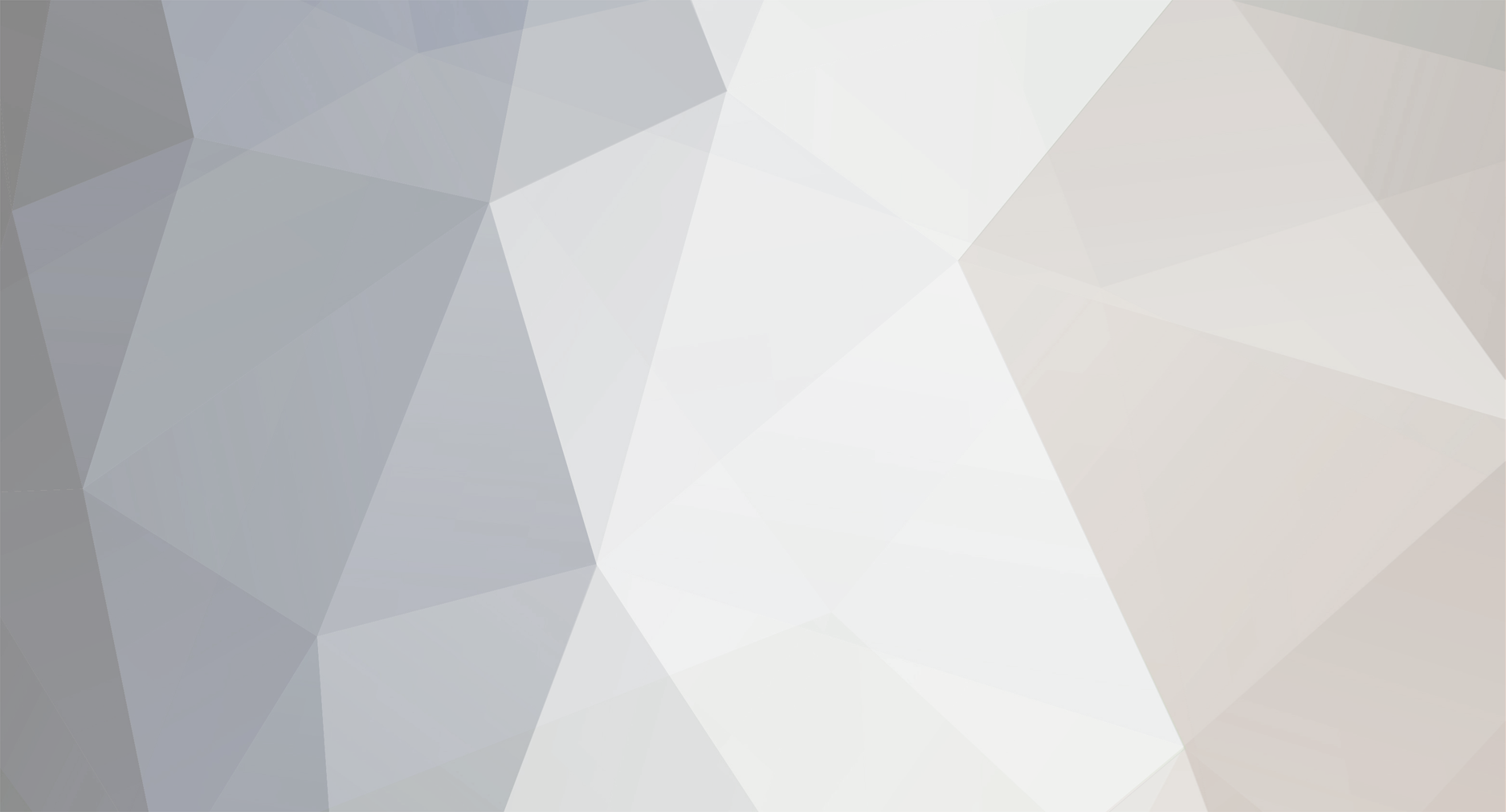
excelmaster
Members-
Posts
51 -
Joined
-
Last visited
Everything posted by excelmaster
-
Help required to fully and properly parameterize a SELECT query
excelmaster replied to excelmaster's topic in PHP Coding Help
@Jacques1 and @mac_gyver: Thank you to the both of you for providing me with much a more elegant and compact code solution (which BTW works just great as-is). I certainly didn't know that I could use the ternary operator in a SQL statement...and I certainly didn't think that I could/should setup permitted values/column-names in an array variable and use that for the query. I have learnt something new from the both of you. Now, while on this subject I would also like to sanitize another input, in order to block the possiblility of SQL-injection, and I would greatly appreciate help with this one as well. So, for/against all of my displayed rows, I display a checkbox to the extreme left of the row..which the user is expected to check for each row that they want updated. Upon clicking the [submit] button, the IDnumber(s) for each of the selected row(s) is passed/POSTed as an array. I would like to be able to sanitize that IDnumber array (to ensure that nothing bad has been included instead of IDnumbers) before it's sent to the query. Here's the relevant bits-and-pieces of my code brought together: // loop through the results foreach($result as $row) { print '<tr>'; print '<td><span class="filler-checkbox"><input type="checkbox" name="IDnumber[]" value="' . $row["idnumber"] . '" /></span></td>'; .... .... print '</tr>'; } ... ... ... print '<div class="full-row-buttons">'; print '<input class="update-button-purchased" name="toggle-purchased" value="Flag selection as *Purchased/Not Purchased*" type="submit"/>'; print '<input class="update-button-later" name="toggle-purchase-later" value="Flag selection as *Buy Later/Buy Now*" type="submit"/>'; print '</div>'; if(isset($functionSelected)){ //Force all passed IDs to be integers and filter out 0/empty values $IDnumbersAry = array_filter(array_map('intval', $_POST['IDnumber'])); //Format list into a comma separated string $IDnumberList = implode(', ', $IDnumbersAry); switch($functionSelected){ case "update-delete": //Detemine the process to run switch($buttonClicked) { case "Flag selection as *Purchased/Not Purchased*": //Toggle purchased flag for selected records where the //purchase_later_flag is NOT 'Y' if(!empty($IDnumberList)) { $query = "UPDATE shoplist SET purchased_flag = IF(purchased_flag='Y', 'N', 'Y') WHERE idnumber IN ($IDnumberList) AND purchase_later_flag <> 'Y'"; $statement = $conn->exec($query); } break; .... .... .... } ... ... ... ... ... ... } } Here's the screenshot: -
Hello All, I'm having trouble trying to "wrap my head around" how I can make the query in my code below more efficient, elegant, and certainly immune to a SQL-injection attack So, I have a form (which can be seen in the attached screenshot) which has the user first select a search-type via a dropdown listbox. The two choices pertain to two columns in the underlying table i.e. store_name and item_description. Once that selection is completed the user is expected to enter a search-term (which is typically in relation to the choice selected in the first prompt). Now, what I would like to happen is that the query be created based on both, the value in the search-type prompt, as well as the value in search-term textbox, and of course, the query must be a parameterized prepared statement (to minimize the possiblity of a SQL-inection attach). Essentially I would like to get rid of the following code (which I believe is potentially redundant, and contains a hard-coded column name between the WHERE and LIKE), to make things less complicated and more elegant/flexible if ($searchtype == "store_name") { $sql = "SELECT * FROM `shoplist` WHERE store_name LIKE :searchterm ORDER BY store_name ASC"; } else if ($searchtype == "item_description") { $sql = "SELECT * FROM `shoplist` WHERE item_description LIKE :searchterm ORDER BY store_name ASC"; } Here's what I tried: // Tried this first but it didn't work...gave some kind of error pertaining to the parameter 2 // Prepare the query ONCE $query = "SELECT * FROM `shoplist` WHERE ? LIKE ? ORDER BY ? ASC"; $searchterm = '%' . $searchterm . '%'; $statement = $conn->prepare($query); $statement->bindValue(1, $searchtype); $statement->bindParam(2, $searchterm); $statement->bindValue(3, $searchtype); $statement->execute(); // ----------------------------------------------------------------------------------------------------------- // // Tried this as well, but was getting unexpected results (mostly all rows being returned, regardless of input) // Prepare the query ONCE $query = "SELECT * FROM `shoplist` WHERE :searchtype LIKE :searchterm ORDER BY :searchtype ASC"; $searchterm = '%' . $searchterm . '%'; $statement = $conn->prepare($query); $statement->bindValue(':searchtype', $searchtype); $statement->bindValue(':searchterm', '%' . $searchterm . '%'); $statement->execute(); Here's the complete code (pertaining to the problem/request): // create short variable names if(isset($_POST['searchtype'])) { $searchtype=$_POST['searchtype']; } if(isset($_POST['searchterm'])) { $searchterm=trim($_POST['searchterm']); } if(isset($_POST['searchtype']) && isset($_POST['searchterm'])) { if (!get_magic_quotes_gpc()) { $searchtype = addslashes($searchtype); $searchterm = addslashes($searchterm); } } if(isset($_POST['searchtype']) && isset($_POST['searchterm'])) { // ****** Setup customized query ****** if ($searchtype == "store_name") { // The following statement is potentially open to SQL-inection attack and has therefore been REM(arked) // $sql = "SELECT * FROM `shoplist` WHERE " . $searchtype . " LIKE :searchterm ORDER BY store_name ASC"; // The following may not be open to SQL-injection attack, but has the column name hard-coded in-between the WHERE and LIKE clauses // I would like the the column name to be set based on the value chosen by the user in the form dropdown listbox for "searchtype" $sql = "SELECT * FROM `shoplist` WHERE store_name LIKE :searchterm ORDER BY store_name ASC"; } else if ($searchtype == "item_description") { // The following statement is potentially open to SQL-inection attack and has therefore been REM(arked) // $sql = "SELECT * FROM `shoplist` WHERE " . $searchtype . " LIKE :searchterm ORDER BY item_description ASC"; // The following may not be open to SQL-injection attack, but has the column name hard-coded in-between the WHERE and LIKE clauses // I would like the the column name to be set based on the value chosen by the user in the form dropdown listbox for "searchtype" $sql = "SELECT * FROM `shoplist` WHERE item_description LIKE :searchterm ORDER BY item_description ASC"; } $statement = $conn->prepare($sql); $statement->bindValue(':searchterm', '%' . $searchterm . '%'); $statement->execute(); ... ... ... } Thanks guys!
-
Why would no rows be returned for a SELECT DISTINCT query?
excelmaster replied to excelmaster's topic in PHP Coding Help
@ginerjm and @Jacques1: I'm new to PHP coding...learning as I go (thanks to you guys)....which is why I had no idea how (and what) to do in order to setup error checking. Thanks for the link @Jacques1...I will take a look at it for future use. @davidannis: Thanks for putting together that code! No worries that it's not tested...I will test and fix if required (to the best of my newbie abilities). Much appreciated Cheers guys! -
Why would no rows be returned for a SELECT DISTINCT query?
excelmaster replied to excelmaster's topic in PHP Coding Help
@ginerjm: >> At this point - you query is so simple that there must be something wrong like an invalid column name or table name. That's exactly what I said to myself last night - "This query is so simple, and not only is it simple, but it's also one that is working for another table"....that I figured there was something crazy going on. Guess what? The problem had nothing to do with any of the code I had in my file! I guess we were all "barking up the wrong tree", 'eh? Anyways, here's how I stumbled across the problem, quite accidentally, if you ask me: Late last night I casually went in to the SQL interactive environment (signing-on as a regular user...rather than as root) to check if my table names were correct. So I issued the command "SHOW TABLES;" and lo and behold, the two lookup tables weren't even listed (even though the main table [shoplist] was indeed listed). It then dawned on me that I had inadvertently forgotten to "GRANT ALL...." to regular users for the two new lookup tables (when I had created the tables as root). Once I executed the "GRANT ALL..." command everything worked hunky-dory. So, lesson for self, and note to moderators/guru's here: should a similar post crop-up in future (where a person can see results from one table, but not from others), first thing to ask is if access rights were granted for the "problem" tables to regular users. @davidannis: What's the best way for you to provide me the code for "adding a value not in the drop down" - as and when you have the time to find/code it - now that I've marked this thread "Solved"? -
Why would no rows be returned for a SELECT DISTINCT query?
excelmaster replied to excelmaster's topic in PHP Coding Help
@davidannis: >> You need something between option and /option or nothing will show in your list except blank lines. That certainly was a very good catch, but alas (inspite of changing it to the corrected statement that you provided) it still isn't doing anything to help show the values from the lookup table. Thanks for providing me the code to turn on error reporting. I seem to have a slew of errors being reported, but they don't seem to be related to the current problem though....I'm still investigating (and trying to eradicate the errors). >> I will come back when I have more time and try to post an example of adding a value not in the drop down. Much thanks in advance for this offer. It will certainly help provide a much improved user-experience. With my newbie knowledge and experience I would never be able to achieve this in a million years. @ginerjm: >> On what are you basing your conclusion that there are no results? The bare echo of the count? It's not based just on the bare echo of the count (which of course indicates 0), but also due to the fact that there are no (looked-up) values being shown in the dropdown listbox. -
Why would no rows be returned for a SELECT DISTINCT query?
excelmaster replied to excelmaster's topic in PHP Coding Help
@ginerjm: I guess I do not have error-checkign turned on....and that's what I wanted to know about as well i.e. how to turn on error-checking, and what must be turn to check for errors? To answer your second question, yes I do have other preceding code (a mixture of PHP and HTML), most of which is/was working just fine...including other queries....and if you're asking specifically about the code that creates $conn, then that code is in a separate file (which is being included), and that too works just fine (because other queries are using it, and they seem to be returning the correct results). Anyways, if it helps, here's the code for $conn: <?php function dbConnect($type) { $dbtype = "mysql"; $dbhost = "localhost"; $dbname = "mydb"; $dbuser = "myuser"; $dbpass = "mypass"; // database connection try { $conn = new PDO("mysql:host=$dbhost;dbname=$dbname",$dbuser,$dbpass); return $conn; } catch (PDOException $e) { print "Error!: " . $e->getMessage() . "<br/>"; exit; } } ?> @Psycho: I do not really have to use "DISTINCT"...and I even tried removing it and using "SELECT * FROM store_master" but yet no joy. So yeah, how do I go about debugging the DB errors? >> As for your second request, that is quite a lot to ask in a forum post. Hmmm....I guess I'll just make-do with a plain Jane dropdown listbox and manually enter the products into the table (before-hand). -
Hello folks, In trying to improve the user experience for my first WebApp I have decided to create two new tables - one a master file to contain a list of all stores, and the second a master file to contain a list of all products that are normally purchased - and I would like to use the values from these two tables as lookup values in dropdown listboxes, to which the user can also add new entries. As it turns out, I'm stuck on the very first objective i.e. to lookup/pull-in the distinct values from the master tables. The problem I'm having is that the query seems to return no rows at all...in spite of the fact that there a records in the table, and the exact same query (when run within the MySQL environment) returns all the rows correctly. Is there something wrong with my code, or how can I debug to see whether or not the query is being executed? Objective # 2, which is to allow new values to be entered into the dropdown listbox, and then inserted into the respective table is certainly waaay beyond my beginner skills, and I'll most certainly need to some help with that as well..so if I can get some code/directions in that regard it will be most appreciated. Thank you. <?php $sql = "SELECT DISTINCT store_name FROM store_master ORDER BY store_name ASC"; $statement = $conn->prepare($sql); $statement->execute(); $count = $statement->rowCount(); echo $count; // fetch ALL results pertaining to the query $result = $statement->fetchAll(); echo "<select name=\"store_name\">"; echo '<option value="">Please select...</option>'; foreach($result as $row) { echo "<option value='" . $row['store_name'] . "'></option>"; } echo "</select>"; ?>
-
@Psycho: The code you provided was almost a "drop-in replacement" for the code I had in my app. I only had to make a a few minor changes (here and there) and it (my app) is now working like a dream....and I can safely say that you've helped me get to end-of-job on this project. One thing I will do thought (in the next few days) is to learn/read up more on PHP/MySQL security, SQL injection etc. and then re-visit this project to make it as water-tight as possible. Thank you once again
-
@Psycho: Thanks once again (a million times over) for providing what (at first glance) seems exactly "what the doctor ordered"! That is indeed some amazing code that you've provided me with. The only processes that use the checkboxes are the update and delete processes...and that's why I decided to merge them into one. That being said, I'm sure my code for the "search" function is also overly complicated and unnecessarily long. Should I include that code as part of this post to have it looked over and potentially changed? >> I don't know what logic you have to determine the value used for the switch()..... The answer to this question of yours is provided in the code block below: So the question I have is this: is that a safe way to do it...or is it still open to SQL injection? If it is indeed open to SQL injection then how do I fix/change it? if(isset($_POST["action"])){ switch($_POST["action"]){ case "insert": .... .... case "update-delete": .... .... case "search": .... .... } } @Ch0cu3r: I certainly wasn't aware that checkboxes could be used to as a mechanism to perform SQL injection. Likewise, I didn't think that a dropdown listbox (allowing only fixed/coded values) could be used as a means to perform SQL injection. I was under the impression that only free-format textboxes could be used for malicious purposes. >> And as I stated you are using a prepared queries incorrectly (read my comment at the bottom of post #4) I certainly did read your comment at the bottom of post #4, and that's what prompted me to ask the question ("Can you tell me which part(s) of my code is still open to SQL injection?")...because you did not state which part was still to prone to SQL injection, and as I said above, I wasn't aware that checkboxes could be use for SQL injection. So...how do I deal with checkboxes and dropdown listboxes....to make them SQL-injection-proof? Thanks once again to both of you for the amazing help and patience. Much appreciated! I'm learning so much, so soon...it's not even funny! Cheers
-
@Ch0cu3r: Actually, the two flags (and therefore the two buttons) are independent of each other (with each serving a different/specific purpose). The "already_purchased" flag is used during the time of shopping (on the weekend) right after an item is picked up (in the grocery store)...so we can easily see which items have been bought, and which are yet to be bought (red = bought, black = yet to be bought). Now, some items we may already have at home (so we don't need to purchase them on the weekend) but will need to buy a few days after the weekend, and therefore we'd like to see those items highlighted in a different color (blue in this case) So when we first head to the grocery store the list will only show rows in black and/or blue...and only the black items will be purchased that day (Saturday). As we go along, picking up the items on the list, we'll click the "red-text button" to indicate that that items has been purchased, and the row will be highlighted in red text. So typically once the shopping is complete, all those black rows will show up as red (unless an item was out of stock). The above being said, the code I provided was specific only to the red-text button i.e. "already_purchased" logic/functionality. I have an almost identical piece of code/logic that deals with the blue-text button ("to be purchased later" items), the only difference being the column-name. However, the logic for the red-text button must/does check to see if a selected row is highlighted in blue i.e. it's a "purchase_later" item then it must be skipped over (since we are clicking the red button, and only want to toggle between red and black or alread_purchased and not_purchased). So, I understand the first "$sql...." statement that you provided, and I kow how to fix it as per my requirement...but I'm not sure about the second "$sql..." statement! As @psycho correctly pointed out, a reverse logic must be developed for the very same button, in order to toggle the value when clicked a second time. Also, where exactly do I place this code? >> Currently your code is still open to SQL injection. Can you tell me which part(s) of my code is still open to SQL injection? @Psycho: thanks for provide such a detailed explanation. I'm gonna need some time to first digest all of that, then understand it, and finally put it to use. I will most certainly have more questions and/or clarifications...but it may come later today or possibly even tomorrow.
-
Forgot to mention the problem I'm having with my current code! So right now, the correct number of rows are being returned for the first query i.e. the one that extracts the selected/checked rows...but when I click the first/red-text button, nothing happens to any of the rows i.e. none of the flags/column values are being changed
-
Hello all, Based on the suggestion of you wonderful folks here, I went away for a few days (to learn about PDO and Prepared Statements) in order to replace the MySQLi commands in my code. That's gone pretty well thus far...with me having learnt and successfully replaced most of my "bad" code with elegant, SQL-Injection-proof code (or so I hope). The one-and-only problem I'm having (for now at least) is that I'm having trouble understanding how to execute an UPDATE query within the resultset of a SELECT query (using PDO and prepared statements, of course). Let me explain (my scenario), and since a picture speaks a thousand words I've also inlcuded a screenshot to show you guys my setup: In my table I have two columns (which are essentially flags i.e. Y/N), one for "items alreay purchased" and the other for "items to be purchased later". The first flag, if/when set ON (Y) will highlight row(s) in red...and the second flag will highlight row(s) in blue (when set ON). I initially had four buttons, two each for setting the flags/columns to "Y", and another two to reverse the columns/flags to "N". That was when I had my delete functionality as a separate operation on a separate tab/list item, and that was fine. Now that I've realized I can include both operations (update and delete) on just the one tab, I've also figured it would be better to pare down those four buttons (into just two), and set them up as a toggle feature i.e. if the value is currently "Y" then the button will set it to "N", and vice versa. So, looking at my attached picture, if a person selects (using the checkboxes) the first four rows and clicks the first button (labeled "Toggle selected items as Purchased/Not Purchased") then the following must happen: 1. The purchased_flag for rows # 2 and 4 must be switched OFF (set to N)...so they will no longer be highlighted in red. 2. The purchased_flag for row # 3 must be switched ON (set to Y)...so that row will now be highlighted in red. 3. Nothing must be done to rows # 1 and 5 since: a) row 5 was not selected/checked to begin with, and b) row # 1 has its purchase_later_flag set ON (to Y), so it must be skipped over. Looking at my code below, I'm guessing (and here's where I need the help) that there's something wrong in the code within the section that says "/*** loop through the results/collection of checked items ***/". I've probably made it more complex than it should be, and that's due to the fact that I have no idea what I'm doing (or rather, how I should be doing it), and this has driven me insane for the last 2 days...which prompted me to "throw in the towel" and seek the help of you very helpful and intellegent folks. BTW, I am a newbie at this, so if I could be provided the exact code, that would be most wonderful, and much highly appreciated. Thanks to you folks, I'm feeling real good (with a great sense of achievement) after having come here and got the great advice to learn PDO and prepared statements. Just this one nasty little hurdle is stopping me from getting to "end-of-job" on my very first WebApp. BTW, sorry about the long post...this is the best/only way I could clearly explaing my situation. Cheers guys! case "update-delete": if(isset($_POST['highlight-purchased'])) { // ****** Setup customized query to obtain only items that are checked ****** $sql = "SELECT * FROM shoplist WHERE"; for($i=0; $i < count($_POST['checkboxes']); $i++) { $sql=$sql . " idnumber=" . $_POST['checkboxes'][$i] . " or"; } $sql= rtrim($sql, "or"); $statement = $conn->prepare($sql); $statement->execute(); // *** fetch results for all checked items (1st query) *** // $result = $statement->fetchAll(); $statement->closeCursor(); // Setup query that will change the purchased flag to "N", if it's currently set to "Y" $sqlSetToN = "UPDATE shoplist SET purchased = 'N' WHERE purchased = 'Y'"; // Setup query that will change the purchased flag to "Y", if it's currently set to "N", "", or NULL $sqlSetToY = "UPDATE shoplist SET purchased = 'Y' WHERE purchased = 'N' OR purchased = '' OR purchased IS NULL"; $statementSetToN = $conn->prepare($sqlSetToN); $statementSetToY = $conn->prepare($sqlSetToY); /*** loop through the results/collection of checked items ***/ foreach($result as $row) { if ($row["purchased"] != "Y") { // *** fetch one row at a time pertaining to the 2nd query *** // $resultSetToY = $statementSetToY->fetch(); foreach($resultSetToY as $row) { $statementSetToY->execute(); } } else { // *** fetch one row at a time pertaining to the 2nd query *** // $resultSetToN = $statementSetToN->fetch(); foreach($resultSetToN as $row) { $statementSetToN->execute(); } } } break; }
-
Okay then...so I guess I'm gonna have to spend the time to understand/learn how to use "prepared statements" and then update my code accordingly. Thanks for the suggestions to avoid use of $_REQUEST and MySQL_real_escape_string calls....and thanks also for the links on how to use MySQLi and "prepared statements".
-
Ooops! Didn't realize I had to post new code...but anyways, here it is: Thanks. if(isset($_REQUEST["action"])){ switch($_REQUEST["action"]){ case "insert": $SQL="INSERT INTO stores (store_name, store_description) VALUES ("; $SQL=$SQL."'" . $_REQUEST["store_name"] . "',"; $SQL=$SQL."'" . $_REQUEST["item_description"] . "',"; $SQL=$SQL.");"; if ($mysqli->query($SQL)=== FALSE) { printf("Error – Unable to insert data to table " . $mysqli->error); } break; ... ...
-
Potential Script Conflict - How to get both functions working?
excelmaster replied to excelmaster's topic in Javascript Help
Yes, my bad...and I apologize for that....will not include extraneous code in future! >> You refer to two PHP blocks, but there are four in the code provided << Acutally it's JS code (not PHP)...and here's the code I talking about: <script> // Function to return user to same tab/function after POST/SUBMIT $(document).ready(function(){ $('a[href="' + window.location.hash + '"]').click(); }); </script> <script> // Function to display a calendar for DATE selection $(function() { $( "#datepicker" ).datepicker(); }); </script> I have gotten chunks of this code from books (and even various online sites)...so I wouldn't know better - about how not to mix and match PHP and HTML. Is there an online place (or perhaps even a book) that I can use to learn how to do that i.e. write clean and simple code (I would much prefer to do it that way myself)? -
Hello all, Another problem I have is that I'm unable to get two different functionalities working together, probably due to a potential script conflict. So, I have one small script which allows for me to remain on a specific DIV/function (such as "Updating Records") after a Form Submit/Post...and another script which provides me a JQueryUI calendar/date-picker. If I have the former (script) above the latter (script), then only the corresponding functionality will work (and the calendar/date-picker will not work). If on the other hand, I move the calendar/date-picker code above the other piece of code then only the calendar/date-picker functionality works. Is there something wrong with my code, or do I have to change the order of anything, in order to get them both play nice? Here's my code: <!doctype html> <html lang="en"> <head> <meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0" /> <title>Shopping List WebApp</title> <!-- Latest compiled and minified CSS --> <link rel="stylesheet" href="//netdna.bootstrapcdn.com/bootstrap/3.1.1/css/bootstrap.min.css"> <link rel="stylesheet" type="text/css" href="default.css"> <link href="demo.css" type="text/css" rel="stylesheet"> <link rel="stylesheet" type="text/css" media="all" href="style.css" /> <!-- Optional theme --> <link rel="stylesheet" href="//netdna.bootstrapcdn.com/bootstrap/3.1.1/css/bootstrap-theme.min.css"> <!-- jQuery --> <script src="//code.jquery.com/jquery-1.11.1.min.js"></script> <!-- Latest compiled and minified JavaScript --> <script src="//netdna.bootstrapcdn.com/bootstrap/3.1.1/js/bootstrap.min.js"></script> <link rel="stylesheet" href="//code.jquery.com/ui/1.10.4/themes/smoothness/jquery-ui.css"> <script src="//code.jquery.com/jquery-1.10.2.js"></script> <script src="//code.jquery.com/ui/1.10.4/jquery-ui.js"></script> <link rel="stylesheet" href="/resources/demos/style.css"> <script> // Function to return user to same tab/function after POST/SUBMIT $(document).ready(function(){ $('a[href="' + window.location.hash + '"]').click(); }); </script> <script> // Function to display a calendar for DATE selection $(function() { $( "#datepicker" ).datepicker(); }); </script> </head> <body> <!-- <div class="container"> --> <div class="wrapper"> <div class="body_wrapper"> <div class="row"> <div class="page-header"> <h1>Stores List WebApp</h1> </div> </div> <?php $mysqli = new mysqli('localhost', 'blah', 'blah-blah-blah', 'blah'); if ($mysqli->connect_error) { die('Connect Error (' . $mysqli->connect_errno . ') ' . $mysqli->connect_error); } if(isset($_REQUEST["action"])){ switch($_REQUEST["action"]){ case "insert": $SQL="INSERT INTO stores (store_name, store_description) VALUES ("; $SQL=$SQL."'" . $_REQUEST["store_name"] . "',"; $SQL=$SQL."'" . $_REQUEST["item_description"] . "',"; $SQL=$SQL.");"; if ($mysqli->query($SQL)=== FALSE) { printf("Error – Unable to insert data to table " . $mysqli->error); } break; case "delete": if(isset($_REQUEST['delete-selected'])) { $SQL="DELETE FROM shoplist WHERE"; for($i=0; $i < count($_REQUEST['checkboxes']); $i++){ $SQL=$SQL . " idnumber=" . $_REQUEST['checkboxes'][$i] . " or"; } $SQL= rtrim($SQL, "or"); if ($mysqli->query($SQL)== FALSE) { echo '<div class="highlight-error">Error: Unable to delete value (perhaps you have not selected anything to delete!)</div>'; } break; } else if(isset($_REQUEST['delete-all'])) { $SQL="DELETE FROM shoplist"; if ($mysqli->query($SQL)== FALSE) { echo '<div class="highlight-error">Error: Unable to delete value (perhaps there is nothing to delete!)</div>'; } break; } case "update": if(isset($_REQUEST['highlight-purchased'])) { $SQL="UPDATE shoplist SET purchased = 'Y' WHERE"; for($i=0; $i < count($_REQUEST['checkboxes']); $i++){ $SQL=$SQL . " idnumber=" . $_REQUEST['checkboxes'][$i] . " or"; } $SQL= rtrim($SQL, "or"); if ($mysqli->query($SQL)== FALSE) { echo '<div class="highlight-error">Error: Unable to update value (perhaps you have not selected anything to update!)</div>'; } else { // echo mysqli_affected_rows($mysqli). ' Records UPDATED successfully<br />'; } break; } else if(isset($_REQUEST['highlight-later'])) { $SQL="UPDATE shoplist SET later_in_week = 'Y' WHERE"; for($i=0; $i < count($_REQUEST['checkboxes']); $i++){ $SQL=$SQL . " idnumber=" . $_REQUEST['checkboxes'][$i] . " or"; } $SQL= rtrim($SQL, "or"); if ($mysqli->query($SQL)== FALSE) { echo '<div class="highlight-error">Error: Unable to update value (perhaps you have not selected anything to update!)</div>'; } else { // echo mysqli_affected_rows($mysqli). ' Records UPDATED successfully<br />'; } break; } else if(isset($_REQUEST['highlight-remove-purchased'])) { $SQL="UPDATE shoplist SET purchased = 'N' WHERE"; for($i=0; $i < count($_REQUEST['checkboxes']); $i++){ $SQL=$SQL . " idnumber=" . $_REQUEST['checkboxes'][$i] . " or"; } $SQL= rtrim($SQL, "or"); if ($mysqli->query($SQL)== FALSE) { echo '<div class="highlight-error">Error: Unable to update value (perhaps you have not selected anything to update!)</div>'; } else { // echo mysqli_affected_rows($mysqli). ' Records UPDATED successfully<br />'; } break; } else if(isset($_REQUEST['highlight-remove-later'])) { $SQL="UPDATE shoplist SET later_in_week = 'N' WHERE"; for($i=0; $i < count($_REQUEST['checkboxes']); $i++){ $SQL=$SQL . " idnumber=" . $_REQUEST['checkboxes'][$i] . " or"; } $SQL= rtrim($SQL, "or"); if ($mysqli->query($SQL)== FALSE) { echo '<div class="highlight-error">Error: Unable to update value (perhaps you have not selected anything to update!)</div>'; } else { // echo mysqli_affected_rows($mysqli). ' Records UPDATED successfully<br />'; } break; } } } ?> <div class="row"> <!-- Nav tabs --> <ul class="nav nav-tabs"> <li class="active"><a href="#add" data-toggle="tab">Add</a></li> <li><a href="#update" data-toggle="tab">Update/View</a></li> <li><a href="#delete" data-toggle="tab">Delete/View</a></li> <li><a href="#search" data-toggle="tab">Search/View</a></li> </ul> <!-- Tab panes --> <div class="tab-content"> <div class="tab-pane active" id="add"> <h3>Add Items:</h3> <p>Use this section to add new item(s).</p> <!-- <form action="#add"> --> <form class="add-data-form" id="add-data-form" action="#add" method="POST"> <input type="hidden" name="action" value="insert" /> <div class="full-row"> <div class="field-label"> Store Name: </div> <select class="store-name" name="store_name"> <option value="No Frills">No Frills</option> <option value="Super Store">Super Store</option> <option value="Food Basics">Food Basics</option> <option value="Freshco">Freshco</option> <option value="Wal Mart">Wal Mart</option> <option value="Giant Tiger">Giant Tiger</option> <option value="Foodland">Foodland</option> <option value="Metro">Metro</option> <option value="Sobeys">Sobeys</option> <option value="SDM">Shoppers Drug Mart</option> <option value="Target">Target</option> </select> </div> <div class="full-row"><div class="field-label">Store Description: </div><div class="field-input"><input class="item-desc" name="store_description" /></div></div> <input class="add-button" value="Add row" type="submit" /> </form> </div> <div class="tab-pane" id="update"> <h3>View and Update Items:</h3> <!-- <form action="#update"> --> <form class="update-data-form" id="update-data-form" action="#update" method="POST"> <input type="hidden" name="action" value="update" /> <div class="full-row-heading"> <table> <tr> <th><span class="spacer-store-name">Store</span></th> <th><span class="spacer-item-desc">Desc.</span></th> </tr> </table> </div> <table> <?php $result = $mysqli->query("SELECT * FROM shoplist ORDER BY store_name ASC"); while($row = $result->fetch_assoc()){ print '<tr>'; print '<td><span class="filler-checkbox"><input type="checkbox" name="checkboxes[]" value="' . $row["idnumber"] . '" /></span></td>'; if ($row["purchased"] == "Y") { print '<td><span class="highlight-purchased">' . $row["store_name"] . '</span></td>'; print '<td><span class="highlight-purchased">' . $row["store_description"] . '</span></td>'; } else if (($row["later_in_week"] == "Y") && ($row["purchased"] != "Y")) { print '<td><span class="highlight-later">' . $row["store_name"] . '</span></td>'; print '<td><span class="highlight-later">' . $row["store_description"] . '</span></td>'; } else { print '<td>' . $row["store_name"] . '</td>'; print '<td>' . $row["store_description"] . '</td>'; } print '</tr>'; } ?> </table> <div class="full-row-buttons"> <input class="update-button-purchased" name="highlight-purchased" value="Highlight selected item(s) as *Purchased*" type="submit"/> <input class="update-button-later" name="highlight-later" value="Highlight selected item(s) as *Later in Week*" type="submit"/> </div> <div class="full-row-buttons"> <input class="update-button-remove-purchased" name="highlight-remove-purchased" value="Unhighlight selected *Purchased* item(s)" type="submit"/> <input class="update-button-remove-later" name="highlight-remove-later" value="Unhighlight selected *Later in Week* item(s)" type="submit"/> </div> </form> </div> <div class="tab-pane" id="delete"> <h3>View and Delete Items:</h3> <!-- <form action="#delete"> --> <form class="delete-data-form" id="delete-data-form" action="#delete" method="POST"> <input type="hidden" name="action" value="delete" /> <div class="full-row-heading"> <table> <tr> <th><span class="spacer-store-name">Store</span></th> <th><span class="spacer-item-desc">Desc</span></th> </tr> </table> </div> <table> <?php $result = $mysqli->query("SELECT * FROM shoplist ORDER BY store_name ASC"); while($row = $result->fetch_assoc()){ print '<tr>'; print '<td><span class="filler-checkbox"><input type="checkbox" name="checkboxes[]" value="' . $row["idnumber"] . '" /></span></td>'; if ($row["purchased"] == "Y") { print '<td><span class="highlight-purchased">' . $row["store_name"] . '</span></td>'; print '<td><span class="highlight-purchased">' . $row["store_description"] . '</span></td>'; } else if (($row["later_in_week"] == "Y") && ($row["purchased"] != "Y")) { print '<td><span class="highlight-later">' . $row["store_name"] . '</span></td>'; print '<td><span class="highlight-later">' . $row["store_description"] . '</span></td>'; } else { print '<td>' . $row["store_name"] . '</td>'; print '<td>' . $row["store_description"] . '</td>'; } print '</tr>'; } ?> </table> <input class="delete-button-selected" name="delete-selected" value="Delete selected row(s)" type="submit"/> <input class="delete-button-all" name="delete-all" value="Delete ALL row(s)" type="submit"/> </form> </div> <div class="tab-pane" id="search"> <h3>Search and View Items:</h3> <form class="search-data-form" id="search-data-form" action="#search" method="POST"> <div class="search-container"> <div class="full-row"> <div class="field-label"> Choose search field: </div> <div class="field-input"> <select name="searchtype"> <option value="store_name">Store Name</option> <option value="item_description">Store Description</option> </select> </div> <br /> </div> <div class="full-row"> <div class="field-label"> Enter search term: </div> <div class="field-input"> <input name="searchterm" type="text" size="40"/> </div> <br /> </div> </div> <input class="search-button" type="submit" name="submit" value="Search"/> <div class="full-row-heading"> <table> <tr> <th><span class="spacer-store-name">Store</span></th> <th><span class="spacer-store-desc">Desc.</span></th> </tr> </table> </div> <table> <?php // create short variable names $searchtype=$_POST['searchtype']; $searchterm=trim($_POST['searchterm']); if (!get_magic_quotes_gpc()) { $searchtype = addslashes($searchtype); $searchterm = addslashes($searchterm); } $query = "select * from shoplist where " . $searchtype . " like '%" . $searchterm . "%' ORDER BY " . $searchtype . " ASC"; $result = $mysqli->query($query); $num_results = $result->num_rows; for ($i=0; $i < $num_results; $i++) { $row = $result->fetch_assoc(); print "<tr>"; if ($row["purchased"] == "Y") { print '<td><span class="highlight-purchased">' . ' ' . $row["store_name"] . '</span></td>'; print '<td><span class="highlight-purchased">' . $row["store_description"] . '</span></td>'; } else if (($row["later_in_week"] == "Y") && ($row["purchased"] != "Y")) { print '<td><span class="highlight-later">' . ' ' . $row["store_name"] . '</span></td>'; print '<td><span class="highlight-later">' . $row["store_description"] . '</span></td>'; } else { print '<td>' . ' ' . $row["store_name"] . '</td>'; print '<td>' . $row["store_description"] . '</td>'; } print "</tr>"; } $result->free(); $mysqli->close(); ?> </table> </form> </div> </div> </div> </div> </div> <!-- </div> --> </body> </html>
-
Hello all, Newbie here....and I've tried a number of differet ways (using the code included below) to incorporate the "mysqli_real_escape_string" function into my code but have been unsuccessful. How (or shoudl I say where) would I include this function, in order to be able to have my data inserted correctly, whilst also avoiding a potential SQL injection attack? Appreciate all the help you guys provide here on this wonderful forum. <!doctype html> <html lang="en"> <head> <meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0" /> <title>Shopping List WebApp</title> <!-- Latest compiled and minified CSS --> <link rel="stylesheet" href="//netdna.bootstrapcdn.com/bootstrap/3.1.1/css/bootstrap.min.css"> <link rel="stylesheet" type="text/css" href="default.css"> <link href="demo.css" type="text/css" rel="stylesheet"> <link rel="stylesheet" type="text/css" media="all" href="style.css" /> <!-- Optional theme --> <link rel="stylesheet" href="//netdna.bootstrapcdn.com/bootstrap/3.1.1/css/bootstrap-theme.min.css"> <!-- jQuery --> <script src="//code.jquery.com/jquery-1.11.1.min.js"></script> <!-- Latest compiled and minified JavaScript --> <script src="//netdna.bootstrapcdn.com/bootstrap/3.1.1/js/bootstrap.min.js"></script> <link rel="stylesheet" href="//code.jquery.com/ui/1.10.4/themes/smoothness/jquery-ui.css"> <script src="//code.jquery.com/jquery-1.10.2.js"></script> <script src="//code.jquery.com/ui/1.10.4/jquery-ui.js"></script> <link rel="stylesheet" href="/resources/demos/style.css"> <script> // Function to return user to same tab/function after POST/SUBMIT $(document).ready(function(){ $('a[href="' + window.location.hash + '"]').click(); }); </script> <script> // Function to display a calendar for DATE selection $(function() { $( "#datepicker" ).datepicker(); }); </script> </head> <body> <!-- <div class="container"> --> <div class="wrapper"> <div class="body_wrapper"> <div class="row"> <div class="page-header"> <h1>Stores List WebApp</h1> </div> </div> <?php $mysqli = new mysqli('localhost', 'blah', 'blah-blah-blah', 'blah'); if ($mysqli->connect_error) { die('Connect Error (' . $mysqli->connect_errno . ') ' . $mysqli->connect_error); } if(isset($_REQUEST["action"])){ switch($_REQUEST["action"]){ case "insert": $SQL="INSERT INTO stores (store_name, store_description) VALUES ("; $SQL=$SQL."'" . $_REQUEST["store_name"] . "',"; $SQL=$SQL."'" . $_REQUEST["item_description"] . "',"; $SQL=$SQL.");"; if ($mysqli->query($SQL)=== FALSE) { printf("Error – Unable to insert data to table " . $mysqli->error); } break; case "delete": if(isset($_REQUEST['delete-selected'])) { $SQL="DELETE FROM shoplist WHERE"; for($i=0; $i < count($_REQUEST['checkboxes']); $i++){ $SQL=$SQL . " idnumber=" . $_REQUEST['checkboxes'][$i] . " or"; } $SQL= rtrim($SQL, "or"); if ($mysqli->query($SQL)== FALSE) { echo '<div class="highlight-error">Error: Unable to delete value (perhaps you have not selected anything to delete!)</div>'; } break; } else if(isset($_REQUEST['delete-all'])) { $SQL="DELETE FROM shoplist"; if ($mysqli->query($SQL)== FALSE) { echo '<div class="highlight-error">Error: Unable to delete value (perhaps there is nothing to delete!)</div>'; } break; } case "update": if(isset($_REQUEST['highlight-purchased'])) { $SQL="UPDATE shoplist SET purchased = 'Y' WHERE"; for($i=0; $i < count($_REQUEST['checkboxes']); $i++){ $SQL=$SQL . " idnumber=" . $_REQUEST['checkboxes'][$i] . " or"; } $SQL= rtrim($SQL, "or"); if ($mysqli->query($SQL)== FALSE) { echo '<div class="highlight-error">Error: Unable to update value (perhaps you have not selected anything to update!)</div>'; } else { // echo mysqli_affected_rows($mysqli). ' Records UPDATED successfully<br />'; } break; } else if(isset($_REQUEST['highlight-later'])) { $SQL="UPDATE shoplist SET later_in_week = 'Y' WHERE"; for($i=0; $i < count($_REQUEST['checkboxes']); $i++){ $SQL=$SQL . " idnumber=" . $_REQUEST['checkboxes'][$i] . " or"; } $SQL= rtrim($SQL, "or"); if ($mysqli->query($SQL)== FALSE) { echo '<div class="highlight-error">Error: Unable to update value (perhaps you have not selected anything to update!)</div>'; } else { // echo mysqli_affected_rows($mysqli). ' Records UPDATED successfully<br />'; } break; } else if(isset($_REQUEST['highlight-remove-purchased'])) { $SQL="UPDATE shoplist SET purchased = 'N' WHERE"; for($i=0; $i < count($_REQUEST['checkboxes']); $i++){ $SQL=$SQL . " idnumber=" . $_REQUEST['checkboxes'][$i] . " or"; } $SQL= rtrim($SQL, "or"); if ($mysqli->query($SQL)== FALSE) { echo '<div class="highlight-error">Error: Unable to update value (perhaps you have not selected anything to update!)</div>'; } else { // echo mysqli_affected_rows($mysqli). ' Records UPDATED successfully<br />'; } break; } else if(isset($_REQUEST['highlight-remove-later'])) { $SQL="UPDATE shoplist SET later_in_week = 'N' WHERE"; for($i=0; $i < count($_REQUEST['checkboxes']); $i++){ $SQL=$SQL . " idnumber=" . $_REQUEST['checkboxes'][$i] . " or"; } $SQL= rtrim($SQL, "or"); if ($mysqli->query($SQL)== FALSE) { echo '<div class="highlight-error">Error: Unable to update value (perhaps you have not selected anything to update!)</div>'; } else { // echo mysqli_affected_rows($mysqli). ' Records UPDATED successfully<br />'; } break; } } } ?> <div class="row"> <!-- Nav tabs --> <ul class="nav nav-tabs"> <li class="active"><a href="#add" data-toggle="tab">Add</a></li> <li><a href="#update" data-toggle="tab">Update/View</a></li> <li><a href="#delete" data-toggle="tab">Delete/View</a></li> <li><a href="#search" data-toggle="tab">Search/View</a></li> </ul> <!-- Tab panes --> <div class="tab-content"> <div class="tab-pane active" id="add"> <h3>Add Items:</h3> <p>Use this section to add new item(s).</p> <!-- <form action="#add"> --> <form class="add-data-form" id="add-data-form" action="#add" method="POST"> <input type="hidden" name="action" value="insert" /> <div class="full-row"> <div class="field-label"> Store Name: </div> <select class="store-name" name="store_name"> <option value="No Frills">No Frills</option> <option value="Super Store">Super Store</option> <option value="Food Basics">Food Basics</option> <option value="Freshco">Freshco</option> <option value="Wal Mart">Wal Mart</option> <option value="Giant Tiger">Giant Tiger</option> <option value="Foodland">Foodland</option> <option value="Metro">Metro</option> <option value="Sobeys">Sobeys</option> <option value="SDM">Shoppers Drug Mart</option> <option value="Target">Target</option> </select> </div> <div class="full-row"><div class="field-label">Store Description: </div><div class="field-input"><input class="item-desc" name="store_description" /></div></div> <input class="add-button" value="Add row" type="submit" /> </form> </div> <div class="tab-pane" id="update"> <h3>View and Update Items:</h3> <!-- <form action="#update"> --> <form class="update-data-form" id="update-data-form" action="#update" method="POST"> <input type="hidden" name="action" value="update" /> <div class="full-row-heading"> <table> <tr> <th><span class="spacer-store-name">Store</span></th> <th><span class="spacer-item-desc">Desc.</span></th> </tr> </table> </div> <table> <?php $result = $mysqli->query("SELECT * FROM shoplist ORDER BY store_name ASC"); while($row = $result->fetch_assoc()){ print '<tr>'; print '<td><span class="filler-checkbox"><input type="checkbox" name="checkboxes[]" value="' . $row["idnumber"] . '" /></span></td>'; if ($row["purchased"] == "Y") { print '<td><span class="highlight-purchased">' . $row["store_name"] . '</span></td>'; print '<td><span class="highlight-purchased">' . $row["store_description"] . '</span></td>'; } else if (($row["later_in_week"] == "Y") && ($row["purchased"] != "Y")) { print '<td><span class="highlight-later">' . $row["store_name"] . '</span></td>'; print '<td><span class="highlight-later">' . $row["store_description"] . '</span></td>'; } else { print '<td>' . $row["store_name"] . '</td>'; print '<td>' . $row["store_description"] . '</td>'; } print '</tr>'; } ?> </table> <div class="full-row-buttons"> <input class="update-button-purchased" name="highlight-purchased" value="Highlight selected item(s) as *Purchased*" type="submit"/> <input class="update-button-later" name="highlight-later" value="Highlight selected item(s) as *Later in Week*" type="submit"/> </div> <div class="full-row-buttons"> <input class="update-button-remove-purchased" name="highlight-remove-purchased" value="Unhighlight selected *Purchased* item(s)" type="submit"/> <input class="update-button-remove-later" name="highlight-remove-later" value="Unhighlight selected *Later in Week* item(s)" type="submit"/> </div> </form> </div> <div class="tab-pane" id="delete"> <h3>View and Delete Items:</h3> <!-- <form action="#delete"> --> <form class="delete-data-form" id="delete-data-form" action="#delete" method="POST"> <input type="hidden" name="action" value="delete" /> <div class="full-row-heading"> <table> <tr> <th><span class="spacer-store-name">Store</span></th> <th><span class="spacer-item-desc">Desc</span></th> </tr> </table> </div> <table> <?php $result = $mysqli->query("SELECT * FROM shoplist ORDER BY store_name ASC"); while($row = $result->fetch_assoc()){ print '<tr>'; print '<td><span class="filler-checkbox"><input type="checkbox" name="checkboxes[]" value="' . $row["idnumber"] . '" /></span></td>'; if ($row["purchased"] == "Y") { print '<td><span class="highlight-purchased">' . $row["store_name"] . '</span></td>'; print '<td><span class="highlight-purchased">' . $row["store_description"] . '</span></td>'; } else if (($row["later_in_week"] == "Y") && ($row["purchased"] != "Y")) { print '<td><span class="highlight-later">' . $row["store_name"] . '</span></td>'; print '<td><span class="highlight-later">' . $row["store_description"] . '</span></td>'; } else { print '<td>' . $row["store_name"] . '</td>'; print '<td>' . $row["store_description"] . '</td>'; } print '</tr>'; } ?> </table> <input class="delete-button-selected" name="delete-selected" value="Delete selected row(s)" type="submit"/> <input class="delete-button-all" name="delete-all" value="Delete ALL row(s)" type="submit"/> </form> </div> <div class="tab-pane" id="search"> <h3>Search and View Items:</h3> <form class="search-data-form" id="search-data-form" action="#search" method="POST"> <div class="search-container"> <div class="full-row"> <div class="field-label"> Choose search field: </div> <div class="field-input"> <select name="searchtype"> <option value="store_name">Store Name</option> <option value="item_description">Store Description</option> </select> </div> <br /> </div> <div class="full-row"> <div class="field-label"> Enter search term: </div> <div class="field-input"> <input name="searchterm" type="text" size="40"/> </div> <br /> </div> </div> <input class="search-button" type="submit" name="submit" value="Search"/> <div class="full-row-heading"> <table> <tr> <th><span class="spacer-store-name">Store</span></th> <th><span class="spacer-store-desc">Desc.</span></th> </tr> </table> </div> <table> <?php // create short variable names $searchtype=$_POST['searchtype']; $searchterm=trim($_POST['searchterm']); if (!get_magic_quotes_gpc()) { $searchtype = addslashes($searchtype); $searchterm = addslashes($searchterm); } $query = "select * from shoplist where " . $searchtype . " like '%" . $searchterm . "%' ORDER BY " . $searchtype . " ASC"; $result = $mysqli->query($query); $num_results = $result->num_rows; for ($i=0; $i < $num_results; $i++) { $row = $result->fetch_assoc(); print "<tr>"; if ($row["purchased"] == "Y") { print '<td><span class="highlight-purchased">' . ' ' . $row["store_name"] . '</span></td>'; print '<td><span class="highlight-purchased">' . $row["store_description"] . '</span></td>'; } else if (($row["later_in_week"] == "Y") && ($row["purchased"] != "Y")) { print '<td><span class="highlight-later">' . ' ' . $row["store_name"] . '</span></td>'; print '<td><span class="highlight-later">' . $row["store_description"] . '</span></td>'; } else { print '<td>' . ' ' . $row["store_name"] . '</td>'; print '<td>' . $row["store_description"] . '</td>'; } print "</tr>"; } $result->free(); $mysqli->close(); ?> </table> </form> </div> </div> </div> </div> </div> <!-- </div> --> </body> </html>
-
How do I return to a page/section after a "Form Submit"?
excelmaster replied to excelmaster's topic in PHP Coding Help
@maxxd: WOW!!! that's a ton of techno-mambo-jambo you've thrown at me (a newbie) right there!!! Anyways, as it turns out, I worked on this till late last night (determined to get to the bottom of the problem, and find a fix)....and find a fix I did! Well, actually it wasn't really a fix, but rather, just a placement-of-code-issue. So I used the code (as-is) from the technique shown in the link below (which is pretty much what you were suggesting earlier - thanks for that suggestion) - and it worked on my site as-is. But then, when I went into the supplied code and started adding in all my bits-and-pieces of code, it wouldn't work the way it was supposed to i.e. remain on the same tab/function/operation after a POST/SUBMIT. https://github.com/N....and/index.html Turns out that the bit of JS code at the very end (just before the closing BODY tag) had to be moved up top (before the closing HEAD tag), and then, and only then did it work. But guess what? That "threw another spanner in the works" and I could no longer get my date-picker to work. I then found out that if I moved the date-picker JS code above the "remain-on-selected-tab" code then the date-picker functionality worked but the "remain-on-selected-tab" functionality no longer worked. So this is my new problem/dilemma!!! I can only get one or the other to work....not both at the same time...which is very much what I would like to have. Needless to say, I'm doing without the date-picker code (for now) since I don't mind typing in a date manually. Any ideas on how I can resolve this new problem? But anways, much thanks to both yourself and @ginerjm for helping me out with this....much appreciated! BTW, those are not my real credentials in the mysqli instantiation...I just made those up. Thanks for the heads-up though. https://github.com/N....and/index.html -
How do I return to a page/section after a "Form Submit"?
excelmaster replied to excelmaster's topic in PHP Coding Help
I've just taken a closer look at my code and found out that the 3 lines of code (just after the <title></title> tags) pertaining to JQueryUI tabs are only left there as remnants of my previous attempt to find a solution to this problem. Other than that, JQueryUI tabs is not being used, per se. I've removed those 3 lines of code and I'm still able to use the app...albeit with the same problem of returning to the "add" form after clicking the submit button from one of the other 3 forms. -
How do I return to a page/section after a "Form Submit"?
excelmaster replied to excelmaster's topic in PHP Coding Help
I have tried a number of different techniques (JQueryUI tabs being just the last one I tried) to achieve this functionality, but I seem to be running into the very same brick-wall each time. Is there something wrong with JQueryUI tabs (which I should know about, and perhaps never use)? As a matter of fact, I've even tried the following solution (which was provided on a different site), and it's exactly what @maxxd suggested above. That too failed to work as soon as I incorporated the required pieces of my code into the basic code provided in the second link below. http://neundorf.vela.uberspace.de/we...ple-Forms-and/ https://github.com/Naxmeify/webdevqs...and/index.html -
How do I return to a page/section after a "Form Submit"?
excelmaster replied to excelmaster's topic in PHP Coding Help
And where would I place that piece of code? Also, I presume it would have to be enclosed with <script></script> tags...correct? -
How do I return to a page/section after a "Form Submit"?
excelmaster replied to excelmaster's topic in PHP Coding Help
@maxxd: I tried what you suggested, but still no joy :-( Just to clarify though: I had this => <form class="add-data-form" action="#add" method="POST">, and you suggested I change it to this => <form class="add-data-form" action="index.php#add" method="POST">...correct? I really don't have the knowledge/skills to write the little script to activate a specific DIV/section on page load...so do you have something like that handy, which you can pass on to me? @ginerjm: Yes, your terminology makes total sense, and mine certainly didn't! So, just forget that I mentioned the word "tabs"....which as you correctly pointed out are essentially items in an unordered list. So, if you look at my screenshot above (3rd post), you'll notice that I have four unordered list items laid out horizontally across the top. Just below those list items is a DIV (four DIV's actually, if you look at the code, but three of them are hidden) which dislays the respective form for the selected list item. Looking at the screenshot again, you will notice that I have a submit button titled "Add Row", for that form. Similarly, I display a delete form with a corresponding button titled "Delete Row" when the "Delete/View" list item is selected. My dilemma is how to achieve what you're suggesting in the last sentence of your paragraph above i.e. >> "....so you can set them while handling the submit and then when you send that page back the settings will be as you wish." Thanks for your patience...much appreciated! -
How do I return to a page/section after a "Form Submit"?
excelmaster replied to excelmaster's topic in PHP Coding Help
Yes indeed, they are all together on the page (to begin with), but depending on which function/tab I'm working on/with, the content section (i.e. the forms, submit buttons etc.) of the other three tabs are being hidden, and they should remain hidden till such time that I've completed working with that function (i.e. add, delete, udpate, search) by selecting one of the other three tabs (up top). Is that any clearer, or is it still confusing? -
How do I return to a page/section after a "Form Submit"?
excelmaster replied to excelmaster's topic in PHP Coding Help
My apologies for not being clear enough and/or for confusing you! The tabs I'm talking about are not "browser tabs", but rather, just text that's enclosed in "<ul><li></li</ul>" and formatted (using CSS) to look like tabs (see attached screenshot). I don't quite understand your 2nd and 3rd senetences...but perhaps they may now be a moot point (with the above clarification). Thanks though, for trying to help..much appreciated!