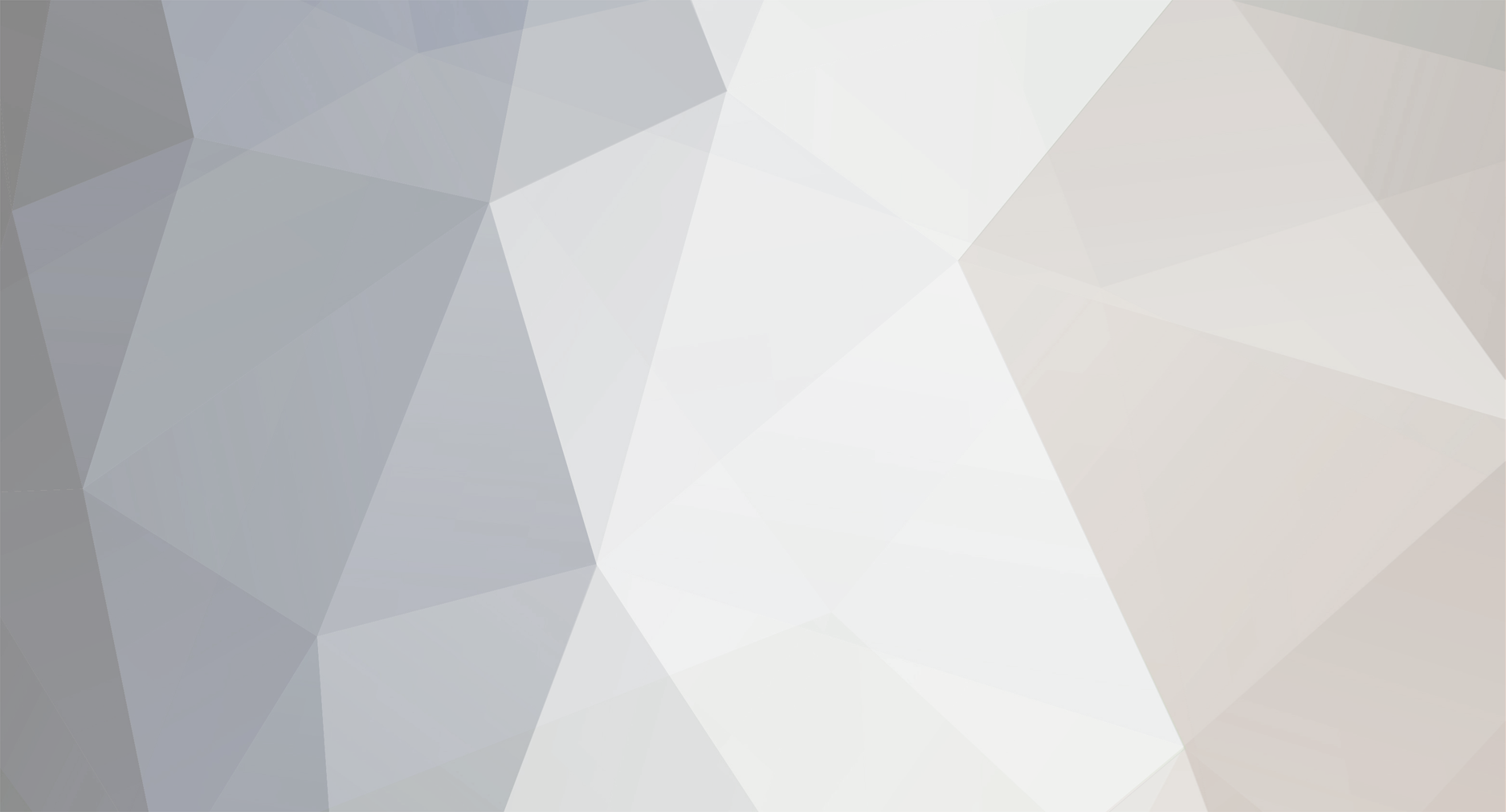
Tom10
-
Posts
108 -
Joined
-
Last visited
Posts posted by Tom10
-
-
Hello, So i'm making a register script and the values are not inserting here is my script.
if(isset($_POST['register'])) { $username = $_POST['username']; $password = $_POST['password']; $cpassword = $_POST['cpassword']; $username = htmlentities($username, ENT_QUOTES); $password = htmlentities($password, ENT_QUOTES); $cpassword = htmlentities($cpassword, ENT_QUOTES); $username = htmlspecialchars($username, ENT_QUOTES); $password = htmlspecialchars($password, ENT_QUOTES); $cpassword = htmlspecialchars($cpassword, ENT_QUOTES); $username = mysqli_real_escape_string($con, $username); $password = mysqli_real_escape_string($con, $password); $cpassword = mysqli_real_escape_string($con, $cpassword); $username = strip_tags($username); $password = strip_tags($password); $cpassword = strip_tags($cpassword); $cpassword = hash('ripemd128', $cpassword); $denymsg = "<h3>The username or password you have entered has been rejected. Check their are not illeagal characters, ie. code, special characters etc. </h3>"; if(preg_match("#[^\w\?\&\=\.]#", $username)) { echo $denymsg; die(); } else { } if(preg_match("#[^\w\?\&\=\.]#", $password)) { echo $denymsg; } else { } if($password !== $_POST['cpassword']) { die("Passwords do not match!"); } if(!$username OR !$password) { die("Make sure you have entered a username and password!"); } $sql = "INSERT INTO `users` (username, password) VALUES ('$username', '$cpassword')"; if($sql === TRUE) { echo "Your account (".$username.") has been created!"; } else { echo "Your account (".$username.") could not be created. "; echo "<br> <br> ".var_dump($sql)." "; } }
I do not get any errors, but here is the result of the variable dump
Your account (user) could not be created. string(92) "INSERT INTO `users` (username, password) VALUES ('user', '602cb6acf8f1d5a8c402bc6b9505730f')"
-
The ^ character inside of a character class ([ ]) inverts the pattern. This is a way of creating a whitelist of allowed characters. If $fullURL contains characters that are outside of the allowed whitelist, then the pattern will match and run the code inside the conditional.
Hopefully that makes sense.
Thank you so much, scootstah.
That made a lot of sense and cleared that up for me
-
Hi, i don't have a problem with this code it works fine, but i don't understand it.
if(preg_match("#[^\w\/\&\\?\=\.]#", $fullURL)) { include 'deny.php'; die(); } else { }
My understanding is that \w includes a-z Z-A so i don't understand when i load the page it doesn't instantly go to deny.php it make's sense to put the include 'deny.php' and die(); in else?
Not sure if you understand what i mean, please say if you don't i will try to explain what i mean better.
-
Characters like < get encoded to something like '%3C'. So, they wouldn't be caught in a preg_match check. You need to be sure to not convert them back to their native characters. But, this should work for what you described:
$fullURL = $_SERVER['SERVER_NAME'] . $_SERVER['REQUEST_URI'] . ''; echo "Full URL: {$fullURL}<br>"; if(preg_match("#[^\w\/\?\&\.\=]#", $fullURL)) { echo "Security error"; } else { echo "URL OK"; }
That regex covers:
\w = a-z, A-Z, 0-9 & _ (underscore)
/ (forwardslash)
? (question mark)
& (ampersand)
. (period)
= (equals sign)
Worked like a charm mate thank you so much
-
So, what are you considering malicious code? I.e. what code are you wanting to allow vs code you don't want to allow?
I am wanting to block out javascript attacks like
index.php?=<script>onload=alert(document.cookie);</script>
It's mainly i'm wondering how to detect using preg match if someone has entered this code or something similar
-
Hey i am trying to use preg_match with my website URL
$url = "".$_SERVER['SERVER_NAME']."".$_SERVER['REQUEST_URI'].""; if(preg_match('#([\^A-Za-z0-9\$]#', $url)) { } else { die("<h1>Security error</h1>"); }
I want to kill the page and say "Security Error" if a client tries to inject code into the url, but i can't get this to work.
All help is very much appreciated
,
Thanks
-
When it comes to security i always use a variety of functions when handling data, forms etc.
Example:
<?php $username = $_POST['username']; $password = trim($_POST['password']); $username = htmlspecialchars($_POST['username']); $password = htmlspecialchars($_POST['password']); $username = mysqli_real_escape_string($con, $username); $password = mysqli_real_escape_string($con, $password); $username = stripslashes($_POST['username']); $password = stripslashes($_POST['password']); $password = hash('ripemd128', $password); $username = strip_tags($username); $password = strip_tags($password); $username = filter_var($username, FILTER_SANITIZE_STRING, FILTER_FLAG_ENCODE_HIGH); $password = filter_var($password, FILTER_SANITIZE_STRING, FILTER_FLAG_ENCODE_HIGH); $username = htmlentities($username, ENT_QUOTES); $password = htmlentities($password, ENT_QUOTES); ?>
-
Your preparing the statement and executing it straight away, You are ment to bind the values and you have not got the result from the query
$q = 'SELECT * FROM users WHERE username=:username AND password=:password'; $query = $dbh->prepare($q); $query->execute(array(':username' => $username, ':password' => $password)); if($query->rowCount() == 0){ header('Location: index.php?err=1');
This won't work because you haven't asked for results. You need to read up on Prepared Statements
Also you are vulnerable to cross site scripting (XSS) and SQL Injection.
-
Yeah like CroNiX said, before continuing on check the query has actually succeeded.
if($sql === TRUE) { //Query was successful, Execute code } else { var_dump($sql); //The Query Failed, dump the data }
-
Make the form in HTML and process it in PHP, sanitize data, check a value has been entered etc.
-
Hi
Am getting this error in my php code
Deprecated: mysql_connect(): The mysql extension is deprecated and will be removed in the future: use mysqli or PDO instead in C:\wamp\www\matu\connect.php on line 9
the line is $con=mysql_connect("$host", "$username", "$password")or die("cannot connect");
Yes like cyberRobot said, mysql functions are now deprecated (out of date) they may be removed from PHP in the future, you are using mysql_connect()
Here's a mysqli example:
$host = "localhost"; $username = "dbUser"; $password = "NULL"; $db_name = "dbName"; $conn = mysqli_connect($host, $username, $password, $db); if($conn->connect_error()) { //If there is an error while connecting to SQL server //Execute code ie: echo $conn->connect_errono(); //Display Error Number } else { //Do Nothing }
Or like cyberRobot also said you can use PDO
-
<?php error_reporting(E_ALL | E_NOTICE); ini_set('display_errors', '1'); require_once("./include/membersite_config.php"); if(!$fgmembersite->CheckLogin()) { $redir_index = "<meta http-equiv='refresh' content='0;index.php'>"; $fgmembersite->$redir_index(); exit; } if($fgmembersite->UserId() == 1261){ $redir_test = "<meta http-equiv'refresh' content='0;testes.php'>"; $fgmembersite->$redir_test(); exit; } $userid = $fgmembersite->UserId(); ?>
Try this
-
Have you tried using preg_match / Regular Expressions to take GCSE and Biology out of the URL and then echo the result?
-
The only thing i can think of is make a cookie, that never expires unless the client clears their browsing cache, history etc.
-
Also, you are vulnerable to SQL injection
//Get name $name = $_GET['name']; $check = $conn->query("select $element_col from name_table WHERE name=$name");
Sanitize the data with functions like real_escape_string, htmlspecialchars and htmlentities because you are also vulnerable to Cross Site Scripting.
i.e
//Get name $name = $_GET['name']; $name = htmlentities($name, ENT_QUOTES); $name = htmlspecialchars($name, ENT_QUOTES); $name = mysqli_real_escape_string($conn, $name);
If you are still getting errors after the advice you have been given then, use var_dump() and post the output on here.
var_dump($check);
-
the reason you are getting errors at the mysqli_num_rows() statement, is because your program logic is not correct and your query is failing due to an error of some kind. the $check variable will be a false value when the query fails due to an error. you are then trying to use that false value in the mysqli_num_rows($check) statement. your program logic should be testing if $check is a true value, without the !.
the reason your query is failing, is because you don't have single-quotes around the '$name' variable inside the sql statement.
finally, you don't need all that program logic anyway. you should not have a database table laid out like that. the data is not normalized, requiring you to write a ton of program logic to find, insert, update, or delete any of the data. you should instead have one row for each same meaning data item, not columns in a row for each same meaning data item.
I agree
if(!check) { }
That won't work, I would do:
if($check === FALSE) { }
or
if($check !== TRUE) { }
-
<?php $url = 'http://' . $_SERVER['SERVER_NAME'] . $_SERVER['REQUEST_URI']; $findMe = array('@', '/', '&', '$', '"', '!', '<', '(', ')', '{'); if (false !== strpos($url, '')) { echo 'Fail!'; } else { } ?>
How can i search for more than one character?
I have got the error
Notice: Array to string conversion in C:\xampp\htdocs\test.php on line 7
-
Yeah but i mean how can i use strpos for the url of my website, i know how to use it with normal html input
-
Like how would i be able to use PHP to detect if they have actually tried to inject malicious code into the url and if so redirect them?
-
Some advice: don't try to detect bad input. You will not be able to protect yourself from everything that way.
Instead just deal with it safely. Output into HTML should use functions like htmlspecialchars and occasionally (raw)urlencode.
People entering PHP code should be perfectly fine because you should never, ever be attempting to execute it. If they want to provide a bad URL like that then it's okay because all you're going to do is output it or maybe redirect people to it, and both of those cases are very easy to protect yourself against. Here's a demonstration:
$url = "http://corruptsecurity.net/chat.php?<?php file_put_contents() ?>"; echo "<html> <head> <title>Redirecting...</title> <meta http-equiv='Refresh' content='10;url=", htmlspecialchars($url), "'> </head> <body> <p>Redirecting you to <a href='", htmlspecialchars($url), "'>", htmlspecialchars($url), "</a>...</p> <script type='text/javascript'> window.setTimeout(function() { document.location = ", json_encode((string)$url), "; }, 3000); </script> </body> </html>";
In the url variable though how can i redirect them to a specific page if they enter malicious code into the url
-
-
preg_match() has to do with regular expressions. It's not a solution to a particular problem but a tool you can use, so using it "properly" depends on what you're using it for. You could use it to validate simple things like usernames or complex things like URLs.
Explain what "detect characters or keywords in the local URL" means.
What i want to do with preg match is detect certain keywords or characters in the URL, it's like with mod security if you enter in the URL <script>onload=alert);</script>
It comes up with 512 security error
I want to use preg_match to detect the keywords or characters that someone enters into the URL and then redirect them or kill the page.
-
Thank you
The first error has gone but i still have the second
<?php error_reporting(E_ALL | E_NOTICE); ini_set('display_errors', '1'); $iduser= $_GET['userid']; include 'conn.php'; mysql_query("SET NAMES 'utf8'"); $rs = mysql_query('SELECT `pae_atividades`.`idativade` , `pae_cargo`.`cargo` , `pae_atividades`.`atividade` , `pae_atividades`.`data` , `pae_atividades`.`hora` , `pae_atividades`.`local` , `pae_atividades`.`inter` , `pae_atividades`.`notas` , `utilizador`.`nome` FROM `ebspma_paad_ebspma`.`pae_atividades` INNER JOIN `ebspma_paad_ebspma`.`utilizador` ON ( `pae_atividades`.`idutilizador` = `utilizador`.`idutilizador` ) INNER JOIN `ebspma_paad_ebspma`.`pae_cargo` ON ( `pae_atividades`.`idcargo` = `pae_cargo`.`idcargo` ) WHERE `pae_atividades`.`data` >= CURDATE( ) AND `pae_atividades`.`idutilizador`= $iduser ORDER BY `pae_atividades`.`data` ASC '); $result = array(); while($row = mysql_fetch_object($rs)){ array_push($result, $row); } echo json_encode($result); ?>
Line 14
Warning: mysql_fetch_object(): supplied argument is not a valid MySQL result resource in /home/ebspma/public_html/atividades/get_atividadesByUserId.php on line 14
[]
mysql is deprecated you should use mysqli, have you tried
while($row = mysql_fetch_object($result)){ array_push($result, $row); }
Your trying to get the result from the query without actually getting the results
Also,
$result = $rs->fetch();
-
Hi, so i'm currently looking into security in PHP and i have looked at the preg_match function on PHP.net and i don't fully understand how it works or how to use it properly for example i don't know how i would use the function to detect characters or keywords in the local url,
Please can someone explain it to me?
Every response is much appreciated, Thanks
If Get Id from mysql db change id to a word !
in PHP Coding Help
Posted
Me too i'm not quite sure what your asking.