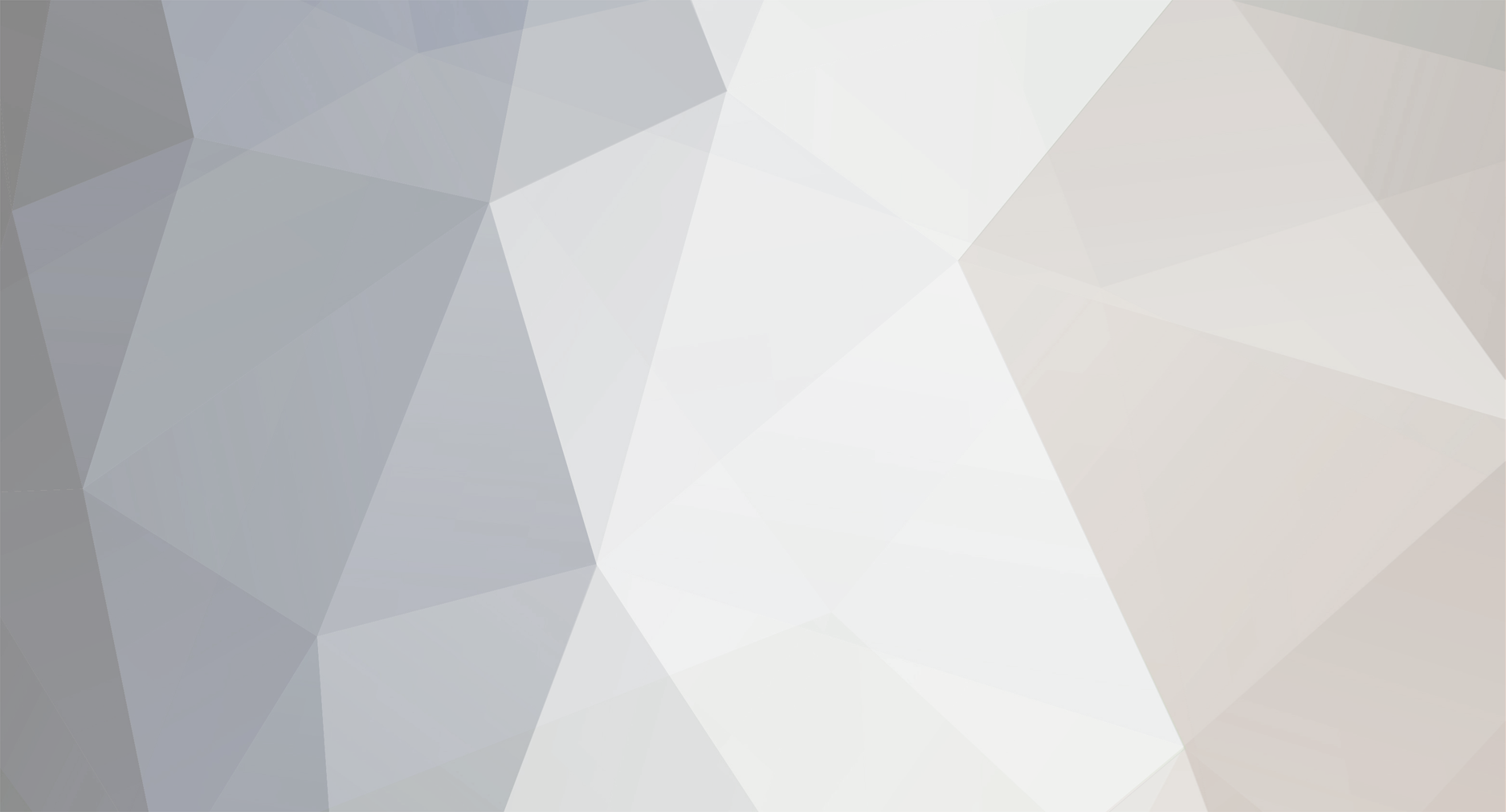
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
No need to change your code completely. The code snippet you provided in your first post is nearly there. try this: <?php $str = "see you soon redarrow"; $words = explode(' ', $str); $bad_words = array("a", "see", "c"); foreach($words as $word) { if(in_array($word, $bad_words)) { echo "'$word' - is a bad word!<br />"; } else { echo "'$word' - is a good word!<br />"; } } ?>
-
Problems with isset() and is_null() on testing server
wildteen88 replied to BigMonkey's topic in PHP Coding Help
Your code seem to be relying on a setting called register_globals to be enabled in order for the code to work correctly. This setting has been disabled by default as it can cause security exploits within your code. When register_globals is disabled you cannot use $(form name here) eg $submit Instead you have use a superglobal array for the type of data you are retrieving. In your case you will want to use the $_POST array variable to fetch post'd data. To get the submit button you'll want to use $_POST['submit'] variable. Here is your new code: <?php if (isset($_POST['submit'])) { ?> <form action="test.php" method="post"> <p> First Name: <input name="firstname" type="text"><br /> Last Name: <input name="lastname" type="text"> <input name="submit" type="submit" value="Send"> </p> </form> <?php } else { echo "You Hit SUBMIT!"; // You mother should be proud! } ?> Also if your production server is running PHP version greater than 4.2.x then you should be able to use the newer superglobal variables when you upgrade your scripts. I would advise you to not reply on register globals when coding scripts and to stick to using superglobals instead. -
<php></php> tags do not even exist. You should use the following as tags: <?php ?>
-
That code is sending an email to the email address provided in the Email field when you fill in the form on the guestbook. It is not being added to your guestbook database - I assume you are using some form a database to store the guestbook entries. If you are using a database you will need to recode that page.
-
If you use escape characters (or whitespace - \n \t \r etc) within single quotes PHP will ignore them. If you use double quotes PHP will parse them eg: \n will give a new line, \t with give a tab. So the code you have provided should be working correctly. Check what your PHP is outputting by viewing the source (right click > View Source). If the output is correct then the problem maybe your javascript.
-
Your code won't write the word NULL into the database table as the art_image variable contains a null value and not the string NULL. You will want to enclose the word NULL within quotes for the art_image variable to hold the world NULL and not the value NULL. If you set the variable as NULL without quotes PHP will store a blank value and so when your code runs it wont write null to the database.
-
All img tags must contain an alt attribute. This is one of the many requirements of XHTML. Also id's can only be used once there cannot be any more than one of the same id used on a page. Either revert duplicated id's to classes or give each id a unique name.
-
As you are using a pre-configured AMP setup it is usually not easy to to upgrade a certain component due to how everything is setup. Normally with these pre-configured AMP setups they tend to place files all over the place. I personally prefer to install Apache, MySQL and PHP manually that way when you want to upgrade a component such as PHP. All you need to do is go to p[hp.net and download the zipped binaries package for the version you want and then extracting the contents of the zip to where PHP is installed - making sure you overwrite all existing files. Job done PHP is now upgraded. However if you are going from PHP4 to PHP5 then you may need to modify Apaches configuration, such as the LoadModule line (if PHP is setup as an Apache Module) or the ScriptAlias line (if PHP is set as CGI). Also if you install AMP manually you'll get the added benefit of how x and y work together such as Apache and PHP or MySQL and PHP etc.
-
[SOLVED] Alright, str_replace is being a b****!
wildteen88 replied to Mathy's topic in PHP Coding Help
Okay so none of the code snippets supplied is not working? If that's the case can you please post your full code here so we can see how you are using the suggested code snippets. Also can everyone quit the insults too. If you are not going to contribute to this thread then don't post at all. -
You should be able to easily upgrade PHP to version 5 easily if download the zipped binaries package from php.net then extract the contents of the zip to where you have installed PHP to - overwriting existing files. Then you will have to reconfigure your webserver. What webserver are you using on Windows 2000? IIS, Apache, etc?
-
[SOLVED] Alright, str_replace is being a b****!
wildteen88 replied to Mathy's topic in PHP Coding Help
Nice, I think you at least know what you're doing. Thanks to everyone else that helped so far, BUT! Can you please give an example to this code? I don't believe I am getting it. Mathy, You'd do this: <?php // get the variables $title = $dat['title']; $creator =$dat['creator']; $subject = $dat['subject']; $forum = $dat['forum']; // You must re-assign $dat its new value in order for // spaces to be changed in to dashes $dat['title'] = str_replace(" ", "-", $title); $dat['creator'] = str_replace(" ", "-", $creator); $dat['subject'] = str_replace(" ", "-", $subject); $dat['forum'] = str_replace(" ", "-", $forum); ?> In the code you supplied earlier all you where doing is creating multiple instances (copies) of $dat and not doing anything with the created variables. -
How I can add GD library support
wildteen88 replied to abdfahim's topic in PHP Installation and Configuration
If you cant find the line then just add the line into the php.ini in the extensions section: extension=php_gd2.dll Before adding it made sure php_gd2.dll is located in PHP's extensions folder. When you haved that line save the php.ini and restart Apache or whatever server software you are using. GD should now be available. -
Always use the full PHP tag syntax (<?php ?>) and not the short hand version (<? ?>). Try: <?php phpinfo() ?> By default PHP has a setting called short_open_tag disabled and thu <? ?> tags do not work. IMO it is best to use the full tag syntax. Also it is important that you restart Apache when you have edited Apache's or even PHP's configuration files (http.conf or php.ini) in order for any changes you have made to take effect.
-
Your regex is incorrect. Your regex is not searching the whole of the string. Currently it only goes as far as the first space - by default regex stops at whitespace (spaces, newlines etc). What you need to tell PHP to do is search the whole entire string from the beginning to the end. You do this by placing the carrot character (^) at the start of the pattern (not within a character class ([]) and then place the dollar sign ($) at the very end of the pattern. Then within the character class you place the characters you wish to allow so letters a-z and digits 0-9 So if you use the the following pattern you code should work as expected: /^[a-zA-Z0-9_]+/$ Your code with new pattern: <?php $_POST['key'] = "some 0255"; if (preg_match("/^[a-zA-Z0-9_]+$/", $_POST['key']) || strlen($_POST['key']) < 2) { echo 'Contains valid characters'; } else { echo 'Contains invalid characters'; } ?>
-
Read Wuhtzu post above, I quote it here read the bit in red:
-
Have a read of this post
-
[SOLVED] Directory Reading Script..Gone Wrong
wildteen88 replied to AbydosGater's topic in PHP Coding Help
When you use is_dir or is_file you will also want to define the path where those files/directory exists. If you don't define the path then it will look in the directory the script is being ran in. It wont check the directory you are reading. Try this: <?php define('DIR', '../Apache/'); $dp = opendir(DIR); $files = array(); $dirs = array(); $unknowns = array(); while (false !== ($file = readdir($dp))) { if (is_file(DIR . $file)) { $files[] = $file; } elseif (is_dir(DIR . $file)) { $dirs[] = $file; } else { $unknowns[] = $file; } } echo '<strong>Files:</strong><br />'; foreach ($files as $file) { echo $file.'<br />'; } echo '<br /><hr /><br />'; echo '<strong>Directorys:</strong><br />'; foreach ($dirs as $dir) { echo $dir.'<br />'; } echo '<br /><hr /><br />'; echo '<strong>Unknown Files:</strong><br />'; foreach ($unknowns as $unkown) { echo $unkown.'<br />'; } echo '<br /><hr /><br />'; ?> -
You cant break out of an if. I don't understand what you mean. Post some code for an example of what you mean.
-
What are you testing? Whats your problem/question? We are not mind readers
-
rather than using echo in the name function use return: function name(){ return 'jay'; }
-
OK jsut remove the else statements: <?php if(isset($_POST['submit'])) { if(isset($_POST['date']) && !empty($_POST['date'])) { echo 'Date is set: - ' . $_POST['date'] . '<br />'; } if(isset($_POST['spent']) && !empty($_POST['spent'])) { echo 'Spent is set: - ' . $_POST['spent'] . '<br />'; } if(isset($_POST['product']) && !empty($_POST['product'])) { echo 'Product is set - ' . $_POST['product'] . '<br />'; } echo '<hr />'; } ?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> Date: <input type="text" name="date" /><br /> Spent: <input type="text" name="spent" /><br /> Product: <input type="text" name="product" /><br /> <input type="submit" name="submit" /> </form>
-
Is this what you mean: <?php if(isset($_POST['submit'])) { if(isset($_POST['date']) && !empty($_POST['date'])) { echo 'Date is set: - ' . $_POST['date'] . '<br />'; } else { echo 'Date is not set!<br />'; } if(isset($_POST['spent']) && !empty($_POST['spent'])) { echo 'Spent is set: - ' . $_POST['spent'] . '<br />'; } else { echo 'Spent is not set!<br />'; } if(isset($_POST['product']) && !empty($_POST['product'])) { echo 'Product is set - ' . $_POST['product'] . '<br />'; } else { echo 'Product is not set!<br />'; } echo '<hr />'; } ?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> Date: <input type="text" name="date" /><br /> Spent: <input type="text" name="spent" /><br /> Product: <input type="text" name="product" /><br /> <input type="submit" name="submit" /> </form>
-
Not really sure what you are asking. Your question is not very clear and your code is not much clearer either. If you could rephase your question along with some visual examples. Then I may be able to help you
-
If you are storing more than 255 chars then use a text data which will allow to store alot of characters (over 65,000 characters) or go for mediumtext or mediumblob which allow to store over 1.5million characters. Varchar is store small amounts of text, such as a persons name, a localtion. It is not designed to store masses of text. Have a look at this cheat sheet to see what data types maximum values
-
for each loop pull information from database
wildteen88 replied to adrian28uk's topic in PHP Coding Help
Ok first of all move your db connection code out side of the foreach. You don't want to make a new connection to the database every time you loop through the foreach loop. Just have the query within the loop. New code: <?php // we moved all the db connection/selection code outside of the foreach // don't wont to connect/select database each time we loop through the foreach include 'dbconn.inc.php'; $dbcnx = mysql_connect($servername, $username, $password) or die('Connection Failed'); mysql_select_db($database) or die('"Unable to locate the shopping basket'); $product_choice = $_POST['choice']; foreach ($product_choice as $numbers) { // Build the query $query = 'SELECT * FROM Products_Options WHERE product_randid=' . $numbers; // Run the query we built above $result = mysql_query($query, $dbcnx) or die (mysql_error()); // check that the query returned only one record if(mysql_num_rows($result) == 1) { // one record has been returned, lets get it. // no need for a while loop here as we are only getting one record // if multiple records are being returned we'd use a while loop $row = mysql_fetch_assoc($result); // display the returned product echo $row['product_option_name']; } } ?>