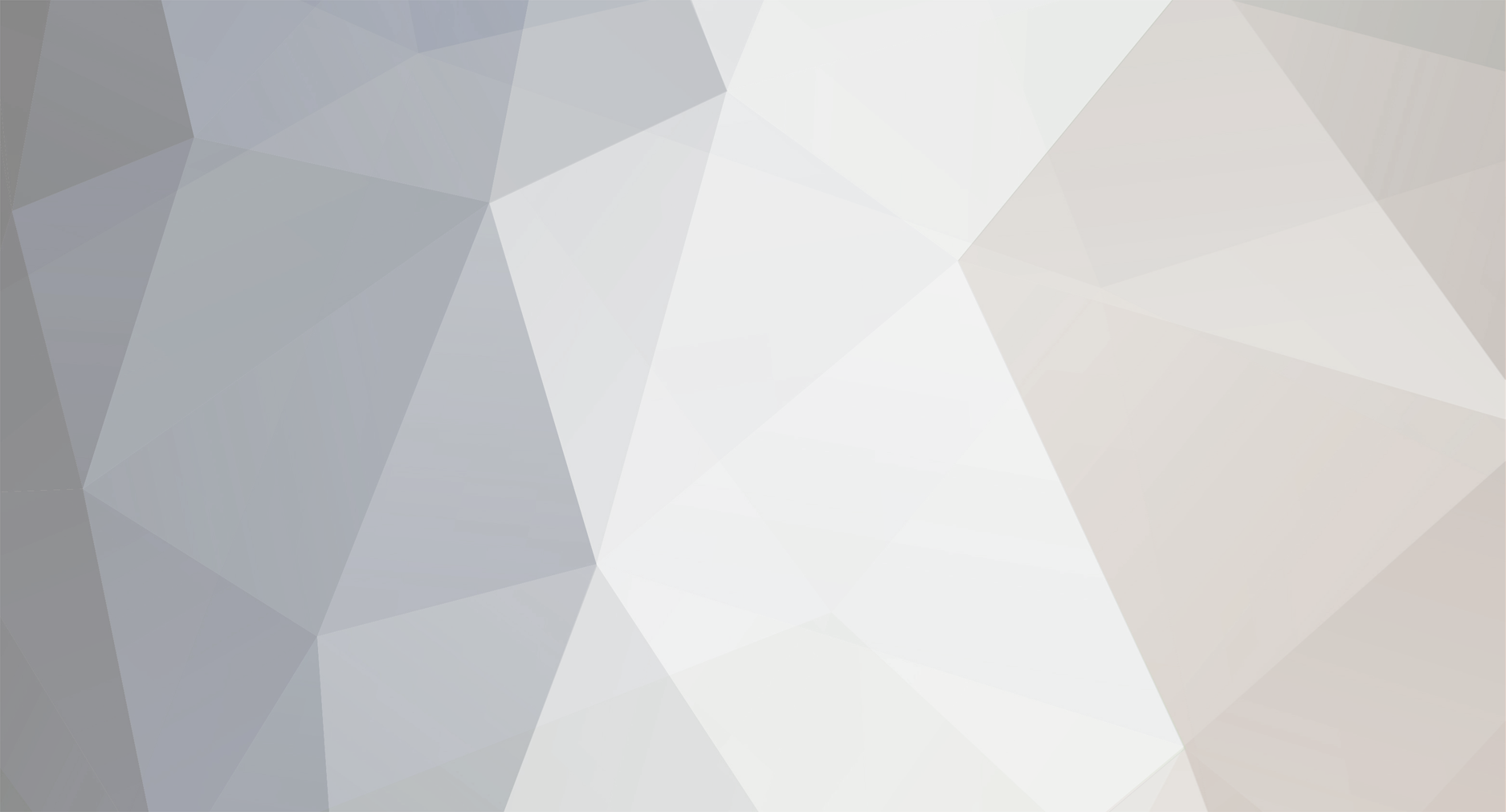
subnet_rx
Members-
Posts
44 -
Joined
-
Last visited
Never
Everything posted by subnet_rx
-
I think I've determined this is a browser issue, but I wouldn't mind someone taking a look at the code to see if I'm doing things correctly <?php $menu_id=filter_input(INPUT_GET, 'q', FILTER_SANITIZE_NUMBER_INT); if (isset($menu_id)) { $url = "http://www.mysite.com/json/mobile_menu/".$menu_id; //load menu items $string=file_get_contents($url); $menu_object=json_decode($string); $m = $menu_object->nodes[0]; //load external links in menu $lurl = "http://www.mysite.com/json/external_links/".$menu_id; //load menu items $lstring=file_get_contents($lurl); $ext_object=json_decode($lstring); } else { header( 'Location: http://m.mysite.com/' ) ; } ?> <!DOCTYPE html> <html> <head> <title><?php print($m->menu->Menu_Title); ?></title> <?php require_once 'includes/header.inc.php'; ?> </head> <body> <div id="nid_menu" data-role="page"> <?php require_once 'includes/header_div.php'; ?> <div data-role="content"> <h1 class="menu_header"><?php print($m->menu->Menu_Title); ?></h1> <ul data-role="listview" data-dividertheme="a"> <?php if (isset($m->menu->Title)) { foreach($menu_object->nodes as $m) { if ($m->menu->Type == "mobile_menu") { echo '<li><a href="./menu.php?q='.$m->menu->Nid.'">'.$m->menu->Title.'</a></li>'; } else { echo '<li><a href="./page.php?q='.$m->menu->Nid.'">'.$m->menu->Title.'</a></li>'; } } } ?> <?php if (array_key_exists("nodes",get_object_vars($ext_object))) { ?> <li data-role="list-divider">Other Resources</li> <?php foreach($ext_object->nodes as $e) { echo '<li>'.$e->link->Link.'</li>'; }} ?> </ul> </div> <?php require_once 'includes/footer.php'; ?> </div> </body> </html>
-
I have a page that processes a variable passed in the url to go get information out of a text file in JSON format. For some reason though, passing one variable loads a page, passing a certain variable (who's data in the file is virtually identical to the first) will cause the browser to just load the page indefinitely. Maybe even more strange is that on my local machine, the variable that won't load is switched, and the other loads fine. How can I debug what's happening when the page never loads so that I never get an error?
-
This is not really a backup. I need a subset of information from a larger database. The larger database cannot be accessed remotely. So, I'm basically getting it to put out a xml feed of the subset of information (that is safe to be public) to then pull into my own local db. If there are other solutions given the restrictions, I'm up for other solutions because my current system is not going to perform very well for large datasets.
-
Even if the database is limited to localhost access only, and your site is not on localhost?
-
Well, not exactly, while the information is in a database, I don't want or need all of it. I'm simply pulling from it as a central source of data. I'm actually putting the data in a RSS feed, then importing into my application.
-
I'm updating my database with a set of data from another database. When there is an item deleted from the source db, how would I make sure it's deleted from the db copy given that I have to run the update in a batch and can't run it when the item is deleted? Would I truncate the db at the beginning of each update, then pull all the of data over?
-
I have urls coming in from an RSS feed. I need to change them to add one thing. index.php should be index2.php. What is the best way to do this?
-
It's not my script, just something open-source, I'm just trying to modify it to get it to work like I want it to.
-
I have rows in a db stored with dates in the form of: 2007-07-07 00:00:00 (type is text). I need to get all dates between now and 14 days from now. Can I get some help on how I should do this? Since the type is text, do I have to use strlen? Or can I do this all in the query somehow?
-
Ok, I'm working on this again today, and I think I have gotten what your saying. When I add a unique index to these columns it gives me errors because there are duplicate entries. How can I force this and remove the extras all at once?
-
I guess I need to read more on this. I don't want to just keep the first occurrence, but in my previous example, just delete the second 1002 2 row. I'd keep the other occurrences of 1002.
-
Thanks for the replies, I'm just getting around to doing this. I don't think that the unique field idea is going to work for me, since it's not really unique. For example, the two columns I'm talking about might look like this: item_id user_id 1000 1 1002 1 1003 1 1002 2 1002 2 So, I would want to delete one of the rows where the item_id and user_id matches on two different rows. Basically, my form checking wasn't good enough, and some people entered their form twice when this shouldn't be possible.
-
I'm trying to remove duplicate records in a database. They will have a unique primary key, but there's another field in the database that should be unique for each person. So, if the same $row['item_id'] exists for the same $row['userid'] on two rows, then I need to delete one of them. What would be the best way of doing this?
-
Thanks, yeah, it said to test with === which I've never personally used before, but I'm about to give this a try. Due to circumstances out of my control, it's going to go on a production server without testing on the particular platform so that's why I'm trying to get my ducks in a row.
-
If it's not found, will it just return false or an error?
-
I've never been that good with regex. I have a variable that contains a string (an HTML file). I'm looking for the phrase: "Unable to open RSS Feed". How would I look for this in this variable?
-
I'm getting this error when a local file tries to get a file off the internet. Both of these settings in php.ini are on: ; Whether to allow the treatment of URLs (like http:// or ftp://) as files. allow_url_fopen = On ; Whether to allow include/require to open URLs (like http:// or ftp://) as files. allow_url_include = On I still get the error.
-
ok, thanks, I'll do that then in case I ever release the script.
-
Since I'm on PHP 5.2, would this work? date_default_timezone_set('America/Chicago'); So, then time() would be set for CST?
-
Will time() always give you the time in relation to the server? If so, how can I compensate for +1 hour in the timestamp? I'm using PEAR:Calendar to set the ending time of an event and then when it is displayed, I check if time() > timestamp and if so, say the event is closed. But I'm on CST time and the server is EST.
-
Here's the complete function at this point: function update_stats($type) { global $db; $a = 200; $points = array(); $wins = array(); $losses = array(); $totals = array(); $outcomes = $db->query_read("SELECT SUM(outcomes) FROM picks_groups WHERE type = ".$type.""); $user_results = $db->query_read("SELECT * FROM picks_users"); while ($users= $db->fetch_array($user_results)) { $wins[$users['userid']] = 0; $losses[$users['userid']] = 0; $points[$users['userid']] = 0; $totals[$users['userid']] = 0; $picks_results = $db->query_read("SELECT picks_bets_placed.userid,picks_bets_placed.outcome,picks_bets_placed.settled,picks_bets_placed.group_id,picks_groups.type FROM picks_bets_placed,picks_groups WHERE userid = ".$users['userid']." AND picks_groups.group_id = picks_bets_placed.group_id"); while ($picks= $db->fetch_array($picks_results)) { if($picks['outcome']=='W' AND $picks['type']==$type AND $picks['settled']=='Y') { $wins[$users['userid']] += 1; } elseif($picks['outcome']=='L' AND $picks['type']==$type AND $picks['settled']=='Y') { $losses[$users['userid']] += 1; } } $totals[$users['userid']] = $wins[$users['userid']] + $losses[$users['userid']]; $win = $wins[$users['userid']]; $loss = $losses[$users['userid']]; //$pow = (($win/2*$j)*(($win/$j)-$loss/$j)); $pow = ($win/($loss+1))*($win-$loss)/$outcomes; $a2 = (.25*$outcomes)+1; // So its always greater than 1 $value = ceil(($a*$a2*exp($pow))+$b); $points[$users['userid']] = $value; } arsort($points); foreach ($points as $key => $val) { $id_results = $db->query_read("SELECT username FROM user WHERE userid = ".$key.""); $id = $db->fetch_array($id_results); $username=$id['username']; $line_item .= "<tr><td>".$username."</td><td>".$wins[$key]."</td><td>".$losses[$key]."</td><td>".$points[$key]."</td></tr>"; } return $line_item; }
-
Ohh, no, I want to have separate rankings for each type. There are 3 now, but there could be 10 in the future. So, I would like rankings for each. That's why when I run this function, I run it dependent on a type variable. The way I store the data now, I can know two totals. The total number of outcomes since the league (type) started and the total number of wins/losses for the player. What I do not know is the total number of outcomes since the player started. I'm not recording time right now, but I intend to institute that very soon.
-
I'll wait and see what you come up with. Right now, I've plugged all the values in and am getting output for all 3 groups (high school, college, nfl), but even though I initialize everything at 0, the points add up to something different in each group. In High School, a person with 0-0 gets 1500 points. In college, which runs second, they get 4650. By the time it gets to NFL, they have 7750. Here's the relevant part of the code: while ($users= $db->fetch_array($user_results)) { $wins[$users['userid']] = 0; $losses[$users['userid']] = 0; $points[$users['userid']] = 0; $totals[$users['userid']] = 0; $picks_results = $db->query_read("SELECT picks_bets_placed.userid,picks_bets_placed.outcome,picks_bets_placed.settled,picks_bets_placed.group_id,picks_groups.type FROM picks_bets_placed,picks_groups WHERE userid = ".$users['userid']." AND picks_groups.group_id = picks_bets_placed.group_id"); while ($picks= $db->fetch_array($picks_results)) { if($picks['outcome']=='W' AND $picks['type']==$type AND $picks['settled']=='Y') { $wins[$users['userid']] += 1; } elseif($picks['outcome']=='L' AND $picks['type']==$type AND $picks['settled']=='Y') { $losses[$users['userid']] += 1; } } $totals[$users['userid']] = $wins[$users['userid']] + $losses[$users['userid']]; $win = $wins[$users['userid']]; $loss = $losses[$users['userid']]; //$pow = (($win/2*$j)*(($win/$j)-$loss/$j)); $pow = ($win/($loss+1))*($win-$loss)/$outcomes; $a2 = (.25*$outcomes)+1; // So its always greater than 1 $value = ceil(($a*$a2*exp($pow))+$b); $points[$users['userid']] = $value; }
-
I'm not really understanding why $b is in there. It starts at 0 and stays at 0 unless I'm missing a line.