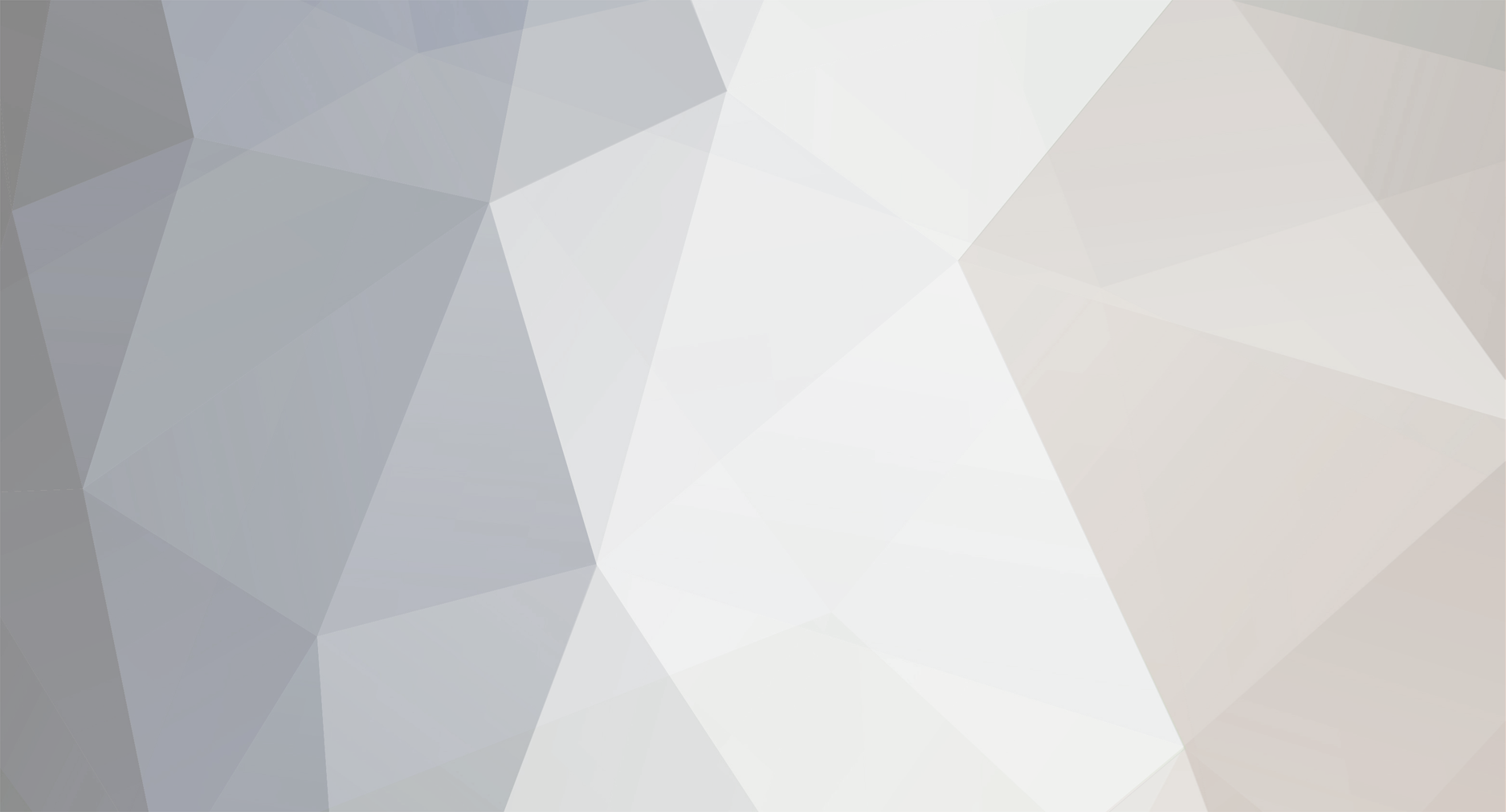
Christian F.
-
Posts
3,072 -
Joined
-
Last visited
-
Days Won
18
We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.