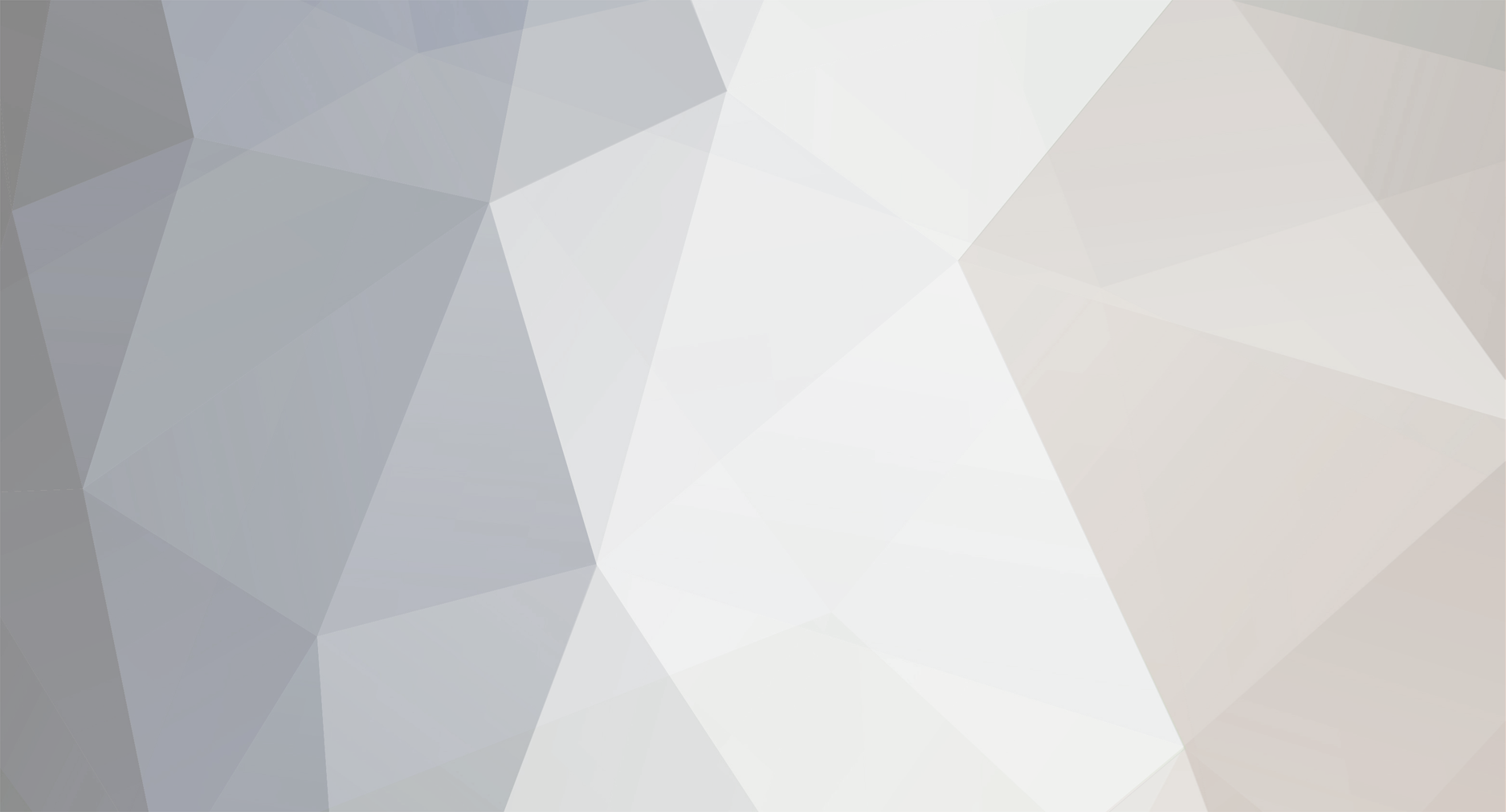
wildteen88
-
Posts
10,480 -
Joined
-
Last visited
Never
Posts posted by wildteen88
-
-
What you'll have to do is calculate your gTotal variable everytime it loops through the while loop. This si what you'll want to do:
[code]$gTotal += $row['cQuantity'] * $row['cartPrice'];[/code]This is the same as:
[code]$gTotal = $gTotoal + $row['cQuantity'] * $row['cartPrice'];[/code]but shorter.
So here is you new code:
[code]<?php
session_start();
include("../../Files/Database.php");
?>
<html>
<head>
<title>MyCart Information</title>
</head>
<body>
<form method=post>
<p align=left> Cart Information for <b><?php echo $_SESSION['username']?></b>,</p>
<table border="1" width="450" id="table1">
<tr>
<td align="center" width="30"> </td>
<td align="center" width="578" colspan="5"><b>My Cart Index</b></td>
<td align="center" width="30" height="26"> </td>
</tr>
<tr>
<td align="center" width="30"><b>No</b></td>
<td align="center" width="50"><b>Image</b></td>
<td align="center" width="100"><b>Product Name</b></td>
<td align="center" width="25"><b>Q</b></td>
<td align="center" width="75"><b>Price ea</b></td>
<td align="center" width="75" height="26"><b>Total</b></td>
<td align="center" width="30"> </td>
</tr>
<?php
if($_SESSION['username'] == "")
{
die ("Please login 1st");
}
else
{
//$query = "Select * from cart where username = $_SESSION[username] ";
$query = "SELECT * FROM cart INNER JOIN products ON cart.pId = products.pId where username = $_SESSION[username] ";
$result = mysql_query($query) or die ("Query Error on listing MyCart");
$gTotal = "";
while($row=mysql_fetch_array($result) )
{
if($row > 1)
{
$a += 1;
$gTotal += $row[cQuantity] * $row[cartPrice];
?>
<tr>
<td align="center" width="30" height="26"><?php echo $a ?></td>
<td align="center" width="50" height="26"><img src="../../Image/<?php echo $row[pImage]; ?>" width="50" height="50"></td>
<td align="center" width="100" height="26"><?php echo $row[pName] ?></td>
<td align="center" width="25" height="26"><input type=text name=quantity size= "1" value="<?php echo $row[cQuantity] ?>"></td>
<td align="center" width="75" height="26"><b>$</b> <? echo $row[cartPrice] ?></td>
<td align="center" width="75" height="26"><?php echo $total1 ?></td>
<td align="center" width="30" height="26"> </td>
</tr>
<?php
}
else
{
echo "Retriving data failed!!";
}
}
}
?>
<tr>
<td align="center" width="30" height="26"> </td>
<td align="center" width="175" height="26" colspan="3"> </td>
<td align="center" width="75" height="26"><b>Total</b></td>
<td align="center" width="75" height="26"><?php echo $gTotal?></td>
<td align="center" width="30" height="26"> </td>
</tr>
</table>
<table border="0" width="450" id="table2">
<tr>
<td align="center"> </td>
<td width="50" align="center"><input type=button name="updateCart" value="Update"></td>
<td width="169" align="center"><input type=button name="checkOut" value="Check Out"></td>
</tr>
</table>
<p align=left><b> Note : Please press Update first before Checkout, if you made any changes.</b></p>
</form>
</body>
</html>[/code] -
Have you got the links in the frame? If you do then use target="_parent" in your anchor (<a>) tags.
If a user clicks a link within the frame the whole page, not just the frame got to the linked page.
Could you explain a bit more please as I dont understand your question. -
Javascript doesnt have a pre built in function like isset. If want to check variable you'll have to do it like this:
[code]if(varName != "")
{
//then do something here
}
else
{
//do something else here
}[/code] -
I guess doing a mnaul install is out of the question then. Have search for apache2triad, wamp5 or XAMPP these are all-in-one installers which will get Apache, PHP and MySQL installed in a snap.
-
Are you using a database? If you do can you show us the code use for:
- connecting to database server
- selecting database, and
- when you query the database. -
You can only get the IP address of the client you can not get any other details that you have stated above from the client computer.
-
Implode syntax:
string [b]implode[/b] ( string glue, array pieces )
the glue bit is the sting or range of characters that gets inserted between values within an array. which is the pieces part. So for example. If you have any array that stores names or words and yiu want an easy way of displaying this arrat you'll want to use implode. Now you want to display your name or words seperated by a coma. This is how you'll do it:
[code]<?php
$num = array("one", "two", "three"," four");
$result = implode(", " , $num);
echo $result;
?>[/code]That will will result in this: [i]one, two, three, four[/i]
Explode syntax:
array [b]explode[/b] ( string separator, string string [, int limit] )
Explode is alittle diferent to implode as Explode custs up strings, depending on the seperator specified and puts each cut section into an array. So take this for example:
So you have string like so:
[i]hello I am redarrow[/i] and you want each word to be put into an array you'll soiemthing like this:
[code]<?php
$string = "Hello I am redarrow";
$words = explode(" ", $string);
?>[/code]You'll now get an any like so:
[i]array("Hello", "I", "am", "redarrow")[/i]
You dont have to use a for loop no. As you can echo out each word sperately like so:
[code]echo $words[0];
echo $words[1];
//ect.[/code]
Thats all there is to it. To get a better explanation always check the PHP manul for thse two functions
[a href=\"http://www.php.net/implode\" target=\"_blank\"]implode[/a]
[a href=\"http://www.php.net/explode\" target=\"_blank\"]explode[/a] -
change:
[code]"at http://".
"$_SERVER['SERVER_NAME']".[/code]to:
[code]at http://" . $_SERVER['SERVER_NAME'] . [/code] -
How would we know what parts we need to see? You arer the one that coded you TMS script no one else. A good start would be if you could post the code that creates the edit/delete links for your tutorials.
-
Instead of echo'ing you $contents file like so:
[code]echo '<B>Picture:</B><BR />'.$contents;[/code] try doing like so:
[code]header('Content-type: image/jpeg');
echo $contents;[/code] -
This is due to a security script that is installed and causes this in certian cases. It thinks your attacking server with your PHP code. for now could place your code over at [a href=\"http://pastebin.com/\" target=\"_blank\"]Paste Bin[/a] and provide a link to you code.
EDIT: I have edited your thread title as sadfasddf didn't make much sense! -
EDIT: OK he has just edited post which is better then what ever got posted before.
Also can you edit your title too so it makes sense!
I will unlock the topic too! -
When you use BBCodes the BBCode parser in IPB parses these BBCode to HTML and then puts it in htmlentities. Once it gets requested IPB then decodes the htmlentities into normal HTML so the browser parses it correctly. When you go to edit your post IPB then goes through a complicated process putting back in the bbcodes!
Why IPB decided to do it this anyones guess as I think it would of been better to parse the BBCode when you request the data! So when it gets stored its just the raw data.
This is why you see old posts with highlighted php code in them!
I got so I excited when say this thread but it was just an old thread that got replied to
-
You might want to use javascript to clear the fields you want clearing instead. Like this:
[code]<script type="text/javascript">
function clearForm()
{
document.formName.name.value = "";
document.formName.email.value = "";
}
</script>
<form name="formName" action="fileName.php" method="get">
Name: <input type="text" name="name"><br />
Email: <input type="text" name="email"><br />
Special Field: <input type="text" name="special" value="doesn't get cleared" /><br />
<input type="button" value="Reset" onClick="clearForm()">
</form>[/code] To add more follow this template:
[code]document.[name of form here].[name of input field].value = "";[/code] -
A few months back just before Xmas the site got hacked. Due to the hack the forum went through an upgrade to the lates version of IPB. During the upgrade we lost a few bits of functionality such as the PHP Tags, Solved button etc.
I think these are being planned to be added, although they was supposed to be. I don't know what is planned to be done. However I would like to have the SOLVED button back. Currently we have to manually edit the title of the thread in order to mark it SOLVED which isn't too hard do, however I havn't been marking posts as solved lately. -
Please do not request/attempt to get help with hacking anyones site or forum account. If anyone does help you do this then both you can the member(s) that do help will probably be banned. This is against our rules and is strickly forbidden!
Thread closed. -
When looking at your phpinfo details. MySQL and MySQLi support is enabled! If phpinof is showing the details about mysql/i then your mysql extensions have enabled and are working.
Also when you make any chnages to both the php.ini or httpd.conf file you must restart the server for the new changes to be made available. -
Yeah I guess j4ymf is absed in the UK like me but his site may be hosted in another time zone otherthan GMT. Didn't think of that.
-
If your on VDS then I think you might be able to access it and edit. In order find where it is use the code that I provided above in my previous post
-
Are you're on a shared hosting or are on you installed PHP/Apache on your PC?
If you're on a shared hosting account then you wont be able to modify the php.ini, however you could ask your host to enable it for you.
However if you have installed it localy then you can eidt your php.ini file. in order to find out where your php.ini is located run the following script on your server:
[code]<?php
//make sure you remove the . between the p and the h before running this script!
p.hpinfo();
?>[/code]When you run that it should a lot of info about PHP and your server. Look for the line [ir]Configuration File (php.ini) Path[/i] which should be located about 5 or so lines from the to. Next to that should be the full path to your PHP.ini file.
Hope that helps. -
PHP gets the time from the server itself. The servers clock should be configured to update automatically it should add an hour or take an hour away when the clocks go forward or back this process is automatic so you shouldn't have to worry about adding/removing hours from your times.
SO I would look into your how your server is configured. All PC/Laptops/Servers or what ever shoud do this. I think computers/servers will only do this if a setting called [b]Daylight saving changes[/b] is set. -
[!--quoteo(post=359718:date=Mar 29 2006, 06:11 PM:name=Spycat)--][div class=\'quotetop\']QUOTE(Spycat @ Mar 29 2006, 06:11 PM) [snapback]359718[/snapback][/div][div class=\'quotemain\'][!--quotec--]
Hi all,
Newbie question here.
I have a form that sends out an e-mail with some pre-defined strings in it.
However, upon getting the e-mail, I see that some unwanted carriage returns
have been inserted. While I realize that this is normal line wrapping, dependent upon the e-mail client being used and the screen resolution, I was wondering if there was a way to force lines to stay together.
Here is what I have from my php:
[code]
".$name." filled out our Goodwill.of Silicon Valley Customer Information Form\r
in order to be notified of upcoming sales in our 16 stores.\r
If you would also like to receive these notifications,\r
we look forward to you paying a visit to: ".$_POST['refurl']."";
[/code]
Everything is sent fine, alsong with the carriage returns I specified by: \r
However, when I get the e-mail, a return after "Customer" has been inserted.
Is there a solution to this?
Thanks,
Rick
[/quote] I do not understand your question. Are yoy saying that when you recieve your email a carriage/new line has been inserted after Customer? If that ios what you're saying then this is out of your control as its the defualt behaviour of the email client its self. Otherwise you'll have to use use the scroll bar at the bottom in order to read your email which is strange!
I would recommend you to chnage the following:
[code]filled out our Goodwill.of Silicon Valley Customer Information Form\r
in order to be notified of upcoming sales in our 16 stores.\r
If you would also like to receive these notifications,\r
we look forward to you paying a visit to: [/code]to[code]filled out our Goodwill.of Silicon Valley Customer Information Form in order to be notified of upcoming sales in our 16 stores.\r
If you would also like to receive these notifications, we look forward to you paying a visit to:[/code]
Now that you have used 1 carriage return your email should now look fine in any screen resolution/email client regardless of the size of the email viewing window.
This is the way I'd recommend.
-
use asreset button like so:
[code]<input type="reset" value="Reset Form Fields">[/code] -
Setup your form like so:
[code]<form name="formName" a.ction="fileName.php" method="get">
<input type="text" name="someText"><br />
<!-- READ THIS and delete after - setup our value=test variable -->
<input type="hidden" name="value" value="test" />
</form>[/code]
refreshing database
in PHP Coding Help
Posted
PHP doesn't have a function that can refresh the database. However MySQL does cache the queries prehaps if you use [a href=\"http://uk2.php.net/manual/en/function.mysql-free-result.php\" target=\"_blank\"]mysql_free_result()[/a] it may solve this.