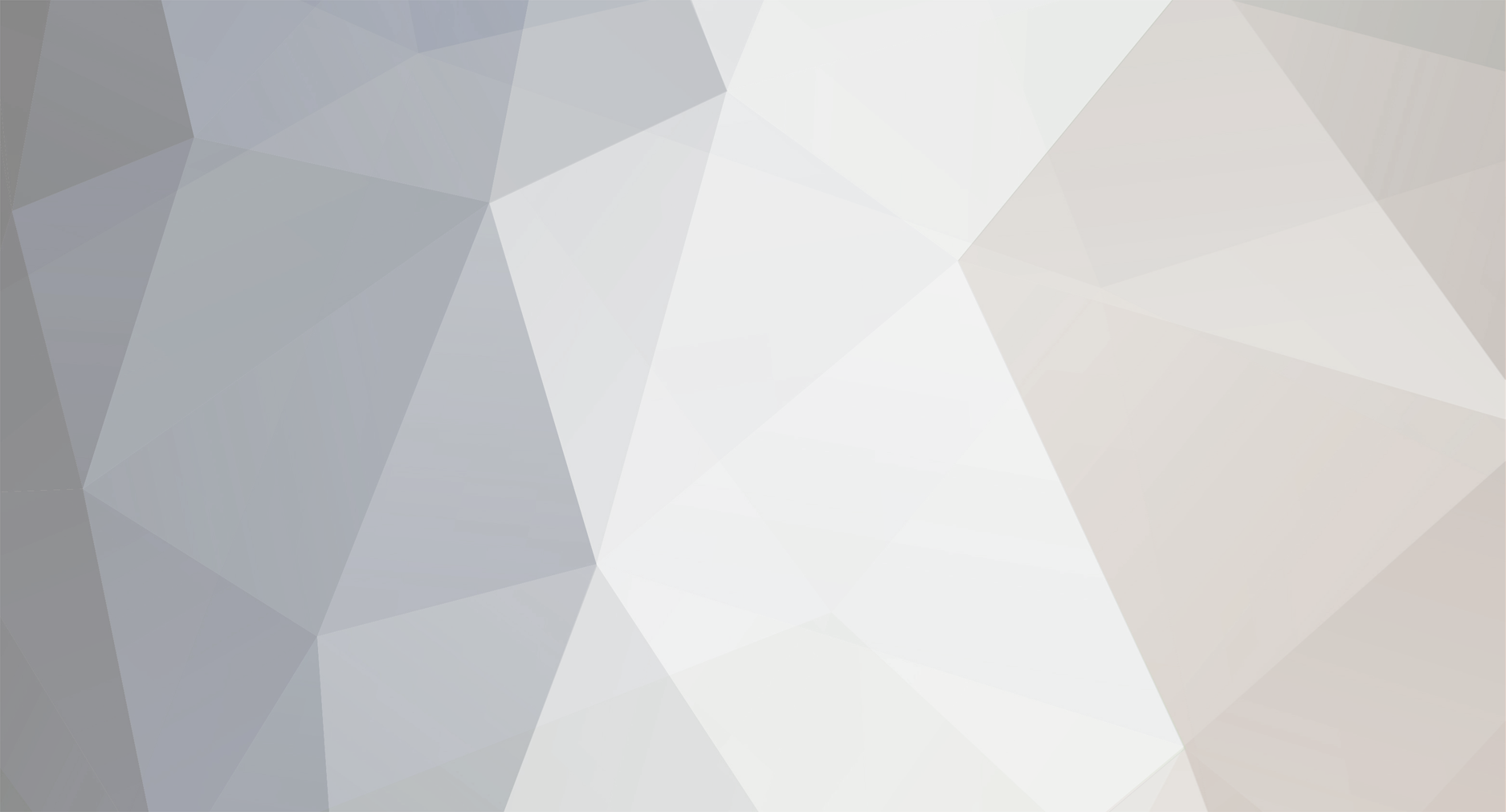
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
@ahvceo Make sure you're placing your code within .php files, as by default PHP is only parsed within these files. You should also make sure your host allows you to use PHP
-
if you're performing a redirect then these need to be header('Location: path/to/page.php'); header("my_profile.php"); } else { header("profile_edit.php"); Also check your servers error logs for why you're getting a 500 Internal Server Error.
-
[SOLVED] loading info to place inside div...
wildteen88 replied to Drags111's topic in PHP Coding Help
Place mainnav.php in your sites root folder (where you upload your sites content too). Now use include($_SERVER['DOCUMENT_ROOT']."/mainnav.php"); PHP will now always include mainnav.php from your sites root folder no matter where its included from. -
You should not be using http:// to include/require files. You should be using local file paths require($_SERVER['DOCUMENT_ROOT'].'/phpfiles.myfile.php');
-
The subdomain will be part of SERVER_NAME
-
Ofcourse it will. Files that are included do not get parsed seperatory. Your should name your variables differently in your included files, otherwise they will overwrite those in your parent file
-
Replace the 0's and 1's in your form with the value of the Accounting_Code field in your database. Now when you process the form use the following if(isset($_POST['books'])) { // loop through the books foreach($_POST['books'] as $account_code => $book) { echo '<h1>'.$book['title'].'</h1>'; echo 'Account Code: ' .$account_code.'<br />'; echo 'ISBN: ' .$book['isbn'].'<br />'; echo 'Price: ' .$book['price'].'<br />'; echo 'Langauge: ' .$book['lang'].'<br />'; echo 'Pages: ' .$book['pages'].'<hr />'; } }
-
Before using any user defined variables POST, GET, COOKIE, REQUEST etc you should check that it exists first, using isset. This will fix the notice if (isset($_REQUEST["o"]) && is_numeric($_REQUEST["o"]) && $_REQUEST["o"]!= 0) { $_SESSION["o"] = $_REQUEST["o"]; $o = $_REQUEST["o"]; } elseif (isset($_SESSION["o"])) { $o = $_SESSION["o"]; } elseif ($default_module) { $o = $default_module; } else { $o = mysql_result(mysql_query("SELECT module_id from ".$table_prefix."modules where active = 1 order by sequence limit 1"),0,0); }
-
[SOLVED] loading info to place inside div...
wildteen88 replied to Drags111's topic in PHP Coding Help
Yes the main file that includes the navbar needs to be in a .php file. However the file the navbar is in can have any file. -
No, I'd setup the form as: <form method="POST" action="test.php"> <p dir="ltr">row 1:<br /> <input type="text" name=books[0][isbn] size="20" value="123456789"><br /> <input type="text" name=books[0][title] size="20" value="Book_1"><br /> <input type="text" name=books[0][price] size="20" value="50"><br /> <input type="text" name=books[0][lang] size="20" value="En"><br /> <input type="text" name=books[0][pages] size="20" value="16"></p> <p dir="ltr">row 2:<br /> <input type="text" name=books[1][isbn] size="20" value="123456790"><br /> <input type="text" name=books[1][title] size="20" value="Book_2"><br /> <input type="text" name=books[1][price] size="20" value="60"><br /> <input type="text" name=books[1][lang] size="20" value="Fr"><br /> <input type="text" name=books[1][pages] size="20" value="17"></p> <p dir="ltr"> </p> <p d That way when you submit the form, all the info for the books will be within the $_POST['books'] array which will like: rray ( [0] => Array ( [isbn] => 123456789 [title] => Book_1 [price] => 50 [lang] => En [pages] => 16 ) [1] => Array ( [isbn] => 123456790 [title] => Book_2 [price] => 60 [lang] => Fr [pages] => 17 ) ) Which will now allow you to easily process the data. Example: if(isset($_POST['books'])) { // loop through the books foreach($_POST['books'] as $book) { echo '<h1>'.$book['title'].'</h1>'; echo 'ISBN: ' .$book['isbn'].'<br />'; echo 'Price: ' .$book['price'].'<br />'; echo 'Langauge: ' .$book['lang'].'<br />'; echo 'Pages: ' .$book['pages'].'<hr />'; } }
-
[SOLVED] loading info to place inside div...
wildteen88 replied to Drags111's topic in PHP Coding Help
Make sure you have saved your code to a .php file not .html PHP code by default only works within .php files. Howver files that are include/require'd can have any file extension -
[SOLVED] loading info to place inside div...
wildteen88 replied to Drags111's topic in PHP Coding Help
Yes. You can place HTML code within included files. -
What do you mean by "exit out of all global variables"? When PHP finishes executing the script all variables/functions/classes will be unset. PHP does not keep anything in memory after once the script has executed.
-
Change if (!$result = mysql_query("SELECT * FROM list1")) { echo "Database is down"; } to if (!$result = mysql_query("SELECT * FROM list1")) { echo "Database is down<br />".mysql_error(); }
-
You shouldn't use HTML to size fonts. You should be using CSS for that.
-
Oh shoot. Forgot about that. There is not built in function for getting the file size remote files (only local files). However I found this custom function http://www.phpcentral.com/207-get-filesize-remote-file.html
-
No problem. to help you understand further you should have a read of variable scope from the manual.
-
Use getimagesize maybe
-
No change the first three lines of your function to what I posted in my post above,
-
Use $_SERVER['REQUEST_URI'] to include the full url. PHP_SELF does not include the query string
-
Where is the variable $rcr_clients defined? functions cannot grab variables that are defined outside of it. In order for your function to retrieve a variable from the global scope you'll need to define it as global in the function. function selectClients() { gloabl $rcr_clients; $qperm_qry = "SELECT * FROM $rcr_clients ";
-
All what ignace's code does is define two functions, scanddir_r and search_r. By looks of it you need to call search_r in order for the code to work. This function requires you to pass a filename and directory to search.
-
Yes it is possible. Here is an example // ,list all items for the menu $items = array('Red Hibiscus', 'Blue Hibiscus', 'Purple Hibiscus'); // start form/menu echo '<from action="'.$_SERVER['PHP_SELF'].'" method="post"> <select name="color">'; // generate the list items foreach($items as $item) { // this part decides whether the current item will be selected $selected = (isset($_POST['color']) && $_POST['color'] == $item) ? ' selected="selected"' : ''; echo '<option value="'.$item.'"'.$selected.'>'.$item.'</option>'; } // end menu/form echo '</select> <input type="submit" name="submit" value="Choose" /> </form>';
-
The use of the mail function is not quite right here Only $name will be seen in the email. $email/$comments will be sent as additional headers which is not what you want. What you should do is: <?php $name = $_POST['name'] ; $email = $_POST['email'] ; $comments = $_POST['comments'] ; $message = "Name: $name\nEmail: $email\nComments: $comments"; mail( "****", "Feedback Form Results", $message); header( "Location: http://www.dkdebtmanagement.co.uk" ) ?> All I did was assign the body of the email to the $message variable. I then passed this to the mail function.