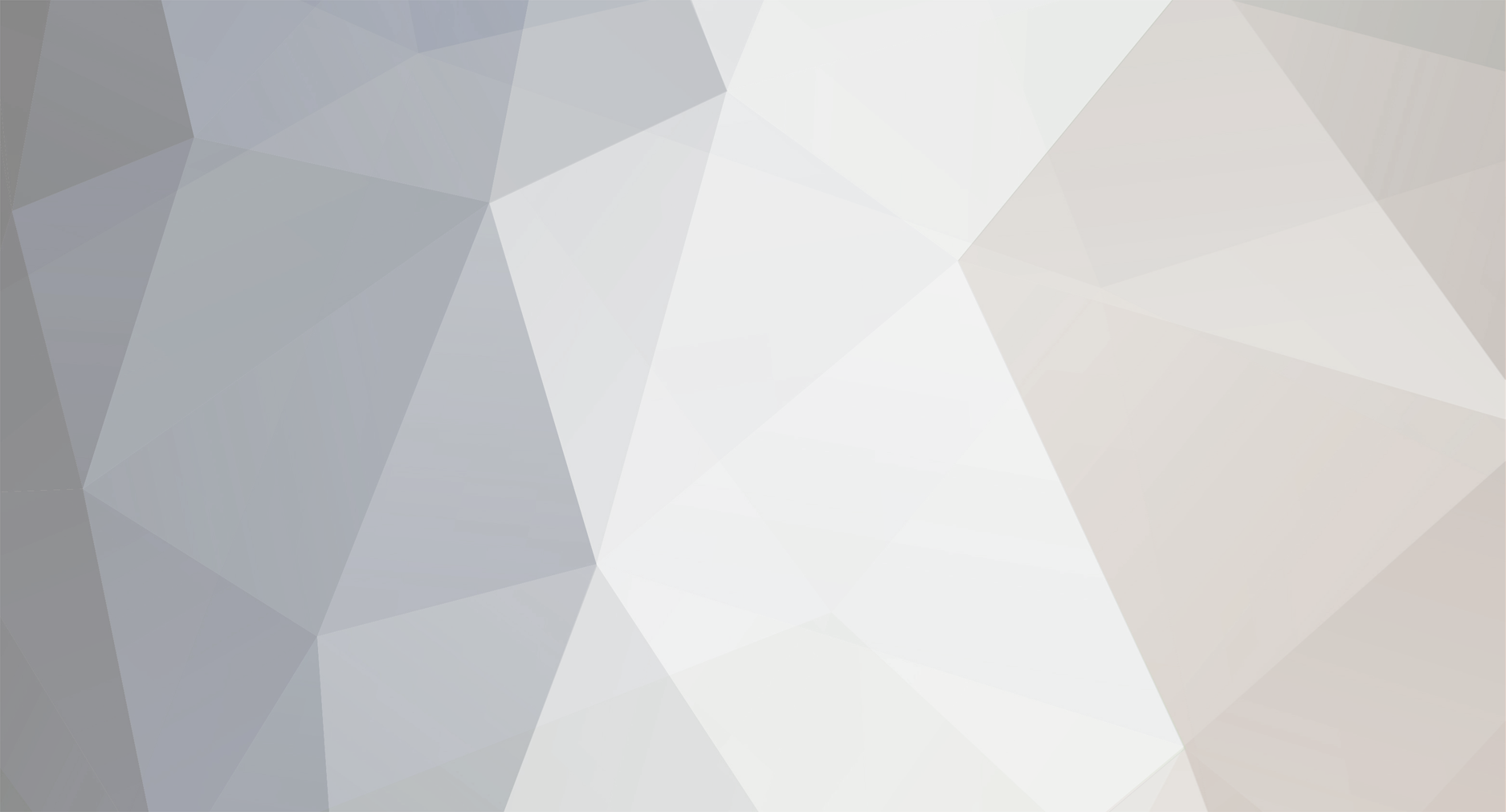
Winstons
-
Posts
52 -
Joined
-
Last visited
Never
Posts posted by Winstons
-
-
-
Did you tried use 'array_multisort' ?
-
Maybe need set 'session_start();' at beginning of 'Session Start.php' file ?
And try remove 'session_unset' from index.php
-
-
If your folder located on higher level you must write
require "../users/menu.php";
Or yet higher
require "../../users/menu.php";
-
Last two xyph's examples is priority, because $_SERVER["DOCUMENT_ROOT"] depends from php.ini or httpd.conf settings.
-
OK. This is residual code. And extra letters is missing
fnction.php
<?php if (isset($_POST['name']) && is_array($_POST['name'])) { $array = $_POST['name']; for($i = 0; $i < sizeof($array); $i++) { $first = !empty($array[$i]['first']) ? $array[$i]['first'] : ''; $last = !empty($array[$i + 1]['last']) ? $array[$i + 1]['last'] : ''; echo first_and_last($first, $last); } } function first_and_last($first_name, $last_name ) { return (!empty($first_name) && !empty($last_name)) ? $first_name[0] . '. ' . $last_name[0] . '.' : ''; } ?>
-
During update, timestamp field is updating automatically, therefore you can not worry
And modify your code a bit.
<? $Sql=" UPDATE `MyTable` SET `client_alias` = '" . $client_alias ."', `testimonial_date` = '" . $testimonial_date . "', `order_ontime` = '" . $order_ontime . "', `how_deal` = '" . $how_deal . "', `order_condition` = '" . $order_condition . "', `happy_colour` = '" . $happy_colour . "', `colour_chosen` = '" . $colur_chosen . "', `easy_use` = '" . $easy_use . "', `effectiveness` = '" . $effectiveness . "', `client_comments_eng` = '" . $client_comments_eng . "', `client_comments_esp` = '" . $client_comments_esp . "', `testimonial_updated_by` = '" . $testimonial_updated_by . "' WHERE `id_testimonial` = '" . $colname_testimonials_update_RS . "'"; mysql_query($Sql) or die('Error, query failed : ' . mysql_error());
-
Your code has 'notice'
Therefore you must correct it, this way:
index.php
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Functions!</title> </head> <body> <p>Please enter your first and last name so I can give you your initials.</p> <form action="functions.php" method="post"> <?php for ($i = 0; $i < 5; $i++) { // Maybe do a fieldset to separate each group. print '<legend>Enter Name</legend>'; printf('<label for="first_name%d">First Name:</label>', $i); printf('<input id="first_name%d" type="text" name="name[][first]" size="20" />', $i); printf('<label for="last_name%d">Last Name:</label>', $i); printf('<input id="last_name%d" type="text" name="name[][last]" size="20" />', $i); //print '</fieldset>'; } ?> <input type="submit" name="submit" value="Get My Initials" /> </body> </html>
functions.php
<?php if (isset($_POST['name']) && is_array($_POST['name'])) { foreach ($_POST['name'] AS $fullname) { list($first, $last) = each($fullname); print first_and_last($first, $last); } echo '<br/>'; } function first_and_last($first_name, $last_name ) { return (!empty($first_name) && !empty($last_name)) ? $first_name[0] . '. ' . $last_name[0] . '.' : ''; } ?>
-
In the code have very many errors.
I've recommended you alter it so:
<?php $con = mysql_connect("localhost","xxxxx","xxxxx") or die('Could not connect: ' . mysql_error()); mysql_select_db("addresses", $con); $sql = "INSERT INTO `addresses` (`first_name`, `last_name`, `extra_info`, `address`, `city`, `state`, `zip`) VALUES ('" . mysql_real_escape_string($_POST['first_name']) . "', '" . mysql_real_escape_string($_POST['last_name']) . "', '" . mysql_real_escape_string($_POST['extra_info']) . "', '" . mysql_real_escape_string($_POST['address']) . "', '" . mysql_real_escape_string($_POST['city']) . "', '" . mysql_real_escape_string($_POST['state']) . "', '" . mysql_real_escape_string($_POST['zip']) . "')"; mysql_query($sql,$con) or die('Error: ' . mysql_error()); echo "1 record added"; echo '<a ref="http://localhost/index.html">Click here to enter another record</a>'; mysql_close($con); ?>
-
Is it ?
function first_and_last() { $names = func_get_args(); $str = ''; foreach($names as $name) $str .= $name[0] . '.<br/>'; return $str; } echo first_and_last('John', 'Brad', 'Joseph');
-
Do you mean this ?
$str = '<li>Some kinda <b>text</b></li> <li>With lot of <i>html</i> tags in it</li> '; preg_match_all("#<li>(.*)</li>#isU", $str, $match); echo '<pre>'.print_r($match[1], 1).'</pre>';
-
Do you mean it ?
function first_and_last() { $names = func_get_args(); return array_map(create_function('$item', 'return $item[0] . ".";'), $names); } echo '<pre>'.print_r(first_and_last('John', 'Brad', 'Joseph'), 1).'</pre>';
-
In function.php you must write
<?php function first_and_last($first_name, $last_name ) { return $first_name[0] . '. ' . $last_name[0] . '.'; } echo first_and_last($_POST['first_name'], $_POST['last_name']);
-
Do you mean this
function first_and_last ($first_name, $last_name){ return substr($first_name, 0, 1) . '. ' . substr($last_name, 0, 1) . '.'; }
After, you can call this function
echo first_and_last('John', 'Smith');
-
Sorry. I've corrected my code.
-
"Agency Stores - Table 1" - is there name of your table ?
Also not initialized variables $dates and $store will cause Notice with error_reporting(E_ALL)
You must initialize their
mysql_connect('localhost','root',''); mysql_select_db('myDB'); $dates = isset($_POST['date']) ? $_POST['date'] : NULL; $store = isset($_POST['store']) ? $_POST['sotore'] : NULL; // these actually display the correct information mysql_query("UPDATE `Agency Stores - Table 1` SET `date` = '".$date."' WHERE `F3` = '".$store."' ") or die(mysql_error());
-
OK.
What do you not obtained do?
-
Do you want parse username from leagueoflegends.com ?
-
Show me, what showing to you var_dump() ?
-
What errors you getting by this script ?
-
so can any one guide me how can i read json in php
You can try decode JSON that way
$data = file_get_contents('GET_JSON_DATA_FROM_SOME_PAGE'); var_dump(json_decode($data));
-
empty - return true if you pass it 0.
Therefore better use 'isset'
elseif(!isset($_POST['agree'])){ echo "You need to check the box"; }
-
Again.
If you wrote
while($rows = mysql_fetch_array($result))
'extract' you must same $rows !!!
extract($rows);
And after extract($rows); you can write instead $rows['weight'], just $weight.
Using PHP OOP concept connecting to MySQL database
in PHP Coding Help
Posted
For connection with database using OOP concepts, better using 'Singleton' pattern.
For creating only one instance of object.