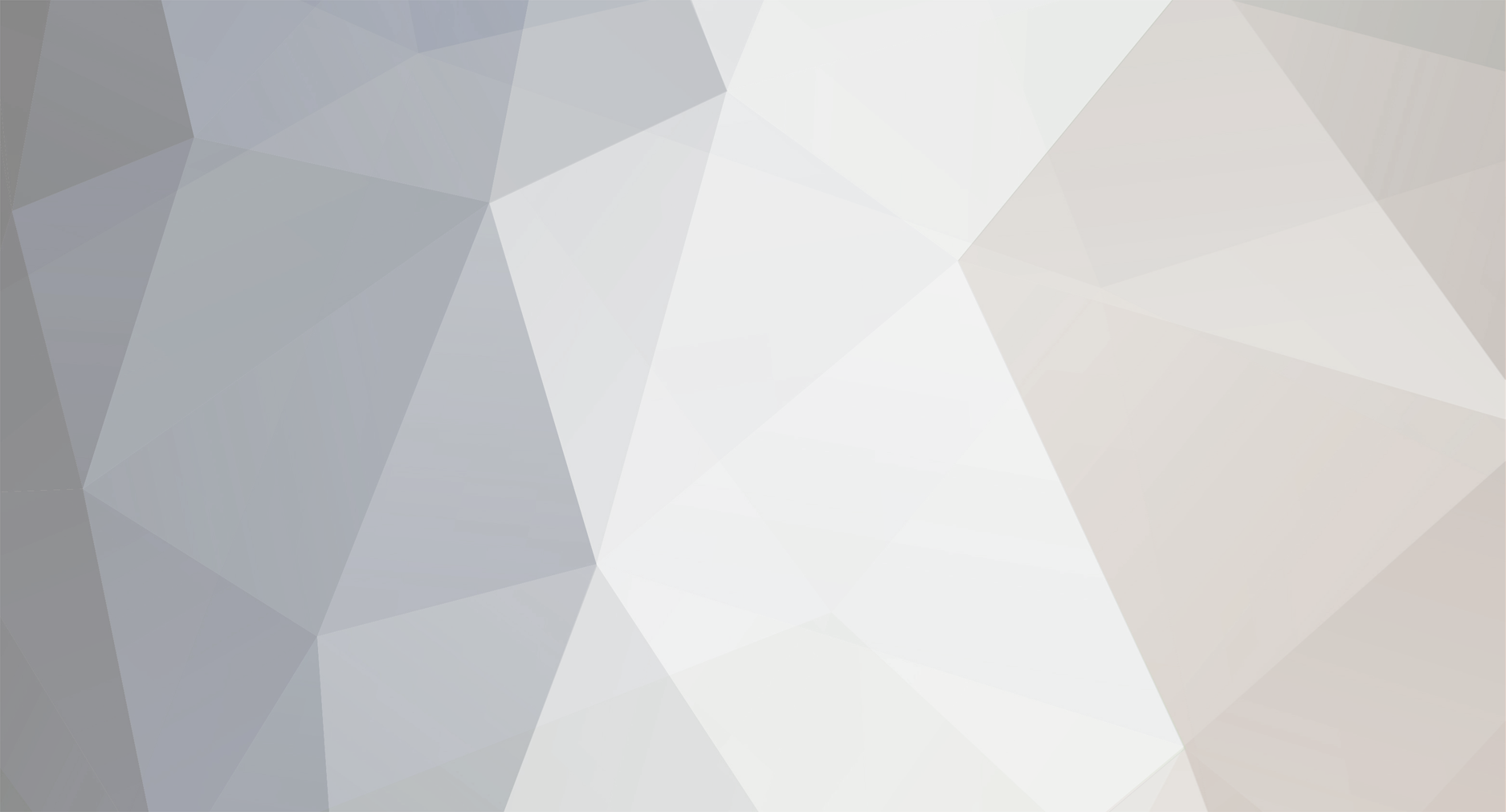
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
When you apply any changes to your php.ini file make sure you are restarting the server in order for the new settings to be applied.
-
Need to turn off Register Globals on shared site
wildteen88 replied to JRS's topic in PHP Coding Help
What register_globals does is extract the variables inside the supergloabls arrays, Superglobal arrays the following variables: $_POST, $_GET, $_SESSION, $_COOKIE etc. Now if you have something like this: [code]<?php if(isset($_POST['submit'])) { echo $_POST['formValue']; } ?> <form action="<?php $_SERVER['PHP_SELF']; ?>" method="post"> <input type="text" name="formValue" /><br /> <input type="submit" name="submit" value="Submit"> </form>[/code]Now that wont work on your ISPs server but this will: [code]<?php if(isset($submit)) { echo $formValue; } ?> <form action="<?php echo $PHP_SELF; ?>" method="post"> <input type="text" name="formValue" /><br /> <input type="submit" name="submit" value="Submit"> </form>[/code] Notice the difference? -
[!--quoteo(post=368843:date=Apr 26 2006, 03:28 PM:name=freshrod)--][div class=\'quotetop\']QUOTE(freshrod @ Apr 26 2006, 03:28 PM) [snapback]368843[/snapback][/div][div class=\'quotemain\'][!--quotec--] I'm relieved to see you guys are having problems with mail as well. In every tutorial, book, article, whatever I read, they make it sound like sending mail with the 'mail()' function is the easiest thing in the world. But I've tried and tried, and even simple code like this: <?php // Your email address $email = "freshrod@freshrod.com"; // The subject $subject = "testing"; // The message $message = "I hope this works!"; mail($email, $subject, $message, "From: $email"); echo "The email has been sent."; ?> just doesn't seem to work. [/quote] Are yoy running that code on a local setup of Apache/PHP? If you are then you will need to configure PHP to use an SMTP server in order for emails to be sent! You can configure the SMTP server in php.ini file. If you are running it off your webhost then it should work or your webhsots has disabled the use of using th mail function.
-
[!--quoteo--][div class=\'quotetop\']QUOTE[/div][div class=\'quotemain\'][!--quotec--]Also, could a mod let me know if I can display the page I have created already?[/quote] Yes you may, You can post it as a screenshot or post a link to your current page. Also you can post your code here too, provided that you use the code blocks ([ code]code here[/code] with out the space in the first code tag).
-
It allows you to define an index in array ie: [code]<?php $myarray = array("index1" => "hello ", "index2" => "world"); //no to called each array for both the iondex1 and index2 array index echo $myarray['index1']; echo $myarray['index2']; //out should be hello world ?>[/code] With out the definning an index you can access your arrays like so $myarray[0] and $myarray[1] also.
-
To the id bit is not a tag it is a HTML attribute. Attributes are the little commands inside a HTML tag ie, class, ie, href etc. To get the id there you just simple type it in! Ie on blah.html you do this: [code]<a href="blah.html" value="selected">Blah page</a><a href="blah2.html">Blah2 page</a>[/code] Then when you cliick on Blah 2 page it goes to blah2.html and in that file you just code id="selected" in the secound acnhor and remove the id from the first anchor tag. This is how this effect is done.
-
Your code does work if you dont use divs and put the id and the classes in the td or tr tag. Then your code will work. You dont have to use divs as nearly all html tags have the onclick attribute. The following is your revised code (NOTE: this only the html inside the body tag h=just replace the following with your old code): [code]<form action="processProfile.php" method="post" name="main"> <table width="756" height="91" border="0"> <tr> <td> </td> <td> </td> <td bordercolor="#CCCCCC"><strong>Create/edit your profile ...</strong></td> </tr> <tr bordercolor="#CCCCCC"> <td colspan="6" class="mH" onclick="toggleMenu('menu1')">Basics</td> </tr> <tr id="menu1" class="mL"> <td width="16%" rowspan="2" valign="top">Height:</td> <td width="1%" rowspan="2"> </td> <td colspan="4"> Between <select name="heightStart" id="heightStart" class="mO"> <option value="3">3'1"</option> <option value="4">3'2"</option> <option value="5">3'3"</option> </select> and <select name="heightEnd" id="heightEnd" class="mO"> <option value="3">3'1"</option> <option value="4">3'2"</option> <option value="5">3'3"</option> </select> </td> </tr> </table> </form>[/code]
-
Need to turn off Register Globals on shared site
wildteen88 replied to JRS's topic in PHP Coding Help
In some cases webhosts may allow the use of .htaccess files. With .htaccess you can change a few settings to the server such as turning off register_globals. If you create a .htaccess file in root of where you store your website files with the following: [code]php_flag register_globals off[/code] This may turn off register_globals through out your site. -
It doesn't really matter if your your ISP is running PHP4 as you are not using any PHP5 specific functions. What I think is the problem is die to your ISP having register_globals turned on. Which means that any use of superglobal arrays will not work, i e($_POSt, $_GET, $_SERVER variables). To see whether Register_globals is on run the following scipt: [code]<?php // remove the space between the p and the h in the code below: p hpinfo(); ?>[/code] Now run that on your ISPs server and find where it says register_globals if it says On in both coloumns this is the reason why your script isn't working.
-
Hi the reason why your cannt connect to mysql was becuaser mysql wasnt running and so you go the error. Whne you install MySQL you shoulf of installed it as a service this whay MySQL will start up automatically in the background when you boot into Windows. [b]EDIT:[/b] Oops Didn't see the following:[!--quoteo--][div class=\'quotetop\']QUOTE[/div][div class=\'quotemain\'][!--quotec--]--RESOLVED! I reinstalled mysql, but this time with tcp/ip connections enabled. works fine now thanks[/quote]
-
What! Whats your question? Not a lot of info is comming from what you have said so fair! [!--quoteo--][div class=\'quotetop\']QUOTE[/div][div class=\'quotemain\'][!--quotec--]That's my current code so far - I think I've got all the AJAX right, but I don't know what to put in the PHP files except setcookie, perhaps? Thanks.[/quote]
-
The reason why it aint working is becuase you are using a link and so the form isn't being submitted and so no $_POST array is created. What you will want to is put id into the link like so: [code]<a href="?do=interactive_requests_stable&accept=true&id=<?=$row['id']?>">Accept</a>[/code] Then change $_POSt['id'] in your SQL Quuery to $_GET['id'] so you SQL Query will be like so: [code]mysql_query("UPDATE $dbname.request_stable SET accepted = 'yes' WHERE id = ".$_GET['id']."");[/code]
-
Oops had a slight typo in the code provided. Change this line: [code]$conn = mysql_connect("localhost", "root", "rootPassword") or die("Unable to connect mysql: " mysql_error());[/code]to[code]$conn = mysql_connect("localhost", "root", "rootPassword") or die("Unable to connect mysql: " . mysql_error());[/code] Also I think you have error reporting turn off. You should turn on error reporting which you can do by editing the php.ini file and finding the line that says [b]display_errors = Off[/b] to [b]display_errors = On[/b] Now save your php.ini file and restart your Apache webserver.
-
New to php and need help with Login script
wildteen88 replied to OzRedBoy's topic in PHP Coding Help
If you want to submit for with a text link you can do this: [code]<span class="style2" onClick="javascript:document.details.submit();">Login</span>[/code] To show the username when they login you will need to setup a session which stores the users username ie: [code]<?php session_start(); $_SESSION['username'] = $_POST['user']; ?>[/code] Then whenever you want to show the users username add [b]session_start();[/b] after [b]<?php[/b] and use the following code which will show the users username: [code]echo $_SESSION['username'];[/code] -
Session variable contains blank data on hosted site
wildteen88 replied to JRS's topic in PHP Coding Help
Could you check also whether [b]register_globals[/b] is on or off on both sites too. As I have feeling your webshot has this enabled and you been coding with register_globals off on your local server, or vise versa -
Yeah that is done by a very simple javascript functions which changes a style on a div/table cell to [b]display: block[/b] as currently the defualt style before you click the link to a poll is [b]display: none[/b]. Then abit more javascript to strip out the link, or to hide it. For little demo of what the javascript function looks like have a look at [a href=\"http://www.phpfreaks.com/forums/index.php?s=&showtopic=90723&view=findpost&p=363935\" target=\"_blank\"]this[/a] post.
-
No errors are displayed
wildteen88 replied to gerkintrigg's topic in PHP Installation and Configuration
Yes To do so you must be able to have access to a file called php.ini and to be able to restart the webserver. If have access to the abpve then find and edit your php.ini. Now fuind the following line: [code]display_errors = Off[/code] change Off to [b]On[/b] If you want your error messages to be red then find the following: [code]; String to output before an error message. ;error_prepend_string = "<font color=ff0000>" ; String to output after an error message. ;error_append_string = "</font>"[/code]and remove the semi-colons infront of [b]error_prepend_string[/b] and [b]error_append_string[/b] Now save your php.ini file and restart your webserver for the changes to be made. -
PHP + PostGreSQL under Apache
wildteen88 replied to jnojr's topic in PHP Installation and Configuration
If you want to use postGreSQL too then you will need to recomiple PHP to use the postGreSQL extension. -
If you you are installing IPB2 then make sure your details yiur are entering upon installation are correct. For now try this: [code]<?php $conn = mysql_connect("localhost", "root", "rootPassword") or die("Unable to connect mysql: " mysql_error()); mysql_select_db("mysql") or die("Unable to select database: " . mysql_error()); echo "PHP was able to succesfully connect to MySQL and manage to select the mysql database too!"; ?>[/code] Before running the above code, make sure you change where it says [b]rootPassword[/b] to the actually password your set for your mysql installation, dont use your computer username password. Now run the code in the browser. If you get the following message: [code]PHP was able to succesfully connect to MySQL and manage to select the mysql database too![/code]Then php was able to connect to mysql and so PHP is talking to mysql and vise versa. If you dont can you post the full error message(s) here.
-
miss configured where is mysqli
wildteen88 replied to kdthiele's topic in PHP Installation and Configuration
I believe you will have to recomile PHP5 to use to the mysqli extension rather than using a pre-packaged rpm unless there is one already available. If you want to see how to recomile PHP you might want to look at [a href=\"http://tedi.heriyanto.net/papers/html-php5-suse.html\" target=\"_blank\"]this[/a], however thats for 9.2 Pro If you enable mysqli but no mysql then you will only be able to use mysqli functions and not any mysql functions. If you want to use both mysqli functions and mysql functions you will want to enable both extensions. -
You'll want to do something like this: [code]$date = date("d.m.y" , strtotime($row['show_date']))[/code]
-
miss configured where is mysqli
wildteen88 replied to kdthiele's topic in PHP Installation and Configuration
Of you want to use mysqli specified functions you will need to configure PHP to use the mysli extension rather than the mysql extension. -
I believe it was becuase you was using single quotes around your query and so PHP wasn't replacing the variable with its value it held as PHP treats variables inside single quotes "as-is".
-
*SOLVED* need help whit date and form functions
wildteen88 replied to zorce's topic in PHP Coding Help
The reason why it aint working is becuase you aint echo;ing/print'ing anything to the browser therefor no result is displayed! EDIt: This is what you want to do: [code]if (isset($_POST['Submit'])) { $base_day = date("d"); $base_hour = date("H"); $base_min = date("i"); $base_sec = date("s"); $new_day = ($base_day + $_POST['skill_day']); $new_hour = ($base_hour + $_POST['skill_hour']); $new_min = ($base_min + $_POST['skill_minute']); $new_sec = ($base_sec + $_POST['skill_second']); // without this nothing will be displayed! echo "Your Project will be completed in: " . $new_day . " days, " . $new_hour . " hours, " . $new_min . " minutes, and " . $new_sec . " secounds"; }[/code] -
The [b]ORDER BY[/b] statement is used at the end of your query like so: [!--sql--][div class=\'sqltop\']SQL[/div][div class=\'sqlmain\'][!--sql1--][span style=\'color:blue;font-weight:bold\']SELECT[/span] city_id, City [color=green]FROM[/color] [color=orange]city[/color] [color=green]WHERE[/color] country_id [color=orange]=[/color][color=red]'$country_id'[/color] [color=green]ORDER BY[/color] city [color=green]ASC[/color] [!--sql2--][/div][!--sql3--] change [b]city[/b] to the row name that you want your sql query to order the results by. ASC means [b]Asc[/b]ending order. If your want your result to be in [b]desc[/b]ending order your'll use [b]DESC[/b] instead of ASC