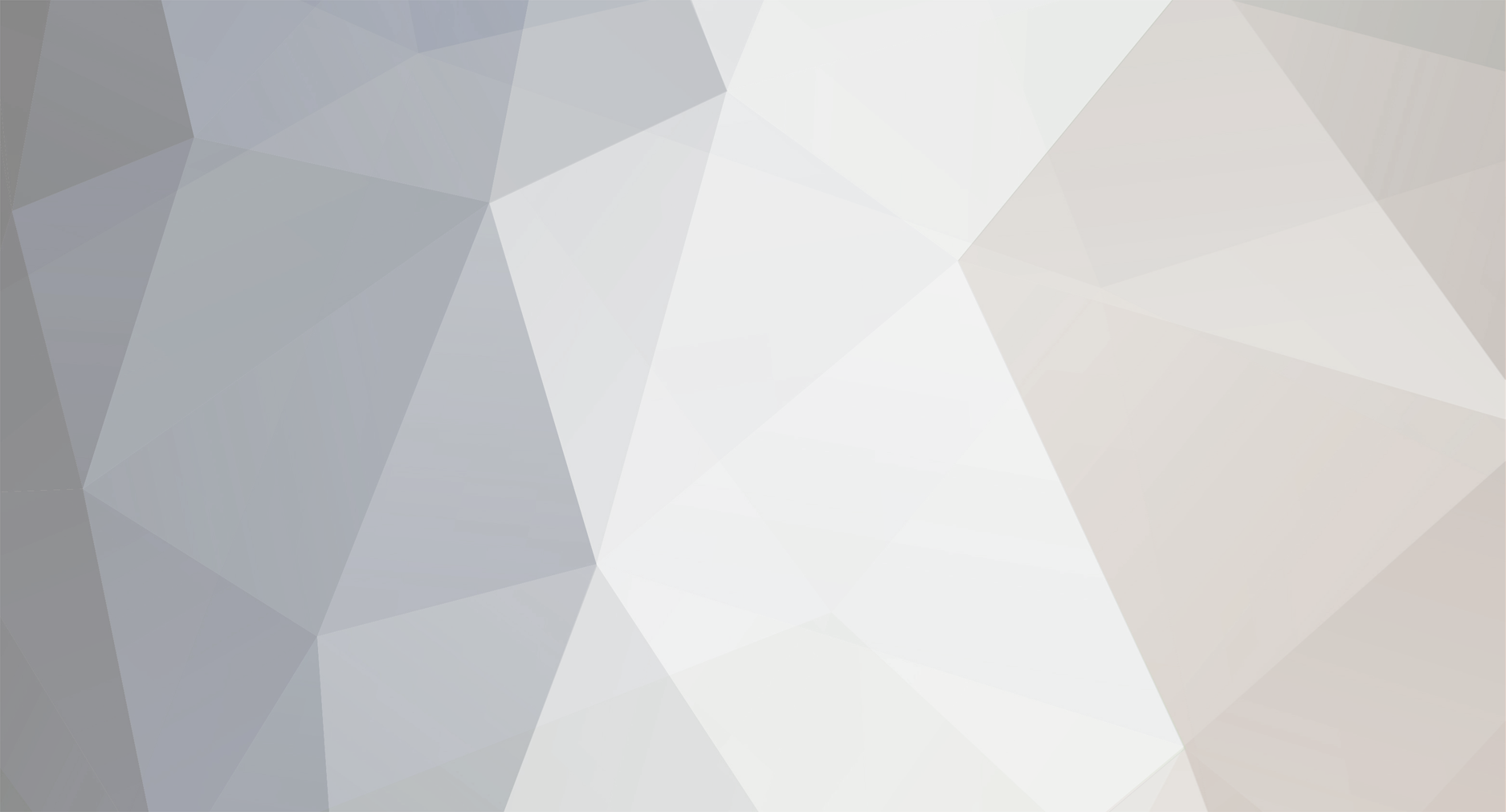
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
Don't forget ternary operators return values. So you could assign a variable a ternary operator, eg instead of doing this ($_SESSION['logged_in'] == 1) ? $this->output = 1 : $this->output = 0; You can do just this: $this->output = ($_SESSION['logged_in'] == 1) ? 1 : 0;
-
When you use header you redirect the user to sheets.php. All POST/GET data from the page you was redirected from will be lost. You'll probably want to use include instead. Also you may aswell use a basic if statement if you want the user to go to sheets.php if $_POST['login'] or $_POST['go'] exists: if(isset($_POST['login']) || isset($_POST['go'])) { include 'sheets.php'; } else { // code here to be executed if $_POST['login'] and $_POST['go'] doesn't exist }
-
The ternary operator is just an if else statement, without the words if/else, instead they are symbols instead. If you understand an if/else statement you'll be able to use a ternary operator. The syntax of a ternary operator is this: (condition) ? true : false The above is the same as: if(condition) { true } else { false } Ternary operators are inline, they cannot execute blocks of code.
-
PhP configuration in Window XP
wildteen88 replied to jackypeh's topic in PHP Installation and Configuration
What is gdbm? I can't seem to find any info about this on php.net. -
[SOLVED] configuration of sessions in php.ini
wildteen88 replied to skein's topic in PHP Coding Help
Where did I say store them in the root folder? I said outside of the root folder. -
[SOLVED] configuration of sessions in php.ini
wildteen88 replied to skein's topic in PHP Coding Help
As long the sessions are stored outside of your websites root folder (the folder where you upload your files too) then your sessions will be "safe". There is no "standard" place to store your sessions files. -
PhP configuration in Window XP
wildteen88 replied to jackypeh's topic in PHP Installation and Configuration
In order to do that you have to recompile PHP. On Windows you don't/should not need to recompile PHP. PHP is already compiled. In order to enable extra libraries you just need to enable either an extension/or an setting within the php.ini. -
[SOLVED] Generating a definition list from 2 arrays
wildteen88 replied to yanisdon's topic in PHP Coding Help
You could of just changed the first foreach loop to this: foreach($dt_data as $k => $dt_val) { $output .= '<dt>'.$dt_val."</dt>\n<dd>" . $dd_data[$k] . "</dd>\n"; } And remove the secound foreach. Rather than rewriting the whole thing. -
Cant connect to mysql????
wildteen88 replied to daboymac's topic in PHP Installation and Configuration
That is to do with mysql. Make sure you have setup the correct user permissions for the lbobmanuel user in mysql. -
Well your configuration works fine here. I'm not sure what the problem might be. Try restoring the httpd.conf to its default settings when Apache was installed, there should be a backup copy of the httpd.conf stored in the conf folder. Look for a file called something like http-default.conf. Rename that backup file to httpd.conf (delete/rename the existing httpd.conf). Restart apache and test a simple html file. If the html file works fine then start by adding in the PHP config lines one by one. Making sure to save the httpd.conf and restart Apache each time.
-
This should not prevent you from accessing localhost (which is an alias to 127.0.0.1). 127.0.0.1 is an internal ip address (or loopback address). My ISP does not condone hosting websites from my computer however I can still use Apache locally. From the problem you are having I'd assume its a configuration issue can you attach your httpd.conf file here.
-
Upgrade PHP5.1.6 to PHP5.2.x. Apache2.2.x is not compatible with PHP5.1.6*. Note: When you upgrade make sure you use the php5apache2_2.dll module (for Windows). Or php5apache2_2.so (for non windows OS). * PHP5.1.6 can be compatible if you download the third party apache2.2.x php module from apachelounge.com Apache2.2.x has changed the way it handles modules. Apache2.0.x modules are no longer compatible with Apache2.2.x
-
That should work. You have modified the correct line. Make sure you have edited the correct httpd.conf file.
-
What version of Apache do you have installed? if its Apache2.2.x then the loadModule line should be: LoadModule php5_module c:/php5/php5apache2_2.dll Make sure you have PHP5.2.x installed in order to get this module. Apache2.2.x changed the way how it handles modules. Apache2.0.x modules are not compatible with Apache2.2.x. php5apache2.dll is for use with Apache 2.0.x
-
Reading extra line in tab-delimited file
wildteen88 replied to ryanbutler's topic in PHP Coding Help
Try: <?php $theFile=fopen("hybrid_spring.txt", "r"); $firstLine=fread($theFile,19); //error check to ensure we can open the text file and get results back, if not, display a //warning. if(!$theFile) { echo "Couldn't open the data file. Try again later."; } else { echo "<table cellspacing=\"2\" cellpadding=\"5\" border=\"1\">\n"; //read the contents of the text file while(!feof($theFile)) { //split the tab delimited file up $data = array_map("trim", explode("\t", fgets($theFile))); if (count($data) == 14) { if($data[5] <= 0 && $data[6] != "LAB" && $data[12] != "Y") { print " <tr class=\"closed\">\n"; } elseif ($data[13] == "Y") { print " <tr class=\"lab\">\n"; } else { print " <tr>\n"; } for ($i=0; $i<13; $i++) { print " <td>$data[$i]</td>\n"; } print " </tr>\n"; } elseif(!empty($data[0])) { echo " <tr>\n <td colspan=\"14\">" . trim($data[0]) . "</td>\n </tr>\n"; } } //done with the file, call the fclose function, passing variable fclose($theFile); echo '</table>'; } ?> -
databases will return back the stored value. Databases wont convert text. This most probably your browser. If you want to see the text & in the browser you need to use &. Your browser will parse and & and display &.
-
Your code wont work as you always have to set the min number. Try: function getmin($nums) { // set min number to first number in the array $min = $nums[0]; // loop through the array foreach($nums as $num) { // check that the number is less than curret min number if($num <= $min) { // set min to current number $min = $num; } } // return minimum number return $min; } $arr = array(25, 60, 8, 1, 22); echo getmin($arr);
-
The server has be configured to parse PHP scripts with the PHP interpreter. You cannot "force" an non-configured server to parse PHP code.
-
If you want to get the smallest value within an array use min. You can also use max to get the biggest number in the array.
-
[SOLVED] Only Using Unique Values in "for()"
wildteen88 replied to JSHINER's topic in PHP Coding Help
Abit like this: <?php $seed = "http://www.site.com/page.html"; $data = file_get_contents($seed); $checked_links = array(); if (preg_match_all("/http:[^\"\s']+/", $data, $links)) { foreach($links[0] as $key => $link) { // check that the link has not been parsed already if(!in_array($link, $checked_links)) { // link has not been parsed we'll add it to the checked links array $checked_links[] = $link; $data_b = file_get_contents('http://www.site.com/sub/'. $link); if (preg_match_all("/http:[^\"\s']+/", $data_b, $links_b)) { @header("Content-type: text/plain"); foreach($links_b[0] as $skey => $sub_link) { // check that the sublink has not been parsed already if(!in_array($sub_link, $checked_links)) { // sublink has not been parsed we'll add it to the checked links array $checked_links[] = $sub_link; echo $sub_link . "\n"; } } } } } } ?> Notice: I changed your for loops to foreach loops. foreach loops are much easier to loop through arrays. -
[SOLVED] Only Using Unique Values in "for()"
wildteen88 replied to JSHINER's topic in PHP Coding Help
Add each link you check into an array, call this array $checked_urls. Then every time you go to parse a url check whether that url has been parsed already using is_array. If its in the array skip to next url, else parse url.