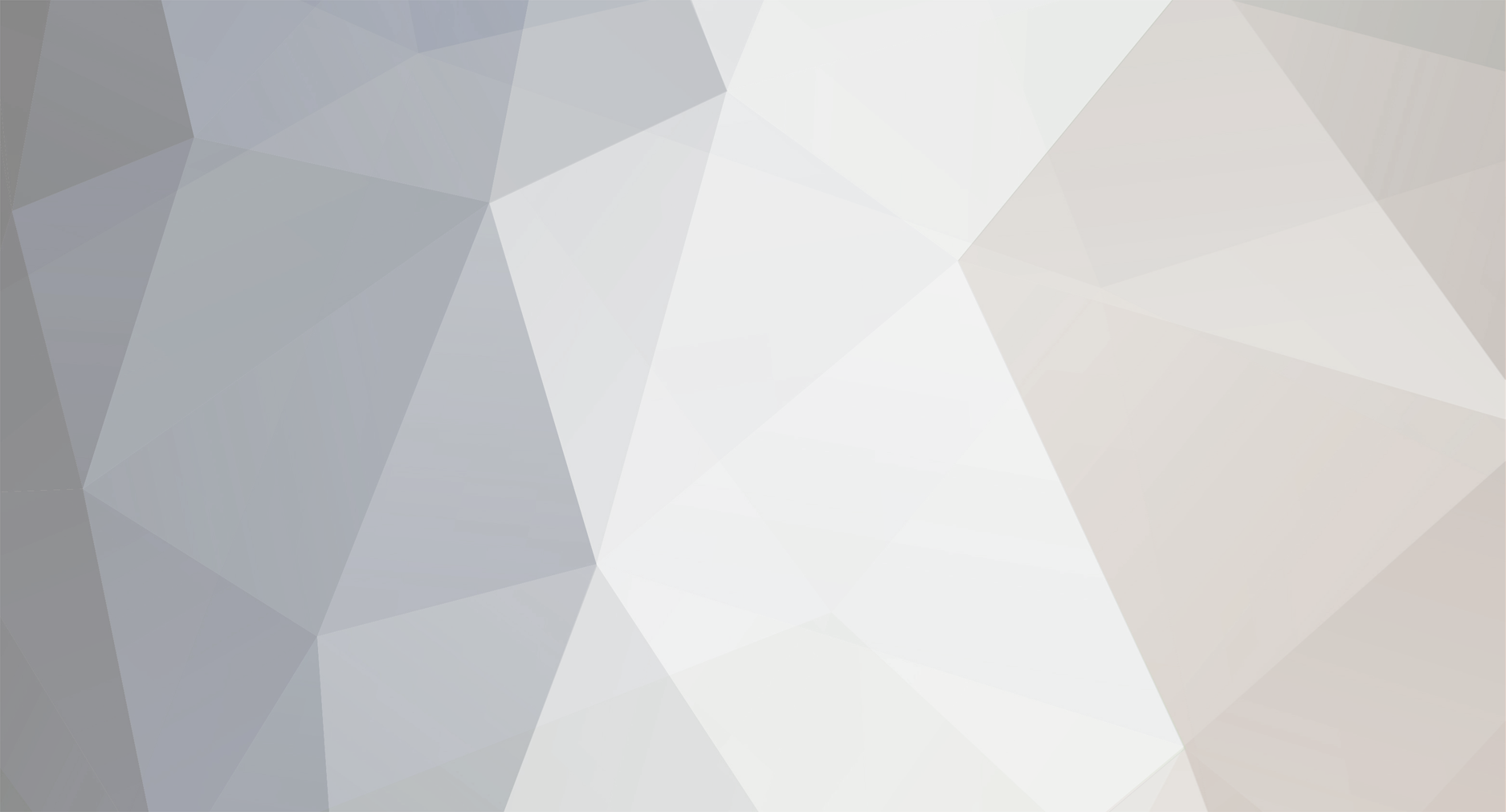
Kimomaru
Members-
Posts
14 -
Joined
-
Last visited
Never
Everything posted by Kimomaru
-
Cool, thanks Jesirose.
-
Hello, I have a simple form for user registration that, right now, requires that all fields be filled out. I would like to change the php code so that two of the fields will not throw an error if they're not filled out (date of birth and middle name), but the rest should be mandatory. The code looks like this; $FirstName=$_POST['FirstName']; $MiddleName=$_POST['MiddleName']; $LastName=$_POST['LastName']; $DateOfBirth=$_POST['DateOfBirth']; $Email=$_POST['Email']; $UserName=$_POST['UserName']; $Password=$_POST['Password']; $Password2=$_POST['Password2']; session_start(); try { if (!filled_out($_POST)) { throw new Exception('You have not filled the form out correctly - please go back and try again.'); } _______________________________ function filled_out($form_vars) { foreach ($form_vars as $key => $value) { if ((!isset($key)) || ($value == '')) { return false; } } return true; } My guess is that the code that needs to be changed is the part that's in bold, but I'm not sure how I can change it to skip the two values that I would like to make optional. Can someone help?
-
Thanks, requinix.
-
My code is generating an error because EREG is deprecated. It's warning me that I should not use it, but am wondering what I should use instead. This is what I'm putting together; function valid_email($address) { if (ereg('^[a-zA-Z0-9_\.\-]+@[a-zA-Z0-9\-]+\.[a-zA-Z0-9\-\.]+$', $address)) { return true; } else { return false; } } Any help or direction is appreciated as always.
-
Loud and clear. Thank you so much for clearing that up, the examples I was looking at confused me. I'm on the right track now.
-
Hey KevinM1, I went back and read your example again, and I think I understand where I was messing up. I needed to understand at what point the PHP opens and closes were happening around the HTML, especially as they pertained to the declaration of the function and code blocks themseves. This was stumping me; <?php function display_html_header() { ?> <html> <head> <title>Blah blah blah</title> </head> <body> <table width="100%" bgcolor="white" cellpadding="12" border="0"> <tr> <td> <p><font size="10">Blah blah blah</font></p> </td> <td align="right"> <? date_default_timezone_set('MST') ?> <? echo date('l, F jS Y'); ?> </td> </tr> </table> </body> </html> <?php } ?> Which is basically that php is being opened and closed for the function, then there's HTML, then it's opened and closed again for the close brace (which was opened during the function). I think I'm going to make a conscious effort to break things down int he code more than I have.
-
Hey Jesirose, That's what it sounds like, but I think I'm starting to understand. Okay, question - Why does this work; <?php function display_html_header() { ?> <html> <head> <title>Blah blah blah</title> </head> <body> <table width="100%" bgcolor="white" cellpadding="12" border="0"> <tr> <td> <p><font size="10">Blah blah blah</font></p> </td> <td align="right"> <? date_default_timezone_set('MST') ?> <? echo date('l, F jS Y'); ?> </td> </tr> </table> </body> </html> <?php } But this does not work; <?php function display_html_header() { ?> <html> <head> <title>Blah blah blah</title> </head> <body> <table width="100%" bgcolor="white" cellpadding="12" border="0"> <tr> <td> <p><font size="10">Blah blah blah</font></p> </td> <td align="right"> <? date_default_timezone_set('MST') ?> <? echo date('l, F jS Y'); ?> </td> </tr> </table> </body> </html> ?> } And I already see most of the problem, there's a closing php tag that is without an opener. The first two refer to the open and close for the function, does this mean that I need to add an opener for the code block?
-
Hi Drummin, Before this morning, I had my header and footers in separate files and called them separately. The PHP book I'm reading suggested to put this content in a different files that contains functions for producing output. Personally, I'm very much into a modular approach as it is easier to track and manage objects, but since I'm new it's difficult to gauge the prevailing "wisdom".
-
Hi Jesirose, First, please recommend a good book. Second - with respect to the open and close PHP syntax, thank you for putting it that way, seriously. In a post I put up yesterday someone replied to me; http://forums.phpfreaks.com/index.php?topic=361252.0 "To close the function you have to go back into PHP code with another <?php. <?php function display_site_info() { ?> <ul> <li>This is test gibberish</li> </ul> <?php }" So, clearly I misunderstood. I could just close it plainly with "?>". The context of the thread would have been referring to a situation where I would be adding another php block of code, in which case (please correct me if I'm wrong), the new opening tag would close the preceding one?
-
Hey Kevin, Now I'm even more confused, but I suspect that it's due mainly to my lack of experience. I'm working off of the book entitled "PHP and MySQL Web Development (4th Edition)", this is how they've laid out the code. Someone in the Amazon Customer Reviews section (named Travis Parks) mentioned that this book does not teach how to write PHP properly. It's puzzling for the newcomer and I can't tell if I'm painting myself into a corner. The first example you mentioned is understandable; <?php // opening tag if($x == true) { // note the brace opening brace // code // code // code } // <-- the closing brace ends the code that is executed if the if-conditional is true closing brace // no closing php tag in this example, but I'm assuming it would be "<?php" ? The second example is harder to understand; <?php open // code // code if(/* something */) { // opening brace brace is opened before the closing php tag but closed after? ?> <-- end of PHP mode, but the rest of the code is STILL taking place within the code block started by the brace above [close] <html> <!-- blah blah blah --> <?php } // closing brace, which closes the block - ALWAYS match braces brace closed after close of block? ?>
-
Hello, It took me about an hour to work through a syntax problem in a PHP file containing functions for test web page I'm learning with. I know how to get something to work, but I don't understand it. In the following syntax I have included functions for a header, some main body information, and a footer. However, I can't figure out how the the opening and closing tags relate to each other. Please refer to commented lines before, thank you for any help or advice. <?php // This is the opening tag function display_html_header() { ?> // This is the closing tag <html> // Here is my HTML <head> <title>Blah Blah Blah</title> </head> <body> <table width="100%" bgcolor="white" cellpadding="12" border="0"> <tr> <td> <p><font size="10">Blah Blah Blah</font></p> </td> <td align="right"> <? date_default_timezone_set('MST') ?> <? echo date('l, F jS Y'); ?> </td> </tr> </table> </body> </html> <?php // Why is this opening tag needed here? I understand that it's needed to make the above syntax work. So, it's a closer? } function display_site_info() { // Does this new section need an opener? What that in the preceding tag? ?> <ul> <li>Blah Blah Blah!</li> </ul> <?php } function display_html_footer() { ?> <table bgcolor="white" cellpadding="12" border="0" align="center"> <tr> <td>Privacy and Terms </td> <td>Contact Us </td> <td>About </td> </tr> </table> </body> </html> <?php }
-
Trouble with functions syntax, please help :(
Kimomaru replied to Kimomaru's topic in PHP Coding Help
Hi requinix, I figured out the problem. My site_fns.php file was calling up .php files that do not exist yet, so it was running into errors and not rendering the site_fns.php file's content. When I commented those out, everything works fine now. Thank you for your help, it was very much appreciated -
Trouble with functions syntax, please help :(
Kimomaru replied to Kimomaru's topic in PHP Coding Help
Hi requinix, Thank you for replying. I originally tried that before posting (and just tried it now) and it still won't render it Does that mean that the problem must be somewhere else on the main page? Confounding . . . -
Hello, I'm working on implementing a very simple web page for my learning of PHP (in preparation for implementing with mySQL). It should be straight forward, but I'm having difficulty understanding how function syntax should work. On my main page, I'm calling a header, footer, and another PHP file; <?php require('header.php'); require_once('site_fns.php'); display_site_info(); require('footer.php');?> The header and footer show up fine up until the site_fns.php syntax, after which everything underneath the header can't be rendered. The site_fns.php file looks like this; <?php require_once('data_valid_fns.php'); require_once('db_fns.php'); require_once('user_auth_fns.php'); require_once('output_fns.php'); ?> The display_site_info() function is in the output_fns.php file, I'm positive that the problem is here; <?php function display_site_info() { ?> <ul> <li>This is test gibberish</li> </ul> } Can someone helpe me understand what I'm doing wrong? I'm very new to this, so any help would be appreciated.