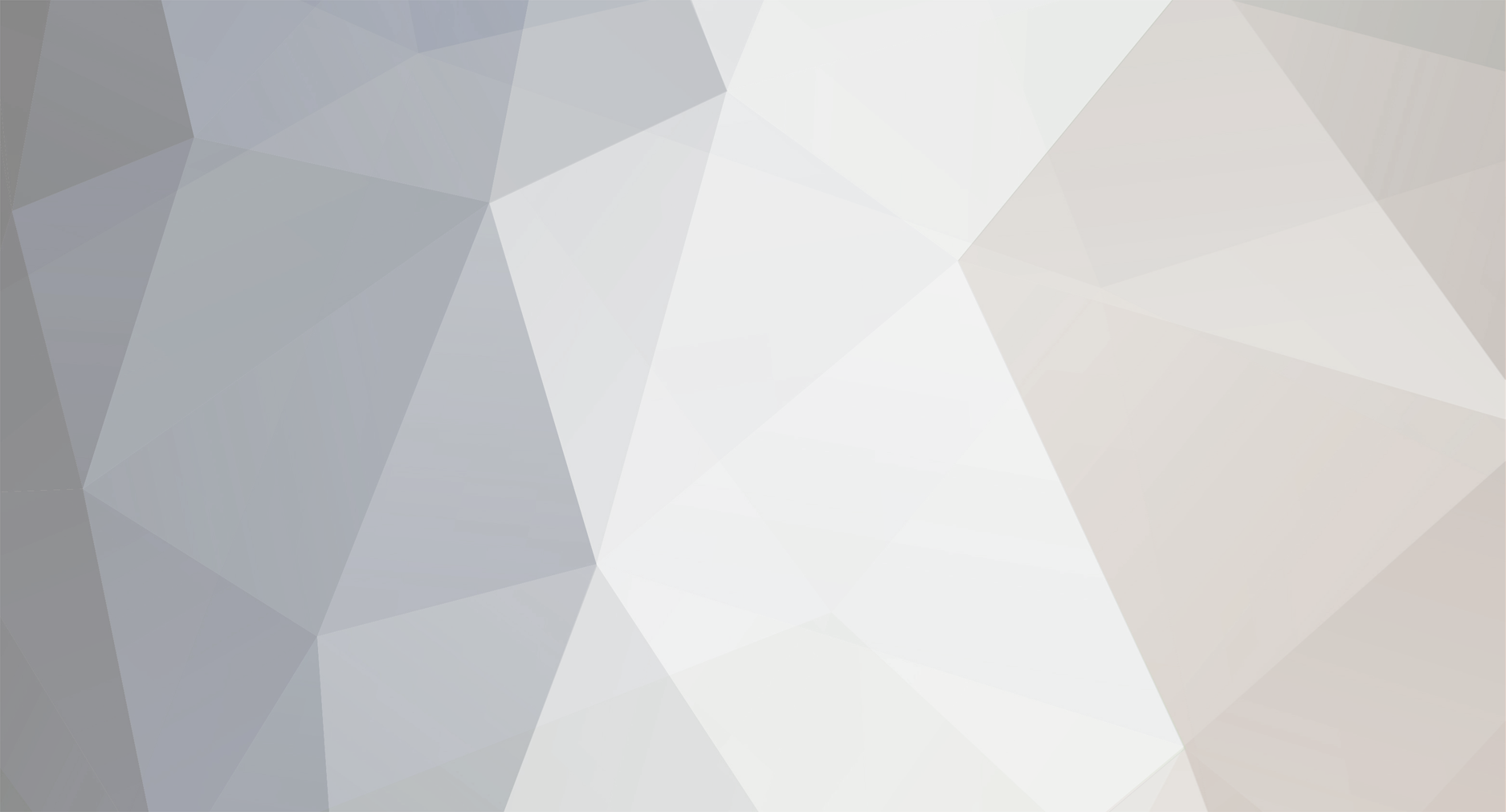
phprocker
-
Posts
91 -
Joined
-
Last visited
Never
Posts posted by phprocker
-
-
Thorpe thank you for the answer. Now what about my first question though. Is it necessary to use it for non-user-input database queries such as a config selection from a config table.
-
It is designed to sanitize data going into the database.
Couldn't there be an SQL injectionwhen there is any call to the database such as a SELECT from the table?
-
Is it necessary to use
mysql_real_escape_string()
when I'm retrieving fields from a table that the user had no input on the result?
Such as if there was a template color that the admin set in the siteconfig table that the script selects automatically with no user input.
Cheers!
-
Daylight savings? I hate the fact we rely on UTC when there is constant mix-ups with American DST. I too was just trying to configure my server a while ago for time. And I wasn't successful unless I put information I know is wrong.
-
That PHP 5 SAMS Unleashed looks good but it's published in 2004. isn't that a bit too long ago? Anything similar and more recent? Past 3 years?
-
I'm looking for a simpler way to handle the config updates rather than a bunch of if statements. Anyone point me in the right direction?
-
Hey all. I've created a script that updates a website's config in a database. The script itself works fine but I feel it is rather uncanny. Where can I look to find better methods of achieving what I'm trying to accomplish?
Here's my script that updates my table with columns id, showlogin, colorscheme, blogmenu, aboutus.
I explain the script below it.
if (isset($_POST['submit'])) { // id will always be 1 and only query if only submit was pressed $sql = "UPDATE config SET id=1"; // showlogin values are 1 for show login form and 2 for don't if(!empty($_POST['showlogin'])) { $showlogin = $_POST['showlogin']; $sql .= ", showlogin='$showlogin'"; } if(!empty($_POST['colorscheme'])) { $colorscheme = $_POST['colorscheme']; $sql .= ", colorscheme='$colorscheme'"; } if(!empty($_POST['blogmenu'])) { $blogmenu = $_POST['blogmenu']; $sql .= ", blogmenu='$blogmenu'"; } else { $sql .= ", blogmenu=2"; } if(!empty($_POST['aboutus'])) { $aboutus = $_POST['aboutus']; $sql .= ", aboutus='$aboutus'"; } else { $sql .= ", aboutus=2"; } mysql_query($sql, $connect) or die (mysql_error()); }
It works like this. I have a form with a few selects and checkboxes. The selects are the colorscheme and showlogin. The checkboxes are blogmenu and about us, both defaulting to the number 2 if they are not checked. 1 means show this item on the live site and 2 means do not show. This is pulled out of the database on page loads.
So, I hope I was clear as my mind is spaghetti right now.
Cheers!
-
Got it, thanks.
-
Hey all. I have a script that checks if a user is an admin and then allows that person to change certain page layouts and other things.
My question is, of the following 2 examples, which is best way to check if the user is an admin.
1st example:
Should I have just a session that is set if the user is an admin and then have the pages displayed if he/she is?
or
2nd example:
Should I have each and every single admin query/request check if the user is an admin or not?
What's the proper method?
Cheers!
-
It worked BlueSkyIS thanks much.
-
Where do you set mysqli, and is it declared global outside of the users class?
Ah you may have the answer. I didn't include that. It's in config.php Let me include and see if that works.
Cheers!
-
Ya I had errors off which was extremely stupid. I turned it off for another project.
Anyway, here's the error.
error on welcome.php
Fatal error: Call to a member function query() on a non-object in C:\xampp\htdocs\site\classes.php on line 24
Code:
classes.php
class users { function is_admin($username) { global $mysqli; $result = $mysqli->query("SELECT isadmin FROM users WHERE username = '$username'"); $value = $result->fetch_object(); if ($value->isadmin == 1) { return 1; } return 0; } }
welcome.php
<?php session_start(); include 'root.php'; include ROOT.DS.'classes.php'; $user = new users(); if (!isset($_SESSION['username'], $_SESSION['imadmin']) || $user->is_admin($_SESSION['username'])==0) { header('Location: index.php'); } ?> //the rest is all html
-
So there's nothing there when you do a View Source?
No.
I thought it was because I wasn't including the classes.php file but I added that and still same thing.
I have to get to sleep, thanks for looking. I'll try again in the morning and post the rest of the code when I can take a look at it through non-bloodshot eyes.
Cheers people thanks!
-
I'm a bit stumped here and as usual I'm sure it's something simple.
I have an object that checks if a user is an admin. It works fine. But it the HTML below it is not getting displayed.
Take a look.
welcome.php
session_start(); $user = new users(); if (!isset($_SESSION['username'], $_SESSION['imadmin']) || $user->is_admin($_SESSION['username'])==0) { header('Location: index.php'); }
welcome.php is getting displayed without getting redirected to the index. But there's no HTML, just the URL to welcome.php in the bar.
Anyone?
Cheers!
-
Try this instead of both lines:
mysql_query("UPDATE users SET last_access = $date WHERE username = $login")or die("QUERY FAILED: " . mysql_error());
-
I'm curious, is there any reason to use
array_pop($value)
at all when you can
explode(' ', $value, -1)
???
Besides being php version < version 5.1.0 compatible of course.
-
Cheers mate!
-
Hey all. My site has a config database table that has a column showlogin to set whether or not login functionality is enabled.
I'm playing around with MySQLi, Classes, and Returns. My return is always 1. Where am I going wrong?
test.php
<?php // Start Session session_start(); // Production Settings ini_set('display_errors', 1); mysqli_report(MYSQLI_REPORT_ALL); include 'db.php'; $mysqli = new mysqli($host, $user, $pass, $db); class styles { function showlogin($show) { global $mysqli; $select = $mysqli->query("SELECT showlogin FROM config LIMIT 1"); $value = $select->fetch_object(); if ($value->showlogin = 1) { return 1; } return 0; } } $login = new styles(); echo $login->showlogin($show); ?>
-
Hey all. I have some CSS styling in my page headers that works only when included on the page itself and not linked from a file. Can anyone see what I'm doing wrong?
index.php
<head> <link rel="stylesheet" type="text/css" href="styles/default.css" /> </head> //code that doesn't load the external style <table class="background1" width="1000px" height="420px" cellspacing="10" cellpadding="15"></table>
styles/default.css
.background1 {background-image:url(images/meh.gif);} .background2 {background-image:url(images/back.png);} img {border-style:none;}
-
If you posted the code you would get a solution 10x faster.
-
-
I actually haven't watched this yet but this guy has really great tutorials. I've been meaning to get around to watching this one myself. Hopefully I get the chance today.
Let me know if that tutorial helps you.
-
Yes, this is one way.
Another is to declare the variables you want to echo and then include your "view".
Like this:
theme.php
<html> <head></head> <body> <form> </form> <?php echo $hellovar; ?> </body> </html>
index.php
<?php $hellovar = "Hello"; include 'theme.php'; ?>
-
Split your html into a header and footer.
Try this.
themeheader.php
<html> <head></head> <body>
themefooter.php
</body> </html>
index.php
<?php // include header include 'themeheader.php'; //echo statement echo "Hello"; //include footer include 'themefooter.php'; ?>
mysql_real_escape_string Question
in PHP Coding Help
Posted
Thank you again. Marked Solved.