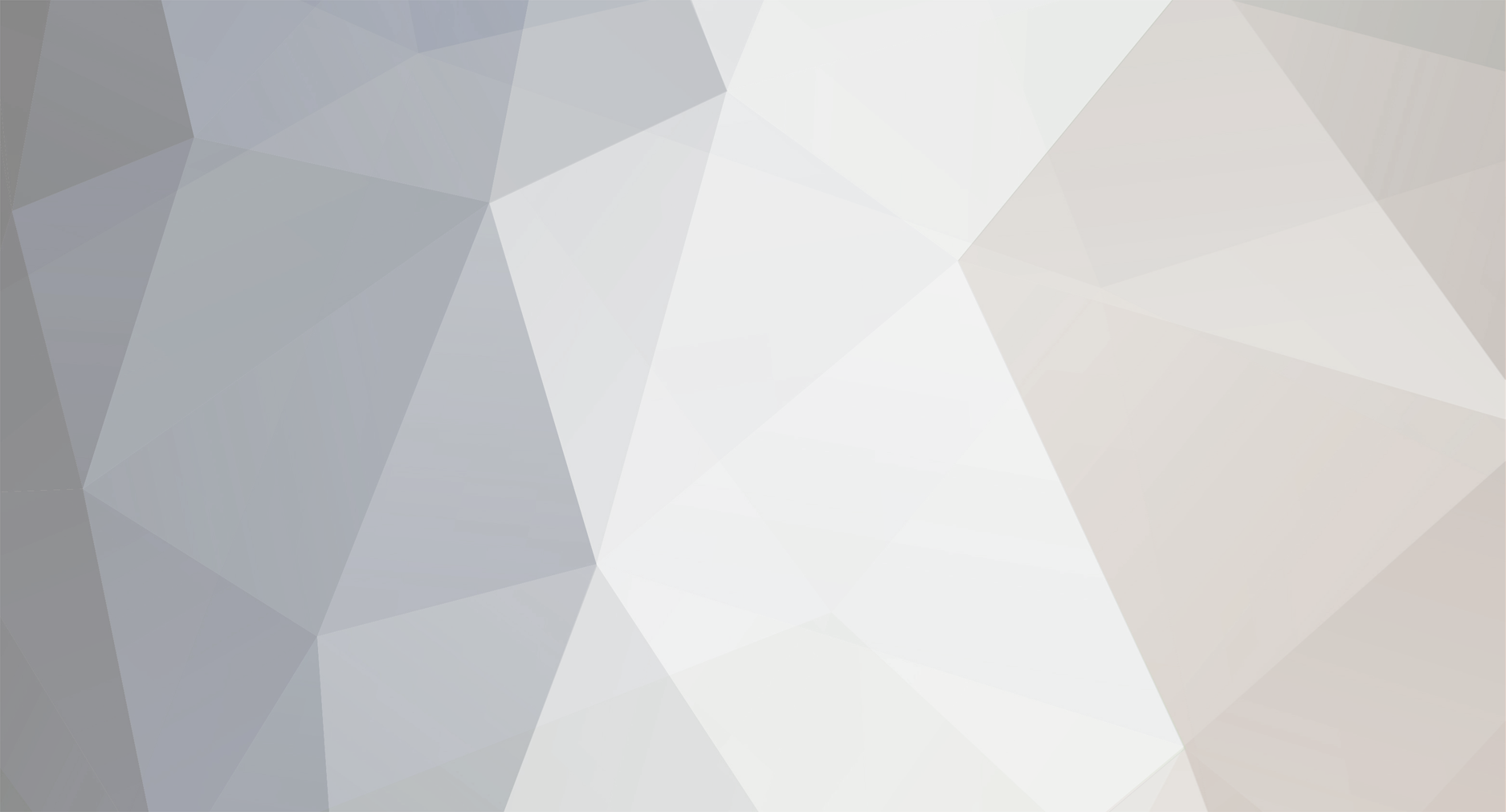
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
Using an Array in a form post--Help please (SOLVED!!!) :)
wildteen88 replied to phencesgirl's topic in PHP Coding Help
This is untested code so it may work. But try this: [code]<?php // if the quantity is being changed for an item if (isset($_POST['update'])) { // get the product's ordered from the cart table $sql = "SELECT * FROM cart WHERE username = '$uid' AND confirmed = 'no'"; $result = mysql_query($sql) or die(mysql_error()); while ($row = mysql_fetch_array($result, MYSQL_ASSOC)) { extract($row); // set the variables foreach ($_POST['pid'] as $pid_key => $pid) { $newquantity = $_POST['newquantity'][$pid_key]; $stock = $row['stock']; // first, check if the user's selected quantity is greater than the stock quantity if ($newquantity > $stock) { $sql = "UPDATE cart SET quantity = '$stock' WHERE username = '$uid' AND confirmed = 'no' AND product_id = '$pid'"; mysql_query($sql) or die(mysql_error()); } else { // put the current newquantity into the cart table $sql = "UPDATE cart SET quantity = '$newquantity' WHERE username = '$uid' AND confirmed = 'no' AND product_id = '$pid'"; mysql_query($sql) or die(mysql_error()); } // end else } // end foreach } // end while } // end if // now let the user view their cart, if it exists. // Select * FROM cart WHERE username == "$uid" $sql = "SELECT * FROM cart WHERE username = '$uid' ORDER BY product_id"; $result = mysql_query($sql) or die(mysql_error()); ?> <form action="/shop/main_shop.php?PGNM=cart" method="post"> <table> <tr> <?php //***make a while loop here that will repeat the table row for each item in the wish table for this user while ($row = mysql_fetch_array($result, MYSQL_ASSOC)) { ?> <td> <input type="hidden" name="pid[]" value="<?php echo $row['product_id']; ?>" /> <input type="text" size="3" name="newquantity[]" maxlength="5"/> </td> <?php } //end while loop ?> <td><input type="submit" name="update" value="Change Qty" /></td> </tr> </table> </form>[/code] -
Using an Array in a form post--Help please (SOLVED!!!) :)
wildteen88 replied to phencesgirl's topic in PHP Coding Help
Then you'll want to do is create seperate fields for each product that user has in thier basket. You give the each form field the same name, but you suffix the name with two square brackets ([]) eg pid[] newquantity[] When you do that the data will be submit as an array. To access product 1 you use $_POST['pid][0] and $_POST['newquantity'][0] to access product 2 you use $_POST['pid][1] and $_POST['newquantity][1] etc. Remeber artrays start at zero. You're best of creatting the form fields dynamically. How do you store each product in the users basket? -
If you wnat to test your PHP scripts without it being hosted on the web then install AMP (Apache PHP and MySQL) by getting either WAMP (for Windows) or XAMPP (for Windows, Mac or Linix) both are free to use. Once installed you can goto http://localhost/ and you'll be able to run your PHP scripts of off your computer. Did you try our suggestions? If you did could you post the updated code here.
-
Using an Array in a form post--Help please (SOLVED!!!) :)
wildteen88 replied to phencesgirl's topic in PHP Coding Help
newquantity is an array, which is $_POST['newquantity']. $_POST is an array of all the POST'd data from the form. Could you define what you mena by an array. DO you want the newquantity text field to hold an array of values, eg 1,3,5,1 each number after the comma is an item within the newquantity array? -
Parse error: parse error, unexpected T_STRING in line 16 (solved)
wildteen88 replied to jrodd32's topic in PHP Coding Help
Post your actuall code here. Not just line 16. Also which example did you use? -
I've had a look but I dont see no use for it. I do have an account for MSN Spaces or MS Live Spaces. I've made an entry and thats it. Hvant used it for like 2 or so years.
-
Parse error: parse error, unexpected T_STRING in line 16 (solved)
wildteen88 replied to jrodd32's topic in PHP Coding Help
You need to escape your quotes within the print statement or use single quotes for the print statement [b]Escaped quotes[/b] [code=php:0]print "<td width=\"30%\" valign=\"top\" align=\"left\" style=\"padding-left:2\" height=\"32\">";[/code] [b]Single Quotes[/b] [code=php:0]print '<td width="30%" valign="top" align="left" style="padding-left:2" height="32">';[/code] You cannot use double quotes within double quotes. You will need to escape the double quotes otherwise PHP will think you have ended the string which you havn't. -
*solved* PHP Variable passing (I should be embarrassed)
wildteen88 replied to laide234's topic in PHP Coding Help
If you are including/requiring scripts then any variables you have set in the script that is including/requiring the files can be using in the included/required script. Eg: [b]test.php[/b] [code=php:0]<?php $varname = 'Hello world'; // include test2.php // we'll be able to use the variable set above in test2.php include 'test2.php'; ?>[/code] [b]test2.php[/b] [code=php:0]<?php // show the value of $varname. // this will use the variable we set in test.php echo $varname; ?>[/code] When you go to test.php you should get Hello World printed to the screen. When using include/require what PHP is affectively doing is basically getting the contents of the required script and pasting it in the location of where the file gets included to as though the code was already there. For example, think of test.php being like this when you run test.php [code=php:0]<?php $varname = 'Hello world'; // include test2.php // we'll be able to use the variable set above in test2.php // show the value of $varname. // this will use the variable we set in test.php echo $varname; ?>[/code] -
Not many people know about this BBCode, but use the nobbc bbcode: [nobbc][nobbc]bbcode [b]you dont[/b] want to be [i]parsed[/i] here[/nobbc][/nobbc] (note this is wrapped in nobbc tags) Actuall result: [nobbc]bbcode [b]you dont[/b] want to be [i]parsed[/i] here[/nobbc] Anyway why you complaining to us. Its not our problem its down to the SMF team. However I do preferer BBCodes parsers that parse BBCode tags in pairs, rather than parsing single BBCode tags.
-
Looks like your server doesnt have register_globals enabled. So you'll need to use the superglobal arrays to access your user input. For example instead of using $PHP_SELF you should use $_SERVER['PHP_SELF'] instead. The same applies ot $joketext you shold use $_POST['joketext'] instead. You'll need to go through your code changing most of the variables to thier superglobal equivalent.
-
[quote author=Gruzin link=topic=107888.msg433334#msg433334 date=1158147727] Offtopic: Fearpig, can you please tell me how did you change the topic title? Thank you. [/quote] It was me that changed the topic title, if you are wondering how *solved* got added into the topic title. I used the inline thread title editor.
-
Prehaps you want to use the mysql_insert_id() function. This function grabs the last generated id by the auto_increment field.
-
The master value is the value set in the php.ini. The local value is usually set within the .htaccess file. Or by an external custom php.ini file. The local value can override the master value.
-
If you are using PHP with Apache2.2.x then thats your problem. PHP isnt compatible with Apache2.2.x. You'll need to download either the latest CVS version of PHP, or download the php5apache2_2.dll from apachelounge.com to get PHP to work with Apache2.2.x A better option would be to downgrade Apache to Apache2.0.x. Your configuration is correct, its the compatiability issue with PHP and Apache2.2.x.
-
In your HTML change [code]<body> <div id="container"> </head> </center> </div></td>[/code] To this: [code]</head> <body> <div id="container">[/code] You'll also want to fix up the HTML issues I suggested too.
-
Use this: [code=php:0]$PartsSearch = $_GET['PartsSearch']; $result = mysql_query("SELECT * FROM tbl_parts WHERE Air_Pressure_Switch='$PartsSearch'", $db); $row = mysql_fetch_array($result); while ($row = mysql_fetch_array($result)) { printf("<b class='Body2'>%s</b><br>", $row["Model"]); }[/code] From looking at your code, you didnt the define the $PartsSearch variable. However thats not the problem. The problem is where you have $_GET['PartsSearch'] on its own. You didnt add a semi-colon after $_GET['PartsSearch']. Thats why your got the parse error.
-
use number format: like this: [code=php:0]$number = '12,589'; // remove any spaces/commas $number = str_replace(array(',', ' '), '', $number); $number2 = number_format($number, 2, '.', ','); echo $number2;[/code] European countries such as france, spain etc use a comma (,) as a decimal seperator rather than a period (.). SO number format is rounding the number. What you should do is remove any commas/spaces from the number. Then pass the number into the format_number function.
-
The other host has a setting called register_globals enabled. You can still use $_GET['variable'] to access the variables from the URL if your other hosts is using a PHP version greater the PHP4.3.
-
Its mainly to do wit your CSS. It is not a good a idea to use positioning when doing a CSS layout, especially when you're trying to center a layout. it is best to use auto margins. The following is your new CSS: [code]body { background-color: #666666; color: #FFF; font-size: 12px; font-family: Verdana, Arial, Helvetica, sans-serif; } a:link, a:visited, a:hover, a:active { color: #FFF; } #container { width: 707px; margin: 0px auto; } #head { width: 707px; } #head img { display: block; } #box1 { width: 345px; } #content { width: 697px; text-aligh: justify; background-color: #2D3855; padding: 5px; } #quote { width: 697px; text-aligh: justify; background-color: #2D3855; padding: 5px; } #foot { width: 707px; font-size: 9px; text-align: center; }[/code] Also you'll want to add to add: [code]<body> <div id="container">[/code] before: [code]</head>[/code] and you'll want to put: [code]</div>[/code] before: [code]</body>[/code] In your HTML. Another thing you'll want to do is clean up your html code by removing the font tags, center tags. Extra line break tags ([nobbc]<br>[/nobbc]) use padding/margins instead rather than adding masess of line breaks to pad out areas etc.
-
Can you post a live example please instead of just code. Its hard to suggest something for you to do, with out seeing the actual problem.
-
No you dont need to chnage anything to the httpd.conf. phpMyAdmin is a PHP script. But what phpMyAdmin is trying to do is include a file called common.lib.php in the libraries folder. Which PHP cannot find and thus results in the the error you are getting. Did try my suggestion by upgrading phpMyAdmin?
-
Using mySql 5.0 functions with PHP
wildteen88 replied to jeva39's topic in PHP Installation and Configuration
The mysql extension is enabled then as you can see the mysql section when you run the phpinfo function. The only problems i see with your code is this: [code]while(mysql_fetch_row($queryexe)) { $id = mysql_result($queryexe, 1); //Puede ser $id= odbc_result($queryexe, "id"); $tema = mysql_result($queryexe, 2); $ritmo = mysql_result($queryexe, 3); $archivo = mysql_result($queryexe, 4); $fecha = mysql_result($queryexe, 5); ?> <tr> <td class="listas" bgcolor="#f7efde"> <?php print ("$tema"); ?></td> <td class="listas" bgcolor="#f7efde"> <?php print ("$ritmo"); ?></td> <td class="listas" bgcolor="#f7efde"> <?php print ("$archivo"); ?></td> <td class="listas" bgcolor="#f7efde"> <?php print ("$fecha"); ?></td> </tr> <?php }?>[/code] Change the highlighted code above to this: [code=php:0]while($row = mysql_fetch_row($queryexe)) { ?> <tr> <td class="listas" bgcolor="#f7efde"><?php print $row['tema']; ?></td> <td class="listas" bgcolor="#f7efde"><?php print $row['ritmo']; ?></td> <td class="listas" bgcolor="#f7efde"><?php print $row['archivo']; ?></td> <td class="listas" bgcolor="#f7efde"><?php print $row['$fecha']; ?></td> </tr> <?php } ?>[/code] Do you get anything now? -
This should do: [code=php:0]$txt = str_replace(array("<br />", "<br>"), "\n", $text);[/code]
-
The problem is you are using single quotes when writring to calendar.php: [code=php:0]fwrite($new_calendar_file, '<?PHP die("You cannot access this file."); ?>\n');[/code] If you use single quotes. PHP will treat \n as-s (meaning normal text). it will not treat \n as a new line character. The solution is to use double quotes: [code=php:0]fwrite($new_calendar_file, "<?PHP die(\"You cannot access this file.\"); ?>\n");[/code]
-
Using mySql 5.0 functions with PHP
wildteen88 replied to jeva39's topic in PHP Installation and Configuration
If mysql functions are not working then the mysql extension hasnt been loaded correctly. Create a new php file called it info.php. now add the following code to the file: [code=php:0]<?php phpinfo(); ?>[/code] Upload to your website, now goto mysite.com/info.php Scroll down the page, do you see a MySQL section at all. To find the MySQL section when running the phpinfo function search for [b]mysql Support[/b] or [b]mysqlI Support[/b]. If you find a section and its the mysqli section you'll need to use the mysql[b]i[/b] functions and not the standard mysql functions, or use the mysql functions if its the standard mysql extension thats enabled. if you find nothing then the mysql extension has been enabled.