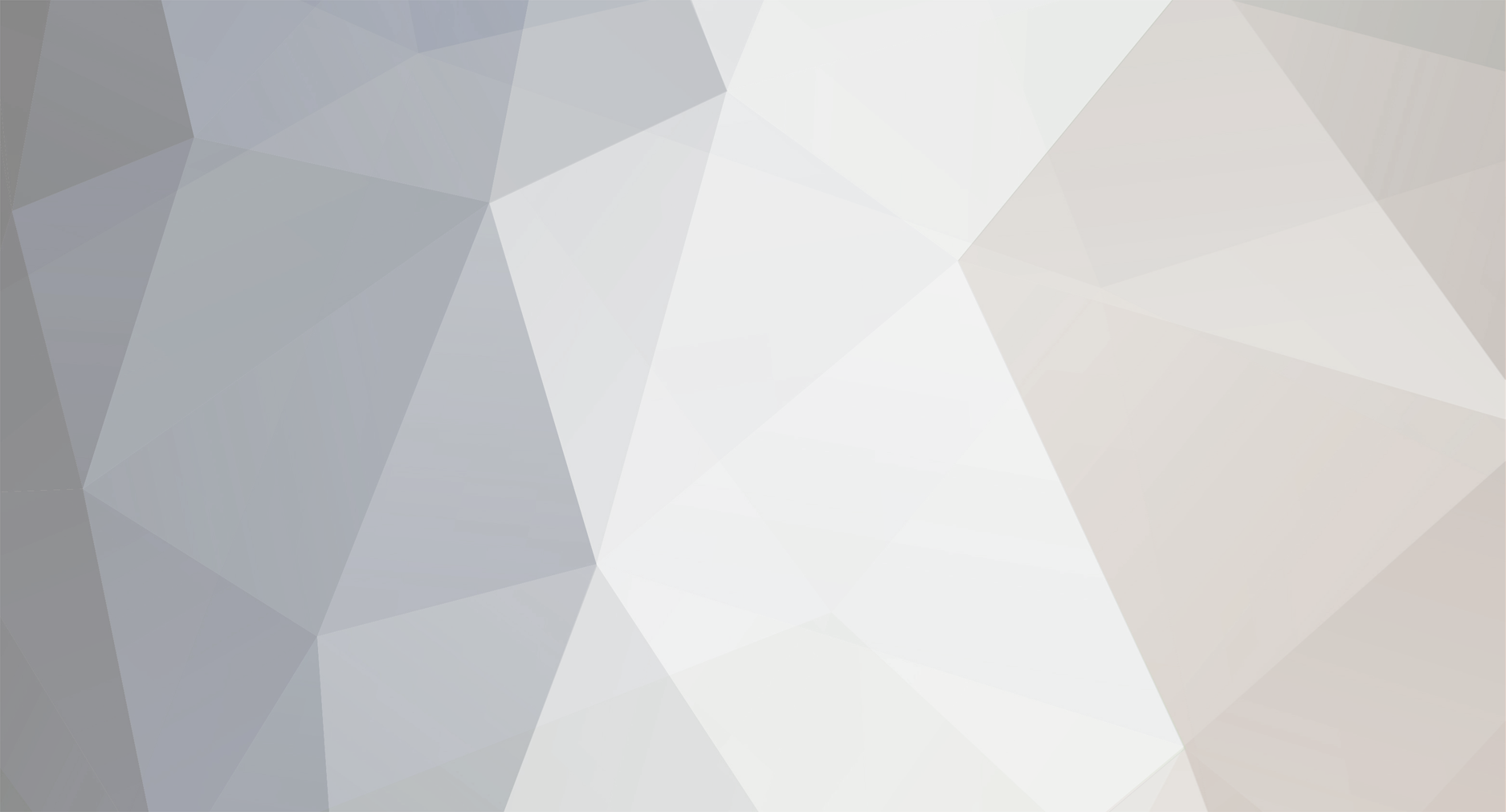
Mistral 🤖
Members-
Posts
46 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Mistral 🤖
-
I have a PHP script that runs in a WIMP environment. The script contacts several remote locations, retrieves data, and then attempts to insert it into our MySQL database. The script is set to run each morning at 5:45am, 6:42am, and 7:42am. The steps: contact store get data delete all old data present for the current store for the current day insert new data I have the script log what it's doing.The problem is that more than half the time when it runs on the scheduled task, it contacts the 1st store and the script stops working when it tries to delete the old data. **However, when I manually run it from the command line it always works.** What could cause the script to stop working when run as a scheduled task? I'm not sure if this matters, but the table contains over 2 million rows. Thanks!
-
That worked, thank you!
-
We have a server with windows 2008 R2 with PHP5 installed. I can run php files from the command line using php -f file.php with no problem. However, when I try to run PHP code inline nothing happens: php -r 'echo "hello world";' Literally no output. Do I need to configure something in php.ini to get the -r to work? Thanks!
-
Turned out there was a stray \r at the end of each element. After removing it the implode works.
-
I have an array like this: $myarray Array ( [0] => [email protected] [1] => [email protected] [2] => [email protected] ) When I do: implode(',', $myarray); I get: ,[email protected] The 1st 2 elements are missing. I have another array that looks exactly like the one above but it has 15 elements, and when I do the same exact implode() statement I get a comma-separated list with all 15 elements. Why is implode() missing the 1st 2 elements? Thanks!
-
We have a Debian Linux server with Apache2. One page, feedback.php has a super-simple form: <form action="feedback.php" method="post"> <div class="type"><input type="radio" name="type" id="compliment" value="compliment" /><label for="compliment">Compliment</label></div> <div class="type"><input type="radio" name="type" id="complaint" value="complaint" /><label for="complaint">Complaint</label></div> <div class="type"><input type="radio" name="type" id="general" value="general" /><label for="general">General</label></div> <br /> <input type="submit" name="submit" class="begin_comments" value="Begin Comments" /> <br /> <br /> <br /> <span class="error"></span> </form> This form posts back to itself, and handles it like this: PHP Code: session_start(); if ($_SERVER['REQUEST_METHOD'] == 'POST') { if (isset($_POST['type'])) { $_SESSION['type'] = $_POST['type']; //echo $_SERVER['REQUEST_METHOD']; header('Location: form.php'); } else { $error = 'You must select a comment type to begin'; } } However, when form.php loads, $_POST, $_GET, or $_REQUEST are all empty arrays. What could cause this? Thanks!
-
Thanks so much for your help!
-
I figured out a way around the issue. The php web page still generates a .bat file that looks like this: cmd /c cscript.exe c:\scripts\labor\scheduled\labor.vbs /store_list:"store1;store2" /start_date:"2013-02-03" /end_date:"2013-02-05" However, it doesn't try to execute the batch file. Instead, I have this: <?php $cmd = 'schtasks /create /sc once /tn "repost_labor" /tr "c:\scripts\labor\scheduled\repost_labor.bat" /st ' . $time; exec($cmd, $out, $retval); ?> where $time is the next minute after the php script runs. In the .bat file on the line before the cmd /c cscript.exe ... line, the php adds this: cmd /c schtasks /delete /tn "repost_labor" /u <uname> /p <pwd> /f /s <domain name> So even though php can't execute the batch file directly, I can have it create a scheduled task that's set to run 1 minute after the script executes that will then run the batch file. When the scheduled task runs the batch file, the batch file then immediately removes the scheduled task and then runs the cscript. It's not a perfect solution, but it's 99% of the way there. Having it execute 1 minute later is totally fine for what this process needs to do.
-
I tried adding in the %ERRORLEVEL% after the line where it's supposed to execute the cscript.exe and it echos back 0 ("success"). If there's something that's just blocking PHP executed in the Web context from running .bat or .vbs files in folders other than the wwwroot folder I might have to use a different approach. I think I'll experiment with virtual folders.
-
I tried what you said and "Running" was echo'ed back, so we know the batch file is running. I also again tried adding cmd /c to the batch file, so now the batch file looks like this: @echo off echo Running cmd /c cscript.exe c:\scripts\labor\scheduled\labor.vbs /store_list:"store1;store2" /start_date:"2013-02-03" /end_date:"2013-02-05" What could be blocking the cscript? Could it be a permissions issue? I tried setting the permissions on cscript.exe, labor.vbs, and repost_labor.bat to allow "Everyone", "Users", "Authenticated Users", and "IUSR" to have read/execute or full access. Could antivirus be blocking it? Is there some way I can have the batch file echo back if/why a command doesn't execute (some error code)? Thanks!!
-
I tried @echo off but it didn't work. Here is the code: $cmd .= 'c:\scripts\labor\scheduled\cscript.exe c:\scripts\labor\scheduled\labor.vbs /store_list:"' . urldecode($store_list) . '" /start_date:"' . urldecode($start_date) . '" /end_date:"' . urldecode($end_date) . '"'; $fname = 'c:\scripts\labor\scheduled\repost_labor.bat'; $fp = fopen($fname, 'w'); fwrite($fp, "@echo off\n" . $cmd); fclose($fp); exec($fname, $out, $retval); print_r($out); echo($retval); $out shows an empty array when I put the @echo off in. If I leave the @echo off out, I get this: Array ( [0] => [1] => C:\inetpub\wwwroot\functions>c:\scripts\labor\scheduled\cscript.exe c:\scripts\labor\scheduled\labor.vbs /store_list:"store1;store2" /start_date:"2013-01-21" /end_date:"2013-01-24" ) And $retval echos back 0.
-
That was just a typo. Typing too fast.
-
I tried: cmd.exe cmd /c.exe psexec in combination with all of the different php commands above (exec, shell_exec, etc.). Could it have something to do with the files I'm trying to run not being in the wwwroot folder?
-
We have a web portal in a WIMP environment. There is a web page that users can fill out a form. When the form submits I need it to create and execute a batch file on the server that is in a folder outside the wwwroot folder. What happens is the user submits the form & the php is able to create the .bat file in c:/scripts/labor/scheduled/labor_repost.bat, no problem. The contents of the batch file is a single line that looks like: c:\scripts\labor\scheduled\cscript.exe c:\scripts\labor\scheduled\labor.vbs /store_list:"store1;store2" /start_date:"2013-01-21" /end_date:"2013-01-24" If I execute the .bat file from the command line manually it works perfectly. The cscript.exe, labor.vbs, and labor_repost.bat files are all in the same folder. In testing, I've tried setting permissions on all these files and the folder they're in to allow these users full control: Everyone Authenticated Users IUSR System Users However, nothing I try from PHP is able to execute the .bat file. I have tried: exec('c:/scripts/labor/scheduled/labor_repost.bat', $out, $retval); $out = shell_exec('c:/scripts/labor/scheduled/labor_repost.bat'); system('c:/scripts/labor/scheduled/labor_repost.bat'); $ws = new COM('WScript.Shell'); $w = $ws->Run('c:/scripts/labor/scheduled/labor_repost.bat', 0, false); I've also tried various other combinations of this by including "psexec" and "cmd /c". None of these will execute the batch file - no matter which one I try, nothing happens. If I echo back the return value from the batch file, it will just echo back the contents of the file. I don't get any error messages. Is it possible that it's a permissions issue? If so, what would need permission to what? We checked the antivirus logs and it doesn't appear to be that. Please help. Thank you!
-
I tried putting in the full path to the file but it still didn't work. I've determined that it's some sort of permissions issue. I get this error when cscript.exe tries to run: CScript Error: Loading your settings failed. (Access is denied. ) It's running on iis Win 2008.
-
I made some changes trying psexec, but it still isn't working. However, it runs perfectly from the command line. I think it's gotta be close and it may have something to do with quoting/escaping double quotation marks or /'s or \'s, but I don't know what it's looking for: error_reporting(E_ALL); ini_set('display_errors', '1'); if($_POST['store_list'] && $_POST['start_date'] && $_POST['end_date']){ echo 'processing<br><br>'; $cmd = 'psexec \\<computername.domainname.com> -u <username> -p <password> -e c:\windows\system32\cscript.exe c:\scripts\labor\scheduled\labor.vbs /store_list:"store1;store2" /start_date:"2013-01-21" /end_date:"2013-01-24"'; echo $cmd.'<br><br>'; $out = shell_exec($cmd); echo '[' . $out.']<br><br>'; echo '<br>end'; } else{ echo 'no data'; }
-
1. I tried both manually typing the command on the command line and pasting in the command line that the script is generating. Both work. 2. I was referencing the path to the script with an absolute path like: c:\path\to\file\my_script.vbs I also tried replacing the \'s with \\ and with /
-
I've spent several hours searching and trying different things, but I can't get this script to run. It is called by a web page: error_reporting(E_ALL); ini_set('display_errors', '1'); if($_POST['store_list'] && $_POST['start_date'] && $_POST['end_date']){ $cmd = 'wscript.exe <path_to_script>/my_script.vbs /store_list:"' . urldecode($_POST['store_list']) . '" /start_date:"' . urldecode($_POST['start_date']) . '" /end_date:"' . urldecode($_POST['end_date']) . '"'; $ws = new COM('WScript.Shell'); $ws->Run('cmd /c ' . $cmd, 0, false); } I tried: with & without wscript.exe, cscript.exe, cmd /c, cmd.exe /c, cmd.exe /c /k referencing the path to the .vbs file with /, \, and \\ exec() shell_exec() When I run the script from the command line it works perfectly. The .vbs file is in a path outside the wwwroot folder. I set the permissions on the .vbs file & the folder it's in to allow read & execute for the Internet Guest Account. The web page seems to indicate the script is being executed but nothing happens. Process Explorer only shows the error: "[Error opening process]" but no other information. Please help.
-
That worked, thank you!
-
I'm trying to write a variable number of arguments to a table. I was looking in the php documentation of bind_param and it mentioned using a ReflectionClass and invokeArgs to do it. I've been trying and I can't get it to work. Say this is my array. The first argument is the types string: $myarr => ( [0] = "sssssi" [1] = "'test'" [2] = "'ttt'" [3] = "'tester'" [4] = "'2015-01-01'" [5] = "'test'" [6] = 0 ) And here is the code: $res = $mysqli->prepare($qry); $ref = new ReflectionClass('mysqli_stmt'); $method = $ref->getMethod("bind_param"); $method->invokeArgs($res,$myarr); $res->execute(); Please help me understand what I'm doing wrong. Thanks!