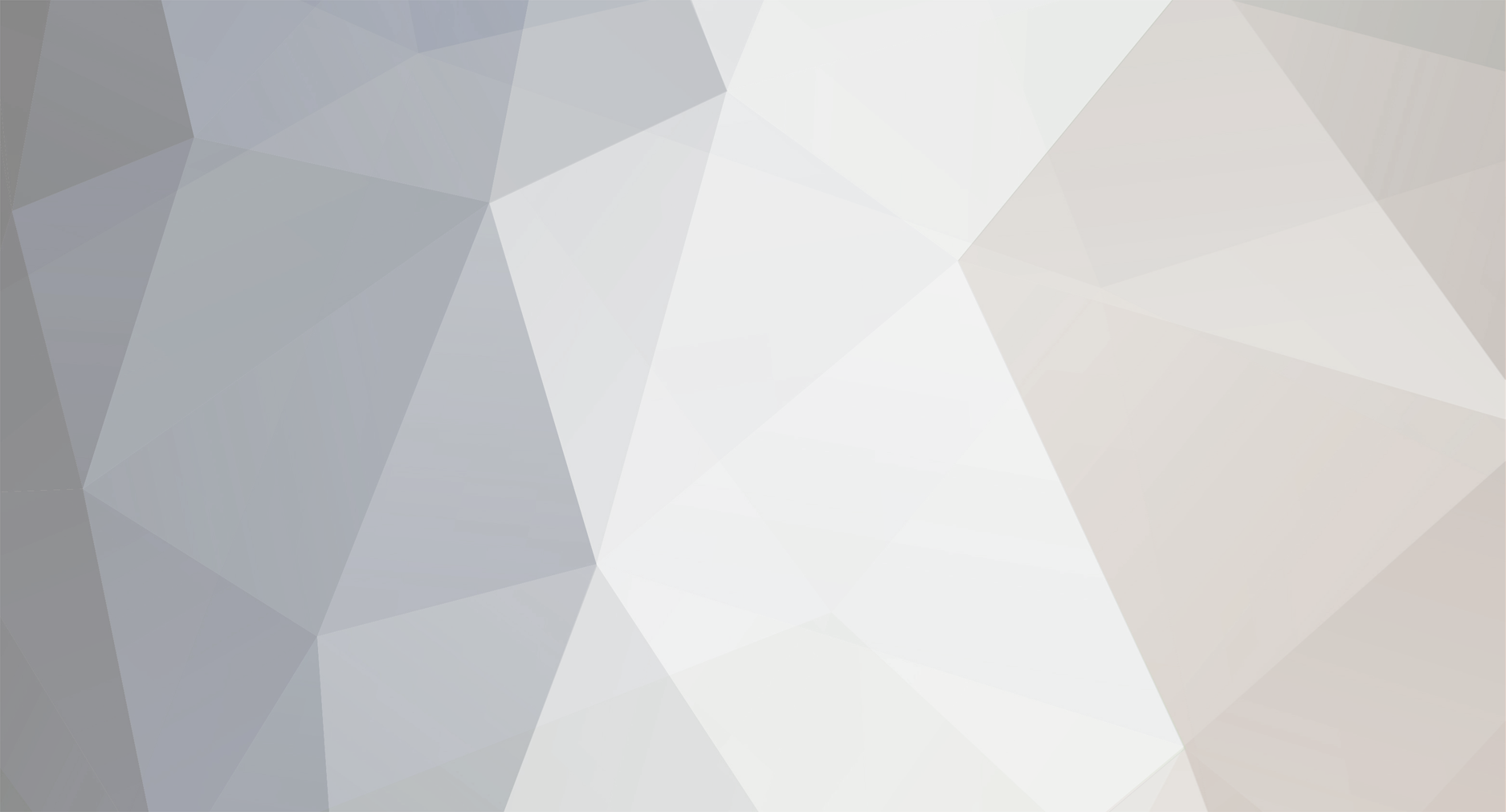
doghouse308
Members-
Posts
9 -
Joined
-
Last visited
doghouse308's Achievements

Newbie (1/5)
0
Reputation
-
Thank you for your suggestion!
-
Thank you for your input and suggestions. There is still some cosmetic work, but the program is now functional. I would like your feedback. I know there are probably better ways to accomplish what I was doing with this program. I have only been working with PHP for about three weeks. Also, since I was using an outdated book, would someone please suggest a good book to learn PHP? <!DOCTYPE html> <!-- Paul Peterson Rock paper Scissors Lizard Spock Survey of Perl Python and PHP Fall 2014 --><?php //initialize stats array $stats = array(); $stats['Win'] = 0; $stats['Lose'] = 0; $stats['Draw'] = 0; $stats['games'] = 0; if(isset($_POST['stats'])) { if(isset($_POST['stats']['Win'])) $stats['Win'] = $_POST['stats']['Win']; if(isset($_POST['stats']['Lose'])) $stats['Lose'] = $_POST['stats']['Lose']; if(isset($_POST['stats']['Draw'])) $stats['Draw'] = $_POST['stats']['Draw']; if(isset($_POST['stats']['games'])) $stats['games'] = $_POST['stats']['games']; } ?> <html> <head> <meta charset="UTF-8"> <title></title> </head> <body> <?php // if human has a value get computer play, compare results and echo results if(isset($_POST['human'])){ $human = $_POST['human']; $computer = computer_play(); $win = $lose = $draw = 0; if(isset($_POST['human'])){ if ($human == $computer){ $draw = 1; $outcome = 'Draw'; }else{ switch($human){ case 'Rock': switch ($computer){ case 'Paper': $outcome = "Lose";$action = "Covers";break; case 'Scissors': $outcome = "Win";$action = "Crushes";break; case 'Lizard': $outcome = "Win";$action = "Crushes";break; case 'Spock': $outcome = "Lose";$action = "Vaporizes";break; } break; case 'Paper': switch ($computer){ case 'Rock': $outcome = "Win";$action = "Covers";break; case 'Lizard': $outcome = "Lose";$action = "Eats";break; case 'Scissors': $outcome = "Lose";$action = "Cuts";break; case 'Spock': $outcome = "Win";$action = "Disproves";break; } break; case 'Scissors': switch ($computer){ case 'Rock': $outcome = "Lose";$action = "Crushes";break; case 'Paper': $outcome = "Win";$action = "Cuts";break; case 'Lizard': $outcome = "Win";$action = "Decapitates";break; case 'Spock': $outcome = "Lose";$action = "Smashes";break; } break; case 'Lizard': switch ($computer){ case 'Rock': $outcome = "Lose";$action = "Crushes";break; case 'Paper': $outcome = "Win";$action = "Eats";break; case 'Scissors': $outcome = "Lose";$action = "Decapitates";break; case 'Spock': $outcome = "Win";$action = "Poisons";break; } break; case 'Spock': switch ($computer){ case 'Rock': $outcome = "Win";$action = "Vaporizes";break; case 'Paper': $outcome = "Lose";$action = "Disproves";break; case 'Scissors': $outcome = "Win";$action = "Smashes";break; case 'Lizard': $outcome = "Lose";$action = "Poisons";break; } break; } } } // if there is an outcome, display results if(isset($outcome) ){ if ($outcome =='Win'){ echo "<br />You ".$outcome."!!<br />Your ".$human." ".$action." my ".$computer." <br />"; }elseif ($outcome =='Lose'){ echo "<br />You ".$outcome."!!<br />My ".$computer." ".$action." your ".$human." <br />"; }elseif ($outcome =='Draw'){ echo "Draw! <br /> We both played ".$human."<br />"; } }; // update stats $stats = game_statistics($outcome, $stats); } else {echo "Please pick something"; } ?> <form method = "post" action = "rpsls_paul.php"> <?php foreach($stats as $key => $val) { echo '<input type = "hidden" name = "stats['.$key.']" value = "'.$val.'" />'; } ?> <h2>Let's Play Rock, Paper, Lizard, Spock</h2> <hr> <table> <tr> <td><input type = "radio" name = "human" value = "Rock" />Rock</td> <td><input type = "radio" name = "human" value = "Paper" />Paper</td> </tr> <tr> <td><input type = "radio" name = "human" value = "Scissors" />Scissors</td> <td><input type = "radio" name = "human" value = "Lizard" />Lizard</td> </tr> <tr> <td colspan = "2"><input type = "radio" name = "human" value = "Spock" />Spock</td> </tr> <tr> <td colspan = "2"><hr></td> </tr> <tr> <td colspan = "2"><input type = "submit" value = "Play!" /></td> </tr> </table> </form> <?php // generate computer play and return results function computer_play(){ $play = rand(1,5); switch ($play) { case 1: $computer = "Rock"; return $computer; case 2: $computer = "Paper"; return $computer; case 3: $computer = "Scissors"; return $computer; case 4: $computer = "Lizard"; return $computer; case 5: $computer = "Spock"; return $computer; } } // update stats array function game_statistics($outcome, $stats ){ $stats['games']++; $stats[$outcome]++; echo "Games: ".$stats['games']; echo " Win: ".$stats['Win']; echo " Loss: ".$stats['Lose']; echo " Draw: ".$stats['Draw']; return $stats; } ?> </body> </html>
-
I wrote the code in pieces and tested as I went along, the parts that are commented out are the problem. When I added those sections (one at a time) is when this stopped working. That is how I know the problem is there.
-
Thank you for your help. I have re-thought this and made significant revisions. The program now runs (mostly) but there are still problems. At the moment there are two sections commented out. The first section is intended to display the results of the hand and the second is to track the game stats. With those sections commented out the program will run but displays nothing in the browser when they are included. One other issue I need to resolve is making the for values required. It will not be good if the user can click submit(play) without selecting anything. I found something at w3 schools site, but was not able to make it work. Please share your comments on this. There is still some house keeping and some finishing touches. But I would like to resolve the issues above before starting to wrap things up. Thanks in advance! <!DOCTYPE html> <!-- To change this license header, choose License Headers in Project Properties. To change this template file, choose Tools | Templates and open the template in the editor. --> <html> <head> <meta charset="UTF-8"> <title></title> </head> <body> <?php $human = human_play(); $computer = computer_play(); echo $human."<br />".$computer; $win = $lose = $draw = 0; if ($human == $computer){ $draw = 1; echo "Draw! <br /> We both played ".$human; }else{ switch($human){ case 'Rock': switch ($computer){ case 'Paper': $outcome = "Lose";$action = "Covers";break; case 'Scissors': $outcome = "Win";$action = "Crushes";break; case 'Lizard': $outcome = "Win";$action = "Crushes";break; case 'Spock': $outcome = "Lose";$action = "Vaporizes";break; } break; case 'Paper': switch ($computer){ case 'Rock': $outcome = "Win";$action = "Covers";break; case 'Lizard': $outcome = "Lose";$action = "Eats";break; case 'Scissors': $outcome = "Lose";$action = "Cuts";break; case 'Spock': $outcome = "Win";$action = "Disproves";break; } break; case 'Scissors': switch ($computer){ case 'Rock': $outcome = "Lose";$action = "Crushes";break; case 'Paper': $outcome = "Win";$action = "Cuts";break; case 'Lizard': $outcome = "Win";$action = "Decapitates";break; case 'Spock': $outcome = "Lose";$action = "Smashes";break; } break; case 'Lizard': switch ($computer){ case 'Rock': $outcome = "Lose";$action = "Crushes";break; case 'Paper': $outcome = "Win";$action = "Eats";break; case 'Scissors': $outcome = "Lose";$action = "Decapitates";break; case 'Spock': $outcome = "Win";$action = "Poisons";break; } break; case 'Spock': switch ($computer){ case 'Rock': $outcome = "Win";$action = "Vaporizes";break; case 'Paper': $outcome = "Lose";$action = "Disproves";break; case 'Scissors': $outcome = "Win";$action = "Smashes";break; case 'Lizard': $outcome = "Lose";$action = "Poisons";break; } break; } } echo "<br />Outcome: ".$outcome." Action: ".$action."<br />"; /* commented out - contains trouble if ($outcome === 'Win'){ $win = 1; echo "<br />You ".$outcome."!!<br />Your ".$human." ".$action." my ".$computer". <br />"; }else{ $lose = 1; echo "<br />You ".$outcome."!!<br />My ".$computer." ".$action." your ".$human". <br />"; } */ if ($outcome =='Win'){ $win = 1; }elseif ($outcome =='Lose'){ $lose = 1; }else{ $draw = 1; }; echo $win." ".$lose." ".$draw; //game_statistics($win, $lose, $draw); function human_play(){ echo <<<_END <form method = "post" action = "temp.php"> <h2>Let's Play Rock, Paper, Lizard, Spock</h2> <hr> <table> <tr> <td><input type = "radio" name = "human" value = "Rock" />Rock</td> <td><input type = "radio" name = "human" value = "Paper" />Paper</td> </tr> <tr> <td><input type = "radio" name = "human" value = "Scissors" />Scissors</td> <td><input type = "radio" name = "human" value = "Lizard" />Lizard</td> </tr> <tr> <td colspan = "2"><input type = "radio" name = "human" value = "Spock" />Spock</td> </tr> <tr> <td colspan = "2"><hr></td> </tr> <tr> <td colspan = "2"><input type = "submit" value = "Play!" /></td> </tr> </table> </form> _END; $human = $_POST['human']; return $human; } function computer_play(){ $play = rand(1,5); switch ($play) { case 1: $computer = "Rock"; return $computer; case 2: $computer = "Paper"; return $computer; case 3: $computer = "Scissors"; return $computer; case 4: $computer = "Lizard"; return $computer; case 5: $computer = "Spock"; return $computer; } } /* function game_statistics($win, $lose, $draw){ static $games = $won = $loss = $ddraw = 0 $won = $won + $win; $loss = $loss + $lose; $ddraw = $ddraw + $draw; echo $won." ".$loss." ".$ddraw; } */ ?> </body> </html>
-
Is it possible to have a static variable in php? I want the value of a variable to remain unchanged the next time a function is called.
-
If I try to run this from MAMP, nothing happens. If I try to run it from an IDE (netbeans) it opens window after window after window until I force the browser to close. This is supposed to be a variant of the game rock, paper, scissors - rock, paper, scissors, lizars, spock After reading the responses, I believe the login script will be included in the program for simplicity. This will never be used on a remote server and I don't see the need for the additional complexity. The existing login file is supposed to generate a form to collect the server, username and password information and establish the connection. If the rpsls and choices table are not present, they will be created (a better method of accomplishing this was presented in a previous response and will be incorporated shortly). The last function in the login file is intended to prevent unintended and potentionally damaging information to be sent using the form. After further reflection, I may simply eliminate that too and simply use the information needed to establish the connection with the database in plain english. Going in order through the rest of the script: The game begins with a call to the ready_to_play function that creates a form with radio buttons to begin the game. If 'Yes' is checked the gameResults table is createed and the game begins by calling the rock_paper_scissors_lizard_spock function once the user clicks on the button. If 'No' is selected the close_rpsls function is called, closing the connection and ending the game. The rock_paper_scissors_lizard_spock function calls the human_play function that creates a table for the human play and returns the value to the rock_paper_scissors_lizard_spock function. Next the computer_play function is called and the value returned to the rock_paper_scissors_lizard_spock function. The computer and human values are passed to the game outcome function that queries the rpsls table for the outcome and action that match the human and computer plays and the results of the game are send to the gameResults table. After each play the user is asked if they want to play again using the play_again function that is very similar to the ready_to_play function. If the user selects 'Yes' the rock_paper_scissors_lizard_spock function is called. If 'No' the close_rpsls function is called The user is asked if they really want to quit. If 'No' the rock_paper_scissors_lizard_spock function is called. If 'Yes' the game_statistic function is called (not currently in the included code. It looks like I forgot that function call). The game_statistics function creates a table to display the number of games, wins, losses and draws, DROPS the gameResults table and closes the connection. I think I also need something there to exit the game. I hope that helps.
-
ginerjm: Yes, this is the first script I wrote in php. And yes, it is my work written using what I learned in an appearantly outdated book. I appreciate your feedback and will work on the issues. Ch0cu3r:As mentioned above, this was written using information from the book selected for a class I am taking. I did not realize that the information was so far out of date. That was a suggestion from a co-worker, who learned php on his own. I found several of the syntax errors after downloading an editor that works with php. Thank you for the assistance. The overkill as you that you justifiably note is due to the way the program was requested. I don't wish to appear insolent, but what I described is what happens when trying to run this program. I have encountered the same issue using Safari and Firefox.
-
I stumbled across this site after being slammed hard elsewhere for being a novice and really not knowing what I am doing. What I have read so far is more encouraging. I just wrote my first program in php and it is not working at all right now. All it keeps doing is opening window after window until I force the browser to close. I am using a Mac running Yosemite and using MAMP. Hopefully that is enough background. I know this is an introduction area, so I will also post this in another forum in case this is closed for being off topic. This is a login file to connect to the server: <?php // login.php // Get connection information echo <<<_END <form method = "post" action = "login.php"> <pre> <input type = "text" name = "localhost" />host server<br /> <input type = "text" name = "username" />Username<br /> <input type = "text" name = "password" /><br /> <br /> <input type = "submit" value = "submit" /> </form> _END $db_server = sanitize_string($localhost); $db_username = sanitize_string($username); $db_password = sanitize_string($password); /* $user = 'root'; $password = 'root'; $db = 'rpsls'; $host = 'localhost'; $port = 3306; $link = mysql_connect( "$host:$port", $user, $password ); $db_selected = mysql_select_db( $db, $link ); */ mysql_connect($db_server, $db_username, $db_password) or die(mysql_error()); // Create rpsls table if it does not exist $tbl = "rpsls"; $query = "CREATE TABLE rpsls(human VARCHAR(10), computer VARCHAR(10), outcome VARCHAR(5), action VARCHAR(15)); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Rock", "Paper", "Lose", "Covers"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Rock", "Scissors", "Win", "Crushes"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Rock", "Lizard", "Win", "Crushes"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Rock", "Spock", "Lose", "Vaporizes"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Paper", "Rock", "Win", "Covers"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Paper", "Scissors", "Lose", "Cuts"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Paper", "Lizard", "Lose", "Eats"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Paper", "Spock", "Win", "Disproves"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Scissors", "Lizard", "Win", "Decapitates"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Scissors", "Spock", "Lose", "Smashes"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Scissors", "Rock", "Lose", "Crushes"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Scissors", "Paper", "Win", "Cuts"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Lizard", "Spock", "Win", "Poisons"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Lizard", "Rock", "Lose", "Crushes"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Lizard", "Paper", "Win", "Eats"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Lizard", "Scissors", "Lose", "Decapitates"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Spock", "Rock", "Win", "Vaporizes"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Spock", "Paper", "Lose", "Disproves"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Spock", "Scissors", "Win", "Smashes"); INSERT INTO rpsls (human, computer, outcome, action) VALUES ("Spock", "Lizard", "Lose", "Poisons");"; check_table($tbl, $query); // Create choices table if it does not exist $tbl = "choices"; $query = "CREATE TABLE choices(id SMALLINT, choice VARCHAR(10)); INSERT INTO choices (id, choice) VALUES (1, "Rock"); INSERT INTO choices (id, choice) VALUES (2, "Paper"); INSERT INTO choices (id, choice) VALUES (3, "Scissors"); INSERT INTO choices (id, choice) VALUES (4, "Lizard"); INSERT INTO choices (id, choice) VALUES (5, "Spock");"; check_table($tbl, $query); // Sanitize user input function sanitize_string($var) { $var = stripslashes($var); $var = htmlentities($var); $var = strip_tags($var); return $var; } function check_table($tbl, $query){ $db = new mysqli(...); $result = $db->query("SHOW TABLES LIKE "$tbl); if ($result->num_rows == 0){ mysql_query($query); } } ?> and this is the program: <?php // log into server and database require_once 'login.php'; $db_server = mysql_connect($db_hostname, $db_username, $db_password); if (!$db_server) die("Unable to connect to MySQL: " . mysql_error()); $conn = mysql_connect($db_server, $db_username, $db_password) or die(mysql_error()); $db_database = 'rpsls'; mysql_select_db($db_database) or die("Unable to select database: " . mysql_error()); // Start Game ready_to_play(); // Rock Paper Scissors Lizard Spock game function rock_paper_scissors_lizard_spock() { $human = human_play(); $computer = computer_play(); game_outcome($human, $computer); play_again(); // Start Game Function function ready_to_play(){ echo <<<_END <form method = "post" action = "rpsls.php"> <h2>Ready to play Rock, Paper, Lizard, Spock?</h2> <hr> <table> <tr> <td><input type = "radio" name = "ready" value = "Yes" />Yes</td> <td><input type = "radio" name = "ready" value = "No" />No</td> </tr> <tr> <td colspan = "2"><input type = "submit" value = "Play!" /></td> </tr> </table> </form> _END if ($ready == "Yes"){ $query = "CREATE TABLE gameResults ( games SMALLINT NOT NULL, win SMALLINT NULL, loss SMALLINT NULL, draw SMALLINT NULL, PRIMARY KEY (games))"; mysql_query($query); rock_paper_scissors_lizard_spock(); }else{ close_rpsls(); } } // Play Again // Start Game Function function play_again() { echo <<<_END <form method = "post" action = "rpsls.php"> <h2>Play Again?</h2> <hr> <table> <tr> <td><input type = "radio" name = "ready" value = "Yes" />Yes</td> <td><input type = "radio" name = "ready" value = "No" />No</td> </tr> <tr> <td colspan = "2"><input type = "submit" value = "Play!" /></td> </tr> </table> </form> _END if ($ready == "Yes"){ rock_paper_scissors_lizard_spock(); }else{ close_rpsls(); } } // Human Play Selection function human_play() { echo <<<_END <form method = "post" action = "rpsls.php"> <h2>Let's Play Rock, Paper, Lizard, Spock</h2> <hr> <table> <tr> <td><input type = "radio" name = "human" value = "Rock" />Rock</td> <td><input type = "radio" name = "human" value = "Paper" />Paper</td> </tr> <tr> <td><input type = "radio" name = "human" value = "Scissors" />Scissors</td> <td><input type = "radio" name = "human" value = "Lizard" />Lizard</td> </tr> <tr> <td colspan = "2"><input type = "radio" name = "human" value = "Spock" />Spock</td> </tr> <tr> <td colspan = "2"><hr></td> </tr> <tr> <td colspan = "2"><input type = "submit" value = "Play!" /></td> </tr> </table> </form> _END return $human; } // Computer Play Selection function computer_play() { $play = rand(1,5); $query = "SELECT choice FROM choices WHERE number = $play"; $computer = mysql_query($query); return $computer; } // Game Outcome Function function game_outcome($human, $computer) { $win = $loss = $draw = 0 if ($human == $computer){ echo "Draw<br />"; echo "We both played ".$human; $draw = 1; }else{ $query = "SELECT outcome, action FROM rpsls WHERE human = $human AND computer = $computer"; $results = mysql_query($query); $results2 = mysql_fetch_array($results); $outcome = $results2[0]; $action = $results2[1]; if ($outcome == "Win"{ echo "You Win!!!<br />" echo "Your ".$human. " ".$action." my ".$computer."<br />"; $win = 1; }else{ echo "You Lose/.<br /> echo "My ".$computer." ".$action." your ".$human."<br />"; $loss = 1; } } $query = "INSERT INTO gameResults VALUES".(NULL, '$win', '$loss', '$draw')"; mysql_query($query); } // Game Statistics Function function game_statistics () { $query = "SELECT * FROM gameResults"; $result = mysql_query($query); $rows = mysql_num_rows($result); $games = $rows; $win = $loss = $draw = 0; for ($index = 0; $index < $rows; ++$index){ $row = mysql_fetch_row($result); $win = $win + $row[1]; $loss = $loss + $row[2]; $draw = $draw + $row[3]; } echo <<<_END <table> <tr> <td>Games</td> <td>Win</td> <td>Loss</td> <td>Draw</td> </tr> <tr> <td>$games</td> <td>$win</td> <td>$loss</td> <td>$draw</td> </tr> </table> _END } // Print Statistics and close the game function close_rpsls(){ echo <<<_END <form method = "post" action = "rpsls.php"> <h3>Are you sure you want to quit?</h3> <hr> <table> <tr> <td><input type = "radio" name = "ready" value = "Yes" />Yes</td> <td><input type = "radio" name = "ready" value = "No" />No</td> </tr> <tr> <td colspan = "2"><input type = "submit" value = "Play!" /></td> </tr> </table> </form> _END if ($ready == "No"){ rock_paper_scissors_lizard_spock(); }else{ $query = "DROP TABLE gameResults"; mysql_query($query); } } // close connection mysql_close($conn); ?> Please forgive my novice errors and help me figure out what is wrong with this program. Thank you. Here is the contents of the error log: 141104 18:36:26 mysqld_safe Starting mysqld daemon with databases from /Applications/MAMP/db/mysql 141104 18:36:28 [Warning] Setting lower_case_table_names=2 because file system for /Applications/MAMP/db/mysql/ is case insensitive 141104 18:36:28 [Note] Plugin 'FEDERATED' is disabled. 141104 18:36:28 InnoDB: The InnoDB memory heap is disabled 141104 18:36:28 InnoDB: Mutexes and rw_locks use GCC atomic builtins 141104 18:36:28 InnoDB: Compressed tables use zlib 1.2.3 141104 18:36:28 InnoDB: Initializing buffer pool, size = 128.0M 141104 18:36:28 InnoDB: Completed initialization of buffer pool 141104 18:36:28 InnoDB: highest supported file format is Barracuda. 141104 18:36:32 InnoDB: Waiting for the background threads to start 141104 18:36:33 InnoDB: 5.5.38 started; log sequence number 1711074 141104 18:36:33 [Note] Server hostname (bind-address): '0.0.0.0'; port: 8889 141104 18:36:33 [Note] - '0.0.0.0' resolves to '0.0.0.0'; 141104 18:36:33 [Note] Server socket created on IP: '0.0.0.0'. 141104 18:36:35 [Note] Event Scheduler: Loaded 0 events 141104 18:36:35 [Note] /Applications/MAMP/Library/bin/mysqld: ready for connections. Version: '5.5.38' socket: '/Applications/MAMP/tmp/mysql/mysql.sock' port: 8889 Source distribution