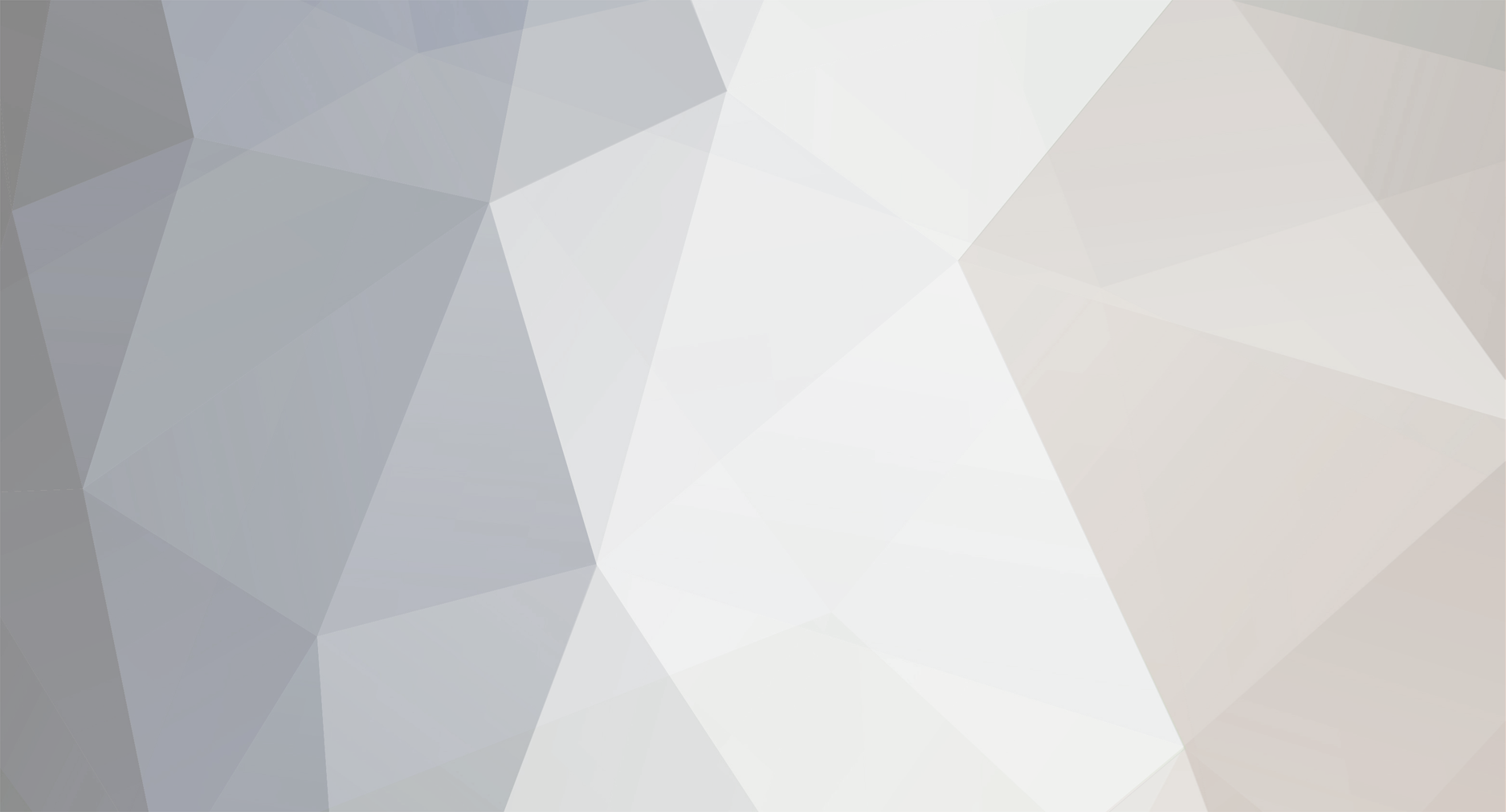
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
Considering it is a loop, just put the email from the database and it will email everyone with an appointment on that day.
-
Then post the rest of the code, something else is at play.
-
That doesn't mean it got to the part where it assigned $firstName...it just means it's not empty. It still has to pass a regex.
-
A Factory pattern is for object creation. It is not an "OOP Switch method". And 100 repeated classes called "ThingA", "ThingB" is better how exactly?
-
Are you sure it actually got to the point that it assigned the variable?
-
Then why not leave the include out and call it a day?
-
That is not at all what the Factory pattern is for, and is in no way cleaner than a simple switch statement.
-
You're getting the error because the file you are trying to include doesn't exist.
-
If you "prefer" making every website from absolute scratch then you probably aren't as proficient as you think you are. Learn frameworks, learn CMS's, and start getting work for them. People probably don't want a hacked together solution, they want to use powerful tools and just don't understand how to implement them. If you build someone a website with Drupal, a year from now they can hire another Drupal developer to easily create a plugin or extend functionality. If you build someone a website with home-brew code, in a year the new developer first has to learn your code, and then figure out how to extend it - which costs them more money in the end.
-
You can do this a little simpler and more modular like this: $pages = array('home', 'services', 'testimonials', 'contact'); $page = isset($_GET['action']) ? $_GET['action'] : 'home'; $func = 'display_' . $page; if (in_array($page, $pages) && function_exists($func)) { $func(); } else { echo 'page not found'; }
-
A good example is with a boolean. If you say "if $var == true", then if $var is actually 1 (integer) then it will pass (since 1 evaluates to true). If you say "if $var === true" then you are saying if $var is a boolean, and is true. EDIT: Actually, if you do "if $var == true" then if $var is equal to ANYTHING (except null, or 0) then it will be true.
-
That is exactly what switch is for... cleaner syntax than "if elseif elseif elseif elseif....".
-
You need to make the dataType json. $.ajax({ dataType: "json"
-
Isn't it pretty obvious? Warning: mysql_query() [function.mysql-query]: Access denied for user 'nobody'@'localhost' (using password: NO) in /home/gaogier/public_html/includes/polls.php on line 4 So check your database connection code.
-
Just keep an unminified version for development. When you go to production minify it. You don't really need it to be minified while you are developing - that's just a PITA. http://jscompress.com/ you can use this site to minifiy javascript.
-
Just make the tables in PHP and send it via JSON to jQuery. $json['tables'] = ''; if($count>=1){ while($result=mysql_fetch_assoc($res)){ $json['tables'] .= '<tr> <td>' . $result['id'] . '</td> <td>' . $result['name'] . '</td> </tr>'; }//end of while }//end of if echo json_encode($json); success:function(data){ $('table').append(data.tables); }
-
Make sure you do the td.title style AFTER the td style.
-
echo "<a href=\"task.php?option=delete\" onclick=\"return confirm('Do you really want to delete this?');\">Delete</a>"; However, this is absolutely not a secure way to do this. You need to use a form, so that you can validate the request to make sure the user actually meant for this to happen.
-
You need to use if conditionals to see if the form was submitted. And don't use $_REQUEST. Use $_GET or $_POST. if (!empty($_POST)) { // query here }
-
:not() isn't implemented yet. So, no there is no way. You'll just have to redefine td.title styles.
-
"hand" is not a cursor. You want pointer. And you don't need Javascript for this, you can just do it with CSS. td:hover { cursor:pointer; }
-
If you make a "success" and "failure" (or error) template, then it enables you to more easily reuse code. At the end of the day, reused code is efficient code. If you had this template: <?php require 'header.php'; ?> <div class="success"> <h1><?php echo $heading; ?><h1> <?php echo $message; ?> </div> <?php require 'footer.php'; ?> Now, anywhere that you want to display a "success" message, you simply do: load_template('success', array( 'title' => 'Member Account Created', 'message' => '<p>Congratulations!</p> <p>Your account has been created, and a confirmation e-mail sent to: "' . $email . '"</p> <p>Please click on the link in that e-mail to activate your account.</p>'; )); Now, you could improve these even further by having a "language" file... which sort of answers your original question. Basically a language file is just a php file containing a big array of messages and text that appears on your website. The idea is that in a multilingual environment, all you need to do is simply translate the english text to spanish (for example) and call it spanish.php, include it, and now all your text and messages are in spanish. But, even if you will only ever use one language, language files are still awesome. So if you had a file named english.php, you could do: $lang['account_create_success'] = '<p>Congratulations!</p> <p>Your account has been created, and a confirmation e-mail sent to: "%s"</p> <p>Please click on the link in that e-mail to activate your account.</p>'; In case you need a variable in the text, you can use sprintf syntax to accomplish that. So in place of $email we used %s. Then when you call it just use sprintf(); load_template('success', array( 'title' => 'Member Account Created', 'message' => sprintf(lang('account_create_success'), $email) )); Also, a side note - you don't need all those <p> tags in there...it's kind of old school. Just use line breaks (<br />).
-
If that's the case, why even bother? Just use those two functions. If you plan to only sanitize input to the point that it is safe to store and query with, then mysql_real_escape_string() is enough (or better yet: prepared statements and you don't even need to sanitize anything on input). There is no magical end-all function for sanitation. htmlspecialchars() will sanitize HTML and make it safe to display HTML from the database to a website without worrying about XSS attacks or people messing with your layout. If that's all you care about, then cool - that's all you need to do. But there is much more to sanitation than that, that you can't solve with 3 lines of code.