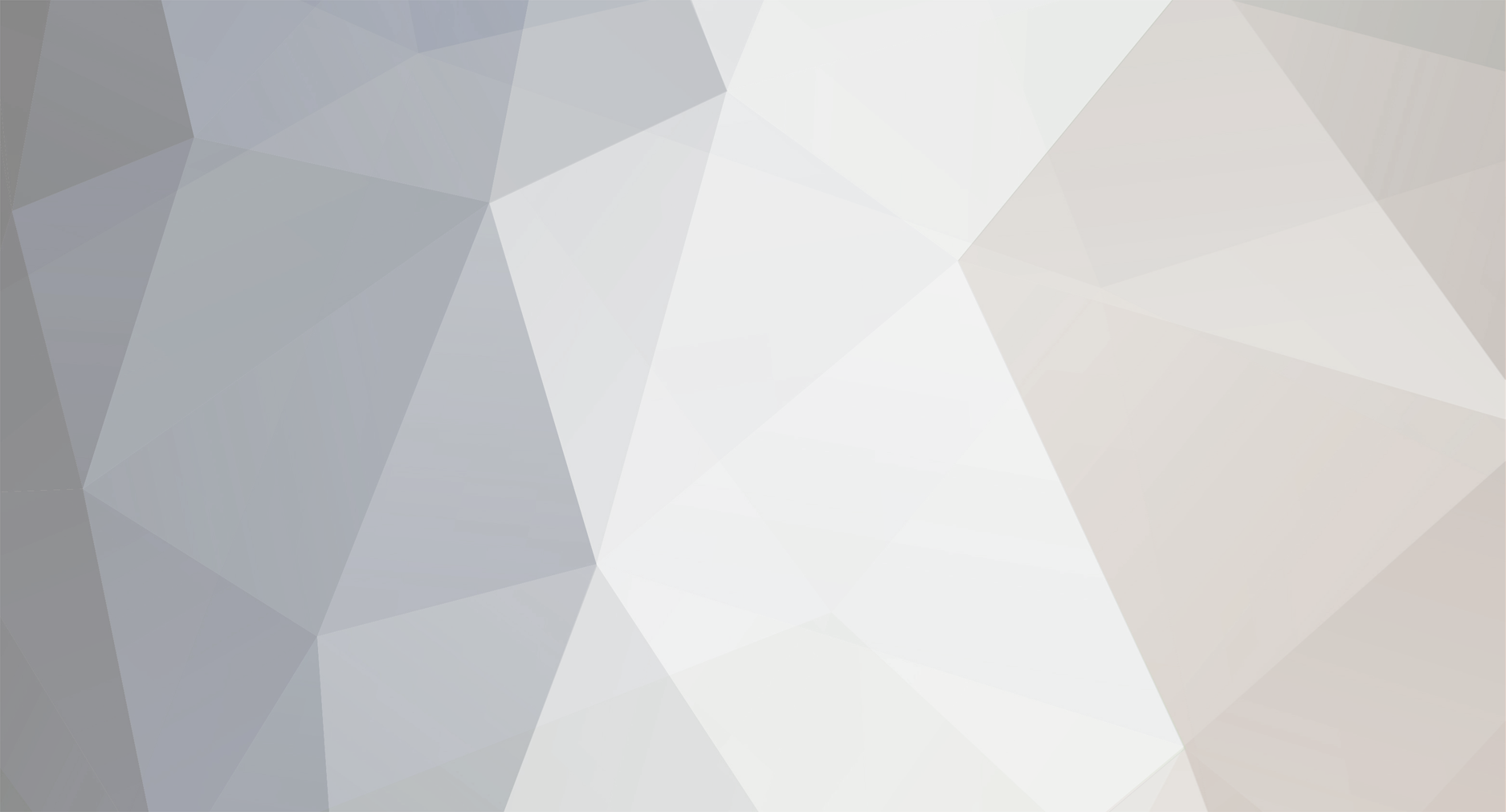
trg86
-
Posts
64 -
Joined
-
Last visited
Posts posted by trg86
-
-
Christian, thank you for your input. I'm not taking offense to the constructive criticism, but I know HTML and CSS like the back of my hand, I've been doing it for years. During development I tend to get into bad habits of using lengthy old font tags and line-breaks just to see how things will look, before I finalize them (in CSS), you will also notice that I do have some permanent css classes already coded in as well.
As for the validation of data in the form processor, I beg to differ sir, as I have a separate validation class already programmed, I have just not implemented it into this system yet.
But, I will take your advice with the PHP and the SQL, those are the areas I am newer in.
-
Thanks again though EG, you have been helpful with several items on my project.
-
Lol, it seems like the simplest problems are always the hardest to find...hehe
-
Lol, yes in answer to your question about the $i, but...
It was a very simple problem that I just fixed....in my database structure. There was a row below the archive row, that I removed since I am not using it at the moment, and that completely solved the problem!
-
You are not issuing any errors if your query is incorrect, which is what I suspect. Without knowing your database structure it would be impossible add
echo mysql_error();
before your mysql_close();
Done...still no errors.
-
Still the same issue, as well as a possible further solution, I believe the problem may be in my processor all of a sudden. Now that I logged into my database via phpMyAdmin, the data is not even being saved to the database anymore, but is still successfully hitting my e-mail. Here is the code for my processor, maybe we can find the culprit there:
<?php // //GATHERED DATA FROM THE USERS FORM ENTRIES AND DATABASE LOGIN // $username="REMOVED_FOR_THIS_POST"; //Database username $password="REMOVED_FOR_THIS_POST"; //Database password $database="REMOVED_FOR_THIS_POST"; //Database name $name = htmlspecialchars($_POST['name']); $email = htmlspecialchars($_POST['email']); $age = htmlspecialchars($_POST['age']); $gender = htmlspecialchars($_POST['gender']); $location = htmlspecialchars($_POST['location']); $homephone = htmlspecialchars($_POST['homephone']); $referrer = htmlspecialchars($_POST['referrer']); mysql_connect("REMOVED_FOR_THIS_POST",$username,$password); //Connection to Database @mysql_select_db($database) or die( "ALERT! Database not found!"); //Database Selection $query = "INSERT INTO leads VALUES ('','$name','$email','$age','$gender','$location','$homephone','$referrer', '0')"; //Insert Form Values To Database mysql_query($query); mysql_close(); ?>
Everything below that is just for sending the data to my e-mail, no relevancy.
-
You see where you're using mysql_close(); ?
Yup, 'tis that the problem? Should I move that statement to the bottom of the document?
-
The following is the entire code for the main page that displays the form data, which WAS WORKING BEFORE. Additionally, I know the form is firing still because the same data is also emailed to me, as well as hitting the database.
<?php session_start(); if (!isset($_SESSION['username'])) { exit(); } ?> <?php error_reporting(E_ALL); ini_set('display_errors', '1'); $db_username="REMOVED_FOR_THIS_POST"; //Database Username $password="REMOVED_FOR_THIS_POST"; //Database Password $database="REMOVED_FOR_THIS_POST"; //Database Name mysql_connect("REMOVED_FOR_THIS_POST",$db_username,$password); //Connection to Database @mysql_select_db($database) or die("ALERT! Database not found!"); //Selection of Database $query="SELECT * FROM leads WHERE `archive` = '0' ORDER by id DESC"; //Database Table to Query //Query Sort Settings $result=mysql_query($query); $num=mysql_numrows($result); mysql_close(); ?> <html> <head> <link href="css/db_style.css" rel="stylesheet" type="text/css" media="screen" /> </head> <body> <div id="wrap"> <div id="top_bar"> <font color="#0092c8"><?php echo "<b>Welcome</b> ".$_SESSION['username'];?></font>, <?php echo('<a href="logout.php">Logout</a>');?> || <b>Current Database:</b> <FONT color="#0092c8" face="verdana" size="2">REMOVED_FOR_THIS_POST</font> || <b>Current Date/Time:</b> <FONT color="#0092c8" face="verdana" size="2"><script type="text/javascript"> document.write ('<span id="date-time">', new Date().toLocaleString(), '<\/span>') if (document.getElementById) onload = function () { setInterval ("document.getElementById ('date-time').firstChild.data = new Date().toLocaleString()", 50) } </script></font> </div> </div><br> <div id="wrap"> <div id="top_user_toolbar"> <b>Control Panel</b> | <a href="leads_archive.php" class="toolbar_link">Archived Leads</a> | <a href="#" class="toolbar_link">Active Leads</a> | <a href="#" class="toolbar_link">Appointment Calender</a> | <a href="pm/message_center.php" class="toolbar_link">Message Center</a></font> </div> </div><br> <div id="wrap"> <div id="top_notification_box"> <b>Notification:</b> <font color="#0992c8">PLEASE REPORT ANY BUGS, THIS IS A WORK IN PROGRESS!</font> </div> </div> <div id="wrap"> <br><br> <table class="leads_table"> <tr><td><center><font class="small_black"><b>New Leads</font></center></b></td></tr> <tr> <th bgcolor="#9e0219"><font face="Arial, Helvetica, sans-serif" size="2" color="#FFFFFF">Name</font></th> <th bgcolor="#9e0219"><font face="Arial, Helvetica, sans-serif" size="2" color="#FFFFFF">E-Mail</font></th> <th bgcolor="#9e0219"><font face="Arial, Helvetica, sans-serif" size="2" color="#FFFFFF">Age</font></th> <th bgcolor="#9e0219"><font face="Arial, Helvetica, sans-serif" size="2" color="#FFFFFF">Gender</font></th> <th bgcolor="#9e0219"><font face="Arial, Helvetica, sans-serif" size="2" color="#FFFFFF">Location</font></th> <th bgcolor="#9e0219"><font face="Arial, Helvetica, sans-serif" size="2" color="#FFFFFF">Phone Number</font></th> <th bgcolor="#9e0219"><font face="Arial, Helvetica, sans-serif" size="2" color="#FFFFFF">Referrer</font></th> <th bgcolor="#9e0219"><font face="Arial, Helvetica, sans-serif" size="2" color="#FFFFFF"><b>- - - Options - - -</b></font></th> </tr> </div> <?php $i=0; while ($i < $num) { $id=mysql_result($result,$i,"id"); //Unique ID Field $name=mysql_result($result,$i,"name"); //Name $email=mysql_result($result,$i,"email"); //EMail Address $age=mysql_result($result,$i,"age"); //Age $gender=mysql_result($result,$i,"gender"); //Gender $location=mysql_result($result,$i,"location"); //City of Residence $homephone=mysql_result($result,$i,"homephone"); //Home Phone Number $referrer=mysql_result($result,$i,"referrer"); //Referrer ?> <tr> <td align="center" bgcolor="#FFFFFF"><font class="lead_txt"><? echo $name; ?></font></td> <td align="center" bgcolor="#FFFFFF"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $email; ?></font></td> <td align="center" bgcolor="#FFFFFF"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $age; ?></font></td> <td align="center" bgcolor="#FFFFFF"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $gender; ?></font></td> <td align="center" bgcolor="#FFFFFF"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $location; ?></font></td> <td align="center" bgcolor="#FFFFFF"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $homephone; ?></font></td> <td align="center" bgcolor="#FFFFFF"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $referrer; ?></font></td> <td align="center" bgcolor="#fdfdfd"><a href="db_edit.php?id=<?php echo $id; ?>"><img src="images/edit.png" border="0" width="32" height="32" alt="Edit" title="Edit Lead Credentials"></a> <a href="#"><img src="images/active.png" border="0" width="32" height="32" alt="Active" title="Move To Active Leads"> <a href="add_to_archive.php?id=<?php echo $id; ?>"><img src="images/archive.png" border="0" width="32" height="32" alt="Delete" title="Archive Lead"> <a href="db_remove.php?id=<?php echo $id; ?>"><img src="images/delete.png" border="0" width="32" height="32" alt="Delete" title="Delete Lead From Database"></a></td> </tr> </center> </font> </body> </html> <?php $i++; } echo "</table>"; ?>
-
Hmm I hope this was just a typing mistake. Your code at the top of the page I overlooked
$query="SELECT * FROM leads WHERE `archive` = '0' ORDER by id DESC;
You're missing the end quote
That was my typing mistake
The end quote is there in the actual file.
-
Can you please post your code? Your sql seems legit
What do you need to see?
-
So it is still not being set as 0 when inserting into the database? Are there any errors?
Nope, no errors on the page, for error reporting, I am using:
error_reporting(E_ALL); ini_set('display_errors', '1');
-
Exactly
Cool deal, I just tried it before you responded, but it is still not wanting to work...hmmm...
-
Oh okay, more like this then?:
$query = "INSERT INTO leads VALUES ('','$name','$email','$age','$gender','$location','$homephone','$referrer', '0');
-
Dont use set in an insert query...it won't work just insert normally and where you would be inserting into archive just put a 0
Thanks for your reply EG, although I am slightly confused with your response...
-
Hey guys, me again. This is all based off of the same couple of items you have helped me with the past couple of days. For those of you that know already, I have been working on a database that stores and displays information via a submitted form from the clients end. By default, all entries show up on a main page, where they can be edited, archived(which moves it to an archive page) or deleted. Now, I have the archive page working, that properly displays only archived entries, using the code below:
$query="SELECT * FROM leads WHERE `archive` = '1' ORDER by id DESC;
The problem that I am having now is that the main page, using this code:
$query="SELECT * FROM leads WHERE `archive` = '0' ORDER by id DESC;
is no longer displaying the entries once they are submitted through the form, even though it was working before, without the extra WHERE statement, which is also how it still works, but will displayed the archived leads as well, which I DO NOT want, any advice on what I may be doing wrong?
I added a new statement to the form processor as well to try and set the archive to 0 when it is inserted into the database, but still no luck, that code is as follows:
$query = "INSERT INTO leads VALUES ('','$name','$email','$age','$gender','$location','$homephone','$referrer') SET `archive` = '0' ";
Thanks guys!!
-
Alrighty, I have received enough help, I have this fully functioning now, thanks everybody!
-
To show archive's just change the query:
$sql = "SELECT * FROM `table` WHERE `archive` = 1";
Already done!
-
Submit the form with an id of what you're archiving in the url such as archive.php?id=id
Just call it by using
$id = mysql_real_escape_string($_GET['id']);
Then use sql to update the information
$sql = "UPDATE `table` SET `archive` = '1' WHERE `id` = '$id'";
To call all of them that aren't archived just use a while loop and a where clause as followed:
$sql = "SELECT * FROM `table` WHERE `archive` = '0'"; $result = mysql_query($sql,$connection) or die("Error connecting to the database"); while($row = mysql_fetch_array($result)) { // How you want to display it here. Examples shown below $title = $row['title']; $info = $row['info']; $id = $row['id']; // Using so many echos to keep it easy to read echo "$title<br>"; echo "$info<br>"; echo "<form action='archive.php?id=$id' method='post'>"; echo "<input type='submit' value='Archive This'>"; echo "</form>"; }
Thank you for the example, although I did not need the whole code, just needed to add this option to my current structure. I also took previous advice that I needed to set it up just like my already complete 'edit' function, which I have done. I have this successfully working now, just need to make the archive page to display just the archived leads...
-
Add a field to the table called "archived." Set that field to 0. Add a button called "archive this" that will flip the "archived" field on a post to 1. Have your main page only show items where archived = 0. Have an archive page if you wish, which only shows archived = 1.
Additionally, what would be the best way to do this?
I already have the button created and in place
-
Boolean (int 1)
I have it:
`archive` INT( 1 ) NOT NULL ;
should work?
-
What would the proper settings be for this column in the database?
-
Just add an archive COLUMN, not a separate table. You would modify this with an update, just as you are with your 'edit' buttons.
Oh okay, I understand better now, sounds simple enough.
Thanks!
I will let you know if I need any further assistance.
-
Do I add the 'archive' table to the same mysql table I have it pulling the results from? or create a new table within the same database?
-
I'm not quite sure of the exact code I should use to do this.
Need Some Quick Help..
in PHP Coding Help
Posted
Okay, advice taken.
Quick question though, even though my current setup of the code works, what is the benefit/difference of doing it this way?