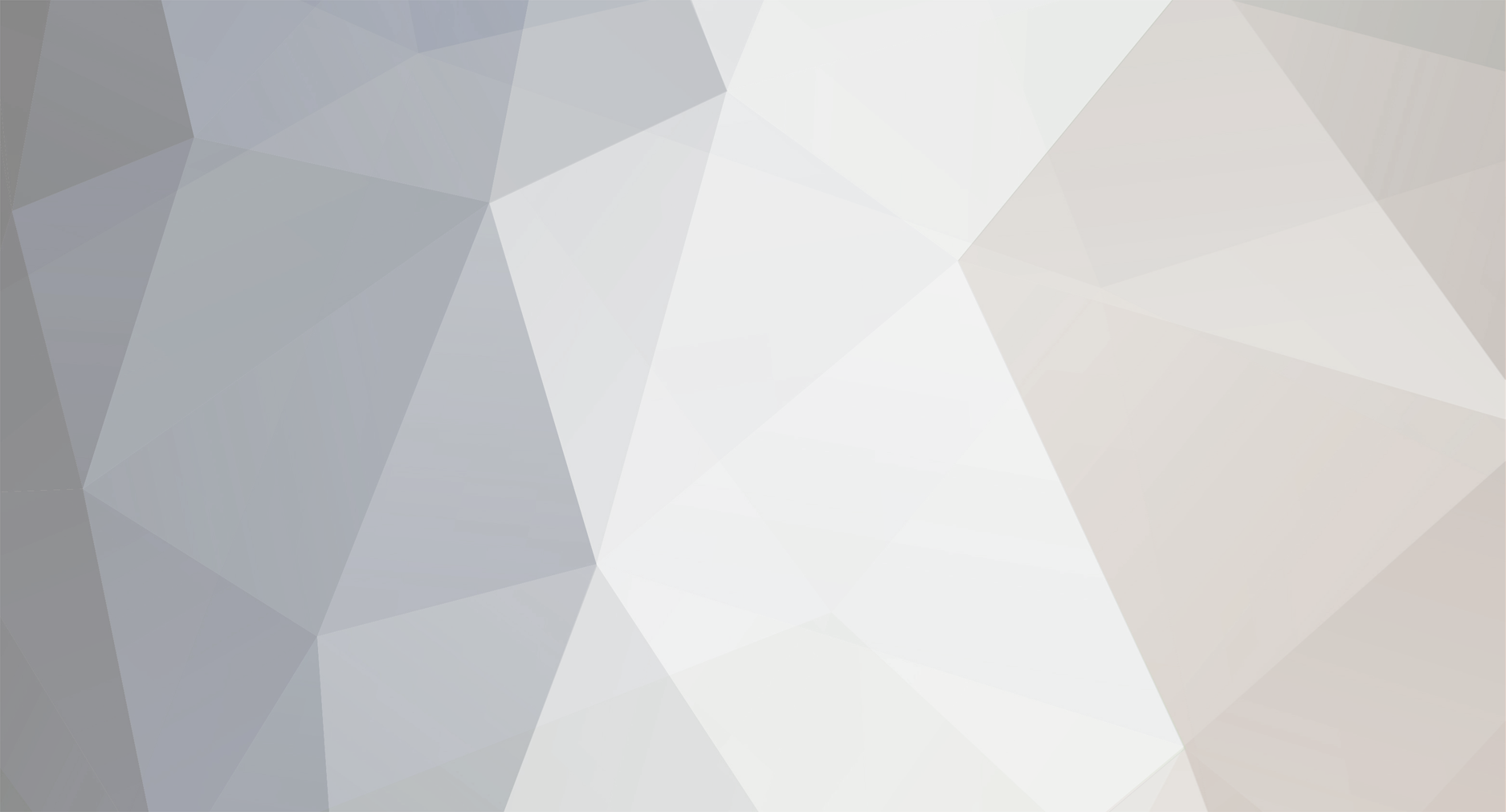
lhemingway
-
Posts
4 -
Joined
-
Last visited
We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.