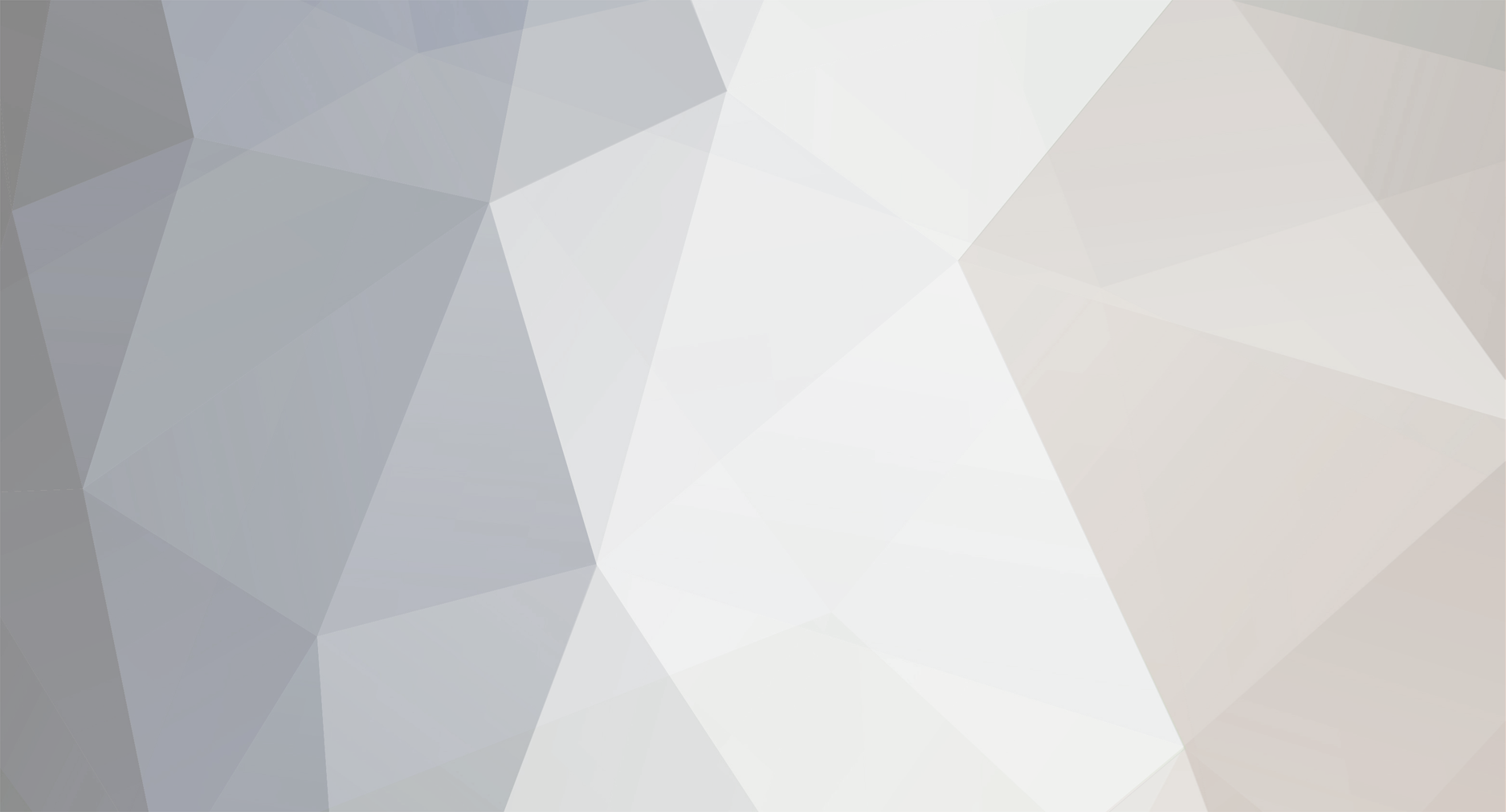
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
[SOLVED] How to do page navigation bar using php codes?
wildteen88 replied to phpRoshan's topic in PHP Coding Help
If you don't want to use javascript then you'll have to use iframes. -
I've got a feeling you may have magic quotes enabled which could be causing this. Change each instance of mysql_real_escape_string($_POST to makeSafe($_POST Now add the following function to your code function makeSafe($value) { if (get_magic_quotes_gpc()) { $value = stripslashes($value); } $value = mysql_real_escape_string($value); return $value; } This will not affect the data that is already within your database. You'll need to loop through the data within your database and apply stripslashes to undo the affects of magic quotes.
-
PHP should not be outputting newlines as literal text \r\n. For some reason newlines are stored like that within your database. You must be doing something with the data when adding the data to the database to cause this. You do not need to doing anything special to have PHP output newlines within text, expect when displaying new lines within the browser you need to use the nl2br function as TeNDoLLA mentioned. For now you will have manually replace each instead of \r\n with a <br /> tag $content = 'hello \r\n world!'; $content = str_replace('\r\n', "\r\n<br />", $content); echo $content; What code are you using to add the data to the database?
-
Have a look at this post for creating multi-column results.
-
How is $subitem constructed? What is the output of echo '<pre>' . print_r($subitem, true) . '</pre>';
-
Image Buttons/Submit not working in IE/Firefox
wildteen88 replied to JasonHarper's topic in PHP Coding Help
When you use an image as the submit button the webbrowsers submit the x and y co-cordinates of the cursor where the user clicked on the button. Doing a print_r on $_POST you'd see this Array ( [submit_x] => 0 [submit_y] => 0 ) If you used a normal submit button (<input type="submit" name="Submit" value="Submit" />) you'd see this outputted Array ( [submit] => submit ) Notice how the name of submit button changes. This is what I meant by checking to see if submit_x or submit_y exists when using an image as the submit button. -
Image Buttons/Submit not working in IE/Firefox
wildteen88 replied to JasonHarper's topic in PHP Coding Help
You need to check if $_POST['Submit_X'] or $_POST['Submit_Y'] exists not $_POST['Submit'] -
use strip_slashes
-
What are you doing there? That query ($check) does not need to be there. However the makeSafe() is necessary to prevent SQL Injection attacks. Now that you have the makeSafe function you can remove these lines $message = htmlentities($_POST['message']); $message2 = htmlentities($_POST['message2']); $message = stripslashes($_POST['message']); $message2 = stripslashes($_POST['message2']); Change your query so it returns the comments in descending order. // Retrieve all the data from the "example" table $result = mysql_query("SELECT * FROM comments ORDER BY id DESC") or die(mysql_error()); I assume you have set up an auto_increment field called id in your comments table?
-
Ooops. I didn't read your code properly earlier change the while loop to while($row = mysql_fetch_assoc( $result )) { $message = $row['message']; $message2 = $row['message2']; // Print out the contents of the entry echo "<div id=\"census41_messages\">"; echo "<div id=\"comments_box\"><div id=\"comment_name\"><p>$message<em>Says: </em></div><div id=\"comment_date\">" . date ("D, M d, Y, g:i a") . "</div><br />$message2</p></div>"; echo "</div>"; }
-
Read file after uploading to website server
wildteen88 replied to jwesneski's topic in PHP Coding Help
This line $str = file_get_contents(home/content/p/o/r/portasabertas/html/upload/$_FILES["file"]["name"]); Should be written as $str = file_get_contents("/home/content/p/o/r/portasabertas/html/upload/" . $_FILES["file"]["name"]); Your file path needs to be enclosed within quotes. -
This topic has been moved to PHP Applications. http://www.phpfreaks.com/forums/index.php?topic=338992.0
-
I cannot see why it is repeating messages/displaying large gaps. What I think is happening is you have a lot of duplicate/blank entries within your comments table. You will want to clear the contents of your comments table now. To clear the contents of the table run the following query in PHPMyAdmin TRUNCATE TABLE comments Then try posting a couple of new comments and see if does it again.
-
Have you tried contacting your host about this issue.
-
This topic has been moved to CSS Help. http://www.phpfreaks.com/forums/index.php?topic=338988.0
-
Yes you can use preg_replace for this. regular-expressions.info is a great place for learning regular expressions.
-
This topic has been moved to PHP Applications. http://www.phpfreaks.com/forums/index.php?topic=339026.0
-
mysql_fetch_* functions return one row at a time. So when your query returns more than one row you need to use a while loop to get all the results. while($row = mysql_fetch_assoc( $result )) { // Print out the contents of the entry echo "<div id=\"census41_messages\">"; echo "Name: ".$row['mesaage']; echo "Message: ".$row['message2']; echo "</div>"; } Now that yo're using a database you no longer need these two lines. // add the newest comment to the start of the file $comments = "<div id=\"comments_box\"><div id=\"comment_name\"><p>$message <em>Says:</em></div><div id=\"comment_date\">" . date ("D, M d, Y, g:i a") . "</div><br />$message2</p></div>"; // this is the new comment to be added $comments .= file_get_contents('messages.txt'); // now we get all the existing comments and append it to the $comments variable. As your code stands now it should be saving and retrieving the posted comments from your database. You now need to validate/sanitize your user input to prevent SQL Injection/XSS attacks. Have a read of this four part guide for writing secure PHP code.
-
Help me please - edituser.php?action=edit
wildteen88 replied to Ptsface12's topic in PHP Coding Help
No, that is not an update query. And update query is formatted like UPDATE table_name SET fieldx='valuey' WHERE some_condition=true -
Help me please - edituser.php?action=edit
wildteen88 replied to Ptsface12's topic in PHP Coding Help
First change your query so it also return the id column $result = mysql_query("SELECT id, username, email FROM users") or die(mysql_error()); To make your edit link change <a href="#" title=""><img SRC="img/icons/icon_edit.png" alt="Edit" /></a> to <a href="edituser.php?action=edit&id=' .$row['id'] .'" title=""><img SRC="img/icons/icon_edit.png" alt="Edit" /></a> -
Help me please - edituser.php?action=edit
wildteen88 replied to Ptsface12's topic in PHP Coding Help
When you outputting your edit link your code will be like this echo '<a href="edituser.php?action=edit&id=' .$row['id'] .'"><img src="edit_button.png" /></a>'; To get the id in edituser.php you'd use $_GET['id'] as I demonstrated in my previous post. -
Only parse the *b* and */b* tags when displaying the comments. Only use htmlspecialchars when you are inserting the comments into table.
-
Help me please - edituser.php?action=edit
wildteen88 replied to Ptsface12's topic in PHP Coding Help
You'll want to pass in the id that is associated to the username when the edit link is clicked, so your edit link will read edituser.php?action=edit&id=id_number_associated_to_user Then in edituser.php you'd fetch the user details from your datebase like $user_id = (int) $_GET['user_id']; $result = mysql_query("SELECT username, email FROM users_table WHERE id=$user_id"); You output the username,email address etc into a form fields so they can be edited. -
Before continuing you'll want to learn how to use MySQL (mysql is the database server that comes with wamp/xampp). The tutorial here will help you to learn the basics of using MySQL with PHP.
-
EDIT: Beaten to it isset only returns TRUE or FALSE. It does not return the value of $_GET['industry']; You need to pass $_GET['industry'] to your function when it is set. You'd write the code like so if(isset($_GET['industry'])) { $companies = fetch_companines($_GET['industry']); } else { $companies = ''; // set companies to an empty string/value }