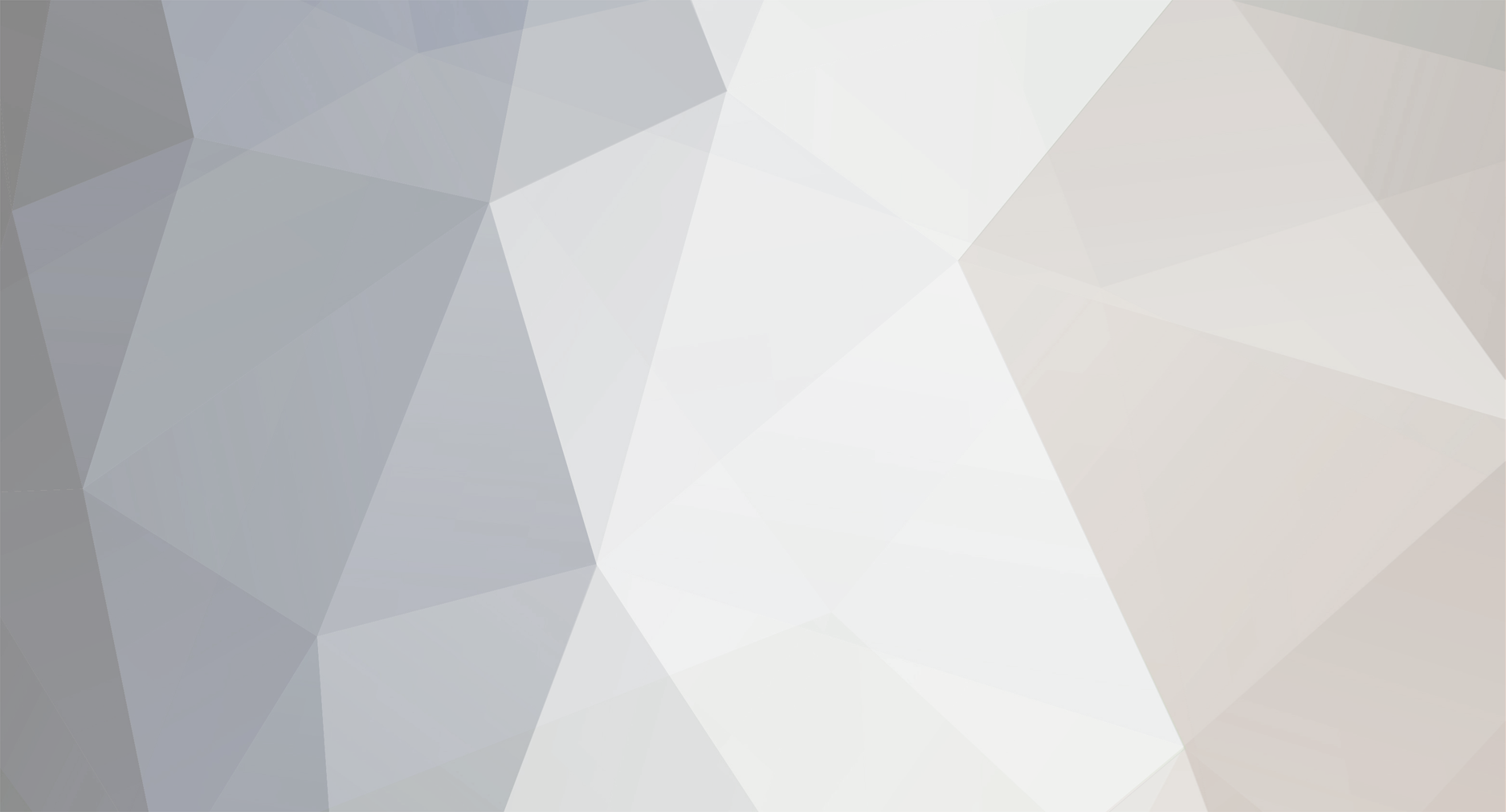
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
change <?php //Use a switch statement to go through the URLS. switch ($nav) { case "login": include ('login.php'); break; case "logout": include ('logout.php'); break; case "editprofile": include ('editprofile.php'); break; case "memberlist": include ('memberlist.php'); break; case "register": include ('register.php'); break; case "viewterms"; include ('terms.php'); break; case "forgot"; include ('reset_pw.php'); break; case "profile"; include ('profile.php'); break; case "admin"; include ('admin/index.php'); break; case "theme"; include ('admin/theme.php'); break; case "news"; include ('admin/news.php'); break; case "general"; include ('admin/general.php'); break; case "download"; include ('download.php'); break; case "game"; include ('game.php'); break; case "tutorial"; include ('tutorial.php'); break; case "contact"; include ('contact.php'); break; default: include ('default.php'); break; } ?> to <?php $pages = array( 'login' => 'login.php', 'logout' => 'logout.php', 'editprofile' => 'editprofile.php', 'memberlist' => 'memberlist.php', 'register' => 'register.php', 'viewterms' => 'terms.php', 'forgot' => 'reset_pw.php', 'profile' => 'profile.php', 'admin' => 'admin/index.php', 'theme' => 'admin/theme.php', 'news' => 'admin/news.php', 'general' => 'admin/general.php', 'download' => 'download.php', 'game' => 'game.php', 'tutorial' => 'tutorial.php', 'contact' => 'contact.php'); if(isset($pages[$nav])) { include './'.$pages[$nav]; } else include './default.php'; ?>
-
Seems the top of index.php should be: <?php /* iSuS - iScripting.net User System v1.0 made by Panzer http://www.iScripting.net Copyright must stay intact */ // Get the configuration file $filename = 'defs.php'; $setup = 'install/index.php'; if (!file_exists($filename)) { die("Please install Isus beafore usage! <a href=\"install/\">Click here</A>"); } if (file_exists($setup)) { die("Please remove the install folder beafore usage. It can be harmful to your site if it exists and someone uses it!"); } include ('config.php'); // get the theme $result = mysql_query("SELECT theme FROM theme WHERE id=1"); $theme = mysql_result($result, 0); //Set the navigation up, secure it's input. $nav = $_GET['action']; secure($nav); ?> rest of your code here
-
Something like <?php $status = array('New', 'Work in Progress', 'Waiting customer reply', 'Updated', 'Work in Progress', 'Updated', 'Work in Progress', 'Waiting customer reply', 'Closed'); $time = array(0.08, 138.75, 25.5, 45.72, 8.13, 0.27, 2.01, 19.12, 215.38); foreach($status as $key => $value) { if(isset($total[$value])) $total[$value] += $time[$key]; else $total[$value] = $time[$key]; } echo '<pre>' . print_r($total, true) . '</pre>'; ?>
-
No, the action is handled by the module. admin.php just includes the requested module. This first part of the code: if(isset($_GET['module']) && file_exists('./'.$_GET['module'].'.php') { // include the module eg news.php include $_GET['module'] . '.php'; } Goes in admin.php. This just includes the module, eg news.php, photo.php etc The second part: if(isset($_GET['action'])) { switch($_GET['action'])) { /* possible actions for module */ case 'add': /* code to add*/ break; case 'edit': /* code to edit*/ break; case 'del': /* code to delete*/ break; default: die('Invalid Command'); break; } Goes in your module eg news.php, photo.php Example setup now attached [attachment deleted by admin]
-
Dont create seperate pages like addnews.php editnews.php delnews.php merge them all it on to one file - news.php then use urls like admin.php?module=news&action=add admin.php?module=news&action=edit admin.php?module=news&action=delete to do whatever you want. Here is how you implement it: In admin.php you'd do something like if(isset($_GET['module']) && file_exists('./'.$_GET['module'].'.php') { // include the module eg news.php include $_GET['module'] . '.php'; } Now in your modules you'll use this base code if(isset($_GET['action'])) { switch($_GET['action'])) { /* possible actions for module */ case 'add': /* code to add*/ break; case 'edit': /* code to edit*/ break; case 'del': /* code to delete*/ break; default: die('Invalid Command'); break; }
-
Are you separating IP addresses by a space within your downloads.log? You'll be better of logging each separate IP on a new line. Then you can just use the following code: $log_file = 'downloads.log'; // file() returns an array of lines $ip_log = file($log_file); // remove new lines $ip_log = array_map('trim', $ip_log); // check to see if the users ip is in the $ip_log array if(in_array($_SERVER['REMOTE_ADDR'], $ip_log)) { echo 'Downloaded'; } else { echo 'Not downloaded'; }
-
The code from admin/theme.php is irrelevant. We need to see the code from index.php
-
PHP SQLite ext. loaded, but not working!!
wildteen88 replied to melkisedek's topic in PHP Installation and Configuration
I believe the sqlite_* based functions are for SQLite 2 only. The SQLite3 extension has its own function set. -
You're not concatenating your string properly. change $theme to .$theme. (including the dots) The period is the concatenation operator.
-
by using the i pattern modifier.
-
PHP can retrieve the users local time by their timezone.
-
EDIT: Beaten to it This line is the issue: if ($rows['check'] = yes) { Use the comparion operator (==) not the assignment operator (=) Also I advise you to avoid using short tags (<? ?> or <?= ?>). Using short tags makes your code less portable as not all PHP installations have short tags enabled.
-
I'd change your code to update.php <?php session_start(); // all errors get reported to the errors session array $_SESSION['errors'] = null; // reset the errors array if(!isset($_SESSION['user'])) { header("Location: login.php"); exit; } $username = $_SESSION['user']; include 'mysql.php'; // check that the password exists if(isset($_POST['password']) && !empty($_POST['password'])) { // validate password length if(strlen($_POST['password']) < { $_SESSION['error']['password'] = "Password too short"; } } else { $_SESSION['error']['password'] = "Password required"; } // check that the email exists if(!isset($_POST['email']) || (isset($_POST['email']) && empty($_POST['email']))) { $_SESSION['error']['email'] = "Nickname required"; } // check that the email exists if(!isset($_POST['nickname']) || (isset($_POST['nickname']) && empty($_POST['nickname']))) { $_SESSION['error']['nickname'] = "Nickname required"; } // check that no errors have been set if(isset($_SESSION['errors']) && !is_array($_SESSION['errors'])) { // no errors set update profile $sql = "UPDATE regusers SET password ='".$_POST['password']."', email ='".$_POST['email']."', nickname ='".$_POST['nickname']."' WHERE username = '" . $username . "'"; $result = mysql_query($sql); if($result) $_SESSION['updated'] = true; } else $_SESSION['updated'] = false; // redirectt to profile.php header("Location: profile.php"); ?> profile.php <?php session_start(); if(!isset($_SESSION['user'])) { header("Location: login.php"); } $username = $_SESSION['user']; mysql_connect("localhost", "root", "") or die(mysql_error()); mysql_select_db("<db>") or die(mysql_error()); $result = mysql_query("SELECT * FROM regusers WHERE username='$username'") or die(mysql_error()); $row = mysql_fetch_array( $result ); ?> <html part> <form action="update.php" method="POST"> <?php // check that the profile updated and that no errors exists if(isset($_SESSION['updated'])) { if(isset($_SESSION['errors']) && is_array($_SESSION['errors']) && ($_SESSION['updated'] == false)) { echo 'Unable to update profile due to: '; echo '<ul><li>' . implode('</li><li>', $_SESSION['errors']) . '</li></ul>'; } else { echo '<b>Profile Updated</b>'; } } ?> </html>
-
You may need to set the appropriate write permissions for the theme/ directory, eg CHMOD 755 should do the trick.
-
[SOLVED] 3 coloumn layout, I thought it would be simple ^^
wildteen88 replied to sean14592's topic in CSS Help
As your main columns is fixed width of 700px why dont you merge your two (left and right) background images in to one then just apply that as the background for the main column. Also try to avoid using absolute/relatively positioning items on your page. -
You'll need to make sure the mod_vhost_alias module is enabled in order for that command to work.
-
Dont absolutely or relatively position your divs. Look in to other methods such as floats. What type of layout are you trying to achive?
-
Variables do not get parsed within strings which start with single quotes. You should do echo ' <img src="'.$theme_dir.'/'.$theme.'/i/start.png" alt="start" /> <a href="index.php"><img src="'.$theme_dir.'/'.$theme.'/i/home.png" alt="home" border="0" /></a> <img src="'.$theme_dir.'/'.$theme.'/i/spacer.png" alt="spacer" /> <a href="index.php?action=download"><img src="'.$theme_dir.'/'.$theme.'/i/download.png" alt="downloads" border="0" /></a> <img src="'.$theme_dir.'/'.$theme.'/i/spacer.png" alt="spacer" /> <a href="index.php?action=game"><img src="'.$theme_dir.'/'.$theme.'/i/game.png" alt="game" border="0" /></a> <img src="'.$theme_dir.'/'.$theme.'/i/spacer.png" alt="spacer" /> <a href="index.php?action=tutorial"><img src="'.$theme_dir.'/'.$theme.'/i/tutorial.png" alt="tutorials" border="0" /></a> <img src="'.$theme_dir.'/'.$theme.'/i/spacer.png" alt="spacer" /> <a href="http://forum.plasmasteelgames.net"><img src="'.$theme_dir.'/'.$theme.'/i/forum.png" alt="forum" border="0" /></a> <img src="'.$theme_dir.'/'.$theme.'/i/spacer.png" alt="spacer" /> <a href="index.php?action=contact"><img src="'.$theme_dir.'/'.$theme.'/i/contact.png" alt="contact" border="0" /></a> <img src="'.$theme_dir.'/'.$theme.'/i/end.png" alt="send" />';
-
Change $theme_dir = $_SERVER['DOCUMENT_ROOT'] . '/theme'; to $theme_dir = '/home/a5880517/public_html/isus/theme'; $_SERVER['DOCUMENT_ROOT'] should return the path to where you should upload files to. Seems your host has screwed something up. You'll have to hard code the full path instead.
-
Do you mean apply rounded corners to item on your webpage? If so this is not handled by PHP but mainly JavaScript. Many Javascript frameworks allow you to apply rounded corners to items on your page. One such framework is JQuery
-
You spelt VirtualHost as VirutalHost (u in wrong place)
-
Why dont you enable display_errors within your php.ini that way you wont get a blank page when an error occurs.
-
Try using an absolute path instead. <?php /* iSuS User System Theme Mod Developed by: plasmagames(http://plasmasteelgames.net) member of iScripting(http://iscripting.net/smf) DO NOT REMOVE THIS */ //If the form hasn't been submitted, show it! if (!$_POST['submit']) { echo "<div class=\"reg\"><div class=\"point\"><img src=\"i/to.png\" alt=\"arrow\"><STRONG>Themes<STRONG></div><form action='index.php?action=theme' method='POST'> <p><strong>Theme:</strong><input class=\"field\" type='text' style width='300' height='500' name='theme' /></p> <input class='submit' type='submit' name='submit' value='Change Themes' /> </form></div> "; } else { //Or else it has.. Secure all the inputs $theme = secure($_POST['theme']); //find the old theme: $select = mysql_query("SELECT * FROM theme WHERE id=1"); //u need a WHERE clause or it won't work and it has to be valid. $row = mysql_fetch_array($select) or die(mysql_error()); //call it $oldtheme $oldtheme = $row['theme']; $theme_dir = $_SERVER['DOCUMENT_ROOT'] . '/theme'; if(file_exists($theme_dir . "/$oldtheme")) { //Insert the user information into the database $update = "UPDATE theme SET theme = '$theme' WHERE id = 1"; //u need a WHERE clause or it won't work and it has to be valid. $rename = rename("$theme_dir/$oldtheme", "$theme_dir/$theme"); //If the update and rename went ok... if (mysql_query($update) && $rename==true) { //Tell them the good news echo "The theme directory was changed to <br /> <strong>$theme</strong><br />"; } else { echo "Error, the theme directory wasn't changed."; } } else { echo "The '$oldtheme' does not exist in $theme_dir"; } } ?>
-
That code looks likes it been generated by Dreamweaver. I do not recommend any PHP code generated by Dreamweaver. You're better of coding it yourself, that you know what the code does/should do plus it will much more readable.
-
Topic solved can be configured so when a topic is solved it'll either change the Post Title, Post Icon or Both. EDIT: This is due to a different template is being used for the Updated Topics page. From what I can tell its handled by Recent.templete.php