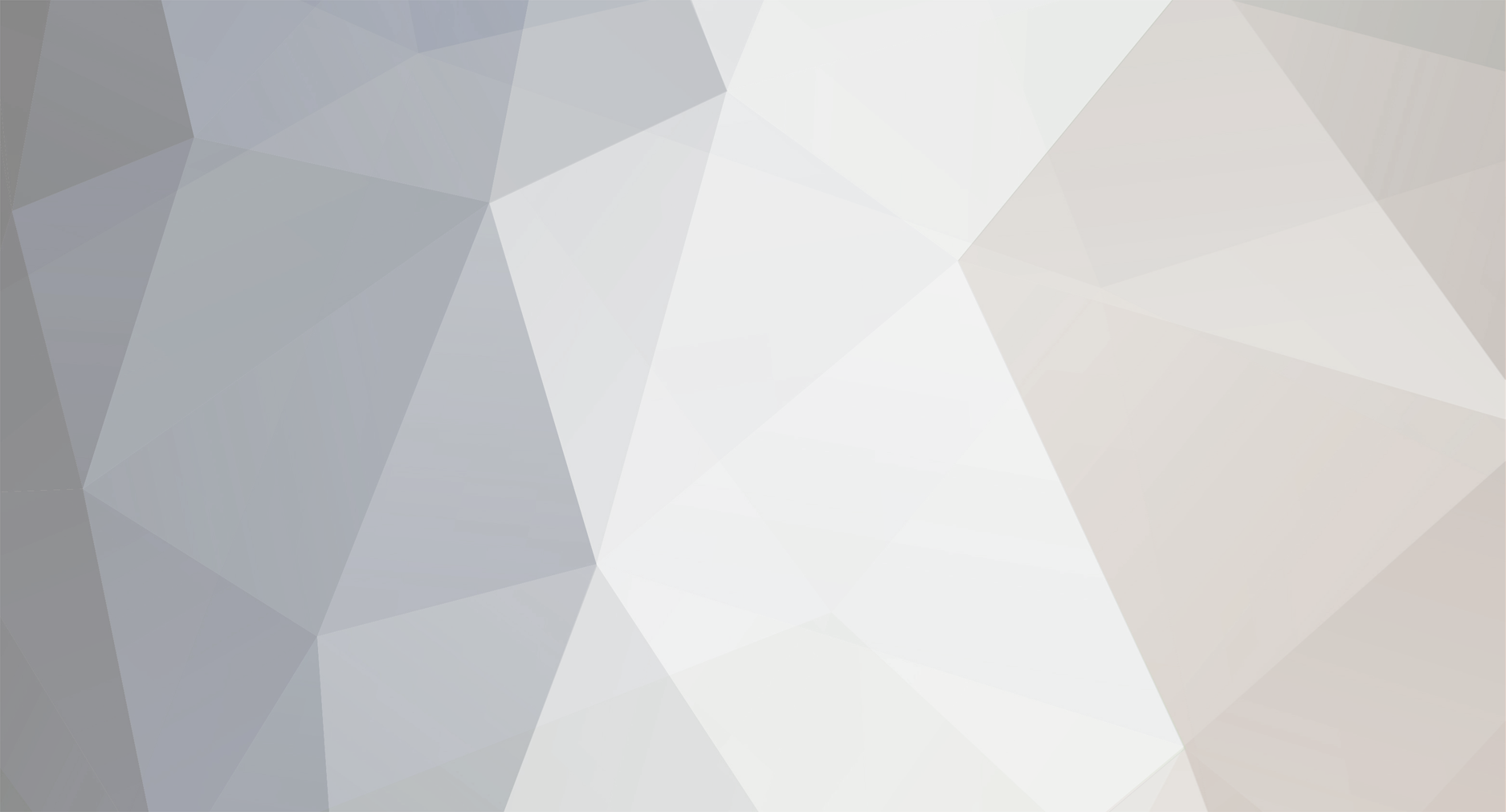
annihilate
Members-
Posts
63 -
Joined
-
Last visited
Everything posted by annihilate
-
Use this, the error then may be a bit more helpful than what you currently have. [code]$result = mysql_query($sql) or die(mysql_error()); [/code]
-
I include the following code on every page, and for any database queries with form data, apply mysql_real_escape_string to the form variables. You don't need to apply any functions to retrieve from the database, the text in there wil be exactly as you submitted it. [code]if ( get_magic_quotes_gpc() ) { function stripslashes_deep($value) { $value = is_array($value) ? array_map('stripslashes_deep', $value) : stripslashes($value); return $value; } $_POST = stripslashes_deep($_POST); $_GET = stripslashes_deep($_GET); $_COOKIE = stripslashes_deep($_COOKIE); }[/code]
-
Hi, I have this function that I found on the net for converting urls posted in a string into hyperlinks when they are printed to the browser. [code]function make_links($text) { $text = eregi_replace('(((f|ht){1}tp://)[-a-zA-Z0-9@:%_\+.~#?&//=]+)', '<a href="\\1" target="_blank">\\1</a>', $text); $text = eregi_replace('([[:space:]()[{}])(www.[-a-zA-Z0-9@:%_\+.~#?&//=]+)', '\\1<a href="http://\\2" target="_blank">\\2</a>', $text); return $text; }[/code] Problem is I want to make the urls produced xhtml valid, so I want to apply htmlspecialchars or htmlentities to the links so that urls with &p=7 for example are turned into &p=7 in the source code. Anyone know if it could be put inside the eregi_replace somewhere. Thanks Colin
-
dynamic image display using php id tag... pls help
annihilate replied to rebeat's topic in PHP Coding Help
No problem. Glad you have it working the way you want. -
0 Not recognised as php required field imput?
annihilate replied to Captain Salad's topic in PHP Coding Help
I would change all the || into &&. And if you want to allow some fileds to be left blank, put the min value as 0. I have quickly done a test of whether it works and it works perfectly. This is the little bit of code I tested on. [code]<?php function checkLength($string, $min, $max) { $length = strlen(trim($string)); if ( ($length < $min) || ($length > $max) ) { return FALSE; } else { return TRUE; } } $name = trim(strip_tags($_POST['Name'])); // Name $email = trim($_POST['Email']); // Email if ( checkLength($email, 1, 50) && checkLength($name, 1, 50)) { echo 'Passed validation'; } else { echo 'Failed'; } ?> <form name="Contact" action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <table class="padform"> <tr><td><label for="Name">Name</label></td><td><input name="Name" id="Name" type="text" size="50" maxlength="50" value="<?php if ( isset($_POST['Name']) ) echo trim(strip_tags($_POST['Name'])); ?>" /></td></tr> <tr><td><label for="Email">Email</label></td><td><input name="Email" id="Email" type="text" size="50" maxlength="50" value="<?php if ( isset($_POST['Email']) ) echo trim($_POST['Email']); ?>" /></td></tr> <tr><td> </td><td align="center"><input name="Submit" type="submit" value="Contact" /></td></tr> </table> </form>[/code] -
But they must have to click on a link to go the edit page? And that link should be passing the jsid in the url so you know what to edit, and can prefill in the form fields with the info from the database.
-
Are you remembering to pass it as a variable in the url. ie on first load of the page, it should be yourpage.php?jsid=7 for example. Then the hidden form variable for jsid will have the value of 7. And then on submission of the form, the update query should have the value of 7 for $jsid.
-
Echo out $sql on the line above 'Your details have been updated' to check that $jsid has a value.
-
0 Not recognised as php required field imput?
annihilate replied to Captain Salad's topic in PHP Coding Help
Put this anywhere on the page, I would put at the top though. [code]// String length // Usage: checkLength(string to check length of, min length, max length) function checkLength($string, $min, $max) { $length = strlen(trim($string)); if ( ($length < $min) || ($length > $max) ) { return FALSE; } else { return TRUE; } }[/code] Then change this code section: [code]if (!isset($_REQUEST['email'])) { header( "Location: http://www.brittalk.com/brittalk/Templates...actUs.htm" ); } elseif (empty($email) || empty($transfer) || empty($title) || empty($firstname) || empty($lastname) || empty($dob) || empty($currentaddress)[/code] To this (I would run the checkLength function on all fields) [code]if (!isset($_REQUEST['email'])) { header( "Location: http://www.brittalk.com/brittalk/Templates...actUs.htm" ); } elseif (checkLength($email,1,40) || checkLength($transfer,1,50) ) // etc etc etc[/code] If you just want it on the yearsataddress field, then change empty($yearsataddress) to checkLength($yearsataddress, 1, 5) or something. The 1 is the min number of digits, and the 5 is the max. Just change those numbers depending on how long or short the field can be. -
0 Not recognised as php required field imput?
annihilate replied to Captain Salad's topic in PHP Coding Help
Try this, substitute the function empty in your script for checkLength Example use: checkLength($title, 0, 50); [code]// String length // Usage: checkLength(string to check length of, min length, max length) function checkLength($string, $min, $max) { $length = strlen(trim($string)); if ( ($length < $min) || ($length > $max) ) { return FALSE; } else { return TRUE; } }[/code] -
0 Not recognised as php required field imput?
annihilate replied to Captain Salad's topic in PHP Coding Help
[a href=\"http://uk2.php.net/empty\" target=\"_blank\"]http://uk2.php.net/empty[/a] The string '0' is considered empty, so will have to use a different method of validation if you want to allow zeros. -
dynamic image display using php id tag... pls help
annihilate replied to rebeat's topic in PHP Coding Help
Try this: If you view the page passing no variables in the url, then home.php is included. If you specify an image to view, them image is shown, nothing is inlcuded. If you specify just an id, then that is included if it exists, else 404.php is included. If you specify a page and image, then both are shown. [code]<?php if ( isset($_GET['id']) && $_GET['id'] <> '' ) // If id is set, then set include to that value { $include = $_GET['id']; } elseif ( (!isset($_GET['id']) || $_GET['id'] == '') && isset($_GET['image']) ) // If id not set, but image is, don't include anything { $include = FALSE; } else // id and image not set, so include a default page { $include = 'home.php'; } if ( $include != FALSE) { if ( is_file($include) ) // If finds file, then include it { include $include; } else // File doesn't exist, so show 404 page { include '404.php'; } } // Show the image if set if ( isset($_GET['image']) && $_GET['image'] <> '' ) { $image = $_GET['image']; // Do some validation on the image string here, if passes then show image // Obviously need to provide full path to image unless it is in same folder as script // eg <img src="images/'.$image.'" /> echo '<img src="'.$image.'" />'; } ?>[/code] -
That is because you have changed my code. [code]echo "<tr bgcolor='#eeeeee'> <td>".$rows['status']."</td><td>".$rows['imagename']."</td><td>".$rows['date']."</td><td>".$rows['description']."</td><td><a href="/memberseditimages.php?editid='.$id.'">Edit</a></td> </tr>";[/code] In the above, that is not what I posted. Use this: [code]echo '<tr bgcolor="#eeeeee"> <td>'.$rows['status'].'</td><td>'.$rows['imagename'].'</td><td>'.$rows['date'].'</td><td>'.$rows['description'].'</td> <td><a href="/memberseditimages.php?editid='.$id.'">Edit</a></td> </tr>';[/code] And the other echo statement should be this: [code]echo '<tr bgcolor="#c0c0c0"> <td>'.$rows['status'].'</td><td>'.$rows['imagename'].'</td><td>'.$rows['date'].'</td><td>'.$rows['description'].'</td> <td><a href="/memberseditimages.php?editid='.$id.'">Edit</a></td> </tr>';[/code] And I would change lines like $color = "2"; to $color = 2;
-
dynamic image display using php id tag... pls help
annihilate replied to rebeat's topic in PHP Coding Help
Try this: [code]<?php if ( isset($_GET['id']) && $_GET['id'] <> '' ) { $include = $_GET['id']; } else // No page specified, so set a default { $include = 'home.php'; } if ( is_file($include) ) { include $include; } else { include '404.php'; } if ( isset($_GET['image']) && $_GET['image'] <> '' ) { $image = $_GET['image']; // Do some validation on the image string here, if passes then show image // Obviously need to provide full path to image unless it is in same folder as script // eg <img src="images/'.$image.'" /> echo '<img src="'.$image.'" />'; } ?>[/code] -
while($rw=mysql_fetch_assoc($feedback)) { replace $feedback with $rs
-
It would go before you do the insert, and the insert would be done depending on the rows found. You need more code than just adding that one line in, but it shouldn't be too difficult to work out. However, I wouldn't do my suggestion anyway, read Fenway's answer, which is much better than mine :-)
-
My guess is that you can't use mysql_fetch_array on a query that uses count. Try $total = mysql_result($totalRun, 0, 0);
-
My fault, didn't close of the last echo statement! Add '; to the end after the last tr.
-
Try this. [code]<?php if ($color == 1) { echo '<tr bgcolor="#eeeeee"> <td>'.$rows['status'].'</td><td>'.$rows['imagename'].'</td><td>'.$rows['date'].'</td><td>'.$rows['description'].'</td><td><a href="/memberseditimages.php?editid='.$id.'">Edit</a></td> </tr>'; // Set $color==2, for switching to other color $color = 2; } // When $color not equal 1, use this table row color else { echo '<tr bgcolor="#c0c0c0"> <td>'.$rows['status'].'</td><td>'.$rows['imagename'].'</td><td>'.$rows['date'].'</td><td>'.$rows['description'].'</td><td><a href="/memberseditimages.php?editid='.$id.'">Edit</a></td></tr> ?>[/code]
-
Have a look at [a href=\"http://uk2.php.net/strip_tags\" target=\"_blank\"]strip_tags[/a] function and some of the user contributed functions and responses on that page.
-
Is there a session variable for a userid or something? Then you can just do your query like so: [code]$query = sprintf("SELECT firstname, lastname FROM users WHERE userid = '".$_SESSION['userid']."'");[/code]
-
Glad you got it working. I didn't realise you were passing hidden values. If I had looked more closely I would have spotted that straight away :-)
-
Echo out the value of $sql to the browser and see if it contains the correct value for id.
-
splitting variable content where a ',' exists
annihilate replied to essjay_d12's topic in PHP Coding Help
[code]$num_in_array = count($arr);[/code] -
Refreshing the page straight after submission is resubmitting the data, so that's probably why it appears again. To prevent this, just query the database to see if the data being submitted is already there. If the username must be unique, then just query the username field. Limit the query to 1 row. If it finds 1 row, then don't insert, else no rows are found so do the insert. [code]SELECT name FROM members WHERE name = $new_username LIMIT 1;[/code]