIX PARAMETERS MAY BE CHANGED TO SUIT YOUR NEEDS */ $o_path ="images/COMPANIES/"; $s_path = "images/thumbs/"; $file = $upload; $save = $file; $t_w = 200; $t_h = 150; /* ============================================== */ /* ============================================== */ /* DO NOT CHANGE THIS NEXT LINE */ Resize_Image($save,$file,$t_w,$t_h,$s_path,$o_path); //Tells you if its all ok /* ============================================== */ /* ============================================== */ /* PROVIDE A WAY FOR THEM TO GO SOMWHERE */ echo "The file ". $file . " has been uploaded, and your information has been added to the directory"; }else { //Gives and error if its not /* ============================================== */ /* ============================================== */ /* PROVIDE A WAY FOR THEM TO GO SOMWHERE */ echo "Sorry, there was a problem uploading your file."; exit(); } /* =============================================== */ /* PREPARE DATA FOR INSERTION INTO TABLE */ /* FUNCTION TO CREATE SALT */ function createSalt() { $string = md5(uniqid(rand(), true)); return substr($string, 0, 3); } //Writes the information to the database /* ============================================== */ /* ============================================== */ /* ALWAYS WRITE YOUR QUERIES AS STRINGS THAT WAY WHEN TESTING, YOU CAN MAKE SURE THAT THE VALUES CONTAIN WHAT YOU EXPECT */ $salt = createsalt(); $password = trim($_POST['password']); $hash = hash('sha256', $salt, $password); $approved = 0; $username = mysql_real_escape_string(trim($_POST['username'])); $email = mysql_real_escape_string(trim($_POST['email'])); $query ="INSERT INTO `companies` (company_name, contact_name, location, postcode, street1, street2, city, phone, email, basicpackage_description, premiumuser_description, password, salt, approved, upload) VALUES ('$company_name', '$contact_name', '$location', '$postcode', '$street1', '$street2', '$city', '$phone', '$email', '$basicpackage_description', '$premiumuser_description', '$password', '$salt', '$approved', '$upload')"; $result = mysql_query($query) or die(mysql_error()); /* =============================================== */ /* at this point we can send an email to the admin as well as the user. DO NOT send the user's password to ANYONE!!!! */ ?> Thank you for registering.<br>; Your account will be approved and activated within 24 hours.<br><br> Click here to return to the <a href="index.php">main page</a>.
-
drummin: line added. jesirose: like this?: echo "$query" or die; If all is correct, i have just tested.... no joy still..
-
Its not doing anything so im guessing its not liking some of the variables or something? Its just reloading to the register form. <?php $query ="INSERT INTO companies (company_name, contact_name, location, postcode, street1, street2, city, phone, email, basicpackage_description, premiumuser_description, password, salt, approved, upload) VALUES ('$company_name', '$contact_name', '$location', '$postcode', '$street1', '$street2', '$city', '$phone', '$email', '$basicpackage_description', '$premiumuser_description', '$password', '$salt', '$approved', '$upload')"; $result = mysql_query($query) or die(mysql_error()); echo "$query"; ?>
-
Thanks, but that hasn't worked? not sure whats wrong with it. <?php $query ="INSERT INTO companies (company_name, contact_name, location, postcode, street1, street2, city, phone, email, basicpackage_description, premiumuser_description, password, salt, approved, upload) VALUES ('$company_name', '$contact_name', '$location', '$postcode', '$street1', '$street2', '$city', '$phone', '$email', '$basicpackage_description', '$premiumuser_description', '$password', '$salt', '$approved', '$upload')"; $result = mysql_query($query) or die(mysql_error()); ?>
-
Any ideas guys? <?PHP session_start(); include('db.php'); /* set some validation variables */ $error_message = ""; /* DEFINE THE FUNCTION */ /* ============================================== */ /* ============================================== */ /* DO NOT MODIFY THIS FUNCTION */ function Resize_Image($save,$file,$t_w,$t_h,$s_path,$o_path) { $s_path = trim($s_path); $o_path = trim($o_path); $save = $s_path . $save; $file = $o_path . $file; $ext = strtolower(end(explode('.',$save))); list($width, $height) = getimagesize($file) ; if(($width>$t_w) OR ($height>$t_h)) { $r1 = $t_w/$width; $r2 = $t_h/$height; if($r1<$r2) { $size = $t_w/$width; }else{ $size = $t_h/$height; } }else{ $size=1; } $modwidth = $width * $size; $modheight = $height * $size; $tn = imagecreatetruecolor($modwidth, $modheight) ; switch ($ext) { case 'jpg': case 'jpeg': $image = imagecreatefromjpeg($file) ; break; case 'gif': $image = imagecreatefromgif($file) ; break; case 'png': $image = imagecreatefrompng($file) ; break; } imagecopyresampled($tn, $image, 0, 0, 0, 0, $modwidth, $modheight, $width, $height) ; imagejpeg($tn, $save, 100) ; return; } /* END OF RESIZE FUNCTION */ //This is the directory where images will be saved $target = "/home/users/web/b109/ipg.removalspacecom/images/COMPANIES/"; $target = $target . basename( $_FILES['upload']['name']); // Connects to your Database session_start(); include ('db.php'); //This gets all the other information from the form /* ============================================== */ /* ============================================== */ /* YOU NEED TO DO SOME VALIDATION AND SANITIZING OF YOUR FORM DATA */ if((!isset($_POST['company_name'])) || (strlen(trim($_POST['company_name'])) <5) || (trim($_POST['company_name']) != preg_replace("/[^a-zA-Z0-9\s\-\'\,\.\_]/", "", trim($_POST['company_name'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid company name<br>"; $error_message = $error_message . "Valid names are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['company_name'] . "</font><hr>"; } /* END validating company_name */ /* =============================================== */ if((!isset($_POST['contact_name'])) || (strlen(trim($_POST['contact_name'])) <5) || (trim($_POST['contact_name']) != preg_replace("/[^a-zA-Z0-9\s\-\'\,\.\_]/", "", trim($_POST['contact_name'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid contact name<br>"; $error_message = $error_message . "Valid names are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['contact_name'] . "</font><hr>"; } /* END validating contact_name */ /* =============================================== */ if((!isset($_POST['phone'])) || (strlen(trim($_POST['phone'])) <5) || (trim($_POST['phone']) != preg_replace("/[^0-9\s\-\_]/", "", trim($_POST['phone'])))) { /* if it is NOT set, then set the error variable and start building the error message */ $error_message = $error_message . "You must enter a valid phone<br>"; $error_message = $error_message . "Valid phones are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid phone was: <font color="red">' . $_POST['phone'] . "</font><hr>"; }else{ $phone = trim($_POST['phone']); } /* END validating phone */ /* =============================================== */ /* =============================================== */ /* validating the email */ /* create a function */ function validateEmailAddress($email) { return filter_var($email, FILTER_VALIDATE_EMAIL) && preg_match('/@.+\./', $email); } if(!isset($_POST['email']) || validateEmailAddress($_POST['email']) !=1) { $error_message = $error_message . "You must enter a valid email address<br>"; $error_message = $error_message . 'The invalid email was: <font color="red">' . $_POST['email'] . "</font><hr>"; } /* END validating email */ /* =============================================== */ if((!isset($_POST['street1'])) || (strlen(trim($_POST['street1'])) <5) || (trim($_POST['street1']) != preg_replace("/[^a-zA-Z0-9\s\-\'\,\.\_]/", "", trim($_POST['street1'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid address<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['street1'] . "</font><hr>"; } /* END validating street1 */ /* =============================================== */ if((!isset($_POST['street2'])) || (strlen(trim($_POST['street2'])) <5) || (trim($_POST['street2']) != preg_replace("/[^a-zA-Z0-9\s\-\'\,\.\_]/", "", trim($_POST['street2'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid address<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['street2'] . "</font><hr>"; } /* END validating street2 */ /* =============================================== */ if((!isset($_POST['premiumuser_description'])) || (strlen(trim($_POST['premiumuser_description'])) <5) || (trim($_POST['premiumuser_description']) != preg_replace("/[^a-zA-Z0-9\s\-\'\,\.\_]/", "", trim($_POST['premiumuser_description'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid address<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['premiumuser_description'] . "</font><hr>"; } /* END validating premiumuser_description */ /* =============================================== * /* =============================================== */ /* this section of code will set up an error message for the username if ANY of the conditions occur 1) checks to see if $_POST['username'] is NOT set 2) if length of username is less than 5 3) if username has anything other than letter, numbers or underscores */ if((!isset($_POST['username'])) || (strlen(trim($_POST['username'])) <5) || (trim($_POST['username']) != preg_replace("/[^a-zA-Z0-9\_]/", "", trim($_POST['username'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid username<br>"; $error_message = $error_message . "Valid names are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['username'] . "</font><hr>"; } /* END validating username */ /* =============================================== */ /* =============================================== */ /* this section of code will set up an error message for the password if ANY of the conditions occur 1) checks to see if $_POST['upassword'] is NOT set 2) if length of upassword is less than 5 3) if upassword has anything other than letter, numbers or underscores */ if((!isset($_POST['password'])) || (strlen(trim($_POST['password'])) <5) || (trim($_POST['password']) != preg_replace("/[^a-zA-Z0-9\_]/", "", trim($_POST['password'])))) { /* if it is NOT set, then set the error variable and start building the error message */ $error_message = $error_message . "You must enter a valid password<br>"; $error_message = $error_message . "Valid passwords are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid password was: <font color="red">' . $_POST['password'] . "</font><hr>"; }else{ $password = trim($_POST['password']); } /* END validating password */ /* =============================================== */ /* =============================================== */ /* check to see if username is already taken */ $username = mysql_real_escape_string(trim($_POST['username'])); $query1 = "SELECT username from companies WHERE username = '$username'"; $result1 = mysql_query($query1) or die(mysql_error()); $count = mysql_num_rows($result1); if($count>0) { $error_message = $error_message . 'The username: <font color="red">' . $_POST['username'] . "</font> is taken.<hr>"; } /* =============================================== */ /* if any of the post variables are invalid */ /* set the session variable and send back to the form page */ if(strlen(trim($error_message))>0) { $_SESSION['error_message'] =$error_message; header("Location: register00.php"); exit(); } /* =============================================== */ $uploadDir = 'images/COMPANIES'; /* main picture folder */ $max_height = 450; /* largest height you allowed; 0 means any */ $max_width = 450; /* largest width you allowed; 0 means any */ $max_file = 2000000; /* set the max file size in bytes */ $image_overwrite = 1; /* 0 means overwite; 1 means new name */ /* add or delete allowed image types */ $allowed_type01 = array( "image/gif", "image/pjpeg", "image/jpeg", "image/png", "image/x-png", "image/jpg"); $do_thumb = 1; /* 1 make thumbnails; 0 means do NOT make */ $thumbDir = "/images/thumbs"; /* thumbnail folder */ $thumb_prefix = ""; /* prefix for thumbnails */ $thumb_width = 90; /* max thumb width */ $thumb_height = 70; // max thumb height //Writes the photo to the server if(move_uploaded_file($_FILES['upload']['tmp_name'], $target)) { /* HERE IS WHERE WE WILL DO THE ACTUAL RESIZING */ /* ============================================== */ /* ============================================== */ /* THESE SIX PARAMETERS MAY BE CHANGED TO SUIT YOUR NEEDS */ $o_path ="images/COMPANIES/"; $s_path = "images/thumbs/"; $file = $upload; $save = $file; $t_w = 200; $t_h = 150; /* ============================================== */ /* ============================================== */ /* DO NOT CHANGE THIS NEXT LINE */ Resize_Image($save,$file,$t_w,$t_h,$s_path,$o_path); //Tells you if its all ok /* ============================================== */ /* ============================================== */ /* PROVIDE A WAY FOR THEM TO GO SOMWHERE */ echo "The file ". $file . " has been uploaded, and your information has been added to the directory"; }else { //Gives and error if its not /* ============================================== */ /* ============================================== */ /* PROVIDE A WAY FOR THEM TO GO SOMWHERE */ echo "Sorry, there was a problem uploading your file."; } /* =============================================== */ /* PREPARE DATA FOR INSERTION INTO TABLE */ /* FUNCTION TO CREATE SALT */ function createSalt() { $string = md5(uniqid(rand(), true)); return substr($string, 0, 3); } //Writes the information to the database /* ============================================== */ /* ============================================== */ /* ALWAYS WRITE YOUR QUERIES AS STRINGS THAT WAY WHEN TESTING, YOU CAN MAKE SURE THAT THE VALUES CONTAIN WHAT YOU EXPECT */ $salt = createsalt(); $passwod = trim($_POST['password']); $hash = hash('sha256', $salt, $password); $approved = 0; $username = mysql_real_escape_string(trim($_POST['username'])); $email = mysql_real_escape_string(trim($_POST['email'])); $query ="INSERT INTO `companies` (company_name, contact_name, location, postcode, street1, street2, city, phone, email, basicpackage_description, premiumuser_description, password, salt, approved, upload) VALUES ('$company_name', '$contact_name', '$location', '$postcode', '$street1', '$street2', '$city', '$phone', '$email', '$basicpackage_description', '$premiumuser_description', '$password', '$salt', '$approved', '$upload')"; $result = mysql_query($query) or die(mysql_error()); /* =============================================== */ /* at this point we can send an email to the admin as well as the user. DO NOT send the user's password to ANYONE!!!! */ ?>
-
Hi everyone... My script register01.php isnt working as expected, it should take variables from register00.php and insert them into the db. It is currently re-loading itself and not inserting, could you take a look at this for me: register00.php <form enctype="multipart/form-data" method="post" action="register01.php"> <table width="316" height="120" border="0"> <tr><td colspan=2><h1>Register/Sign Up</h1></td></tr> <tr><td>Company Name:</td><td> <input name="company_name" type="text" id="company_name"> </td></tr> <tr><td>Contact Name:</td><td> <input name="contact_name" type="text" id="contact_name"> </td></tr> <tr><td>Contact Number:</td><td> <input name="phone" type="number" id="phone" value="incl. area code"> </td></tr> <tr><td>Address line 1:</td><td> <input name="street1" type="text" id="street1"> </td></tr> <tr><td>Address line 2:</td><td> <input name="street2" type="text" id="street1"> </td></tr> <tr><td>Area:</td><td> <input name="location" type="text" id="location"> </td></tr> <tr><td>City:</td><td> <input name="city" type="text" id="city"> </td></tr> <tr><td>Postcode:</td><td> <input name="postcode" type="text" id="postcode"> </td></tr> <tr><td>Username:</td><td> <input name="username" type="text" id="username"> </td></tr> <tr><td>Password:</td><td> <input name="password" type="password" class="style7" id="password"> </td></tr> <tr><td>Email:</td><td> <input name="email" type="text" class="style7" id="email"> </td></tr> <tr><td>Company Logo:</td><td> <input name="upload" type="file" class="style7" id="upload"> </td></tr> <tr><td>Company Description:</td><td> <textarea rows="20" cols="50" name="premiumuser_description" id="premiumuser_description"></textarea> </td></tr> <tr><td> <input name="Submit" type="submit" value="Register" /> </td></tr> </table> register01.php <?PHP session_start(); include('db.php'); /* set some validation variables */ $error_message = ""; /* DEFINE THE FUNCTION */ /* ============================================== */ /* ============================================== */ /* DO NOT MODIFY THIS FUNCTION */ function Resize_Image($save,$file,$t_w,$t_h,$s_path,$o_path) { $s_path = trim($s_path); $o_path = trim($o_path); $save = $s_path . $save; $file = $o_path . $file; $ext = strtolower(end(explode('.',$save))); list($width, $height) = getimagesize($file) ; if(($width>$t_w) OR ($height>$t_h)) { $r1 = $t_w/$width; $r2 = $t_h/$height; if($r1<$r2) { $size = $t_w/$width; }else{ $size = $t_h/$height; } }else{ $size=1; } $modwidth = $width * $size; $modheight = $height * $size; $tn = imagecreatetruecolor($modwidth, $modheight) ; switch ($ext) { case 'jpg': case 'jpeg': $image = imagecreatefromjpeg($file) ; break; case 'gif': $image = imagecreatefromgif($file) ; break; case 'png': $image = imagecreatefrompng($file) ; break; } imagecopyresampled($tn, $image, 0, 0, 0, 0, $modwidth, $modheight, $width, $height) ; imagejpeg($tn, $save, 100) ; return; } /* END OF RESIZE FUNCTION */ //This is the directory where images will be saved $target = "/home/users/web/b109/ipg.removalspacecom/images/COMPANIES/"; $target = $target . basename( $_FILES['upload']['name']); // Connects to your Database session_start(); include ('db.php'); //This gets all the other information from the form /* ============================================== */ /* ============================================== */ /* YOU NEED TO DO SOME VALIDATION AND SANITIZING OF YOUR FORM DATA */ if((!isset($_POST['company_name'])) || (strlen(trim($_POST['company_name'])) <5) || (trim($_POST['company_name']) != preg_replace("/[^a-zA-Z0-9\s\-\'\,\.\_]/", "", trim($_POST['company_name'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid company name<br>"; $error_message = $error_message . "Valid names are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['company_name'] . "</font><hr>"; } /* END validating company_name */ /* =============================================== */ if((!isset($_POST['contact_name'])) || (strlen(trim($_POST['contact_name'])) <5) || (trim($_POST['contact_name']) != preg_replace("/[^a-zA-Z0-9\s\-\'\,\.\_]/", "", trim($_POST['contact_name'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid contact name<br>"; $error_message = $error_message . "Valid names are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['contact_name'] . "</font><hr>"; } /* END validating contact_name */ /* =============================================== */ if((!isset($_POST['phone'])) || (strlen(trim($_POST['phone'])) <5) || (trim($_POST['phone']) != preg_replace("/[^0-9\s\-\_]/", "", trim($_POST['phone'])))) { /* if it is NOT set, then set the error variable and start building the error message */ $error_message = $error_message . "You must enter a valid phone<br>"; $error_message = $error_message . "Valid phones are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid phone was: <font color="red">' . $_POST['phone'] . "</font><hr>"; }else{ $phone = trim($_POST['phone']); } /* END validating phone */ /* =============================================== */ /* =============================================== */ /* validating the email */ /* create a function */ function validateEmailAddress($email) { return filter_var($email, FILTER_VALIDATE_EMAIL) && preg_match('/@.+\./', $email); } if(!isset($_POST['email']) || validateEmailAddress($_POST['email']) !=1) { $error_message = $error_message . "You must enter a valid email address<br>"; $error_message = $error_message . 'The invalid email was: <font color="red">' . $_POST['email'] . "</font><hr>"; } /* END validating email */ /* =============================================== */ if((!isset($_POST['street1'])) || (strlen(trim($_POST['street1'])) <5) || (trim($_POST['street1']) != preg_replace("/[^a-zA-Z0-9\s\-\'\,\.\_]/", "", trim($_POST['street1'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid address<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['street1'] . "</font><hr>"; } /* END validating street1 */ /* =============================================== */ if((!isset($_POST['street2'])) || (strlen(trim($_POST['street2'])) <5) || (trim($_POST['street2']) != preg_replace("/[^a-zA-Z0-9\s\-\'\,\.\_]/", "", trim($_POST['street2'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid address<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['street2'] . "</font><hr>"; } /* END validating street2 */ /* =============================================== */ if((!isset($_POST['premiumuser_description'])) || (strlen(trim($_POST['premiumuser_description'])) <5) || (trim($_POST['premiumuser_description']) != preg_replace("/[^a-zA-Z0-9\s\-\'\,\.\_]/", "", trim($_POST['premiumuser_description'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid address<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['premiumuser_description'] . "</font><hr>"; } /* END validating premiumuser_description */ /* =============================================== * /* =============================================== */ /* this section of code will set up an error message for the username if ANY of the conditions occur 1) checks to see if $_POST['username'] is NOT set 2) if length of username is less than 5 3) if username has anything other than letter, numbers or underscores */ if((!isset($_POST['username'])) || (strlen(trim($_POST['username'])) <5) || (trim($_POST['username']) != preg_replace("/[^a-zA-Z0-9\_]/", "", trim($_POST['username'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid username<br>"; $error_message = $error_message . "Valid names are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['username'] . "</font><hr>"; } /* END validating username */ /* =============================================== */ /* =============================================== */ /* this section of code will set up an error message for the password if ANY of the conditions occur 1) checks to see if $_POST['upassword'] is NOT set 2) if length of upassword is less than 5 3) if upassword has anything other than letter, numbers or underscores */ if((!isset($_POST['password'])) || (strlen(trim($_POST['password'])) <5) || (trim($_POST['password']) != preg_replace("/[^a-zA-Z0-9\_]/", "", trim($_POST['password'])))) { /* if it is NOT set, then set the error variable and start building the error message */ $error_message = $error_message . "You must enter a valid password<br>"; $error_message = $error_message . "Valid passwords are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid password was: <font color="red">' . $_POST['password'] . "</font><hr>"; }else{ $password = trim($_POST['password']); } /* END validating password */ /* =============================================== */ /* =============================================== */ /* check to see if username is already taken */ $username = mysql_real_escape_string(trim($_POST['username'])); $query1 = "SELECT username from companies WHERE username = '$username'"; $result1 = mysql_query($query1) or die(mysql_error()); $count = mysql_num_rows($result1); if($count>0) { $error_message = $error_message . 'The username: <font color="red">' . $_POST['username'] . "</font> is taken.<hr>"; } /* =============================================== */ /* if any of the post variables are invalid */ /* set the session variable and send back to the form page */ if(strlen(trim($error_message))>0) { $_SESSION['error_message'] =$error_message; header("Location: register00.php"); exit(); } /* =============================================== */ $uploadDir = 'images/COMPANIES'; /* main picture folder */ $max_height = 450; /* largest height you allowed; 0 means any */ $max_width = 450; /* largest width you allowed; 0 means any */ $max_file = 2000000; /* set the max file size in bytes */ $image_overwrite = 1; /* 0 means overwite; 1 means new name */ /* add or delete allowed image types */ $allowed_type01 = array( "image/gif", "image/pjpeg", "image/jpeg", "image/png", "image/x-png", "image/jpg"); $do_thumb = 1; /* 1 make thumbnails; 0 means do NOT make */ $thumbDir = "/images/thumbs"; /* thumbnail folder */ $thumb_prefix = ""; /* prefix for thumbnails */ $thumb_width = 90; /* max thumb width */ $thumb_height = 70; // max thumb height //Writes the photo to the server if(move_uploaded_file($_FILES['upload']['tmp_name'], $target)) { /* HERE IS WHERE WE WILL DO THE ACTUAL RESIZING */ /* ============================================== */ /* ============================================== */ /* THESE SIX PARAMETERS MAY BE CHANGED TO SUIT YOUR NEEDS */ $o_path ="images/COMPANIES/"; $s_path = "images/thumbs/"; $file = $upload; $save = $file; $t_w = 200; $t_h = 150; /* ============================================== */ /* ============================================== */ /* DO NOT CHANGE THIS NEXT LINE */ Resize_Image($save,$file,$t_w,$t_h,$s_path,$o_path); //Tells you if its all ok /* ============================================== */ /* ============================================== */ /* PROVIDE A WAY FOR THEM TO GO SOMWHERE */ echo "The file ". $file . " has been uploaded, and your information has been added to the directory"; }else { //Gives and error if its not /* ============================================== */ /* ============================================== */ /* PROVIDE A WAY FOR THEM TO GO SOMWHERE */ echo "Sorry, there was a problem uploading your file."; } /* =============================================== */ /* PREPARE DATA FOR INSERTION INTO TABLE */ /* FUNCTION TO CREATE SALT */ function createSalt() { $string = md5(uniqid(rand(), true)); return substr($string, 0, 3); } //Writes the information to the database /* ============================================== */ /* ============================================== */ /* ALWAYS WRITE YOUR QUERIES AS STRINGS THAT WAY WHEN TESTING, YOU CAN MAKE SURE THAT THE VALUES CONTAIN WHAT YOU EXPECT */ $salt = createsalt(); $passwod = trim($_POST['password']); $hash = hash('sha256', $salt, $password); $approved = 0; $username = mysql_real_escape_string(trim($_POST['username'])); $email = mysql_real_escape_string(trim($_POST['email'])); $query ="INSERT INTO `companies` (company_name, contact_name, location, postcode, street1, street2, city, phone, email, basicpackage_description, premiumuser_description, password, salt, approved, upload) VALUES ('$company_name', '$contact_name', '$location', '$postcode', '$street1', '$street2', '$city', '$phone', '$email', '$basicpackage_description', '$premiumuser_description', '$password', '$salt', '$approved', '$upload')"; $result = mysql_query($query) or die(mysql_error()); /* =============================================== */ /* at this point we can send an email to the admin as well as the user. DO NOT send the user's password to ANYONE!!!! */ ?> Thank you for registering.<br>; Your account will be approved and activated within 24 hours.<br><br> Click here to return to the <a href="index.php">main page</a>.
-
And why is it attempting to find a file that doesnt exist? i thought session start() started a session?
-
on the server?
-
right okay so ive changed it to: session.save_path = "removalspacecom.ipagemysql.com/sessions" I get this: Warning: session_start() [function.session-start]: open(removalspacecom.ipagemysql.com/sessions/sess_08873b0d5b9926bc4d94bc85797f1c4e, O_RDWR) failed: No such file or directory (2) in /hermes/bosweb25a/b109/ipg.removalspacecom/admin01.php on line 2 Warning: session_start() [function.session-start]: Cannot send session cookie - headers already sent by (output started at /hermes/bosweb25a/b109/ipg.removalspacecom/admin01.php:2) in /hermes/bosweb25a/b109/ipg.removalspacecom/admin01.php on line 2 Warning: session_start() [function.session-start]: Cannot send session cache limiter - headers already sent (output started at /hermes/bosweb25a/b109/ipg.removalspacecom/admin01.php:2) in /hermes/bosweb25a/b109/ipg.removalspacecom/admin01.php on line 2 There are 1 removalspace users so far. Company Name Contact Name Contact Number Email Address Line 1 Address Line 2 Location Postcode Basic Members Upgraded Users Company Logo Approved none none none [email protected] none none none none Current level = 1 Level 1 - Free Level 2 - Basic Level 3 - Premium Level 0 - Do Not Display Warning: Unknown: open(removalspacecom.ipagemysql.com/sessions/sess_08873b0d5b9926bc4d94bc85797f1c4e, O_RDWR) failed: No such file or directory (2) in Unknown on line 0 Warning: Unknown: Failed to write session data (files). Please verify that the current setting of session.save_path is correct (removalspacecom.ipagemysql.com/sessions) in Unknown on line 0 Any ideas guys?
-
if i create a new folder on my file manager called 'sessions' and the path to that is: /sessions and i change the session.save_path to: session.save_path = /sessions Will it work without it crashing/messing up? just checking before i try it?
-
On my control panel i locate my php.ini file.... In the session section, session.save_path = home/temp etc... here is the whole section: [session] ; Handler used to store/retrieve data. session.save_handler = files ; Argument passed to save_handler. In the case of files, this is the path ; where data files are stored. Note: Windows users have to change this ; variable in order to use PHP's session functions. ; ; As of PHP 4.0.1, you can define the path as: ; ; session.save_path = "N;/path" ; ; where N is an integer. Instead of storing all the session files in ; /path, what this will do is use subdirectories N-levels deep, and ; store the session data in those directories. This is useful if you ; or your OS have problems with lots of files in one directory, and is ; a more efficient layout for servers that handle lots of sessions. ; ; NOTE 1: PHP will not create this directory structure automatically. ; You can use the script in the ext/session dir for that purpose. ; NOTE 2: See the section on garbage collection below if you choose to ; use subdirectories for session storage ; ; The file storage module creates files using mode 600 by default. ; You can change that by using ; ; session.save_path = "N;MODE;/path" ; ; where MODE is the octal representation of the mode. Note that this ; does not overwrite the process's umask. session.save_path = /home/users/web/b109/ipg.removalspacecom/cgi-bin/tmp ; Whether to use cookies. session.use_cookies = 1 ; This option enables administrators to make their users invulnerable to ; attacks which involve passing session ids in URLs; defaults to 0. ; session.use_only_cookies = 1 ; Name of the session (used as cookie name). session.name = PHPSESSID ; Initialize session on request startup. session.auto_start = 0 ; Lifetime in seconds of cookie or, if 0, until browser is restarted. session.cookie_lifetime = 0 ; The path for which the cookie is valid. session.cookie_path = / ; The domain for which the cookie is valid. session.cookie_domain = ; Handler used to serialize data. php is the standard serializer of PHP. session.serialize_handler = php ; Define the probability that the 'garbage collection' process is started ; on every session initialization. ; The probability is calculated by using gc_probability/gc_divisor, ; e.g. 1/100 means there is a 1% chance that the GC process starts ; on each request. session.gc_probability = 1 session.gc_divisor = 100 ; After this number of seconds, stored data will be seen as 'garbage' and ; cleaned up by the garbage collection process. session.gc_maxlifetime = 1440 ; NOTE: If you are using the subdirectory option for storing session files ; (see session.save_path above), then garbage collection does *not* ; happen automatically. You will need to do your own garbage ; collection through a shell script, cron entry, or some other method. ; For example, the following script would is the equivalent of ; setting session.gc_maxlifetime to 1440 (1440 seconds = 24 minutes): ; cd /path/to/sessions; find -cmin +24 | xargs rm ; PHP 4.2 and less have an undocumented feature/bug that allows you to ; to initialize a session variable in the global scope, albeit register_globals ; is disabled. PHP 4.3 and later will warn you, if this feature is used. ; You can disable the feature and the warning separately. At this time, ; the warning is only displayed, if bug_compat_42 is enabled. session.bug_compat_42 = 1 session.bug_compat_warn = 1 ; Check HTTP Referer to invalidate externally stored URLs containing ids. ; HTTP_REFERER has to contain this substring for the session to be ; considered as valid. session.referer_check = ; How many bytes to read from the file. session.entropy_length = 0 ; Specified here to create the session id. session.entropy_file = ;session.entropy_length = 16 ;session.entropy_file = /dev/urandom ; Set to {nocache,private,public,} to determine HTTP caching aspects ; or leave this empty to avoid sending anti-caching headers. session.cache_limiter = nocache ; Document expires after n minutes. session.cache_expire = 180 ; trans sid support is disabled by default. ; Use of trans sid may risk your users security. ; Use this option with caution. ; - User may send URL contains active session ID ; to other person via. email/irc/etc. ; - URL that contains active session ID may be stored ; in publically accessible computer. ; - User may access your site with the same session ID ; always using URL stored in browser's history or bookmarks. session.use_trans_sid = 0 ; Select a hash function ; 0: MD5 (128 bits) ; 1: SHA-1 (160 bits) session.hash_function = 0 ; Define how many bits are stored in each character when converting ; the binary hash data to something readable. ; ; 4 bits: 0-9, a-f ; 5 bits: 0-9, a-v ; 6 bits: 0-9, a-z, A-Z, "-", "," session.hash_bits_per_character = 4 ; The URL rewriter will look for URLs in a defined set of HTML tags. ; form/fieldset are special; if you include them here, the rewriter will ; add a hidden <input> field with the info which is otherwise appended ; to URLs. If you want XHTML conformity, remove the form entry. ; Note that all valid entries require a "=", even if no value follows. url_rewriter.tags = "a=href,area=href,frame=src,input=src,form=,fieldset="
-
That was just a defualt .ini uploaded.... mines this: session.save_path = /home/users/web/b109/ipg.removalspacecom/cgi-bin/tmp on my server, this should in theory work shouldnt it, meaning that the problem is elswhere? is that true??
-
[session] ; Handler used to store/retrieve data. session.save_handler = files ; Argument passed to save_handler. In the case of files, this is the path ; where data files are stored. Note: Windows users have to change this ; variable in order to use PHP's session functions. ; ; As of PHP 4.0.1, you can define the path as: ; ; session.save_path = "N;/path" ; ; where N is an integer. Instead of storing all the session files in ; /path, what this will do is use subdirectories N-levels deep, and ; store the session data in those directories. This is useful if you ; or your OS have problems with lots of files in one directory, and is ; a more efficient layout for servers that handle lots of sessions. ; ; NOTE 1: PHP will not create this directory structure automatically. ; You can use the script in the ext/session dir for that purpose. ; NOTE 2: See the section on garbage collection below if you choose to ; use subdirectories for session storage ; ; The file storage module creates files using mode 600 by default. ; You can change that by using ; ; session.save_path = "N;MODE;/path" ; ; where MODE is the octal representation of the mode. Note that this ; does not overwrite the process's umask. ;session.save_path = "/tmp" ; Whether to use cookies. session.use_cookies = 1 ; This option enables administrators to make their users invulnerable to ; attacks which involve passing session ids in URLs; defaults to 0. ; session.use_only_cookies = 1 ; Name of the session (used as cookie name). session.name = PHPSESSID ; Initialize session on request startup. session.auto_start = 0 ; Lifetime in seconds of cookie or, if 0, until browser is restarted. session.cookie_lifetime = 0 ; The path for which the cookie is valid. session.cookie_path = / ; The domain for which the cookie is valid. session.cookie_domain = ; Handler used to serialize data. php is the standard serializer of PHP. session.serialize_handler = php ; Define the probability that the 'garbage collection' process is started ; on every session initialization. ; The probability is calculated by using gc_probability/gc_divisor, ; e.g. 1/100 means there is a 1% chance that the GC process starts ; on each request. session.gc_probability = 1 session.gc_divisor = 100 ; After this number of seconds, stored data will be seen as 'garbage' and ; cleaned up by the garbage collection process. session.gc_maxlifetime = 1440 ; NOTE: If you are using the subdirectory option for storing session files ; (see session.save_path above), then garbage collection does *not* ; happen automatically. You will need to do your own garbage ; collection through a shell script, cron entry, or some other method. ; For example, the following script would is the equivalent of ; setting session.gc_maxlifetime to 1440 (1440 seconds = 24 minutes): ; cd /path/to/sessions; find -cmin +24 | xargs rm ; PHP 4.2 and less have an undocumented feature/bug that allows you to ; to initialize a session variable in the global scope, albeit register_globals ; is disabled. PHP 4.3 and later will warn you, if this feature is used. ; You can disable the feature and the warning separately. At this time, ; the warning is only displayed, if bug_compat_42 is enabled. session.bug_compat_42 = 1 session.bug_compat_warn = 1 ; Check HTTP Referer to invalidate externally stored URLs containing ids. ; HTTP_REFERER has to contain this substring for the session to be ; considered as valid. session.referer_check = ; How many bytes to read from the file. session.entropy_length = 0 ; Specified here to create the session id. session.entropy_file = ;session.entropy_length = 16 ;session.entropy_file = /dev/urandom ; Set to {nocache,private,public,} to determine HTTP caching aspects ; or leave this empty to avoid sending anti-caching headers. session.cache_limiter = nocache ; Document expires after n minutes. session.cache_expire = 180 ; trans sid support is disabled by default. ; Use of trans sid may risk your users security. ; Use this option with caution. ; - User may send URL contains active session ID ; to other person via. email/irc/etc. ; - URL that contains active session ID may be stored ; in publically accessible computer. ; - User may access your site with the same session ID ; always using URL stored in browser's history or bookmarks. session.use_trans_sid = 0 ; Select a hash function ; 0: MD5 (128 bits) ; 1: SHA-1 (160 bits) session.hash_function = 0 ; Define how many bits are stored in each character when converting ; the binary hash data to something readable. ; ; 4 bits: 0-9, a-f ; 5 bits: 0-9, a-v ; 6 bits: 0-9, a-z, A-Z, "-", "," session.hash_bits_per_character = 4 ; The URL rewriter will look for URLs in a defined set of HTML tags. ; form/fieldset are special; if you include them here, the rewriter will ; add a hidden <input> field with the info which is otherwise appended ; to URLs. If you want XHTML conformity, remove the form entry. ; Note that all valid entries require a "=", even if no value follows. url_rewriter.tags = "a=href,area=href,frame=src,input=src,form=,fieldset="
-
Yes, i have access to my php.ini on my cpanel. What do i need to change what to??
-
Is it something to do with this: How would i fully check this is correct? cpanel somewhere?
-
Hi im getting the following error message in my admin area: Warning: session_start() [function.session-start]: open(/home/users/web/b109/ipg.removalspacecom/cgi-bin/tmp/sess_9b495d20f1a5e1cb9eaed99c1b5dfed6, O_RDWR) failed: No such file or directory (2) in /hermes/bosweb25a/b109/ipg.removalspacecom/admin01.php on line 2 Warning: session_start() [function.session-start]: Cannot send session cookie - headers already sent by (output started at /hermes/bosweb25a/b109/ipg.removalspacecom/admin01.php:2) in /hermes/bosweb25a/b109/ipg.removalspacecom/admin01.php on line 2 Warning: session_start() [function.session-start]: Cannot send session cache limiter - headers already sent (output started at /hermes/bosweb25a/b109/ipg.removalspacecom/admin01.php:2) in /hermes/bosweb25a/b109/ipg.removalspacecom/admin01.php on line 2 There are 1 removalspace users so far. Company Name Contact Name Contact Number Email Address Line 1 Address Line 2 Location Postcode Basic Members Upgraded Users Company Logo Approved none none none [email protected] none none none none Current level = 0 Level 1 - Free Level 2 - Basic Level 3 - Premium Level 0 - Do Not Display Warning: Unknown: open(/home/users/web/b109/ipg.removalspacecom/cgi-bin/tmp/sess_9b495d20f1a5e1cb9eaed99c1b5dfed6, O_RDWR) failed: No such file or directory (2) in Unknown on line 0 Warning: Unknown: Failed to write session data (files). Please verify that the current setting of session.save_path is correct (/home/users/web/b109/ipg.removalspacecom/cgi-bin/tmp) in Unknown on line 0 Admin01.php <?PHP session_start(); /* really need to use a session variable to insure authorized to be here */ include ('db.php'); /* ======================================== 99% of the time it is better to put your query in a string. It makes debugging much easier ======================================== */ $query = "SELECT * FROM companies"; $result = mysql_query($query ) or die("SELECT Error: ".mysql_error()); $num_rows = mysql_num_rows($result); /* ======================================== I find it easier to locate problems if I indent code properly and drop out of PHP if there are large sections of html ======================================== */ ?> <br><br><br>There are <?PHP echo $num_rows; ?> removalspace users so far.<P> <table width="819" height="114"> <tr> <th>Company Name</th> <th>Contact Name</th> <th>Contact Number</th> <th>Email</th> <th>Address Line 1</th> <th>Address Line 2</th> <th>Location</th> <th>Postcode</th> <th>Basic Members</th> <th>Upgraded Users</th> <th>Company Logo</th> <th>Approved</th> </tr> <tr> <td colspan="6"></td> </tr> <?PHP while ( $row = mysql_fetch_array($result, MYSQL_ASSOC )) { ?> <tr> <td><?PHP echo $row['company_name']; ?></td> <td><?PHP echo $row['contact_name']; ?></td> <td><?PHP echo $row['phone']; ?></td> <td><?PHP echo $row['email']; ?></td> <td><?PHP echo $row['street1']; ?></td> <td><?PHP echo $row['street2']; ?></td> <td><?PHP echo $row['location']; ?></td> <td><?PHP echo $row['postcode']; ?></td> <td><?PHP echo $row['basicpackage_description']; ?></td> <td><?PHP echo $row['premiumuser_description']; ?></td> <?PHP /* ======================================== I presume you want to show the thumb version here ======================================== */ ?> <td><img src="images/thumbs/<?PHP echo $row['upload']; ?>" alt="logo"/></td> </tr> <tr> <td colspan="10"> <table> <tr> <td>Current level = <?PHP echo $row['approved']; ?></td> <td><a href="admin02.php?id=<?PHP echo $row['id']; ?>&level=1">Level 1 - Free</a></td> <td><a href="admin02.php?id=<?PHP echo $row['id']; ?>&level=2">Level 2 - Basic</a></td> <td><a href="admin02.php?id=<?PHP echo $row['id']; ?>&level=3">Level 3 - Premium</a></td> <td><a href="admin02.php?id=<?PHP echo $row['id']; ?>&level=0">Level 0 - Do Not Display</a></td> </tr> </table> </tr> <?PHP } echo "</table>"; ?></table> Then when i click to change something e.g level of user, i get this error message: Warning: session_start() [function.session-start]: open(/home/users/web/b109/ipg.removalspacecom/cgi-bin/tmp/sess_d986479c3603a52a37390b24a12caf03, O_RDWR) failed: No such file or directory (2) in /hermes/bosweb25a/b109/ipg.removalspacecom/admin02.php on line 2 Warning: session_start() [function.session-start]: Cannot send session cookie - headers already sent by (output started at /hermes/bosweb25a/b109/ipg.removalspacecom/admin02.php:2) in /hermes/bosweb25a/b109/ipg.removalspacecom/admin02.php on line 2 Warning: session_start() [function.session-start]: Cannot send session cache limiter - headers already sent (output started at /hermes/bosweb25a/b109/ipg.removalspacecom/admin02.php:2) in /hermes/bosweb25a/b109/ipg.removalspacecom/admin02.php on line 2 Warning: Cannot modify header information - headers already sent by (output started at /hermes/bosweb25a/b109/ipg.removalspacecom/admin02.php:2) in /hermes/bosweb25a/b109/ipg.removalspacecom/admin02.php on line 9 Warning: Unknown: open(/home/users/web/b109/ipg.removalspacecom/cgi-bin/tmp/sess_d986479c3603a52a37390b24a12caf03, O_RDWR) failed: No such file or directory (2) in Unknown on line 0 Warning: Unknown: Failed to write session data (files). Please verify that the current setting of session.save_path is correct (/home/users/web/b109/ipg.removalspacecom/cgi-bin/tmp) in Unknown on line 0 Admin02.php <?PHP session_start(); /* really need to use a session variable to insure authorized to be here */ include('db.php'); $id = (int) $_GET['id']; $level = (int) $_GET['level']; $query = "UPDATE companies set approved = '$level' WHERE id = '$id'"; $result = mysql_query($query); header("Location: admin01.php"); ?> Can someone please help with this please?
-
For LOGIN: a_test2.php: <?PHP include ('db.php'); /* set some validation variables */ $error_message = ""; /* =============================================== */ /*this section of code will set up an error message for the username if ANY of the conditions occur 1) checks to see if $_POST['username'] is NOT set 2) if length of username is less than 5 3) if username has anything other than letter, numbers or underscores*/ if((!isset($_POST['username'])) || (strlen(trim($_POST['username'])) <5) || (trim($_POST['username']) != preg_replace("/[^a-zA-Z0-9\_]/", "", trim($_POST['username'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid username<br>"; $error_message = $error_message . "Valid names are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['username'] . "</font><hr>";} else{ $username = mysql_real_escape_string(trim($_POST['username']));} /* END validating username *//* =============================================== */ /* =============================================== */ /*this section of code will set up an error message for thepassword if ANY of the conditions occur 1) checks to see if $_POST['password'] is NOT set 2) if length of password is less than 5 3) if password has anything other than letter, numbers or underscores*/ if((!isset($_POST['password'])) || (strlen(trim($_POST['password'])) <5) || (trim($_POST['password']) != preg_replace("/[^a-zA-Z0-9\_]/", "", trim($_POST['password'])))) { /* if it is NOT set, then set the error variable and start building the error message */ $error_message = $error_message . "You must enter a valid password<br>"; $error_message = $error_message . "Valid passwords are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid password was: <font color="red">' . $_POST['password'] . "</font><hr>";} else{ $password = trim($_POST['password']);} /* END validating password *//* =============================================== */ /* =============================================== */ /* if any of the post variables are invalid */ /* set the session variable and send back to the form page */ if(strlen(trim($error_message))>0) { $_SESSION['error_message'] =$error_message; header("Location: login.php"); exit();} /* =============================================== */ /* =============================================== */ /* FUNCTION TO CREATE SALT */ function createSalt() { $string = md5(uniqid(rand(), true)); return substr($string, 0, 3);} /* check to see if username is in the table if not send back to login*/ $query01 = "SELECT id, salt FROM companies WHERE username = '$username'"; $result01 = mysql_query($query01) or die(mysql_error()); if(mysql_num_rows($result1 != 1)) { header("Location: login.php"); exit(); } $row = mysq_fetch_array($result01); $salt = $row['salt']; $password = trim($_POST['password']); $hash = hash('sha256', $salt, $password); $query02 = "SELECT id FROM companies WHERE username = '$username' AND password = '$hash'"; $result02 = mysql_query($query02) or die(mysql_error()); if(mysql_num_rows($result2) !=1) { /* not found send back to login */ header("Location: login.php"); exit();} /* =============================================== *//* success!!! send them where you want */ ?> for this table: table width="300" border="0" align="center" cellpadding="0" cellspacing="1" bgcolor="#CCCCCC"> <tr> <form name="form1" method="post" action="a_test2.php"> <td> <table width="100%" border="0" cellpadding="3" cellspacing="1" bgcolor="#FFFFFF"> <tr> <td colspan="3"><strong>Member Login </strong></td> </tr> <tr> <td width="78">Username</td> <td width="6">:</td> <td width="294"><input name="username" type="text" id="username"></td> </tr> <tr> <td>Password</td> <td>:</td> <td><input name="password" type="password" id="password"></td> </tr> <tr> <td> </td> <td> </td> <td><input type="submit" name="Submit" value="Login"></td> </tr> </table> </td> </form> </tr> </table> For REGISTER: andy_test_01.php: <?PHP include('db.php'); /* set some validation variables */ $error_message = ""; /* =============================================== */ /* this section of code will set up an error message for the username if ANY of the conditions occur 1) checks to see if $_POST['username'] is NOT set 2) if length of username is less than 5 3) if username has anything other than letter, numbers or underscores */ if((!isset($_POST['username'])) || (strlen(trim($_POST['username'])) <5) || (trim($_POST['username']) != preg_replace("/[^a-zA-Z0-9\_]/", "", trim($_POST['username'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid username<br>"; $error_message = $error_message . "Valid names are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['username'] . "</font><hr>"; } /* END validating username */ /* =============================================== */ /* =============================================== */ /* this section of code will set up an error message for the password if ANY of the conditions occur 1) checks to see if $_POST['upassword'] is NOT set 2) if length of upassword is less than 5 3) if upassword has anything other than letter, numbers or underscores */ if((!isset($_POST['password'])) || (strlen(trim($_POST['password'])) <5) || (trim($_POST['password']) != preg_replace("/[^a-zA-Z0-9\_]/", "", trim($_POST['password'])))) { /* if it is NOT set, then set the error variable and start building the error message */ $error_message = $error_message . "You must enter a valid password<br>"; $error_message = $error_message . "Valid passwords are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid password was: <font color="red">' . $_POST['password'] . "</font><hr>"; }else{ $password = trim($_POST['password']); } /* END validating password */ /* =============================================== */ /* =============================================== */ /* validating the email */ /* create a function */ function validateEmailAddress($email) { return filter_var($email, FILTER_VALIDATE_EMAIL) && preg_match('/@.+\./', $email); } if(!isset($_POST['email']) || validateEmailAddress($_POST['email']) !=1) { $error_message = $error_message . "You must enter a valid email address<br>"; $error_message = $error_message . 'The invalid email was: <font color="red">' . $_POST['email'] . "</font><hr>"; } /* END validating email */ /* =============================================== */ /* =============================================== */ /* check to see if username is already taken */ $username = mysql_real_escape_string(trim($_POST['username'])); $query1 = "SELECT username from companies WHERE username = '$username'"; $result1 = mysql_query($query1) or die(mysql_error()); $count = mysql_num_rows($result); if($count>0) { $error_message = $error_message . 'The username: <font color="red">' . $_POST['username'] . "</font> is taken.<hr>"; } /* =============================================== */ /* if any of the post variables are invalid */ /* set the session variable and send back to the form page */ if(strlen(trim($error_message))>0) { $_SESSION['error_message'] =$error_message; header("Location: register.php"); exit(); } /* =============================================== */ /* =============================================== */ /* PREPARE DATA FOR INSERTION INTO TABLE */ /* FUNCTION TO CREATE SALT */ function createSalt() { $string = md5(uniqid(rand(), true)); return substr($string, 0, 3); } $salt = createsalt(); $passwod = trim($_POST['password']); $hash = hash('sha256', $salt, $password); $approved = 0; $username = mysql_real_escape_string(trim($_POST['username'])); $email = mysql_real_escape_string(trim($_POST['email'])); $query2 = "INSERT INTO companies (username, password, email, salt, approved) VALUES('$username', '$hash', '$email', '$salt', '$approved')"; $result2 = mysql_query($query2) or die(mysql_error()); /* =============================================== */ /* at this point we can send an email to the admin as well as the user. DO NOT send the user's password to ANYONE!!!! */ ?> Thank you for registering.<br>; Your account will be approved and activated within 24 hours.<br><br> Click here to return to the <a href="index.php">main page</a>. for this table: <form name="form1" method="post" action="andy_test_01.php"> <table width="316" height="120" border="0"> <tr><td colspan=2><h1>Register/Sign Up</h1></td></tr> <tr><td>Username:</td><td> <input name="username" type="text" id="username"> </td></tr> <tr><td>Password:</td><td> <input name="password" type="password" class="style7" id="password"> </td></tr> <tr><td colspan="2" align="right"> <tr><td>Email:</td><td> <input name="email" type="text" class="style7" id="email"> </td></tr> <tr><td colspan="2" align="right"> <input name="Submit" type="submit" class="style7" value="Register" /> </td></tr> </table>
-
so it's: $query1 = "SELECT salt from companies WHERE salt = '$salt'"; thats just hash/salt password right? that produces the following: "Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in /hermes/bosweb25a/b109/ipg.removalspacecom/andy_test_01.php on line 63 Warning: Cannot modify header information - headers already sent by (output started at /hermes/bosweb25a/b109/ipg.removalspacecom/andy_test_01.php:63) in /hermes/bosweb25a/b109/ipg.removalspacecom/andy_test_01.php on line 73"
-
Okay: i have gone away and done a little bit of resaerch. I understand that using salt to check login is a lot more secure as the user's username doesnt change and salt it random. I have changed the query to: $query1 = "SELECT username, salt from companies WHERE salt = '$salt', username = '$username'"; could someone please explain why im getting this error?: You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near ' username = 'testing'' at line 1 'testing' is what i have used as a test username??
-
<?php /* =============================================== */ /* check to see if username is already taken */ $username = mysql_real_escape_string(trim($_POST['username'])); $query1 = "SELECT username, salt from companies WHERE username = '$username', salt = '$salt'"; $result1 = mysql_query($query1) or die(mysql_error()); $count = mysql_num_rows($result); if($count>0) { $error_message = $error_message . 'The username: <font color="red">' . $_POST['username'] . "</font> is taken.<hr>"; } ?>
-
/* =============================================== */ /* check to see if username is already taken */ $username = mysql_real_escape_string(trim($_POST['username'])); $query1 = "SELECT username from companies WHERE username = '$username'"; $result1 = mysql_query($query1) or die(mysql_error()); $count = mysql_num_rows($result); if($count>0) { $error_message = $error_message . 'The username: <font color="red">' . $_POST['username'] . "</font> is taken.<hr>"; } Check USERNAME? not password? this is where the error is, just checking...
-
Inserted the username and password with this code: <?PHP include('db.php'); /* set some validation variables */ $error_message = ""; /* =============================================== */ /* this section of code will set up an error message for the username if ANY of the conditions occur 1) checks to see if $_POST['username'] is NOT set 2) if length of username is less than 5 3) if username has anything other than letter, numbers or underscores */ if((!isset($_POST['username'])) || (strlen(trim($_POST['username'])) <5) || (trim($_POST['username']) != preg_replace("/[^a-zA-Z0-9\_]/", "", trim($_POST['username'])))) { /* if username is bad start building the error message */ $error_message = "You must enter a valid username<br>"; $error_message = $error_message . "Valid names are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid name was: <font color="red">' . $_POST['username'] . "</font><hr>"; } /* END validating username */ /* =============================================== */ /* =============================================== */ /* this section of code will set up an error message for the password if ANY of the conditions occur 1) checks to see if $_POST['upassword'] is NOT set 2) if length of upassword is less than 5 3) if upassword has anything other than letter, numbers or underscores */ if((!isset($_POST['password'])) || (strlen(trim($_POST['password'])) <5) || (trim($_POST['password']) != preg_replace("/[^a-zA-Z0-9\_]/", "", trim($_POST['password'])))) { /* if it is NOT set, then set the error variable and start building the error message */ $error_message = $error_message . "You must enter a valid password<br>"; $error_message = $error_message . "Valid passwords are min 5 characters and use letters, numbers and underscores only.<br>"; $error_message = $error_message . 'Your invalid password was: <font color="red">' . $_POST['upassword'] . "</font><hr>"; }else{ $upassword = trim($_POST['password']); } /* END validating upassword */ /* =============================================== */ /* =============================================== */ /* validating the email */ /* create a function */ function validateEmailAddress($email) { return filter_var($email, FILTER_VALIDATE_EMAIL) && preg_match('/@.+\./', $email); } if(!isset($_POST['email']) || validateEmailAddress($_POST['email']) !=1) { $error_message = $error_message . "You must enter a valid email address<br>"; $error_message = $error_message . 'The invalid email was: <font color="red">' . $_POST['email'] . "</font><hr>"; } /* END validating email */ /* =============================================== */ /* =============================================== */ /* check to see if username is already taken */ $username = mysql_real_escape_string(trim($_POST['username'])); $query1 = "SELECT username from companies WHERE username = '$username'"; $result1 = mysql_query($query1) or die(mysql_error()); $count = mysql_num_rows($result); if($count>0) { $error_message = $error_message . 'The username: <font color="red">' . $_POST['username'] . "</font> is taken.<hr>"; } /* =============================================== */ /* if any of the post variables are invalid */ /* set the session variable and send back to the form page */ if(strlen(trim($error_message))>0) { $_SESSION['error_message'] =$error_message; header("Location: register.php"); exit(); } /* =============================================== */ /* =============================================== */ /* PREPARE DATA FOR INSERTION INTO TABLE */ /* FUNCTION TO CREATE SALT */ function createSalt() { $string = md5(uniqid(rand(), true)); return substr($string, 0, 3); } $salt = createsalt(); $upasswod = trim($_POST['password']); $hash = hash('sha256', $salt, $password); $approved = 0; $username = mysql_real_escape_string(trim($_POST['username'])); $email = mysql_real_escape_string(trim($_POST['email'])); $query2 = "INSERT INTO companies (username, password, email, salt, approved) VALUES('$username', '$hash', '$email', '$salt', '$approved')"; $result2 = mysql_query($query2) or die(mysql_error()); /* =============================================== */ /* at this point we can send an email to the admin as well as the user. DO NOT send the user's password to ANYONE!!!! */ ?> Thank you for registering.<br>; Your account will be approved and activated within 24 hours.<br><br> Click here to return to the <a href="index.php">main page</a>.