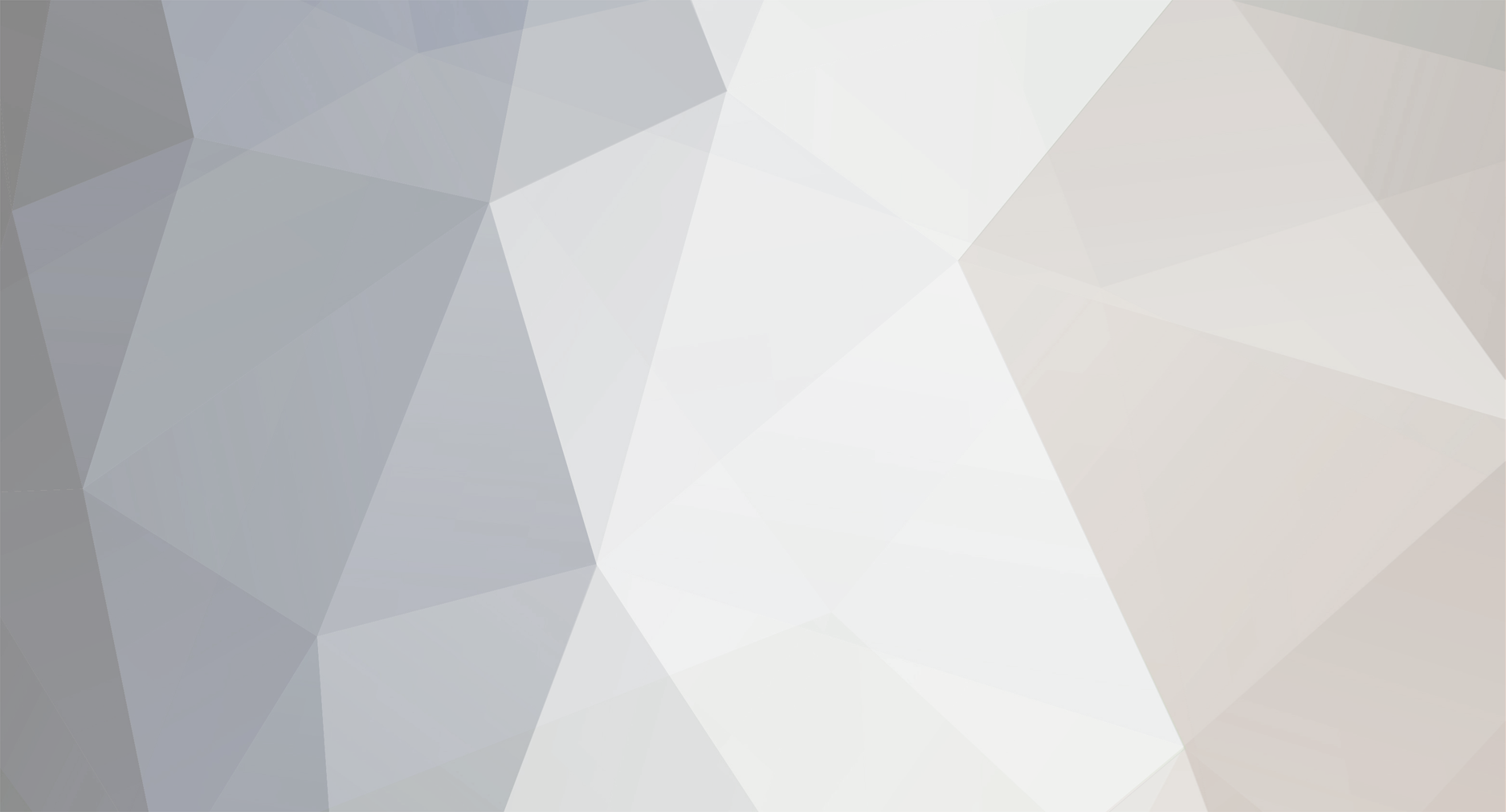
jameshay
-
Posts
7 -
Joined
-
Last visited
jameshay's Achievements

Newbie (1/5)
1
Reputation
jameshay has no recent activity to show
1
We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.