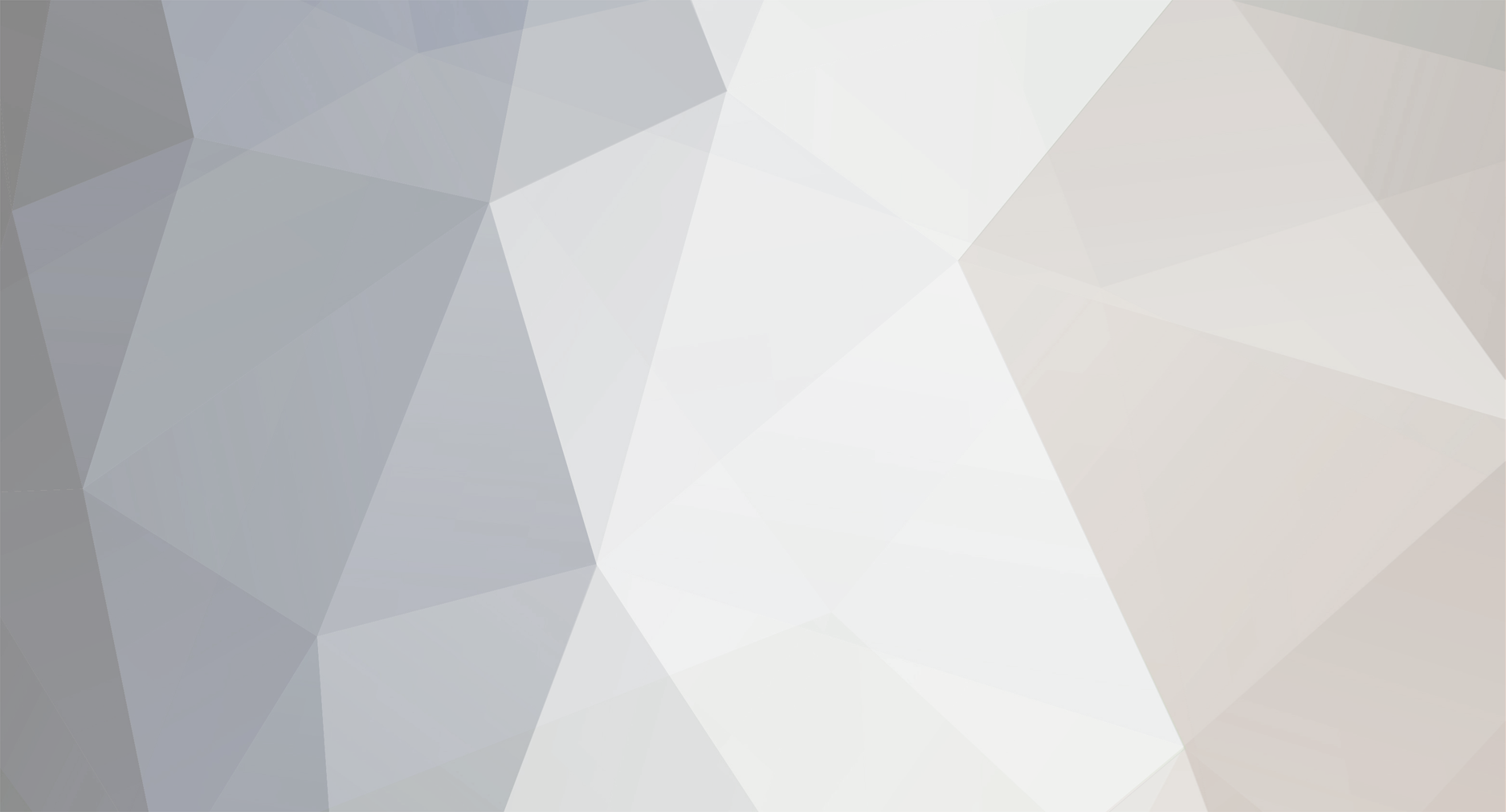
Tom10
-
Posts
108 -
Joined
-
Last visited
Posts posted by Tom10
-
-
I have turned on error reporting and switched to PDO, However i still recieve a blank page when logging in
<?php require('./includes/connect.php'); error_reporting(E_ALL); if ($_SERVER['REQUEST_METHOD'] == "POST") { $username = $_POST['username']; $username = htmlentities($username); $password = $_POST['password']; $query = $mysqli->prepare("SELECT username FROM apna_users WHERE username=?"); $query->bindParam('?', $username, PDO::PARAM_STR, 50); $query->execute(); $row = $query->fetch(PDO::FETCH_ASSOC); if (password_verify($password, $row['password']) && $query->num_rows() > 0) { echo "Login Successful"; } else { echo "Login Failed."; } } ?> <html> <title>Apna Bhaiii - Login</title> <body> <center> <div id="login"> <h1>Login to your account</h1><br> <form action="" method="POST"> <h3>Username:</h3> <input type="text" name="username" placeholder="Enter your username" /> <br> <h3>Password:</h3> <input type="password" name="password" placeholder="Enter your password" /> <br><br> <input type="submit" name="loginbtn" value="Log In" /> <br> </form> <h3>Don't have an account? <a href="register.php">Create one today</a></h3> </div> </center> </body> </html>
-
Ok thanks @Jacques1 and @Barand
-
The input password will not match that in the user table, so your selection criteria should just be where the username matches. Then verify the retrieved password hash.
I have changed that,
if ($query = $mysqli->prepare("SELECT username, password FROM apna_users WHERE username=?")) { $query->bindParam("username", $username); $query->execute(); $result->fetch(); }
if (password_verify($password, $result['password']) && $result->num_rows() > 0) { ?> <html> <h2>Login Successful</h2> </html> <?php } else { ?> <html> <h2>Login Failed</h2> </html> <?php }
When i login, nothing is displayed it's just a blank page.
-
<?php require('./includes/connect.php'); error_reporting(E_ALL | E_NOTICE); if ($_SERVER['REQUEST_METHOD'] == "POST") { $username = $_POST['username']; $username = htmlentities($username); $password = $_POST['password']; if ($query = $mysqli->prepare("SELECT username, password FROM apna_users WHERE username=? AND password=?")) { $query->bindParam("username", $username); $query->bindParam("password", $password); $query->execute(); $query->bind_result($result); $result->fetch(); } if (password_verify($password, $result['password']) && $result->num_rows() > 0) { ?> <html> <h2>Login Successful</h2> </html> <?php $query->close(); } else { ?> <html> <h2>Login Failed</h2> </html> <?php $query->close(); } } ?> <html> <title>Apna Bhaiii - Login</title> <body> <center> <div id="login"> <h1>Login to your account</h1><br> <form action="" method="POST"> <h3>Username:</h3> <input type="text" name="username" placeholder="Enter your username" /> <br> <h3>Password:</h3> <input type="password" name="password" placeholder="Enter your password" /> <br><br> <input type="submit" name="loginbtn" value="Log In" /> <br> </form> <h3>Don't have an account? <a href="register.php">Create one today</a></h3> </div> </center> </body> </html>
I have made those changes is this any better?, forgive me if i have mistakes in the code i am quite new to coding just trying to get my head around it
-
Hello, I am having issues with the login system that i am currently working on, it is showing login failed on the page when the login details for the user are correct.
Login.php
<?php require('./includes/connect.php'); error_reporting(E_ALL | E_NOTICE); if ($_SERVER['REQUEST_METHOD'] == "POST") { $username = $_POST['username']; $username = htmlentities($username); $password = $_POST['password']; $password = password_hash($password, PASSWORD_BCRYPT); $query = "SELECT username, password FROM apna_users WHERE username='$username' AND password='$password'"; $result = mysqli_query($mysqli, $query); $row = $result->fetch_array(); if (password_verify($password, $row['password']) && $result->num_rows() > 0) { ?> <html> <h2>Login Successful</h2> </html> <?php } else { ?> <html> <h2>Login Failed</h2> </html> <?php } } ?> <html> <title>Apna Bhaiii - Login</title> <body> <center> <div id="login"> <h1>Login to your account</h1><br> <form action="" method="POST"> <h3>Username:</h3> <input type="text" name="username" placeholder="Enter your username" /> <br> <h3>Password:</h3> <input type="password" name="password" placeholder="Enter your password" /> <br><br> <input type="submit" name="loginbtn" value="Log In" /> <br> </form> <h3>Don't have an account? <a href="register.php">Create one today</a></h3> </div> </center> </body> </html>
Register.php (The register script works perfectly)
<?php require('./includes/connect.php'); if($_SERVER['REQUEST_METHOD'] == "POST") { $email = $_POST['email']; $email= filter_var($email, FILTER_VALIDATE_EMAIL); $username = $_POST['username']; $username = htmlentities($username); $password = $_POST['password']; $cpassword = $_POST['cpassword']; if (!filter_var($email) || empty($username)) { echo "<b>Email address is invalid.</b>"; } if (empty($username)) { echo PHP_EOL . "<b>Username is empty</b>"; } if (empty($password)) { echo PHP_EOL . "<b>Password is empty or invalid</b>"; } if($cpassword != $password) { die("The passwords do not match!"); } $enc_password = password_hash($password, PASSWORD_BCRYPT); if (mysqli_query($mysqli, "INSERT INTO apna_users (email, username, password) VALUES ('$email', '$username', '$enc_password')")) { echo "Your account has been successfully created."; echo '<meta http-equiv="refresh" content="1;login.php">'; exit(); } else { echo "An error has occured whilst creating your account, please try again later." . PHP_EOL . "If the problem persists please contact support."; } } ?> <html> <title>Apna Bhaiii - Register</title> <style> input {padding: 10px; border-radius: 20px; } #registerbtn1 input {width: 400px;} </style> <body> <center> <div id="register"> <h1>Create your account</h1><br> <form action="" method="POST"> <h3>E-mail Address:</h3> <input type="text" name="email" placeholder="Enter your E-Mail Address" required /> <br> <h3>Username:</h3> <input type="text" name="username" placeholder="Enter your username" required /> <br> <h3>Password:</h3> <input type="password" name="password" placeholder="Enter your password" required /> <br> <h3>Confirm Password:</h3> <input type="password" name="cpassword" placeholder="Confirm your password" required /> <br><br> <input type="submit" name="registerbtn" id="registerbtn1" value="Create" /> <br> </form> </div> </center> </body> </html>
Does anyone know why it is doing this?,
Thanks
-
<a href="<?php echo htmlspecialchars(htmlspecialchars($_SERVER['PHP_SELF'])) . '?page=manage&new='.hash('ripemd128', rand()).'' ?>"> + New User </a>
Hi i'm trying to load a create page by adding &new= to the url but it won't work if i create case 'new': ?
-
Because $res IS an array of all usernames, which is what your query asked for by using fetchAll(). So you'd need to iterate over that array.
foreach($res as $user) { echo $user['username'] . "<br>"; }
Ah i get you
-
Hi i'm trying to echo all usernames from sql to html my code is working but it's echoing to the page as an array
$select = "SELECT username FROM users"; $stmt = $handler->prepare($select); $stmt->execute(); if($stmt->rowCount()) { $res = $stmt->fetchAll(); print_r($res); }
-
Depends on what you need to search for, but let's say you want to search for the users last name.
Create a form with an input for the last name. When submitting the form, just do a search for the last name in the db using WHERE lastname = 'lastname_from_form' to get an exact match, or WHERE lastname LIKE 'lastname_from_form%' to get all that start with lastname_from_form (the % at the end is a wildcard).
Then display the list of matched users
Thanks, CroNiX i will try this!
-
Hi, i've never created a search in php so i'm not exactly sure on the method you can use do create a search, basically i have created an admin panel and it has a list of users but if there are too many users i will need to create a search.
Please can someone tell me how i can do this?
Thanks in advance!
-
Not sure but there is one thing that should change.
The following should change:
.. .. $stmt->execute(); $row = $stmt->fetchAll(); if($stmt->rowCount()) { if($row['rank'] == 1) .. ..
to this:
$stmt->execute(); if($stmt->rowCount()) { $row = $stmt->fetch(); if($row['rank'] == 1) .. ..
Note the use of rowcount to determine what to do next and also the use of 'fetch' instead of fetchall. Fetchall will produce an array of rows whereas you only want one row of data to work with here (and should have only one row).
If all you want to do is to exit this script and go somewhere else, why the meta echo? Just call header("Location: $url") to go where you want.
Hi i just changed the code and it still does the same thing, but the reason i used meta echo is incase a browser has headers disabled.
-
I am trying to get my account to redirect to admin.php but it's not working it just goes to user.php.
here is my code
<?php @ini_set('display_errors', 1); @error_reporting(1); @ini_set('allow_url_include', Off); @set_time_limit(0); session_start(); require 'connect.php'; if($_SERVER['REQUEST_METHOD'] == "POST") { if(!isset($_POST['token'])) { die("Possible Attack!"); } $username = $_POST['username']; $password = $_POST['password']; $username = htmlspecialchars($username); $username = htmlentities($username); $username = strip_tags($username); if(preg_match("#[^\w]#", $username)) { die("Your username must be numbers or letters only!"); } $hash = hash('ripemd320', $password); if(empty($username) || empty($password)) { die("Please enter both your username and password!"); } $sql = "SELECT username, password, rank FROM users WHERE BINARY username = :username AND BINARY password = :password"; $stmt = $handler->prepare($sql); $stmt->bindParam(':username', $username, PDO::PARAM_STR, 12); $stmt->bindParam(':password', $hash, PDO::PARAM_STR, 12); $stmt->execute(); $row = $stmt->fetchAll(); if($stmt->rowCount()) { if($row['rank'] == 1) { $_SESSION['loggedIn'] = 1; $_SESSION['username'] = $username; $_SESSION['rank'] = $row['rank']; echo '<meta http-equiv="refresh" content="0;admin.php">'; } $_SESSION['loggedIn'] = 1; $_SESSION['username'] = $username; $_SESSION['rank'] = $row['rank']; echo '<meta http-equiv="refresh" content="0;user.php">'; } else { die("Username or Password is incorrect!"); } } ?>
I appreciate any help at all
-
yeah i figured that out before i don't know how i didn't spot that prepare error
-
$sql_string = "INSERT INTO users SET username = :a, password = :b"; $sql->prepare($sql_string); $sql->bindParam(':a', $username, PDO::PARAM_STR, 50); $sql->bindParam(':b', $hash, PDO::PARAM_STR, 30); $sql->execute();
I've done exactly what the documentation says.
-
we are really trying to help you, but when you don't bother to read the php.net documentation and its examples for the statements you are trying to use and really learn what each statement does, and in this case learn what each statement returns as a value, it's not possible for you to write code that does anything. you are operating in an uncontrolled random trial (and mostly error) mode, where you are not using the documentation as an input to determine the correct way of using any statement.
I do read the documentation i just don't understand their bindParam tutorial
-
I'm now getting this error
Fatal error: Call to undefined method PDO::bindParam() in C:\xampp\htdocs\register.php on line 41
this is the code i have updated:
$sql_string = "INSERT INTO users SET username = :a, password = :b"; $sql->prepare($sql_string); $sql->bindParam(':a', $username); $sql->bindParam(':b', $hash); $sql->execute(); if($sql->query($sql_string) === TRUE) { echo "Your account has been successfully created!"; }
-
-
I am getting the following error
Fatal error: Call to a member function bind_param() on string in C:\xampp\htdocs\register.php on line 40
Here is my script:
<?php require 'connect.php'; error_reporting(E_ALL | E_NOTICE); if($_SERVER['REQUEST_METHOD'] == "POST") { $username = $_POST['username']; $password = $_POST['password']; $cpassword = $_POST['cpassword']; if($cpassword !== $password) { die("Passwords do not match!"); } $username = htmlspecialchars($username); $username = htmlentities($username); $username = strip_tags($username); if(preg_match("#[^\w]#", $username)) { die("Your username must be numbers or letters only!"); } $hash = hash('ripemd320', $password); if(empty($username) || empty($password)) { die("Please enter both your username and password!"); } $sql = "INSERT INTO users (username, password) VALUES ($username, $password)"; $sql->bind_param("ss", $username, $password); $sql->execute(); if($handler->query($sql) === TRUE) { echo "Your account has been successfully created!"; } } ?>
-
ok thanks, and this is my connection script
<?php try { $handler = new PDO("mysql:host=localhost;dbname=test;", "root", ""); $handler->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); } catch (PDOException $e) { echo "A connection could not be established to ".$_SERVER['SERVER_ADDR']." The following error has occurred: ".$e->getMessage()." "; } ?>
-
Did you connect to your database and assign $stmt? You don't check any results so you can't be sure, can you?
I have required the connect file and
$stmt = $handler->prepare($sql); $stmt->bind_param("ss", $username, $hash); $stmt->execute();
-
<?php @ini_set('display_errors', 1); @error_reporting(1); @ini_set('allow_url_include', Off); @set_time_limit(0); require 'connect.php'; if($_SERVER['REQUEST_METHOD'] == "POST") { $username = $_POST['username']; $password = $_POST['password']; $username = htmlspecialchars($username); $username = htmlentities($username); $username = strip_tags($username); if(preg_match("#[^\w]#", $username)) { die("Your username must be numbers or letters only!"); } $hash = hash('ripemd320', $password); if(empty($username) || empty($password)) { die("Please enter both your username and password!"); } $sql = "SELECT username, password FROM users WHERE BINARY username = :username AND BINARY password = :password"; $stmt = $handler->prepare($sql); $stmt->bind_param("ss", $username, $hash); $stmt->execute(); if($stmt->rowCount()) { if($row['rank'] == 1) { $_SESSION['loggedIn'] = 1; $_SESSION['username'] = $username; $_SESSION['rank'] = $row['rank']; echo '<meta http-equiv="refresh" content="0;admin.php">'; } $_SESSION['loggedIn'] = 1; $_SESSION['username'] = $username; $_SESSION['rank'] = $row['rank']; echo '<meta http-equiv="refresh" content="0;user.php">'; } } ?>
I'm getting the following error Call to undefined method PDOStatement::bind_param()
-
<?php session_start(); // Store Session Data $_SESSION['login_user'] = $username; // Initializing Session with value of PHP Variable echo $_SESSION['login_user']; ?>
session_start doesn't store session data it starts the session, the $_SESSION function stores session data.
By the looks of it you are trying to store the username on login and echo it into the index page?
Try this:
login
<?php session_start(); //Start session //This is only an example you store the session data when you are logging in if($count->rowCount > 0) { //Store session data $_SESSION['username'] = $username; } ?>
index
<?php session_start(); if(isset($_SESSION['username'])) { } else { echo "Couldn't set session!"; } ?>
Then you can use
<?php echo $username; ?>
-
if ($_SESSION['tarjouskori']) { // Estetään tuplasähköpostit. $message = "Lähettäjä: ".$_POST[billto_first_name]."\n<br>"; $message.= "Puhelin: ".$_POST[billto_phone_number]."\n<br>"; $message.= "Sähköposti: ".$_POST[billto_email]."\n<br>"; $message.= "Lisätoiveet: ".$_POST[special_requests]."\n\n<br>"; $message.= "Tuotteet\n<br><br>";
".$_POST['billto_first_name']."
-
Sorry about that i'm still learning PHP
PHP Login System
in PHP Coding Help
Posted
I keep getting this error