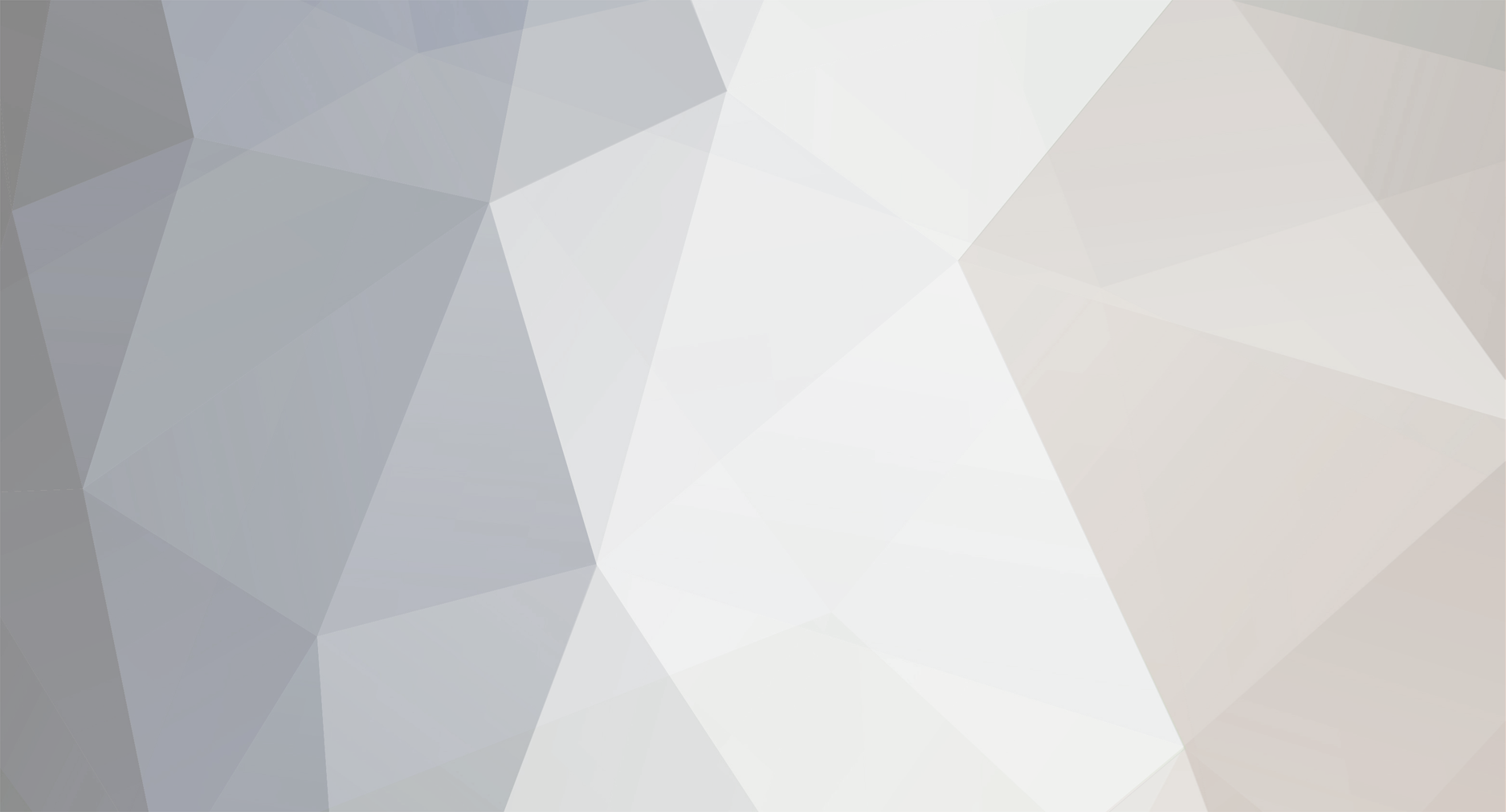
designanddev
Members-
Posts
54 -
Joined
-
Last visited
Everything posted by designanddev
-
what about the return what do i do, should i return false like i current had done first time round
-
when i use $this is breaks and comes up with an error message
-
This is the whole user class <?php require_once("database.php"); class User extends DatabaseObject{ protected static $table_name = "users"; protected static $db_fields = array('id', 'username', 'password', 'first_name', 'last_name', 'email'); public $id; public $username; public $password; public $first_name; public $last_name; public $email; public $errors = array(); // Initialize an error array. // Check for an email first name: public static function Reg($first_name, $last_name) { if(!empty($first_name) && !empty($last_name)) { $register = new User(); $register->first_name = $first_name; $register->last_name = $last_name; return $register; } else{ if(empty($first_name)){ $errors[] = 'You forgot to enter your username.'; } if(empty($last_name)){ $errors[] = 'You forgot to enter your lastname.'; } return $errors; }} public static function Authenticate($username="", $password=""){ global $database; $username = $database->escape($username); $password = $database->escape($password); $sql = "SELECT * FROM users "; $sql .= "WHERE username = '{$username}' "; $sql .= "AND password = '{$password}' "; $sql .= "LIMIT 1"; $result_array = self::find_by_sql($sql); return !empty($result_array)? array_shift($result_array): false; } public function fullname(){ if(isset($this->first_name) && isset($this->last_name)){ return "first name: ".$this->first_name."</br> last name: ".$this->last_name; }else{ return ""; } } //Common Database Methods public static function find_all(){ global $database; $result_set = self::find_by_sql("SELECT * FROM ".self::$table_name); return $result_set; } public static function find_id($id=0){ global $database; $result_array = self::find_by_sql("SELECT * FROM ".self::$table_name." WHERE ID = {$id}"); return !empty($result_array) ? array_shift($result_array) : false; } public static function find_by_sql($sql=""){ global $database; $result_set = $database->query($sql); $object_array = array(); while($row = $database->mysql_fetch($result_set)){ $object_array[] = self::instantiate($row); } return $object_array; } private function instantiate($record){ $object = new self; //$object->username = $record['username']; //$object->password = $record['password']; //$object->first_name = $record['first_name']; //$object->last_name = $record['last_name']; foreach($record as $attribute => $value){ if($object->has_attribute($attribute)){ $object->$attribute = $value; } } return $object; } private function has_attribute($attribute){ $object_vars = $this->attributes(); return array_key_exists($attribute, $object_vars); } protected function attributes() { // return an array of attribute names and their values $attributes = array(); foreach(self::$db_fields as $field) { if(property_exists($this, $field)) { $attributes[$field] = $this->$field; } } return $attributes; } protected function sanitized_attributes() { global $database; $clean_attributes = array(); // sanitize the values before submitting // Note: does not alter the actual value of each attribute foreach($this->attributes() as $key => $value){ $clean_attributes[$key] = $database->escape($value); } return $clean_attributes; } public function save() { // A new record won't have an id yet. return isset($this->id) ? $this->update() : $this->create(); } public function create() { global $database; // Don't forget your SQL syntax and good habits: // - INSERT INTO table (key, key) VALUES ('value', 'value') // - single-quotes around all values // - escape all values to prevent SQL injection $attributes = $this->sanitized_attributes(); $sql = "INSERT INTO ".self::$table_name." ("; $sql .= join(", ", array_keys($attributes)); $sql .= ") VALUES ('"; $sql .= join("', '", array_values($attributes)); $sql .= "')"; if($database->query($sql)) { $this->id = $database->insert_id(); return true; } else { return false; } } public function update() { global $database; // Don't forget your SQL syntax and good habits: // - UPDATE table SET key='value', key='value' WHERE condition // - single-quotes around all values // - escape all values to prevent SQL injection $attributes = $this->sanitized_attributes(); $attribute_pairs = array(); foreach($attributes as $key => $value) { $attribute_pairs[] = "{$key}='{$value}'"; } $sql = "UPDATE ".self::$table_name." SET "; $sql .= join(", ", $attribute_pairs); $sql .= " WHERE id=". $database->escape($this->id); $database->query($sql); return ($database->affected_rows() == 1) ? true : false; } public function delete(){ global $database; // Don't forget your SQL syntax and good habits: // - DELETE FROM table WHERE condition LIMIT 1 // - escape all values to prevent SQL injection // - use LIMIT 1 $sql = "DELETE FROM ".self::$table_name; $sql .= " WHERE id=". $database->escape($this->id); $sql .= " LIMIT 1"; $database->query($sql); return ($database->affected_rows() == 1) ? true : false; // NB: After deleting, the instance of User still // exists, even though the database entry does not. // This can be useful, as in: // echo $user->first_name . " was deleted"; // but, for example, we can't call $user->update() // after calling $user->delete(). } }//END OF USER ?>
-
Ok i have been displaying my errors without using foreach loops my code was very clean after i tried to add more feature from after the tutorial, i want a way to display my errors like the example i photo example i posted earlier, i do not get the difference, im trying to use the same technique when displaying errors
-
Ok heres an example of error messages i have been using.... Submit form if(isset($_POST['submit'])){ $photo = new Photograph(); $photo->caption = $_POST['caption']; $photo->category_id = $_POST['category']; $photo->created = strftime("%Y-%m-%d %H:%M:%S", time()); $photo->attach_file($_FILES['file_upload']); $photo->admin_id = $session->user_name; //Success if($photo->save()){ $session->message("Photograph uploaded successfully."); redirect_to('list_photos.php'); }else{ //Failure $message = join("<br />", $photo->errors); } } Function... public function attach_file($file) { // Perform error checking on the form parameters if(!$file || empty($file) || !is_array($file)) { // error: nothing uploaded or wrong argument usage $this->errors[] = "No file was uploaded."; return false; } elseif($file['error'] != 0) { // error: report what PHP says went wrong $this->errors[] = $this->upload_errors[$file['error']]; return false; } else { // Set object attributes to the form parameters. $this->temp_path = $file['tmp_name']; $this->filename = basename($file['name']); $this->type = $file['type']; $this->size = $file['size']; // Don't worry about saving anything to the database yet. return true; } } public function save() { // A new record won't have an id yet. if(isset($this->id)) { // Really just to update the caption $this->update(); } else { // Make sure there are no errors // Can't save if there are pre-existing errors if(!empty($this->errors)) { return false; } // Make sure the caption is not too long for the DB if(strlen($this->caption) > 255) { $this->errors[] = "The caption can only be 255 characters long."; return false; } if(empty($this->caption)) { $this->errors[] = "please add a caption."; return false; } // Can't save without filename and temp location if(empty($this->filename) || empty($this->temp_path)) { $this->errors[] = "The file location was not available."; return false; } // Determine the target_path $target_path = "/home/designanddev.co.uk/public_html/blog/".$this->upload_dir."/".$this->filename; // Make sure a file doesn't already exist in the target location if(file_exists($target_path)) { $this->errors[] = "The file {$this->filename} already exists."; return false; } // Attempt to move the file if(move_uploaded_file($this->temp_path, $target_path)) { // Success // Save a corresponding entry to the database if($this->create()) { // We are done with temp_path, the file isn't there anymore unset($this->temp_path); return true; } } else { // File was not moved. $this->errors[] = "The file upload failed, possibly due to incorrect permissions on the upload folder."; return false; } } } And this function here allows me to locate the error messages anywhere within my script <?php echo output_message($message); ?> Hope this is understandable
-
Thank you i see but was there any need for the is_object function and is_array function? also i have my error message function that i used to place the errors anywhere on my page now how will i but this back into my function which is.. $message = join("<br />", $newuser->errors); <?php echo output_message($message); ?> i hope you understand Thanks for your help
-
im not quiet sure what you mean
-
ive tried this... public static function Reg($first_name, $last_name) { if(!empty($first_name) && !empty($last_name)) { $register = new User(); $register->first_name = $first_name; $register->last_name = $last_name; return $register; } elseif(empty($first_name)){ $errors[] = 'You forgot to enter your username.'; return false; }elseif(empty($last_name)){ $errors[] = 'You forgot to enter your lastname.'; return false;} } and my submit is now if(isset($_POST['submit'])) { $first_name = trim($_POST['first_name']); $last_name = trim($_POST['last_name']); $new_user = User::Reg($first_name, $last_name); if($new_user && $new_user->save()) { // comment saved // No message needed; seeing the comment is proof enough. // Important! You could just let the page render from here. // But then if the page is reloaded, the form will try // to resubmit the comment. So redirect instead: redirect_to("photo.php?id={$photo->id}"); } else { // Failed $message = $new_user->errors; } } else { $first_name = ""; $last_name = ""; }
-
something like this... But its not working public static function Reg($first_name, $last_name) { if(!empty($first_name) && !empty($last_name)) { $register = new User(); $register->first_name = $first_name; $register->last_name = $last_name; return $register; } else { if(empty($first_name)){ $errors[] = 'You forgot to enter your username.'; return false; }elseif(empty($last_name)){ $errors[] = 'You forgot to enter your lastname.'; return false;} } }
-
I have this code here below but i would like to display errors so if the user doesn't fill out the specific fields they will get a message to fill that in, i can only figure out how to make it display one error message. heres the code, you guys have been great so far, sorry for duplicating my messages. Kind Regards Submit form..... if(isset($_POST['submit'])) { $first_name = trim($_POST['first_name']); $last_name = trim($_POST['last_name']); $new_user = User::Reg($first_name, $last_name); if($new_user && $new_user->save()) { redirect_to("photo.php?id={$photo->id}"); } else { // Failed $message = "There was an error that prevented the comment from being saved."; } } else { $first_name = ""; $last_name = ""; } This Reg function..... public static function Reg($first_name, $last_name) { if(!empty($first_name) && !empty($last_name)) { $register = new User(); $register->first_name = $first_name; $register->last_name = $last_name; return $register; } else { return false; } }
-
can some define the differences between the 4 indexes please... Primary Unique Index Fulltext Kind regards
-
not a problem at all, just wanted to know if this could be done any other way
-
Thanks for your help Barand, you've bin great
-
how would i go about doing this ive added this (return $catdata[0]->category_name;) to my category function but is there any other way of accessing my category name without using this $catdata[0] public function category(){ $catdata = Category::get_category($this->category_id); return $catdata[0]->category_name; }
-
Thanks Barand ive been up all last night working this out, i understand a little more how this is working now but how could i add this within my class to keep it more clean, i think i need to work on how the returns are working.
-
ive tried using this to pull just the category_names.... foreach ($photos as $photo){ echo $photo->caption; $catdata = $photo->category(); echo $catdata->category_name; echo "</br></br>"; } still not working can anyone please help with get around this issue. Kind Regards
-
Hope this here is more clear for you jordan 26 Array ( [0] => Category Object ( [id] => 1 [category_name] => php ) ) jordans Array ( [0] => Category Object ( [id] => 2 [category_name] => HTML ) ) jordan 8 Array ( [0] => Category Object ( [id] => 1 [category_name] => php ) )
-
i want to be able to pull the category name out but it just returns the array at the moment the category name is set as an empty field in the database thats why its blank with the id of 4
-
Thank You This is what it returned jordan 26 Array ( ) jordans Array ( [0] => Category Object ( [id] => 4 [category_name] => ) ) jordan 8 Array ( )
-
Thanks for the reply i have tried this but the $photo->category(); keeps return Array instead of the information i need inside that Array This is the function inside the $photo->category(); public function category(){ return Category::get_category($this->id); } and this is the Category::get_category function public static function get_category($category_id=0) { global $database; $sql = "SELECT * FROM ".self::$table_name; $sql .= " WHERE id=" .$database->escape($category_id); return self::find_by_sql($sql); } The $photo->category(); keeps returning the Array but what i would like to do is access the the $category_name attribute within the Category:: class which is stored in my database. I hope some understands what i am trying to accomplish here. Kind Regards
-
i have tried this but the category::get_category(2) which i would like to be category::get_category($photo->id) is being set by another class the echo statement outputs either the numbers of (Array) but i want it to be outputting the the content string. i hope you understand i am trying to make sense of all the joins and the instantiation. // Find all the photos $photos = Photograph::find_all(); $text = Category::get_category(2); foreach ($photos as $photos){ echo $photos->caption; echo $photos->category(); echo "</br></br>"; } KInd Regards
-
i have this code here but i can not seem to to loop the $text = Category::get_category($photo->id); with the $photo. ive tried working around this but i keep getting all my loop fields saying Array, <?php // Find all the photos $photos = Photograph::find_all(); foreach ($photos as $photos){ $text = Category::get_category($photo->id); echo $photos->caption; echo "</br></br>"; } ?>
-
im using this code here to instantiate all my records from the data, but i am having trouble trying to use the join another table cell to this private function instantiate($record){ $object = new self; //$object->username = $record['username']; //$object->password = $record['password']; //$object->first_name = $record['first_name']; //$object->last_name = $record['last_name']; foreach($record as $attribute => $value){ if($object->has_attribute($attribute)){ $object->$attribute = $value; } } return $object; }