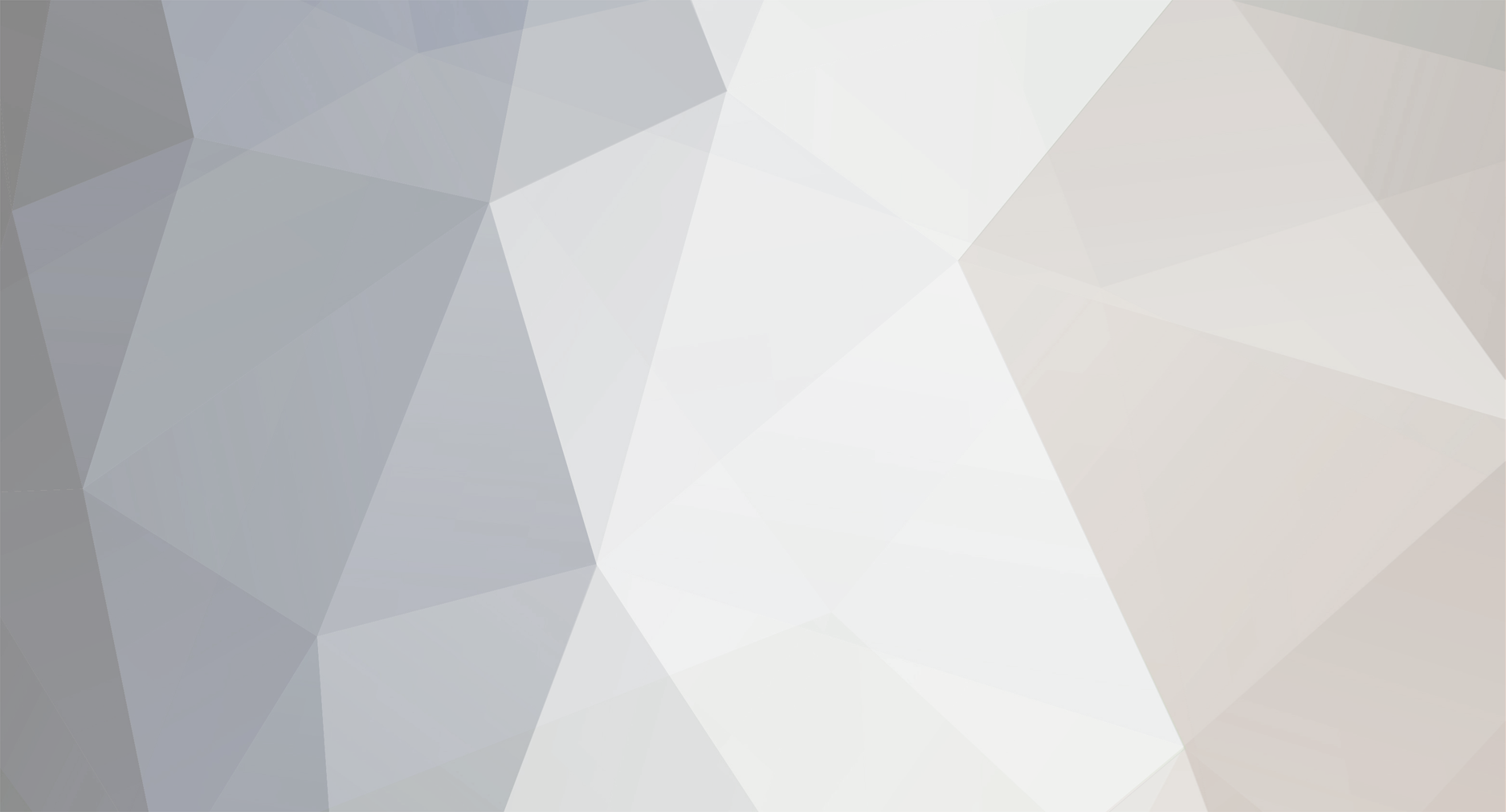
batwimp
Members-
Posts
319 -
Joined
-
Last visited
Everything posted by batwimp
-
Ok. Back to basics. This may look ugly, but do this: $results = search_results($keywords); var_dump($results); Also, we should have output $results instead of $row. Let's see what the var_dump says.
-
Was that with my code or yours? If it was with yours, put my code back and add those two lines to the top inside the php tags and tell me what the error says.
-
See those two lines of code in my signature? Put them at the top of the page, inside the php tags. Let me know if you get an error output to the screen and what it says.
-
OK. I see. You can switch it back now.
-
It looks like you it's still outputting your old code. If you view the source, you can still see the echo statements on each $row, which I removed in my code.
-
I can't test your code because I don't have your database, etc. set up. But try this and tell me what errors it puts out: <?php include 'func.inc.php'; ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd"> <html> <head> <title>search</title> </head> <body> <form action="" method="POST"> <p> <input type="text" name="keywords" size="17" maxlength="9" value="Enter B/L No." onfocus="if(this.value == 'Enter B/L No.'){this.value = '';}" /> <input type="submit" class="formbutton" value="Track" /> </p> </form> <?php if (isset($_POST['keywords'])) { $suffix = ""; $keywords = mysql_real_escape_string(htmlentities(trim($_POST['keywords']))); $errors = array(); if (empty($keywords)) { $errors[] = ''; } else if (strlen($keywords)<9) { $errors[] = '<br><strong>Your Bill of Lading Number must contain 9-digits.</strong>'; } else if (search_results($keywords) === false) { $errors[] = '<br><strong>Please enter a valid Bill of Lading Number.</strong>'; } if (empty($errors)) { $results = search_results($keywords); $results_num = count($results); $suffix = ($results_num !=1) ? 's' : ''; foreach($results as $result) { echo "<br><table> <thead> <tr> <th><strong>B/L No.</strong></th> <th><strong>Origin City</strong></th> <th><strong>Origin Country</strong></th> <th><strong>Destination City</strong></th> <th><strong>Destination Country</strong></th> <th><strong>Status</strong></th> <th><strong>Current Location</strong></th> </tr> </thead> <tbody>"; while ($row = mysql_fetch_assoc($results) { echo " <tr> <td> {$row['Bill_No']}</td> <td> {$row['Origin_City']}</td> <td> {$row['Origin_Country']}</td> <td> {$row['Destination_City']}</td> <td> {$row['Destination_Country']}</td> <td> {$row['Status']}</td> <td> {$row['Current_Location']}</td> </tr> "; } echo" </tbody> </table>"; } } else { foreach($errors as $error) { echo $error, '</br>'; } } } ?> </body> </html>
-
You also don't want to echo out the php tags such as: <?php echo $row['Bill_No'] ?> Since that is already inside an echo, just concatenate it into the string.
-
When you echo out the table, try switching the single quotes with double quotes (and vice-versa). It will not parse PHP variables that are inside singles quotes, but it will if they are within double quotes. If you look at the source for the page it produces, you will see your PHP code in your HTML.
-
xyph, how long did it take your server to process those 20,000 iterations and give you back your final hash? Just curious.
-
What have you coded so far? Remember to put any code you post inside code tags.
-
Did you remember to call session_start() at the top of the page?
-
There's some debate on whether MD5 is good for hashing passwords. But either way, it's a lot better than nothing. And yes, use salt. Much tastier!
-
It doesn't look like you are hashing your passwords. You may want to consider this for added security. Also, you need to take a few more steps to log the user out. You're going to want to destroy the session and delete any cookies: http://us2.php.net/manual/en/function.session-destroy.php
-
hw can i get drop down form to change sort by form asc or desc?
batwimp replied to Lisa23's topic in PHP Coding Help
This this: <select name="sortby" onChange="this.form.submit()"> -
It seems like you could make this a lot more efficient by adding more tables and joining them (relational database). Are you familiar with this concept?
-
Depending on which version of PHP you are using, empty() doesn't like being passed string offsets in arrays. You may want to consider storing your object property in a single variable and then checking to see if that variable is empty. Then you can also just use that variable for inserting it into your HTML when needed.
-
Get value from newly inserted row through form?
batwimp replied to xwishmasterx's topic in PHP Coding Help
http://us2.php.net/manual/en/function.mysql-insert-id.php $sql = mysql_query("SELECT invite_id FROM phpfox_invite WHERE email='".mysql_real_escape_string(stripslashes($_REQUEST['email']))."'"); $result = mysql_fetch_array( $sql ); $inviteid = mysql_insert_id(); -
You don't necessarily want a catch-all function to sanitize your inputs unless you put checks in to see what data type each input is (string, integer, etc.) because each different data type requires a different kind of sanitation. So strings should use trim and mysql_real_escape_string, integers should be cast to integers -- (int)$value -- or use some other type of method such as intval(). Most programmers know what kind of data they expect for a given variable, and sanitize it accordingly. Ultimately if you want to pass off your sanitizing to a function, you need some kind of checks inside that function to verify what type of data it is and apply sanitation accordingly. Search for some tutorials on google and youtube for php data sanitizing and you should get some good info.
-
Oops, right. You're passing in scalar data instead of arrays. My bad. Inside your function you may want to do something like: function sanitize_data($data) // create the function sanitize_data { $data = mysql_real_escape_string(trim($data)); return $data; }
-
Your sanitize_data function is no longer doing anything but calling itself. I think he meant for you to keep the function the way it was, but put the post values into an array and call sanitize_data from outside the function. Something like this: function sanitize_data($data) // create the function sanitize_data { $data = array_map('trim',$data); $data = array_map('strip_tags',$data); $data = array_map('htmlspecialchars',$data); $data = array_map('mysql_real_escape_string',$data); return $data; } $post = array(); foreach($_POST as $key =>$value){ $post[$key] = sanitize_data($value); // call sanitize_data using each value from the $post array } Am I right, Muddy?
-
Prints out three images instead of two? - for loop?
batwimp replied to Adrienk's topic in PHP Coding Help
Are there only two images in that directory? If so, add a third image, run your code, and tell us what happens. -
does your config.php or adauth.php includes write anything out?
-
Right. But were you able to tell if the requirements of loop termination have been met by the variables from within the loop? You would have to break out yourself (or put a different counter inside that loop that would break out), but you should still be able to see if the variables are filling up correctly.
-
Echo out your $count and $revrows to see what they are.
-
As an aside, it's pretty weird to have one of your column names the same as your table name. I don't know if this would cause problems or not.