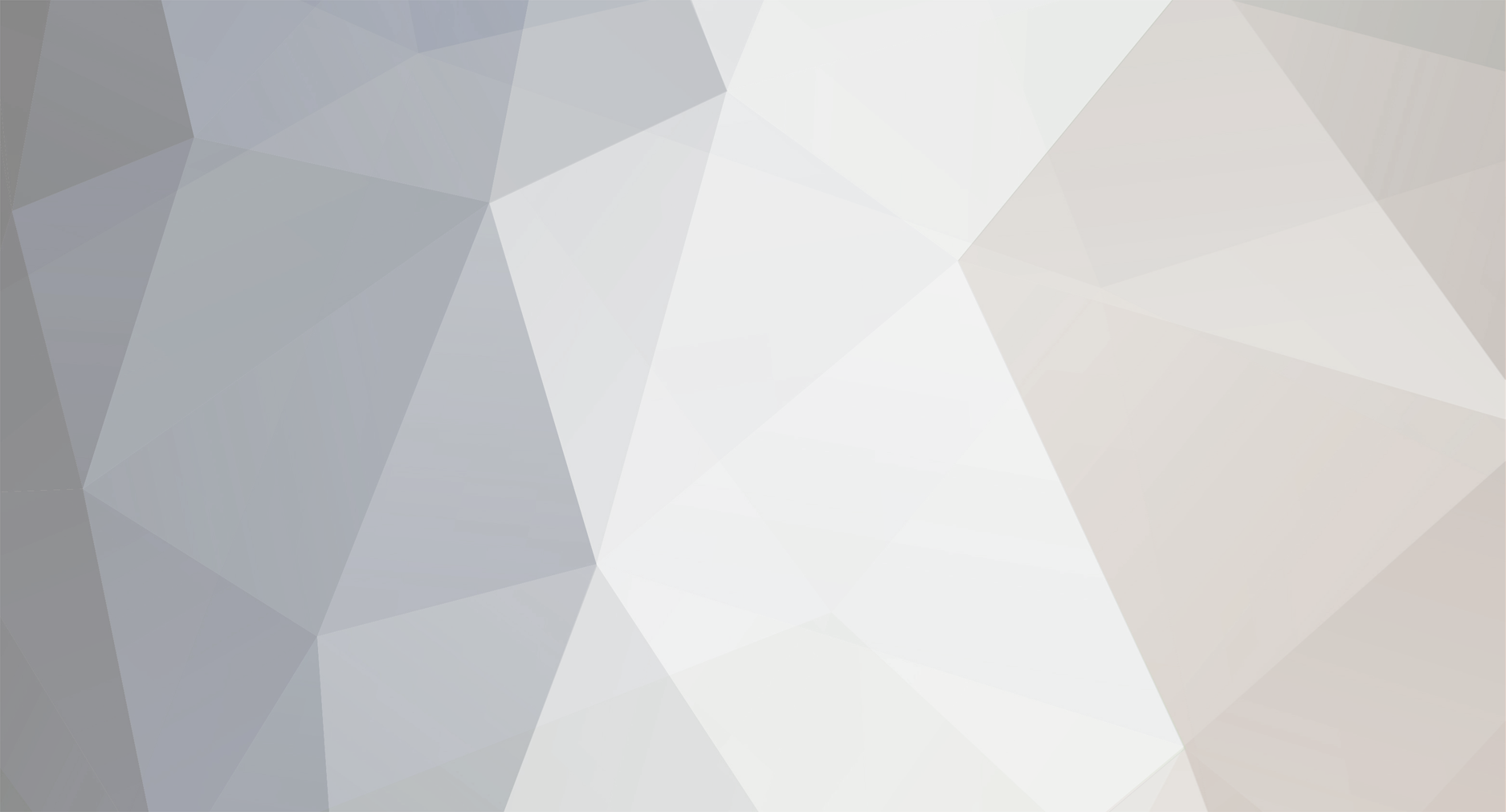
danny232
Members-
Posts
12 -
Joined
-
Last visited
danny232's Achievements

Newbie (1/5)
0
Reputation
-
Thanks for the advice. Here is the code: <?php // Make a MySQL Connection $query = "SELECT * FROM jobs ORDER BY id DESC"; $result = mysql_query($query) or die(mysql_error()); while($row = mysql_fetch_array($result)){ echo "<strong>"; echo $row['title']. " <br /> </strong>". $row['body'] . " <br /><br />Salary: ". $row['wage'] . " <br />Closing Date: ". $row['expiry'] . " <br /><br />To apply for this position, please email your CV to ". $row['apply']; echo "<br /><br />"; } ?> <?php $charLimit = 25; $myText = "$body"; $teaserText = substr($myText,0,$charLimit) . "... "; echo $teaserText; ?> I understand this won't work the way I want it to due to the ordering but i've tried altering the code around and I get several errors. Basically I want it to read from the table "jobs" and input the row from the column "body" but only display 30 words then have "..." at the end.
-
I've got a script running where I submit data into a form and that posts it into the database then posts the information onto a php page. I'm wanting the results returned to cutoff after 20 characters with "...." after it to show there is more (there will be then a 'click here to see more') I've tried searching for this numerous times but the results seem to direct to here: http://php.net/manual/en/function.substr.php I've read through that but that seems to discount everything and displays an error message saying there's too much to display. Can anyone help please?
-
I've managed to get a new php page to retrieve data from the database but i'm struggling to repeat the same command so it displays all the tables. Can anyone help please? <?php include 'admin/config.php'; ?> <?php Echo "<strong>"; ?> <?php // Make a MySQL Connection $query = "SELECT * FROM jobs ORDER BY id ASC"; $result = mysql_query($query) or die(mysql_error()); $row = mysql_fetch_array($result) or die(mysql_error()); echo $row['title']. " <br /> </strong>". $row['body'] . " <br /><br />Salary: ". $row['wage'] . " <br />Closing Date: ". $row['expiry'] . " <br /><br />To apply for this position, please email your CV to ". $row['apply']; ?>
-
Thanks for all your help, it finally works! In regards to security, the script will be in an admin area which will be in a protected directory, is it still worth creating a php function to block sql injections?
-
<?php include 'config.php'; ?> <? $title = $_POST['title']; $body = $_POST['body']; $wage = $_POST['wage']; $expiry = $_POST['expiry']; $apply = $_POST['apply']; if (!$title || !$body || !$wage || !$expiry || !$apply) { echo "<center><br /><br /><br /><br /><br />Missing Fields.<br />" ."<a href='javascript: history.go(-1)'>Go Back</a></center>"; exit; } mysqli_select_db("db477825879"); $query = "INSERT INTO jobs (title, body, wages, expiry, apply) VALUES ('title','body','wages','expiry','apply')" $result = mysql_query($query) or die(mysql_error()); if ($result) echo mysql_affected_rows()." Job entered into database."; ?> I'm now getting a new error on line 21 about the syntax: Parse error: syntax error, unexpected '$query' (T_VARIABLE) in /homepages/23/d477349413/htdocs/beta/admin/insert.php on line 21 I'm hoping to get the script running first then do all the security mesaures afterwards. Thanks for your help
-
I've changed the column name now to "body". <?php include 'config.php'; ?> <? $title = $_POST['title']; $body = $_POST['body']; $wage = $_POST['wage']; $expiry = $_POST['expiry']; $apply = $_POST['apply']; if (!$title || !$body || !$wage || !$expiry || !$apply) { echo "<center><br /><br /><br /><br /><br />Missing Fields.<br />" ."<a href='javascript: history.go(-1)'>Go Back</a></center>"; exit; } mysql_select_db("db477825879"); $query = "INSERT INTO jobs (title, body, wage, expiry, apply) VALUES ('$_POST[title]','$_POST[body]','$_POST[wage]','$_POST[expiry]','$_POST[apply]'"; $result = mysql_query($query) or die(mysql_error()); if ($result) echo mysql_affected_rows()." Job entered into database."; ?> index.html <center> <form action="insert.php" method="post"> Title<br /> <input type="text" name="title" maxlength="30" size="30"><br /><br /> Description<br /> <textarea name="body" maxlength="30" size="30" rows="8" cols="30"></textarea><br /><br /> Wage<br /> £<input type="text" name="wage" maxlength="8" size="5"><br /><br /> Expiry Date<br /> <input type="text" name="expiry" maxlength="15" size="15"><br /><br /> Apply to:<br /> <input type="text" name="apply" maxlength="30" size="30"><br /><br /> <input type="submit" value="Submit Job"> </form> and here's a screenshot of the database in phpmyadmin
-
It's now recognising the database and the form fields, but now coming back with: Highlighted in bold is what was entered into the form fields. <?php include 'config.php'; ?> <? $title = $_POST['title']; $desc = $_POST['desc']; $wage = $_POST['wage']; $expiry = $_POST['expiry']; $apply = $_POST['apply']; if (!$title || !$desc || !$wage || !$expiry || !$apply) { echo "<center><br /><br /><br /><br /><br />Missing Fields.<br />" ."<a href='javascript: history.go(-1)'>Go Back</a></center>"; exit; } mysql_select_db("jobs"); $query = "INSERT INTO Persons (title, desc, wage, expiry, apply) VALUES ('$_POST[title]','$_POST[desc]','$_POST[wage]','$_POST[expiry]','$_POST[apply]'"; $result = mysql_query($query) or die(mysql_error()); if ($result) echo mysql_affected_rows()." Job entered into database."; ?>
-
I now get "No database selected". I've run the config.php and the statement comes back as "Connected to MYSQL". Would the error mean it's not connecting to the actual database or the table within the database? <?php include 'config.php'; ?> <? $title = $_POST['title']; $desc = $_POST['desc']; $wage = $_POST['wage']; $expiry = $_POST['expiry']; $apply = $_POST['apply']; if (!$title || !$desc || !$wage || !$expiry || !$apply) { echo "<center><br /><br /><br /><br /><br />Missing Fields.<br />" ."<a href='javascript: history.go(-1)'>Go Back</a></center>"; exit; } mysql_select_db("jobs"); $query = "INSERT INTO jobs (title,wage,desc,expiry,apply)"; $result = mysql_query($query) or die(mysql_error()); if ($result) echo mysql_affected_rows()." Job entered into database."; ?> P.s i'm new to PHP if you couldn't tell...
-
<?php include 'config.php'; ?> <? $title = $_POST['title']; $desc = $_POST['desc']; $wage = $_POST['wage']; $expiry = $_POST['expiry']; $apply = $_POST['apply']; if (!$title || !$desc || !$wage || !$expiry || !$apply) { echo "Missing Fields.<br />" ."Go back."; exit; } mysql_select_db("jobs"); $_POST = "INSERT into Jobs values ('".$title."', '".$desc."', '".$wage."', '".$expiry."', '".$apply."')"; $result = mysql_query($query); if ($result) echo mysql_affected_rows()." Job entered into database."; ?> i've changed it to this now, and it doesn't seem to give the 'Missing fields' error. However it's still not posting to the database.
-
Thanks for your replies. insert.php <?php include 'config.php'; ?> <? if (!$title || !$desc || !$wage || !$expiry || !$apply) { echo "Missing Fields.<br />" ."Go back."; exit; } $title = $_POST['title']; $desc = $_POST['desc']; $wage = $_POST['wage']; $expiry = $_POST['expiry']; $apply = $_POST['apply']; mysql_select_db("Jobs"); $_POST = "INSERT into Jobs values ('".$title."', '".$desc."', '".$wage."', '".$expiry."', '".$apply."')"; $result = mysql_query($query); if ($result) echo mysql_affected_rows()." Job entered into database."; ?> index.html <center> <form action="insert.php" method="post"> Title<br /> <input type="text" name="title" maxlength="30" size="30"><br /><br /> Description<br /> <textarea name="title" maxlength="30" size="30" rows="8" cols="30"></textarea><br /><br /> Wage<br /> £<input type="text" name="wage" maxlength="8" size="5"><br /><br /> Expiry Date<br /> <input type="text" name="expiry" maxlength="15" size="15"><br /><br /> Apply to:<br /> <input type="text" name="apply" maxlength="30" size="30"><br /><br /> <input type="submit" value="Submit Job"> </form>
-
<center> <form action="insert.php" method="post"> Title<br /> <input type=text name=title maxlength=30 size=30><br /><br /> Description<br /> <textarea name=title maxlength=30 size=30 rows="8" cols="30"></textarea><br /><br /> Wage<br /> £<input type=text name=wage maxlength=8 size=5><br /><br /> Expiry Date<br /> <input type=expiry name= maxlength=15 size=15><br /><br /> Apply to:<br /> <input type=text name=apply maxlength=30 size=30><br /><br /> <input type="submit" value="Submit Job"> </form> The insert.php <?php include 'config.php'; ?> <? if (!$title || !$desc || !$wage || !$expiry || !$apply) { echo "Missing Fields.<br />" ."Go back."; exit; } $title = addslashes($title); $desc = addslashes($desc); $wage = addslashes($wage); $expiry = addslashes($expiry); $apply = addslashes($apply); mysql_select_db("jobs"); $query = "insert into jobs values ('".$title."', '".$desc."', '".$wage."', '".$expiry."', '".$apply."')"; $result = mysql_query($query); if ($result) echo mysql_affected_rows()." Job entered into database."; ?> When I submit the form I just get the error message "Missing fields, go back"
-
I'm new to php so i'm not the best with the terms at the minute! I'm trying to create a website where I can display our job vacancies on our main website and edit/add job vacancies in an admin panel in the back end. I've got the database setup correctly and i've got the php page to connect to the database, i've created a php form to submit to the mysql database but i've had no luck (it keeps saying posting error). Can anyone show me an example of how to do this please? Thanks Danny