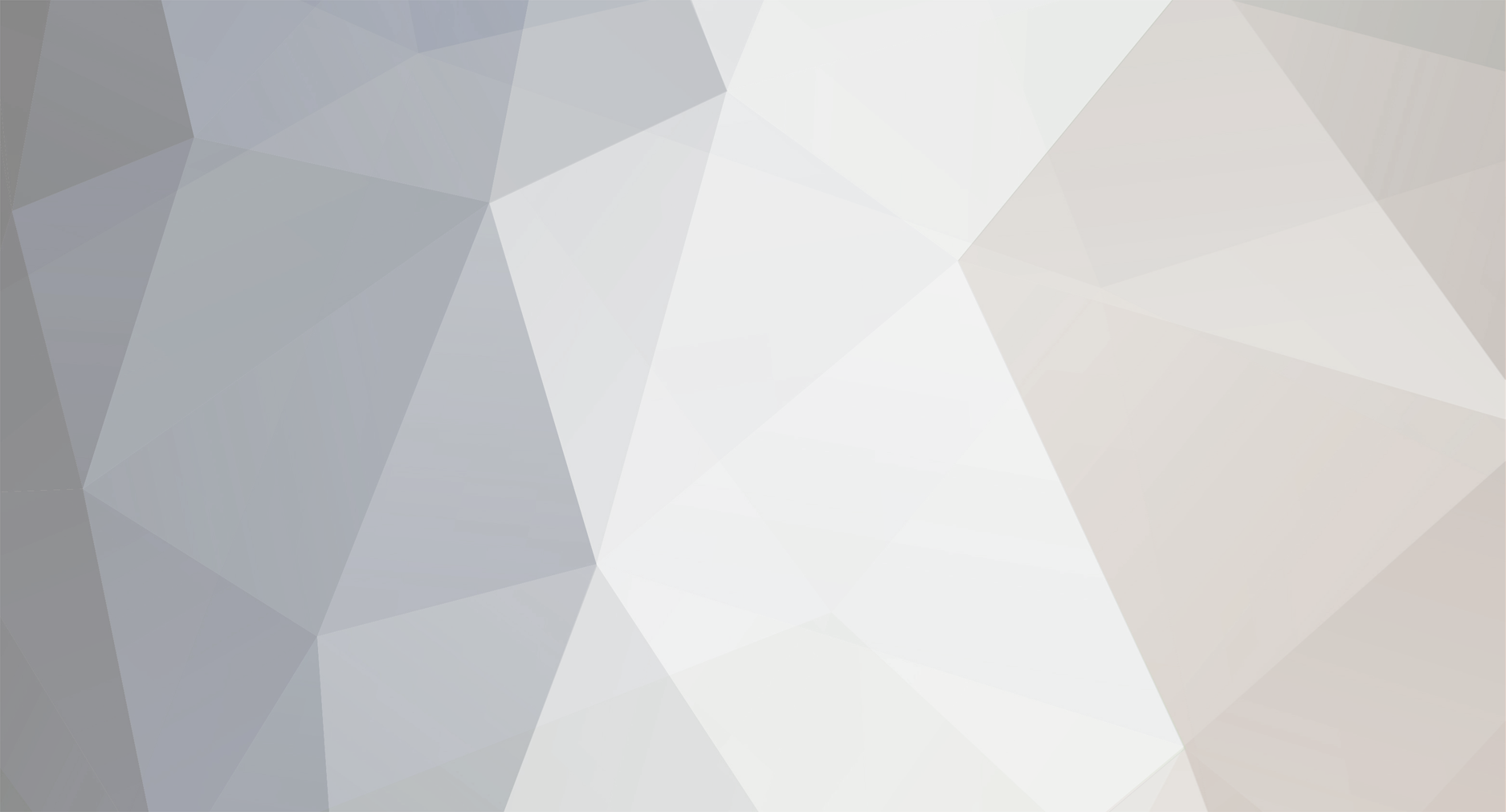
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
I'll take this approach to generating sql queries based on input from a form. <?php $conn = mysql_connect($dbhost, $dbuser, $dbpass) or die('Error connecting to mysql'); mysql_select_db($dbnameprim); $pagedb = "members"; function make_safe($value) { if(is_numeric($value)) { $value = (int) $value; } elseif(is_string($value)) { $value = "'" . mysql_real_escape_string($value) . "'"; } return $value; } // list form fields to be inserted into the database $fields = array('firstname', 'lastname', 'password', 'email'); $db = null; if (isset($_POST['save'])) { // list through the $fields array foreach($fields as $field) { // check to make sure the field exists in the $_POST array // and that the value is not empty if(isset($_POST[$field]) && trim($_POST[$field]) != '') { // populate the $db array $db['fields'][] = $field; $db['values'][] = make_safe($_POST[$field]); } } if(is_array($db)) { /// generate our query! $sql = "INSERT INTO `$dbname.clients` (`".implode('`, `', $db['fields']).') VALUES ('.implode(', ', $db['values']).')'; mysql_query($sql) or die(mysql_error() .": $sql"); } } mysql_close($conn); ?>
-
[SOLVED] mysql_real_escape_string - where to use?
wildteen88 replied to damianjames's topic in PHP Coding Help
mysql_real_escape_string should only be used when inserting data into your database. you dont use mysql_real_escape_string for data coming out of the database. -
[SOLVED] Retain a carriage return from a text box.
wildteen88 replied to jeff5656's topic in PHP Coding Help
Haha! #3 Look into using nl2br echo nl2br($row['problist']); all newlines are stored in the database. Its the web browser that ignores the new lines. -
PHP uses the built in serialize/unserialize functions when writing/reading data to the session file.
-
Open Apaches httpd.conf file and add the following line at the bottom: ServerName localhost Save the httpd.conf and restart Apache. The error should now disappear.
-
Best way to limit records by way of 30 per page etc.
wildteen88 replied to johnsmith153's topic in PHP Coding Help
Look into pagination tutorials. -
A long winded version: $text = 'Hello World! Good: [img=http://www.google.co.uk/images/firefox/sprite.png] Bad: [img=http://www.google.co.uk/images/firefox/sprite.php]'; $text = preg_replace_callback('#\[img\](.+?)\[/img\]#s', 'parse_image', $text); function parse_image($data) { $trusted_ext = array('gif', 'jpg', 'jpeg', 'bmp', 'png'); $info = pathinfo($data[1]); if(in_array($info['extension'], $trusted_ext)) { return '<img src="'.$data[1].'" />'; } // this could be just "return false;" return '<span style="color:red"><b>REMOVED</b></span>'; } echo nl2br($text);
-
This is really annoying me now! PHP array from file PLEASE HELP!
wildteen88 replied to eits's topic in PHP Coding Help
Already been suggested and didn't work http://www.phpfreaks.com/forums/index.php/topic,225111.msg1037215.html#msg1037215 -
This is really annoying me now! PHP array from file PLEASE HELP!
wildteen88 replied to eits's topic in PHP Coding Help
That wont work! Use function checkcard($newcard) { $dealtcards = file("file.crd"); $dealtcards = array_map('trim', $dealtcards); // remove the new lines. if (in_array($newcard, $dealtcards)) { return true; } } -
You are setting the $this->meta variable in correctly. Here if ( !empty($iptc['2#110'][0]) ) $meta['credit'] = utf8_encode(trim($iptc['2#110'][0])); elseif ( !empty($iptc['2#080'][0]) ) $meta['credit'] = utf8_encode(trim($iptc['2#080'][0])); if ( !empty($iptc['2#055'][0]) and !empty($iptc['2#060'][0]) ) $meta['created_timestamp'] = strtotime($iptc['2#055'][0] . ' ' . $iptc['2#060'][0]); if ( !empty($iptc['2#120'][0]) ) // caption $meta['caption'] = utf8_encode(trim($iptc['2#120'][0])); if ( !empty($iptc['2#116'][0]) ) // copyright $meta['copyright'] = utf8_encode(trim($iptc['2#116'][0])); if ( !empty($iptc['2#005'][0]) ) // title $meta['title'] = utf8_encode(trim($iptc['2#005'][0])); } You're populating your $meta array. $this->meta and $meta are not the same. You'll need to change all instances of $meta to $this->meta or add the following line after the above block of code $this->meta = $meta;
-
[SOLVED] Global function, echoing lots of Variables?
wildteen88 replied to Mutley's topic in PHP Coding Help
Beaten to it .= is the concatenation assignment operator Examples // assignment operator $var1 = 'one'; $var1 = 'two'; echo $var1 // ouputs: two // concatenation assignment operator $var1 .= 'one'; $var1 .= 'two'; echo $var1 // ouputs: onetwo -
You do not have a closing brace for this if: if ($ok == "1") { However looking at your script. This portion of code $allowed_ext = "doc, pdf, png, jpg"; ... $extension = pathinfo($_FILES['file']['name']); $extension = $extension[extension]; $allowed_paths = explode(", ", $allowed_ext); for($i = 0; $i < count($allowed_paths); $i++) { if ($allowed_paths[$i] == "$extension") { $ok = "1"; } } if ($ok == "1") { can be changed to $allowed_ext = array("doc", "pdf", "png", "jpg"); ... $img = pathinfo($_FILES['file']['name']); if (in_array($img['extension'], $allowed_ext)) Which is much cleaner. Everything put together: <?php $uploaddir = "fileupz"; $allowed_ext = array("doc", "pdf", "png", "jpg"); $max_size = "460800"; // 450kb $max_height = ""; //if intent on uploading images hxw.. $max_width = ""; $img = pathinfo($_FILES['file']['name']); if (in_array($img['extension'], $allowed_ext)) { if($_FILES['file']['size'] > $max_size) { print "File size is too big!"; exit; } elseif($_FILES['file']['size'] <= "0") { print "File size is too Small 0kb!"; exit; } if ($max_width && $max_height) { list($width, $height, $type, $w) = getimagesize($_FILES['file']['tmp_name']); if($width > $max_width || $height > $max_height) { print "File height and/or width are too big!"; exit; } } if(is_uploaded_file($_FILES['file']['tmp_name'])) { $new_filename = microtime(true) . getmypid() . $_FILES['file']['name']; move_uploaded_file($_FILES['file']['tmp_name'],$uploaddir.'/'.$new_filename); print "Your file has been uploaded successfully! Yay!<br />" . $new_filename; } else { print "Incorrect file extension!"; } } ?>
-
That code should work fine. This is the test data I used $calc[] = 99999999; $calc[] = 28931; $calc[] = 21389183981; The output of your script is ["99 999 999","28 931","21 389 183 981"] Nothing wrong there
-
What are you using to connect locally/externally. Also post all errors in full here
-
Use if(is_uploaded_file($_FILES['file']['tmp_name'])) { $new_filename = (microtime(true) . getmypid()) . $_FILES['file']['name']; move_uploaded_file($_FILES['file']['tmp_name'],$uploaddir.'/'.$new_filename); print "Your file has been uploaded successfully! Yay!<br />" . $new_filename; } else { print "Incorrect file extension!"; }
-
phpinfo
-
Yeah had a typo fixed it.
-
If you array is a single dimension and it only contains numbers -- which you want to format then use array_walk eg $numbers = array(1, 12.06593, 1253689, .0669); echo '<pre>' . print_r($numbers, true) . '</pre>'; array_walk($numbers, 'format_number_array'); echo '<p>FORMATTED:<pre>' . print_r($numbers, true) . '</pre>'; function format_number_array(&$item) { $item = number_format($item, 0, ',', ' '); }
-
Yes PHP supports two image libraries GD and ImageMagic
-
[SOLVED] Extensions not working?
wildteen88 replied to Strahan's topic in PHP Installation and Configuration
When installing PHP extensions ensure they are of the same version as the version of PHP (php.exe) installed. Extensions for PHP5.2.5 (or lower) are not compatible with PHP5.2.6. I recommend you to go to php.net and download the "PHP 5.2.6 zip package". Once downloaded, open the .zip and extract the files withiin the ext/ folder to wherever your PHP ext/ folder is to. -
[SOLVED] Debugging: stop if variable undefined
wildteen88 replied to KenDRhyD's topic in PHP Coding Help
This is handled by E_ALL for me. -
Change if ($color == "FBFBF7") { $color = "FFFFFF"; } else { $color = "FBFBF7"; } to $color = (isset($color) && $color == "FBFBF7") ? "FFFFFF" : "FBFBF7";
-
Its should still be fixed. EDIT: <?php $botnick = "Chatterup"; $server = "SERVER"; $port = "6667"; $channel = ""; ?> <html> <head> <meta http-equiv="Content-Language" content="en"> <meta name="GENERATOR" content="Microsoft FrontPage 5.0"> <meta name="ProgId" content="FrontPage.Editor.Document"> <meta http-equiv="Content-Type" content="text/html; charset=windows-1252"> <title>Room List</title> </head> <body> <table width="98%" border="0" align="center" cellpadding="0" cellspacing="0" id="AutoNumber1" style="border-collapse: collapse"> <tr> <td width="8%" height="20" align="center" valign="middle" bgcolor="#FFFFCC" style="border-left:1px solid #333333;border-bottom:1px solid;border-top:1px solid #333333;FONT-FAMILY: verdana;FONT-SIZE: 8pt;TEXT-DECORATION: none;"> Users</td> <td width="15%" height="20" align="left" valign="middle" bgcolor="#FFFFCC" style="border-bottom:1px solid;border-top:1px solid #333333;FONT-FAMILY: verdana;FONT-SIZE: 8pt;TEXT-DECORATION: none;"> Roomname:</td> <td width="77%" height="20" align="left" valign="middle" bgcolor="#FFFFCC" style="border-right:1px solid #333333;border-top:1px solid #333333;FONT-FAMILY: verdana;FONT-SIZE: 8pt;TEXT-DECORATION: none;"> Topic:</td> </tr> <?php $fp = fsockopen($server, $port, $errno, $errstr, 30); if (!$fp) { echo "$errstr ($errno)<br />\n"; } else { fputs($fp,"USER $botnick $botnick 127.0.0.1 :php\n"); $nick = $botnick . rand(10000,99999); fputs($fp,"NICK $nick\n"); while (!feof($fp)) { usleep(50); $fget = @fgets($fp, 128); //echo "$fget <br>\n"; $match = explode(" ", $fget); $fget = ereg_replace ("\n", "", $fget); $fget = ereg_replace ("\r", "", $fget); if (isset($match[0]) && $match[0] == "PING") { fputs($fp, "PONG :" . $match[1]); } elseif(isset($match[1])) { if ($match[1] == "001") { fputs($fp,"LIST\n"); } else if ($match[1] == "433") { $nick = $botnick . rand(10000,99999); fputs($fp,"NICK $nick\n"); } else if ($match[1] == "323") { fputs($fp, "QUIT :bye!\n"); break; } else if ($match[1] == "322") { $match[3] = ereg_replace ("#", "", $match[3]); if (eregi ("$channel(.*)", $match[3], $chan)) { $topic = explode(":", $fget); unset($topic[0]); unset($topic[1]); $topic = implode(":", $topic); if ($color == "FBFBF7") { $color = "FFFFFF"; } else { $color = "FBFBF7"; } ?> <tr> <td width="8%" align="center" valign="middle" bgcolor="#<?php echo $color; ?>" style="border-top:1px solid #333333;border-left:1px solid #333333;border-bottom:1px solid #333333;FONT-FAMILY: verdana;FONT-SIZE: 8pt;TEXT-DECORATION: none;"><?php echo $match[4]; ?></td> <td width="15%" style="border-bottom:1px solid #333333;bordertop:1px solid #333333;border-top:1px solid #333333;FONT-FAMILY: verdana;FONT-SIZE: 8pt;TEXT-DECORATION: none;" bgcolor="#<?php echo $color; ?>"> <a href="./chat.php?action=Chat&rmname=[EN]<?php echo $chan[1]; ?>"> <?php echo $chan[1]; ?> </a></td> <td width="77%" style="border-right:1px solid #333333;border-bottom:1px solid #333333;FONT-FAMILY:;border-top:1px solid #333333; verdana;FONT-SIZE: 8pt;TEXT-DECORATION: none;"bgcolor="#<?php echo $color; ?>"> <?php echo $topic; ?></td> </tr> <?php } } } } fclose($fp); } ?> </table> </body> </html>
-
[SOLVED] Debugging: stop if variable undefined
wildteen88 replied to KenDRhyD's topic in PHP Coding Help
For errors to be displayed during runtime you'll also need to to make display_errors is enabled. You can set this within your php.ini display_errors = On Or via ini_set in your scripts error_reporting(E_ALL); ini_set('display_errors', 'On');