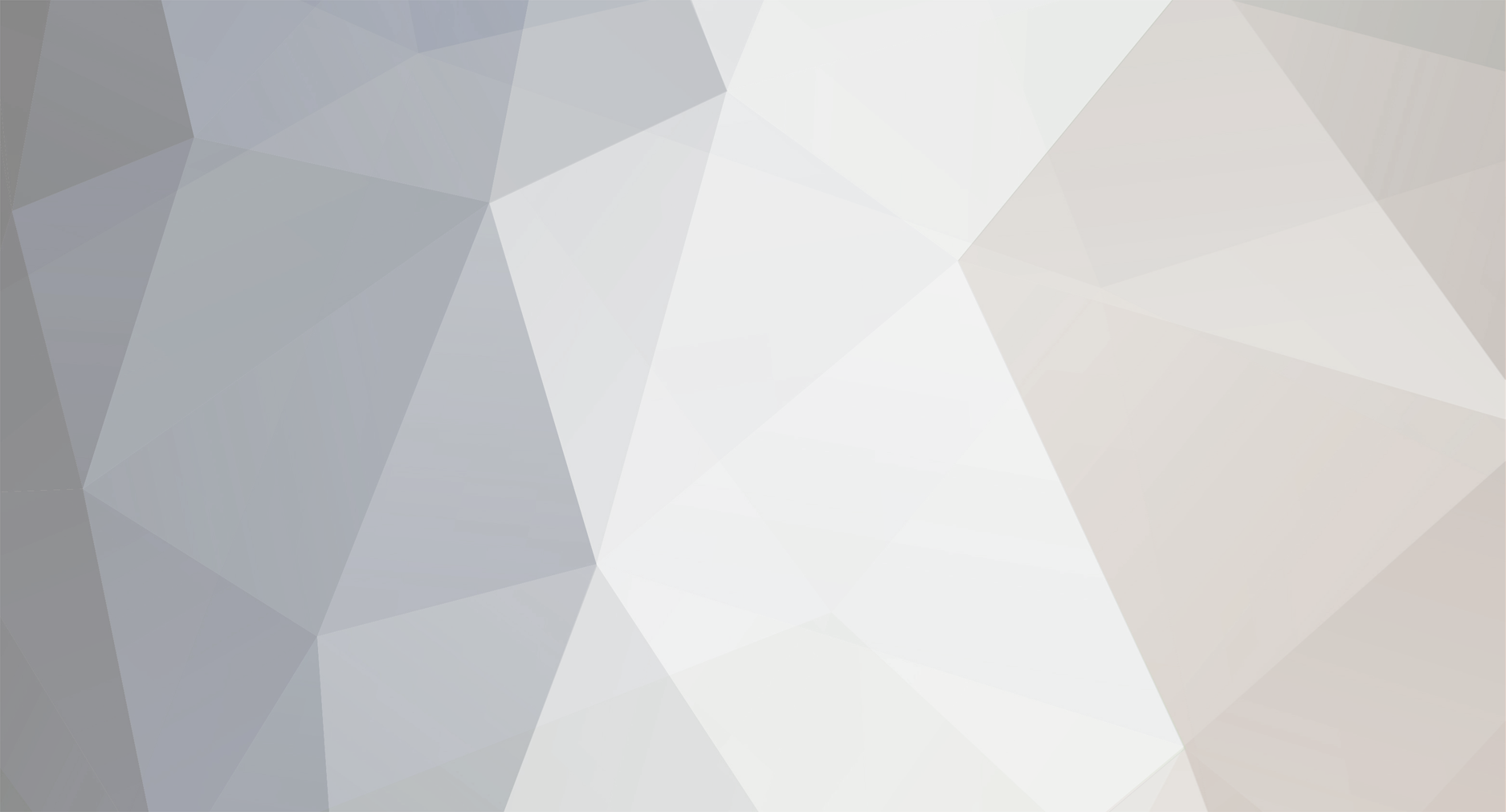
play_
-
Posts
717 -
Joined
-
Last visited
-
Days Won
1
Posts posted by play_
-
-
Hi.
I retrieve news from my database.
and to print out, i use echo nl2br($row['news']).
But it doesnt work.
Then, i click "edit news" which takes me to a form with the news as the value in the text area.
Now i see the news with <br> where i had pressed enter when i submitted.
So all i have to do is click submit and itll update the database with the new news.
however, that doesnt sound right. I shouldnt have to submit the news, then edit it for the nl2br to work.
Does anyone know what's wrong?
-
Edit. Found a solution. i had to type cast the paremeter.
in case anyone plan on using this script, here is the correct form:
[code] function disable(id) {
var obj = document.getElementById(id);
obj.value='Loading. . .';
obj.disabled=true;
}
function dis(num) {
id = Number(num)
setTimeout('disable(id)', 1)
}[/code]
enjoy -
I made a function that, calls another function after 1milisecond (its being used on a submit button, so when the button is pressed, it disables).
Anywyas, if i use this format, it works:
[code]
function disable(id) {
var obj = document.getElementById(id);
obj.value='Loading. . .';
obj.disabled=true;
}
function dis() {
setTimeout('disable(1)', 1000)
}[/code]
and the button:
<input type="submit" name="submit" value="Add this shirt" onmouseover="dis(1)" id="1" />
However, this is no good because on the 'dis' function, i hand-coded the '1' in there.
So ive been trying to pass a paremeter to dis(), and use that paremeter on:
setTimeout('disable(1)', 1000) <--- 1 here would be the paremeter passed.
So i tried this:
[code]
function disable(id) {
var obj = document.getElementById(id);
obj.value='Loading. . .';
obj.disabled=true;
}
function dis(num) {
setTimeout('disable(num)', 1000)
}[/code]
That way, dis() would call disable() with the paremeter of 1.
However, it keeps saying 'num' is not defined.
Any ideas? -
I got 1 hour of sleep last night so bare with me, im not sure i understand.
Your page loads a listing of houses, and each of them has a button, that you would like to say 'no', but when you click, you want it to display 'yes'?
If so, why not have a column in your database, let's say "sold", that can hold either "yes" or "no".
Then, you could retrieve it and put it as the button value like
<input type="submit" value="<?php echo $row['sold']; ?>">
Is that it? sorry if im way off. -
post errors.
and try
[code]
echo $INR;
[/code]
instead of
[code]
echo$(INR)
[/code] -
Sometimes it ends up in the bulkmail. try checking it there.
-
Just to be on the safeside, ill add 2 lines of code to high - tek's post.
[code]
<?php
ob_start();
$var=1;
$vat++;
header("Location: index.php");
ob_end_flush();
?>
[/code] -
Sorry, forgot to add.
When the mysql_insert_id is only retrieved once; when the submit button is first clicked. When the page reloads, i skip the query that gets mysql_insert_id.
here's a bit of the code:
(this bit of code only runs once, when i click submit for the first time, then $_SESSION[i] becomes > 1, and does not perform this query)
[code]if ($_SESSION['i'] == 1) {
$query1 = "INSERT INTO shirts (title, type, category, date_added) VALUES ('$title', '$type', '$category', NOW())";
$result1 = mysql_query($query1);
$sql_id = mysql_insert_id();
}[/code]
There are other queries performed, and i need to insert mysql_insert_id into the other query that comes next. So i want to get mysql_insert_id once, and use it again, and again... -
Pretty much, i wanna pass a variable to the same page.
I have a form that does a query, and gets the id (mysql_insert_id), the form leads back to current page($_SERVER['PHP_SELF'])
Anyways, when the page loads, i'd like to echo out the mysql_insert_id.
and here's the thing, i will submit the form a couple more times, and it wont do the query again, but i'd like to print out the mysql_insert_id from when i first clicked submit.
Can i do this without sessions? -
[!--quoteo(post=358327:date=Mar 25 2006, 01:40 PM:name=tgs)--][div class=\'quotetop\']QUOTE(tgs @ Mar 25 2006, 01:40 PM) [snapback]358327[/snapback][/div][div class=\'quotemain\'][!--quotec--]
No that wasn't what I meant. I meant uhm...like using text links to run those.
[/quote]
Yes, the code the other person posted will work.
[code]
<?php
switch($_GET['id']) {
default :
include("defaultpage.php");
break;
case "1" :
include("page1.php");
break;
case "2" :
include("page2.php");
break;
}
?>
[/code]
then on the link, you'd have
[code]echo '<a href="otherpage.php?id=1">click here to go to otherpage.php and do everything under case "1"</a>';[/code] -
[!--quoteo(post=358352:date=Mar 25 2006, 02:55 PM:name=AV1611)--][div class=\'quotetop\']QUOTE(AV1611 @ Mar 25 2006, 02:55 PM) [snapback]358352[/snapback][/div][div class=\'quotemain\'][!--quotec--]
Here is what I'm trying to do, but I need to do it differnt...
<A HREF="<?php s_y_s_t_e_m('c:\links\logoff.exe');?>">Log Off System</a><br/>
I only want it to run when I click the links, but it runs when I load the page every time...
I understand that PHP parsed before HTML, but I expected it to behave differntly I guess...
Do I do a javascript onClick or something? (I Don't know JS)
I added the underscores because the site won't let me post code...
[/quote]
I guess you could make it a button, and then use if(isset($_POST['submit']))... -
Alright i get it.
Thanks. I think this will work out fine -
Hey. Thanks.
I understand what you are saying, and it does make sense.
One thing, on "t-shirt details" table, what would the index key be? Should i give it another index? (detailed_shirt_id)
So let's say i wanna show all the colors and sizes available for "donkey kong" which has the id of lets say, 3.
select * from detailed_shirt_id where shirt_id = 3
then i could order by size and color.
that seems like it'd work, right?
So far, the sites ive made uses 1 to 2 tables at the most, and they were fairly small. so this is a first time approach to a not-so-small database.
-
Hello,
I've decided to go ahead and sell T-shirts online. Ill order blank shirts, print designs on them and sell on my site.
I was making the site and setting up a good/efficient DB boggled me.
K here's how my plan:
2 tables :: men_shirts | women_shirts
Let's say [i]each shirt comes in ONE and only one size[/i]. So for example, i make a shirt called "donkey kong", all sizes would be Yellow. Here's how i have a table set up for this:
men_shirts
------------------------------
shirt_id
name
category
color
small_quantity
medium_quantity
large_quantity
xtralarge_quantity
Let me explain:
shirtid :: self explanatory
name :: the name for the shirt (ex: donkey kong, power up, old skool, etc)
category :: games, geek, humor.
color :: white, black, pink, green
small_quantity :: number of t-shirts sized "S" i ordered
medium_quantity :: number of t-shirts sized "M" i ordered
large_quantity :: number of t-shirts sized "L" i ordered
xtralarge_quantity :: number of t-shirts sized "XL" i ordered
Remember, this is if shirt gets only 1 color.
So on the "add product" page, which will add the shirt to the database to be displayed on the site, id have this:
[a href=\"http://ficti0n.com/work/shirts/upload.php\" target=\"_blank\"]http://ficti0n.com/work/shirts/upload.php[/a]
Ok. Notice how I can select only 1 color, but different quantities for the sizes.
Now, the confusing part:
What if id like to get different colors, for different sizes?
I could add these columns to the table:
small_quantity_blue
small_quantity_white,
small_quantity_pink ....
small_quantity_(keep doing this for every color)
and
medium_quantity_blue
medium_quantity_white,
medium_quantity_pink ....
smallmedium_quantity_(keep doing this for every color)
And same thing for Large and Xtra large.
Then what if i add more sizes and colors? Thatd be more stuff on the table.
Is this the right thing to do if i plan on giving each size different colors?
Or should i make a new table or what?
Then of course, i'd hve a women_shirts table, which would be just like the men's.
Sorry this was rather long.
Thanks for reading! (if anyone actually did) -
[a href=\"http://www.amazon.com/gp/product/0764583328/sr=8-1/qid=1143223758/ref=sr_1_1/104-8906384-9563142?%5Fencoding=UTF8\" target=\"_blank\"]http://www.amazon.com/gp/product/076458332...5Fencoding=UTF8[/a]
-
Anyone? the reason i ask is because i just thought of using a temporary table instead of cookies, for small shopping site i plan on building
-
Can someone tell me a good use for temporary tables?
The only thing i can think of, is for a shopping cart. it could be used for a shopping cart right? -
Anytime.
While im at it, let me give you some more advice.
Instead of using a bunch of IF/ELSE IF statements like you did, use the SWITCH statement.
I'm not sure if it makes your code faster or not, but it makes it easier to read and apparently, its more programmer-like (in other words, looks less newb :) )
so instead of:
[code]
if ($page_type == 1) {
$new_type = "Home"
echo $new_type;
} else if ($page_type == 2) {
$new_type = "Series"
echo $new_type;
} else if ($page_type == 3) {
$new_type = "Movies"
echo $new_type;
} else if ($page_type == 4) {
$new_type = "Special"
echo $new_type;
} else if ($page_type == 5) {
$new_type = "Gaming"
echo $new_type;
} else {
$new_type = "Unknown"
echo $new_type;
}
[/code]
You could/should have:
[code]
$new_type = NULL;
switch ($page_type) {
case 1:
$new_type = "Home";
echo $new_type;
break;
case 2:
$new_type = "Series";
echo $new_type;
break;
[/code]
and you could finish it off with the others :) -
Oh sorry.
I forgot to put ; after some lines
$new_type = "Home"
should be
$new_type = "Home";
and for all the others below that too lol. sorry -
Try this:
<?
require_once ("config.php");
$sql = "SELECT ID, PAGE_TITLE, PAGE_TYPE
FROM $sTableName
ORDER BY PAGE_TYPE ASC";
$sql_result = mysql_query($sql);
echo "<TABLE BORDER=1>";
echo "<TR><TH>Page Type </TH><TH>Page Title</TH><TH>Page ID</TH><TH>Edit Page</TH>";
while ($row = mysql_fetch_array($sql_result)) {
$page_id = $row["ID"];
$page_title = $row["PAGE_TITLE"];
$page_type = $row["PAGE_TYPE"];
$edit = '<a href ="cms.php?page_id='.$page_id.'">Edit Page</a>';
// change page type #'s to words
$new_type = NULL;
if ($page_type == 1) {
$new_type = "Home"
echo $new_type;
} else if ($page_type == 2) {
$new_type = "Series"
echo $new_type;
} else if ($page_type == 3) {
$new_type = "Movies"
echo $new_type;
} else if ($page_type == 4) {
$new_type = "Special"
echo $new_type;
} else if ($page_type == 5) {
$new_type = "Gaming"
echo $new_type;
} else {
$new_type = "Unknown"
echo $new_type;
}
echo "<TR><TD>$new_type</TD><TD>$page_title</TD><TD>$page_id</TD><TD>$edit</TD></TR>";
}
echo "</TABLE>";
?> -
Im not sure what your problem is.
You got all those IF statements to work but removing the"" from each number, right?
What was the other problem? -
change this:
if ($page_type == "1")
to this:
if ($page_type == 1)
-
[code]<form action="list.php" method="post">
<div class="left_search">
<div>Search for:</div>
<div>
<input type="text" maxlength="20" name="what" class="signup_textfield" /> in
<select name="selection">
<option value="">... select ...</option>
<?
foreach ($selections as $key => $value) {
echo "<option value='$key'";
if (isset($selection)) {
echo " selected";
}
echo "> {$value} </option>";
}
?>
</select> <input type="submit" value="Go!" name="go" /><br />
<input type="radio" name="exact" /> Exact match.<br />
<input type="radio" name="contain" /> Contain these words.
</div>
</div> <!--/left_search -->
</form>[/code]
I can click both radio buttons and theyll be selected. I only want 1 to be selected. how do i fx this?
K i solved it.
god, i can't believe i posted this :( -
lol, anytime.
nl2br
in PHP Coding Help
Posted
It's a RedHat Linux server.
To insert, i have a form, and heres the php code for it:
[code]
<?php
if (isset($_POST['submit'])) {
$news = escape_data($_POST['news']);
$title = escape_data($_POST['title']);
$query = "INSERT INTO news (title, news, date_submitted, author) VALUES ('$title', '".wordwrap($news, 45, "\n", 1)."', NOW(), '". $_SESSION['user_name'] ."')";
$result = mysql_query($query) or die (mysql_error());
if ($result) {
header ('Location: index.php');
} else {
echo 'Could not add news.';
}
}
?>[/code]