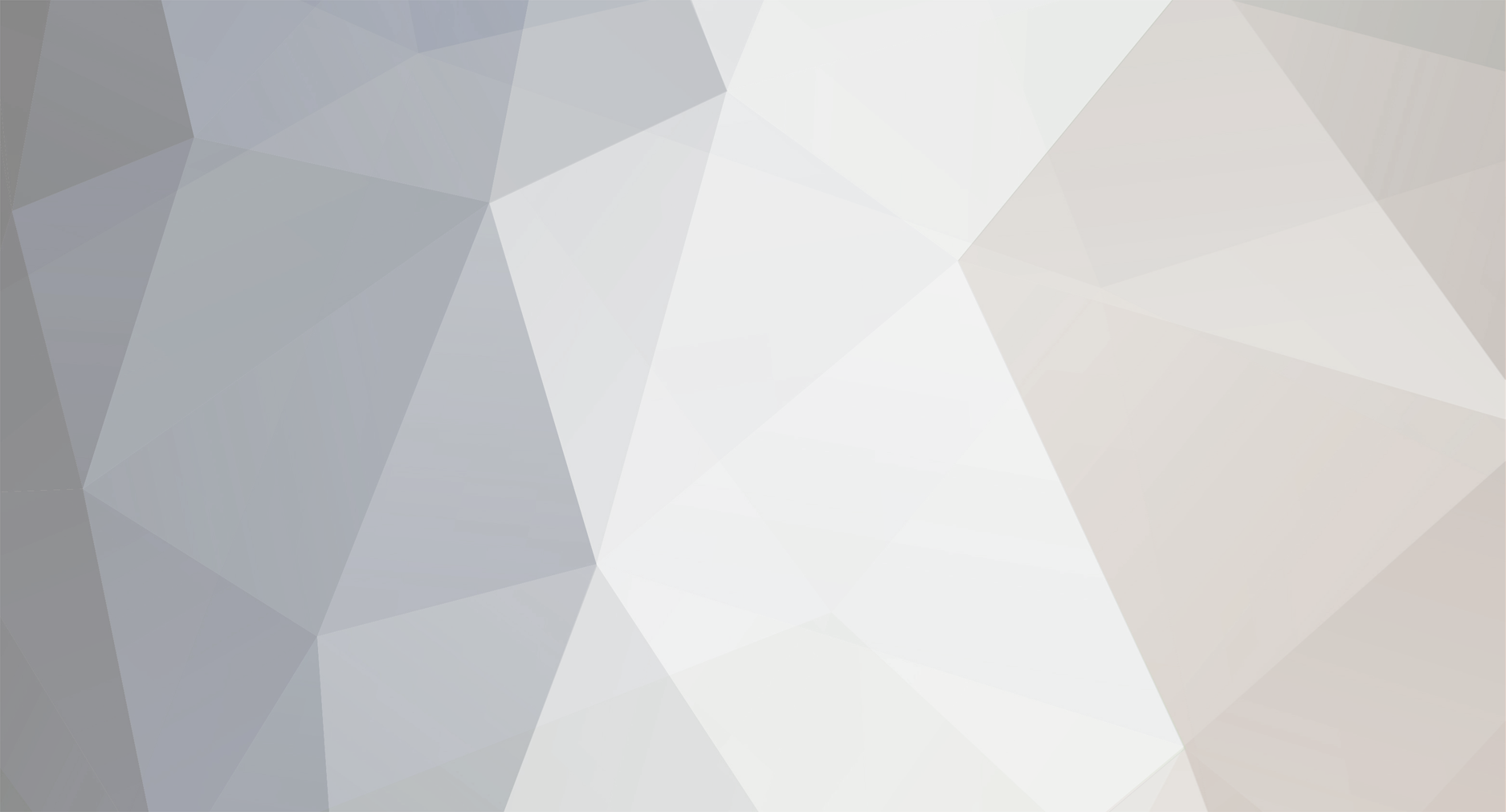
Richard_Grant
-
Posts
71 -
Joined
-
Last visited
Posts posted by Richard_Grant
-
-
Tbh iv'e never had any issues with Godaddy at all, i think your just incapable of managing your own website.
If you don't like basic webhosting then maybe you should get a VPS. Their job is only to make sure your SERVER is running not your WEBSITE.
-
Can someone dumb the function session_write_close() to me?
Ive read this http://php.net/manual/en/function.session-write-close.php
and i still don't understand!
-
Mysql is depreciated you should use mysqli or PDO in PHP.
-
The answer to this post describes my situation.
http://stackoverflow.com/questions/4451398/is-it-safe-to-keep-the-user-password-hash-on-session-php
It should be safe, since a client can only access his own session values. But you should:
- make sure the client cannot access it
- use another encryption, I wouldn't consider md5 safe, better use SHA-512 or something else.
But most importantly: Do you really need the hash in your session? If the user has been authenticated, he will always receive the same session (if your server is configured correctly). If you are anxious about session hijacking, you are going down the wrong path.
-
I have a login system Username and Password.
My password is encrypted with bcrypt, if it okay to store that bcrypt in a session as $_SESSION["hash"]
To verify that the user is who they say they are?
Or do i only need to do
$_SESSION["username"]
-
RewriteRule ^user/([0-9]+)/(.*)/(.*)(/|$) ./board/user.php?ID=$1&FIRSTNAME=$2&LASTNAME=$3 [L,NC]
That last(.*)
is going to match everything up to the end of the string, including the slash. When the regex engine has to choose between/
and the end of the string, it'll choose the latter because that's already true and it doesn't want to backtrack to match the slash.Be more restrictive than just
.*
for everything. Even if you want to allow all sorts of characters you know slashes won't be allowed, right? You should also enforce the end of the string, otherwise/1/Richard/Grant/other/stuff
will be allowed.RewriteRule ^user/([0-9]+)/([^/]+)/([^/]+)/?$ ./board/user.php?ID=$1&FIRSTNAME=$2&LASTNAME=$3 [L,NC]
Works
just like i needed!
-
I got these 2 rewrites:
RewriteRule ^user/([0-9]+)/(.*)/(.*)/ ./board/user.php?ID=$1&FIRSTNAME=$2&LASTNAME=$3 [L,NC] RewriteRule ^user/([0-9]+)/(.*)/(.*) ./board/user.php?ID=$1&FIRSTNAME=$2&LASTNAME=$3 [L,NC]
And i want to combine them but when i do this:
RewriteRule ^user/([0-9]+)/(.*)/(.*)(/|$) ./board/user.php?ID=$1&FIRSTNAME=$2&LASTNAME=$3 [L,NC]
It doesnt work..
when i type this in the url https://www.mysite/board/users.php?ID=1&FIRSTNAME=Richard&LASTNAME=Grant/ the variables are retrieved like this: (has trailing slash)
ID="1"
FIRSTNAME="Richard/Grant"
LASTNAME=""
when i type this in the url https://www.mysite/board/users.php?ID=1&FIRSTNAME=Richard&LASTNAME=Grant the variables are retrieved like this: (doesn't have trailing slash)
ID="1"
FIRSTNAME="Richard"
LASTNAME="Grant"
-
Ahhh! i just found out that if i type
https://www.mysite.com/user/RichardGrant/1
it doesnt recongnize the url because the trailing slash...
but this works fine!
-
im using this:
RewriteCond %{REQUEST_URI} user/(.*)/(.*)/ RewriteRule user/(.*)/(.*)/ %{DOCUMET_ROOT}/board/profile.php?ID=$1&NAME=$2
Is that fine? its working fine but is it depreciated or anything?
-
You will first need to edit your PHP script so it outputs the links in the new format. Eg
<a href="/user/1">FooBar</a>
Then in .htaccess file in the your sites document root. You can use the following rule
RewriteEngine On RewriteRule user/(\d+) board/profile.php?user=$1 [NC]
To map /user/x to /board/profile.php?user=x
Thank you
im sure that will work exactly the way i need it to, i will let you know as soon as i finish making the user profile!
-
Okay so i have a URL like this..
https://www.mysite.com/board/profile.php?user=1
I want the URL to be like this..
https://www.mysite.com/user/1
.htaccess rewrites have always confused me.
-
Setting the expiration time to the past is a compatibility feature for Internet Explorer.
What people miss, however, is that PHP automatically sets the expiration time when you give it an empty value. So the time() stuff doesn't do anything and is arguably a waste of space.
oh so i can just put ?
setcookie($name, '', NULL, "/", "", 0);
Without any compatibility issues?
-
This is prob a stupid question.. but i've always wondered..
When deleting a cookie why do we use
why do we use:
setcookie($name, '', time() - 3600, "/", "", 0);
when this works just fine:
setcookie($name, '', 0, "/", "", 0);
isn't the time() just a waste of space?
I ask this because everywhere i look i see:
setcookie($name, '', time() -3600 , "/", "", 0);
-
Richard,
I am not sure I understand either your original point or your train of thought. To quote you:
I agree that sniffing is a potential problem. It is far less of a potential problem than people think however. In order to sniff someone's packets, you need to be able to technically intercept their packets. With the advent of high speed switching, there are far fewer places for people to sniff -- although the pervasive use of wifi hotspots are a problem.
What I don't understand is your assertion that the use of TLS/SSL doesn't secure your communication, when in fact it does --- via strong encryption.
If there's some misunderstanding here, then you should probably respond with the specifics.
Because you can force when the user goes to https://www.mysite... to go to http://www.mysite
-
#closed
-
Never invent your own security protocol. As a layman (which we all are), your chance of success is exactly zero. And even if you were a security expert, designing protocols is a matter of many years and extensive peer reviews. It's not something you do on your own in 5 minutes.
The solution to your problem is TLS/SSL. This reliably prevents people from sniffing or manipulating the network traffic. You find it too heavy for your purposes? Well, there's a reason why it's heavy. If there was a shortcut, trust me, we'd already use it.
So for an actual website, any custom protocol is out of the question. If you're doing this purely for educational purposes, the whole idea is still heavily flawed:
First of all, it's not even clear what you're trying to protect. The MD5 hash is the password to your application, which means you still transmit it as plaintext. Anybody who intercepts the hash can log in as that user. The only theoretical benefit is that the attacker doesn't immediately see the original input, so if the client reuses the same password on a different site (which of course you should never do), then the account on that other site may survive a bit longer. But for your own site, there's absolutely no benefit whatsoever.
Then of course there's a conceptual error: As you've already realized, anybody who is able to manipulate the network traffic might as well remove all the fancy JavaScript code and grab the original user input. There's no solution to this. You simply cannot create trust within an entirely untrusted environment. You need something to start with. In the case of TLS, that's what certificates are for.
So at best, you get protection against passive attackers which only listen and never manipulate the traffic. But since we cannot choose our attackers, this isn't very useful.
Last but not least, JavaScript is not always available. For example, many people turn it off for security reasons. What now? Does it mean the plaintext password gets sent around? Does the entire site cease to function?
Note that those are not suggestions for fixing the protocol. It cannot be fixed. The only option for a production website is TLS.
I stopped reading this at
All log in and registration scripts should be in SSL, Which mine are. The data can still be grabbed from https.
-
I just realized that hashing the username is pointless because the session will just get the right username after.
_ so instead _
Im not going to store the username in a session like i usually do,
Im going to store the ID of the user and make the db get the information when needed.
-
Data "ciphering"? Maybe you mean data siphoning? Honestly, I stopped reading at that point.
Yes, now go back and reread it. The terminology is irrelevant
-
Data siphoning is becoming more common every day,
Data siphoning is when you intercept the data and sniff between a client and a host, also known as sniffing a connection. ( i am focusing on session hijack)
To protect clients I've decided to write an MD5 calculation function which changes a secure string (such as a password) to plain MD5
Then once the MD5_password reaches PHP i BCRYPT with cost 20 using password_hash
_
MD5 is not ideal at all and i would like to write a better encryption but i only know how to do MD5 for java script, but i really don't need that much security here.
the purpose is to not show sensitive information, that's going to be hashed on the server, during a data siphon attack.
_
Data siphoning can not be protected against on the host server, the siphoning happens on the clients side usually when they don't have a strong firewall or such.
What are some good techniques you would practice to protect from data siphoning?
Before added security i was able to siphon this:
Username: Richard
Password: mypassword
After added security i was able to siphon this:
Username: 6ae199a93c381bf6d5de27491139d3f9
Password: 5f4dcc3b5aa765d61d8327deb882cf99
Now the only vulnerability between the client and server is if the hacker dns hacks the client which could redirect them to a website that looks like mine with the same EXACT url. which i can't help.
The real username can be retrieved in a session on login.
The real username and password can be found if a hacker injects js to remove the MD5 function, so if you know how to detect JavaScript injection i would like to know that as well.
______
Pretty much it looks like this..
Form -> Send md5(username) & md5(password) -> Server check if match in datbase -> If so login.
^ cypher ^cypher (session)
-
you need to be using a float datatype.
4.90205690308E-6 is exponential.
-
This has nothing to do with PDO, it's the same thing with MySQLi. In fact, you'd have to write twice as much code for MySQLi.
I was talking about not using prepared statements my bad about that.
-
I think this is a misunderstanding. If you put the table name into an SQL string, you'll get a syntax error:
SELECT * FROM 'this_is_invalid';
Identifiers are not strings, so you cannot use PDO::quote().
Like I said, there's no easy solution. In fact, it's impossible to prevent SQL injections with the function itself, because you want it to accept complete SQL clauses (like the WHERE clause). Your only chance is to carefully validate all input before you pass it to the function.
You should also consider using professional database frameworks like Doctrine. They don't entirely solve the problem either, but they're more mature and easier to control.
. Its really spaghetti and if i didn't spend hours setting up my server to use PDO i would switch to mysqli
-
You mean for identifiers? This doesn't work. mysqli_real_escape_string() only works within quoted string values. It only prevents users from “breaking out” of the quoted expression. If there are no quotes, the whole function is useless.
By the way, the PDO equivalent of mysqli_real_escape_string() is PDO::quote().
My table name and col are passed in as a string parameter so PDO::quote should work just fine for me
-
You indeed cannot pass identifiers like column or table names to a prepared statements, and that's indeed a problem.
There are basically two solutions: Either you avoid this scenario altogether, or you use a whitelist of acceptable identifiers. Theoretically, you might also try a kind of generic validation so that you can use entirely dynamic queries (which seems to be your goal). But this is risky and fragile, so I'd avoid it at all cost. Even the big database frameworks shy away from this.
Would you scold me for using mysqli_real_escape_string ? (if it would even let me use my pdo connection as the connection variable)
Bash syntax
in Linux
Posted
This is a simple permutation function that i was converting from php:
What is wrong with the syntax?
Link to PHP version: http://stackoverflow.com/questions/12293870/algorithm-to-get-all-possible-string-combinations-from-array-up-to-certain-lengt