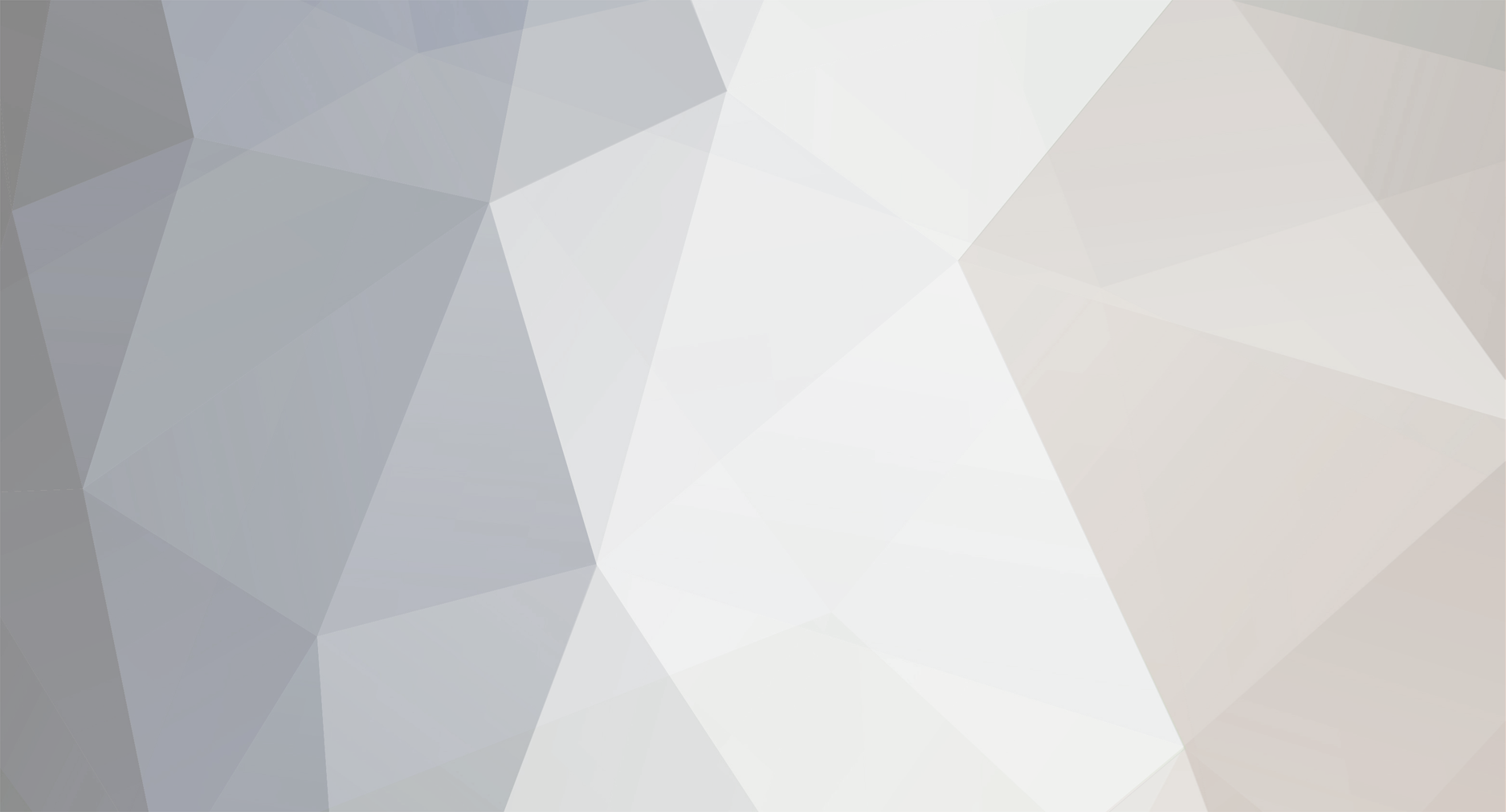
zelig
Members-
Posts
117 -
Joined
-
Last visited
Never
Everything posted by zelig
-
Okay, I have a script that if someone is a particular "class", they can't get additional bonus points for their character. However, if they aren't that class, then they get additional points. But, it seems to be ignoring the elseif and gives a strength point no matter what the class is. I don't see where I coded wrong, so I would appreciate any help! Thanks! if ($expgain + $player->mine_exp >= $player->mine_maxexp) { echo "<br /><b>Your mining leveled up!</b>"; echo "$blurb $randeffect<p>"; if(($strength + $agility + $wisdom + $vitality >=100) && ($class != 'Apprentice' || $class != 'Mage' || $class != 'Priest' || $class != 'Rogue' || $class != 'Scholar' || $class != 'Wanderer' || $class != 'Warrior' || $class != 'Woodsman')) { echo "<br /><b>You gained 1 strength point!</b>"; $query = $db->execute("update `users` set `energy`=?, `mine_level`=?, `mine_exp`=?, `minecount`=?, `strength`=?, `mine_maxexp`=? where `id`=?", array($player->energy - 1, $player->mine_level + 1, $player->mine_exp + 1, $player->minecount + 1, $player->strength + 1, $player->mine_maxexp + $newmax, $player->id)); } elseif (($strength + $agility + $wisdom + $vitality >= 100) && ($class = 'Apprentice' || $class = 'Mage' || $class = 'Priest' || $class = 'Rogue' || $class = 'Scholar' || $class = 'Wanderer' || $class = 'Warrior' || $class = 'Woodsman')) { echo "You have already maxed out your stats at 100!"; $query = $db->execute("update `users` set `energy`=?, `mine_level`=?, `mine_exp`=?, `minecount`=?, `mine_maxexp`=? where `id`=?", array($player->energy - 1, $player->mine_level + 1, $player->mine_exp + 1, $player->minecount + 1, $player->mine_maxexp + $newmax, $player->id)); } elseif($strength + $vitality + $wisdom + $agility < 100) { echo "<br /><b>Your mining leveled up and you gained 1 strength point!</b>"; $query = $db->execute("update `users` set `energy`=?, `mine_level`=?, `mine_exp`=?, `minecount`=?, `strength`=?, `mine_maxexp`=? where `id`=?", array($player->energy - 1, $player->mine_level + 1, $player->mine_exp + 1, $player->minecount + 1, $player->strength + 1, $player->mine_maxexp + $newmax, $player->id)); } } else { $query = $db->execute("update `users` set `energy`=?, `mine_exp`=?, `minecount`=? where `id`=?", array($player->energy - 1, $player->mine_exp + 1, $player->minecount + 1, $player->id)); echo "$blurb $randeffect<p>"; }
-
Okay, for some reason, I'm showing an error on this: The error occurs with the bracket after the else, and I don't understand why... if ($strength + $vitality + $wisdom + $agility >= 100) { echo "You have already maxed out your stats at 100!"; $query = $db->execute("update `users` set `energy`=?, `mine_exp`=?, `minecount`=?, `small_gold`=? where `id`=?", array($player->energy - 1, $player->mine_exp + 1, $player->minecount + 1, $player->small_gold + 1, $player->id)); echo "$blurb $randeffect<p>"; } else($strength + $vitality + $wisdom + $agility < 100) { echo "<br /><b>Your mining leveled up and you gained 1 strength point!</b>"; $query = $db->execute("UPDATE `users` set `energy`=?, `mine_level`=?, `mine_exp`=?, `minecount`=?, `small_gold`=?, `strength`=?, `mine_maxexp`=? where `id`=?", array($player->energy - 1, $player->mine_level + 1, $player->mine_exp + 1, $player->minecount + 1, $player->small_gold + 1, $player->strength + 1, $player-> mine_maxexp + $newmax, $player->id)); }
-
Yeah, it's not doing exactly what I want. There's no error in it. I'll move this along to a mysql help. Thanks!
-
I'm trying to pull from my database every spell or attack that is equal to `All`, but it doesn't seem to be working. All it is doing is pulling all the spells, regardless of class = `All`, and also 0 of the attacks. It should be producing a single attack that is = to `All` as no spells are = `All`. Any help would be greatly appreciated! Thanks! (This is my first attempt at a JOIN statement...) $query = "SELECT attacks.id, attacks.name, attacks.price, attacks.class, attacks.descript, spells.id, spells.name, spells.price, spells.class, spells.descript ". "FROM attacks, spells ". "WHERE attacks.class = 'All' || spells.class = 'All' order by attacks.name, spells.name asc";
-
I'm trying to make it so that someone can add an item to the shop based upon the current items within the database. How do I go about doing that? I know i have to change <input> to <select>, but beyond that, how do I code it so I can run an array to get all the current items from the table `items` and list them by their `name` field? Thanks in advance! Here is what I have so far: function addshopinv($id) { error_reporting(E_ALL); ini_set('display_errors', 1); if (isset($_POST["submit"])) { extract($_POST); $errors = 0; $errorlist = ""; if ($name == "") { $errors++; $errorlist .= "Name is required.<br />"; } else if ($errors == 0) { $dbh=dbconnect() or die ("Userlist read error: " . mysql_error()."<br>"); mysql_select_db("XXX"); $query = mysql_query("SELECT id FROM items WHERE name='$name'"); while ($result = mysql_fetch_array($query)){ $item_id = $result['id']; $query1 = mysql_query("INSERT INTO sale SET shop_id='$id', item_id='$item_id'"); } admindisplay("Inventory Item Added.","Add New Inventory Item"); } else { admindisplay("<b>Errors:</b><br /><div style=\"color:red;\">$errorlist</div><br />Please go back and try again.", "Add New Item to Shop"); } } $page = <<<END <b><u>Add New Inventory Item</u></b><br /><br /> <form action="admin_panel.php?do=addshopinv:$id" method="post"> <table width="90%"> <tr><td width="20%">Name:</td><td><input type="text" name="name" size="30" maxlength="255" value="" />*255 character max</td></tr> </table> <input type="submit" name="submit" value="Submit" /> <input type="reset" name="reset" value="Reset" /> </form> END; $page = parsetemplate($page, $row); admindisplay($page, "Add New Inventory Item"); }
-
Can't figure out why it won't add to the database...
zelig replied to zelig's topic in PHP Coding Help
I found it... *headsdesk* -
Okay, the function appears to work just fine, except it doesn't add to the database. I don't have any errors, so I'm not quite sure what is going on. I've got an echo on the $id and $item_id, and both were pulling correctly. Just not being added to the table `sale`... Anyone help? Thanks in advance! function addshopinv($id) { error_reporting(E_ALL); ini_set('display_errors', 1); if (isset($_POST["submit"])) { extract($_POST); $errors = 0; $errorlist = ""; if ($name == "") { $errors++; $errorlist .= "Name is required.<br />"; } else if ($errors == 0) { $dbh=dbconnect() or die ("Userlist read error: " . mysql_error()."<br>"); mysql_select_db("XXX"); $query = mysql_query("SELECT id FROM items WHERE name='$name'"); while ($result = mysql_fetch_array($query)){ $item_id = $result['id']; echo $id; $query1 = mysql_query("INSERT INTO sale SET shop_id='$id', item_id='$item_id"); } admindisplay("Inventory Item Added.","Add New Inventory Item"); } else { admindisplay("<b>Errors:</b><br /><div style=\"color:red;\">$errorlist</div><br />Please go back and try again.", "Add New Item to Shop"); } }
-
Okay, here's what I'm doing. It's sort of like a market script. When someone wants to buy something, they choose from a drop down the item they wish to buy. However, there is a setup cost for it to be started. Then, based upon the length they choose (anywhere from 1 - 12 months), the cost will add up. My questions are: [*]Is it possible to assign a setup cost? [*]How can I make it so that, while some things will be, say $2 a month, others will be $3 or other amounts? Here's what I have so far, to try and figure this out. Feel free to laugh... Or if you could point me to an example of something like this, I'd be very appreciative! Thanks!! function ChangeInitialCost(){ $cic = ($_POST['newpremium']); if (($cic == "clan") || ($cic == "guild") || ($cic == "house") || ($cic == "militia")){ } And the form I have: (I haven't entered a submit button yet...) <table width="200" border="1"> <form action="premium.php" method="post" name="purchase"> <tr> <td>Premium:</td> <td><select id="newpremium" name="newpremium"> <option value="casino">Casino</option> <option value="clan">Clan</option> <option value="guild">Guild</option> <option value="house">House</option> <option value="militia">Militia</option> </select></td></form> </tr> <tr><form action="premium.php" method="post" name="length"> <td>Length:</td> <td><select id="length" name="length"> <option value="0">-----</option> <option value="1">One Month</option> <option value="2">Two Months</option> <option value="3">Three Months</option> <option value="4">Four Months</option> <option value="5">Five Months</option> <option value="6">Six Months</option> <option value="7">Seven Months</option> <option value="8">Eight Months</option> <option value="9">Nine Months</option> <option value="10">Ten Months</option> <option value="11">Eleven Months</option> <option value="12">Twelve Months</option> </select></td></form> </tr> <tr> <td>Cost:</td> <td>SOME TYPE OF FUNCTION</td> </table>
-
I got a bit of what I'm doing... I'm calling the URL to a main.php where this is not located. How do I setup the Delete Post URL to run a function on the same page? And I think I see what you're talking about. I've added in the code: $id = $row['id']; Is that what you are trying to get me to see?
-
Okay, so when I call out the messages to be read with this query: $result = mysql_query("SELECT * FROM boards WHERE boardname='$board' ORDER BY id LIMIT 10"); I try to follow it up with: $id = $_GET['id']; But that doesn't seem to work either. How can I get the $id variable to capture the id that is called during that result query?
-
Each message has its own unique id. That's the auto-increment primary key of id on the boards table. How do I define what the $id variable is? I tried the $id=$_GET['id'] , but that didn't seem to pull it.
-
Okay, I guess the term "forum" is incorrect. There are no threads or overall board for everything in that sense. I have a message system that allows people to post to "boards" inside of my game. Each room can have a board inside it, thus needing the boardname field within the boards table. Each of these boards is its own separate entity that contain messages. So you can think of it as a message system tied to a specific room based upon its boardname (which is the room's title). Does that make more sense? It's just a bunch of single "topics" (if you want to go with forum terminology) that don't exist within any board or thread. Here are all the functions that are in use for this system: Post HTML: if (getlevel(get("plane"),coords(),"board") == "") echo "There is no bulletin board here.<br>\n"; else{ $action = filter($action); $data = explode("Post: ",getlevel(get("plane"),coords(),"board")); $board = getlevel(get("plane"),coords(),"board"); $size = count($data); $message = stripslashes(nl2br($action)); $thetime = gmdate("D, M jS, Y - g:i A") . " GMT"; $thisdata = explode(":::",$data[$data[0]]); $thisdata[0] = get("name"); $thisdata[1] = $thetime; $thisdata[2] = $message; $thisdata = implode(":::",$thisdata); $data[$data[0]] = $thisdata; $data[0]++; if ($data[0] > $size) $data[0] = 1; $data = implode($data); $dbh=dbconnect() or die ("Userlist read error: " . mysql_error()."<br>"); mysql_select_db("XXX"); $result = mysql_query("INSERT INTO `boards` SET `boardname`='$board', `message`='$message', `username`='$username', `time`='$thetime'") or die (mysql_error()); echo "Message posted.<br>\n"; } Post function: <? $command = 1; $dat = substr($action2,5); if (getlevel(get("plane"),coords(),"board") == "") echo "There is no bulletin board here.<br>\n"; else{ echo "<center><form action=post.php method=post>\n<textarea name=action rows=10 cols=95%>$dat</textarea>\n<input type=hidden name=username value=$username>\n<input type=hidden name=password value=$password><br>\n<input type=submit value=Post>\n</form></center>\n"; } ?> Readboard/Delete <? function delpost() { if (getlevel(get("plane"),coords(),"board") == "" && ( get("admin") == "Y+" || get("admin") == "Y")) echo "There is no bulletin board here.<br>\n"; else{ $board = getlevel(get("plane"),coords(),"board"); $dbh=dbconnect() or die ("Userlist read error: " . mysql_error()."<br>"); mysql_select_db("XXX"); $id = $_GET['id']; $result = mysql_query("DELETE FROM `boards` WHERE `boardname`='$board' AND `id`='$id'") or die(mysql_error());; echo "Message deleted.<br>\n"; }} $command = 1; if (getlevel(get("plane"),coords(),"board") == "") echo "There is no bulletin board here.<br>\n"; else{ $board = getlevel(get("plane"),coords(),"board"); if ($admin == "Y+" || $admin == "Y"){ $x = " --- <a href=main.php?username=$username&password=$password&action=delpost&id=$id>Delete Post</a>";} $dbh=dbconnect() or die ("Userlist read error: " . mysql_error()."<br>"); mysql_select_db("XXX"); $result = mysql_query("SELECT * FROM boards WHERE boardname='$board' ORDER BY id LIMIT 10"); $post = mysql_fetch_assoc($result); while ($row = mysql_fetch_array($result)){ echo stripslashes($row['message']) . "$x<br>" . " --- ".stripslashes($row['username']). " on ".stripslashes($row['time']) ."<hr width=90%>"; } } ?>
-
Didn't do anything.
-
I'm not deleting a board. Boards is the title of the table within the database. Inside boards are the fields: id, boardname, message. I'm wanting to delete a specific message based on the id of the message. I have multiple boards (thus the need for boardname) but each message has a unique id (id) to it. I do notice that when I mouseover the link, it doesn't have the id of the message on the end of the url... So, I guess it's not pulling $id correctly then, right?
-
A single post within a thread.
-
Hmm, no errors, but it doesn't delete it either. Here's what I have now: $command = 1; if (getlevel(get("plane"),coords(),"board") == "") echo "There is no bulletin board here.<br>\n"; else{ $board = getlevel(get("plane"),coords(),"board"); if ($admin == "Y+" || $admin == "Y"){ $x = " --- <a href=main.php?username=$username&password=$password&action=delpost&id=$id>Delete Post</a>";} $dbh=dbconnect() or die ("Userlist read error: " . mysql_error()."<br>"); mysql_select_db("XX"); $result = mysql_query("SELECT * FROM boards WHERE boardname='$board' ORDER BY id LIMIT 10"); $post = mysql_fetch_assoc($result); while ($row = mysql_fetch_array($result)){ echo stripslashes($row['message']) . "$x<br>" . " --- ".stripslashes($row['username']). " on ".stripslashes($row['time']) ."<hr width=90%>"; } } function delpost() { if (getlevel(get("plane"),coords(),"board") == "" && ( get("admin") == "Y+" || get("admin") == "Y")) echo "There is no bulletin board here.<br>\n"; else{ $board = getlevel(get("plane"),coords(),"board"); $dbh=dbconnect() or die ("Userlist read error: " . mysql_error()."<br>"); mysql_select_db("XX"); $id = $_GET['id']; $result = mysql_query("DELETE * FROM `boards` WHERE `boardname`='$board', `id`='$id'"); echo "Message deleted.<br>\n"; }}
-
Yes, each post has a unique id, simply the id field (primary). I didn't create a separate postid field.
-
I'm trying to be able to delete a specific post from a forum. How do I make it so that my function knows which post to delete? I know it needs to pull the id from the boards table, but how do I do that? In the readboard function, I've added a link to try and pull the ID of that specific post, but I don't think I have it right... Readboard function: <? $command = 1; if (getlevel(get("plane"),coords(),"board") == "") echo "There is no bulletin board here.<br>\n"; else{ $board = getlevel(get("plane"),coords(),"board"); if ($admin == "Y+" || $admin == "Y"){ $x = " --- <a href=main.php?username=$username&password=$password&action=delpost>Delete Post</a>";} $dbh=dbconnect() or die ("Userlist read error: " . mysql_error()."<br>"); mysql_select_db("XX"); $result = mysql_query("SELECT * FROM boards WHERE boardname='$board' ORDER BY id LIMIT 10"); $post = mysql_fetch_assoc($result); while ($row = mysql_fetch_array($result)){ echo stripslashes($row['message']) . "$x<br>" . " --- ".stripslashes($row['username']). " on ".stripslashes($row['time']) ."<hr width=90%>"; } } ?> Here's my current delpost function: function delpost() { if (getlevel(get("plane"),coords(),"board") == "" && ( get("admin") == "Y+" || get("admin") == "Y")) echo "There is no bulletin board here.<br>\n"; else{ $board = getlevel(get("plane"),coords(),"board"); $dbh=dbconnect() or die ("Userlist read error: " . mysql_error()."<br>"); mysql_select_db("XX"); $result = mysql_query("DELETE * FROM `boards` WHERE `boardname`='$board', `id`='$id'"); echo "Message deleted.<br>\n"; }}
-
Worked. Thanks litebearer!
-
Hmm... I tried doing a loop, but I have to confess, I'm not overly familiar with them. I got an error: Warning: mysql_fetch_assoc(): supplied argument is not a valid MySQL result resource Here is the code I have now: result = mysql_query("SELECT * FROM boards WHERE boardname='$board' ORDER BY id LIMIT 10"); $post = mysql_fetch_assoc($result); while ($row = mysql_fetch_assoc($post)){ echo stripslashes($row['message']) . "<br>\n" . " --- ".stripslashes($row['username']). " on ".stripslashes($row['time']) ."\n<hr width=90%>\n";} What did I do wrong, so I can learn from it?
-
For some reason, I can only get 1 row to echo out when there are actually multiple rows in the database that should be echoing. Here is what I have: $result = mysql_query("SELECT * FROM boards WHERE boardname='$board' ORDER BY id LIMIT 10"); $post = mysql_fetch_assoc($result); echo stripslashes($post['message']) . "<br>\n" . " --- ".stripslashes($post['username']). " on ".stripslashes($post['time']) ."\n<hr width=90%>\n"; It pulls the one record great, but it only shows one record... I want to keep it to 10 records, thus the LIMIT in there (which I think I did right...), but it won't even show the ones in there right now (under 10, so that's not an issue yet).
-
Through the input form near the top of the post.
-
Marked as null. In the MySQL DB, there's a checkmark to indicate that it is null.
-
I can't seem to figure this error out... Any help would be appreciated!! echo stripslashes($post['message']) . " --- stripslashes($post['username'])<br>\n" . " on stripslashes($post['time']) ."\n<hr width=90%>\n"; Basically, what I want it to say is: 'message' --- 'username' on 'time' With, of course, 'message', 'username', and 'time' filled in with the info from the database.