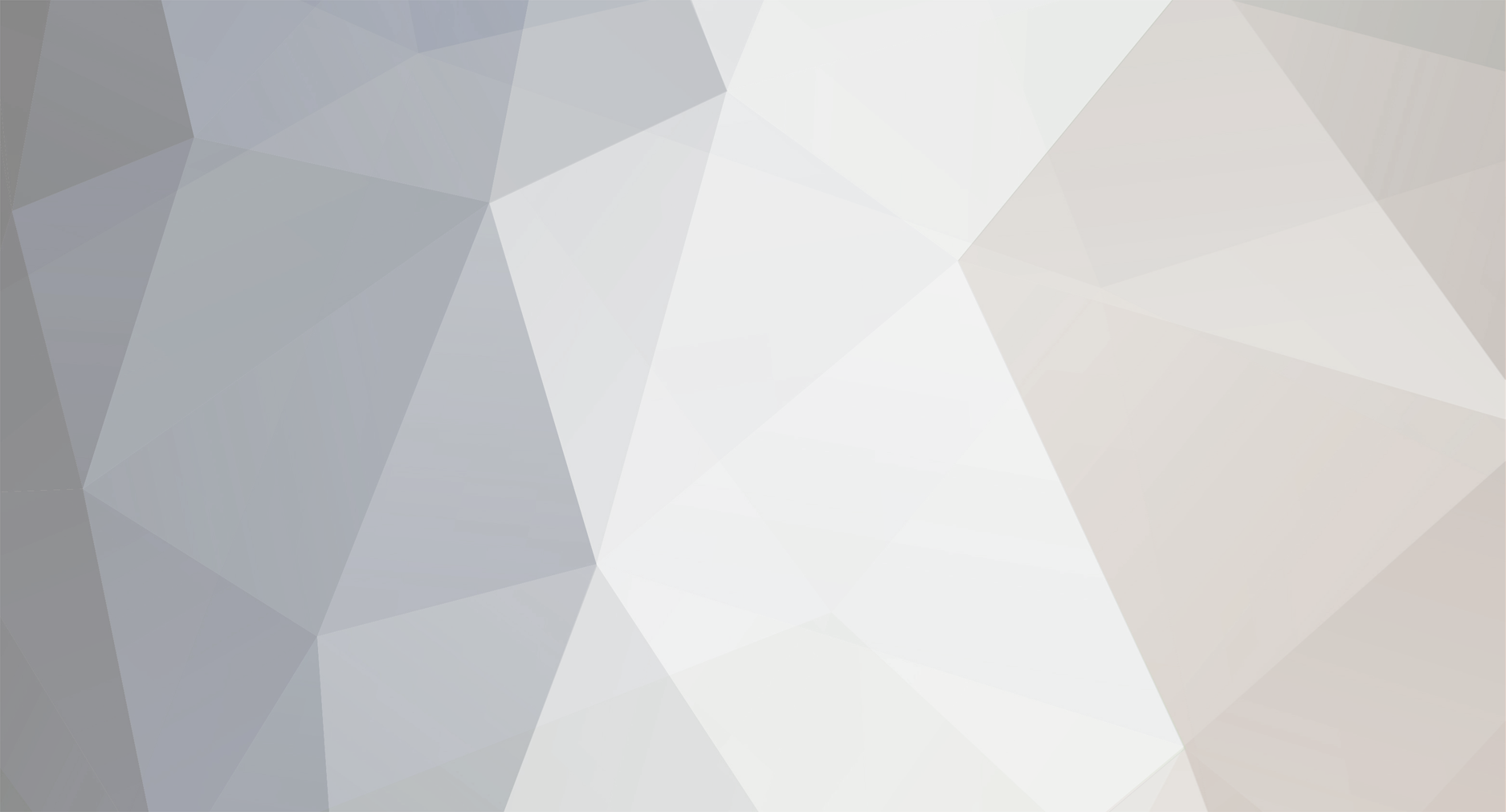
tazmith
Members-
Posts
14 -
Joined
-
Last visited
-
Days Won
1
tazmith last won the day on June 17 2014
tazmith had the most liked content!
tazmith's Achievements

Newbie (1/5)
4
Reputation
-
Exactly as "Jacques1" said. You have a .php file with unparsed HTML. Look at the beginning of your script. Perfect example of what "Jacques1" is explaining. Basically, you can't have any HTML output before the header() function is declared.. just as a test throw in ob_start(); after your include_once("init.php");
-
oh woops, I forgot you mentioned websockets too.
-
Thanks for the reply phpzer. I think what's happening is there's constantly a request being opened, the move function and create function both send a request at the same time. AJAX seems to be the best option here. I used to run it just as php but letting the actual page load is a hell of a lot slower than using AJAX. Someone was telling me about websockets, but i'm not familiar with it.
-
you can run everything through 1 update if you wanted. You don't need to use if (empty($_POST['first']) === false) { } instead you could just update everything on if (isset($_POST['submit'])) { //run query that updates all $_POST data here. }
-
I've got these 2 functions, and I'm wondering if anyone could give me some advice on it, or help me with a better option. Basically i'm running into a problem with the page it glitches out a lot.. The functions are for an RPG game world (map) Basically a user can move north, south, east and west, however; after about 3 moves, the script glitches out, and freezes for a minute and than either you need to refresh the page, or just wait it out until it allows you to move again. The movements are based off the "WASD" keys, thats how you navigate north, south, east and west. If anyone could help me with this, that'd be awesome, thanks. //function that moves the user on the map. function move(direction) { var ajaxRequest; try { //Opera 8.0+, Firefox, Safari ajaxRequest = new XMLHttpRequest(); } catch (e) { //Internet Explorer Browsers try { ajaxRequest = new ActiveXObject("Msxml2.XMLHTTP"); } catch (e) { try { ajaxRequest = new ActiveXObject("Microsoft.XMLHTTP"); } catch (e) { alert("Your browser broke!"); return false; } } } //function that will recieve data sent to the server. ajaxRequest.onreadystatechange = function() { if (ajaxRequest.readyState == 4) { } } ajaxRequest.open("GET", "move.php?direction=" + direction, true); ajaxRequest.send(null); setTimeout("clearatk()", 10); setTimeout("create()", 30); setTimeout("getmob()", 40); setTimeout("getname()", 50); } //function that creates the actual map. function create() { var ajaxRequest; try { //Opera 8.0+, Firefox, Safari ajaxRequest = new XMLHttpRequest(); } catch (e) { //Internet Explorer Browsers try { ajaxRequest = new ActiveXObject("Msxml2.XMLHTTP"); } catch (e) { try { ajaxRequest = new ActiveXObject("Microsoft.XMLHTTP"); } catch (e) { alert("Your browser broke!"); return false; } } } //function that will recieve data sent to the server. ajaxRequest.onreadystatechange = function() { if (ajaxRequest.readyState == 4) { document.getElementById("create").innerHTML = ajaxRequest.responseText; } } ajaxRequest.open("GET", "create.php?ajax=1", true); ajaxRequest.send(null); }
-
No problem, glad I could help.
-
Hope this helps you. It's basic, this can be done in many ways, but here's a re-vamped version of what I did in my 1st post. Note: I wen't with the PDO version, because mysql_ is just bad practice in my opinion, but that's only my opinion <?php $host = 'host'; $dbname = 'db_name'; $user = 'db_user'; $pass = 'db_pass'; try { $dbh = new PDO("mysql:host=$host;dbname=$dbname", $user, $pass); } catch(PDOException $e) { echo $e->getMessage(); } if (!isset($_POST['selectid']) && !isset($_POST['submit'])) { echo '<form method="POST">Select ID to edit: <select name="id">'; $query = $dbh->query('SELECT id FROM contacts ORDER BY id ASC'); foreach ($query->fetchAll(PDO::FETCH_ASSOC) as $row) { echo '<option value="'.$row['id'].'">'.$row['id'].'</option>'; } echo '</select>'; echo '<input type="submit" name="selectid" id="selectid" value="Submit" /> </form>'; } //selecting the id. if (isset($_POST['selectid'])) { $query = $dbh->prepare('SELECT first,last,id FROM contacts WHERE ' . 'id=:id '); $query->bindParam(':id', $_POST['id'], PDO::PARAM_INT); $query->execute(); $row = $query->fetch(PDO::FETCH_ASSOC); $count = $query->rowCount(); //incase of POST data manipulation if ($count < 1) { die('Error: User does not exist!'); } echo 'Viewing ID: '.$row['id'].' Firstname: '.$row['first'].''; //the form. echo '<br /><br /> <form method="POST"><div> First Name: <input type="text" name="first" value="'.$row['first'].'" /><br /> Last Name: <input type="text" name="last" value="'.$row['last'].'" /><br /> <input type="hidden" name="id" value="'.$row['id'].'" /> <input type="submit" name="submit" id="submit" value="Submit" />'; echo '<br /><br /><a href="pagehere.php">go back</a>'; } //submitting. if (isset($_POST['submit'])) { if (empty($_POST['first']) === false) { //update firstname, since it was updated. $firstname_update = $dbh->prepare('UPDATE contacts SET ' . 'first=:first WHERE ' . 'id=:id '); $firstname_update->bindParam(':firstname', $_POST['first']); $firstname_update->bindParam(':id', $_POST['id'], PDO::PARAM_INT); $firstname_update->execute(); //echo if wanted. echo 'Updating Firstname of ID: '.htmlspecialchars($_POST['id'],ENT_QUOTES).' to '.htmlspecialchars($_POST['first'],ENT_QUOTES).''; //just continue onward for lastname, phone, whatever you wan't really. echo '<br /><br /><a href="pagehere.php">go back</a>'; } } ?>
-
if you wanted to you could have a drop down with the ID'S, and submit leading to a page like update.php?updateid=userid which will than show the input fields for first, last, ect. And than on that submit, create the POST submit.
-
not updating database and prepared statements
tazmith replied to jacko_162's topic in PHP Coding Help
http://php.net/pdo.prepared-statements would be a good start to check out PDO and prepared statements. but heres an example based off the script you've posted. <?php /* PDO example for a database connection */ $host = 'host'; $dbname = 'db_name'; $user = 'db_user'; $pass = 'db_pass'; try { $dbh = new PDO("mysql:host=$host;dbname=$dbname", $user, $pass); } catch(PDOException $e) { echo $e->getMessage(); } /* PDO example of select using 'bindParam' and fetchAll*/ $id = $_SESSION['SESS_MEMBER_ID']; $stmt = $dbh->prepare('SELECT settings,member_id FROM members WHERE ' . 'member_id=:member_id '); $stmt->bindParam(':member_id', $id, PDO::PARAM_INT); $stmt->execute(); foreach ($stmt->fetchAll(PDO::FETCH_ASSOC) as $row) { $arr = array(); $arr = explode(';',$row['settings']); $member_id = $row['member_id']; } /* PDO example of insert using 'bindParam' method */ $member_id = $_SESSION['SESS_MEMBER_ID']; $date = date("y-m-d"); $time = date("H:i:s", time() - 3600); $stmt = $dbh->prepare("INSERT INTO tests (member_id, test1, time, date, month, day, active1) VALUES (:member_id, :test1, :time, :date, :month, :day, :active1)"); $stmt->bindParam(':member_id', $_SESSION['SESS_MEMBER_ID'], PDO::PARAM_INT); $stmt->bindParam(':test1', $_POST['test1'], PDO::PARAM_STR); //by default it will be param_str. $stmt->bindParam(':time', $time); $stmt->bindParam(':date', $date); $stmt->bindParam(':month', $_POST['month']); $stmt->bindParam(':day', $_POST['date']); $stmt->bindParam(':active1', $_POST['active1']); $stmt->execute(); If your going to stick with mysql_ when your using $_POST, get into the habit of storing it using mysql_real_escape_string if you're worried about 'sql injection.' Otherwise your completely vulnerable. example: $test1 = $_POST['test1']; //becomes $test1 = mysql_real_escape_string($_POST['test1']); hope that helps, good luck. -
<?php /* * PDO version. */ $host = 'host'; $dbname = 'db_name'; $user = 'db_user'; $pass = 'db_pass'; try { $dbh = new PDO("mysql:host=$host;dbname=$dbname", $user, $pass); } catch(PDOException $e) { echo $e->getMessage(); } echo '<form method="POST">Select id to edit: <select name="id">'; $query = $dbh->query('SELECT id FROM contacts ORDER BY id ASC'); foreach ($query->fetchAll(PDO::FETCH_ASSOC) as $row) { echo '<option value="'.$row['id'].'">'.$row['id'].'</option>'; } echo '</select>'; echo '<br /><div> First Name: <input name="first" type="text" /><br />'; echo '<input type="submit" name="submit" id="submit" value="Submit" /> </form>'; if (isset($_POST['submit'])) { echo '<br />Editing ID: '.htmlspecialchars($_POST['id'],ENT_QUOTES). ''; if (empty($_POST['first']) === false) { //firstname has been edited, lets update. $update_query = $dbh->prepare('UPDATE contacts SET ' . 'first=:first WHERE ' . 'id=:id '); $update_query->bindParam(':first', $_POST['first']); $update_query->bindParam(':id', $_POST['id'], PDO::PARAM_INT); $update_query->execute(); echo '<br />Updated firstname to '.htmlspecialchars($_POST['first'],ENT_QUOTES).''; } } /* /* * mysql_ version. */ echo '<form method="POST">Select id to edit: <select name="id" id="ids">'; $query = "SELECT id FROM contacts ORDER BY id ASC"; $result = mysql_query($query) or die ( mysql_error ()); while ($row = mysql_fetch_array($result,MYSQL_ASSOC)) { echo '<option value="'.$row['id'].'">'.$row['id'].'</option>'; } echo '</select>'; echo '<br /><div> First Name: <input name="first" type="text" /><br />'; echo '<input type="submit" name="submit" id="submit" value="Submit" /> </form>'; if (isset($_POST['submit'])) { echo '<br /><br />Editing id: ' .htmlspecialchars($_POST['id'],ENT_QUOTES). ''; if (empty($_POST['first']) === false) { $id = (int)$_POST['id']; $firstname = mysql_real_escape_string($_POST['first']); $update_query = "UPDATE contacts SET first = '$firstname' WHERE id = '$id'"); $update_result = mysql_query($update_result) or die ( mysql_error ()); echo '<br />Updated firstname to '.htmlspecialchars($_POST['first'],ENT_QUOTES).''; } } ?>